
Visual CSharp 2005 Recipes (2006) [eng]
.pdf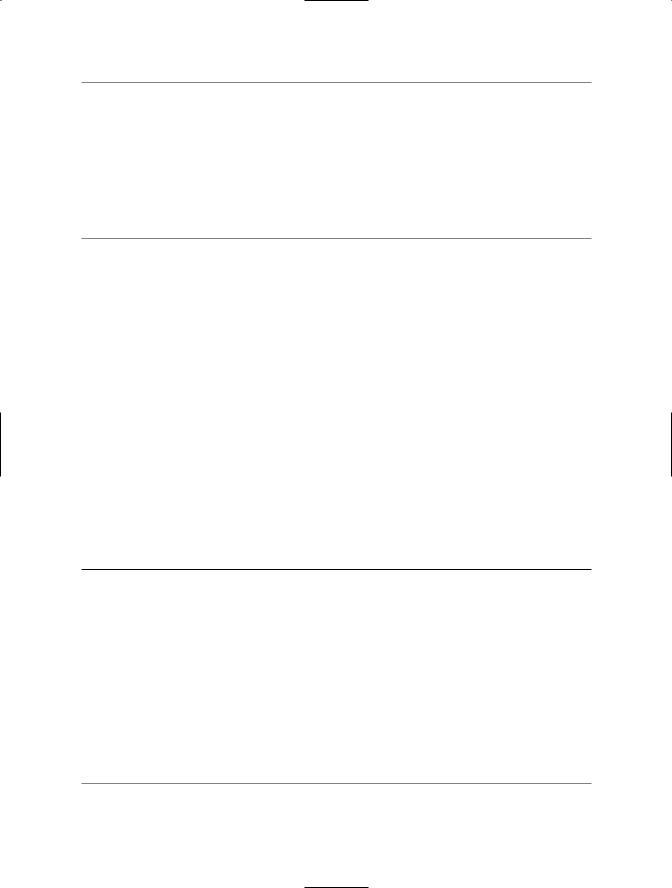
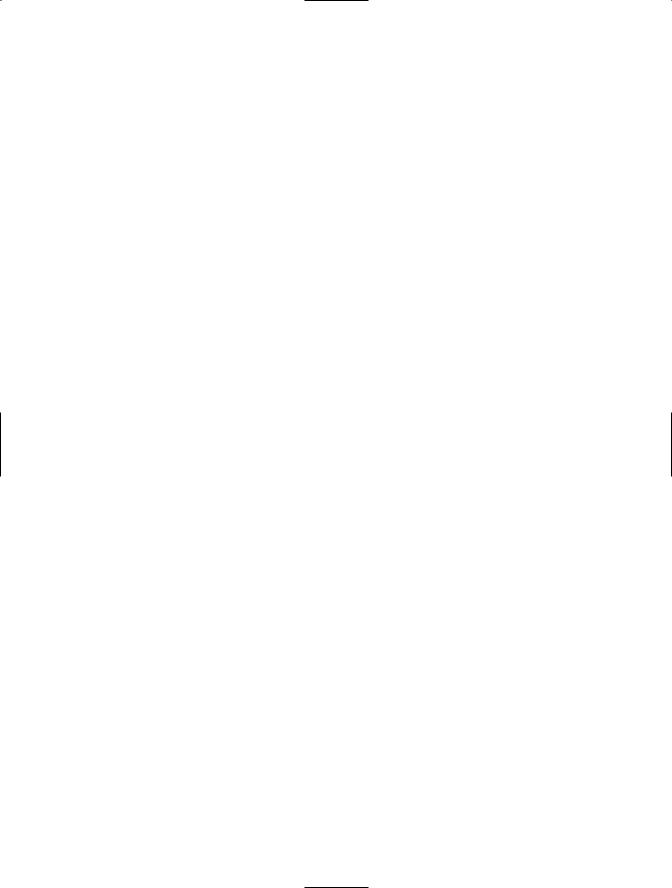

410 C H A P T E R 1 1 ■ S E C U R I T Y A N D C RY P TO G R A P H Y
The Evidence class actually contains two collections, representing different types of evidence:
•Host evidence includes those evidence objects assigned to the assembly by the runtime or the trusted code that loaded the assembly.
•Assembly evidence represents custom evidence objects embedded into the assembly at build time.
The Evidence class implements three methods for enumerating the evidence objects it contains: GetEnumerator, GetHostEnumerator, and GetAssemblyEnumerator. The GetHostEnumerator and GetAssemblyEnumerator methods return a System.Collections.IEnumerator instance that enumerates only those evidence objects from the appropriate collection. The GetEnumerator method returns an IEnumerator instance that enumerates all of the evidence objects contained in the Evidence collection.
■Note Evidence classes do not extend a standard base class or implement a standard interface. Therefore, when working with evidence programmatically, you need to test the type of each object and know what particular types you are seeking. (See recipe 3-11 for details on how to test the type of an object at runtime.)
The Code
The following example demonstrates how to display the host and assembly evidence of an assembly to the console. The example relies on the fact that all standard evidence classes override the Object. ToString method to display a useful representation of the evidence object’s state. Although interesting, this example does not always show the evidence that an assembly would have when loaded from within your program. The runtime host (such as the Microsoft ASP.NET or Internet Explorer runtime host) is free to assign additional host evidence as it loads an assembly.
using System;
using System.Reflection; using System.Collections; using System.Security.Policy;
namespace Apress.VisualCSharpRecipes.Chapter11
{
public class Recipe11_09
{
public static void Main(string[] args)
{
//Load the specified assembly. Assembly a = Assembly.LoadFrom(args[0]);
//Get the Evidence collection from the
//loaded assembly.
Evidence e = a.Evidence;
// Display the Host Evidence. IEnumerator x = e.GetHostEnumerator();
Console.WriteLine("HOST EVIDENCE COLLECTION:"); while(x.MoveNext())
{
Console.WriteLine(x.Current.ToString()); Console.WriteLine("Press Enter to see next evidence."); Console.ReadLine();
}
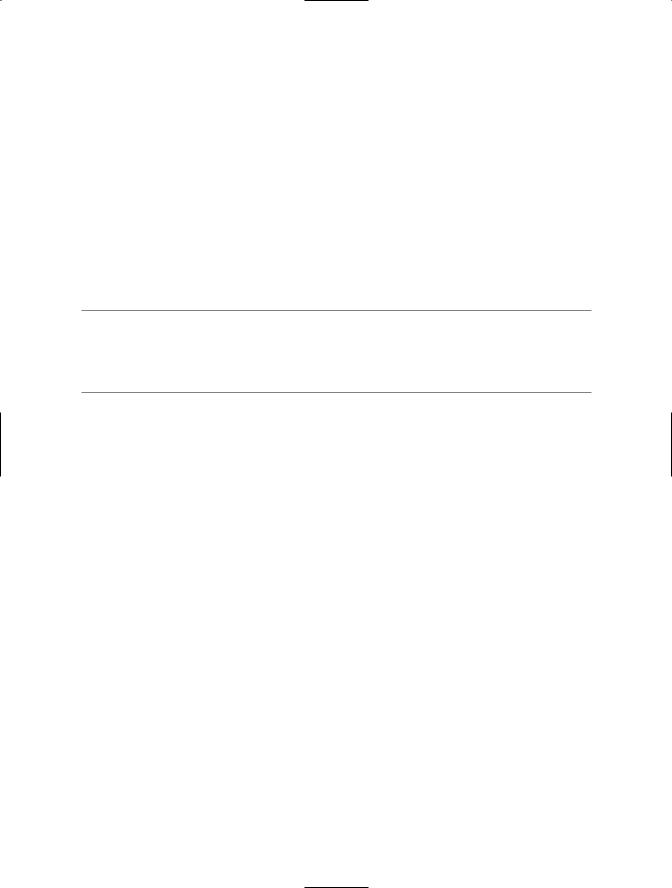
C H A P T E R 1 1 ■ S E C U R I T Y A N D C RY P TO G R A P H Y |
411 |
//Display the Assembly Evidence. x = e.GetAssemblyEnumerator();
Console.WriteLine("ASSEMBLY EVIDENCE COLLECTION:"); while(x.MoveNext())
{
Console.WriteLine(x.Current.ToString()); Console.WriteLine("Press Enter to see next evidence."); Console.ReadLine();
}
//Wait to continue.
Console.WriteLine("Main method complete. Press Enter."); Console.ReadLine();
}
}
}
■Note All of the standard evidence classes provided by the .NET Framework are immutable, ensuring that you cannot change their values after the runtime has created them and assigned them to the assembly. In addition, you cannot add or remove items while you are enumerating across the contents of a collection using an IEnumerator; otherwise, the MoveNext method throws a System.InvalidOperationException exception.
11-10. Determine If the Current User Is a Member of a Specific Windows Group
Problem
You need to determine if the current user of your application is a member of a specific Windows user group.
Solution
Obtain a System.Security.Principal.WindowsIdentity object representing the current Windows user by calling the static method WindowsIdentity.GetCurrent. Create a System.Security.Principal. WindowsPrincipal class using the WindowsIdentity class, and then call the method IsInRole of the
WindowsPrincipal object.
How It Works
The role-based security (RBS) mechanism of the .NET Framework abstracts the user-based security features of the underlying operating system through the following two key interfaces:
•The System.Security.Principal.IIdentity interface, which represents the entity on whose behalf code is running; for example, a user or service account.
•The System.Security.Principal.IPrincipal interface, which represents the entity’s IIdentity and the set of roles to which the entity belongs. A role is simply a categorization used to group entities with similar security capabilities, such as a Windows user group.
To integrate RBS with Windows user security, the .NET Framework provides the following two Windows-specific classes that implement the IIdentity and IPrincipal interfaces:
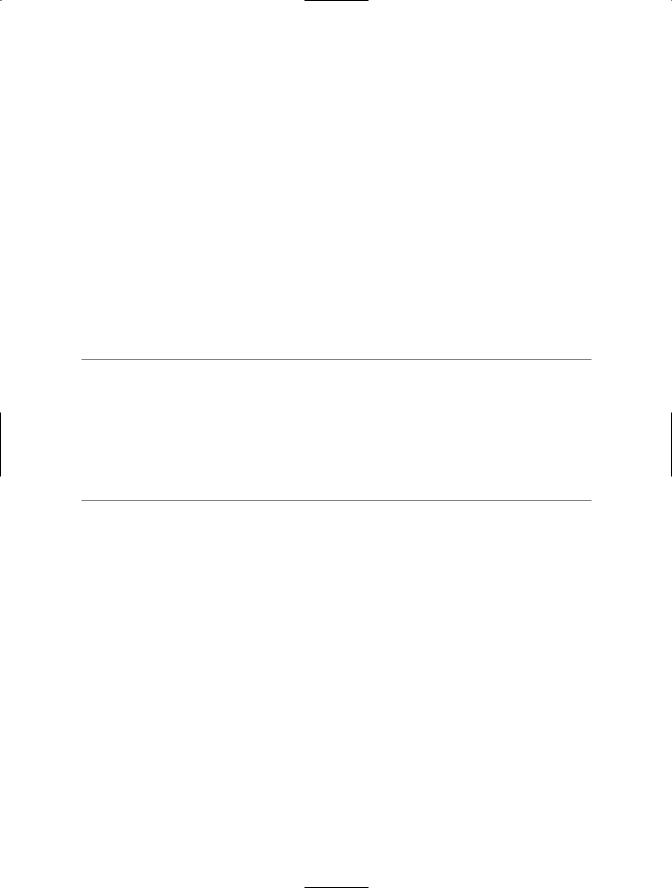
412C H A P T E R 1 1 ■ S E C U R I T Y A N D C RY P TO G R A P H Y
•System.Security.Principal.WindowsIdentity, which implements the IIdentity interface and represents a Windows user.
•System.Security.Principal.WindowsPrincipal, which implements IPrincipal and represents the set of Windows groups to which the user belongs.
Because .NET RBS is a generic solution designed to be platform-independent, you have no access to the features and capabilities of the Windows user account through the IIdentity and IPrincipal interfaces, and you must frequently use the WindowsIdentity and WindowsPrincipal objects directly.
To determine if the current user is a member of a specific Windows group, you must first call the static method WindowsIdentity.GetCurrent. The GetCurrent method returns a WindowsIdentity object that represents the Windows user on whose behalf the current thread is running. An overload of the GetCurrent method new to .NET Framework 2.0 takes a bool argument and allows you to control what is returned by GetCurrent if the current thread is impersonating a user different from the one associated with the process. If the argument is true, GetCurrent returns a WindowsIdentity representing the impersonated user, or it returns null if the thread is not impersonating a user. If the argument is false, GetCurrent returns the WindowsIdentity of the thread if it is not impersonating
a user, or it returns the WindowsIdentity of the process if the thread is currently impersonating a user.
■Note The WindowsIdentity class provides overloaded constructors that, when running on Microsoft Windows Server 2003 or later platforms, allow you to obtain a WindowsIdentity object representing a named user. You can use this WindowsIdentity object and the process described in this recipe to determine if that user is a member of a specific Windows group. If you try to use one of these constructors when running on an earlier version of Windows, the WindowsIdentity constructor will throw an exception. On Windows platforms preceding Windows Server 2003, you must use native code to obtain a Windows access token representing the desired user. You can then use this access token to instantiate a WindowsIdentity object. Recipe 11-12 explains how to obtain Windows access tokens for specific users.
Once you have a WindowsIdentity, instantiate a new WindowsPrincipal object, passing the WindowsIdentity object as an argument to the constructor. Finally, call the IsInRole method of the WindowsPrincipal object to test if the user is in a specific group (role). IsInRole returns true if the user is a member of the specified group; otherwise, it returns false. The IsInRole method provides four overloads:
•The first overload takes a string containing the name of the group for which you want to test. The group name must be of the form [DomainName]\[GroupName] for domain-based groups and [MachineName]\[GroupName] for locally defined groups. If you want to test for membership of a standard Windows group, use the form BUILTIN\[GroupName] or the other overload that takes a value from the System.Security.Principal.WindowsBuiltInRole enumeration. IsInRole performs a case-insensitive test for the specified group name.
•The second IsInRole overload accepts an int, which specifies a Windows role identifier (RID). RIDs provide a mechanism to identify groups that is independent of language and localization.
•The third IsInRole overload accepts a member of the System.Security.Principal. WindowsBuiltInRole enumeration. The WindowsBuiltInRole enumeration defines a set of members that represent each of the built-in Windows groups.
•The fourth IsInRole overload (new to .NET Framework 2.0) accepts a System.Security. Principal.SecurityIdentifier object that represents the security identifier (SID) of the group for which you want to test.
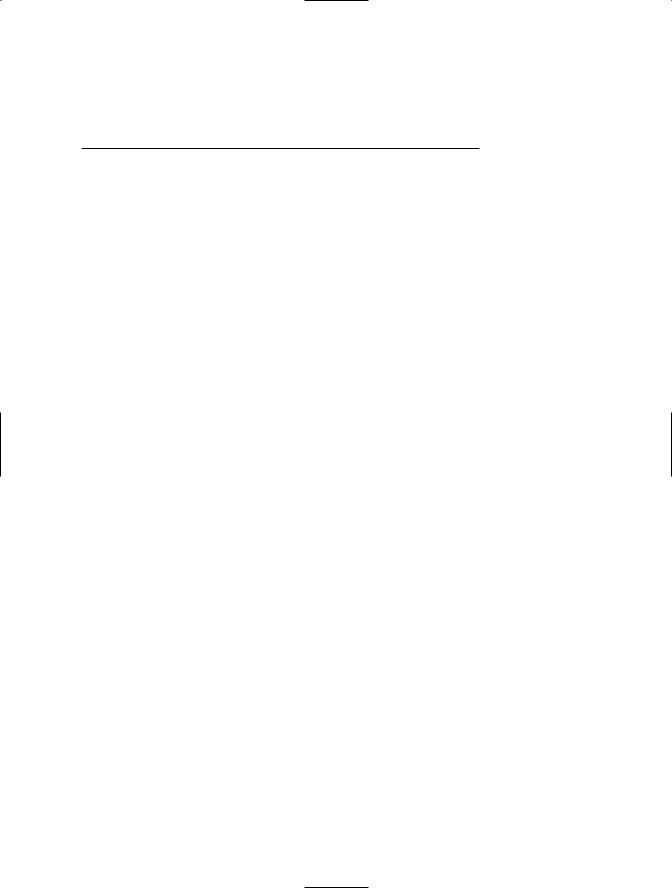
C H A P T E R 1 1 ■ S E C U R I T Y A N D C RY P TO G R A P H Y |
413 |
Table 11-2 lists the name, RID, and WindowsBuiltInRole value for each of the standard Windows groups.
Table 11-2. Windows Built-In Account Names and Identifiers
Account Name |
RID (Hex) |
WindowsBuiltInRole Value |
BUILTIN\Account Operators |
0x224 |
AccountOperator |
BUILTIN\Administrators |
0x220 |
Administrator |
BUILTIN\Backup Operators |
0x227 |
BackupOperator |
BUILTIN\Guests |
0x222 |
Guest |
BUILTIN\Power Users |
0x223 |
PowerUser |
BUILTIN\Print Operators |
0x226 |
PrintOperator |
BUILTIN\Replicators |
0x228 |
Replicator |
BUILTIN\Server Operators |
0x225 |
SystemOperator |
BUILTIN\Users |
0x221 |
User |
|
|
|
The Code
The following example demonstrates how to test whether the current user is a member of a set of named Windows groups. You specify the groups that you want to test for as command-line arguments. Remember to prefix the group name with the machine or domain name, or BUILTIN for standard Windows groups.
using System;
using System.Security.Principal;
namespace Apress.VisualCSharpRecipes.Chapter11
{
class Recipe11_10
{
public static void Main (string[] args)
{
//Obtain a WindowsIdentity object representing the currently
//logged on Windows user.
WindowsIdentity identity = WindowsIdentity.GetCurrent();
//Create a WindowsPrincipal object that represents the security
//capabilities of the specified WindowsIdentity; in this case,
//the Windows groups to which the current user belongs. WindowsPrincipal principal = new WindowsPrincipal(identity);
//Iterate through the group names specified as command-line
//arguments and test to see if the current user is a member of
//each one.
foreach (string role in args)
{
Console.WriteLine("Is {0} a member of {1}? = {2}", identity.Name, role, principal.IsInRole(role));
}
// Wait to continue.
Console.WriteLine("\nMain method complete. Press Enter."); Console.ReadLine();
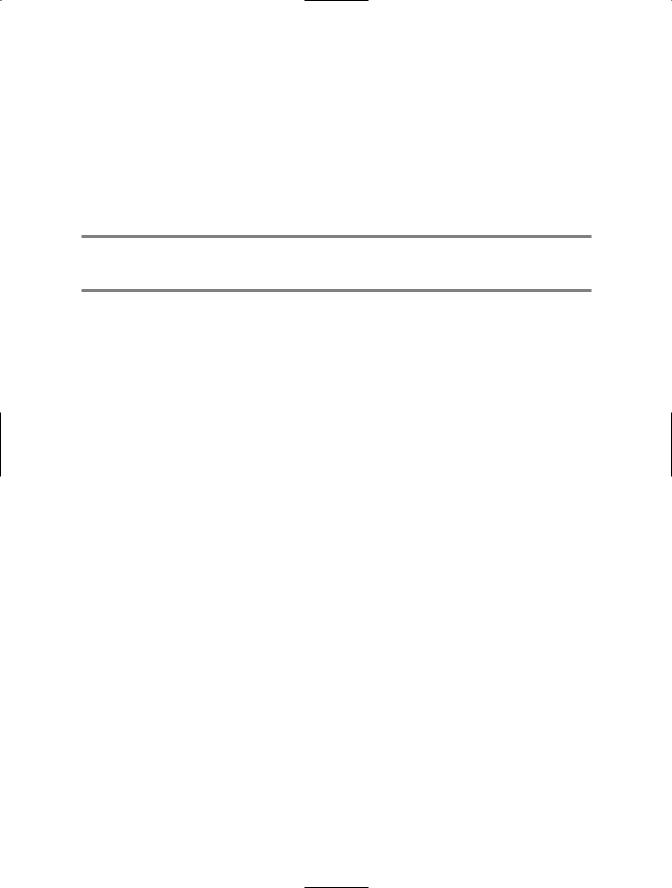
414 C H A P T E R 1 1 ■ S E C U R I T Y A N D C RY P TO G R A P H Y
}
}
}
Usage
If you run this example as a user named Darryl on a computer named MACHINE using this command:
Recipe11-10 BUILTIN\Administrators BUILTIN\Users MACHINE\Accountants
you will see console output similar to the following:
Is MACHINE\Darryl a member of BUILTIN\Administrators? = False
Is MACHINE\Darryl a member of BUILTIN\Users? = True
Is MACHINE\Darryl a member of MACHINE\Accountants? = True
11-11. Restrict Which Users Can Execute Your Code
Problem
You need to restrict which users can execute elements of your code based on the user’s name or the roles of which the user is a member.
Solution
Use the permission class System.Security.Permissions.PrincipalPermission and its attribute counterpart System.Security.Permissions.PrincipalPermissionAttribute to protect your program elements with RBS demands.
How It Works
The .NET Framework supports both imperative and declarative RBS demands. The class PrincipalPermission provides support for imperative security statements, and its attribute counterpart PrincipalPermissionAttribute provides support for declarative security statements. RBS demands use the same syntax as CAS demands, but RBS demands specify the name the current user must have, or more commonly, the roles of which the user must be a member. An RBS demand instructs the runtime to look at the name and roles of the current user, and if that user does not meet the requirements of the demand, the runtime throws a System.Security.SecurityException exception.
To make an imperative security demand, you must first create a PrincipalPermission object specifying the username and role name you want to demand, and then you must call its Demand method. You can specify only a single username and role name per demand. If either the username or the role name is null, any value will satisfy the demand. Unlike with code access permissions, an RBS demand does not result in a stack walk; the runtime evaluates only the username and roles of the current user.
To make a declarative security demand, you must annotate the class or member you want to protect with a correctly configured PrincipalPermissionAttribute attribute. Class-level demands apply to all members of the class, unless a member-specific demand overrides the class demand.
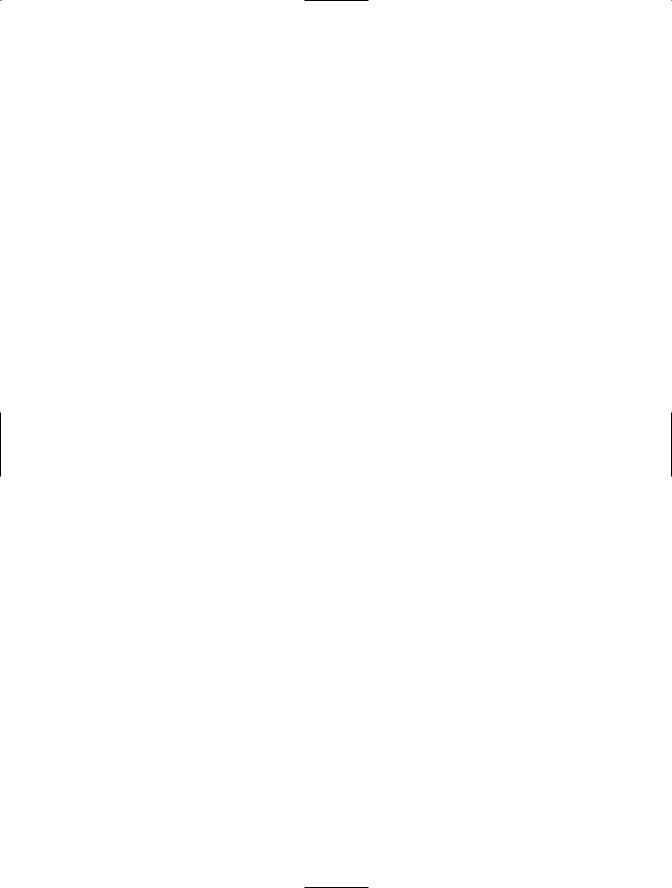
C H A P T E R 1 1 ■ S E C U R I T Y A N D C RY P TO G R A P H Y |
415 |
Generally, you are free to choose whether to implement imperative or declarative demands. However, imperative security demands allow you to integrate RBS demands with code logic to achieve more sophisticated demand behavior. In addition, if you do not know the role or usernames to demand at compile time, you must use imperative demands. Declarative demands have the advantage that they are separate from code logic and easier to identify. In addition, you can view declarative demands using the Permview.exe tool (discussed in recipe 11-6). Whether you implement imperative or declarative demands, you must ensure that the runtime has access to the name and roles for the current user to evaluate the demand correctly.
The System.Threading.Thread class represents an operating system thread running managed code. The static property CurrentPrincipal of the Thread class contains an IPrincipal instance representing the user on whose behalf the managed thread is running. At the operating system level, each thread also has an associated Windows access token, which represents the Windows account on whose behalf the thread is running. The IPrincipal instance and the Windows access token are two separate entities. Windows uses its access token to enforce operating system security, whereas the .NET runtime uses its IPrincipal instance to evaluate application-level RBS demands. Although they may, and often do, represent the same user, this is by no means always the case.
The benefit of this approach is that you can implement a user and an RBS model within your application using a proprietary user accounts database, without the need for all users to have Windows user accounts. This is a particularly useful approach in large-scale, publicly accessible Internet applications.
By default, the Thread.CurrentPrincipal property is undefined. Because obtaining user-related information can be time-consuming, and only a minority of applications use this information, the
.NET designers opted for lazy initialization of the CurrentPrincipal property. The first time code gets the Thread.CurrentPrincipal property, the runtime assigns an IPrincipal instance to the property using the following logic:
•If the application domain in which the current thread is executing has a default principal, the runtime assigns this principal to the Thread.CurrentPrincipal property. By default, application domains do not have default principals. You can set the default principal of an application domain by calling the method SetThreadPrincipal on a System.AppDomain object that represents the application domain you want to configure. Code must have the ControlPrincipal element of SecurityPermission to call SetThreadPrincipal. You can set the default principal only once for each application domain; a second call to SetThreadPrincipal results in the exception System.Security.Policy.PolicyException.
•If the application domain does not have a default principal, the application domain’s principal policy determines which IPrincipal implementation to create and assign to Thread.CurrentPrincipal. To configure principal policy for an application domain, obtain an AppDomain object that represents the application domain and call the object’s
SetPrincipalPolicy method. The SetPrincipalPolicy method accepts a member of the enumeration System.Security.Principal.PrincipalPolicy, which specifies the type of IPrincipal object to assign to Thread.CurrentPrincipal. Code must have the
ControlPrincipal element of SecurityPermission to call SetPrincipalPolicy. Table 11-3 lists the available PrincipalPolicy values; the default value is UnauthenticatedPrincipal.
•If your code has the ControlPrincipal element of SecurityPermission, you can instantiate your own IPrincipal object and assign it to the Thread.CurrentPrincipal property directly. This will prevent the runtime from assigning default IPrincipal objects or creating new ones based on principal policy.
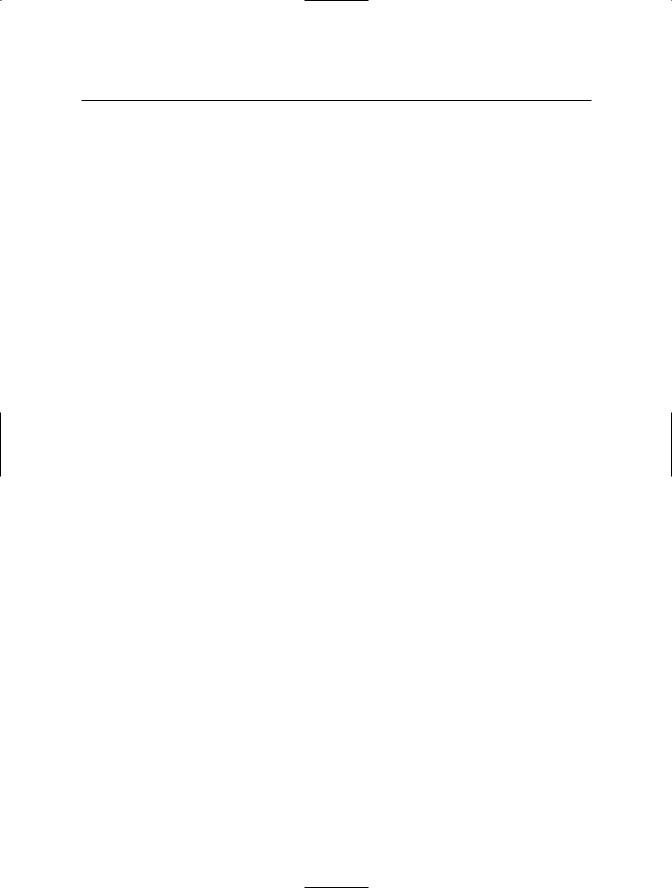
416 C H A P T E R 1 1 ■ S E C U R I T Y A N D C RY P TO G R A P H Y
Table 11-3. Members of the PrincipalPolicy Enumeration
Member Name |
Description |
NoPrincipal |
No IPrincipal object is created. Thread.CurrentPrincipal returns |
|
a null reference. |
UnauthenticatedPrincipal |
An empty System.Security.Principal.GenericPrincipal object is |
|
created and assigned to Thread.CurrentPrincipal. |
WindowsPrincipal |
A WindowsPrincipal object representing the currently logged-on |
|
Windows user is created and assigned to Thread.CurrentPrincipal. |
|
|
Whatever method you use to establish the IPrincipal for the current thread, you must do so before you use RBS demands, or the correct user (IPrincipal) information will not be available for the runtime to process the demand. Normally, when running on the Windows platform, you would set the principal policy of an application domain to PrincipalPolicy.WindowsPrincipal (as shown here) to obtain Windows user information.
//Obtain a reference to the current application domain. AppDomain appDomain = System.AppDomain.CurrentDomain;
//Configure the current application domain to use Windows-based principals. appDomain.SetPrincipalPolicy(
System.Security.Principal.PrincipalPolicy.WindowsPrincipal);
The Code
The following example demonstrates the use of imperative and declarative RBS demands. The example shows three methods protected using imperative RBS demands (Method1, Method2, and Method3), and then three other methods protected using the equivalent declarative RBS demands (Method4, Method5, and Method6).
using System;
using System.Security.Permissions;
namespace Apress.VisualCSharpRecipes.Chapter11
{
class Recipe11_11
{
public static void Method1()
{
//An imperative role-based security demand for the current principal
//to represent an identity with the name Anya. The roles of the
//principal are irrelevant.
PrincipalPermission perm =
new PrincipalPermission(@"MACHINE\Anya", null);
// Make the demand. perm.Demand();
}
public static void Method2()
{
//An imperative role-based security demand for the current principal
//to be a member of the roles Managers OR Developers. If the
//principal is a member of either role, access is granted. Using the
//PrincipalPermission, you can express only an OR type relationship.
//This is because the PrincipalPolicy.Intersect method always
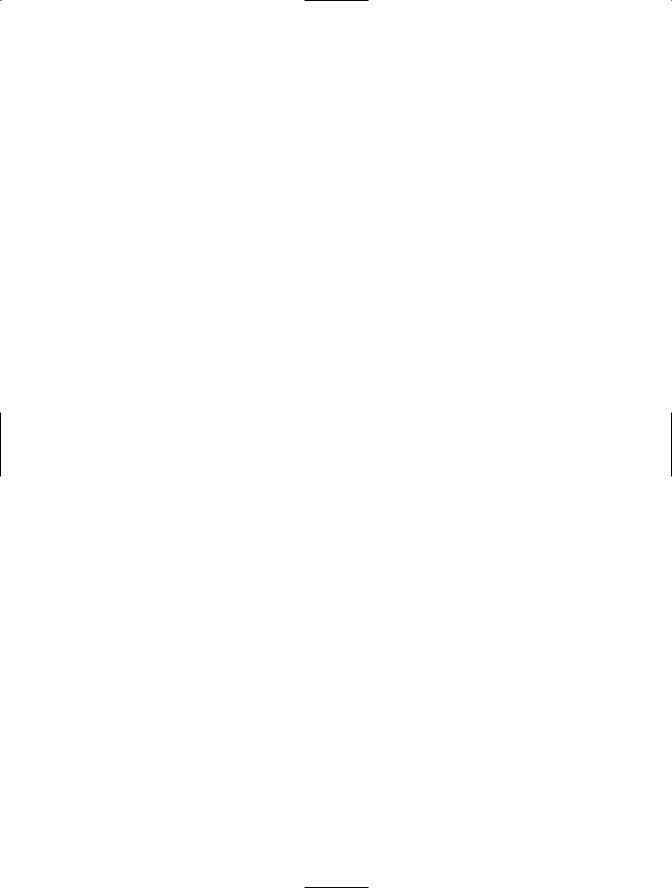
C H A P T E R 1 1 ■ S E C U R I T Y A N D C RY P TO G R A P H Y |
417 |
//returns an empty permission unless the two inputs are the same.
//However, you can use code logic to implement more complex
//conditions. In this case, the name of the identity is irrelevant. PrincipalPermission perm1 =
new PrincipalPermission(null, @"MACHINE\Managers");
PrincipalPermission perm2 =
new PrincipalPermission(null, @"MACHINE\Developers");
// Make the demand. perm1.Union(perm2).Demand();
}
public static void Method3()
{
//An imperative role-based security demand for the current principal
//to represent an identity with the name Anya AND be a member of the
//Managers role.
PrincipalPermission perm =
new PrincipalPermission(@"MACHINE\Anya", @"MACHINE\Managers");
// Make the demand perm.Demand();
}
//A declarative role-based security demand for the current principal
//to represent an identity with the name Anya. The roles of the
//principal are irrelevant. [PrincipalPermission(SecurityAction.Demand, Name = @"MACHINE\Anya")] public static void Method4()
{
//Method implementation. . .
}
//A declarative role-based security demand for the current principal
//to be a member of the roles Managers OR Developers. If the
//principal is a member of either role, access is granted. You
//can express only an OR type relationship, not an AND relationship.
//The name of the identity is irrelevant. [PrincipalPermission(SecurityAction.Demand, Role = @"MACHINE\Managers")] [PrincipalPermission(SecurityAction.Demand, Role = @"MACHINE\Developers")] public static void Method5()
{
//Method implementation. . .
}
//A declarative role-based security demand for the current principal
//to represent an identity with the name Anya AND be a member of the
//Managers role.
[PrincipalPermission(SecurityAction.Demand, Name = @"MACHINE\Anya", Role = @"MACHINE\Managers")]
public static void Method6()
{
// Method implementation. . .
}
}
}