
- •Table of Content
- •Why We Wrote This Book
- •What This Book Is About
- •Who Should Read This Book
- •Conventions Used
- •Figure 1. Example class diagram
- •How This Book Is Organized
- •How to Use This Book
- •Companion Web Site
- •Acknowledgments
- •Why Patterns?
- •History of the Patterns Movement
- •Basic Concepts in Patterns
- •Software Abstraction and Reuse
- •Summary
- •Chapter 1. Creational Patterns
- •Introduction to Creational Patterns
- •Abstract Factory
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 1.1. Abstract Factory class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 1.1 AddressFactory.java
- •Example 1.2 Address.java
- •Example 1.3 PhoneNumber.java
- •Example 1.4 USAddressFactory.java
- •Example 1.5 USAddress.java
- •Example 1.6 USPhoneNumber.java
- •Example 1.7 FrenchAddressFactory.java
- •Example 1.8 FrenchAddress.java
- •Example 1.9 FrenchPhoneNumber.java
- •Builder
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 1.2. Builder class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 1.10 AppointmentBuilder.java
- •Example 1.11 Appointment.java
- •Example 1.12 Scheduler.java
- •Example 1.13 MeetingBuilder.java
- •Factory Method
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 1.3. Factory Method class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 1.14 Editable.java
- •Example 1.15 ItemEditor.java
- •Example 1.16 Contact.java
- •Prototype
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 1.4. Prototype class diagram
- •Benefits and Drawbacks
- •Figure 1.5. Example of Prototype use
- •Pattern Variants
- •Example 1.17 Copy constructor
- •Related Patterns
- •Example
- •Example 1.18 Copyable.java
- •Example 1.19 Address.java
- •Singleton
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 1.6. Singleton class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 1.20 HistoryList.java
- •Introduction to Behavioral Patterns
- •Chain of Responsibility
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Figure 2.1. Chain of Responsibility sequence diagram
- •Implementation
- •Figure 2.2. Chain of Responsibility class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 2.1 ProjectItem.java
- •Example 2.2 Project.java
- •Example 2.3 Task.java
- •Command
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Figure 2.3. Sequence diagram for invocation of Command
- •Implementation
- •Figure 2.4. Command class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Figure 2.5. Class diagram showing both the undo and MacroCommand variant
- •Related Patterns
- •Example
- •Example 2.4 Command.java
- •Example 2.5 UndoableCommand.java
- •Example 2.6 Appointment.java
- •Example 2.7 ChangeLocationCommand.java
- •Interpreter
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 2.6. Interpreter class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 2.8 Expression.java
- •Example 2.9 ConstantExpression.java
- •Example 2.10 VariableExpression.java
- •Example 2.11 CompoundExpression.java
- •Example 2.12 AndExpression.java
- •Example 2.13 OrExpression.java
- •Example 2.14 ComparisonExpression.java
- •Example 2.15 EqualsExpression.java
- •Example 2.16 ContainsExpression.java
- •Example 2.17 Context.java
- •Example 2.18 ContactList.java
- •Iterator
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 2.7. Iterator class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 2.19 Iterating.java
- •Example 2.20 ToDoList.java
- •Example 2.21 ToDoListCollection.java
- •Example 2.22 ToDoListCollectionImpl.java
- •Example 2.23 ToDoListImpl.java
- •Example 2.24 ListPrinter.java
- •Mediator
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 2.8. Mediator class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 2.25 MediatorGui.java
- •Example 2.26 ContactDisplayPanel.java
- •Example 2.27 ContactSelectorPanel.java
- •Example 2.28 ContactEditorPanel.java
- •Example 2.29 ContactMediator.java
- •Example 2.30 ContactMediatorImpl.java
- •Memento
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 2.9. Memento class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 2.31 AddressBook.java
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 2.10. Observable class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 2.32 TaskChangeObservable.java
- •Example 2.33 TaskChangeObserver.java
- •Example 2.34 ObserverGui.java
- •Example 2.35 TaskEditorPanel.java
- •Example 2.36 TaskHistoryPanel.java
- •Example 2.37 TaskSelectorPanel.java
- •State
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Figure 2.11. State transition diagram for a door
- •Implementation
- •Figure 2.12. State class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Figure 2.13. State transition diagram for a file
- •Example 2.38 State.java
- •Example 2.39 CalendarEditor.java
- •Example 2.40 StateGui.java
- •Strategy
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Benefits and Drawbacks
- •Implementation
- •Figure 2.14. Strategy class diagram
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 2.41 ContactList.java
- •Example 2.42 SummarizingStrategy.java
- •Example 2.43 NameSummarizer.java
- •Example 2.44 OrganizationSummarizer.java
- •Visitor
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 2.15. Visitor class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 2.45 ProjectItem.java
- •Example 2.46 Deliverable.java
- •Example 2.47 DependentTask.java
- •Example 2.48 Project.java
- •Example 2.49 Task.java
- •Example 2.50 ProjectVisitor.java
- •Example 2.51 ProjectCostVisitor.java
- •Template Method
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 2.16. Template Method class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 2.52 ProjectItem.java
- •Example 2.53 Deliverable.java
- •Example 2.54 Task.java
- •Introduction to Structural Patterns
- •Adapter
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 3.1. Adapter class diagram interface
- •Figure 3.2. Sequence diagram for action mapping
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 3.1 Chovnatlh.java
- •Example 3.3 Contact.java
- •Example 3.4 ContactAdapter.java
- •Bridge
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Comparison of Inheritance Pattern and Bridge Pattern
- •Implementation
- •Figure 3.3. Bridge class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 3.5 ListImpl.java
- •Example 3.6 OrderedListImpl.java
- •Example 3.7 BaseList.java
- •Example 3.8 NumberedList.java
- •Composite
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 3.4. Composite class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Figure 3.5. Composite class diagram for the code example
- •Example 3.9 ProjectItem.java
- •Example 3.10 Deliverable.java
- •Example 3.11 Project.java
- •Example 3.12 Project.java
- •Example 3.13 Task.java
- •Decorator
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 3.6. Decorator class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 3.14 ProjectItem.java
- •Example 3.15 Deliverable.java
- •Example 3.16 Task.java
- •Example 3.17 ProjectDecorator.java
- •Example 3.18 DependentProjectItem.java
- •Example 3.19 SupportedProjectItem.java
- •Facade
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 3.7. Facade object diagram
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 3.20 InternationalizationWizard.java
- •Example 3.21 Currency.java
- •Example 3.22 InternationalizedText.java
- •Example 3.23 PhoneNumber.java
- •Example 3.24 Nation.java
- •Flyweight
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 3.8. Flyweight class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 3.25 State.java
- •Example 3.26 CleanState.java
- •Example 3.27 DirtyState.java
- •Example 3.28 StateFactory.java
- •Half-Object Plus Protocol (HOPP)
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 3.9. HOPP class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 3.29 Calendar.java
- •Example 3.30 CalendarImpl.java
- •Example 3.31 CalendarHOPP.java
- •Proxy
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 3.10. Proxy class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 3.32 AddressBook.java
- •Example 3.33 AddressBookProxy.java
- •Example 3.34 AddressBookImpl.java
- •Introduction to System Patterns
- •Model-View-Controller (MVC)
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 4.1. MVC component diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 4.1 ContactModel.java
- •Example 4.2 ContactView.java
- •Example 4.3 ContactDisplayView.java
- •Example 4.4 ContactEditView.java
- •Example 4.5 ContactEditController.java
- •Session
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Stateful and Stateless Communication
- •Applications Often Require Stateful Communication
- •Session Pattern and Stateful Communication
- •Real-World Stateful Communication
- •Implementation
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Figure 4.2. Session component for a client-matching session
- •Figure 4.3. Session component for server-maintained sessions
- •Figure 4.4. Session tracker
- •Example 4.6 SessionClient.java
- •Example 4.7 SessionServer.java
- •Example 4.8 SessionServerImpl.java
- •Example 4.9 SessionServerDelegate.java
- •Worker Thread
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 4.5. Worker Thread class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 4.10 Queue.java
- •Example 4.11 RunnableTask.java
- •Example 4.12 ConcreteQueue.java
- •Example 4.13 AddressRetriever.java
- •Example 4.14 ContractRetriever.java
- •Callback
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 4.6. Callback component diagram
- •Figure 4.7. Callback sequence diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 4.15 CallbackServer.java
- •Example 4.16 CallbackServerImpl.java
- •Example 4.17 CallbackServerDelegate.java
- •Example 4.18 CallbackClient.java
- •Example 4.19 CallbackClientImpl.java
- •Successive Update
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Client Pull
- •Figure 4.8. Successive Update sequence diagram (client pull)
- •Server Push
- •Figure 4.9. Successive Update sequence diagram (server push)
- •Implementation
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 4.20 PullClient.java
- •Example 4.21 ClientPullRequester.java
- •Example 4.22 ClientPullServer.java
- •Example 4.23 ClientPullServerImpl.java
- •Example 4.24 UpdateServerDelegate.java
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 4.10. Router class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 4.25 Message.java
- •Example 4.26 InputChannel.java
- •Example 4.27 OutputChannel.java
- •Example 4.28 Router.java
- •Transaction
- •Pattern Properties
- •Purpose
- •Introduction
- •Applicability
- •Description
- •Implementation
- •Figure 4.11. Transaction class diagram
- •Benefits and Drawbacks
- •Pattern Variants
- •Related Patterns
- •Example
- •Example 4.29 AppointmentTransactionParticipant.java
- •Example 4.30 AppointmentBook.java
- •Chapter 5. Introduction to Java Programming Language Patterns
- •Event Handling
- •Packages
- •Overview
- •Pattern Use
- •JavaBeans
- •Packages
- •Overview
- •AWT and Swing – The Graphical User Interface APIs
- •Packages
- •Common Features
- •The AWT Architectural Model
- •Benefits
- •Drawbacks
- •The Swing Architectural Model
- •General Pattern Use
- •Pattern Use in AWT
- •Pattern Use in Swing
- •Collections Framework
- •Packages
- •Description
- •Example 6.1 Collections class and threadsafe versions of collections
- •Pattern Use
- •Input-Output (I/O)
- •Packages
- •Description
- •Example 6.2 Streams in Java
- •Pattern Use
- •Reflection
- •Packages
- •Overview
- •Example 6.3 Using instances of unknown origin
- •Pattern Use
- •Java Naming and Directory Interface (JNDI)
- •Packages
- •Description
- •Example 7.1 Using JNDI
- •Pattern Use
- •JDBC
- •Packages
- •Overview
- •Example 7.2 Obtaining results from a database
- •Pattern Use
- •Packages
- •Overview
- •Pattern Use
- •CORBA
- •Packages
- •Overview
- •Java and CORBA
- •Pattern Use
- •Jini
- •Packages
- •Description
- •Lookup Service
- •Distributed Events
- •Pattern Use
- •Java 2, Enterprise Edition (J2EE)
- •Overview
- •Core J2EE Concepts
- •J2EE Tiers
- •Core Technical Concepts
- •Core Component Technologies
- •Communication and the Connector Technologies
- •Main J2EE Resources
- •Component Patterns
- •Servlets and JSPs
- •Packages
- •Overview
- •Main API Elements
- •Life Cycle
- •JavaServer Pages
- •Pattern Use
- •Enterprise JavaBeans
- •Packages
- •Description
- •General Pattern Use
- •Connector Pattern Use: Factory Method
- •Architectural Pattern Use
- •System Requirements
- •Creational Pattern Code Examples
- •Example A.1 AddressFactory.java
- •Example A.2 Address.java
- •Example A.3 PhoneNumber.java
- •Example A.4 USAddressFactory.java
- •Example A.5 USAddress.java
- •Example A.6 USPhoneNumber.java
- •Example A.7 FrenchAddressFactory.java
- •Example A.8 FrenchAddress.java
- •Example A.9 FrenchPhoneNumber.java
- •Example A.10 RunPattern.java
- •Example A.11 AppointmentBuilder.java
- •Example A.12 Appointment.java
- •Example A.13 Scheduler.java
- •Example A.14 MeetingBuilder.java
- •Example A.15 InformationRequiredException.java
- •Example A.16 Location.java
- •Example A.17 LocationImpl.java
- •Example A.18 Contact.java
- •Example A.19 ContactImpl.java
- •Example A.20 RunPattern.java
- •Example A.21 Editable.java
- •Example A.22 ItemEditor.java
- •Example A.23 Contact.java
- •Example A.24 EditorGui.java
- •Example A.25 RunPattern.java
- •Example A.26 Copyable.java
- •Example A.27 Address.java
- •Example A.28 RunPattern.java
- •Example A.29 HistoryList.java
- •Example A.30 SingletonGUI.java
- •Example A.31 RunPattern.java
- •Behavioral Pattern Code Examples
- •Example A.32 ProjectItem.java
- •Example A.33 Project.java
- •Example A.34 Task.java
- •Example A.35 Contact.java
- •Example A.36 ContactImpl.java
- •Example A.37 DataCreator.java
- •Example A.38 DataRetriever.java
- •Example A.39 RunPattern.java
- •Example A.40 Command.java
- •Example A.41 UndoableCommand.java
- •Example A.42 Appointment.java
- •Example A.43 ChangeLocationCommand.java
- •Example A.44 CommandGui.java
- •Example A.45 LocationEditor.java
- •Example A.46 Contact.java
- •Example A.47 ContactImpl.java
- •Example A.48 Location.java
- •Example A.49 LocationImpl.java
- •Example A.50 RunPattern.java
- •Example A.51 Expression.java
- •Example A.52 ConstantExpression.java
- •Example A.53 VariableExpression.java
- •Example A.54 CompoundExpression.java
- •Example A.55 AndExpression.java
- •Example A.56 OrExpression.java
- •Example A.57 ComparisonExpression.java
- •Example A.58 EqualsExpression.java
- •Example A.59 ContainsExpression.java
- •Example A.60 Context.java
- •Example A.61 ContactList.java
- •Example A.62 Contact.java
- •Example A.63 ContactImpl.java
- •Example A.64 RunPattern.java
- •Example A.65 Iterating.java
- •Example A.66 ToDoList.java
- •Example A.67 ToDoListCollection.java
- •Example A.68 ToDoListCollectionImpl.java
- •Example A.69 ToDoListImpl.java
- •Example A.70 ListPrinter.java
- •Example A.71 DataCreator.java
- •Example A.72 DataRetriever.java
- •Example A.73 RunPattern.java
- •Example A.74 MediatorGui.java
- •Example A.75 ContactDisplayPanel.java
- •Example A.76 ContactSelectorPanel.java
- •Example A.77 ContactEditorPanel.java
- •Example A.78 ContactMediator.java
- •Example A.79 ContactMediatorImpl.java
- •Example A.80 Contact.java
- •Example A.81 ContactImpl.java
- •Example A.82 RunPattern.java
- •Example A.83 AddressBook.java
- •Example A.84 Address.java
- •Example A.85 AddressImpl.java
- •Example A.86 Contact.java
- •Example A.87 ContactImpl.java
- •Example A.88 RunPattern.java
- •Example A.89 TaskChangeObservable.java
- •Example A.90 TaskChangeObserver.java
- •Example A.91 ObserverGui.java
- •Example A.92 TaskEditorPanel.java
- •Example A.93 TaskHistoryPanel.java
- •Example A.94 TaskSelectorPanel.java
- •Example A.95 Task.java
- •Example A.96 RunPattern.java
- •Figure A.1. State transition diagram for a file
- •Example A.97 State.java
- •Example A.98 CalendarEditor.java
- •Example A.99 StateGui.java
- •Example A.100 Appointment.java
- •Example A.101 Contact.java
- •Example A.102 ContactImpl.java
- •Example A.103 Location.java
- •Example A.104 LocationImpl.java
- •Example A.105 DataCreator.java
- •Example A.106 FileLoader.java
- •Example A.107 RunPattern.java
- •Example A.108 ContactList.java
- •Example A.109 SummarizingStrategy.java
- •Example A.110 NameSummarizer.java
- •Example A.111 OrganizationSummarizer.java
- •Example A.112 Contact.java
- •Example A.113 ContactImpl.java
- •Example A.114 DataCreator.java
- •Example A.115 DataRetriever.java
- •Example A.116 RunPattern.java
- •Example A.117 ProjectItem.java
- •Example A.118 Deliverable.java
- •Example A.119 DependentTask.java
- •Example A.120 Project.java
- •Example A.121 Task.java
- •Example A.122 ProjectVisitor.java
- •Example A.123 ProjectCostVisitor.java
- •Example A.124 Contact.java
- •Example A.125 ContactImpl.java
- •Example A.126 DataCreator.java
- •Example A.127 DataRetriever.java
- •Example A.128 RunPattern.java
- •Example A.129 ProjectItem.java
- •Example A.130 Deliverable.java
- •Example A.131 Task.java
- •Example A.132 RunPattern.java
- •Structural Pattern Code Examples
- •Example A.133 Chovnatlh.java
- •Example A.134 ChovnatlhImpl.java
- •Example A.135 Contact.java
- •Example A.136 ContactAdapter.java
- •Example A.137 Contact.java
- •Example A.138 ListImpl.java
- •Example A.139 OrderedListImpl.java
- •Example A.140 BaseList.java
- •Example A.141 NumberedList.java
- •Example A.142 OrnamentedList.java
- •Example A.143 RunPattern.java
- •Example A.144 ProjectItem.java
- •Example A.145 Deliverable.java
- •Example A.146 Project.java
- •Example A.147 Project.java
- •Example A.148 Task.java
- •Example A.149 Contact.java
- •Example A.150 ContactImpl.java
- •Example A.151 DataCreator.java
- •Example A.152 DataRetriever.java
- •Example A.153 RunPattern.java
- •Example A.154 ProjectItem.java
- •Example A.155 Deliverable.java
- •Example A.156 Task.java
- •Example A.157 ProjectDecorator.java
- •Example A.158 DependentProjectItem.java
- •Example A.159 SupportedProjectItem.java
- •Example A.160 Contact.java
- •Example A.161 ContactImpl.java
- •Example A.162 RunPattern.java
- •Example A.163 InternationalizationWizard.java
- •Example A.164 Currency.java
- •Example A.165 InternationalizedText.java
- •Example A.166 PhoneNumber.java
- •Example A.167 Nation.java
- •Example A.168 FacadeGui.java
- •Example A.169 DataCreator.java
- •Example A.170 RunPattern.java
- •Example A.171 State.java
- •Example A.172 CleanState.java
- •Example A.173 DirtyState.java
- •Example A.174 StateFactory.java
- •Example A.175 ManagedList.java
- •Example A.176 Address.java
- •Example A.177 AddressImpl.java
- •Example A.178 Contact.java
- •Example A.179 ContactImpl.java
- •Example A.180 RunPattern.java
- •Example A.181 Calendar.java
- •Example A.182 CalendarImpl.java
- •Example A.183 CalendarHOPP.java
- •Example A.184 Appointment.java
- •Example A.185 Contact.java
- •Example A.186 ContactImpl.java
- •Example A.187 Location.java
- •Example A.188 LocationImpl.java
- •Example A.189 FileLoader.java
- •Example A.190 RunPattern.java
- •Example A.191 AddressBook.java
- •Example A.192 AddressBookProxy.java
- •Example A.193 AddressBookImpl.java
- •Example A.194 FileLoader.java
- •Example A.195 Address.java
- •Example A.196 AddressImpl.java
- •Example A.197 DataCreator.java
- •Example A.198 RunPattern.java
- •System Pattern Code Examples
- •Example A.199 ContactModel.java
- •Example A.200 ContactView.java
- •Example A.201 ContactDisplayView.java
- •Example A.202 ContactEditView.java
- •Example A.203 ContactEditController.java
- •Example A.204 RunPattern.java
- •Example A.205 SessionClient.java
- •Example A.206 SessionServer.java
- •Example A.207 SessionServerImpl.java
- •Example A.208 SessionServerDelegate.java
- •Example A.209 SessionException.java
- •Example A.210 Address.java
- •Example A.211 AddressImpl.java
- •Example A.212 Contact.java
- •Example A.213 ContactImpl.java
- •Example A.214 RunPattern.java
- •Example A.215 Queue.java
- •Example A.216 RunnableTask.java
- •Example A.217 ConcreteQueue.java
- •Example A.218 AddressRetriever.java
- •Example A.219 ContractRetriever.java
- •Example A.220 ServerDataStore.java
- •Example A.221 ServerDataStoreImpl.java
- •Example A.222 Address.java
- •Example A.223 AddressImpl.java
- •Example A.224 Contact.java
- •Example A.225 ContactImpl.java
- •Example A.226 RunPattern.java
- •Example A.227 CallbackServer.java
- •Example A.228 CallbackServerImpl.java
- •Example A.229 CallbackServerDelegate.java
- •Example A.230 CallbackClient.java
- •Example A.231 CallbackClientImpl.java
- •Example A.232 Project.java
- •Example A.233 ProjectItem.java
- •Example A.234 Task.java
- •Example A.235 RunPattern.java
- •Example A.236 PullClient.java
- •Example A.237 ClientPullRequester.java
- •Example A.238 ClientPullServer.java
- •Example A.239 ClientPullServerImpl.java
- •Example A.240 UpdateServerDelegate.java
- •Example A.241 Task.java
- •Example A.242 TaskImpl.java
- •Example A.243 UpdateException.java
- •Example A.244 RunPattern.java
- •Example A.245 Message.java
- •Example A.246 InputChannel.java
- •Example A.247 OutputChannel.java
- •Example A.248 Router.java
- •Example A.249 InputKey.java
- •Example A.250 RouterClient.java
- •Example A.251 Receiver.java
- •Example A.252 RouterGui.java
- •Example A.253 RunPattern.java
- •Example A.254 AppointmentTransactionParticipant.java
- •Example A.255 AppointmentBook.java
- •Example A.256 TransactionException.java
- •Example A.257 Appointment.java
- •Example A.258 AppointmentImpl.java
- •Example A.259 Contact.java
- •Example A.260 ContactImpl.java
- •Example A.261 Location.java
- •Example A.262 LocationImpl.java
- •Example A.263 RunPattern.java
- •Appendix B.
- •Pattern Origins
- •Creational Patterns
- •Behavioral Patterns
- •Structural Patterns
- •System Patterns
- •[Bloch01]
- •[Coplien]
- •[DPCS]
- •[Fowler00]
- •[Jini01]
46.}
Two classes, AddressRetriever and ContactRetriever, implement the RunnableTask interface in this
example. The classes are very similar; both use RMI to request that a business object be retrieved from a server. As their names suggest, each class retrieves a specific kind of business object, making Address and Contact objects from the server available to clients.
Example A.218 AddressRetriever.java
1.import java.rmi.Naming;
2.import java.rmi.RemoteException;
3.public class AddressRetriever implements RunnableTask{
4.private Address address;
5.private long addressID;
6.private String url;
7.
8.public AddressRetriever(long newAddressID, String newUrl){
9.addressID = newAddressID;
10.url = newUrl;
11.}
12.
13.public void execute(){
14.try{
15. ServerDataStore dataStore = (ServerDataStore)Naming.lookup(url);
16. address = dataStore.retrieveAddress(addressID);
17.}
18.catch (Exception exc){
19.}
20.}
21.
22.public Address getAddress(){ return address; }
23.public boolean isAddressAvailable(){ return (address == null) ? false : true; }
24.}
Example A.219 ContractRetriever.java
1.import java.rmi.Naming;
2.import java.rmi.RemoteException;
3.public class ContactRetriever implements RunnableTask{
4.private Contact contact;
5.private long contactID;
6.private String url;
7.
8.public ContactRetriever(long newContactID, String newUrl){
9.contactID = newContactID;
10.url = newUrl;
11.}
12.
13.public void execute(){
14.try{
15. ServerDataStore dataStore = (ServerDataStore)Naming.lookup(url);
16. contact = dataStore.retrieveContact(contactID);
17.}
18.catch (Exception exc){
19.}
20.}
21.
22.public Contact getContact(){ return contact; }
23.public boolean isContactAvailable(){ return (contact == null) ? false : true; }
24.}
The RMI server in this example is defined by the ServerDataStore interface and its implementer,
ServerDataStoreImpl.
Example A.220 ServerDataStore.java
1.import java.rmi.Remote;
2.import java.rmi.RemoteException;
3.public interface ServerDataStore extends Remote{
4.public Address retrieveAddress(long addressID) throws RemoteException;
5.public Contact retrieveContact(long contactID) throws RemoteException;
6.}
Example A.221 ServerDataStoreImpl.java
1.import java.rmi.Naming;
2.import java.rmi.server.UnicastRemoteObject;
339
3.public class ServerDataStoreImpl implements ServerDataStore{
4.private static final String WORKER_SERVER_SERVICE_NAME = "workerThreadServer";
6.public ServerDataStoreImpl(){
7.try {
8. |
UnicastRemoteObject.exportObject(this); |
9. |
Naming.rebind(WORKER_SERVER_SERVICE_NAME, this); |
10.}
11.catch (Exception exc){
12.System.err.println("Error using RMI to register the ServerDataStoreImpl " + exc);
13.}
14.}
15.
16.public Address retrieveAddress(long addressID){
17.if (addressID == 5280L){
18. return new AddressImpl("Fine Dining", "416 Chartres St.", "New Orleans", "LA", "51720");
19.}
20.else if (addressID == 2010L){
21. return new AddressImpl("Mystic Yacht Club", "19 Imaginary Lane", "Mystic", "CT", "46802");
22.}
23.else{
24. return new AddressImpl();
25.}
26.}
27.public Contact retrieveContact(long contactID){
28.if (contactID == 5280L){
29.return new ContactImpl("Dwayne", "Dibley", "Accountant", "Virtucon");
30.}
31.else{
32. return new ContactImpl();
33.}
34.}
36.}
The Address and Contact interfaces define the business objects used in this example, and the implementers AddressImpl and ContactImpl provide underlying functional behavior.
Example A.222 Address.java
1.import java.io.Serializable;
2.public interface Address extends Serializable{
3.public static final String EOL_STRING = System.getProperty("line.separator");
4.public static final String SPACE = " ";
5.public static final String COMMA = ",";
6.public String getType();
7.public String getDescription();
8.public String getStreet();
9.public String getCity();
10.public String getState();
11.public String getZipCode();
12.
13.public void setType(String newType);
14.public void setDescription(String newDescription);
15.public void setStreet(String newStreet);
16.public void setCity(String newCity);
17.public void setState(String newState);
18.public void setZipCode(String newZip);
19.}
Example A.223 AddressImpl.java
1.public class AddressImpl implements Address{
2.private String type;
3.private String description;
4.private String street;
5.private String city;
6.private String state;
7.private String zipCode;
8.
9.public AddressImpl(){ }
10.public AddressImpl(String newDescription, String newStreet,
11.String newCity, String newState, String newZipCode){
12.description = newDescription;
13.street = newStreet;
14.city = newCity;
340
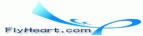
15.state = newState;
16.zipCode = newZipCode;
17.}
18.
19.public String getType(){ return type; }
20.public String getDescription(){ return description; }
21.public String getStreet(){ return street; }
22.public String getCity(){ return city; }
23.public String getState(){ return state; }
24.public String getZipCode(){ return zipCode; }
25.
26.public void setType(String newType){ type = newType; }
27.public void setDescription(String newDescription){ description = newDescription; }
28.public void setStreet(String newStreet){ street = newStreet; }
29.public void setCity(String newCity){ city = newCity; }
30.public void setState(String newState){ state = newState; }
31.public void setZipCode(String newZip){ zipCode = newZip; }
32.
33.public String toString(){
34.return street + EOL_STRING + city + COMMA + SPACE +
35. state + SPACE + zipCode + EOL_STRING;
36.}
37.}
Example A.224 Contact.java
1.import java.io.Serializable;
2.public interface Contact extends Serializable{
3.public static final String EOL_STRING = System.getProperty("line.separator");
4.public static final String SPACE = " ";
5.public String getFirstName();
6.public String getLastName();
7.public String getTitle();
8.public String getOrganization();
9. |
public void setFirstName(String newFirstName); |
|||
10. |
||||
11. |
public void setLastName(String newLastName); |
|||
12. |
public void setTitle(String newTitle); |
|||
13. |
public void setOrganization(String newOrganization); |
|||
14. |
} |
|
|
Y |
|
|
|
|
|
Example A.225 ContactImpl.java |
|
|
L |
|
|
|
F |
||
1. |
|
|
|
|
public class ContactImpl implements Contact{ |
||||
2. |
private String firstName; |
|
|
M |
3. |
private String lastName; |
|
A |
|
4. |
private String title; |
E |
|
|
5. |
private String organization;T |
|
|
|
6. |
|
|
|
|
7.public ContactImpl(){}
8.public ContactImpl(String newFirstName, String newLastName,
9.String newTitle, String newOrganization){
10. firstName = newFirstName;
11. lastName = newLastName;
12. title = newTitle;
13. organization = newOrganization;
14. }
15.
16.public String getFirstName(){ return firstName; }
17.public String getLastName(){ return lastName; }
18.public String getTitle(){ return title; }
19.public String getOrganization(){ return organization; }
21.public void setFirstName(String newFirstName){ firstName = newFirstName; }
22.public void setLastName(String newLastName){ lastName = newLastName; }
23.public void setTitle(String newTitle){ title = newTitle; }
24.public void setOrganization(String newOrganization){ organization = newOrganization; }
26.public String toString(){
27.return firstName + SPACE + lastName + EOL_STRING;
28.}
29.}
RunPattern creates a ConcreteQueue, then uses it to retrieve a sample Contact and two Addresses. The worker
thread in the queue processes these requests as |
queue. The ConcreteQueue and its |
associated worker thread can be used throughout |
client application, performing any background |
TEAM FLY PRESENTS
task required by the client as RunnableTask objects are added to its queue.
341
Example A.226 RunPattern.java
1.import java.io.IOException;
2.public class RunPattern{
3.private static final String WORKER_SERVER_URL = "//localhost/ workerThreadServer";
4.public static void main(String [] arguments){
5.System.out.println("Example for the WorkerThread pattern");
6.System.out.println("In this example, a ConcreteQueue object which uses a");
7.System.out.println(" worker thread, will retrieve a number of objects from");
8.System.out.println(" the server.");
9.System.out.println();
10.
11.System.out.println("Running the RMI compiler (rmic)");
12.System.out.println();
13.try{
14. Process p1 = Runtime.getRuntime().exec("rmic ServerDataStoreImpl");
15. p1.waitFor();
16.}
17.catch (IOException exc){
18.System.err.println("Unable to run rmic utility. Exiting application.");
19. System.exit(1);
20.}
21.catch (InterruptedException exc){
22.System.err.println("Threading problems encountered while using the rmic utility.");
23.}
24.
25.System.out.println("Starting the rmiregistry");
26.System.out.println();
27.Process rmiProcess = null;
28.try{
29. rmiProcess = Runtime.getRuntime().exec("rmiregistry");
30. Thread.sleep(15000);
31.}
32.catch (IOException exc){
33.System.err.println("Unable to start the rmiregistry. Exiting applica-
tion.");
34. System.exit(1);
35.}
36.catch (InterruptedException exc){
37.System.err.println("Threading problems encountered when starting the rmiregistry.");
38.}
39.
40.System.out.println("Creating the queue, which will be managed by the worker thread");
41.System.out.println();
42.ConcreteQueue workQueue = new ConcreteQueue();
43.
44.System.out.println("Creating the RMI server object, ServerDataStoreImpl");
45.System.out.println();
46.ServerDataStore server = new ServerDataStoreImpl();
47.
48.System.out.println("Creating AddressRetrievers and ContactRetreivers.");
49.System.out.println(" These will placed in the queue, as tasks to be");
50.System.out.println(" performed by the worker thread.");
51.System.out.println();
52.AddressRetriever firstAddr = new AddressRetriever(5280L, WORKER_SERVER_URL);
53.AddressRetriever secondAddr = new AddressRetriever(2010L, WORKER_SERVER_URL);
54.ContactRetriever firstContact = new ContactRetriever(5280L, WORKER_SERVER_URL);
56.workQueue.put(firstAddr);
57.workQueue.put(firstContact);
58.workQueue.put(secondAddr);
60.while (!secondAddr.isAddressAvailable()){
61. |
try{ |
62. |
Thread.sleep(1000); |
63. |
} |
64. |
catch (InterruptedException exc){} |
65. |
} |
66. |
|
67.System.out.println("WorkerThread completed the processing of its Tasks");
68.System.out.println("Printing out the retrieved objects now:");
69.System.out.println();
70.System.out.println(firstAddr.getAddress());
342
71.System.out.println(firstContact.getContact());
72.System.out.println(secondAddr.getAddress());
74. }
75.
76.}
343