
CSharp Bible (2002) [eng]-1
.pdf
MyArray[4] = 4;
foreach(int ArrayElement in MyArray) System.Console.WriteLine(ArrayElement);
}
}
The ArrayElement identifier is a variable defined in the foreach loop. It contains the value of an element in the array. The foreach loop visits every element in the array, which is handy when you need to work with each element in an array without having to know the size of the array.
Using jump statements to move around in your code
Jump statements jump to a specific piece of code. Jump statements always execute and are not controlled by any Boolean expressions.
The break statement
You saw the break statement in the section about switch statements. C# also enables you to use the break statement to break out of the current block of statements. Usually, the break statement is used to break out of an iteration statement block:
int MyVariable = 0;
while(MyVariable < 10)
{
System.Console.WriteLine(MyVariable); if(MyVariable == 5)
break;
MyVariable++;
}
System.Console.WriteLine("Out of the loop.");
The preceding code prints the following to the console:
0
1
2
3
4
Out of the loop.
The code reads: "If the value of MyVariable is 5, break out of the while loop." When the break statement executes, C# transfers control to the statement following an iteration statement's embedded statements. The break statement is usually used in switch, while, do, for, and foreach statement blocks.
The continue statement
The continue statement returns control to the Boolean expression that controls an iteration statement, as shown in the following code:
int MyVariable;
for(MyVariable = 0; MyVariable < 10; MyVariable++)
{
if(MyVariable == 5) continue;
System.Console.WriteLine(MyVariable);
}
The preceding code prints the following to the console:
0
1
2
3
4
6
7
8
9
This code reads: "If the value of MyVariable is 5, continue to the next iteration of the for loop without executing any other embedded statements." This is why 5 is missing from the output. When the value of MyVariable is 5, control returns to the top of the for loop and the call to WriteLine() is never made for that iteration of the for loop.
Like the break statement, the continue statement is usually used in switch, while, do, for, and foreach statement blocks.
The goto statement
The goto statement unconditionally transfers control to a labeled statement. You can label any statement in C#. Statement labels are identifiers that precede a statement. A colon follows a statement label. A label identifier follows the goto keyword, and the goto statement transfers control to the statement named by the label identifier, as shown in the following code:
int MyVariable = 0;
while(MyVariable < 10)
{
System.Console.WriteLine(MyVariable); if(MyVariable == 5)
goto Done; MyVariable++;
}
Done: System.Console.WriteLine("Out of the loop.");
The preceding code prints the following to the console:
0
1
2
3
4
Out of the loop.
When the value of MyVariable is 5, the goto statement executes and control transfers to the statement with a label of Done. The goto statement always executes, regardless of any iteration statements that may be executing.
You can also use the goto keyword in conjunction with the case labels in a switch statement, instead of the break statement:
switch(MyVariable)
{
case 123:
System.Console.WriteLine("MyVariable == 123"); goto case 124;
case 124:
System.Console.WriteLine("MyVariable == 124"); break;
}
Note Using the goto statement in a lot of places can make for confusing, unreadable code. The best practice is to avoid using a goto statement if at all possible. Try to restructure your code so that you don't need to use any goto statements.
Using statements to perform mathematical calculations safely
You've already seen how the checked and unchecked keywords can control the behavior of error conditions in your mathematical expressions. You can also use these keywords as statements that control the safety of mathematical operations. Use the keywords before a block of statements that should be affected by the checked or unchecked keywords, as in the following code:
checked
{
Int1 = 2000000000;
Int2 = 2000000000; Int1PlusInt2 = Int1 + Int2;
System.Console.WriteLine(Int1PlusInt2);
}
Summary
C# provides several ways to control the execution of your code, giving you options to execute a block of code more than once, or sometimes not at all, based on the result of a Boolean expression.
The if statement executes code once, but only if the accompanying Boolean expression evaluates to true. The if statement can include an else clause, which executes a code block if the Boolean expression evaluates to false.
The switch statement executes one of many possible code blocks. Each code block is prefixed by a case statement list. C# evaluates the expression in the switch statement and then looks for a case statement list whose value matches the expression evaluated in the switch statement.
The while, do, and for statements continue to execute code as long as a specified Boolean expression is true. When the Boolean expression evaluates to false, the embedded statements
are no longer executed. The while and for statements can be set up so that their Boolean expressions immediately evaluate to false, meaning that their embedded statements never actually execute. The do statement, however, always executes its embedded statements at least once.
The foreach statement provides a nice way to iterate through elements in an array quickly. You can instruct a foreach loop to iterate through the elements in an array without knowing the size of the array or how its elements were populated. The foreach statement sets up a special identifier that is populated with the value of an array element during each iteration of the foreach loop.
The break, continue, and goto statements disrupt the normal flow of an iteration statement, such as while or foreach. The break statement breaks out of the iteration loop, even if the Boolean expression that controls the loop's execution still evaluates to true. The continue statement returns control to the top of the iteration loop without executing any of the embedded statements that follow it. The goto statement always transfers control to a labeled statement.
You can surround mathematical operations in checked or unchecked statements to specify how you want to handle mathematical errors in your C# code.
Chapter 6: Working with Methods
In This Chapter
Methods are blocks of statements that when executed return some type of value. They can be called by name, and calling a method causes the statements in the method to be executed.
You've already seen one method: the Main() method. Although C# enables you to put all of your code in the Main() method, you will probably want to design your classes to define more than one method. Using methods keeps your code readable because your statements are placed in smaller blocks, rather than in one big code block. Methods also enable you to take statements that may be executed multiple times and place them in a code block that can be called as often as needed.
In this chapter, you learn to create functions that do and do not return data. You learn how to pass parameters into methods, and you learn how best to structure a method to make your applications modularized.
Understanding Method Structure
At a minimum, a method is made up of the following parts:
•Return type
•Method name
•Parameter list
•Method body
Note All methods are enclosed in a class. A method cannot exist outside of a class.
Methods have other optional parts, such as attribute lists and scope modifiers. The following sections focus on the basics of a method.
Return type
A method starts out by defining the type of data that it will return when it is called. For example, suppose you want to write a method that adds two integers and returns the result. In that case, you write its return type as int.
C# permits you to write a method that doesn't return anything. For example, you may write a method that simply writes some text to the console, but doesn't calculate any data worth returning to the code that called the method. In that case, you can use the keyword void to tell the C# compiler that the method does not return any data.
If you want to return a value from your method, you use the return keyword to specify the value that should be returned. The return keyword is followed by an expression that evaluates to the type of value to be returned. This expression can be a literal value, a variable, or a more complicated expression.
Cross-Reference Expressions are covered in more detail in Chapter 4.
Method name
All methods must have a name. A method name is an identifier, and method names must follow the naming rules of any identifier. Remember that identifiers must begin with an uppercase or lowercase letter or an underscore character. The characters following the first character can be an uppercase or lowercase letter, a digit, or an underscore.
Parameter list
Methods may be called with parameters that are used to pass data to the method. In the preceding example, in which a method adds two numbers together, you would need to send the method the values of the two integers to be added. This list of variables is called the method's parameter list.
The method's parameter list is enclosed in parentheses and follows the method name. Each parameter in the parameter list is separated by a comma, and includes the parameter's type followed by its name.
You can also prefix parameters in the parameter list with modifiers that specify how their values are used within the method. You'll look at those modifiers later in this chapter.
You can define a method that doesn't take any parameters. If you want to write a method that doesn't take any parameters, simply write the parentheses with nothing inside them. You've already seen this in the Main() methods you've written. You can also place the keyword void inside the parentheses to specify that the method does not accept any parameters.
Method body

The method body is the block of statements that make up the method's code. The method body is enclosed in curly braces. The opening curly brace follows the method's parameter list, and the closing curly brace follows the last statement in the method body.
Calling a Method
To call a method, write its name in the place where the method's code should be executed in your code. Follow the method name with a pair of parentheses, as shown in Listing 6-1. As with all statements in C#, the method call statement must end with a semicolon.
Listing 6-1: Calling a Simple Method
class Listing6_1
{
public static void Main()
{
Listing6_1 MyObject;
MyObject = new Listing6_1(); MyObject.CallMethod();
}
void CallMethod()
{
System.Console.WriteLine("Hello from CallMethod()!");
}
}
Note You need the statements in Main() that create a new Listing6_1 object before you can call the object's methods. Chapter 9 discusses C#'s class and object concepts.
If the method is defined with a parameter list, the parameter values must be specified when you call the method. You must specify the parameters in the same order as they are specified in the method's parameter list, as shown in Listing 6-2.
Listing 6-2: Calling a Method with a Parameter
class Listing6_2
{
public static void Main()
{
int MyInteger; Listing6_2 MyObject;
MyObject = new Listing6_2(); MyObject.CallMethod(2); MyInteger = 3; MyObject.CallMethod(MyInteger);
}
void CallMethod(int Integer)
{
System.Console.WriteLine(Integer);
}
}
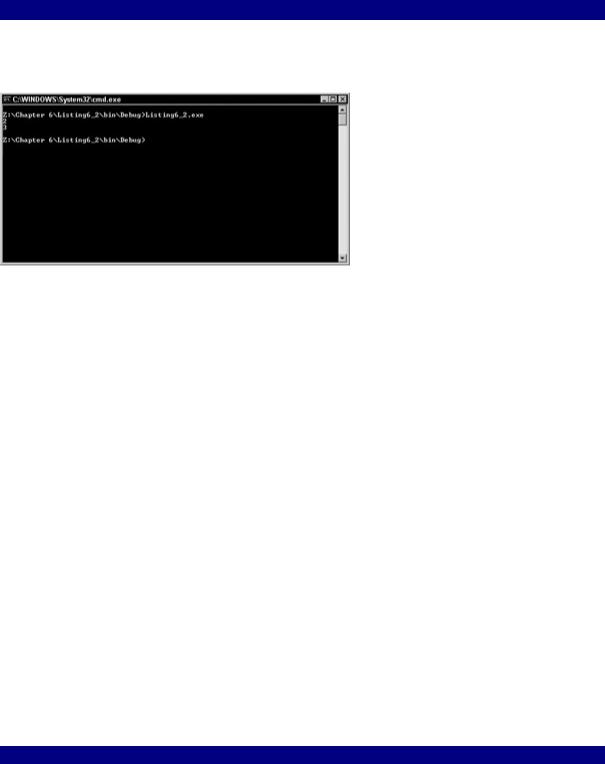
When you compile and run Listing 6-2, you see output similar to that of Figure 6-1.
Figure 6-1: A simple call to a method results in the output shown here.
This is because the Main() method calls the CallMethod() twice: once with a value of 2 and once with a value of 3. The method body of the CallMethod() method writes the supplied value to the console.
When you supply a value for a method parameter, you can use a literal value, as in the 2 in Listing 6-2, or you can supply a variable whose value is used, as in the MyInteger variable in Listing 6-2.
When a method is called, C# takes the values specified and assigns those values to the parameters used in the method. During the first call to CallMethod() in Listing 6-2, the literal 2 is used as the parameter to the method, and the method's Integer parameter is given the value of 2. During the second call to CallMethod() in Listing 6-2, the variable MyInteger is used as the parameter to the method, and the method's Integer parameter is given the value of the MyInteger variable's value: 3.
The parameters that you specify when calling a method must match the types specified in the parameter list. If a parameter in a method's parameter list specifies an int, for example, the parameters you pass into it must be of type int, or a type that can be converted to an int. Any other type produces an error when your code is compiled. C# is a type-safe language, which means that variable types are checked for legality when you compile your C# code. Where methods are concerned, this means that you must specify the correct types when specifying parameters. Listing 6-3 shows the correct types during parameter specification.
Listing 6-3: Type Safety in Method Parameter Lists
class Listing6_3
{
public static void Main()
{
Listing6_3 MyObject;
MyObject = new Listing6_3(); MyObject.CallMethod("a string");
}
void CallMethod(int Integer)

{
System.Console.WriteLine(Integer);
}
}
This code does not compile, as you can see in Figure 6-2.
Figure 6-2: Calling a method with an invalid data type generates compiler errors.
The C# compiler issues these errors because the CallMethod() method is being executed with a string parameter, and the CallMethod() parameter list specifies that an integer must be used as its parameter. Strings are not integers, and cannot be converted into integers, and this mismatch causes the C# compiler to issue the errors.
If the method returns a value, you should declare a variable to hold the returned value. The variable used to hold the return value from the function is placed before the method name, and an equal sign separates the variable's identifier from the method name, as shown in Listing 6- 4.
Listing 6-4: Returning a Value from a Method
class Listing6_4
{
public static void Main()
{
Listing6_4 MyObject;
int ReturnValue;
MyObject = new Listing6_4();
ReturnValue = MyObject.AddIntegers(3, 5); System.Console.WriteLine(ReturnValue);
}
int AddIntegers(int Integer1, int Integer2)
{
int Sum;
Sum = Integer1 + Integer2; return Sum;
}
}

Several things are happening in this code:
•A method called AddIntegers() is declared. The method has two parameters in its parameter list: an integer called Integer1 and an integer called Integer2.
•The method body of the AddIntegers() method adds the two parameter values together and assigns the result to a local variable called Sum. The value of Sum is returned.
•The Main() method calls the AddIntegers() method with values of 3 and 5. The return value of the AddIntegers() method is placed in a local variable called ReturnValue.
•The value of ReturnValue is printed out to the console.
Figure 6-3 contains the results of the program shown in Listing 6-4.
Figure 6-3: Data is returned from a method and shown in the console window.
Understanding Parameter Types
C# allows four types of parameters in a parameter list:
•Input parameters
•Output parameters
•Reference parameters
•Parameter arrays
Input parameters
Input parameters are parameters whose value are sent into the method. All the parameters used up to this point have been input parameters. The values of input parameters are sent into the function, but the method's body cannot permanently change their values.
Listing 6-4, shown in the previous example, defines a method with two input parameters: Integer1 and Integer2. The values for these parameters are input to the method, which reads their values and does its work.
Input parameters are passed by value into methods. The method basically sees a copy of the parameter's value, but is not allowed to change the value supplied by the caller. See Listing 6- 5 for an example.
Listing 6-5: Modifying Copies of Input Parameters
class Listing6_5
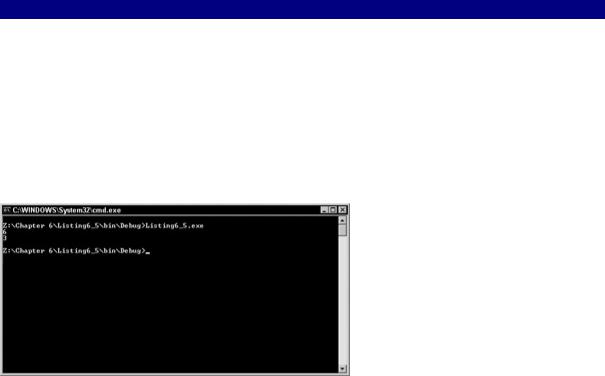
{
public static void Main()
{
int MyInteger; Listing6_5 MyObject;
MyObject = new Listing6_5(); MyInteger = 3; MyObject.CallMethod(MyInteger); System.Console.WriteLine(MyInteger);
}
void CallMethod(int Integer1)
{
Integer1 = 6; System.Console.WriteLine(Integer1);
}
}
In Listing 6-5, the Main() method sets up an integer variable called MyInteger and assigns it a value of 3. It then calls MyMethod() with MyInteger as a parameter. The CallMethod() method sets the value of the parameter to 6 and then prints out its value to the console. When the CallMethod() method returns, the Main() method continues, and prints the value of MyInteger.
When you run this code, the output should resemble that of Figure 6-4.
Figure 6-4: Demonstrate input parameters with the CallMethod() function.
This output occurs because the CallMethod() method modifies its copy of the input parameter, but that modification does not affect the value of the original method supplied by Main(). The value of MyInteger is still 3 after the CallMethod() method returns, because CallMethod() cannot change the value of the caller's input parameter. It can only change the value of its copy of the value.
Output parameters
Output parameters are parameters whose values are not set when the method is called. Instead, the method sets the values and returns the values to the caller through the output parameter.