
Beginning Apache Struts - From Novice To Professional (2006)
.pdf
8 |
C H A P T E R 2 ■ S E R V L E T A N D J S P R E V I E W |
■Note If you’re already using Tomcat, be sure you’re using 4.x or (better) 5.0.x, because higher versions (5.5.x and above) require JSDK 1.5 and versions lower than 4.x require some fiddling to work with Struts.
3.Unzip the contents of the zip file, ensuring that the Create Folders option is selected. The directory jakarta-tomcat-5.0.28 should appear.
4.Locate the directory of your Java SDK installation. This directory is known as the JAVA_HOME directory.
■Note I’m assuming you have installed the 1.4 JSDK.
5.Open .\jakarta-tomcat-5.0.28\bin\startup.bat with a text editor such as Notepad and put in an extra line at the start to set up the JAVA_HOME path. For example, if the JDK is in C:\jdk1.4.2\, you’d use
JAVA_HOME=C:\jdk1.4.2\
6.Repeat Step 5 for the shutdown.bat batch file.
■Note If you’re using Linux or some *nix OS, the files to amend are startup.sh and shutdown.sh.
Now test your Tomcat installation:
7.Double-click on the startup.bat batch file. You should see a DOS screen appear (see Figure 2-1).
8.Open the URL http://localhost:8080 with your favorite web browser. You should see the default Tomcat welcome web page (Figure 2-2).
9.When you’re done, double-click the shutdown.bat batch file to stop Tomcat.

C H A P T E R 2 ■ S E R V L E T A N D J S P R E V I E W |
9 |
Figure 2-1. Tomcat starting up
Figure 2-2. The Apache Tomcat welcome page

10 |
C H A P T E R 2 ■ S E R V L E T A N D J S P R E V I E W |
SHOULD YOU ENCOUNTER PROBLEMS...
If you encounter problems, check the following:
•That you’ve got the JAVA_HOME path right. If startup.bat terminates abruptly, this is likely the problem.
•That you don’t have another web server (like IIS or PWS) or application latched on to port 8080.
•If you have a firewall running on your PC, ensure that it isn’t blocking traffic to and from 8080. Also ensure that it doesn’t block shutdown.bat.
If none of this works, try putting a pause DOS command at the end of the batch file to read the error message. Try using Google with the error message. Nine times out of ten, someone else has had the same problem and posted a solution on the Internet.
Servlet Container Basics
Now that you have installed Tomcat, you have to know how to install your web applications on it. The information in this section applies to any conformant servlet container, not just Tomcat.
Apart from the bin directory in which startup.bat and shutdown.bat live, the most other important directory is the webapps directory, which will contain your web applications.
A web application is bundled as a WAR file (for Web Application aRchive), which is just an ordinary JAR file with the .war extension. To deploy a web application, you simply drop its WAR file in the webapps directory and presto! Tomcat automatically installs it for you. You know this because Tomcat will create a subdirectory of webapps with the same name as your web application.
■Note If you want to re-deploy a web application, you have to delete the existing web application’s
subdirectory. Most servlet containers will not overwrite an existing subdirectory.
The WAR archive file must contain the following:
•A WEB-INF directory, into which you put a file called web.xml, used to configure your webapp. Struts comes with a web.xml file that you must use.
•A WEB-INF\lib directory, into which you put all Struts JAR files, including any thirdparty JAR files your application uses.

C H A P T E R 2 ■ S E R V L E T A N D J S P R E V I E W |
11 |
•A WEB-INF\classes directory, into which go the .class files for your webapp.
•Your JSP, HTML, image, CSS, and other files. JSP and HTML files are usually on the “root” of the WAR archive, CSS usually in a styles subdirectory, and images in a (surprise!) images subdirectory.
Figure 2-3 illustrates this information.
Figure 2-3. Structure of a simple WAR file
Don’t worry too much about having to memorize these details for the time being. Future labs should make them second nature to you.

12 |
C H A P T E R 2 ■ S E R V L E T A N D J S P R E V I E W |
Important Servlet Classes
In the introduction to this chapter, I told you that you didn’t have to know much about servlets in order to use Struts.
This is true, except for two servlet helper classes that are very important to Struts. You need to pay special attention to these. They will certainly be used in your Struts applications:
•HttpServletRequest: Used to read parameter values on an incoming HTTP request and read or set “attributes” that you can access from your JSPs.
•HttpSession: Represents the current user session. Like HttpServletRequest, HttpSession also has get and set functions for attributes but they are associated with the user session instead of the request.
These objects represent “scopes,” or lifetimes, of variables on your webapp. I’ll explain scopes in more detail in the next chapter.
Figure 2-4 illustrates the most used functions on these two classes.
Figure 2-4. HttpServletRequest and HttpSession
Note that you can obtain an instance of HttpSession from HttpRequestServlet using the latter’s getSession() function. The important functions on both classes are also listed in Appendix B.

C H A P T E R 2 ■ S E R V L E T A N D J S P R E V I E W |
13 |
JavaServer Pages (JSP)
Earlier, I mentioned that servlets are Java classes that generate an appropriate response to an HTTP request. This response is usually an HTML page displaying the results of a computation.
Unfortunately, a pure Java class is often not the easiest way to generate HTML. Your typical web page contains lots of text, and your servlet subclass would have to contain or access this text somewhere. In the worst-case scenario, you’d have Java classes containing lots of text and HTML tags. And don’t forget, you’d have to escape every double quote. Trust me, it’s not a pretty sight.
The problem is that Java classes (and therefore servlet subclasses) are code-centric, and are not geared to allow the programmer to easily display large quantities of text, like a web page.
JavaServer Pages (JSP) is a solution to this problem. A JSP page is text-centric, but allows developers full access to the Java programming language via scriptlets. These consist of Java code embedded in the JSP file between special marker tags, <% ... %>, as shown in Listing 2-1.
■Note The servlet container (like Tomcat) actually converts a JSP page to a regular servlet, then compiles it behind the scenes. This happens every time the JSP page is changed.
In the non-Java world, the closest analog to JSP would be PHP or Active Server Pages (ASP). Listing 2-1 illustrates a simple JSP page, which I’ll briefly analyze in the following
subsections:
Listing 2-1. Hello.jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <%@ taglib uri="/tags/time" prefix="time" %>
<html>
<h1>Hello <%= "world".toUpperCase() %></h1>, and the time is now <time:now format="hh:mm"/>.
</html>
Figure 2-5 shows how Hello.jsp might appear in the web browser.
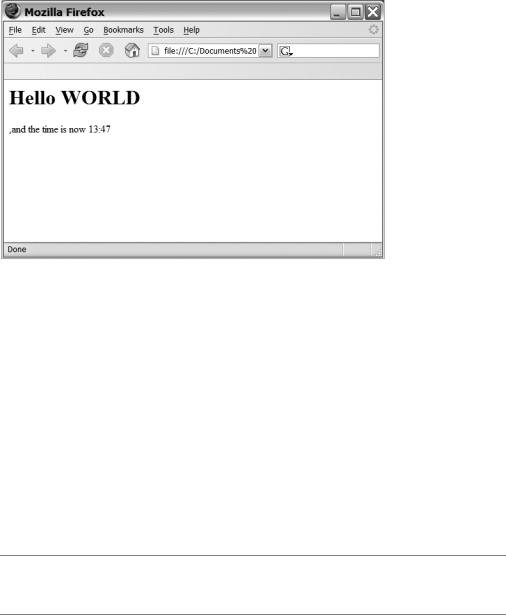
14 |
C H A P T E R 2 ■ S E R V L E T A N D J S P R E V I E W |
Figure 2-5. Hello.jsp viewed in a web browser
Deconstructing Hello.jsp
The first line in Hello.jsp is a header, indicating the type of output (text/html, using UTF-8 encoding), and the scripting language used (Java).
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
Immediately following this header is a tag library declaration:
<%@ taglib uri="/tags/time" prefix="time" %>
This declaration tells the servlet container that this JSP page uses a tag library. A tag library defines custom tags that you can create and use to put some prepackaged functionality into a JSP page.
■Note In this book, I’ll use the term custom tag, but the correct term is custom action. I’ve avoided being politically correct because “action” is an often-used word in Struts. Also, I find the term custom tag more intuitive.
In this instance, the prepackaged functionality is a time display:
<time:now format="hh:mm"/>
I should warn you that this “time” tag library is purely fictitious. I made it up just to show you how custom tags might be used. Struts uses custom tags extensively, and I’ll cover this in detail in Chapter 4.

C H A P T E R 2 ■ S E R V L E T A N D J S P R E V I E W |
15 |
Finally, as Listing 2-1 shows, I’ve made gratuitous use of a scriptlet:
<%= "world".toUpperCase() %>
This is simply an embedding of Java code in the JSP page. Notice the all-important equal sign (=) right after the start of the scriptlet tag <%. This means the evaluated value of the Java code is to be displayed. It is also possible to have scriptlets that do not display anything but instead produce some side effect, as shown in Listing 2-2.
Listing 2-2. Scriptlets in Action
<%@ page contentType="text/html;charset=UTF-8" language="java" %> <%
double g = (Math.sqrt(5) + 1)/2;
%>
The golden ratio is: <%= g %>
In Listing 2-2 there are two scriptlets. The first silently calculates a number (no = sign), and the second displays the calculated value (with an = sign).
The other instructive thing about Listing 2-2 is that it demonstrates how to define a variable in one scriptlet and access it in other scriptlets within the same page.
■Note Every JSP page has a predefined variable called request that is the HttpServletRequest instance associated with the current page.
The current trend in JSP has been to try to replace the use of scriptlets with custom tags. This is largely because using scriptlets results in less readable JSPs than using custom tags. I’ll revisit this idea again in Chapter 10, where I’ll introduce the JSP Standard Tag Library (JSTL).
Final Thoughts
This section should be old hat to readers who have even a little experience using JSP. If you’re from the ASP/PHP camps, you should have little difficulty mapping your knowledge in those technologies to JSP.
The next two chapters give you an in-depth look at two aspects of JSP technology that even many experienced JSP developers might not grasp fully, but that are crucial to your understanding of Struts.
16 |
C H A P T E R 2 ■ S E R V L E T A N D J S P R E V I E W |
Useful Links
•The Tomcat website http://jakarta.apache.org/tomcat/
•Further information on servlets: http://java.sun.com/products/servlet/docs.html
•The Servlet specification: http://java.sun.com/products/servlet/download.html
•Pro Jakarta Struts (Apress 2005): http://www.apress.com/book/bookDisplay. html?bID=228
Summary
•Tomcat’s .\webapps directory is where you deploy your web application.
•Webapps are deployed as WAR files, which are just JAR files with a .war extension.
•WAR files contain a .\WEB-INF directory, with a web.xml file to configure Tomcat.
•Your Java classes and third-party JAR files go into WEB-INF\classes and WEB-INF\lib, respectively.
•Two very important servlet classes are HttpServletRequest and HttpSession.

C H A P T E R 3
■ ■ ■
Understanding Scopes
In servlet-speak, a variable’s “scope” refers to its lifetime in a web application—how long the variable exists in the course of a user’s interaction with the system. A variable’s scope also determines its visibility—who can read and change the variable. Web applications routinely utilize this concept, so you must become thoroughly familiar with it. You’ll do just that in this chapter.
Servlet technology uses four types of scope. Listed in decreasing lifetimes, they are as follows:
•Application scope: Application-scoped variables are visible to every page of the web application, all the time. The variable is visible to all users of the system and exists across multiple user sessions. In other words, different users can potentially view and change the same variable at any time and from any page.
•Session scope: Session-scoped variables exist only for a single-user session, but they are visible to every page in that session. Users would not be able to view or change each other’s instance of the variable.
•Request scope: Request-scoped variables exist only for a single request. This includes page redirects. So, if request-scoped variable x = 7 on the first page is redi-
rected to a second page, then x = 7 on the second page too.
•Page scope: A page-scoped variable exists only on the current page. This does not include redirects. So, if a page-scoped variable x = 8 on the first page is redirected to a second page, then x is undefined on the second page.
■Note A redirect occurs when you ask for one page but are given another. The page you asked for redirects you to the page you actually get. You can do this in JSP with the <jsp:forward> tag, which you will see in action
in Lab 3 later in this chapter.
17