
Beginning REALbasic - From Novice To Professional (2006)
.pdf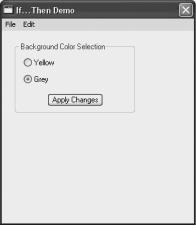
166 |
C H A P T E R 6 ■ M A K I N G D E C I S I O N S W I T H C O N D I T I O N A L L O G I C |
The If…Then…Else Block
You can expand the If…Then block to include an optional Else statement. When you do so, you provide an alternative course of action that should be taken if the tested condition turns out to be False instead of True. For example, suppose you create the application you see in Figure 6-1.
Figure 6-1. Using conditional logic to modify a window’s background color
In this example, a GroupBox control has been added to the application’s window and populated with two RadioButton controls, each of which is associated with a different color. A PushButton has also been added to the GroupBox control. By adding the following code statements to the PushButton control, you provide the user with the capability to change the window’s background color.
If rbnYellow.Value = True Then
Window1.HasbackColor = True
Window1.BackColor = &cFFFF80
End If
If rbnGrey.Value = True Then
Window1.HasbackColor = True
Window1.BackColor = &cC0C0C0
End If

C H A P T E R 6 ■ M A K I N G D E C I S I O N S W I T H C O N D I T I O N A L L O G I C |
167 |
■Note A RadioButton control is used to present users with a choice between two options. By default, all RadioButton controls on a window function as a group, meaning only one RadioButton can be selected at a time. By grouping RadioButton controls together, using the GroupBox control as an example, you can create separate groupings of RadioButton controls, each of which is independent of other groups.
You can use the Properties window to specify a default RadioButton selection by enabling the Value property for one of the RadioButton controls in a grouping. Programmatically, you can change the currently selected RadioButton by setting its Value property to True. Similarly, you can check to see if a RadioButton control’s Value property has been set, as the previous example shows.
As you can see, two If…Then blocks are set up. The first If…Then block checks to see if the Value property belonging to the first RadioButton control is set to True (for example, that it has been selected). If it was selected, then the next statement executes and sets the BackColor property of the window to Yellow.
The second If…Then block performs a second test, this time looking to see if the value of the second RadioButton control was selected. Figure 6-2 demonstrates how the selection of the RadioButton labeled Yellow changes the window’s background color.
Figure 6-2. Using If…Then blocks to process the value of RadioButton controls
168 |
C H A P T E R 6 ■ M A K I N G D E C I S I O N S W I T H C O N D I T I O N A L L O G I C |
The problem with the previous example is this: if the user selected the first RadioButton control, REALbasic is still required to process the second If…Then block, even though it is not selected. Rather than creating multiple If…Then blocks, as was done in the previous example, you can set things up to be more efficient using the Else statement to set up an If…Then…Else block as the following shows.
If rbnYellow.Value = True Then
Window1.BackColor = &cFFFF80
Else
Window1.BackColor = &cC0C0C0
End If
As you can see, this example is one line shorter than the previous example, yet it performs exactly the same thing, while simplifying the code so REALbasic only has to perform one conditional test.
The If…Then…ElseIf Blocks
REALbasic also provides you with the capability to set up If…Then…ElseIf blocks, which test for any number of possible conditions and execute the code statements associated with the first matching condition. For example, you could add an additional RadioButton control to the previous example, and then modify the code statements assigned to the PushButton control, as the following shows.
If rbnYellow.Value = True Then
Window1.BackColor = &cFFFF80
ElseIf rbnGrey.Value = True Then
Window1.BackColor = &cC0C0C0
ElseIf rbnWhite.Value = True Then
Window1.BackColor = &cFFFFFF
End If
In this example, three separate conditions are tested and, whichever one proves True, is executed, while the others are skipped.
Nesting If…Then Blocks
REALbasic also enables you to embed, or nest, If…Then blocks within one another. In doing so, you can create complex conditional tests that begin by testing for one condition, and then perform further testing when required, as the following example shows.
If blnGameOver = True Then
If intNoOfPoints <= 1000 Then
MsgBox("Continue your training my young padewan learner.")
End If

C H A P T E R 6 ■ M A K I N G D E C I S I O N S W I T H C O N D I T I O N A L L O G I C |
169 |
If intNoOfPoints >= 1001 Then
If intNoOfPoints <= 10000 Then
MsgBox("Your Jedi skills are indeed most impressive!")
End If
End If
If intNoOfPoints >= 10001 Then
MsgBox("Congratulations Master Jedi, you are truly strong with the force.") End If
End If
In this example, the value of blnGameOver is tested to see if it is equal to True (for example, if it is time to end the game). If it is time, a number of nested If…Then blocks are executed to determine the player’s score.
■Tip You can create extremely complex testing logic by embedding If…Then statements and blocks. However, remember, nesting too deeply can make your program code difficult to maintain and understand. As an alternative to embedding If…Then statements, you can use logical operators to combine comparison operations. Logical operators are covered in the section “Logical Operators.”
The Select…Case Block
At times, you might want to test a single condition against a number of possible values. While you can certainly perform this type of test using an If...Then…ElseIf block, REALbasic also provides you with the Select Case block, which is better suited to performing this type of test.
While an If…Then…ElseIf block evaluates each ElseIf statement, a Select Case block stops executing once a matching Case statement is found, making it more efficient. The following outlines the syntax for the Select Case block.
Select Case expression
Case value statements
Case value statements
Case Else statements
End Select
The Select…Case block begins with the Select Case statement and ends with the End Select statement. Individual Case statements are defined inside the Select Case block that identifies possible matching values. When a match occurs, the code statements in between the matching Case statement and the next Case statement are executed.

170 |
C H A P T E R 6 ■ M A K I N G D E C I S I O N S W I T H C O N D I T I O N A L L O G I C |
■Note Select…Case statements can include an optional Case Else statement that executes only when none of the defined Case statements match up against the tested value. Note, the Select Case block accepts either Case Else or Else as the format of the optional Else clause.
As you can see, a Select Case block is easier to read when compared to nested If…Then blocks. In addition, Select Case blocks typically require less code statements to set up. The following example demonstrates how to set up a typical Select Case block.
Select Case strCustomerName
Case "Walmart"
MsgBox("Customer account number is 6765765765765.")
Return
Case "Target"
MsgBox("Customer account number is 6769382576767.")
Return
Case "Roses"
MsgBox("Customer account number is 1231435 456755.")
Return
Case "Sears"
MsgBox("Customer account number is 98978562546573.")
Return
Case Else
MsgBox("An account must be set up for this new customer.")
End Select
In this example, a message is displayed depending on whether the value assigned to the strCustomerName variables match any of the values specified in the Case statements. However, if no match is found, the Case Else statement executes.
Case statements are flexible. For example, you can set them up to check for a range of values using the To keyword, as the following shows.
Select Case intNoOfPoints Case 1 To 1000
MsgBox("Continue your training my young padewan learner.") Return
Case 1001 To 10000
MsgBox("Your Jedi skills are indeed most impressive!") Return
Case 10001 To 1000000
MsgBox("Congratulations Master Jedi, you are truly strong with the force.") Return
End Select

C H A P T E R 6 ■ M A K I N G D E C I S I O N S W I T H C O N D I T I O N A L L O G I C |
171 |
You can also set up Case statements using different ranges or types of values, as the following shows.
Select Case intMonthOfBirth Case 1, 4, 7, 10
MsgBox("Department birthday celebrations occur on the 15th of the month.") Return
Case 2, 5, 8, 11
MsgBox("Department birthday celebrations occur on the 21st of the month.") Return
Case 3, 6, 9, 12
MsgBox("Department birthday celebrations occur on the 1st of the month.") Return
End Select
#If…#EndIf
One of REALbasic’s primary selling features is its capability to compile applications that can run on different OS platforms. Each OS platform has certain, completely unique features. For example, only Windows OSs support the registry.
To develop applications that can execute on certain OSs, at times, you need to customize portions of your application to leverage OS-specific features. To provide you with a mechanism for handling these situations, REALbasic provides the #If…#EndIf block.
The #If…#EndIf block can be used to handle other situations, too, such as supporting the creation of applications that require features found in certain versions of REALbasic or that run differently in debug mode versus as a standalone application. REALbasic limits the #If…#EndIf block’s functionality by only allowing it to work with a specific set of Boolean constants, which Table 6-1 shows.
Table 6-1. REALbasic Boolean Constants
Constant |
Classification |
Description |
DebugBuild |
Debug vs. Standalone |
Evaluates to True when the application is run |
|
|
within the REALbasic IDE. |
RBVersion |
REALbasic Version |
Returns a True or False value, indicating the |
|
|
version level being used to run a REALbasic |
|
|
application. |
TargetHasGUI |
Application Type |
Returns a value of True if the application being |
|
|
run is a Desktop application (as opposed to a |
|
|
Console, Service, or Event Driven Console |
|
|
application). |
TargetBigEndian |
OS |
Returns a value of True if the application being |
|
|
run uses the Big Endian byte order (e.g., |
|
|
Macintosh systems). |
Continued

172 C H A P T E R 6 ■ M A K I N G D E C I S I O N S W I T H C O N D I T I O N A L L O G I C
Table 6-1. Continued
Constant |
Classification |
Description |
TargetLittleEndian |
OS |
Returns a value of True if the application being |
|
|
run uses the Little Endian byte order (e.g., PCs). |
TargetCarbon |
OS |
Returns a value of True if the application being |
|
|
run executes Carbon/Mac OS X code (e.g., Mac |
|
|
Classic and Mac OS X). |
TargetMachO |
OS |
Returns a value of True if the application is |
|
|
being run on a Macintosh computer running |
|
|
Mac OS X. |
TargetMacOS |
OS |
Returns a value of True if the application is |
|
|
being run on a Macintosh computer running |
|
|
either Mac Classic or Mac OS X. |
TargetMacOSClassic |
OS |
Returns a value of True if the application is |
|
|
being run on a Macintosh computer running |
|
|
Mac Classic. |
TargetLinux |
OS |
Returns a value of True if the application is |
|
|
being run on a Linux computer. |
TargetWin32 |
OS |
Returns a value of True if the application is |
|
|
being run on a Windows computer. |
|
|
|
To understand the #If…#EndIf statement, seeing it in action can help. The following statements demonstrate how to test for the OS being used to run the application.
#If TargetMachO
MsgBox("This application is running on a computer running on Mac OS X.") #ElseIf TargetWin32
MsgBox("This application is running on a computer running on Windows.") #ElseIf TargetLinux
MsgBox("This application is running on a computer running on Linux.") #EndIf
■Note As the previous example demonstrates, the Then keyword is optional when working with the #If…#EndIf statement.
For another example of how to use the #If…#EndIf code block, check out the RB Word Processor application in Chapter 9.

C H A P T E R 6 ■ M A K I N G D E C I S I O N S W I T H C O N D I T I O N A L L O G I C |
173 |
REALbasic Operators
As you have already seen in this book, REALbasic uses comparison operators in the formulation of program statements that involve conditional logic. REALbasic also uses mathematical operators when working with numbers. In addition to comparison and mathematical operators, REALbasic also supports the use of logical operators that facilitate the testing of more than one condition at a time.
Comparison Operators
For REALbasic to make any type of comparison, it must be told what type of comparison to make. In most of the examples you have seen up to this point, comparisons were made based on equality. In other words, the equals (=) operator was used to ask REALbasic to determine if two values were equal.
In addition to checking for equality, REALbasic provides you with a collection of other comparison operators that give you with the capability to check for a range of values. Table 6-2 provides a complete list of REALbasic’s comparison operators.
Table 6-2. REALbasic Comparison Operators
Operator |
Type |
Example |
= |
Equals |
If X = 18 Then |
<> |
Not Equals |
If X <> 18 |
> |
Greater Than |
If X > 18 |
< |
Less Than |
If X < 18 |
>= |
Greater Than or Equal To |
If X >= 21 |
<= |
Less Than or Equal To |
If X <= 21 |
|
|
|
You have the chance to work with a number of these operators when you create the RB Number Guess game in the section “Creating a Computer Game.”
■Note In addition to using REALbasic’s comparison operators for the obvious task of comparing numeric data, you can also use them when you work with string values. String comparisons are case-insensitive, meaning “Bob” and “bob” are equal to one another. However, “Ann” is considered less than “Bob” because, alphabetically, “Ann” comes before “Bob.”

174 |
C H A P T E R 6 ■ M A K I N G D E C I S I O N S W I T H C O N D I T I O N A L L O G I C |
Mathematical Operators
REALbasic’s mathematical operators are based on the same set of operators we all use for common everyday math. Anyone familiar with a modern calculator should have no trouble recognizing each of the mathematical operators listed in Table 6-4 or understanding their function.
OPERATOR PREDEDENCE
One important concept you need to be aware of when working with mathematical operators is the order of precedence in which REALbasic performs mathematic operations. Table 6-3 lists the order in which REALbasic mathematic operator precedence occurs. Note, operators listed at the top of Table 6-3 are processed before those listed later in the table.
Table 6-3. Order of Precedence for REALbasic Mathematic Operators
Operator |
Description |
*, /, \ |
Multiplication and division occur first and are processed from left to right |
Mod |
Modulo is processed after all multiplication and division |
+, - |
Addition and subtraction are processed last, starting from left to right |
|
|
To help better understand the order in which REALbasic processes mathematical operators, consider the following statement.
intTestValue = 10 /2 + 3 – 1 * 3
REALbasic solves this equation, as the following outlines.
1.Divide 10 by 2 to get 5.
2.Multiply 1 * 3 to get 3.
3.Add 5 + 3 – 3 to get 5.
If you want, you can use parentheses to control the order in which REALbasic solves an equation. For example, if the equation were rewritten as you see in the following:
intTestValue = 10 /2 + (3 – 1) * 3
REALbasic would solve it as shown here.
1.Subtract 1 from 3 to get 2.
2.Divide 10 by 2 to get 5.
3.Multiply 2 * 3 to get 6.
4.Add 5 + 6 to get 11.

C H A P T E R 6 ■ M A K I N G D E C I S I O N S W I T H C O N D I T I O N A L L O G I C |
175 |
Table 6-4. REALbasic Mathematical Operators
Operator |
Type |
Example |
||
+ |
Add |
5 + 5 = 10 |
||
- |
Subtract |
10 – 5 = 5 |
||
* |
Multiply |
5 * 5 = 25 |
||
\ |
Division (Integer) |
15 |
\ 2 |
= 7 |
/ |
Division (Floating Point) |
15 |
/ 2 |
= 7.5 |
Mod |
Modulo |
15 Mod 2 = 1 |
||
|
|
|
|
|
An example of how to work with most of these mathematical operators was provided in RB Calculator application presented in Chapter 5.
Logical Operators
At times, you may want to combine comparison operations together to make a decision. For example, you might want to execute a given set of code statements only when both the values of X and Y are greater than to zero. You could set up this test as the following shows.
If X > 0 Then
If Y > 0 Then
MsgBox("Both X and Y are greater than zero.")
End If
End If
Using the REALbasic And logical operator, however, you could rewrite these statements as in the following.
If X > 0 And Y > 0 Then
MsgBox("Both X and Y are greater than zero.")
End If
As you can see, using the And operator saves you two lines of code and makes things easier to read and understand. Table 6-5 lists the logical operators supported by REALbasic and describes their function.
Table 6-5. REALbasic Logical Operators
Operator Type |
Example |
And |
Both comparisons are True |
X >= 0 And Y >= 5 |
Or |
Either comparison is True |
X = 0 Or Y = 0 |
Not |
Reverses the value of a Boolean value |
Not (X > Y) |
|
|
|