
- •Table of Contents
- •Foreword
- •Preface
- •Acknowledgments
- •Item 1: Consider providing static factory methods instead of constructors
- •Item 2: Enforce the singleton property with a private constructor
- •Item 3: Enforce noninstantiability with a private constructor
- •Item 4: Avoid creating duplicate objects
- •Item 5: Eliminate obsolete object references
- •Item 6: Avoid finalizers
- •Item 7: Obey the general contract when overriding equals
- •Item 8: Always override hashCode when you override equals
- •Item 9: Always override toString
- •Item 10: Override clone judiciously
- •Item 11: Consider implementing Comparable
- •Item 12: Minimize the accessibility of classes and members
- •Item 13: Favor immutability
- •Item 14: Favor composition over inheritance
- •Item 15: Design and document for inheritance or else prohibit it
- •Item 16: Prefer interfaces to abstract classes
- •Item 17: Use interfaces only to define types
- •Item 18: Favor static member classes over nonstatic
- •Item 19: Replace structures with classes
- •Item 20: Replace unions with class hierarchies
- •Item 21: Replace enum constructs with classes
- •Item 22: Replace function pointers with classes and interfaces
- •Item 23: Check parameters for validity
- •Item 24: Make defensive copies when needed
- •Item 25: Design method signatures carefully
- •Item 26: Use overloading judiciously
- •Item 27: Return zero-length arrays, not nulls
- •Item 28: Write doc comments for all exposed API elements
- •Item 29: Minimize the scope of local variables
- •Item 30: Know and use the libraries
- •Item 31: Avoid float and double if exact answers are required
- •Item 32: Avoid strings where other types are more appropriate
- •Item 33: Beware the performance of string concatenation
- •Item 34: Refer to objects by their interfaces
- •Item 35: Prefer interfaces to reflection
- •Item 36: Use native methods judiciously
- •Item 37: Optimize judiciously
- •Item 38: Adhere to generally accepted naming conventions
- •Item 39:Use exceptions only for exceptional conditions
- •Item 40:Use checked exceptions for recoverable conditions and run-time exceptions for programming errors
- •Item 41:Avoid unnecessary use of checked exceptions
- •Item 42:Favor the use of standard exceptions
- •Item 43: Throw exceptions appropriate to the abstraction
- •Item 44:Document all exceptions thrown by each method
- •Item 45:Include failure-capture information in detail messages
- •Item 46:Strive for </vetbfailure atomicity
- •Item 47:Don't ignore exceptions
- •Item 48: Synchronize access to shared mutable data
- •Item 49: Avoid excessive synchronization
- •Item 50: Never invoke wait outside a loop
- •Item 51: Don't depend on the thread scheduler
- •Item 52: Document thread safety
- •Item 53: Avoid thread groups
- •Item 54: Implement Serializable judiciously
- •Item 55:Consider using a custom serialized form
- •Item 56:Write readObject methods defensively
- •Item 57: Provide a readResolve method when necessary
- •References
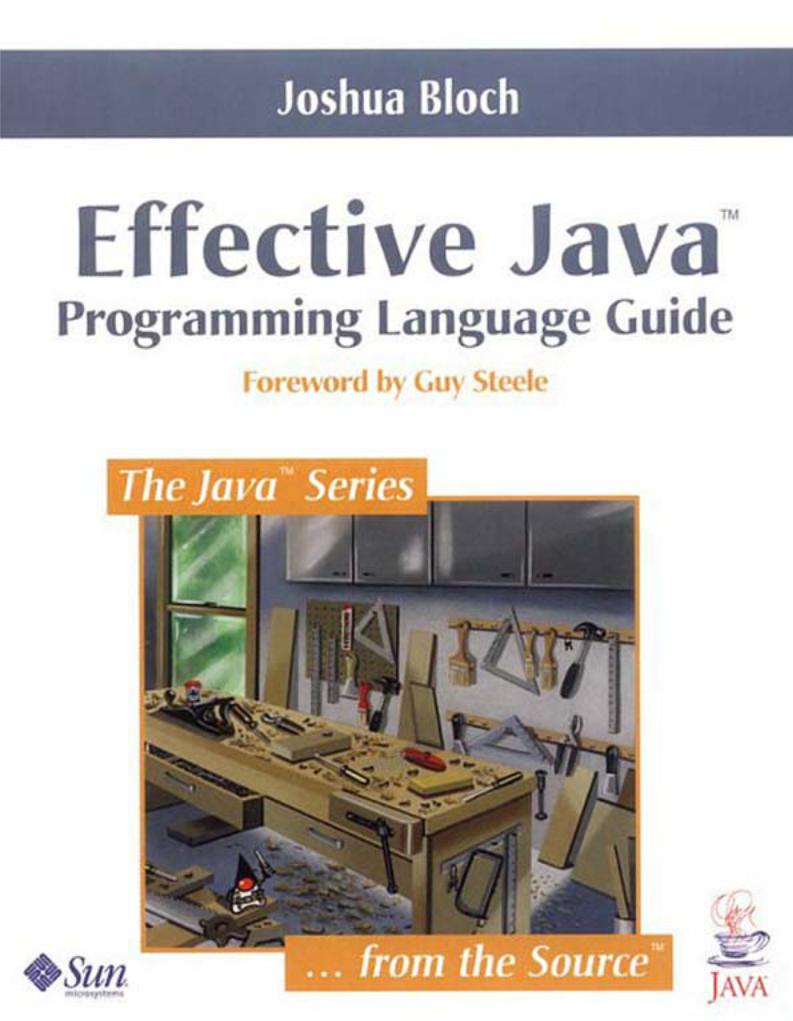
Effective Java: Programming Language Guide
Joshua Bloch
Publisher: Addison Wesley
First Edition June 01, 2001
ISBN: 0-201-31005-8, 272 pages
Are you ready for a concise book packed with insight and wisdom not found elsewhere? Do you want to gain a deeper understanding of the Java programming language? Do you want to write code that is clear, correct, robust, and reusable? Look no further! This book will provide you with these and many other benefits you may not even know you were looking for.
Featuring fifty-seven valuable rules of thumb, Effective Java Programming Language Guide contains working solutions to the programming challenges most developers encounter each day. Offering comprehensive descriptions of techniques used by the experts who developed the Java platform, this book reveals what to do - and what not to do - in order to produce clear, robust and efficient code.
Table of Contents |
|
Foreword ............................................................................................................................... |
1 |
Preface ................................................................................................................................... |
3 |
Acknowledgments................................................................................................................. |
4 |
Chapter 1. Introduction....................................................................................................... |
5 |
Chapter 2. Creating and Destroying Objects .................................................................... |
8 |
Item 1: Consider providing static factory methods instead of constructors....................... |
8 |
Item 2: Enforce the singleton property with a private constructor................................... |
11 |
Item 3: Enforce noninstantiability with a private constructor.......................................... |
13 |
Item 4: Avoid creating duplicate objects.......................................................................... |
13 |
Item 5: Eliminate obsolete object references ................................................................... |
16 |
Item 6: Avoid finalizers.................................................................................................... |
19 |
Chapter 3. Methods Common to All Objects .................................................................. |
23 |
Item 7: Obey the general contract when overriding equals............................................ |
23 |
Item 8: Always override hashCode when you override equals...................................... |
31 |
Item 9: Always override toString.................................................................................. |
35 |
Item 10: Override clone judiciously ............................................................................... |
37 |
Item 11: Consider implementing Comparable................................................................. |
44 |
Chapter 4. Classes and Interfaces..................................................................................... |
48 |
Item 12: Minimize the accessibility of classes and members .......................................... |
48 |
Item 13: Favor immutability ............................................................................................ |
50 |
Item 14: Favor composition over inheritance .................................................................. |
57 |
Item 15: Design and document for inheritance or else prohibit it.................................... |
61 |
Item 16: Prefer interfaces to abstract classes ................................................................... |
65 |
Item 17: Use interfaces only to define types .................................................................... |
69 |
Item 18: Favor static member classes over nonstatic....................................................... |
71 |
Chapter 5. Substitutes for C Constructs .......................................................................... |
75 |
Item 19: Replace structures with classes.......................................................................... |
75 |
Item 20: Replace unions with class hierarchies ............................................................... |
76 |
Item 21: Replace enum constructs with classes ................................................................ |
80 |
Item 22: Replace function pointers with classes and interfaces....................................... |
88 |
Chapter 6. Methods............................................................................................................ |
91 |
Item 23: Check parameters for validity............................................................................ |
91 |
Item 24: Make defensive copies when needed................................................................. |
93 |
Item 25: Design method signatures carefully................................................................... |
96 |
Item 26: Use overloading judiciously .............................................................................. |
97 |
Item 27: Return zero-length arrays, not nulls................................................................. |
101 |
Item 28: Write doc comments for all exposed API elements......................................... |
103 |
Chapter 7. General Programming.................................................................................. |
107 |
Item 29: Minimize the scope of local variables ............................................................. |
107 |
Item 30: Know and use the libraries............................................................................... |
109 |
Item 31: Avoid float and double if exact answers are required.................................. |
112 |
Item 32: Avoid strings where other types are more appropriate .................................... |
114 |
Item 33: Beware the performance of string concatenation ............................................ |
116 |
Item 34: Refer to objects by their interfaces .................................................................. |
117 |
Item 35: Prefer interfaces to reflection........................................................................... |
118 |
Item 36: Use native methods judiciously ....................................................................... |
121 |
Item 37: Optimize judiciously........................................................................................ |
122 |
Item 38: Adhere to generally accepted naming conventions ......................................... |
124 |
Chapter 8. Exceptions ...................................................................................................... |
127 |
Item 39:Use exceptions only for exceptional conditions ............................................... |
127 |
Item 40:Use checked exceptions for recoverable conditions and |
run-time exceptions |
for programming errors .................................................................................................. |
129 |
Item 41:Avoid unnecessary use of checked exceptions ................................................. |
130 |
Item 42:Favor the use of standard exceptions................................................................ |
132 |
Item 43: Throw exceptions appropriate to the abstraction............................................. |
133 |
Item 44:Document all exceptions thrown by each method ............................................ |
135 |
Item 45:Include failure-capture information in detail messages.................................... |
136 |
Item 46:Strive for </vetbfailure atomicity ..................................................................... |
138 |
Item 47:Don't ignore exceptions .................................................................................... |
139 |
Chapter 9. Threads .......................................................................................................... |
141 |
Item 48: Synchronize access to shared mutable data ..................................................... |
141 |
Item 49: Avoid excessive synchronization..................................................................... |
145 |
Item 50: Never invoke wait outside a loop ................................................................... |
149 |
Item 51: Don't depend on the thread scheduler .............................................................. |
151 |
Item 52: Document thread safety ................................................................................... |
154 |
Item 53: Avoid thread groups......................................................................................... |
156 |
Chapter 10. Serialization ................................................................................................. |
158 |
Item 54: Implement Serializable judiciously ............................................................ |
158 |
Item 55:Consider using a custom serialized form.......................................................... |
161 |
Item 56:Write readObject methods defensively .......................................................... |
166 |
Item 57: Provide a readResolve method when necessary ............................................ |
171 |
References ......................................................................................................................... |
174 |
Effective Java: Programming Language Guide
Foreword
If a colleague were to say to you, “Spouse of me this night today manufactures the unusual meal in a home. You will join?” three things would likely cross your mind: third, that you had been invited to dinner; second, that English was not your colleague's first language; and first, a good deal of puzzlement.
If you have ever studied a second language yourself and then tried to use it outside the classroom, you know that there are three things you must master: how the language is structured (grammar), how to name things you want to talk about (vocabulary), and the customary and effective ways to say everyday things (usage). Too often only the first two are covered in the classroom, and you find native speakers constantly suppressing their laughter as you try to make yourself understood.
It is much the same with a programming language. You need to understand the core language: is it algorithmic, functional, object-oriented? You need to know the vocabulary: what data structures, operations, and facilities are provided by the standard libraries? And you need to be familiar with the customary and effective ways to structure your code. Books about programming languages often cover only the first two, or discuss usage only spottily. Maybe that's because the first two are in some ways easier to write about. Grammar and vocabulary are properties of the language alone, but usage is characteristic of a community that uses it.
The Java programming language, for example, is object-oriented with single inheritance and supports an imperative (statement-oriented) coding style within each method. The libraries address graphic display support, networking, distributed computing, and security. But how is the language best put to use in practice?
There is another point. Programs, unlike spoken sentences and unlike most books and magazines, are likely to be changed over time. It's typically not enough to produce code that operates effectively and is readily understood by other persons; one must also organize the code so that it is easy to modify. There may be ten ways to write code for some task T. Of those ten ways, seven will be awkward, inefficient, or puzzling. Of the other three, which is most likely to be similar to the code needed for the task T' in next year's software release?
There are numerous books from which you can learn the grammar of the Java Programming Language, including The Java Programming Language by Arnold, Gosling, and Holmes [Arnold00] or The Java Language Specification by Gosling, Joy, yours truly, and Bracha [JLS]. Likewise, there are dozens of books on the libraries and APIs associated with the Java programming language.
This book addresses your third need: customary and effective usage. Joshua Bloch has spent years extending, implementing, and using the Java programming language at Sun Microsystems; he has also read a lot of other people's code, including mine. Here he offers good advice, systematically organized, on how to structure your code so that it works well, so that other people can understand it, so that future modifications and improvements are less
1
Effective Java: Programming Language Guide
likely to cause headaches—perhaps, even, so that your programs will be pleasant, elegant, and graceful.
Guy L. Steele Jr.
Burlington, Massachusetts
April 2001
2