
- •Preface
- •Who Should Read This Book
- •Organization and Presentation
- •Contacting the Authors
- •Acknowledgments
- •Contents
- •Introduction
- •Why Microsoft .NET?
- •The Microsoft .NET Architecture
- •Internet Standards
- •The Evolution of ASP
- •The Benefits of ASP.NET
- •What Is .NET?
- •.NET Experiences
- •.NET Clients
- •.NET Services
- •.NET Servers
- •Review
- •Quiz Yourself
- •Installation Requirements
- •Installing ASP.NET and ADO.NET
- •Installing the .NET Framework SDK
- •Testing Your Installation
- •Support for .NET
- •Review
- •Quiz Yourself
- •Designing a Database
- •Normalization of Data
- •Security Considerations
- •Review
- •Quiz Yourself
- •Creating a Database
- •Creating SQL Server Tables
- •Creating a View
- •Creating a Stored Procedure
- •Creating a Trigger
- •Review
- •Quiz Yourself
- •INSERT Statements
- •DELETE Statements
- •UPDATE Statements
- •SELECT Statements
- •Review
- •Quiz Yourself
- •The XML Design Specs
- •The Structure of XML Documents
- •XML Syntax
- •XML and the .NET Framework
- •Review
- •Quiz Yourself
- •ASP.NET Events
- •Page Directives
- •Namespaces
- •Choosing a Language
- •Review
- •Quiz Yourself
- •Introducing HTML Controls
- •Using HTML controls
- •How HTML controls work
- •Intrinsic HTML controls
- •HTML Control Events
- •The Page_OnLoad event
- •Custom event handlers
- •Review
- •Quiz Yourself
- •Intrinsic Controls
- •Using intrinsic controls
- •Handling intrinsic Web control events
- •List Controls
- •Rich Controls
- •Review
- •Quiz Yourself
- •Creating a User Control
- •Adding User Control Properties
- •Writing Custom Control Methods
- •Implementing User Control Events
- •Review
- •Quiz Yourself
- •Common Aspects of Validation Controls
- •Display property
- •Type Property
- •Operator Property
- •Using Validation Controls
- •RequiredFieldValidator
- •RegularExpressionValidator
- •CompareValidator
- •RangeValidator
- •CustomValidator
- •ValidationSummaryx
- •Review
- •Quiz Yourself
- •Maintaining State Out of Process for Scalability
- •No More Cookies but Plenty of Milk!
- •Out of Process State Management
- •Review
- •Quiz Yourself
- •Introducing the Key Security Mechanisms
- •Web.config and Security
- •Special identities
- •Using request types to limit access
- •New Tricks for Forms-based Authentication
- •Using the Passport Authentication Provider
- •Review
- •Quiz Yourself
- •ASP.NET Updates to the ASP Response Model
- •Caching with ASP.NET
- •Page Output Caching
- •Absolute cache expiration
- •Sliding cache expiration
- •Fragment Caching
- •Page Data Caching
- •Expiration
- •File and Key Dependency and Scavenging
- •Review
- •Quiz Yourself
- •A Brief History of Microsoft Data Access
- •Differences between ADO and ADO.NET
- •Transmission formats
- •Connected versus disconnected datasets
- •COM marshaling versus text-based data transmission
- •Variant versus strongly typed data
- •Data schema
- •ADO.NET Managed Provider Versus SQL Managed Provider
- •Review
- •Quiz Yourself
- •Review
- •Quiz Yourself
- •Creating a Connection
- •Opening a Connection
- •Using Transactions
- •Review
- •Quiz Yourself
- •Building a Command
- •Connection property
- •CommandText property
- •CommandType property
- •CommandTimeout property
- •Appending parameters
- •Executing a Command
- •ExecuteNonQuery method
- •Prepare method
- •ExecuteReader method
- •Review
- •Quiz Yourself
- •Introducing DataReaders
- •Using DataReader Properties
- •Item property
- •FieldCount property
- •IsClosed property
- •RecordsAffected property
- •Using DataReader Methods
- •Read method
- •GetValue method
- •Get[Data Type] methods
- •GetOrdinal method
- •GetName method
- •Close method
- •Review
- •Quiz Yourself
- •Constructing a DataAdapter Object
- •SelectCommand property
- •UpdateCommand, DeleteCommand, and InsertCommand properties
- •Fill method
- •Update method
- •Dispose method
- •Using DataSet Objects
- •DataSetName property
- •CaseSensitive property
- •Review
- •Quiz Yourself
- •Constructing a DataSet
- •Tables property
- •TablesCollection Object
- •Count property
- •Item property
- •Contains method
- •CanRemove method
- •Remove method
- •Add method
- •DataTable Objects
- •CaseSensitive property
- •ChildRelations property
- •Columns property
- •Constraints property
- •DataSet property
- •DefaultView property
- •ParentRelations property
- •PrimaryKey property
- •Rows property
- •Dispose method
- •NewRow method
- •Review
- •Quiz Yourself
- •What Is Data Binding?
- •Binding to Arrays and Extended Object Types
- •Binding to Database Data
- •Binding to XML
- •TreeView Control
- •Implement the TreeView server control
- •Review
- •Quiz Yourself
- •DataGrid Control Basics
- •Binding a set of data to a DataGrid control
- •Formatting the output of a DataGrid control
- •Master/Detail Relationships with the DataGrid Control
- •Populating the Master control
- •Filtering the detail listing
- •Review
- •QUIZ YOURSELF
- •Updating Your Data
- •Handling the OnEditCommand Event
- •Handling the OnCancelCommand Event
- •Handling the OnUpdateCommand Event
- •Checking that the user input has been validated
- •Executing the update process
- •Deleting Data with the OnDeleteCommand Event
- •Sorting Columns with the DataGrid Control
- •Review
- •Quiz Yourself
- •What Is Data Shaping?
- •Why Shape Your Data?
- •DataSet Object
- •Shaping Data with the Relations Method
- •Review
- •Quiz Yourself
- •OLEDBError Object Description
- •OLEDBError Object Properties
- •OLEDBError Object Methods
- •OLEDBException Properties
- •Writing Errors to the Event Log
- •Review
- •Quiz Yourself
- •Introducing SOAP
- •Accessing Remote Data with SOAP
- •SOAP Discovery (DISCO)
- •Web Service Description Language (WSDL)
- •Using SOAP with ASP.NET
- •Review
- •Quiz Yourself
- •Developing a Web Service
- •Consuming a Web Service
- •Review
- •Quiz Yourself
- •ASP and ASP.NET Compatibility
- •Scripting language limitations
- •Rendering HTML page elements
- •Using script blocks
- •Syntax differences and language modifications
- •Running ASP Pages under Microsoft.NET
- •Using VB6 Components with ASP.NET
- •Review
- •Quiz Yourself
- •Preparing a Migration Path
- •ADO and ADO.NET Compatibility
- •Running ADO under ASP.NET
- •Early Binding ADO COM Objects in ASP.NET
- •Review
- •Quiz Yourself
- •Answers to Part Reviews
- •Friday Evening Review Answers
- •Saturday Morning Review Answers
- •Saturday Afternoon Review Answers
- •Saturday Evening Review Answers
- •Sunday Morning Review Answers
- •Sunday Afternoon Review Answers
- •What’s on the CD-ROM
- •System Requirements
- •Using the CD with Windows
- •What’s on the CD
- •The Software Directory
- •Troubleshooting
- •ADO.NET Class Descriptions
- •Coding Differences in ASP and ASP.NET
- •Retrieving a Table from a Database
- •Displaying a Table from a Database
- •Variable Declarations
- •Statements
- •Comments
- •Indexed Property Access
- •Using Arrays
- •Initializing Variables
- •If Statements
- •Case Statements
- •For Loops
- •While Loops
- •String Concatenation
- •Error Handling
- •Conversion of Variable Types
- •Index
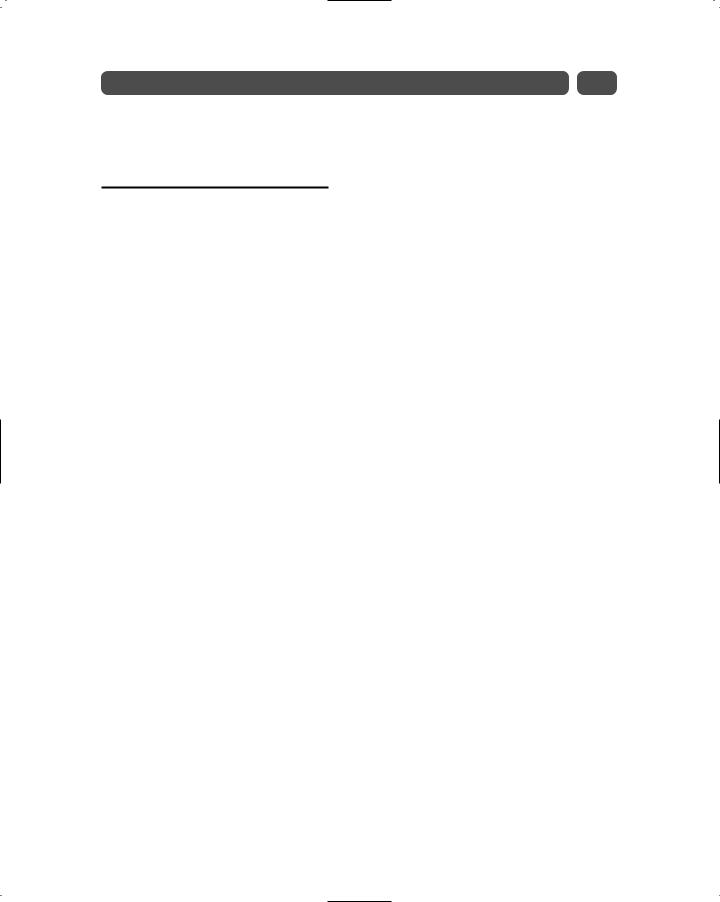
Session 6—XML: A Primer |
59 |
quotes, which are required. You can create attributes to help describe your elements. You could have also used another child element called <TYPE> rather than using an attribute. Either way is fine. It’s really a matter of preference.
XML and the .NET Framework
The important thing to remember about XML is that it is simply a way of describing data so that any application that knows the structure of the document can use it. Why do you need to know about XML when dealing with ASP.NET? Well, much of the .NET Framework revolves around the concept of universal data and application access. In fact, pretty much everything in the .NET world revolves around the premise of universal access.
Probably two of the most evident examples of XML usage in ASP.NET are the config.web and global.asax files. Without going into too much detail about these files, they store application and configuration data. Here is an example of a config.web file:
<configuration>
<sessionstate timeout=”120”/> <assemblies>
<add assembly=”mscorlib”/> <add assembly=”System.Web”/> <add assembly=”System.Data”/>
</assemblies>
<appsettings>
<add key=”DSN” value=”Server=127.0.0.1;Database=hootie; UID=sa”/> </appsettings>
</configuration>
From your knowledge of XML, you can see that the document element for the config.web file is <configuration>. The document element contains several child elements including
<sessionstate>, <assemblies>, and <appsettings>. The <sessionstate> element has an attribute named timeout that has a value of 120. The <sessionstate> element is empty, which means that it does not contain any character data. When an element is empty is must be closed with the /> or </ELEMENT> syntax. The same could have been accomplished by writing:
<sessionstate timeout=”120”></sessionstate>
XML is also used in ASP.NET with Web Services. A Web Service’s results are always returned in XML format. Suppose you created a Web Service named Math that has a method named Add that sums two numbers. If you invoke the Web Service and call the Add function by passing 2 and 6 as the parameters you might get the following result:
<?xml version=”1.0” ?>
<int xmlns=”http://tempuri.org/”>8</int>
You’ll notice that the result is returned in XML format. The XML document contains a prolog and a document element. That’s it.

60 |
Saturday Morning |
This brief introduction of XML and how it is used by the .NET Framework will help you understand some of the concepts introduced later in the book. For further information about XML, we recommend visiting the World Wide Web Consortium’s (W3C) XML Web site at www.w3c.com.
.NET Web Services, like the global.asax and config.web files, are based heavily on XML. All Web Services responses are serialized as XML. We’ll talk more about Web Services in Session 28.
REVIEW
“XML is the future . . .” If you’ve heard that once, you’ve heard it a million times. You may be sick of hearing it, but you accept it because it’s probably true. Simply put, the purpose of XML is to describe data so it can easily be exchanged. The nice thing about XML is that it’s easy to learn as its syntax is very similar to its cousin HTML.
QUIZ YOURSELF
1.What is the purpose of XML? (See session introduction.)
2.How many root elements are in an XML document? (See “The XML Design Specs.”)
3.What are the three logical parts of an XML document? (See “The Structure of XML Documents.”)
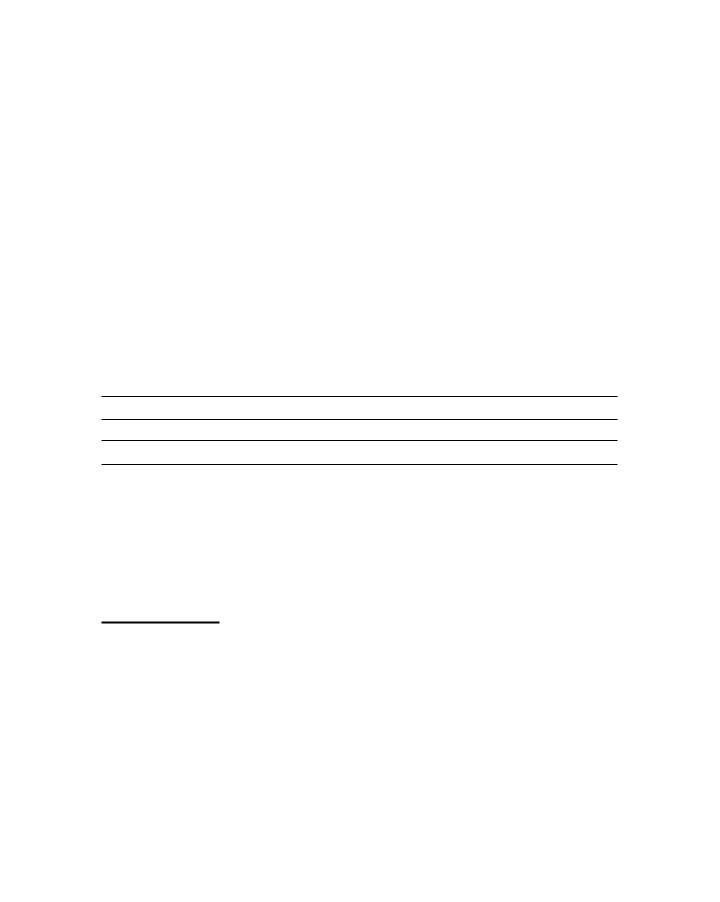
S E S S I O N
7
Developing ASP.NET Pages
Session Checklist
Handling ASP.NET events
Using page directives and namespaces
Choosing a language
In this session, we are going to walk you through writing an ASP.NET page. Writing and ASP.NET page is a little more complicated than writing an ASP page, but once you get the hang of it, you’ll end up writing a lot less code. ASP.NET pages are event-oriented
rather than procedural. This means that instead of starting at the top of a page and writing code that is executed as the page is interpreted, you write event-handling code. An event can be pretty much any type of action, for example, a user submitting a form, a page loading, or clicking a button, and so on.
ASP.NET Events
When an ASP.NET page is loaded, a structured series of events is fired in a set order. You write code that responds to these events rather than interspersing it with HTML as the general practice with ASP. Figure 7-1 shows the ASP.NET event order.
As you can see, the first event to be fired when a page is loaded is the Page_Load event and the last to be fired is the Page_Unload event. The Page_Load event is fired every time a page is loaded. In between the Page_Load and the Page_Unload events, control events are fired. A control event is an event that is wired to a control of some sort. ASP.NET provides many types of controls including HTML controls, Web controls, user controls, validation controls, and so on. Don’t concern yourself with the differences between controls right now (they’re discussed in greater detail in Session 8, “Using HTML Controls”, and Session 9, “Using Web Controls”.), just be aware that they can all respond to events, like being clicked.
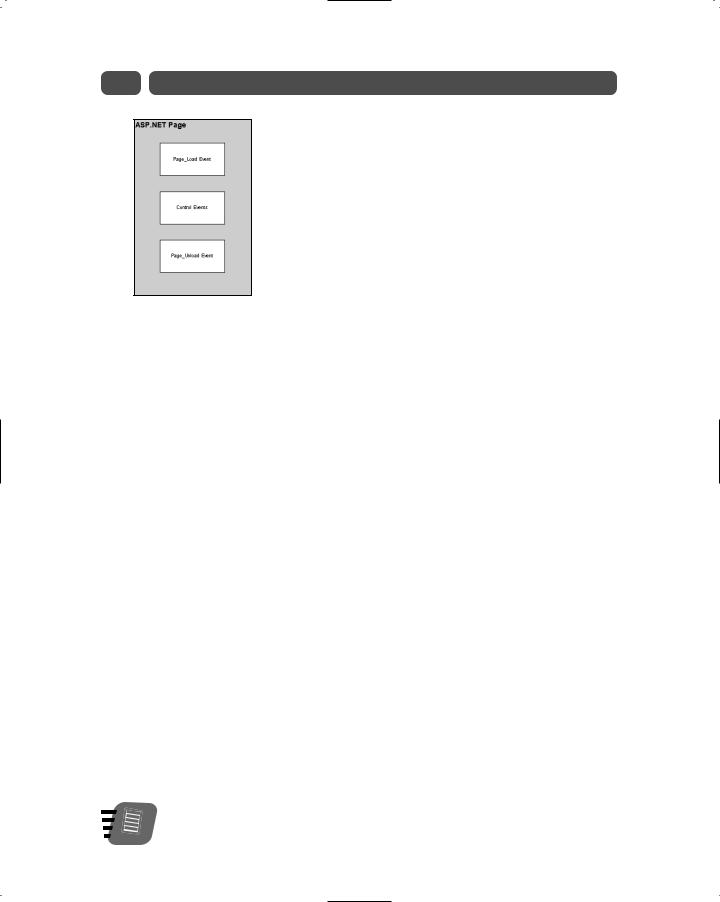
62 |
Saturday Morning |
Figure 7-1 ASP.NET page events
In order to write code for these events, you need to include them in a code declaration block. In ASP.NET, a code declaration block looks like this:
<SCRIPT [LANGUAGE=”codeLangauge”] RUNAT=”SERVER” [SRC=”externalfilename”]> ‘ Event Handling Code
</SCRIPT>
The LANGUAGE attribute in the <SCRIPT> element specifies the language used in the code block. The value can be any .NET language like VB or C#. The RUNAT=”SERVER” attribute/value pair specifies that the script block should be executed on the server-side rather than the client-side. The SRC attribute enables you to specify an external file where the code is located.
So, based on what we know at this point, an ASP.NET page should look like this:
<SCRIPT LANGUAGE=”VB” RUNAT=”server”>
Sub Page_Load(Source As Object, E As EventArgs)
‘Page_Load Code End Sub
Sub Control_Click(Source As Object, E As EventArgs) ‘Control_Click Code
End Sub
Sub Page_Unload(Source As Object, E As EventArgs)
‘Page_Unload
End Sub </SCRIPT> <html> <head>
<title>ASP.NET Page</title> </head>
<body>
</body>
</html>
Note
ASP.NET files have an .aspx extension. The .aspx extension simply tells IIS that an ASP.NET page is being requested and should be handled accordingly. All code in this session should be written in files with an .aspx extension.
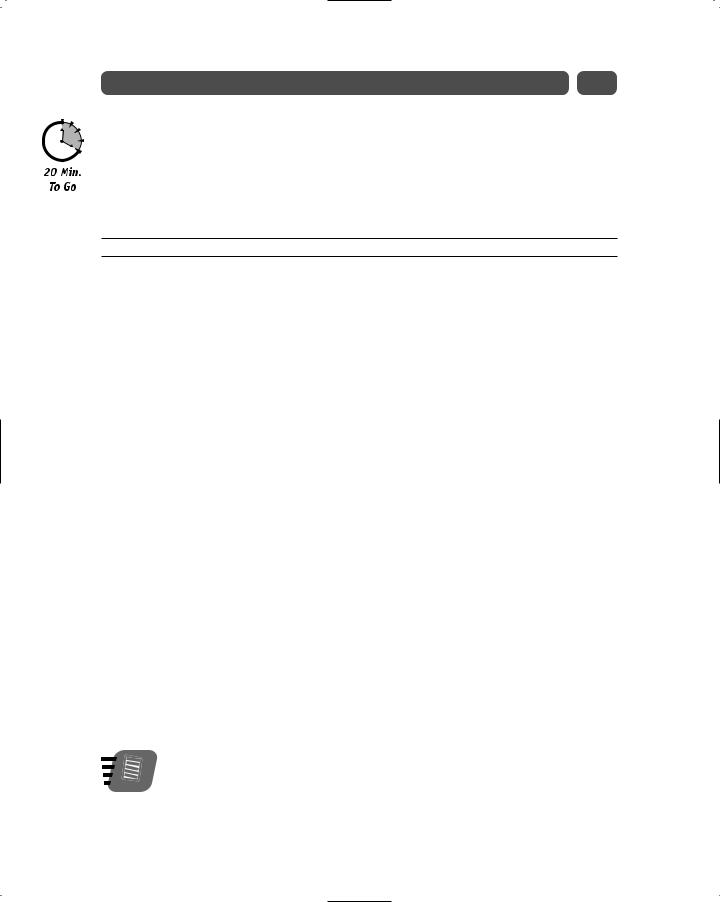
Session 7—Developing ASP.NET Pages |
63 |
In ASP.NET, a page is an object, which means it has properties, events and methods. The Page object has one very important property: isPostBack. The isPostBack property returns a Boolean value indicating whether the page is being loaded in response to a client post back. This is important because in many cases you will be initializing controls when a page is loaded. Since ASP.NET manages control state between requests, you probably don’t want to initialize a control if the page is responding to a post back. Right? Listing 7-1 shows an example of using the isPostBack property:
Listing 7-1 An isPostBack property example
<SCRIPT LANGUAGE=”VB” RUNAT=”server”>
Sub Page_Load(Source As Object, E As EventArgs) ‘ Page_Load Code
lblTest.Text = Page.isPostBack End Sub
Sub Control_Click(Source As Object, E As EventArgs) ‘Control_Click Code
End Sub
Sub Page_Unload(Source As Object, E As EventArgs) ‘ Page_Unload
End Sub </SCRIPT> <html> <head>
<title>ASP.NET Page</title> </head>
<body>
<form ID=”frmTest” RUNAT=”SERVER”> <asp:Label ID=”lblTest” RUNAT=”SERVER”/> </BR>
<asp:Button ID=”btnSubmit” TEXT=”Submit” RUNAT=”SERVER”/> </form>
</body>
</html>
As shown in Listing 7-1, we added a snippet of code to the Page_Load event that checks if the page is being posted back using the isPostBack property. Something that may look a little foreign are the server-side control declarations a little further down the page. We declared three server controls including a Form HTML control, a Label Web control and a
Button Web control. You’ll notice that each of these declarations contains a “RUNAT=SERVER” attribute/value pair. This simply means that the control is rendered on the server and its events are handled on the server side rather than the client side. We’ll talk more about controls in later sessions.
The Page class contains many useful properties and methods. Refer to your
.NET documentation for a complete treatment of the Page class.
Note
OK, try running the previous example. When the page is first loaded the word “False” appears above the Submit button. If you click the Submit button, the form is posted and