
Beginning ActionScript 2.0 2006
.pdf
Chapter 5
function drawBox(targetMovieClip:MovieClip, boxWidth:Number, ; boxHeight:Number, boxColor:Number, lineColor:Number):Void
{
targetMovieClip.beginFill(boxColor); targetMovieClip.lineStyle(1, lineColor); targetMovieClip.moveTo(0, 0); targetMovieClip.lineTo(boxWidth, 0); targetMovieClip.lineTo(boxWidth, boxHeight); targetMovieClip.lineTo(0, boxHeight); targetMovieClip.lineTo(0, 0);
}
createButton(“playButton”, “Play”, 10, 10, invokePlay); createButton(“stopButton”, “Stop”, 70, 10, invokeStop); setupMovie();
20.Save the file, return to the Flash project file, and select Control Test Movie. The project should work identically as before.
How It Works
The issues in the code at the beginning of this example include entanglement of drawing routines with button creation code, entanglement of animation control code with the button handlers, duplication of code, hard-coded movie clip references, and code that is inflexible overall. You fixed some of these problems through refactoring.
The first issue is that the button handlers contain code that directly starts and stops the animation. Even though each contains only one line of code, it should still be externalized into its own function (without this, later refactoring steps won’t work):
function invokePlay():Void
{
trace(“Pressed play”); presentationArea.play();
}
function invokeStop():Void
{
trace(“Pressed stop”); presentationArea.stop();
}
playButton.onRelease = function()
{
invokePlay();
}
stopButton.onRelease = function()
{
invokeStop();
}
138

Getting Started with Coding
Next, the code that sets up and loads an external animation should be grouped in its own function:
function setupMovie():Void
{
// Create presentation area, and load a movie clip into it _level0.createEmptyMovieClip(“presentationArea”, 3); presentationArea._x = 10;
presentationArea._y = 40; presentationArea.loadMovie(“http://www.nathanderksen.com/book/;
tryItOut_refactorAnimation.swf”);
}
There is very clearly duplicated code, which calls for creating a function that can convert the duplicated code into a single command to be called whenever needed:
function createButton(buttonName:String, buttonLabel:String, ; xPos:Number, yPos:Number, callback:Function):Void
{
var buttonHandle:MovieClip;
_level0.createEmptyMovieClip(buttonName, _level0.getNextHighestDepth()); buttonHandle = _level0[buttonName];
buttonHandle.onRelease = function()
{
callback();
}
buttonHandle._x = xPos; buttonHandle._y = yPos; buttonHandle.beginFill(0xAAAAFF); buttonHandle.lineStyle(1, 0x333333); buttonHandle.moveTo(0, 0); buttonHandle.lineTo(50, 0); buttonHandle.lineTo(50, 20); buttonHandle.lineTo(0, 20); buttonHandle.lineTo(0, 0);
buttonHandle.createTextField(“labelField”, 1, 4, 4, 50, 15); buttonHandle.labelField.text = buttonLabel;
}
Next, drawing routines are entangled within the createButton() function. These can easily be removed and placed in their own function that can potentially be reused elsewhere:
function createButton(buttonName:String, buttonLabel:String, ; xPos:Number, yPos:Number, callback:Function):Void
{
var buttonHandle:MovieClip;
_level0.createEmptyMovieClip(buttonName, _level0.getNextHighestDepth()); buttonHandle = _level0[buttonName];
buttonHandle.onRelease = function()
{
callback();
}
buttonHandle._x = xPos; buttonHandle._y = yPos;
139
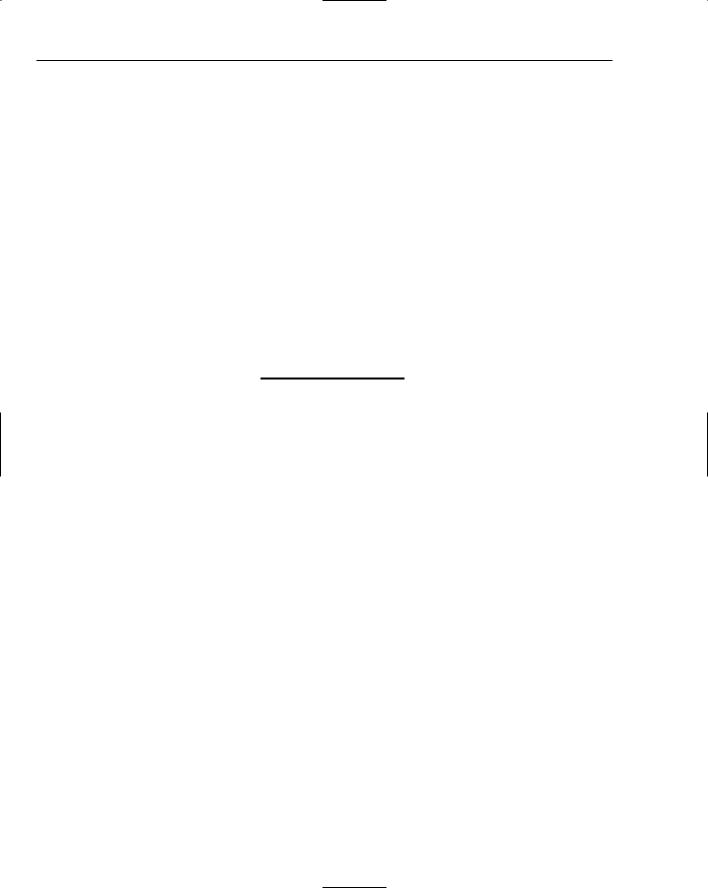
Chapter 5
drawBox(buttonHandle, 50, 20, 0xAAAAFF, 0x333333); buttonHandle.createTextField(“labelField”, 1, 4, 4, 50, 15); buttonHandle.labelField.text = buttonLabel;
}
function drawBox(targetMovieClip:MovieClip, boxWidth:Number, ; boxHeight:Number, boxColor:Number, lineColor:Number):Void
{
targetMovieClip.beginFill(boxColor); targetMovieClip.lineStyle(1, lineColor); targetMovieClip.moveTo(0, 0); targetMovieClip.lineTo(boxWidth, 0); targetMovieClip.lineTo(boxWidth, boxHeight); targetMovieClip.lineTo(0, boxHeight); targetMovieClip.lineTo(0, 0);
}
At this point, significant improvements have been made to the quality of this code. A couple issues still exist, such as a hard-coded level reference within createButton(), and although the drawing routine can now support variable-sized backgrounds, the button cannot. An exercise at the end of this chapter gives you a chance to fix these issues.
Summar y
This chapter explored the principles of OOP (object-oriented programming) and examined what’s meant by best practices in coding. Here are some of the points about OOP that you’ll want to remember:
OOP involves packaging data and the code that acts on that data into a single entity.
The OOP term for a function is a method, and the term for a variable is a property.
A class is a template for an object. Objects are copies derived from a class through the process of instantiation.
Class inheritance allows a sub-class to acquire methods and properties from a parent class.
Goals of OOP include keeping code focused, promoting re-use, reducing impact of change, and keeping code understandable.
You also learned a number of things about coding best practices. Some of the key points include the following:
Variable names should be readable, reflect the kind of data contained in the variable, and not be a reserved word.
Variable types should be declared along with the variable.
Strong typing helps to make code clearer and easier to debug.
Comments should supplement code, but clean, easy-to-read variables and syntax are essential to understanding the code.
A variable’s scope refers to its visibility to other parts of the project. The three types of scope are local, timeline, and global.
140

Getting Started with Coding
Variables should be scoped as narrowly as possible. Avoid global and timeline variables whenever possible.
Do not directly modify global variables. Create accessor functions that are responsible for modifying global variables.
The best way to store global data is through the use of a custom class.
Variables can be set and retrieved in other movie clips using dot syntax.
All function input parameters and return values should be strongly typed.
Functions should be small, well-defined units of code. Function code that becomes too large, too complicated, too repetitive, or too entangled should be refactored.
In the next chapter, you continue to learn about project setup, including some best practices for organizing the library, working with screens, and placement of code. Before proceeding to the next chapter, work through the following exercises to help solidify the material that you just learned.
Exercises
1.Which of the following are valid, correct statements? For the ones that are not valid or correct, explain why.
a.var myString:String = new String(“the quick brown fox”);
b.var myNextString:String = “the quick brown fox”;
c.var myDate:Date = new Date(“2005/05/12”);
d.var myNextDate:Date = Date(2005, 05, 12);
e.var myObject:Object = {property1:”foo”, property2:”bar”};
f.var myNextObject:Object = new Object();
g.var myArray = new Array();
h.var myNumber:Number = “20”;
2.Without running the code, determine what the output of each trace statement in the following code snippet would be.
var myString:String = “baz”;
var mySecondString:String = “foo”; _global.mySecondString = “bar”;
function testFunction():Void |
|
{ |
|
var myString:String = “qux”; |
|
trace(“testFunction->myString: “ + myString); |
//a. |
trace(“testFunction->mySecondString: “ + mySecondString); |
//b. |
} |
|
testFunction(); |
|
trace(“myString: “ + myString); |
//c. |
trace(“mySecondString: “ + mySecondString); |
//d. |
141

Chapter 5
delete mySecondString; |
|
trace(“mySecondString: “ + mySecondString); |
//e. |
3.Which of the following function declarations are valid?
a.
function myFunction1():Void
{
// Function contents
}
b.
var myFunction2:Function = function():Void
{
// Function contents
}
c.
var myFunction3:Function = new function():String
{
// Function contents
}
d.
function myFunction4(inputString:String):String
{
// Function contents return true;
}
e.
var myFunction5():Void
{
// Function contents
}
f.
function myFunction6(inputString, inputNumber)
{
// Function contents
}
4.Modify the final code for the “Refactor Problematic Code” Try It Out to allow the following:
Modify the createButton() function so that a width and height parameter can be sent in to create a variable-sized button. The function should automatically assign dimensions if the input width and height values are 0, null, or undefined.
Modify any statements within createButton() that refer directly to _level0 so that they instead refer to a movie clip that is passed in through an additional function argument.
142

6
Setting Up Flash Projects
ActionScript programming involves more than just coding. Working on a Macromedia Flash project includes organizing timelines, structuring symbols in the library, structuring elements on state, working with media, and creating a structured movie clip environment that can be controlled with code. As you’ve seen, a number of guidelines help you to make the most of your project.
In this chapter, you learn to set up your library, work with bitmap images, and use movie clips and frames.
Setting Up the Librar y
In even a medium-sized project, the number of items that accumulate in the library can become quite large, so it’s a good idea to organize library folders before placing anything in the project. Figure 6-1 shows a suggested system for organizing the library.
At the base, two folders are created, one (global) for content that is not specific to any particular screen, and one (screens) for content that belongs to a specific screen. The screens folder shows one subfolder for each screen in the project: home, photography, and tutorials. Within each of the screen folders and the global folder exist five subfolders:
bitmaps — Stores bitmap images that have been imported into the project.
buttons — Holds complete button clips.
graphics — Contains artwork that is just illustrative in nature.
movie clips — Stores clips containing animation or holding other clips or media.
tweens — Holds clips that are automatically created whenever tweens are created on the timeline.

Chapter 6
Figure 6-1
The _buttons folder within the bitmaps folder is for bitmaps to be used in buttons. It is prepended with the underscore character to force the folder to the top of the sort order. You can use this technique any time you want a folder to come before any other folders or movie clips on the same folder level.
Try out this system, and modify it as you learn what works best for you.
Working with Bitmap Images
Different situations call for different treatment of bitmap images. Sometimes you’ll find it preferable to load them dynamically with ActionScript, whereas at other times it’s preferable to have them in the library. Following are several reasons why it might be better to embed images into the library rather than to load them on-the-fly:
Images are easier to implement, and no separate preloading is needed.
Macromedia Flash player version 8 supports loading of JPG, GIF, and PNG images, although versions 6 and 7 support only dynamic loading of JPG images. To take advantage of images that
144

Setting Up Flash Projects
make use of transparency effects and to target player versions earlier than 8, the images need to be in the library.
Images that are embedded in the library can be used more easily in tweened animations.
The following sections examine a few things to keep in mind when handling bitmap images.
Keep Images Organized on the Desktop
Maintain one folder within the project directory that is dedicated to holding images. This keeps the project folder neat and the images easily accessible for making changes.
Use a standard naming convention for each image, preferably so that when they are sorted by name, they are grouped by function or by screen. Also, keep a directory structure within the library to organize the images.
Figure 6-2 shows a possible organizational system where an images folder has been placed next to the Flash project file. Within the images folder, the global folder holds images that are global in nature, such as for common navigation. The screens folder contains one subfolder per screen for all images specific to that screen.
Figure 6-2
Keep Images Organized in the Library
The library is arranged in a similar manner to the desktop images folder. A global folder holds media that is common to the project, and individual screen folders hold media specific to each screen.
Figure 6-3 shows the library panel for portfolio.fla.
Embed Images into Movie Clips
It is generally not a good idea to place bitmap images directly on the timeline. If the image is placed in several places within the project, and it later becomes necessary to swap the image with another, it will be necessary to find and replace each image individually.
Effects, animation, and scripting cannot be done directly on a bitmap image; you can deal with both of those by always nesting bitmap images within a movie clip. Then, rather than dragging the bitmap to the stage whenever it is needed, drag the movie clip containing the bitmap to the stage. Once done, effects can be applied to the movie clip, the movie clip can be easily tweened, and updating a bitmap image involves just updating the contents of the one movie clip.
In the next Try It Out you work with symbols in the library, and begin putting together a multi-screen project.
145

Chapter 6
Figure 6-3
Try It Out |
Working with Bitmap Images |
Follow these steps to get acquainted with the process of working with bitmap images:
1.Open portfolio.fla from the book’s source files at <source file directory>/Chapter 6/ portfolio v1/.
2.Select File Import Import to Library and choose headerImage.png from the folder portfolio v1/images/global.
3.Select File Import Import to Library and choose featuredPhoto.png from the folder portfolio v1/images/screens/home.
4.Open the library (Window Library) and drag headerImage.png from the base of the library into the folder global/bitmaps.
146

Setting Up Flash Projects
5.Drag featuredPhoto.png from the base of the library into the folder screens/home/bitmaps.
6.Double-click anywhere on the gray part of the header bar on the top of the stage. You should be put into editing mode for the headerBackground movie clip.
7.Click the empty keyframe for the layer named Background Image. From the library, drag headerImage.png onto the stage so that it is aligned top-right within the movie clip.
8.Click the image on the stage to select it. Take a look at the options currently available in the properties panel (Window Properties).
9.With the image still selected, right-click and select Convert to Symbol, or choose Modify Convert to Symbol. Name the symbol headerImage, and ensure that the Movie Clip radio button has been selected. Click OK.
10.Take another glance at the properties panel. It now shows the text field on the top left for entering an instance ID, and it also provides a drop-down menu for color selection.
11.Drag the headerImage movie clip at the root of the library into the global/graphics library folder.
12.Return to the main stage by clicking the Scene 1 button just above the timeline. Open the Home layer set, and select the keyframe in the Featured Photo layer.
13.Drag featuredPhoto.png from the library’s screens/home/bitmaps folder onto the stage, just below the featured photo heading.
14.Click the image to select it. Right-click and select Convert to Symbol or select Modify Convert to Symbol. Name the symbol featuredPhoto and ensure that the Movie Clip radio button has been selected. Click OK.
15.Drag the featuredPhoto movie clip at the root of the library into the screens/home/graphics library folder.
16.Click the featuredPhoto movie clip on the stage to select it again. Right-click and select Convert to Symbol, or choose Modify Convert to Symbol. Name the symbol featuredPhotoButton and ensure that the Button radio button has been selected. Click OK.
17.With the symbol still selected on the stage, go to the properties panel and type featuredPhoto_btn in the top-left text field.
18.Double-click the thumbnail image, which is now a button, to go into editing mode for the button clip.
19.Click the Down keyframe in the timeline. Select Insert Timeline Keyframe.
20.Click the thumbnail image on the stage. In the properties panel, select Brightness from the Color drop-down menu and choose a negative value, such as –10%.
21.Return to the main stage by clicking the Scene 1 button just above the timeline. Drag the featuredPhotoButton movie clip at the root of the library into the screens/home/buttons library folder. Your stage and library should now look something like Figure 6-3.
22.Save the file and select Control Test Movie. Clicking the featured photo image should cause the button color effect to show when the mouse button is down.
147