
Beginning ActionScript 2.0 2006
.pdf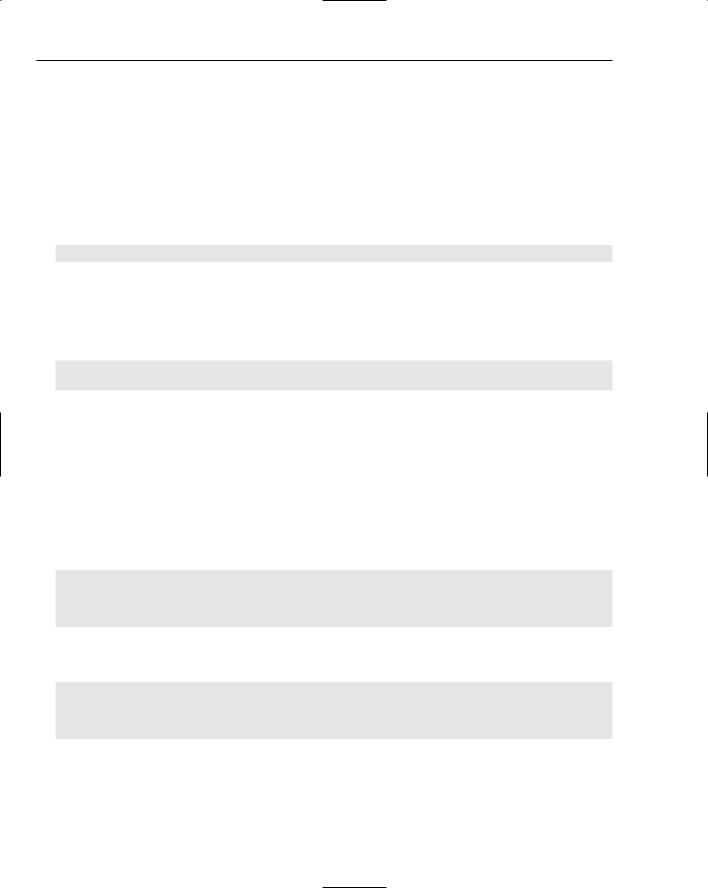
Chapter 2
The data type (String) comes next, separated from the variable name by a colon (:). The data type declaration tells the compiler the kind of data — a chunk of text, a numerical value, or a date — can be stored in the variable. The data type is optional; if no data type is to be declared, there should be no colon after the variable name. Although declaring a data type is optional, it’s a good practice to follow, as you see shortly. Finally, a semicolon indicates the end of the statement.
Assigning Data to a Variable
For a variable to be truly useful, it needs to be assigned some data. One way to do this is by assigning an initial value during the variable declaration:
var myTitle:String = “Programmer”;
The equals sign is an assignment operator that says to assign the data that follows — in this example, the literal string Programmer — to the referenced variable. The literal string is surrounded by quotation marks to distinguish it as text data instead of code to execute.
Data can also be assigned to a variable in a separate statement, like this:
var myTitle:String;
myTitle = “Programmer”;
The first statement declares the variable myTitle, but leaves it empty. The second statement assigns the string literal Programmer to the variable.
Viewing the Contents of a Variable
To find out what data a variable contains, the contents need to be read and then output to the screen. A built-in function called trace() enables you to see the contents of a variable. When you place the variable name between the function’s round brackets, trace() reads the contents of the variable and displays it in the Output panel. Here’s an example that declares a variable, assigns a String value to it, and then runs trace on the variable. The variable’s content, the string Programmer, is output to the screen.
var myTitle:String; myTitle = “Programmer”;
trace(myTitle);
// Outputs: Programmer
You see the trace() statement in the examples throughout this book. To better read the output, use the string concatenation operator (+) to add a description to the output, like this:
var myTitle:String; myTitle = “Programmer”;
trace(“My title is: “ + myTitle); // Outputs: My title is: Programmer
If the variable passed into the trace() function is not of type String, Flash converts the data to a string the best way it knows how.
28

Getting Started with ActionScript 2.0
Two keywords represent the absence of data in a variable: null and undefined. Retrieving the contents of a variable that has been declared but has not yet had data assigned to it results in the null value being returned. Attempting to test the contents of a variable that has not been declared results in the undefined value being returned. Here are examples:
var myEmptyVar:String; trace(myEmptyVar);
// Outputs: null
trace(myUndefinedVar); // Outputs: undefined
Passing Data from Variable to Variable
Data can also be passed from one variable to another in the same way that a literal string value is assigned to a variable:
var myTitle:String; var yourTitle:String;
myTitle = “Programmer”;
yourTitle = myTitle;
trace(yourTitle);
// Outputs: Programmer
The data contained by the myTitle variable is assigned to the yourTitle variable. Both variables end up containing the string Programmer.
Naming Variables
Variable names can be named pretty much anything you want, with the restrictions that they can only include letters, numbers, and the underscore characters, and cannot start with a number. In addition, they cannot consist of any of the words that are reserved by the ActionScript compiler. The easiest way to tell if there is a conflict is if your variable name turns blue in the editor. The compiler warns you if you use any core language keywords, such as default, if, while, new, Key, or Date. Chapter 5 includes a list of keywords in its discussion of best practices. You can find a complete list at http://livedocs
.macromedia.com/flash/8/main/wwhelp/wwhimpl/common/html/wwhelp.htm?context= LiveDocs_Parts&file=00001236.html.
Variables are case sensitive, so myVariable and myvariable are two different variables. Having variable names differ only by case is, as you can imagine, not a good practice and can cause quite a bit of confusion down the road.
One common variable naming convention is camelBack notation, which consists of multiple words strung together with a capitalized first letter for each, beginning with the second word: myLong VariableName. Whatever naming convention you use, make sure the names make it quickly obvious what each variable is used for and what kind of data it stores.
29

Chapter 2
Here are a few bad examples of variable naming:
var fp:Boolean; |
// Not explicit |
var users:Number; |
// Does not describe the nature of the data |
var scores:Array; |
// Give no clue about the kind of data the variable contains |
var sound:Sound; |
// Uses only case to differentiate from a reserved keyword |
|
|
Better names for these variables would be
var isFirstPass:Boolean;
var numRegisteredUsers:Number;
var highScoreArray:Array;
var backgroundSoundLoop:Sound;
It is common for programmers to choose the shortest variable names they can under the misconception that it will save time typing. Whatever time might be saved typing will be lost several-fold in debugging. A worthy goal of programming is to write self-documenting code, where the variable names that you choose contribute to quickly understanding what your code does.
Introducing Constants
A close companion of the variable is the constant. The constant holds some piece of data, but that is defined only when the constant is declared, and is not changed from that point on. This is very useful for referring to values that are referenced in multiple places in the code. These values may need to be changed in future versions of a project, but do not change during the execution of the application. When the value of a constant is to be changed, that change is made only where the constant is defined, and every place in the code that uses the constant automatically uses the new value. Constants are declared in the same way as variables, but use a naming convention of all uppercase letters with underscore characters separating words. Constants are restricted by convention to storing only Boolean, String, and Number values. Here are some examples:
var APP_NAME:String = “Yet Another Test Application”; var ROOT_SERVER_PATH:String = “/Applications/YATA/”; var BASE_FILE_NAME:String = “YATA.swf”;
trace(“Loading “ + APP_NAME + “ from “ + ROOT_SERVER_PATH + BASE_FILE_NAME);
// Outputs: Loading Yet Another Test Application from /Applications/YATA/YATA.swf
Technically, there is nothing to prevent you from assigning a new value to a constant during the execution of the program, but it should never be done. The naming convention of using all uppercase letters is used as a reminder during coding that you should treat the constant data as read-only.
Using Strong Variable Typing
Variables can store different types of data, such as a string, a number, or a date. The type of data used has an impact on how you can work with it. Consider the following code:
var numCars = 1; var numTrucks = “2”;
trace(numCars + numTrucks); // Outputs: 12
30

Getting Started with ActionScript 2.0
This example shows what happens when care is not taken with data types. The variable numCars contains a number, whereas the variable numTrucks contains a string. The + operator has two possible meanings depending on the type of data on either side of the operator. Only if the values on both sides are Numbers does the operator perform a mathematical addition; otherwise it converts non-String data to a String and then joins the two Strings together. This is an example of weak typing.
Making use of strong typing lets the compiler help you with data type issues. Strong typing includes the variable type information with the variable declaration. The compiler then remembers the data type for each subsequent use of that variable, and each time new data is to be assigned to a variable, it checks that the data being assigned is of the same type as the variable being assigned the new data. Any time that the compiler sees a potential data type incompatibility, it reports the error and refuses to finish the compile. Modifying the preceding example to take advantage of strong typing, you get the following code:
var numCars:Number = 1; var numTrucks:Number = “2”; trace(numCars + numTrucks);
//Outputs: **Error** Scene=Scene 1, layer=Layer 1, frame=1:Line 2: Type ; mismatch in assignment statement: found String where Number is required.
The compiler correctly flags that there is a String in a place on line 2 where a Number has been specified, and prevents the compile from succeeding until the error is fixed.
The practice of using strong typing is a huge time-saver for debugging because the compiler shows you errors that could otherwise take hours to find. For strong typing to be most effective, you must be consistent in using it every time you declare a new variable. Strong typing also helps prevent Flash from changing the type of a variable on-the-fly, a capability ActionScript 2.0 inherited from ActionScript 1.0 to maintain backward compatibility. Variables that are created without a type in their declarations are sometimes referred to as variants because their types can vary during execution of the code through automatic type conversions. Variants are generally looked down upon because they can introduce hard- to-find errors into your programs.
The following Try It Out illustrates how strong typing can help prevent data type errors such as those brought on by variants.
In keeping with this best practice, all examples in this book declare variable types any time a variable is created.
Try It Out |
Using Strong Typing to Find Errors Faster |
The following steps show some of the impact of using strong typing to find errors that would otherwise be difficult to trace.
1.Create a new Macromedia Flash document by selecting File New and choosing Flash Document from the New Document panel.
2.Click the first frame in the timeline, open the Actions panel (Window Actions), and type in the following ActionScript code:
var isFirstTime; isFirstTime = “false”; if (isFirstTime == false)
31

Chapter 2
{
trace(“isFirstTime is false”);
}
3.Select Control Test Movie and take a look at the contents of the output panel.
4.Modify the code to look like this:
var isFirstTime:Boolean; isFirstTime = “false”; if (isFirstTime == false)
{
trace(“isFirstTime is false”);
}
5.Select Control Test Movie and take a look at the contents of the output panel.
6.Fix the error by removing the quotes from around false:
var isFirstTime:Boolean; isFirstTime = false;
if (isFirstTime == false)
{
trace(“isFirstTime is false”);
}
7.Select Control Test Movie and take a look at the contents of the output panel.
8.Add the following code to the end of the listing:
var numRecords; numRecords = “23”;
for (var i:Number = 0; i < numRecords + 1; i++){ trace(“Parsing row “ + i);
}
9.Select Control Test Movie and take a look at the contents of the output panel.
10.Modify the added code to look like this:
var numRecords:Number; numRecords = “23”;
for (var i:Number = 0; i < numRecords + 1; i++){ trace(“Parsing row “ + i);
}
11.Select Control Test Movie and take a look at the contents of the output panel.
12.Fix the error by removing the quotes from around 23:
var numRecords:Number; numRecords = 23;
for (var i:Number = 0; i < numRecords + 1; i++){ trace(“Parsing row “ + i);
}
13.Select Control Test Movie and take a look at the contents of the output panel.
32

Getting Started with ActionScript 2.0
How It Works
A Boolean data type is very simple, given that it can hold only a true or a false value, yet it is a common source for errors. One error is to surround the keywords true and false with quotes, which turns them into String values instead of Boolean values. The first line assigns a String value to what should be a Boolean variable. The next line does a check to see if the variable isFirstTime contains the Boolean value false, but because isFirstTime contains the String value “false”, the trace() statement does not run.
When the first line is changed to read var isFirstTime:Boolean;, the compiler gives the following warning and does not complete the compilation:
**Error** Scene=Scene 1, layer=Layer 1, frame=1:Line 1: Type mismatch in assignment statement: found String where Boolean is required.
isFirstTime = “false”;
Total ActionScript Errors: 1 |
Reported Errors: 1 |
This error is a good thing because it reports a potential problem that could otherwise go initially undetected and be difficult to debug. The error reports which line of the source code is at issue, prints out the line, and describes the problem. In this example, the line number is preceded by a description of the timeline that holds the line of code in question, which is generally the first layer of the first frame in the main timeline. If the code is from an external file, the name of the file containing the problematic code is shown instead. Here a type mismatch is reported, where the type of “false”, which is a string, does not match the declared type of isFirstTime, which is a Boolean. Removing the quotes from around “false” is enough to fix the problem.
The example using the numRecords variable is a bit nefarious because Flash actually changes the type of the variable on-the-fly. First there’s the variable declaration where numRecords is assigned the String “23”. This is distinct from the number 23, which would not be surrounded by quotes.
var numRecords = “23”;
Loops are discussed later in this book, so don’t worry about the details of the loop itself right now. What you should note is that the code between the curly brackets is supposed to run 23 + 1 = 24 times. Instead, it runs 231 times! What happens is that numRecords contains a String, and the operation “23” + 1 causes the + operator to be used for concatenating strings instead of mathematical addition. The number 1 is converted to a string and joined with “23”, resulting in the string “231”. Unfortunately, the loop then uses the string “231” to determine when to stop. Seeing that it looks like a number, it converts the string on-the-fly and happily loops through 231 times.
When you add the strong typing to declare numRecords as a Number, the compiler catches the problem and spits out a type mismatch error. Once again, removing the quotes fixes the problem.
Exploring Data Types
Variables can store many different types of data. To see what data types are all available, open a new Macromedia Flash document, open the Actions panel (Window Development Panels Actions Panel), and type var myData:. After typing the colon, the auto-complete feature shows you a drop-down of all the data types from which you can choose. The built-in data types include the following:
33
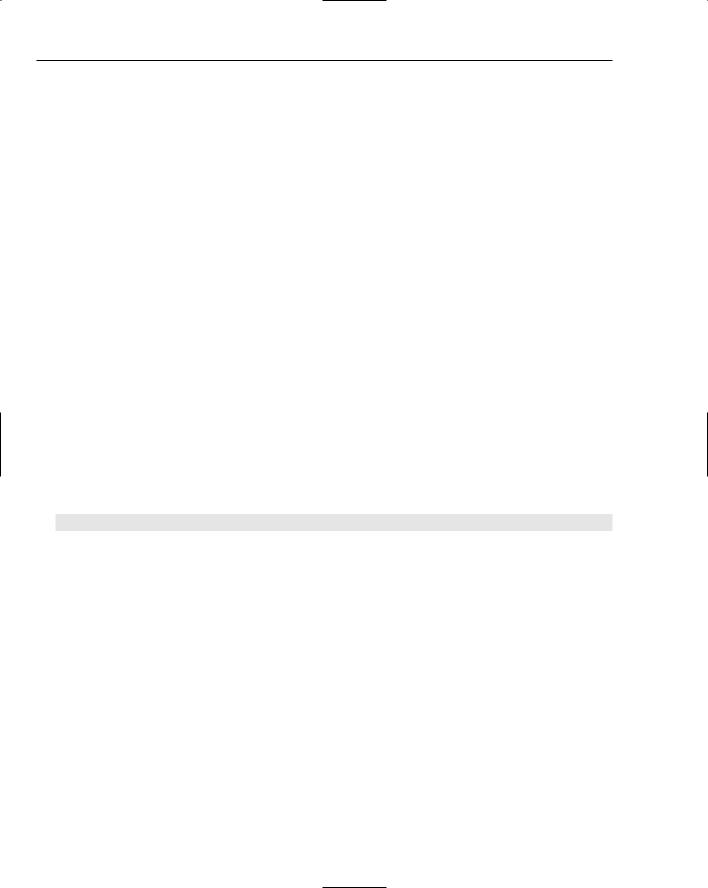
Chapter 2
Array — Stores a collection of data.
Boolean — Stores either the value true or the value false.
Date — Stores dates and times.
MovieClip — Stores a data structure that defines an onstage movie clip.
Number — Stores an integer or a decimal value.
Object — The base type from which the other types are derived.
String — Stores text.
TextField — Allows access to an onscreen text field.
The data types Boolean, Number, and String are referred to as primitive data types because they cannot be broken down into anything simpler. Other types, such as Date, MovieClip, and TextField, place some sort of structure on the data stored within. These are referred to as composite data types. The Date type consists of a numerical representation of the date, plus methods that enable you to retrieve just a portion of the date, such as the month, the day, or the hour of the day. The MovieClip type is a complex representation of all the elements contained within a movie clip, plus additional information regarding position, transparency, attached variables, and much more. The TextField type stores information about text placed onscreen, such as the actual string to display, the position onscreen, the font in which to display it, and the font size. Another composite data type, the Array, is a structured collection of data of any type. (Arrays are discussed in detail later in this chapter.) In general, composite data types internally consist of a collection of data types, plus they provide code that enables you interact with the data in a controlled way.
Data types exist to represent many entities within Macromedia Flash, including components. (Components are examined in detail in Chapters 9–11.) When working with components, you may from time to time see notation like the following:
var saveButton:mx.controls.Button;
A component definition is treated as a data type. A special dotted data type notation indicates that the definition for the Button component is organized in a hierarchy, similar to the way in which you organize your computer files into folders. This example declaration of myButton tells the compiler to look for the definition for the particular data type in a specific location, namely the file Button.as in <Flash Application Root>/First Run/Classes/mx/controls/.
Distinguishing Primitive and Composite Data Types
The reason for classifying data types into two categories is that their data is stored differently (see Figure 2-2) in main memory, and as a result, the variables behave differently. There’s only one value to store for primitive data types, and that can fit directly in the variable’s assigned memory space. There are multiple data elements to store for composite data types, and they require additional structure to be stored in main memory.
Composite data is too large to store directly in the variable, so it’s stored elsewhere in main memory and the variable holds only a numerical address describing where to find the actual data. This address is sometimes referred to as a pointer because it points to where in memory the actual data can be found. You never see this address, nor will you work with it directly. You need to know about it only because it affects the way you work with passing variables.
34

Getting Started with ActionScript 2.0
numBoxes:Number
1
anniversaryDate:Date
data is at memory |
|
|
|
year = 2006 |
location 143568754 |
|
|
|
month = 6 |
|
|
|
|
day = 3 |
|
|
|
||
|
|
... |
||
|
|
|
|
|
Figure 2-2
Passing by Value Versus Pass by Reference
When primitive data types are passed from one variable to another, the data is passed by value, which means that a complete duplicate of the data is made and passed along to the receiving variable.
Composite data types behave differently, in that assigning a composite data type from one variable to another does not make a copy of the data, but only of the numerical address describing where the data is located. This behavior is called passing by reference.
Here’s an example that shows what happens when you use variable assignment to copy a date. Many people try to make a copy of a date by assigning a variable holding a date to another variable, but as you’ve learned, copying the contents of a variable containing composite data does not work as expected.
var anniversaryDate:Date = new Date(2006, 5, 3); // Create a new date var nextAnniversaryDate:Date = anniversaryDate; // Assign the date to // nextAnniversaryDate
nextAnniversaryDate.setYear(2007); // Change the year to 2007 trace(“anniversaryDate: “ + anniversaryDate); trace(“nextAnniversaryDate: “ + nextAnniversaryDate);
Line 1 creates a new Date. A Date is a composite data type, so anniversaryDate does not store the actual date; it only stores the reference to where the date data is located. Line 2 assigns the contents of anniversaryDate to nextAnniversaryDate. It is easy to think that this makes a copy of the date, but it does not. Instead, just the reference to the date data is copied from anniversaryDate to nextAnniversaryDate, so that they point to the same date data, as shown in Figure 2-3. In line 3, the year is changed to 2007. In the last two lines, the trace() statements retrieve the data held by anniversaryDate and by nextAnniversaryDate, and reveal that they hold the same value.
Basically, you just can’t use variable assignment to duplicate anything other than Number, Boolean, and String values. But that doesn’t mean you can’t duplicate the data of other data types.
35

Chapter 2
anniversaryDate:Date
data is at memory location 143568754
nextAnniversaryDate:Date
data is at memory location 143568754
Figure 2-3
year = 2007 month = 6 day = 3
...
Duplicating Data for Composite Types
Given that assigning a variable containing a composite data type to another variable does not actually copy the data, how is a copy made? In general, the data to be duplicated needs to either be copied manually, or it needs to provide the capability to clone itself. In the previous section, you saw how trying to make a copy of the Date class did not quite work as expected. This class does not support the capability to clone itself, so you have to create a second copy manually:
var anniversaryDate:Date = new Date(2006, 6, 3); // Create a new date
var nextAnniversaryDate:Date = new Date(2007, 6, 3); // Create a second date //manually
The MovieClip class provides a means of cloning itself. To duplicate a movie clip, use the duplicate() method that the class makes available. To see how this works, first put a movie clip on the stage:
var ball1Clip:MovieClip;
ball1Clip = _level0.createEmptyMovieClip(“ball1_mc”, 1);
ball1Clip._x = 100; trace(ball1._x); // Outputs: 100;
This code creates a single empty movie clip on level 0, which is the bottom-most level in any Flash movie. It then moves the movie clip to an x coordinate of 100, which places it 100 pixels from the left edge of the movie. If you try this, you won’t actually see anything because there is nothing in the movie clip. The trace() statement indicates, though, that the movie clip has moved to its starting position. Now make a copy of the movie clip:
var ball1Clip:MovieClip;
ball1Clip = _level0.createEmptyMovieClip(“ball1_mc”, 1);
var ball2Clip:MovieClip = myMC1.duplicateMovieClip(“ball2_mc”, 2); ball1Clip._x = 100;
ball2Clip._x = 200; trace(ball1Clip._x); // Outputs: 100; trace(ball2Clip._x); // Outputs: 200;
In the third line, duplicateMovieClip() makes a complete copy of the movie clip referenced by ball1Clip, and assigns a handle to the new movie clip to ball2Clip. When the _x properties for each of the two variables are assigned, it’s apparent that the two variables now point to two separate movie clips because they report two different _x coordinates.
36

Getting Started with ActionScript 2.0
As you saw with the Date class, not all data types have convenient methods for making copies, and there is no consistency between data types in how copies are made. This is not unusual, and is not as much of a limitation as you might think. Each data type works in a way that is appropriate for the nature of the data contained within, and that means that making a complete copy of the data is not always the best way to duplicate it.
Now that you have an understanding of variables and data types, you’re ready to see what you can actually do with the different types of data. But first, you need to take a look at one more concept: dot syntax.
Using Dot Syntax
ActionScript is an object-oriented language, which means that it combines data with the code that works on the data into a single entity. Say you have a variable containing an important date. The variable contains not only the date, but also provides you access to some ways of working with the data that make up the date, such as advancing the year or getting the day of the week. You access the code that can work with data through what is called dot syntax.
Every type of data that you work with is represented a class. A class provides the definition for what you can do with data of that type. It defines the nature of the data, the code that acts on the data (called methods), and the properties that describe the data. Dot syntax is used to call a class method or to refer to a class property. (You learn more about classes, objects, methods, and properties as you work your way through this book.)
In dot syntax, the variable is followed by a dot, which is followed the name of a code routine (a method) or of a data descriptor (a property). The general formats for using the dot syntax are
variable.method();
variable.property;
To see listings of all the properties and methods for the standard ActionScript classes, open the Help panel within the authoring environment, and then select ActionScript 2.0 Language Reference ActionScript Classes. Clicking a class brings up a synopsis of all the properties and methods that you can use with that class.
To illustrate the syntax, take a look at how to call properties and methods with the Date class. First, create a new Date instance (you can create the Date instance at the same time you declare the variable, for brevity):
var specialDate:Date = new Date(2006, 5, 3);
This creates a new date on June 3, 2006. (The month is 0-based — month 0 is January — so 5 represents June, not May.) Want to find out which day of the week this date falls on? Checking the language reference for the Date class within the Flash help panel (ActionScript 2.0 Reference ActionScript Classes Date) turns up a method called getDay() , which gives you that information:
var specialDate:Date = new Date(2006, 5, 3);
trace(currentDate.getDay()); // Returns 6, which is Saturday
37