
Beginning ActionScript 2.0 2006
.pdf
Chapter 4
// Outputs: Thu Jul 14 21:24:08 GMT-0700 2005
trace(getDateNextWeek(currentDate));
// Outputs: Thu Jul 21 21:24:08 GMT-0700 2005
trace(currentDate);
// Outputs: Thu Jul 21 21:24:08 GMT-0700 2005
The variable currentDate is passed to getDateNextWeek() as a parameter. The function adds seven days to the date passed in and then returns the new date. Unfortunately, the Date data type is a composite data type, so the function actually changes the original value in doing its calculation. To keep this from happening, a new Date instance needs to be created within the function:
var currentDate:Date = new Date();
function getDateNextWeek(inputDate:Date):Date
{
var tempDate = new Date(); tempDate.setTime(inputDate.getTime()); tempDate.setTime(tempDate.getTime() + 7 * 24 * 60 * 60 * 1000); return tempDate;
}
trace(currentDate);
// Outputs: Thu Jul 14 21:38:59 GMT-0700 2005
trace(getDateNextWeek(currentDate));
// Outputs: Thu Jul 21 21:38:59 GMT-0700 2005
trace(currentDate);
// Outputs: Thu Jul 14 21:38:59 GMT-0700 2005
This time the argument isn’t changed, so there are no longer any side effects.
Side effects are not always terrible, and many functions are written specifically for their side effects, such as to send data to a server through the LoadVars or the XML class. You see examples of these classes in use later in this book. Side effects are bad primarily when it is not immediately obvious what kind of side effect a function has. Clear, explicit naming of functions and variables goes a long way in making
it easier to work with and to debug functions that use side effects. For instance, a function called getFormFields()grabs the contents of several text fields and drop-down boxes, and sends that data to the server; but it isn’t obvious from its name that the function is actually responsible for sending data to a server. If the function were named sendFormDataToServer() or even just sendFormData(), the side effect would be more apparent.
Summar y
This chapter finishes up the introduction to the core ActionScript language. Some points to remember include the following:
A function is the core container for integrating chunks of code into a single command that can be reused.
98

Exploring ActionScript Functions and Scope
Different variables have different visibility, depending on where and how they are declared. The three types of variable scope are local, timeline, and global.
Be careful about introducing side effects to a function. A function should not modify outside data unless it is clear from the function’s name that that is the function’s intended purpose.
Exercises
1.Create a function that takes two string arguments and returns the one that alphabetically comes first. (Hint: Check out the behavior of the comparison operators.)
2.Modify the following function so that it does not directly access the timeline variable from within the function, nor modify the original array:
var fruitArray:Array = new Array(“kumquat”, “apple”, “pear”, “strawberry”, “banana”);
trace(fruitArray);
function sortArray():Void
{
fruitArray.sort();
}
sortArray();
trace(fruitArray);
3.Modify the convertToButton() function so that it also works with rollover and rollout events. Choose a new color to use for the mouseover state.
function drawRectangle(targetMC:MovieClip, rectWidth:Number, rectHeight:Number, bgColor:Number):Void
{
targetMC.moveTo(0, 0); targetMC.lineStyle(1, 0x000000); targetMC.beginFill(bgColor, 100); targetMC.lineTo(rectWidth, 0); targetMC.lineTo(rectWidth, rectHeight); targetMC.lineTo(0, rectHeight); targetMC.lineTo(0, 0);
}
function buttonClickHandler():Void
{
trace(“Button pressed”);
}
function convertToButton(targetMC:MovieClip, callbackFunction:Function):Void
{
drawRectangle(targetMC, 100, 30, 0x333399);
targetMC.onPress = function()
{
this.clear();
drawRectangle(this, 100, 30, 0x3333CC);
99
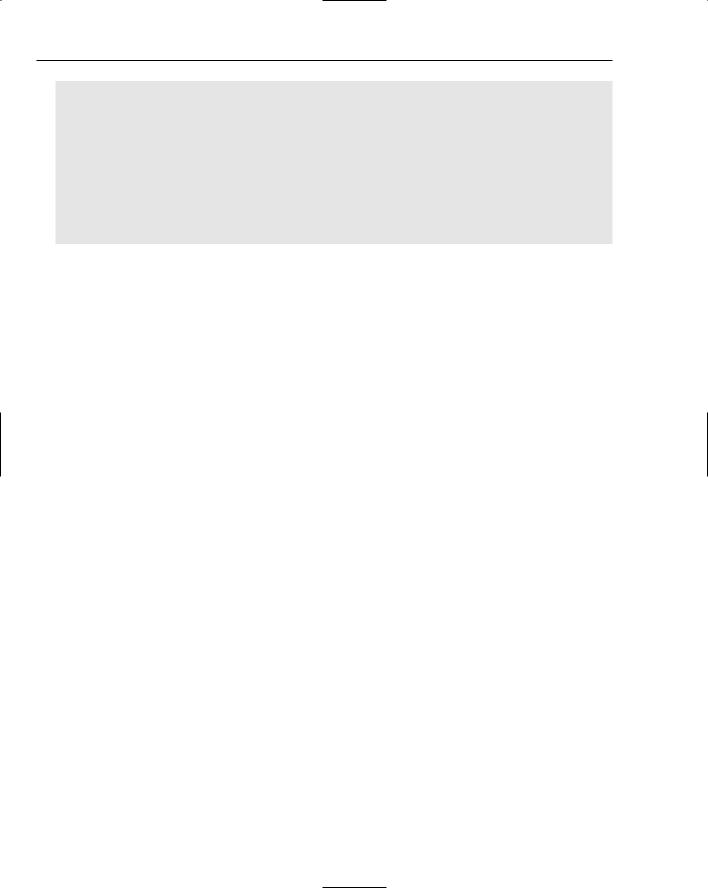
Chapter 4
}
targetMC.onRelease = function()
{
this.clear();
drawRectangle(this, 100, 30, 0x333399);
callbackFunction();
}
}
this.createEmptyMovieClip(“testButton”, 1); convertToButton(testButton, buttonClickHandler);
100
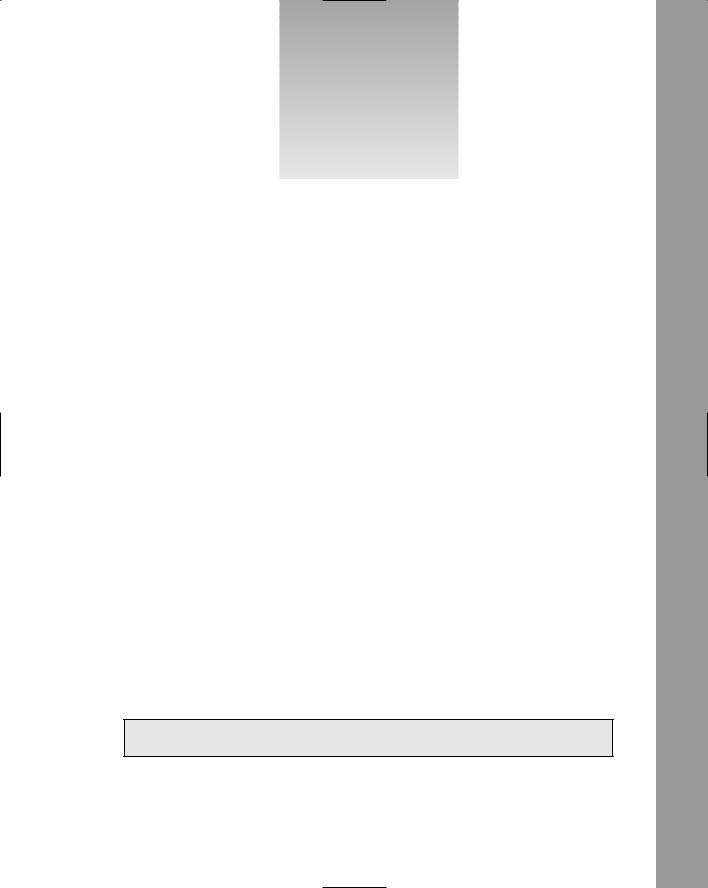
5
Getting Star ted with Coding
With an understanding of the mechanics of programming with ActionScript, it is time to apply some structure to that knowledge. Part of developing programming skills is to learn some larger constructs that help you move from the realm of a coder to the realm of a developer. Even if you are learning ActionScript purely as a personal hobby, you will benefit from these constructs.
This chapter begins by presenting a primer on object-oriented programming (OOP) principles. You learn some of the key object-oriented terminology and why the principles behind object-oriented programming are important. Then the bulk of the chapter teaches you about some programming best practices, and finally you learn some techniques for creating your own functions. You’ve got a lot ahead of you, so let’s get started.
Introduction to Object-Oriented
Programming
The concept of object-oriented programming is core to how many modern programming and scripting languages operate. By learning about OOP principles, you’ll have a better understanding of why ActionScript works the way it does, and if you decide to proceed to making your own custom classes, the knowledge will help you to better grasp that process, too.
Defining Object-Oriented Programming
Numerous definitions of object-oriented programming exist, and there can be many debates about some of the finer details, but the basic premise boils down to the concept of an object, which is
A single entity that holds data and code that acts on that data.

Chapter 5
The use of objects as units of organization is different from the older procedural model where code is basically seen as a list of instructions given to the computer. Technically all programs are just a list of instructions, but object-oriented programming practitioners work at applying a particular organization to those instructions. Various programming languages include features that help in this effort, such as the dot syntax used in ActionScript, JavaScript, and Java.
The package of code and data consists of three things: the data, the methods that act on the data, and the properties that describe the data (see Figure 5-1). The methods that act on the data are really functions, just with a different name. Properties are basically variables, again just with a different name.
Object
Data
“the quick brown fox”
Methods (Functions)
indexOf()
substring()
toLowerCase()
toUpperCase()
...
Properties (Variables)
length
...
Figure 5-1
The dot syntax is used to access methods and properties from an object. In the following example, some text is assigned to a variable called stringObject:
var stringObject:String = “the quick brown fox”;
trace(stringObject.length); // Outputs: 19
trace(stringObject.toUpperCase()); // Outputs: THE QUICK BROWN FOX
This variable now contains a String object, which contains the data “the quick brown fox” as well as different properties and methods that you can use on that data. For instance, the length property reports on the number of characters in the string, and the toUpperCase() method returns a copy of the string with all letters in the string converted to capitals.
It is definitely convenient to have all the functionality that could possibly apply to a particular data type attached directly to that data. There are no libraries to import, no associations to create, and by knowing the data type, a quick search through its documentation reveals all the properties and methods that can be accessed.
102
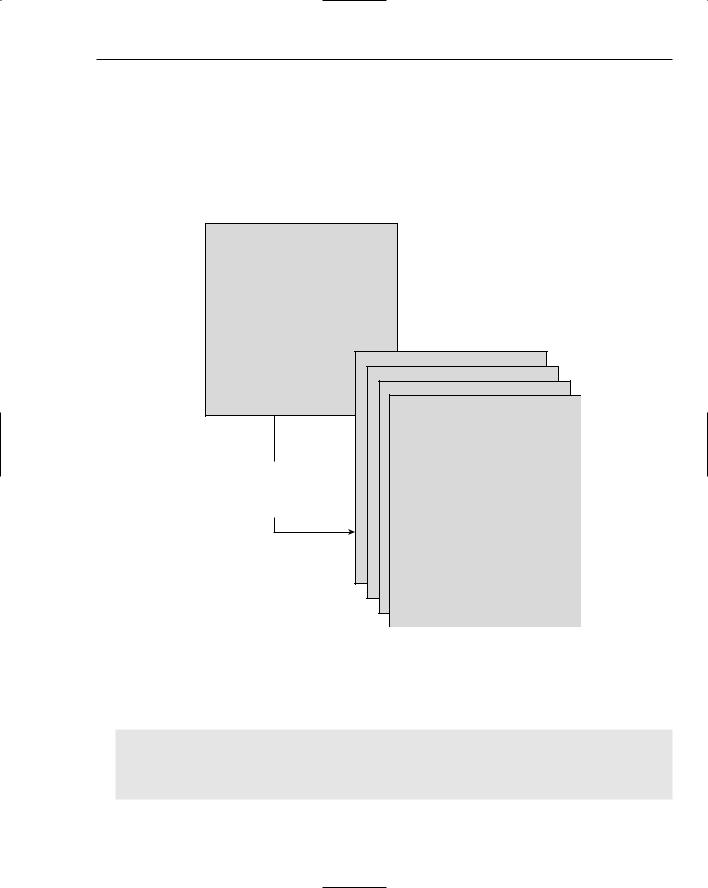
Getting Started with Coding
Classes Versus Objects
A class is a definition or a template from which an object is derived. There is only one copy of any single class definition in memory at any time. The class includes all the code needed by an object, but does not include the data. An object stores the actual data that the class code is to work on, and each object has its own independent data store. Even though you hear the terms object and class used interchangeably, they are not the same thing.
Figure 5-2 illustrates a basic class that is built into the Flash player: the String class.
String Class
Methods
indexOf()
substring()
toLowerCase()
toUpperCase()
Properties
length
The String class is instantiated multiple times to create individual objects.
Figure 5-2
String Object
String Object
String Object
String Object
Data
“the quick brown fox”
Methods
indexOf()
substring()
toLowerCase()
toUpperCase()
Properties
length
There is always exactly one String class, but there can be any number of String objects. Any time that data is assigned to a variable of type String, a String object is created. The following code shows that the properties and methods apply equally well to a String literal as they do to a variable containing a String object:
trace(“the quick brown fox”.length); // Outputs: 19
trace(“the quick brown fox”.toUpperCase()); // Outputs: THE QUICK BROWN FOX
103
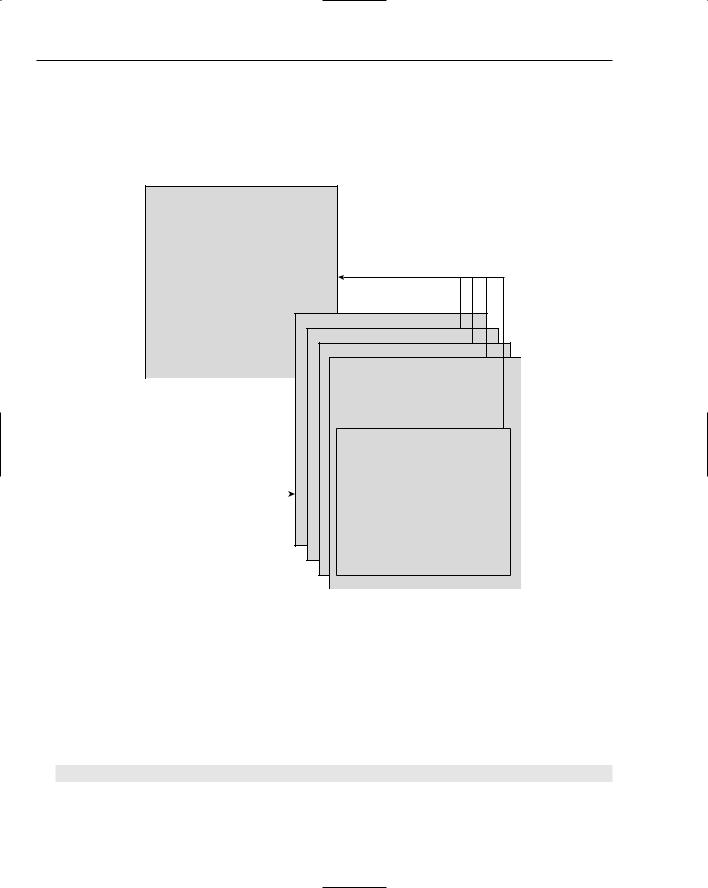
Chapter 5
The term for a single String object is an instance, and the process of creating an instance is called instantiation. Because no real work is needed to create an instance of the String class, it is called implicit instantiation.
Figure 5-2 shows that with each object, all the methods and properties defined by the class are available in the object. Although it looks like all the code is duplicated for each object, this is not the case. Figure 5-3 gives a more accurate picture of what happens.
String Class |
|
Methods |
|
indexOf() |
|
substring() |
|
toLowerCase() |
|
toUpperCase() |
|
Properties |
String Object |
length |
String Object |
|
String Object |
|
String Object |
|
|
|
|
Data |
|
|
|
|
“the quick brown fox” |
The String |
|
class is |
|
Methods |
|
|
|||
instantiated multiple |
|
|||
|
|
|||
times to create |
|
indexOf() |
||
individual objects. |
|
substring() |
||
|
|
|
|
toLowerCase() |
|
|
|
|
toUpperCase() |
|
|
|
Properties
length
Figure 5-3
Duplicating the code for all the properties and methods would take up too much memory, so each object simply points to the code within the class.
The same relationships exist in the Sound class (see Figure 5-4), a more complex data type.
The process for instantiating a new Sound object from the Sound class is a little bit different than the String class’s process. The new operator is used to indicate which class is to be instantiated. The following code snippet creates a new Sound instance:
var introSound:Sound = new Sound();
104

Getting Started with Coding
Sound Class
Methods
attachSound()
getBytesLoaded()
getBytesTotal()
getVolume()
Properties
duration position
The Sound class is instantiated multiple times to create individual objects.
Figure 5-4
Sound Object
Sound Object
Sound Object
Sound Object
Data
<mysong.mp3>
Methods
attachSound()
getBytesLoaded()
getBytesTotal()
getVolume()
Properties
duration position
The new operator creates objects explicitly, and therefore the process is called explicit instantiation. Once instantiated, the object needs to be assigned to a variable so that it can be accessed. The variable to hold the new object is declared to be of the same data type as the class being instantiated.
New instances of the primitive data types — String, Number, and Boolean — are created through implicit instantiation, although explicit instantiation is also possible through the new operator. New instances for all other data types are explicitly created through the new operator.
The following code snippet loads a sound file into the Sound object and starts the playback once the sound file has completely loaded:
var introSound:Sound = new Sound(); introSound.onLoad = function()
{
this.start();
}
introSound.loadSound(“myFirstArrangement.mp3”);
105

Chapter 5
There is a naming convention for classes and objects as well. Class names all start with a capital letter, whereas the names of variables holding object instances, which really encompasses all variables, start with a lowercase letter.
Class Inheritance
The concept of inheritance is also part of object-oriented programming. A good OOP technique is to manage relationships between different classes. Where relationships exist, inheritance allows one class, and thus all objects created from that class, to inherit properties and methods from another class. Inheritance starts from a base class, which defines core methods and properties. Classes can extend (add on to) the base class. Classes that extend a class inherit all of that class’s properties and methods, and can add their own or modify existing ones. Creating your own custom classes is a more advanced topic that is fully explained in Chapter 27.
A good example of inheritance at work in real life is the components that come with the Macromedia Flash development environment. Open the Flash Help panel, navigate to Components Language Reference ComboBox Component ComboBox Class, and you see a number of tables listing the properties, methods, and events available to use with the ComboBox component. Headings include “Method summary for the ComboBox class,” “Methods inherited from the UIObject class,” and “Methods inherited from the UIComponent class.” The UIObject and UIComponent classes are inherited by all components, automatically providing functionality without having to redefine each method, property, and event for each component. For instance, the ComboBox component inherits the setSize() method from the UIObject class. To resize a ComboBox on the stage with the instance name chooseColorCombo, you’d use the following line of code:
chooseColorCombo.setSize(200, 22);
The setSize() method is automatically included with each component that inherits from the UIObject class, without any additional effort by you.
Inheritance allows common code to be implemented once, rather than duplicated in similar classes, and applies a common set of methods to similar classes. This results in consistent usage of the classes and helps keep code clean and focused.
Goals of Object-Oriented Programming
Although a number of goals for object-oriented programming exist, the overriding one is to make code easier to work with, whether you’re part of a group project or on your own. Stemming from this one are the other OOP goals:
Keep code focused. Focused code performs a small number of closely related tasks, rather than a large number of loosely related tasks. Methods within a class are there specifically to support the goal of the class, so classes tend to be fairly focused by design.
Promote code re-use. Being able to reuse and share code brings significant benefits to the programming process, such as simplifying the code, and saving time from not having to re-implement the same task. Smaller elements often work best. If one class has multiple roles, for example, it may work better to break the class into several single-role classes. Those smaller classes can be more readily reused in other parts of the project, or in different projects completely.
106

Getting Started with Coding
Reduce impact of change. It is common for changes to be required either in the middle of development or after all primary development has wrapped up. Even in personal undertakings, it isn’t unusual to alter a project’s goal in mid-development. These changes can be more readily accommodated by code that is easily adapted to different needs — self-contained and focused code.
Keep code understandable. Be organized, use good variable and function naming conventions, and make use of white space. Keep functions small, focused, and well defined. Object-oriented programming helps give code a structure that is easy to follow.
Coding Best Practices
The term best practice is common in the business world. It refers to the process of discovering which organizations excel in a given field, analyzing how they execute their projects, and then looking for common traits in the execution of each of those projects. Those common traits are called the best practices for that field and are used as a model for others to follow. Best practices change over time as technologies change and as new ideas take hold. These practices are not divine gospel, but are an opportunity to learn from the experience of others, and to apply to and expand with your own development work.
A number of best practices taken from other ActionScript programmers will help you in getting started with ActionScript. The following sections explore those practices in the following topics:
Variable naming
Variable typing
Commenting
Formatting code
Variable scope
Accessing variables in another timeline
Creating your own functions
Understanding Variable Naming
How variables are named contributes to how understandable the code is. Developing good variable naming habits will save hours of frustration during debugging and during later development. Here are a few strategies to follow for naming variables.
Making Variable Names Readable
One common variable formatting style is called camelBack. It consists of separating multiple words in a variable name by capitalizing the first letter of each word beginning with the second word. Here are some examples of variables named in the camelBack style:
var applicationName:String; var isFirstPass:Boolean; var highScoresArray:Array;
107