
- •Table of Contents
- •C# and the .NET Platform, Second Edition
- •Introduction
- •Part One: Introducing C# and the .NET Platform
- •Part Two: The C# Programming Language
- •Part Three: Programming with .NET Assemblies
- •Part Four: Leveraging the .NET Libraries
- •Part Five: Web Applications and XML Web Services
- •Obtaining This Book's Source Code
- •The .NET Solution
- •What C# Brings to the Table
- •The Role of the Assembly Manifest
- •Summary
- •Chapter 2: Building C# Applications
- •Summary
- •Chapter 3: C# Language Fundamentals
- •Defining Program Constants
- •Defining Custom Class Methods
- •C# Enumerations
- •Summary
- •The Second Pillar: C#'s Inheritance Support
- •Summary
- •Catching Exceptions
- •Finalizing a Type
- •Garbage Collection Optimizations
- •Summary
- •Chapter 6: Interfaces and Collections
- •Building Comparable Objects (IComparable)
- •Summary
- •Summary
- •Internal Representation of Type Indexers
- •Summary
- •An Overview of .NET Assemblies
- •Understanding Delayed Signing
- •Using a Shared Assembly
- •GAC Internals
- •Summary
- •Spawning Secondary Threads
- •A More Elaborate Threading Example
- •Summary
- •Summary
- •Object Persistence in the .NET Framework
- •The .NET Remoting Namespaces
- •Understanding the .NET Remoting Framework
- •All Together Now!
- •Terms of the .NET Remoting Trade
- •Testing the Remoting Application
- •Revisiting the Activation Mode of WKO Types
- •Deploying the Server to a Remote Machine
- •Summary
- •Control Events
- •The Form Class
- •Summary
- •Regarding the Disposal of System.Drawing Types
- •Understanding the Graphics Class
- •Summary
- •The TextBox Control
- •Working with Panel Controls
- •Configuring a Control's Anchoring Behavior
- •Summary
- •Chapter 16: The System.IO Namespace
- •The Static Members of the Directory Class
- •The Abstract Stream Class
- •Summary
- •The Role of ADO.NET Data Providers
- •The Types of System.Data
- •Selecting a Data Provider
- •The Types of the System.Data.OleDb Namespace
- •Working with the OleDbDataReader
- •Summary
- •Submitting the Form Data (GET and POST)
- •Some Benefits of ASP.NET
- •Creating an ASP.NET Web Application by Hand
- •The Composition of an ASP.NET Page
- •The Derivation of an ASP.NET Page
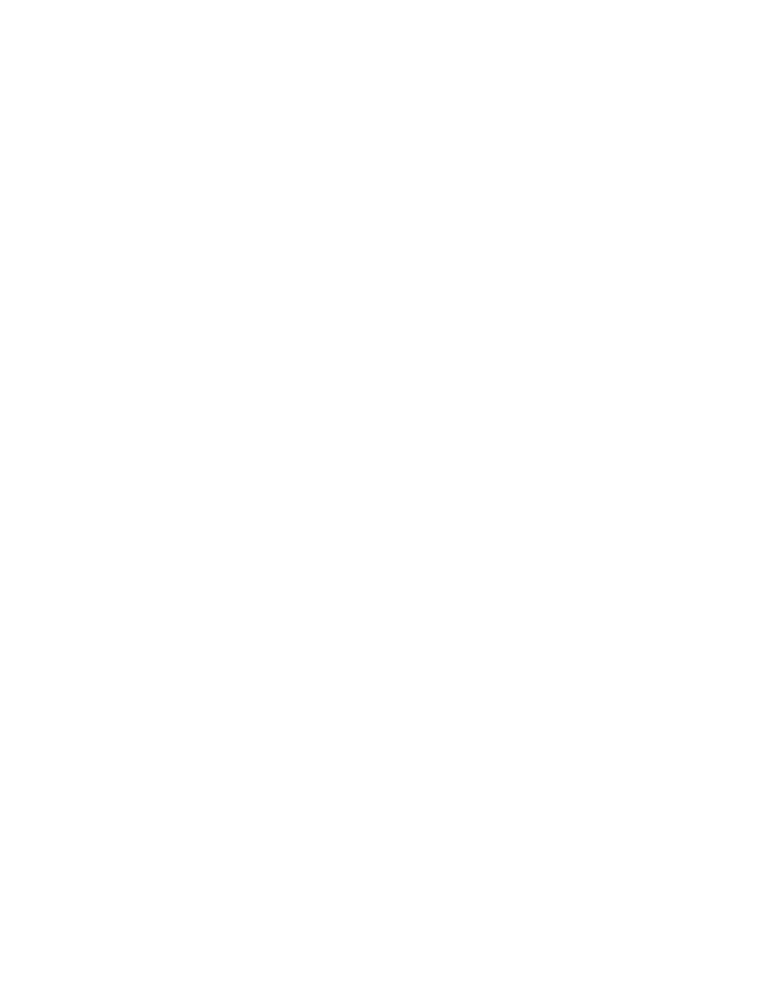
The Role of the Assembly Manifest |
|
C# and the .NET Platform, Second Edition |
|
by Andrew Troelsen |
ISBN:1590590554 |
Last but not least, recall that a valid .NET assembly will also contain metadata that describes the code
Apress © 2003 (1200 pages)
library itself (technically termed a manifest). Like type metadata, it is always the job of the compiler to
This comprehensive text starts with a brief overview of the
generate the assembly'sC# languagemanifestand .thenForquicklyexample,movheres toarekeysometechnicalrelevantand details of the CSharpCalculator
assembly: architectural issues for .NET developers.
.assembly extern mscorlib
Table of Contents
{
C# and the .NET Platform, Second Edition
.publickeytoken = (B7 7A 5C 56 19 34 E0 89)
Introduction
.ver 1:0:3300:0
Part One - Introducing C# and the .NET Platform
}
Chapter 1 - The Philosophy of .NET
.assembly CSharpCalculator
Chapter 2 - Building C# Applications
{
Part Two - The C# Programming Language
.hash algorithm 0x00008004
Chapter 3 - C# Language Fundamentals
.ver 1:0:932:40235
Chapter 4 - Object-Oriented Programming with C#
}
Chapter.module5 - ExceptionsCSharpCalculatorand Object Lifetime.exe
Chapter.imagebase6 - Interfaces0x00400000and Collections
Chapter.subsystem7 - Callback0x00000003Interfaces, Delegates, and Events
.file alignment 512
Chapter 8 - Adva ced C# Type Construction Techniques
.corflags 0x00000001
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
In a nutshell, this manifest metadata documents the list of external assemblies that have been referenced by the CSharpCalculator.exe (via the .assembly extern directive) as well as various characteristics of the
binary itself (via the .assembly, .ver, .module (etc.) directives).
Chapter 12 - Object Serialization and the .NET Remoting Layer Chapter 13 - Building a Better Window (Introducing Windows Forms) Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

Compiling CIL# andtothePlatform.NET Platform,-SpecificSe ond EditionInstructions
by Andrew Troelsen |
ISBN:1590590554 |
Due to the fact that assemblies contain CIL instructions and metadata, rather than platform-specific
Apress © 2003 (1200 pages)
instructions, the underlying CIL must be compiled on the fly before use. The entity that compiles the CIL
This comprehensive text starts with a brief overview of the
into meaningful CPUC# lainstructionsguage and isthentermedquicklya justmoves-in-timeto key(JIT)technicalcompilerandthat sometimes goes by the friendly name of "Jitterarchitectural." The .issuesNET runtimefor .NETenvironmentdevelopers. leverages a JIT compiler for each CPU targeting the CLR, each of which is optimized for the platform it is targeting.
TableFor example,of Contentsif you are building a .NET application that is to be deployed on a handheld device, the C#correspondingand the .NET JitterPlatform,is wellSecond-equippedEditionto run within a low-memory environment. On the other hand, if you
are deploying your assembly to a back end server (where memory is seldom an issue), the related Jitter
Introduction
will be optimized to function in a high-memory environment. In this way, developers can write a single body
Part One - Introducing C# and the .NET Platform
of code that can be efficiently JIT-compiled and executed on machines with different architectures.
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
Furthermore, as a given Jitter compiles CIL instructions into corresponding machine code, it will cache the
Part Two - The C# Programming Language
results in memory in a manner suited to the target OS. In this way, if a call is made to a method named
Chapter 3 - C# Language Fundamentals
Bar() defined within a class named Foo, the Bar() CIL instructions are compiled into platform-specific
Chapter 4 - Object-Oriented Programming with C#
instructions on the first invocation and retained in memory for later use. Therefore, the next time Bar() is
Chapter 5 - Exceptions and Object Lifetime
called, there is no need to recompile the CIL. As you will see in Chapter 9, the .NET SDK also provides a
Chapter 6 - Interfaces and Collections
tool called ngen.exe that will compile CIL code to a native image at the time of installation.
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables
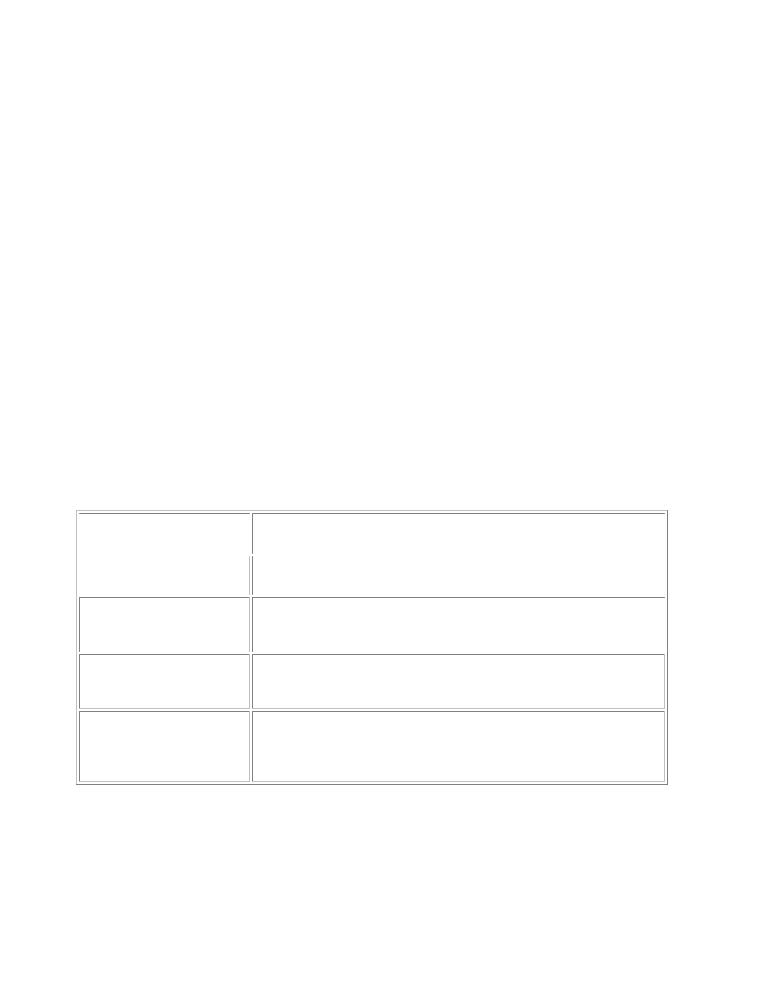
UnderstandingC# andthet .CommonNET Platf rm,TypeSecond SystemEdition
by Andrew Troelsen |
ISBN:1590590554 |
As already mentioned, a given assembly (single file or multifile) may contain any number of distinct
Apress © 2003 (1200 pages)
"types." In the world of .NET, a type is simply a generic term used to collectively refer to an entity from the
This comprehensive text starts with a brief overview of the
set {class, structure,C# languinterface,ge andenumeration,then quicklydelegate}moves to. keyWhentechnicalyou buildandsolutions using a .NET-aware language (such asarchitecturalC#), you willissuesmostforlikely.NETinteractd veloperswith. each of these types. For example, your assembly may define a single class that implements some number of interfaces. Perhaps one of the interface methods takes a custom enum type as an input parameter and returns a populated structure to the caller.
Table of Contents
C#Recalland thethat.NETthe CommonPlatform, SecondType SystemEdition (CTS) is a formal specification that describes how a given type
(class, structure, interface, etc.) must be defined in order to be hosted by the CLR. Also recall that the
Introduction
CTS defines a number of syntactic constructs (such as the use of unsigned types) that may or may not be
Part One - Introducing C# and the .NET Platform
supported by a given .NET-aware language. When you wish to build assemblies that can be used by all
Chapter 1 - The Philosophy of .NET
possible .NET-aware languages, you need to conform your exposed types to the rules of the CLS (defined
Chapter 2 - Building C# Applications
shortly). For the time being, let's preview the formal definitions of all possible CTS types.
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
ChapterCTS4Class- ObjectTypes-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Every .NET-aware language supports, at the very least, the notion of a "class type," which is the
Chapter 6 - Interfaces and Collections
cornerstone of object-oriented programming. A class may be composed of any number of members (such
Chapter 7 - Callback Interfaces, Delegates, and Events
as constructors, operators, properties, methods, and events) and data points (fields). As you would expect,
Chapter 8 - Advanced C# Type Construction Techniques
the CTS allows a given class to support virtual and abstract members that define a polymorphic interface for derived classes. On the limiting side of the equation, CTS classes may only derive from a single base class (multiple inheritance is not allowed for class types). Chapter 4 provides all the gory details of building
CTS class types with C#, however, Table 1-2 documents a number of characteristics pertaining to class
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming types.
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer |
||||||
Table 1-2: CTS Class Characteristics |
||||||
|
Chapter 13 - Building a Better |
Window (Introducing Windows Forms) |
|
|
||
|
ChapterClass14 - A Better Painting |
FrameworkMeaning(GDI+)in Life |
|
|
||
|
ChapterCharacteristic15 - Programming with |
Windows Forms Controls |
|
|
||
|
|
|
|
|
|
|
|
Chapter 16 - The System.IO Namespace |
|
|
|||
|
Is the class "sealed" or |
|
|
Sealed classes are types that cannot function as a base class to |
|
|
|
Chapter 17 - Data Access with ADO.NET |
|
|
|||
|
not? |
|
|
other types. |
|
|
Part Five - Web Applications and |
|
|
XML Web Services |
|
|
|
|
|
|||||
ChaptDoesr 18the-classASP.NETimplementWeb Pages |
|
|
Ana dinterfaceWeb Controlsis a collection of abstract members that provide a |
|
|
|
Chapterany interfaces?19 - ASP.NET Web Applicationscontract between the object and object user. The CTS allows a |
|
|
||||
|
Chapter 20 - XML Web Services |
|
|
class to implement any number of interfaces. |
|
|
Index
Is the class abstract or
Listconcrete?f Figures
List of Tables
What is the "visibility" of this class?
Abstract classes cannot be directly created, but are intended to define common behaviors for derived types. Concrete classes can be created directly.
Each class must be configured with a visibility attribute. Basically this trait defines if the class may be used by external assemblies, or only from within the containing assembly (e.g., a private helper class).
CTS Structure Types
The concept of a structure is also formalized under the CTS. If you have a C background, you should be pleased to know that these user-defined types (UDTs) have survived in the world of .NET (although they behave a bit differently under the hood). In general, a structure can be thought of as a lightweight class type having value semantics. For more details on the subtleties see Chapter 3. For example, CTS structures may define any number of parameterized constructors (the no-argument constructor is reserved). In this way, you are able to establish the value of each field during the time of construction.
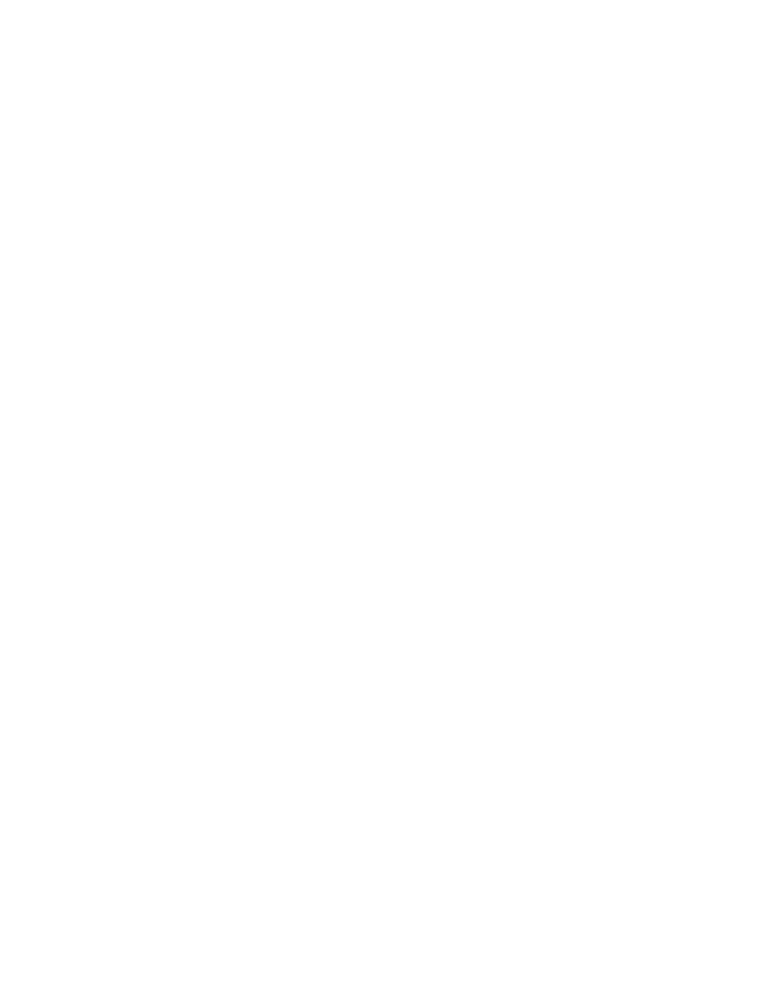
While structures are best suited for modeling geometric and mathematical types, the following type offers
C# and the .NET Platform, Second Edition a bit more pizzazz (at the risk of offending the C++ purists):
|
by Andrew Troelsen |
ISBN:1590590554 |
// Create a |
Apress © 2003 (1200 pages) |
|
C# structure. |
|
|
struct Baby |
This comprehensive text starts with a brief overview of the |
|
C# language and then quickly moves to key technical and |
{architectural issues for .NET developers.
//Structures can contain fields.
public string name;
Table of Contents
// Structures can contain constructors (with arguments).
C# and the .NET Pla form, Seco d Edition
public Baby(string name)
Introduction
{ this.name = name; }
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
// Structures may take methods.
Chapter 2 - Building C# Applications
public void Cry()
Part Two - The C# Programming Language
{ Console.WriteLine("Waaaaaaaaaaaah!!!"); }
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
public bool IsSleeping() { return false; }
Chapter 5 - Exceptions and Object Lifetime
public bool IsChanged() { return false; }
Chapter 6 - Interfaces and Collections
}
Chapter 7 - Callback Interfaces, Delegates, and Events Chapter 8 - Advanced C# Type Construction Techniques
PartHereThreeis our- Programmstructure ingactionw th .(assumeNET Ass mbliesthis logic is contained in some Main() method):
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
// Welcome to the world Max Barnaby!!
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Baby barnaBaby = new Baby("Max");
Part Four - Leveraging the .NET Libraries
Console.WriteLine("Changed?: {0} ", barnaBaby.IsChanged());
Chapter 12 - Object Serialization and the .NET Remoting Layer
Console.WriteLine("Sleeping?: {0} ", barnaBaby.IsSleeping());
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter// Show14 -yourA BettertruePaintingcolorsFrameworkMax...(GDI+)
Chapterfor(int15 -iProgramming= 0; < with10000;Windowsi++)Forms Controls
barnaBaby.Cry();
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
As you will see, all CTS structures are derived from a common base class: System.ValueType. This base class configures a type to behave as a stack-allocated entity rather than a heap-allocated entity. Finally,
be aware that the CTS permits structures to implement any number of interfaces; however, structures may
Chapter 20 - XML Web Services
not function as the base type to other classes or structures and are therefore explicitly "sealed."
Index
List of Figures
CTS Interface Types
List of Tables
Interfaces are nothing more than a named collection of abstract member definitions, which may be supported (i.e., implemented) by a given class or structure. Unlike classic COM, .NET interfaces do not derive a common base interface such as IUnknown. In fact, interfaces are the only .NET type that do not derive from a common base type (not even System.Object). This point should be clear, given that interfaces typically express pure protocol and do not provide an implementation.
On their own, interfaces are of little use. However when a class or structure implements a given interface in its unique way, you are able to request access to the supplied functionality using an interface reference in a polymorphic manner. As well, when you create custom interfaces using a .NET-aware programming language, the CTS permits a given interface to derive from multiple base interfaces (unlike classic COM). As you might suspect, this allows us to build some rather exotic behaviors. Interface-based programming in will be fully detailed in Chapter 6.
CTS Enumeration Types
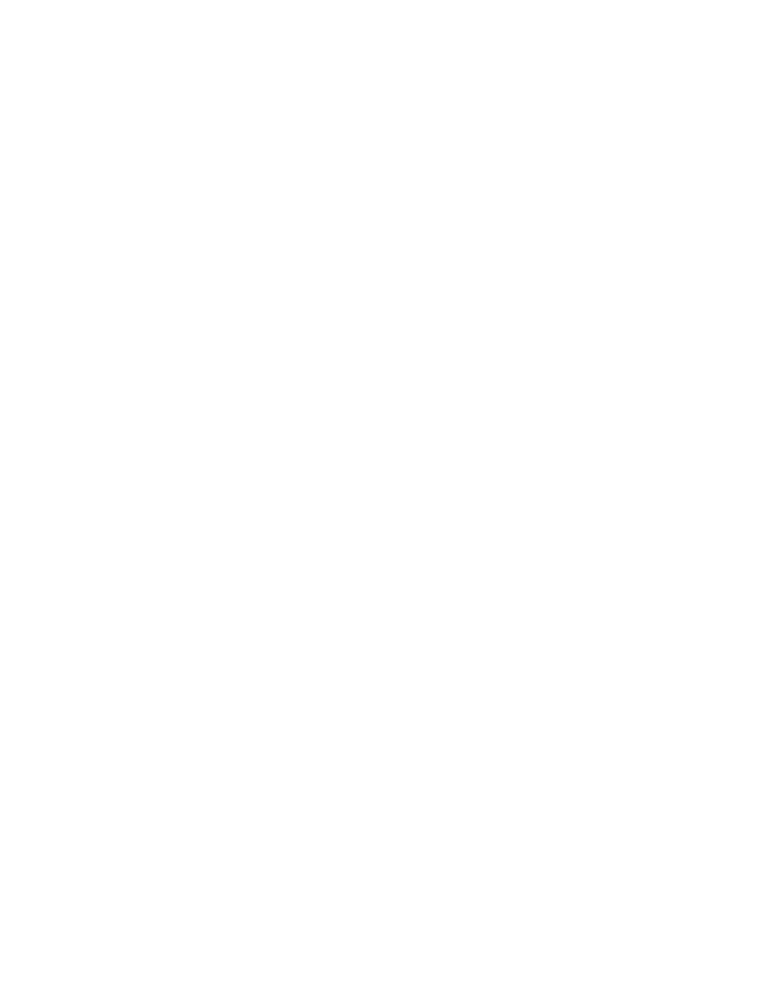
Enumerations are a handy programming construct that allows you to group name/value pairs under a
C# and the .NET Platform, Second Edition
specific name. For example, assume you are creating a video game application that allows the user to
by Andrew Troelsen ISBN:1590590554
select one of three player types (Wizard, Fighter, or Thief). Rather than keeping track of raw numerical
Apress © 2003 (1200 pages)
values to represent each possibility, you could build a custom enumeration:
This comprehensive text starts with a brief overview of the
C# language and then quickly moves to key technical and
// A C# enumeration.
architectural issues for .NET developers.
public enum PlayerType
{Wizard = 100, Fighter = 200, Thief = 300 } ;
Table of Contents
C# and the .NET Platform, Second Edition
By default, the storage used to hold each item is a System.Int32 (i.e., a 32-bit integer), however it is
Introduction
possible to alter this storage slot if need be (e.g., when programming for a low memory device such as a Pocket PC). Also, the CTS demands that enumerated types derive from a common base class, System.Enum. As you will see in Chapter 3, this base class defines a number of interesting members that
allow you to extract, manipulate, and transform the underlying name/value pairs at runtime.
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
CTS Delegate Types
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Delegates are the .NET equivalent of a type safe C style function pointer. The key difference is that a .NET
Chapter 6 - Interfaces and Collections
delegate is a class that derives from System.MulticastDelegate, rather than a simple pointer to a raw
Chapter 7 - Callback Interfaces, Delegates, and Events
memory address. These types are useful when you wish to provide a way for one entity to forward a call to
Chapter 8 - Advanced C# Type Construction Techniques
another entity. Furthermore, delegates provide intrinsic support for multicasting (i.e., forwarding a request
Part Three - Programming with .NET Assemblies
to multiple recipients) and asynchronous method invocations. As you will see in Chapter 7, delegates
Chapter 9 - Understanding .NET Assemblies provide the foundation for the .NET event protocol.
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
CTS Type Members
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Now that you have previewed each of the .NET types formalized by the CTS, realize that each may take
Chapter 13 - Building a Better Window (Introducing Windows Forms)
any number of members. Formally speaking, a type member is constrained by the set {constructor, static
Chapter 14 - A Better Painting Framework (GDI+)
constructor, nested type, operator, method, property, field, constant, event}.
Chapter 15 - Programming with Windows Forms Controls
The CTS defines the various "adornments" that may be associated with a given member. For example,
Chapter 16 - The System.IO Namespace
each member has a given "visibility" trait (e.g., public, private, protected, and so forth). A member may be
Chapter 17 - Data Access with ADO.NET
declared as "abstract" to enforce a polymorphic behavior on derived types as well as "virtual" to define a
Part Five - Web Applications and XML Web Services
canned (but overridable) implementation. As well, most members may be configured as "static" (bound at
Chapter 18 - ASP.NET Web Pages and Web Controls
the class level) or "instance" level (bound at the object level) entities. The construction of type members is
Chapter 19 - ASP.NET Web Applications
examined over the course of the next several chapters.
Chapter 20 - XML Web Services
Index
ListIntrinsicof Figures CTS Data Types
List of Tables
The final aspect of the CTS to be aware of for the time being, is that it establishes a well-defined set of intrinsic data types used by all .NET-aware languages. Although a given language typically has a unique keyword used to declare an intrinsic data type, all languages ultimately resolve to the same type defined in mscorlib.dll. Consider Table 1-3, which documents how key CTS data types are expressed in various
.NET languages.
Table 1-3: The Intrinsic CTS Data Types
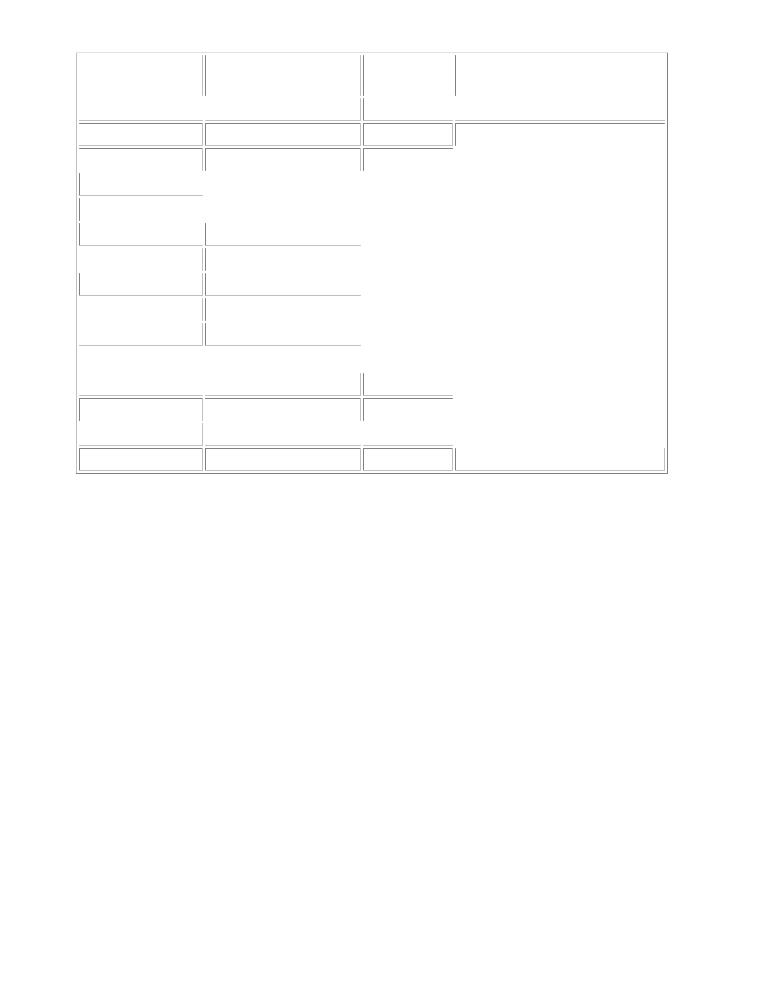
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
.NET Base |
C# andVisualthe .NETBasicPlatform, SecondC#Edition |
|
|
C++ with Managed |
|
||||||||
|
Type |
by Andrew.NETroelsenKeyword |
|
|
|
Keyword |
|
|
ISBN:1590590554Extensions Keyword |
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
System.Byte |
Apress |
|
|
© 2003 (1200 pages) |
|
|
|
byte |
|
|
char |
|
|
|
|
|
|
|
Byte |
|
|
|
|
|
|
|||
|
|
This |
|
|
|
comprehensive text starts with a brief overview |
|
of the |
|
|||||
|
|
|
|
|
|
|
||||||||
|
System.SByte |
C# |
|
|
|
language and then quickly moves to key technical and |
|
|||||||
|
|
|
|
|
Not supported |
|
|
|
sbyte |
|
|
signed char |
|
|
|
|
architectural issues for .NET developers. |
|
|
|
|
||||||||
|
|
|
|
|
|
|||||||||
|
System.Int16 |
|
|
|
|
Short |
|
|
|
short |
|
|
short |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
System.Int32 |
|
|
|
|
Integer |
|
|
|
int |
|
|
int or long |
|
Table of Contents |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
C# and the .NET Platform, |
|
|
|
Second Edition |
|
|
|
long |
|
|
__int64 |
|
||
|
System.Int64 |
|
|
|
|
Long |
|
|
|
|
|
|
||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Introduction |
|
|
|
|
Not supported |
|
|
|
ushort |
|
|
unsigned short |
|
|
System.UInt16 |
|
|
|
|
|
|
|
|
|
|
|||
Part One - Introducing |
|
C# and the .NET Platform |
|
|
|
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Chapter 1 - The Philosophy of .NET |
|
|
|
uint |
|
|
unsigned int or unsigned long |
|
|||||
|
System.UInt32 |
|
|
|
|
Not supported |
|
|
|
|
|
|
||
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
Chapter 2 - Building C# |
|
Applications |
|
|
|
ulong |
|
|
unsigned __int64 |
|
|||
|
System.UInt64 |
|
|
|
|
Not supported |
|
|
|
|
|
|
||
Part Two - The C# Programming Language |
|
|
|
|
|
|
|
|
||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Chapter 3 - C# Language |
Fundamentals |
|
|
|
float |
|
|
float |
|
||||
|
System.Single |
|
|
|
|
Single |
|
|
|
|
|
|
||
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
Chapter 4 - Object-Oriented Programming with C# |
|
|
|
double |
|
|
double |
|
|||||
|
System.Double |
|
|
|
|
Double |
|
|
|
|
|
|
||
|
Chapter 5 - Exceptions |
|
|
|
and Object Lifetime |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||||
|
System.Object |
|
|
|
|
Object |
|
|
|
object |
|
|
Object* |
|
|
Chapter 6 - Interfaces |
|
|
|
and Collections |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
|
Chapter 7 - Callback |
|
|
Interfaces, Delegates, and Events |
|
|
__wchar_t |
|
||||||
|
System.Char |
|
|
|
|
Char |
|
|
|
char |
|
|
|
|
|
Chapter 8 - Advanced |
|
|
|
C# Type Construction Techniques |
|
|
|
|
|||||
|
|
|
|
|
|
|
||||||||
|
System.String |
|
|
|
|
String |
|
|
|
string |
|
|
String* |
|
Part Three - Pro ramming |
|
with .NET Assemblies |
|
|
|
|
|
|
|
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
|
||
|
Chapter 9 - Understanding |
|
.NET Assemblies |
|
|
|
decimal |
|
|
Decimal |
|
|||
|
System.Decimal |
|
|
|
Decimal |
|
|
|
|
|
|
|||
|
Chapter 10 - Processes, |
|
|
|
AppDomains, Contexts, and |
|
|
|
Threads |
|
|
|
|
|
|
|
|
|
|
|
|
|
|||||||
|
|
|
|
|
|
|
|
|
|
ChapterSystem11.Boolean- Type Reflection,BooleanL te Binding, and Attributebool-Based Programmingbool
Part Four - Leveraging the .NET Libraries
ChapterAs you12can- see,ObjectnotSerializationall languagesandaretheable.NETtoRemotingrepresentLayerthe same intrinsic data types of the CTS using Chapterspecific13keywords- Buil ing. Asa Betteryou mightWindowimagine,(I troducingit wouldWindowsbe very helpfulForms) to create a well-known subset of the CTS
that defines a common, shared set of programming constructs (and data types) for all .NET-aware
Chapter 14 - A Better Painting Framework (GDI+)
languages. Enter the CLS.
Chapter 15 - Programming with Windows Forms Controls Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables
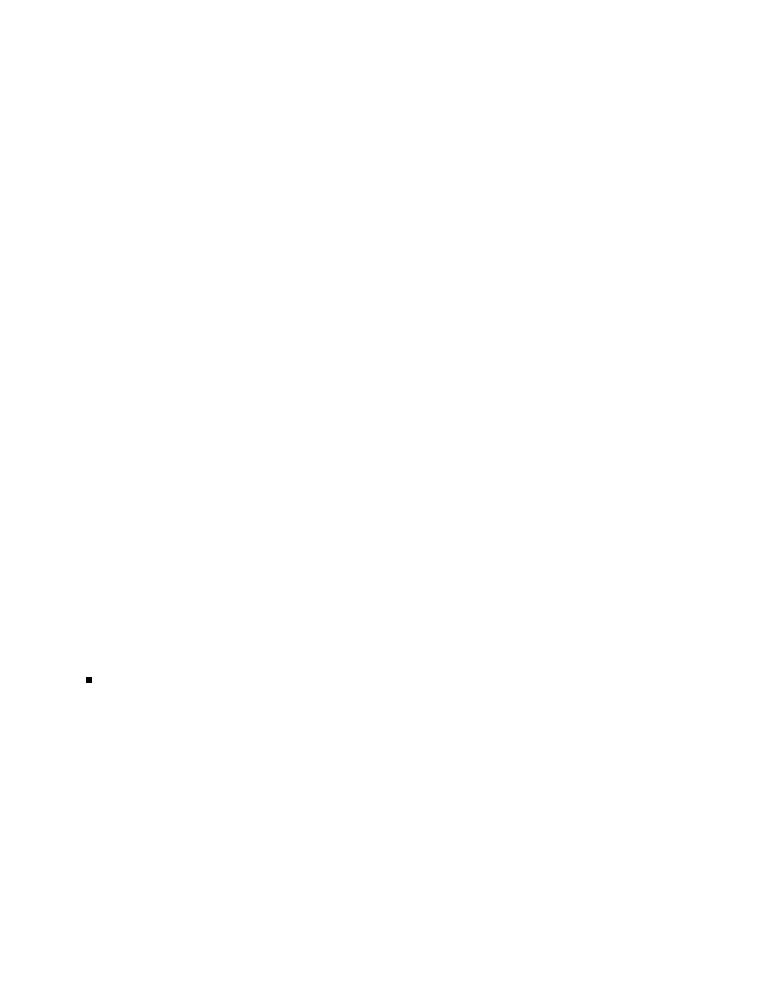
UnderstandingC# andthet .CommonNET Platf rm,LanguageSecond EditionSpecification
by Andrew Troelsen |
ISBN:1590590554 |
As you are aware, different languages express the same programming constructs in unique, language-
Apress © 2003 (1200 pages)
specific terms. For example, in C#, string concatenation is denoted using the plus operator while in Visual
This comprehensive text starts with a brief overview of the
Basic you typicallyC#makel nguageuse ofandthethenampersandquickly moves. Eventowhenkey technicaltwo distinctandlanguages express the same programmatic idiomarchitectur(for example,l issuesaforfunction.NET developewith no returns. value) the chances are very good that the syntax will appear quite different on the surface:
Table of Contents
' VB .NET function returning void (aka VB .NET subroutines).
C# and the .NET Platform, Second Edition
Public Sub Foo()
Introduction
' stuff...
Part One - Introducing C# and the .NET Platform
End Sub
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
// C# function returning void.
Part Two - The C# Programming Language
public void Foo()
Chapter 3 - C# Language Fundamentals
{
Chapter 4 - Object-Oriented Programming with C#
// stuff...
Chapter} 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
As you have already seen, these minor syntactic variations are inconsequential in the eyes of the .NET
Chapter 8 - Advanced C# Type Construction Techniques
runtime, given that the respective compilers (csc.exe or vbc.exe in this case) are configured to emit the
Part Three - Programming with .NET Assemblies
same CIL instruction set. However, languages can also differ with regard to their overall level of
Chapter 9 - Understanding .NET Assemblies
functionality. For example, some languages (C#) allow you to overload operators for a given type while others (VB .NET) do not. Some languages may support the use of unsigned data types, which will not map
correctly in other languages. What we need is to have a baseline to which all .NET aware languages are
Part Four - Leveraging the .NET Libraries
expected to conform.
Chapter 12 - Object Serialization and the .NET Remoting Layer
ChapterThe CLS13is- aBuildingset of guidelinesa Better Windowthat describe(IntroducingvividWindowsdetail, theForminimals) and complete set of features a
Chaptergiven .NET14 --awareA BettercompilerPaintingmustFrameworksupport(GDI+)to produce code that can be hosted by the CLR, while at the
Chsamept rtime15 -beProgrammingaccessed inwitha uniformWindowsmannerForms byControlsall languages that target the .NET platform. In many ways Chapterthe CLS16can- Thebe Systemviewed.IOas Namephysicalpacesubset of the full functionality defined by the CTS.
Chapter 17 - Data Access with ADO.NET
The CLS is ultimately a set of rules that compiler builders must conform to, if they intend their products to function seamlessly within the .NET universe. Each rule is assigned a simple name (e.g., "CLS Rule 6"), and describes how this rule affects those who build the compilers as well as those who (in some way)
interact with them. For example, the crème de la crème of the CLS is the mighty Rule 1:
Chapter 20 - XML Web Services
Index
RULE 1: CLS rules apply only to those parts of a type that are exposed outside the defining assembly.
List of Figures
List of Tables
Given this key rule you can (correctly) infer that the remaining rules of the CLS do not apply to the internal logic used to build the inner workings of a .NET type. Assume you are building a .NET application that exposes three classes, each of which defines a single function. Given the first rule of the CLS, the only aspects of the classes that must conform to the CLS are the member definitions (i.e., naming conventions, parameters, and return types). The internal implementations of each method may use any number of nonCLS techniques, as the outside world won't know the difference.
For example, if you were to add the following member to the C# Calc class type seen previously in this chapter, you would have just written a non-CLS-compliant method, as the parameters and return values make use of an unsigned data type:
public class Calc
{
// CLS compliant!
public int Add(int x, int y)
{ return x + y; }
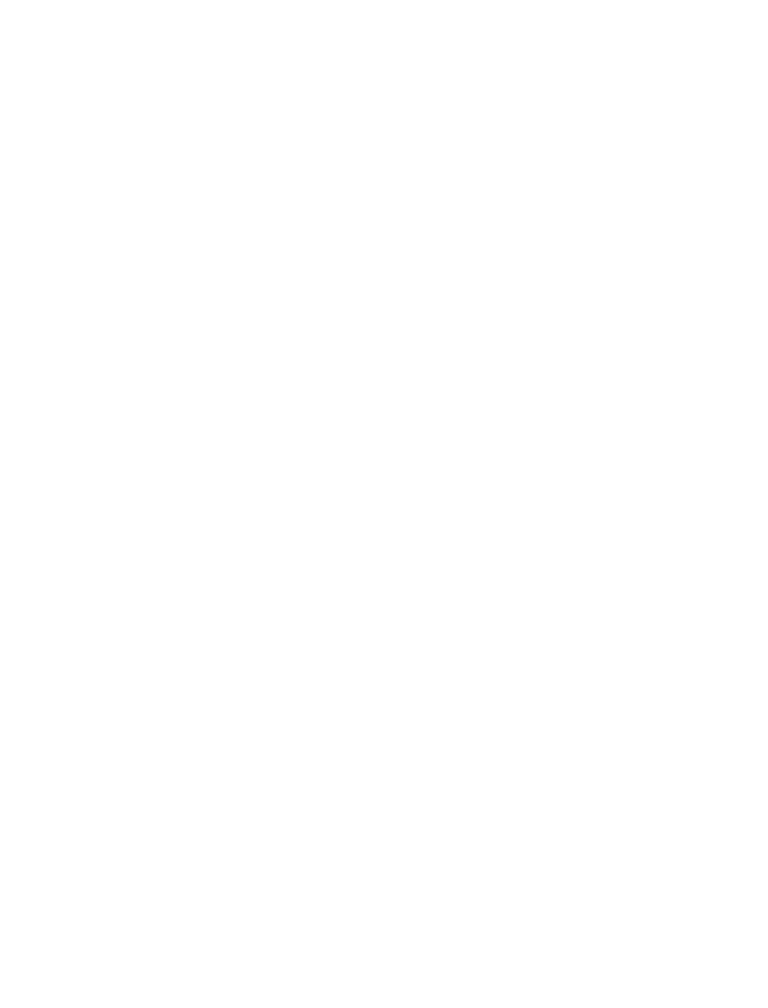
|
C# and the .NET Platform, Second Edition |
|
|
|
// Not CLS compliant! |
y) |
ISBN:1590590554 |
|
by Andrew Troelsen |
||
|
public ulong Add(ulong x, ulong |
|
|
|
Apress © 2003 (1200 pages) |
|
|
} |
{ return x + y;} |
|
|
This comprehensive text starts with a brief overview of the |
|||
|
C# language and then quickly moves to key technical and |
||
|
architectural issues for .NET developers. |
|
However, if you were to update your CLS-compliant method as follows:
Table of Contents
public class Calc
C# and the .NET Platform, Second Edition
{
Introduction
// Still CLS compliant!
Part One - Introducing C# and the .NET Platform
public int Add(int x, int y)
Chapter{1 |
- The Philosophy of .NET |
|
Chapter |
2 |
- Building C# Applications |
|
|
// As this ulong is only used internally, we are still |
Part Two - The C# Programming Language |
||
|
|
// CLS compliant. |
Chapter |
3 |
- C# Language Fundamentals |
|
|
ulong theAnswer = (ulong)(x + y); |
Chapter |
4 |
- Object-Oriented Programming with C# |
|
|
Console.Write("Answer as ulong: {0}\n", |
Chapter |
5 |
- Exceptions and Object Lifetime |
|
|
theAnswer); |
Chapter |
6 |
- In erfaces and Collections |
Chapter}7 |
return x + y; |
|
- Callback Interfaces, Delegates, and Events |
||
Chapter... |
8 |
- Advanced C# Type Construction Techniques |
Part} Three - Programming with .NET Assemblies |
||
Chapter |
9 |
- Understanding .NET Assemblies |
Chapter |
10 |
- Processes, AppDomains, Contexts, and Threads |
Chapter |
11 |
- Type Reflection, Late Binding, and Attribute-Based Programming |
You have still conformed to the rules of the CLS, and can rest assured that all .NET languages are able to
Part Four - Leveraging the .NET Libraries
interact with this implementation of the Add() method.
Chapter 12 |
- Object Serialization and the .NET Remoting Layer |
Chapter 13 |
- Building Better Window (Introducing Windows Forms) |
Of course, in addition to Rule 1, the CLS defines numerous other rules. For example, the CLS describes |
|
C apter 14 |
- A Better Painting Framework (GDI+) |
how a given language must represent text strings, how enumerations should be represented internally (the
Chbaseptertype15 -usedProgrammingfor storage),withhowWindowsto useFormsstatic Controlstypes, and so forth. Again, remember that in most cases
Chapttheserrules16 - doThenotSystemhave.IOto beNamespacecommitted to memory (unless you need to build a LISP .NET compiler!).
Chapter 17 - Data Access with ADO.NET
PartEnsuringFive - Web ApplicationsCLS Complianceand XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
ChapterAs you19will-seeASPover.NET theW bcourseApplicationsof this book, C# does define a number of constructs that are not CLS
Chaptercompliant20 .-TheXMLgoodWeb Snews,rviceshowever, is that you are always free to inform the C# compiler to check your Indexcode for CLS compliance using a single .NET attribute (which must be placed outside the scope of any
namespace or type definitions):
List of Figures
List of Tables
// Tell the C# compiler to check for CLS compliance.
[assembly: System.CLSCompliant(true)]
Chapter 11 dives into the details of attribute-based programming in detail. For the time being, simply understand that this line of code will instruct the C# compiler to check your code for complete CLS compliance. If any non-CLS-compliant syntactic tokens are discovered, you are issued a compiler error and a description of the offending code.
Note If you are interested in investigating each constraint imposed by the CLS, check out Partition I of the Common Language Infrastructure (CLI, not to be confused with CIL). By default, this Word document is located under <drive>:\Program Files\Microsoft Visual Studio .NET 2003\SDK\v1.1\ Tool Developers Guide\Docs. If you can't locate this path on your machine, simply do a search for a folder named "Tool Developers Guide".
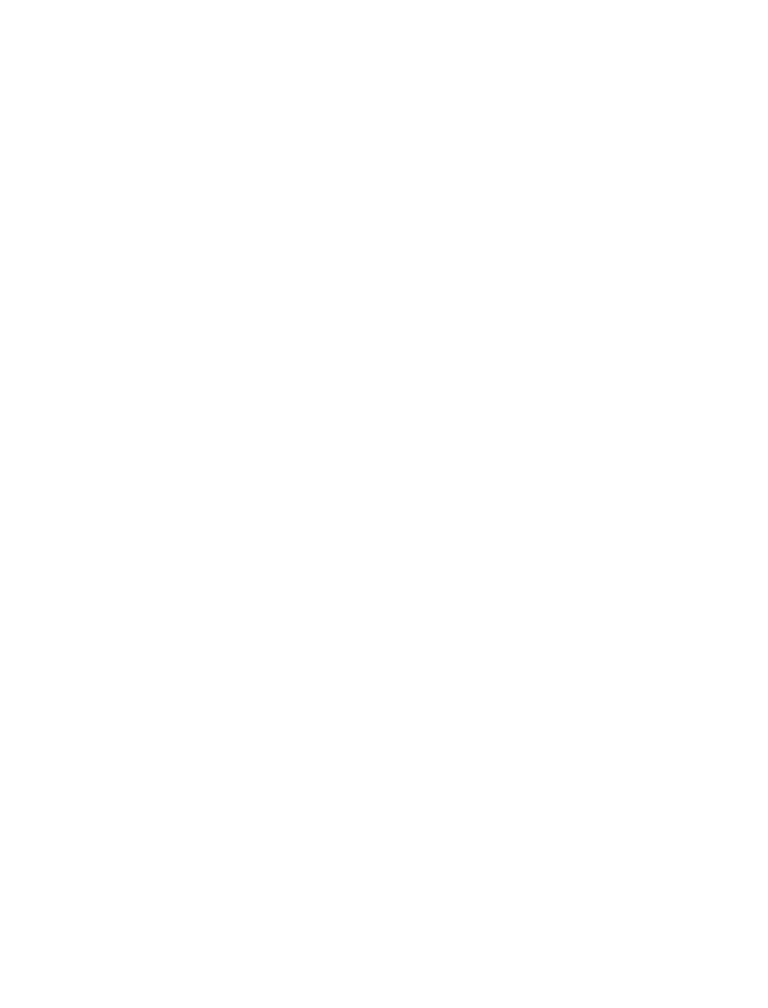
UnderstandingC# andthet .CommonNET Platf rm,LanguageSecond EditionRuntime
by Andrew Troelsen |
ISBN:1590590554 |
In addition to the CTS and CLS specifications, the final TLA (three-letter-acronym) to contend with at the
Apress © 2003 (1200 pages)
moment is the CLR. Programmatically speaking, the term runtimecan be understood as a collection of
This comprehensive text starts with a brief overview of the
external servicesC#thatlanguageare requiredand thento executequicklyamogivens tocompiledkey t chnicalunit ofandcode. For example, when developers makearchitecturaluse of the Microsoftissues forFoundation.NET developersClasses. (MFC) to create a new application, they are (painfully) aware that their binary is required to link with the rather hefty MFC runtime library (e.g., mfc42.dll). Other popular languages also have a corresponding runtime. Visual Basic 6.0 programmers
Table of Contents
are also tied to a runtime module or two (e.g., msvbvm60.dll). Java developers are tied to the Java Virtual
C# and the .NET Platform, Second Edition
Machine (e.g., JVM) and so forth.
Introduction
The .NET platform offers yet another runtime system. The key difference between the .NET runtime and
Part One - Introducing C# and the .NET Platform
the various other runtimes I have just mentioned is the fact that the .NET runtime provides a single well-
Chapter 1 - The Philosophy of .NET
defined runtime layer that is shared by all languages and platforms that are .NET aware. As mentioned
Chapter 2 - Building C# Applications
earlier in this chapter, the .NET runtime is officially termed the common language runtime, or simply CLR.
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
The crux of the CLR is physically represented by an assembly named mscoree.dll (aka, the Common
Chapter 4 - Object-Oriented Programming with C# |
|
Object Runtime Execution Engine). When an assembly is referenced for use, mscoree.dll is loaded |
|
Chapter 5 |
- Exceptions and Object Lifetime |
automatically, which in turn loads the required assembly into memory. The runtime engine is responsible |
|
Chapter 6 |
- Interfaces and Collections |
for a number of tasks. First and foremost, it is the entity in charge of resolving the location of an assembly |
|
Chapter 7 |
- Callback Int rfaces, Delegates, and Events |
and finding the requested type (e.g., class, interface, structure, etc.) within the binary by reading the |
|
Chap er 8 |
- Advanced C# Type Construct on Techniques |
contained metadata. The execution engine lays out the type in memory, compiles the associated CIL into
PartplatformTh ee-specific- Programminginstructions,with .performsNET Assembliesany (optional) security checks and then executes the code in
Chapterquestion9. - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
In addition to loading your custom assemblies and creating your custom types, the CLR will also interact
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
with the types contained within the .NET base class libraries. Although the entire base class library has
Part Four - Leveraging the .NET Libraries
been broken into a number of discrete assemblies, the key binary is mscorlib.dll. This .NET assembly
Chapter 12 - Object Serialization and the .NET Remoting Layer
contains a large number of core types that encapsulate a wide variety of common programming tasks as well as the core data types used by all .NET languages. When you build .NET solutions, you always make
use of this particular assembly, and perhaps numerous other .NET binaries (both system supplied and
Chapter 15 - Programming with Windows Forms Controls custom).
Chapter 16 - The System.IO Namespace
ChapterFigure 17-3-illustratesData AccessthewithworkflowADO.NETthat takes place between your source code (which is making use of
PartbaseFiveclass- WeblibraryApplicationstypes), aandgivenXML.NETWebcompiler,Servicesand the .NET execution engine.
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables
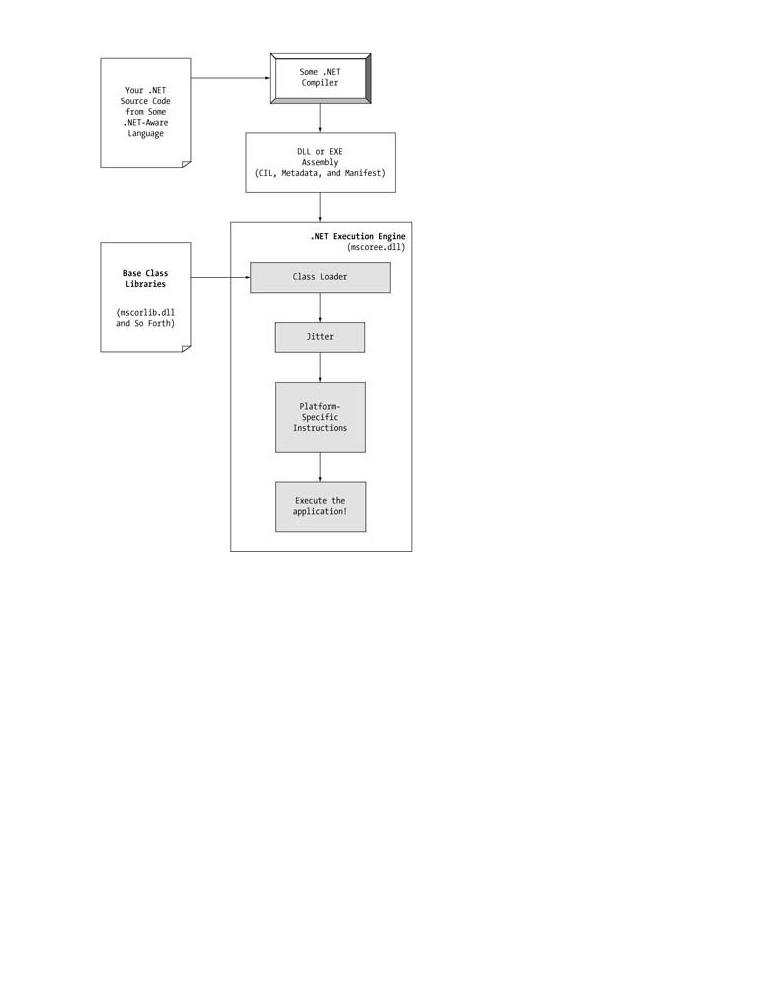
ISBN:1590590554
|
overview of the |
|
technical and |
Table |
|
C# and |
|
Part |
|
Chapter |
|
Chapter |
|
Part |
|
Chapter |
|
Chapter |
|
Chapter |
|
Chapter |
|
Chapter |
|
Chapter |
|
Part |
|
Chapter |
|
Chapter |
|
Chapter |
Programming |
Part |
|
Chapter |
|
Chapter |
Forms) |
Chapter |
|
Chapter |
|
ChapterFigure16 - 1The-3:System.IOmscoree.dllNamespacein action |
|
Chapter 17 - Data Access with ADO.NET |
|
Part Five - Web Applications and XML Web Services |
|
Chapter 18 - ASP.NET Web Pages and Web Controls |
|
Chapter 19 - ASP.NET Web Applications |
|
Chapter 20 - XML Web Services |
|
Index |
|
List of Figures |
|
List of Tables |
|
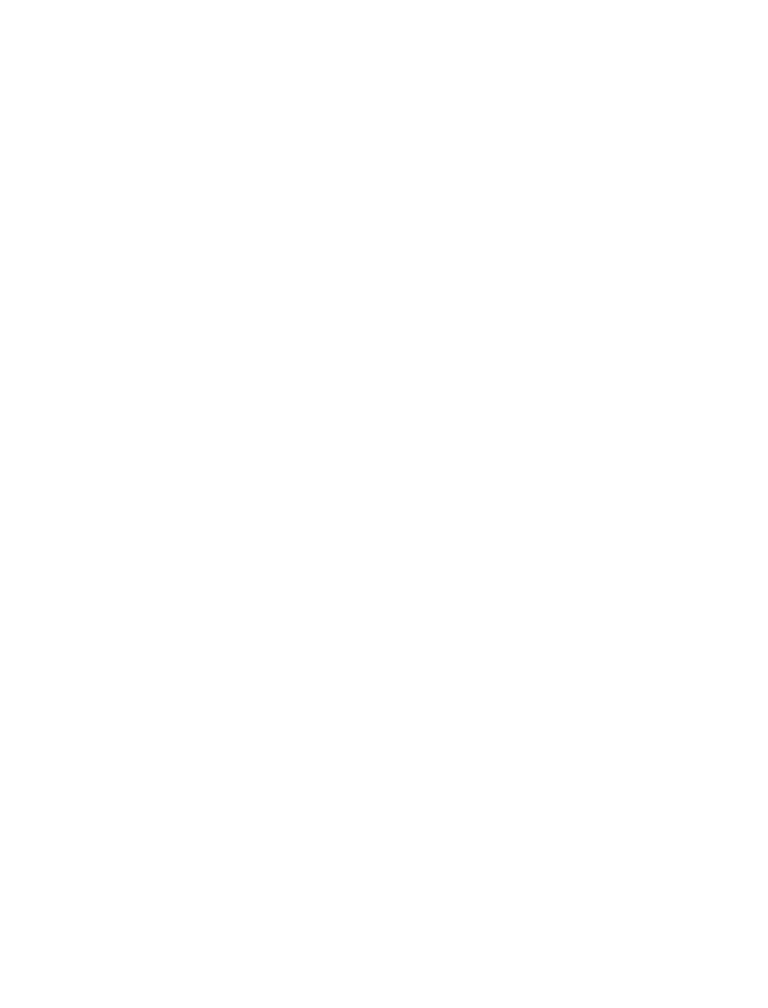
A Tour of theC# and.NETthe Namespaces. ET Platform, S cond Edition
by Andrew Troelsen |
ISBN:1590590554 |
Each of us understands the importance of code libraries. The point of libraries such as MFC, J2EE, or ATL
Apress © 2003 (1200 pages)
is to give developers a well-defined set of existing code to leverage in their applications. For example, MFC
This comprehensive text starts with a brief overview of the
defines a numberC#oflanguageC++ classesand thatenprovidequickly movescannedtoimplementationskey technical andof dialog boxes, menus, and toolbars. This is aarchitecturalgood thing forissuestheforMFC.NETprogrammersdevelopers. of the world, as they can spend less time reinventing the wheel, and more time building a custom solution. Visual Basic and Java offer similar notions: intrinsic types/global functions and packages, respectively.
Table of Contents
C#Unlikeand theMFC,.NETJava,Pl tform,or VisualSecondBasicEdition6.0, the C# language does not come with a predefined set of language-
specific classes. Ergo, there is no C# class library. Rather, C# developers leverage existing types supplied
Introduction
by the .NET Framework. To keep all the types within this binary well organized, the .NET platform makes
Part One - Introducing C# and the .NET Platform
extensive use of the namespace concept.
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
The key difference between this approach and a language-specific library such as MFC, is that any
Part Two - The C# Programming Language
language targeting the .NET runtime makes use of the same namespaces and same types as a C#
Chapter 3 - C# Language Fundamentals
developer. For example, the following three programs all illustrate the ubiquitous "Hello World"
Chapter 4 - Object-Oriented Programming with C#
application, written in C#, VB .NET, and C++ with managed extensions (MC++):
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
// Hello world in C#
Chapter 7 - Callback Interfaces, Delegates, and Events
using System;
Chapter 8 - Advanced C# Type Construction Techniques
public class MyApp
Part Three - Programming with .NET Assemblies
{
Chapter 9 - Understanding .NET Assemblies public static void Main()
Chapter 10 - Processes, AppDomains, Contexts, and Threads
{
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Console.WriteLine("Hi from C#");
Part Four - Leveraging the .NET Libraries
}
Chapter 12 - Object Serialization and the .NET Remoting Layer
}
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapt' Hellor 14 -worldA BetterinPaintingVB .FrameworkNET (GDI+)
ChapterImports15 -SystemProgramming with Windows Forms Controls
Public Module MyApp
Chapter 16 - The System.IO Namespace
Sub Main()
Chapter 17 - Data Access with ADO.NET
Console.WriteLine("Hi from VB .NET")
Part Five - Web Applications and XML Web Services
End Sub
Chapter 18 - ASP.NET Web Pages and Web Controls
End Module
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
// Hello world in Managed C++ (MC++)
Index
#using <mscorlib.dll>
List of Figures
using namespace System;
List of Tables
void main()
{
Console::WriteLine(S"Hi from MC++");
}
Notice that each language is making use of the Console class defined in the System namespace. Beyond minor syntactic variations, these three applications look and feel very much alike, both physically and logically. As you can see, the .NET platform has brought a streamlined elegance to the world of software engineering.
Clearly, your primary goal as a .NET developer is to get to know the wealth of types defined in the numerous base class namespaces. The most fundamental namespace to get your hands around is named "System." This namespace provides a core body of types that you will need to leverage time and again as a .NET developer. In fact, you cannot build any sort of functional C# application without at least making a reference to the System namespace.
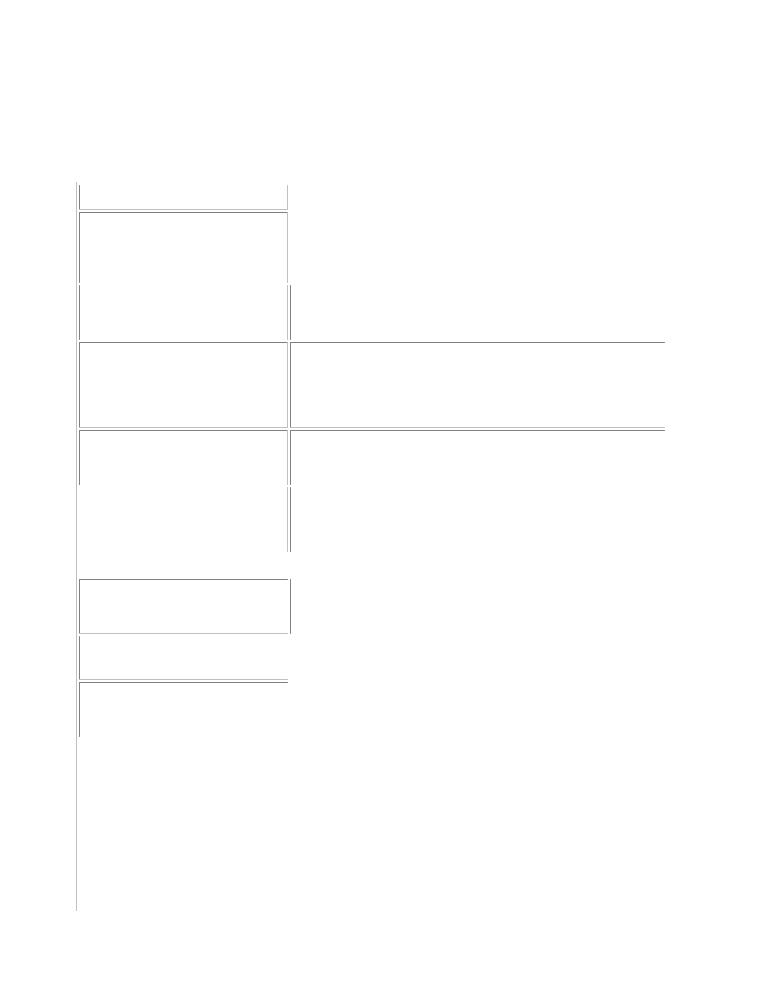
System is the root namespace for numerous other .NET namespaces. Simply put, namespaces are a way
C# and the .NET Platform, Second Edition |
|
to group semantically related types (classes, enumerations, interfaces, delegates, and structures) under a |
|
by Andrew Troelsen |
ISBN:1590590554 |
single umbrella. For example, the System.Drawing namespace contains a number of types to assist you in
Apress © 2003 (1200 pages)
rendering images onto a given graphics device. Other namespaces exist for data access, Web
This comprehensive text starts with a brief overview of the
development, threading, and programmatic security (among many others). From a very high level, Table
|
|
|
C# language and then quickly moves to key technical and |
|||||
|
1-4 offers a rundown of some (but certainly not all) of the .NET namespaces. |
|||||||
|
|
|
architectural issues for .NET developers. |
|||||
|
Table 1-4: A Sampling of .NET Namespaces |
|||||||
|
|
|
|
|
|
|
|
|
Table of Contents |
|
|
Meaning in Life |
|
|
|||
|
|
.NET Namespace |
|
|
|
|
||
C# and the .NET Platform, Second Edition |
|
|
|
|||||
|
|
|
|
|||||
IntroductionSystem |
|
Within System you find numerous low-level classes dealing |
|
|
||||
Part One - Introducing C# and the .NET |
Platformwith primitive types, mathematical manipulations, garbage |
|
|
|||||
Chapter 1 |
- The Philosophy of .NET |
|
collection, as well as a number of commonly used exceptions |
|
|
|||
|
Chapter 2 |
- Building C# Applications |
|
|
and predefined attributes. |
|
|
|
|
|
|
|
|
||||
Part Two - The C# Programming Language |
|
|
||||||
|
|
System.Collections |
This namespace defines a number of stock container objects |
|
|
|||
Chapter 3 - C# Language Fundamentals(ArrayList, Queue, etc.) as well as base types and interfaces |
|
|
||||||
Chapter 4 |
- Object-Oriented Programming with C# |
|
|
|||||
|
|
|
|
|
|
that allow you to build customized collections. |
|
|
|
Chapter 5 - Exceptions and Object Lifetime |
|
|
|||||
|
|
|
||||||
|
|
System.Data |
These namespaces are (of course) used for database |
|
|
|||
|
Chapter 6 |
- Interfaces and Collections |
manipulations (ADO.NET). You will see each of these later in |
|
|
|||
|
|
|
|
|
|
|
|
|
|
ChapterSystem7 .Data- C .llbackCommonInterfaces, Delegates, and Events |
|
|
|||||
|
|
|
|
|
|
this book. |
|
|
|
Chapter 8 - Advanced C# Type Construction Techniques |
|
|
|||||
|
|
System.Data.OleDb |
|
|
|
|||
Part Three - Programming with .NET Assemblies |
|
|
||||||
|
|
System.Data.SqlClient |
|
|
|
|||
|
|
Chapter 9 - Understanding .NET Assemblies |
|
|
||||
|
|
|
|
|||||
|
ChapterSystem10.Diagnostics- Processe , AppDomains, Contexts,Here, youandfindThreadsnumerous types that can be used by any |
|
|
|||||
|
Chapter 11 - Type Reflection, Late Binding,.NETand-awareAttributelanguage-Based Programmingto programmatically debug and trace |
|
|
|||||
Part Four - Leveraging the .NET Librariesyour source code. |
|
|
||||||
|
|
|
|
|
||||
|
|
Chapter 12 - Object Serialization and the .NET Remoting Layer |
|
|
||||
|
|
System.Drawing |
Here, you find numerous types wrapping GDI+ primitives |
|
|
|||
|
Chapter 13 - Building a Better Window (Introducing Windows Forms) |
|
|
|||||
|
|
System.Drawing.Drawing2D |
such as bitmaps, fonts, icons, printing support, and advanced |
|
|
|||
|
Chapter 14 - A Better Painting Framework (GDI+) |
|
|
|||||
|
|
ChapterSystem.Drawing.Printing15 - Programming with Windows |
|
|
graphical rendering support. |
|
|
|
|
|
Forms Controls |
|
|
||||
|
|
|
|
|||||
|
|
Chapter 16 - The System.IO Namespace |
|
|
This namespace includes file IO, buffering, and so forth. |
|
|
|
|
|
System.IO |
|
|
|
|
||
|
|
Chapter 17 - Data Access with ADO.NET |
|
|
|
|
|
|
|
|
|
|
|
|
|
||
PartSystem.NetFive - Web Applications and XML |
WebThisServicesnamespace (as well as other related namespaces) |
|
|
|||||
Chapter 18 - ASP.NET Web Pages and |
|
|
contains types related to network programming |
|
|
|||
Web Controls |
|
|
||||||
|
Chapter 19 - ASP.NET Web Applications |
|
(requests/responses, sockets, end points, etc.). |
|
|
|||
|
|
|
|
|
||||
|
|
Chapter 20 - XML Web Services |
|
|
Defines types that support runtime type discovery and |
|
|
|
|
|
System.Reflection |
|
|
|
|
||
Index |
|
|
|
dynamic creation and invocation of custom types. |
|
|
||
|
|
System.Reflection.Emit |
|
|
|
|
||
List of Figures |
|
|
|
|
|
|||
|
|
|
|
|
||||
ListSystem.Runtime.InteropServicesof Tables |
|
|
Provides facilities to allow .NET types to interact with |
|
|
|||
|
|
|
|
|
|
"unmanaged code" (e.g., C-based DLLs and classic COM |
|
|
|
|
|
|
|
|
servers) and vice versa. |
|
|
|
|
|
|
|||||
|
|
System.Runtime.Remoting |
|
|
This namespace (and other related namespaces) define |
|
|
|
|
|
|
|
|
|
types used to build solutions that incorporate the new .NET |
|
|
|
|
|
|
|
|
Remoting layer (which has nothing to do with classic DCOM |
|
|
|
|
|
|
|
|
whatsoever). |
|
|
|
|
|
|
|||||
|
|
System.Security |
|
|
Security is an integrated aspect of the .NET universe. In the |
|
|
|
|
|
|
|
|
|
security-centric namespaces you find numerous types |
|
|
|
|
|
|
|
|
dealing with permissions, cryptography, and so on. |
|
|
|
|
|
|
|||||
|
|
System.Threading |
|
|
You guessed it, this namespace deals with threading issues. |
|
|
|
|
|
|
|
|
|
Here you will find types such as Mutex, Thread, and Timeout. |
|
|
|
|
|
|
|
|
|
|
|
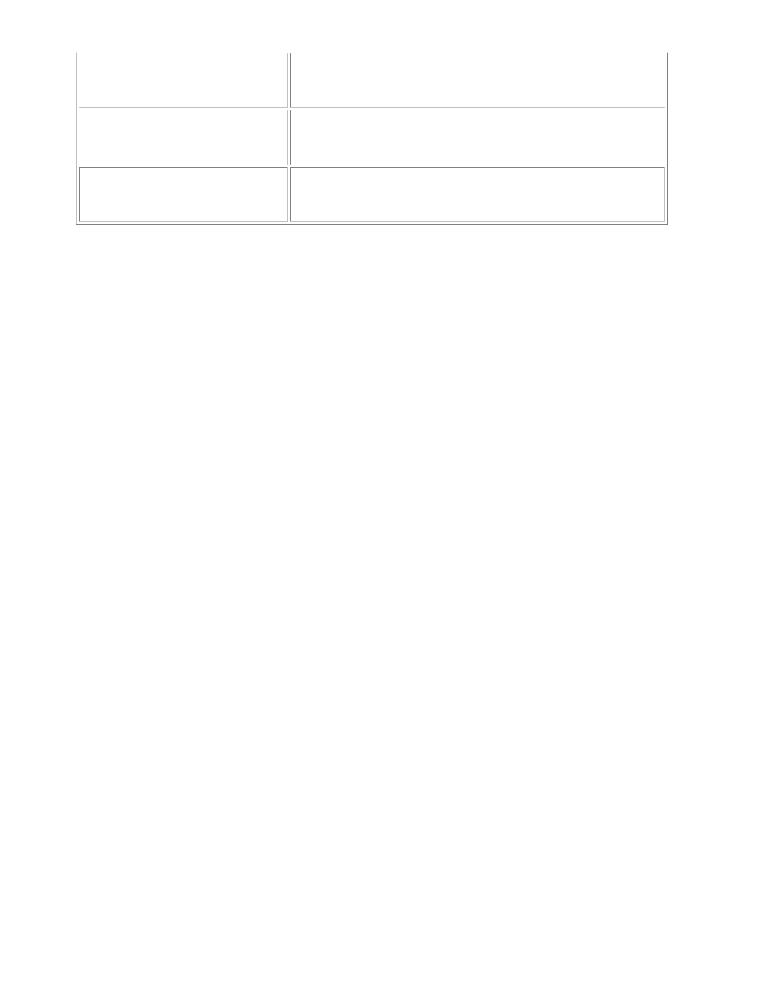
System.Web C# and the .NET |
|
Platform,A numberSecondof namespacesEdition are specifically geared toward the |
by Andrew Troelsen |
|
development of .NET Web applications, including ASP.NET |
|
ISBN:1590590554 |
|
|
|
and XML Web services. |
Apress © 2003 (1200 pages) |
||
|
||
This comprehensive |
|
text tarts with a brief overview of the |
System.Windows.Forms |
|
Despite the name, the .NET platform does contain |
C# language and then quickly moves to key technical and |
||
|
|
namespaces that facilitate the construction of more |
architectural issues for .NET developers. |
||
|
|
traditional main windows, dialog boxes, and custom widgets. |
The XML-centric namespaces contain numerous types that represent core XML primitives and types used to interact with
C# and the .NET Platform, Second EditionXML data.
Introduction
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Accessing a Namespace Programmatically
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
It is worth reiterating that a namespace is nothing more than a convenient way for us mere humans to
Chapter 3 - C# Language Fundamentals
logically understand and organize related types. For example, consider again the System namespace.
Chapter 4 - Object-Oriented Programming with C#
From your perspective, you can assume that System.Console represents a class named Console that is Chapcontaineder 5 -withinExceptionsa namespaceand Objecalledt LifetimeSystem. However, in the eyes of the .NET runtime, this is not so. The
Chapterruntime6engine- I terfacesonly seesandaCollectionssingle entity named System.Console.
Chapter 7 - Callback Interfaces, Delegates, and Events
As you build your custom types, you have the option of organizing your items into a custom namespace.
Chapter 8 - Advanced C# Type Construction Techniques
Again, a namespace is a logical naming scheme used by .NET languages to group related types under a
Part Three - Programming with .NET Assemblies
unique umbrella. When you group your types into a namespace, you provide a simple way to circumvent
Chapter 9 - Understanding .NET Assemblies
possible name clashes between assemblies. For example, if you were building a new Windows Forms application that references two external assemblies, and each assembly contained a type named GoCart, you would be able to specify which GoCart class you are interested in by appending the type name to its
containing namespace (i.e., "Intertech.CustomVehicles.GoCart" not "SlowVehicles.GoCart").
Chapter 12 - Object Serialization and the .NET Remoting Layer
ChapterIn C#, the13 -"using"Buildingkeyworda BettersimplifiesWindow (Introducingthe processWindowsof declaringForms)types defined in a particular namespace.
ChapterHere is14how- AitBetterworks.PaintingLet's sayFrameworkyou are interested(GDI+) in building a traditional desktop application. The main
Chapterwindow15renders- Programmingbar chartwithbasedWindonwssomeFormsinformationControls obtained from a back-end database and displays Chapteryour company16 - ThelogoSystemusing.IOaNamespaceBitmap type. While learning the types each namespace contains takes study
and experimentation, here are some obvious candidates to reference in your program:
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter// Here18 -areASP.NETallWebthePagesnamespacesand Web Controlsused
Chapterusing19System;- ASP.NET Web Applications
Chapterusing20System- XML Web.Drawing;Services
using System.Windows.Forms;
Index
using System.Data;
List of Figures
using System.Data.OleDb;
List of Tables
to build this application.
//General base class library types.
//Rendering types.
//GUI widget types.
//General data centric types.
//OLE DB data access types.
Once you have referenced some number of namespaces (and set a reference to the associated external assembly), you are free to create instances of the types they contain. For example, if you are interested in creating an instance of the Bitmap class (defined in the System.Drawing namespace), you can write:
// Explicitly list the namespaces used by this file...
using System;
using System.Drawing; class MyClass
{
public void DoIt()
{
// Create a 20×20 pixel bitmap. Bitmap bm = new Bitmap(20, 20);
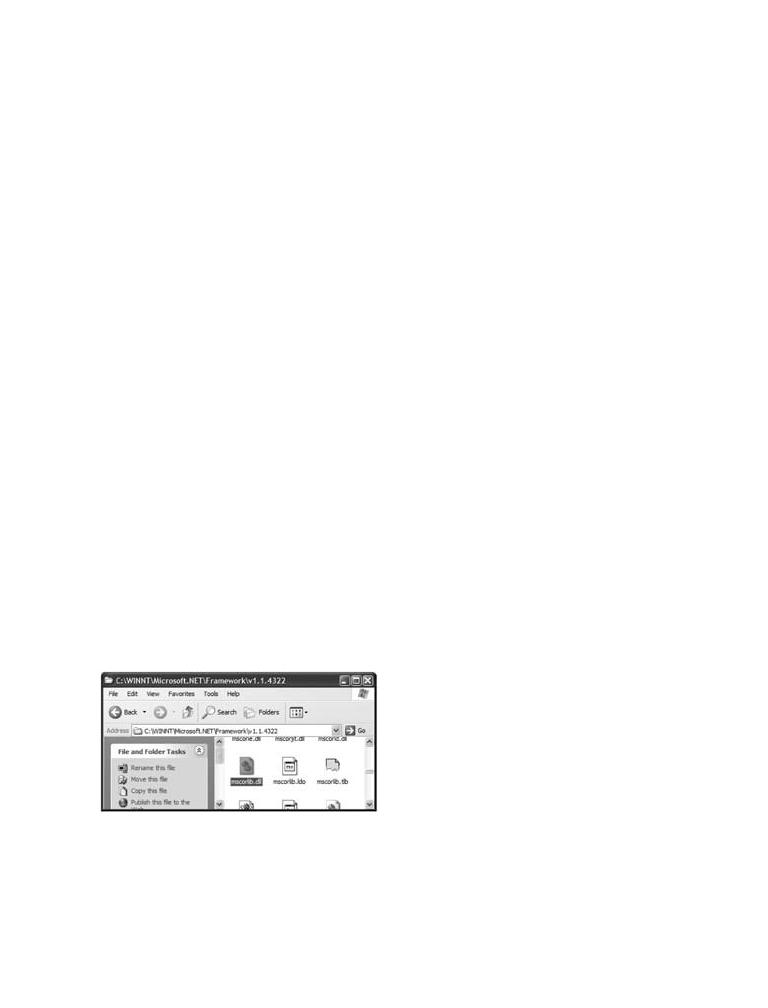
// Use the bitmap...
|
} |
C# and the .NET Platform, Second Edition |
||
} |
by Andrew Troelsen |
ISBN:1590590554 |
||
|
||||
|
Apress © 2003 (1200 pages) |
|
||
|
|
|
||
|
|
This comprehensive text starts with a brief overview of the |
||
|
|
C# language and then quickly |
oves to key technical and |
Because your application is referencing System.Drawing, the compiler is able to resolve the Bitmap class
architectural issues for .NET developers.
as a member of this namespace. If you did not directly reference System.Drawing in your application, you
would be issued a compiler error. However, you are free to declare variables using a "fully qualified name"
as well:
Table of Contents
C# and the .NET Platform, Second Edition
// Not listing System.Drawing namespace!
Introduction
using System;
Part One - Introducing C# and the .NET Platform
class MyClass
Chapter 1 - The Philosophy of .NET
{
Chapter 2 - Building C# Applications
public void DoIt()
Part Two - The C# Programming Language
{
Chapter 3 - C# Language Fundamentals
// Using fully qualified name.
Chapter 4 - Object-Oriented Programming with C#
System.Drawing.Bitmap bm = new System.Drawing.Bitmap(20, 20);
Chapter 5 - Exceptions and Object Lifetime
...
Chapter 6 - Interfaces and Collections
}
Chapter 7 - Callback Interfaces, Delegates, and Events
}
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
While defining a type using the fully qualified name provides greater readability, I think you'd agree that the
Chapter 10 - Processes, AppDomains, Contexts, and Threads
C# using keyword reduces keystrokes. In this text, I will avoid the use of fully qualified names (unless there
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
is a definite ambiguity to be resolved) and opt for the simplified approach of the C# using keyword.
Part Four - Leveraging the .NET Libraries
However, always remember that this technique is simply a shorthand notation for specifying a type's fully
Chapter 12 - Object Serialization and the .NET Remoting Layer
qualified name, and each approach results in the exact same underlying CIL.
Chapter 13 - Building a Better Window (Introducing Windows Forms) Chapter 14 - A Better Painting Framework (GDI+)
Referencing External Assemblies
Chapter 15 - Programming with Windows For s Controls
Chapter 16 - The System.IO Namespace
In addition to specifying a namespace via the C# using keyword, you also need to tell the C# compiler the name of the assembly containing the actual CIL definition for the referenced type. As mentioned, many core .NET namespaces live within mscorlib.dll. System.Drawing however, is contained in a separate binary
named System.Drawing.dll. By default, the system-supplied assemblies are located under <drive>: \
Chapter 19 - ASP.NET Web Applications
%windir%\ Microsoft.NET\ Framework\ <version>, as seen in Figure 1-4.
Chapter 20 - XML Web Services
Index
List of
List of
Figure 1-4: The base class libraries
Depending on the development tool you are using to build your .NET types, you will have various ways to inform the compiler which assemblies you wish to include during the compilation cycle. You examine how to do so in the next chapter, so I'll hold off on the details for now.
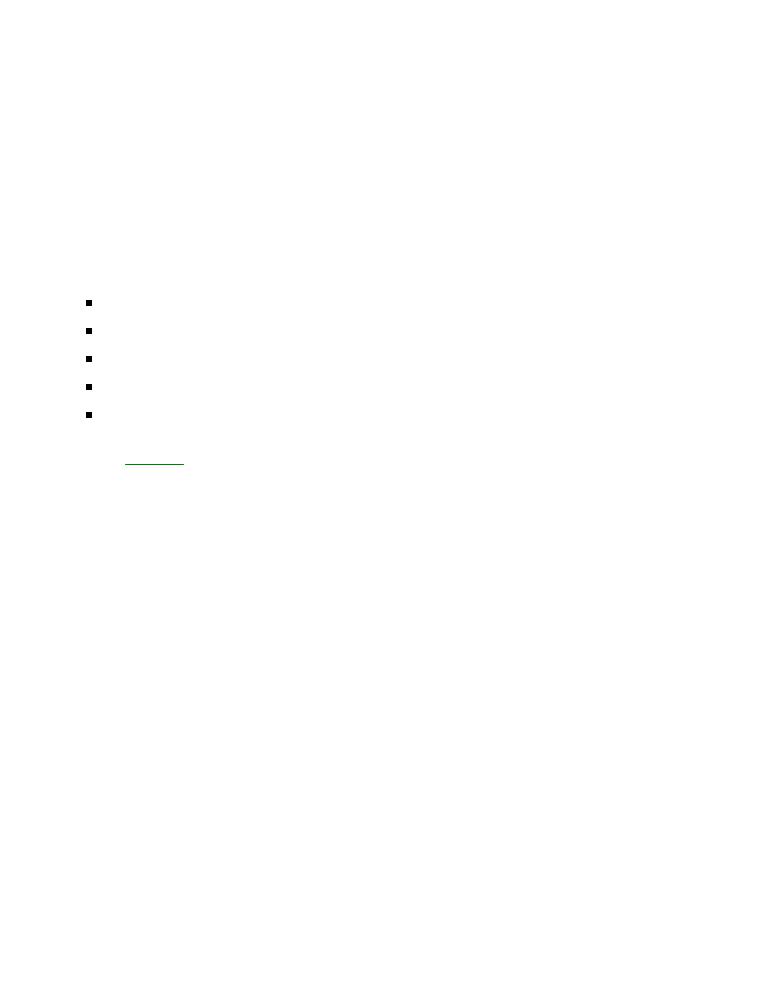
Increasing C#Yourand theNamespace.NET Platform,NomenclatureSecond Edition
by Andrew Troelsen |
ISBN:1590590554 |
If you are beginning to feel a tad overwhelmed at the thought of gaining mastery over every nuance of the
Apress © 2003 (1200 pages)
.NET universe, just remember that what makes a namespace unique is that the types it defines are all
This comprehensive text starts with a brief overview of the somehowsemanticallyC# langurelatedge and. Therefore,th n quicklyif youmoveshavetonokeyneedtechnicfor aluserand interface beyond a simple
console application,architecturalyou can forgetissu s allforabout.NET developersthe System. .Windows.Forms and System.Drawing namespaces (among others). If you are building a painting application, the database programming namespaces are most likely of little concern. Like any new set of prefabricated code, you learn as you go.
Table of Contents
C#Overandthethechapters.NET Platform,that follow,SecondyouEditionare exposed to numerous aspects of the .NET platform and related
namespaces. As it would be impractical to detail every type contained in every namespace in a single
Introduction
book (honestly, there are many thousands of types in .NET version 1.0 and even more with the release of
Part One - Introducing C# and the .NET Platform
version 1.1), you should be aware of the following techniques that can be used to learn more about the
Chapter 1 - The Philosophy of .NET
.NET libraries:
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
.NET SDK online documentation (MSDN)
Chapter 3 - C# Language Fundamentals
ChapterThe4 ildasm- Object.exe-Orientedutility Programming with C#
Chapter 5 - Exceptions and Object Lifetime
The Class Viewer Web application
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
The wincv.exe desktop application
Chapter 8 - Advanced C# Type Construction Techniques
Part ThreeThe Visual- ProgrammingStudio .NETwithintegrated.NET AssemObjectlies Browser
Chapter 9 - Understanding .NET Assemblies
I think it's safe to assume you know what to do with the supplied online Help (remember, F1 is your friend),
Chapter 10 - Processes, AppDomains, Contexts, and Threads
howeverChapter 2 points out some strategies used when navigating MSDN. In addition, it is important that
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
you understand how to work with the ildasm.exe, the Class Viewer Web application, and wincv.exe utilities,
Part Four - Leveraging the .NET Libraries
each of which is shipped with the .NET SDK (the VS .NET Object Browser is examined in Chapter 2).
Chapter 12 - Object Serialization and the .NET Remoting Layer Chapter 13 - Building a Better Window (Introducing Windows Forms) ChapterUsing14 ildasm- A Better .Paintingexe Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Recall that at one time in the evolution of .NET, CIL was simply named IL. Given this, the first tool under
Chapter 16 - The System.IO Namespace
examination in this chapter is not named cildasm.exe, but ildasm.exe. The Intermediate Language Dissasembler utility (ildasm.exe) allows you to load up any .NET assembly and investigate its contents (including the associated manifest, CIL instruction set and type metadata) using a friendly GUI. The
ildasm.exe is most likely found in <drive>:\Program Files\Microsoft Visual Studio .NET 2003\SDK\v1.1\Bin.
Chapter 19 - ASP.NET Web Applications
Once you launch this tool, proceed to the "File | Open" menu command and navigate to the assembly you
Chapter 20 - XML Web Services
wish to explore. For the time being, open up mscorlib.dll (Figure 1-5). Note the path of the opened
Index
assembly is documented in the caption of the ildasm.exe utility.
List of Figures
List of Tables

ISBN:1590590554
of the
and
Table
C# and
Part
Chapter
Chapter
Part
Chapter
Chapter
Chapter
Chapter
Chapter
Chapter
Part
Chapter
Chapter
Chapter |
Programming |
Part
Chapter
Chapter
Figure 1-5: Your new best friend, ildasm.exe
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - see,The System.IO Namespace
As you can the structure of an assembly is presented in a familiar tree view format. While exploring a Chaptergiven type,17 -noticeData Accessthat thewithmembersADO.NETfor a given type (methods, properties, nested classes, and so forth)
Paret Fiveidentified- WebbyApplicationsspecific iconand.XMLTableWeb1-5Serviceslists some of the more common iconic symbols and text dump
Chaptabbreviationsr 18 - ASP. .NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
ChTablept r 120-5:-ildasmXML Web.exeServicTreesView Icons
|
|
|
|
|
|
Index |
|
Text Dump |
|
Meaning in Life |
|
|
ildasm.exe |
|
|
||
List of Figures |
|
Abbreviation |
|
|
|
|
TreeView Icon |
|
|
|
|
List of Tables |
|
|
|
|
|
|
(dot) |
|
This icon signifies that additional information is |
||
|
|
|
|
||
|
|
|
|
|
available for a given type. Also marks the |
|
|
|
|
|
assembly manifest. |
|
|
|
|
|
|
|
|
|
[NSP] |
|
Represents a namespace. |
|
|
|
|
|
|
|
|
|
[CLS] |
|
Signifies a class type. |
|
|
|
|
|
Be aware that nested types are marked with the |
|
|
|
|
|
dollar sign notation (for example, <outer |
|
|
|
|
|
class>$<inner class>). |
|
|
|
|
|
|
|
|
|
[VCL] |
|
Represents a structure type. |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
[INT] |
|
Represents an interface type. |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
[MET] |
|
Represents a method of a given type. |

|
|
|
|
|
|
|
|
|
|
|
|
C# and |
|
the[STM].NET Platform, SecondRepresentsEdition a static method of a given type |
|
||
|
|
|
by Andrew Troelsen |
|
(note the "s" marker). |
|
||
|
|
|
ISBN:1590590554 |
|
||||
|
|
|
|
|
|
|
|
|
|
|
|
Apress © |
2003 (1200 pages) |
|
Represents a field (e.g., public data) defined by |
|
|
|
|
|
|
|
[FLD] |
|
|
|
|
|
|
This |
comprehensive text starts with |
|
brief overview of the |
|
|
|
|
|
|
|
|
|
a given type. |
|
|
|
|
C# language and then quickly moves to key technical and |
|
||||
|
|
|
|
|||||
|
|
|
architectural issues for .NET developers. |
|
||||
|
|
|
|
|
[STF] |
Represents a static field defined by a given type |
|
|
|
|
|
|
|
|
|
(again note the "s" marker). |
|
|
|
|
|
|
|
|
|
|
Table of Contents |
|
|
[PTY] |
|
Signifies a property supported by the type. |
|
||
|
|
|
|
|
|
|
|
|
C# and the .NET Platform, Second Edition |
|
|
IntroductionBeyond allowing you to explore the types (and members of a specific type) contained in a given assembly,
PartildasmOne.exe- Introducingalso allowsC#youandtotheview.NETthePlatformunderlying CIL instructions for a given member. To illustrate, locate
Chapterand double1 --TheclickPhilosophythe defaultofconstructor.NET icon for the System.IO.BinaryWriter class. This launches a
Chaseparateter 2 window,- BuildingdisplayingC# Applicationsthe CIL shown in Figure 1-6.
Part Two - The C# Programming Language
Chapter
Chapter
Chapter
Chapter
Chapter
Chapter
Part
Chapter
Chapter
Chapter |
Programming |
Part
Chapter
Chapter
Chapter 14 - A Better Painting Framework (GDI+)
Figure 1-6: Viewing the underlying CIL
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
ChapterDumping17 - DataNamespaceAccess with ADOInformation.NET to File
Part Five - Web Applications and XML Web Services
The next point of interest with regard to ildasm.exe is the very useful ability to dump the relational hierarchy
Chapter 18 - ASP.NET Web Pages and Web Controls
of an assembly into a text file. In this way, you can make hard copies of your favorite assemblies to read at
Chapter 19 - ASP.NET Web Applications
your neighborhood coffeehouse (or brew pub). To do so, select "File | Dump TreeView" and provide a
Chapter 20 - XML Web Services
name for the resulting *.txt file. As you look over the dump, notice that the identifying icons have been
Index
replacedwith their corresponding textual abbreviations (see the previous table). For example, ponder
List of Figures
Figure 1-7.
List of Tables
Figure 1-7: Dumping namespace information to file

C# and the .NET Platform, Second Edition |
|
Dumping CILbyInstructionsAndrew Troelsento File |
ISBN:1590590554 |
Apress © 2003 (1200 pages)
On a related note, you are also able to dump the CIL instructions for a given assembly to file, using the
This comprehensive text starts with a brief overview of the
"File | Dump" menu option. Once you configure your dump options, you are asked to specify a location for
C# language and then quickly moves to key technical and
the *.IL file. Assuming you have dumped the contents of mscorlib.dll to file you can view its contents using architectural issues for .NET developers.
notepad. For example, Figure 1-8 shows the CIL for a method you will come to know (and love) in Chapter 11, GetType().
Table of Contents
C# and
Part
Chapter
Chapter
Part
Chapter |
|
Chapter |
C# |
Chapter |
|
Chapter |
|
Chapter |
Events |
Chapter |
Techniques |
Part |
|
ChapterFigure9 - 1Understanding-8: Dumping CIL.NETtoAssfilemblies |
|
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Ildasm.exe has additional options that can be discovered from the supplied online Help. Although I
Part Four - Leveraging the .NET Libraries
assume you will investigate these options on your own, as I mentioned earlier, one item of interest is the
Chapter 12 - Object Serialization and the .NET Remoting Layer
"Ctrl+M" keystroke. As you recall, .NET-aware compilers emit CIL and metadata that is used by the CLR
Chapter 13 - Building a Better Window (Introducing Windows Forms) |
|||
to interact with a given type. Once you load an assembly into ildasm.exe, press Ctrl+M to view the |
|||
Chapter 14 |
- A Bett r Painting Framework (GDI+) |
||
generated type metadata. To offer a preview of things to come, Figure 1-9 shows the metadata for the |
|||
Chap er 15 |
- Progra ming with Windows Forms Controls |
||
TestApp.exe assembly you will create in Chapter 2. |
|||
Chapter 16 |
- The System.IO Namespace |
|
|
Chapter 17 |
- Data Access with ADO.NET |
Part
Chapter
Chapter
Chapter
Index
List of
List of
Figure 1-9: Viewing type metadata via ildasm.exe
Viewing Assembly Metadata
Finally, if you are interested in viewing the contents of the assembly's manifest (and I know you are), simply double click the MANIFEST icon (Figure 1-10).

Second Edition
ISBN:1590590554
with a brief overview of the
moves to key technical and
developers.
Table
C# and
IntroductionFigure 1-10: Double click here to view the assembly manifest.
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
If you have not realized it by now, ildasm.exe is in many ways the .NET equivalent of the OLE/COM Object
Chapter 2 - Building C# Applications
Viewer utility. Oleview.exe is the tool of choice to learn about classic COM servers and examine the
Part Two - The C# Programming Language
underlying IDL and registry settings behind a given binary. Ildasm.exe is the tool of choice to examine .NET
Chapter 3 - C# Language Fundamentals
assemblies, the underlying CIL and related metadata.
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
The Class Viewer Web Application
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
In addition to ildasm.exe, the Class Viewer Web application (shipped with the .NET SDK sample
Chapter 8 - Advanced C# Type Construction Techniques
applications) is yet another way to explore the .NET namespaces. Once you have configured the samples
Part Three - Programming with .NET Assemblies
provided with the .NET SDK (via the Start | All Programs | Microsoft .NET Framework SDK v1.1 | Samples
Chapter 9 - Understanding .NET Assemblies
and QuickStart Tutorials menu item), launch your browser of choice and navigate to
Chttp://localhost/quickstart/aspplus/samples/classbrowser/vb/classbrowserapter 10 - Processes, AppDomains, Contexts, and Threads .aspx
Chapter(do note11that- Typethe exactReflection,locationLateofBinding,this WebandapplicationAttribute-Basedmay changeProgrammingfuture releases of the .NET SDK).
PartThisFourenables- L veragingyou to examinethe .NETtheLibrelationshiparies of various types in a more Web-savvy manner (Figure 1-11).
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter
Chapter
Chapter
Chapter
Part
Chapter
Chapter
Chapter
Index
List of
List of
Figure 1-11: Viewing types using the ClassViewer Web application
The wincv.exe Desktop Application
The final tool to be aware of is wincv.exe (Windows Class Viewer). Again, by default this tool is installed in the same directory as ildasm.exe (most likely in <drive>:\Program Files\ Microsoft Visual Studio .NET 2003\SDK\V1.1\Bin directory). This Windows-based application allows you to browse the underlying C# type definition in the base class libraries. The use of this tool is quite simple: Type in the name of the item
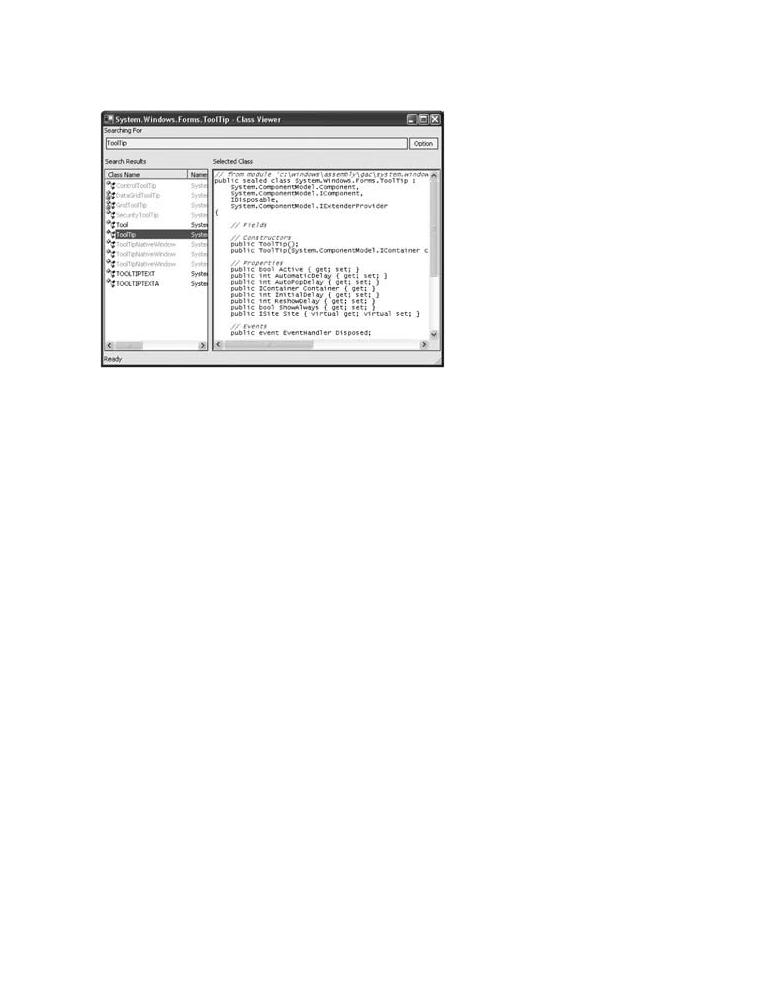
you wish to explore, and the underlying C# source code definitions are displayed on the right side. Figure
C# and the .NET Platform, Second Edition
1-12 shows the member set for the System.Windows.Forms.ToolTip class.
by Andrew Troelsen |
ISBN:1590590554 |
Apress © 2003 (1200 pages)
of the
and
Table
C# and
Part
Chapter
Chapter
Part
Chapter
Chapter
Chapter
Chapter
Figure 1-12: Working with wincv.exe
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Cool! Now that you have a number of strategies that you can use to explore the entirety of the .NET
universe, you are just about ready to examine how to build some C# applications. But first, let me
Chapter 9 - Understanding .NET Assemblies
comment on one final "minor" (read: "not-so-minor") detail.
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables

Deploying theC# and.NETthe .NETRuntimePlatform, Second Edition
by Andrew Troelsen |
ISBN:1590590554 |
As you have most likely surmised, .NET assemblies can only be loaded and executed on a machine that
Apress © 2003 (1200 pages)
has been configured to host the .NET runtime. As an individual who builds .NET software, this should
This comprehensive text starts with a brief overview of the
never be an issue,C#aslanguageyour developmentand then quicklymachinemoveswilltobekeyproperlytechnicalconfiguredand at the time you install the
.NET SDK (or VisualarchitecturalStudio .NET)issues. However,for .NET developersassume you. have just created a fantastic .NET application and wish to copy it to a brand new machine. Of course, this *.exe will fail to run if the target computer does not have .NET installed.
Table of Contents
C#Ratherand thethan.NEToptingPlatform,for overkillSecondandEditioninstalling the full .NET SDK on each and every machine that may run
your application, Microsoft has created a specific redistribution package named Dotnetfx.exe that can be
Introduction
freely shipped and installed along with your custom software. This installation program is included with the
Part One - Introducing C# and the .NET Platform
.NET SDK (or VS .NET), however, it is also freely downloadable (simply do a search on
Chapter 1 - The Philosophy of .NET
http://www.microsoft.com).
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Dotnetfx.exe will correctly configure a "virgin machine" to execute .NET assemblies, as long as said
Chapter 3 - C# Language Fundamentals
machine is one of the following flavors of Microsoft Windows (of course, future versions of the Windows
Chapter 4 - Object-Oriented Programming with C#
OS will have native .NET support):
Chapter 5 - Exceptions and Object Lifetime
ChapterMicrosoft6 - InterfacesWindowsand98Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Microsoft Windows NT 4.0 (SP 6a or greater)
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Microsoft Windows Millennium Edition (aka Windows Me)
Chapter 9 - Understanding .NET Assemblies
ChapterMicrosoft10 - Processes,WindowsAppDomains,2000 (SP2 orContgreaxter)s, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Microsoft Windows XP Home / Professional
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Once installed, the target machine will now host the necessary base class libraries, mscoree.dll, and
Chapter 13 - Building a Better Window (Introducing Windows Forms)
additional .NET infrastructure (such as the Global Assembly Cache). Do understand however, that if you
Chapter 14 - A Better Painting Framework (GDI+)
are building a Web application using .NET technologies, the end user's machine does not need to be
Chapter 15 - Programming with Windows Forms Controls
configured with the .NET redistribution kit (as the browser will simply display generic HTML).
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Running .NET on Non-Microsoft Operating Systems
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
As mentioned at various places in this chapter, the .NET platform is already being ported to other
Chapter 19 - ASP.NET Web Applications
operating systems. The key that makes this possible is yet another .NET specification termed the ChapterCommon20 Language- XML WebInfrastructureServices (CLI, which previously went under the code name "rotor"). Simply put,
IndthexCLI is the ECMA standard that describes the core libraries of the .NET universe. Microsoft has
Listreleasedof Figuresan open-source implementation of the CLI (The Shared Source CLI) which can be compiled on ListWindowsof TablesXP, FreeBSD Unix as well as the Macintosh (OS X and higher).
Note For more information regarding the Shared Source CLI, do a search for "Shared Source Common Language Infrastructure" on http://www.microsoft.com.
Although the role of this book is to confine the creation, deployment and execution of .NET applications to the Windows family of operating systems, Table 1-6 provides a few helpful links you may find interesting (understand the exact URL may change in the future).
Table 1-6: Select Links to the Platform-Agnostic Nature of .NET
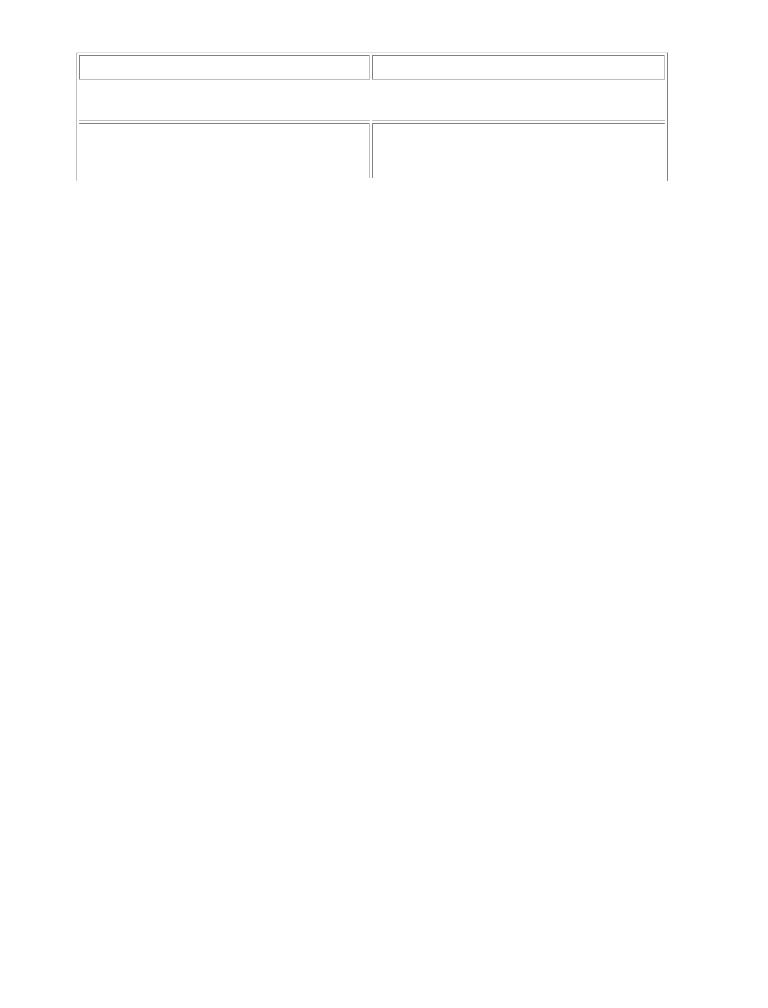
.NET-CentricC#Weband Linkthe .NET Platform, SecondMeaningEdition in Life
|
|
by Andrew Troelsen |
|
ISBN:1590590554 |
|
|
|
http://msdn.microsoft.com/net/ecma |
|
Details the standardization of C# and the .NET |
|
|
|
Apress © 2003 (1200 pages) |
|
platform |
|
|
|
This comprehensive text starts with |
|
a brief overview of the |
|
|
|
|
|
||
|
|
C# language and then quickly moves |
|
to key technical and |
|
|
|
http://www.go-mono.com |
|
Homepage for the Mono project, which is a |
|
|
|
architectural issues for .NET developers. |
|
||
|
|
|
|
porting of C# and .NET to various flavors of |
|
|
|
|
|
Linux |
|
|
|
|
|
|
|
Table of Contents |
|
|
|
||
C# and the .NET Platform, Second Edition |
|
|
|
||
Introduction |
|
|
|
Part One - Introducing C# and the .NET Platform
Chapter 1 - The Philosophy of .NET
Chapter 2 - Building C# Applications
Part Two - The C# Programming Language
Chapter 3 - C# Language Fundamentals
Chapter 4 - Object-Oriented Programming with C#
Chapter 5 - Exceptions and Object Lifetime
Chapter 6 - Interfaces and Collections
Chapter 7 - Callback Interfaces, Delegates, and Events
Chapter 8 - Advanced C# Type Construction Techniques
Part Three - Programming with .NET Assemblies
Chapter 9 - Understanding .NET Assemblies
Chapter 10 - Processes, AppDomains, Contexts, and Threads
Chapter 11 - Type Reflection, Late Binding, and Attribute-Based Programming
Part Four - Leveraging the .NET Libraries
Chapter 12 - Object Serialization and the .NET Remoting Layer
Chapter 13 - Building a Better Window (Introducing Windows Forms)
Chapter 14 - A Better Painting Framework (GDI+)
Chapter 15 - Programming with Windows Forms Controls
Chapter 16 - The System.IO Namespace
Chapter 17 - Data Access with ADO.NET
Part Five - Web Applications and XML Web Services
Chapter 18 - ASP.NET Web Pages and Web Controls
Chapter 19 - ASP.NET Web Applications
Chapter 20 - XML Web Services
Index
List of Figures
List of Tables