
- •Table of Contents
- •Objectives
- •Embedded Microcomputer Applications
- •Microcomputer and Microcontroller Architectures
- •Digital Hardware Concepts
- •Voltage, Current, and Resistance
- •Diodes
- •Transistors
- •Mechanical Switches
- •Transistor Switch ON
- •Transistor Switch OFF
- •The FET as a Logic Switch
- •NMOS Logic
- •CMOS Logic
- •Mixed MOS
- •Real Transistors Don’t Eat Q!
- •Logic Symbols
- •Tri-State Logic
- •Timing Diagrams
- •Multiplexed Bus
- •Loading and Noise Margin Analysis
- •The Design and Development Process
- •Chapter One Problems
- •Organization: von Neumann vs. Harvard
- •Microprocessor/Microcontroller Basics
- •Microcontroller CPU, Memory, and I/O
- •Design Methodology
- •The 8051 Family Microcontroller
- •Processor Architecture
- •Introduction to the 8051 Architecture
- •8051 Memory Organization
- •8051 CPU Hardware
- •Oscillator and Timing Circuitry
- •The 8051 Microcontroller Instruction Set Summary
- •Direct and Register Addressing
- •Indirect Addressing
- •Immediate Addressing
- •Generic Address Modes and Instruction Formats
- •8051 Address Modes
- •The Software Development Cycle
- •Software Development Tools
- •Hardware Development Tools
- •Chapter Two Problems
- •Timing Diagram Notation Conventions
- •Rise and Fall Times
- •Propagation Delays
- •Setup and Hold Time
- •Tri-State Bus Interfacing
- •Pulse Width and Clock Frequency
- •Fan-Out and Loading Analysis—DC and AC
- •Calculating Wiring Capacitance
- •Fan-Out When CMOS Drives LSTTL
- •Transmission Line Effects
- •Ground Bounce
- •Logic Family IC Characteristics and Interfacing
- •Interfacing TTL Compatible Signals to 5 Volt CMOS
- •Design Example: Noise Margin Analysis Spreadsheet
- •Worst-Case Timing Analysis Example
- •Chapter Three Review Problems
- •Memory Taxonomy
- •Secondary Memory
- •Sequential Access Memory
- •Direct Access Memory
- •Read/Write Memories
- •Read-Only Memory
- •Other Memory Types
- •JEDEC Memory Pin-Outs
- •Device Programmers
- •Memory Organization Considerations
- •Parametric Considerations
- •Asynchronous vs. Synchronous Memory
- •Error Detection and Correction
- •Error Sources
- •Confidence Checks
- •Memory Management
- •Cache Memory
- •Virtual Memory
- •CPU Control Lines for Memory Interfacing
- •Chapter Four Problems
- •Read and Write Operations
- •Address, Data, and Control Buses
- •Address Spaces and Decoding
- •Address Map
- •Chapter Five Problems
- •The Central Processing Unit (CPU)
- •Memory Selection and Interfacing
- •Preliminary Timing Analysis
- •External Data Memory Cycles
- •External Memory Data Memory Read
- •External Data Memory Write
- •Design Problem 1
- •Design Problem 2
- •Design Problem 3
- •Completing the Analysis
- •Chapter Six Problems
- •Introduction to Programmable Logic
- •Technologies: Fuse-Link, EPROM, EEPROM, and RAM Storage
- •PROM as PLD
- •Programmable Logic Arrays
- •PAL-Style PLDs
- •Design Examples
- •PLD Development Tools
- •Simple I/O Decoding and Interfacing Using PLDs
- •IC Design Using PCs
- •Chapter Seven Problems
- •Direct CPU I/O Interfacing
- •Port I/O for the 8051 Family
- •Output Current Limitations
- •Simple Input/Output Devices
- •Matrix Keyboard Input
- •Program-Controlled I/O Bus Interfacing
- •Real-Time Processing
- •Direct Memory Access (DMA)
- •Burst vs. Single Cycle DMA
- •Cycle Stealing
- •Elementary I/O Devices and Applications
- •Timing and Level Conversion Considerations
- •Level Conversion
- •Power Relays
- •Chapter Eight Problems
- •Interrupt Cycles
- •Software Interrupts
- •Hardware Interrupts
- •Interrupt Driven Program Elements
- •Critical Code Segments
- •Semaphores
- •Interrupt Processing Options
- •Level and Edge Triggered Interrupts
- •Vectored Interrupts
- •Non-Vectored Interrupts
- •Serial Interrupt Prioritization
- •Parallel Interrupt Prioritization
- •Construction Methods
- •Power and Ground Planes
- •Ground Problems
- •Electromagnetic Compatibility
- •Electrostatic Discharge Effects
- •Fault Tolerance
- •Hardware Development Tools
- •Instrumentation Issues
- •Software Development Tools
- •Other Specialized Design Considerations
- •Thermal Analysis and Design
- •Battery Powered System Design Considerations
- •Processor Performance Metrics
- •Benchmarks
- •Device Selection Process
- •Analog Signal Conversion
- •Special Proprietary Synchronous Serial Interfaces
- •Unconventional Use of DRAM for Low Cost Data Storage
- •Digital Signal Processing / Digital Audio Recording
- •Detailed Checklist
- •1. Define Power Supply Requirements
- •2. Verify Voltage Level Compatibility
- •3. Check DC Fan-Out: Output Current Drive vs. Loading
- •4. AC (Capacitive) Output Drive vs. Capacitive Load and De-rating
- •5. Verify Worst Case Timing Conditions
- •6. Determine if Transmission Line Termination is Required
- •7. Clock Distribution
- •8. Power and Ground Distribution
- •9. Asynchronous Inputs
- •10. Guarantee Power-On Reset State
- •11. Programmable Logic Devices
- •12. Deactivate Interrupt and Other Requests on Power-Up
- •13. Electromagnetic Compatibility Issues
- •14. Manufacturing and Test Issues
- •Books
- •Web and FTP Sites
- •Periodicals: Subscription
- •Periodicals: Advertiser Supported Trade Magazines
- •Index
8 |
|
CHAPTER EIGHT |
161 |
Basic I/O Interfaces
Ultimately computers are useless unless they are connected somehow to the outside world. This chapter emphasizes the connection of simple I/O (input/ output) devices to a microcontroller, directly and mapped into the processor’s memory or I/O address space using a bus. We’ll also discuss more advanced I/O techniques.
For embedded processors, I/O capabilities are among the most important factors to consider when selecting a CPU. Typical microcontroller ICs have on-chip bi-directional parallel ports, serial ports, and timer/counter devices. Many also have specialized I/O for driving LCDs, analog-to-digital converters, pulse- width-modulated (PWM) digital-to-analog outputs, complex pulse trains of programmable width, and timers for period and frequency measurement, etc. Some devices also incorporate special serial interfaces, intended for inter-chip connections. These types of I/O are very specific to a particular processor chip, and while they may require a lot of programming effort, they don’t require much effort in the way of hardware design. However, interfacing an I/O device to a processor data bus is a significant process that is equivalent to the memory to processor interface design, and is subject to the same timing and loading analysis.
Direct CPU I/O Interfacing
The processors I/O pins may often be connected directly to simple devices, such as key switches and LEDs. In some cases an interface circuit may be required to convert the processor’s I/O voltage and current levels to those appropriate for the I/O device. In order to understand which approach is appropriate, we’ll investigate the capabilities of the processor’s I/O pins, using the 8051 as the primary example.
162EMBEDDED CONTROLLER
Hardware Design
Our objectives in this section are to understand how the I/O port circuitry is designed, how to interpret the relevant specifications, and the capabilities limitations of the circuits. The 8051 Port 1 I/O pins will be used to illustrate the unique characteristics of the quasi bi-directional circuits. The I/O port DC specifications and absolute maximum ratings will be compared to the requirements for driving a simple LED circuit. In addition, the I/O voltage specifications will be explained and we’ll examine related protective circuits.
The characteristics of an external device must be considered in both the hardware and software design. For instance, mechanical switches used for manual input to microcontroller-based designs are prone to contact bounce, which causes the connection to open and close several times within a few milliseconds. The programmer must ignore these bounce conditions to prevent multiple key actions.
Port I/O for the 8051 Family
The I/O ports are mapped into the SFR (special function register) address space of the 8051, using direct access to the upper half of the internal data memory, addresses 80 through FFh (h = hexadecimal). In this example, we will use Port 1 on the basic 8051 device, which is the easiest port to describe since it has no alternate functions. For example, Port 1 is mapped to internal location 90h. This port can be used for general purpose I/O. Port 1 also appears in the bit addressable space as locations 90h to 97h. Port 1’s LSB (least significant bit) is available at address 90h, and the MSB (most significant bit) is at address 97h in the bit-addressable space.
Port 1 on the standard 8051 family parts can sink a few milliamperes, however it can only source only 10 to100 microamperes. The entire port can be reset to zero by moving the value zero to location 90h by executing the instruction: MOV 90h,#0. The MSB (P1.7) could be set to logic one by setting bit number 97h executing the following instruction: SETB 97h. Bit P1.7 can be cleared to logic zero by executing the instruction CLR 97h. Likewise, a single input bit can be tested using a conditional jump instruction (such as JB 90h,address) that will jump to the address only if the LSB of Port 1 (P1.0) is high when the instruction is executed. You can easily observe this operation by using a logic probe or meter connected to pin 1 of the processor chip, which is the LSB of Port 1 (P1.0). The I/O pins will be in the logic one state after reset, but executing the CLR 90h instruction will clear P1.0. I/O pins can also be input directly
163CHAPTER EIGHT
Basic I/O Interfaces
to another bit, such as the carry bit, which is very useful when sending and receiving information by a serial bit sequence. This is a useful way to transfer data and addresses between the processor and serial I/O and memory devices.
For example, to output eight bits to Port 1 the following instructions can be used:
MOV |
90h, A ; Accumulator is output to |
port 1 |
||
MOV |
P1, A |
; |
same as above, using the |
symbolic name for port 1 |
MOV |
P1,0ffh |
; |
Output FF hex (all ones) |
to port 1 |
It is also possible to output a single bit, as shown below:
CLR |
P1.0 |
; |
The |
LSB |
of |
Port |
1 |
is |
cleared (made equal to 0, ~0 Volts) |
SETB |
P1.0 |
; |
The |
LSB |
of |
Port |
1 |
is |
set (made equal to 1, ~5 Volts) |
Likewise, eight bits can be input into the accumulator, using:
MOV |
A, P1 |
; Acc<=port 1 |
Single bit input can be accomplished from Port one bit 1 to the carry bit:
MOV |
C, P1.1 ; Carry bit is loaded with the current state of P1.1 |
An input bit can also be used to control program flow:
JB |
P1.0, address ; Jump to address if bit P1.0 is 1, otherwise continue |
Monitor commands can also be used to access the I/O pins on the SDK:
#P1 |
allows direct R/W of port 1 |
#SB 92 allows observing and set/clr of P1.2 bit
Port 1 can be accessed one bit at a time in the bit addressable address space from 90h to 97h, which correspond to each of the eight bits of port 1. The MSB (P1.7) can be accessed at bit address location 97h. The entire port can be reset to zero by moving the value zero to location 90h executing the instruction:
MOV 90h,#0
The MSB (P1.7) could be set to logic one by setting bit number 97h executing the following instruction:
SETB 97h
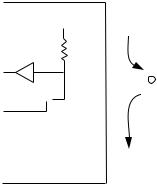
164EMBEDDED CONTROLLER
Hardware Design
Bit P1.7 can be cleared to logic zero by executing the instruction CLR 97h. Likewise, a single input bit can be tested using a conditional jump instruction, such as: JB 90h,address which will jump to the address only if the LSB of Port 1 (P1.0) is high when the instruction is executed. You can easily observe this operation by using a logic probe or meter connected to pin 1 of the processor chip, which is the LSB of Port 1 (P1.0). Normally the pin will be in the logic one state after reset, but executing the CLR 90h instruction will clear P1.0. I/O pins can also be input directly to another bit, such as the carry bit, which is very useful when sending and receiving information by a serial bit sequence. This is exactly how the data and addresses are sent and received between the processor and serial I/O and memory devices.
It’s important to recognize that some instructions modify the output latch, rather than the input pin. This applies to instructions that read-modify-write the output pins, such as ANDing the port with a constant value to mask certain bits. This is necessary because the I/O pins can serve as input or output. Pins which are to be used as inputs must be written with a logic one output first, so that an external device such as a switch to ground, can pull the line low.
If the pins were used directly, then a pin that was being used as an input but just happened to be low at the time that the logical AND operation was carried out, would become stuck low! By performing the logical AND with the output register instead, the state of the input pin will not be affected.
The internal circuits for the I/O pin are shown in simplified form in the figure. The 8051 uses a modified open-drain output structure, which allows it to oper-
ate as either input or output, or even both at the same time. It consists of a constant |
|||||||||||
current pull-up (current source), an |
|
|
|
|
|
|
|
|
|
|
|
N-channel MOSFET switch as a pull- |
|
|
|
+V |
|
||||||
down device (FET sinks current). |
|
|
|
|
|||||||
|
|
|
|
|
|
|
|
|
|
Resistor |
|
The FET is an active switch, so it can |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
IOH Sources |
|||
sink more current. That is why the |
In |
Current |
|||||||||
|
|||||||||||
8051’s sink current is large compared |
|
|
|
|
|
|
|
|
Port |
|
|
|
|
|
|
|
|
|
|
Pin |
|
||
to source. |
|
|
|
|
|
|
|
|
|
||
Out |
|
|
|
Output |
|||||||
|
|
|
|
|
|
|
|
|
|
|
|
The simplified I/O port circuit diagram |
|
|
|
|
|
|
|
|
IOL |
Sinks |
|
|
|
|
|
|
|
|
|
||||
NMOS FET |
|
|
|
|
|
Current |
|||||
in Figure 8-1 shows a pull-up resistor |
|
|
|
||||||||
|
|
|
|
|
|
|
|
|
|
|
|
providing a weak current source, and a |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
FET pull-down capable of sinking more |
|
|
|
|
|
|
|
|
|
|
|
current. The input pin can also be read |
Figure 8-1: Simplified I/O port circuit. |
|
|||||||||
|
|
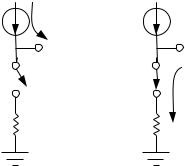
165CHAPTER EIGHT
Basic I/O Interfaces
by the input buffer. This allows the pin to be used as either input or output. When using the pin as an input, the FET must be turned off by writing a one to the output pin. Then an external device, such as a switch connected between the pin and ground, will pull the input low when the switch is closed. When using one of these pins as an input, an external pull-up is usually not required, as the pin is pulled up internally.
While the simplified representation approximates the behavior of the circuit, in order to thoroughly understand how it behaves, we must go deeper. The diagram in Figure 8-2 shows a somewhat more accurate version of the circuit,
which is referred to as a “quasi-
+V
bi-directional” circuit. The pull-up is actually a current source, which
can source one of two currents. When the output is static in the
high state, the current source provides about 50 microamperes of current to an external load.
When the output pin transitions
from one to zero, the FET switches on, sinking the source current and
the current from any output load to
ground. The switch is not perfect, and has some resistance, which causes the output voltage to rise somewhat above ground. If the current source is a resistor, then the low-to-high output voltage transition would be very slow, due to the R-C time constant formed by the resistor and the load capacitance. Even with a small constant current source, the output voltage will ramp up slowly. The current source in the 8051 behaves differently on a zero-to-one transition. When the output pin transitions from zero to one, the current source provides a much higher current for a very short time, pulling the output voltage up quickly. Then the current source reverts to its lower value. This unique feature of the output addresses the slow rise time problem by lowering the time constant during the zero-to-one transition, without requiring an external input device to sink more than 50 microamperes. A secondary benefit is that the pin circuitry does not have to be explicitly programmed as an input or output, as is the case with all other microcontroller families. This also means that the pin can be used alternately for input and output, like an open-collector or opendrain bus without concern for bus contention. This is useful for things like