
Introduction to 3D Game Programming with DirectX.9.0 - F. D. Luna
.pdf


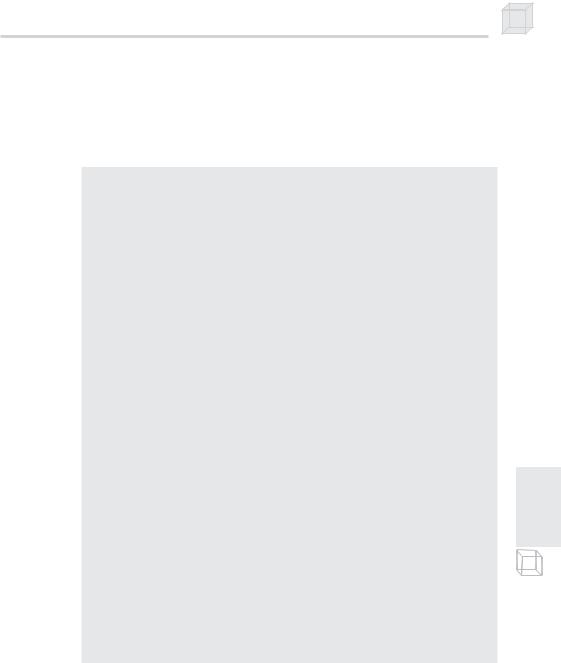


Building a Flexible Camera Class 209
void Camera::fly(float units)
{
if( _cameraType == AIRCRAFT ) _pos += _up * units;
}
12.3 Sample Application: Camera
This chapter’s sample program creates and renders the scene shown in Figure 12.8. You are free to fly around this scene using input from the keyboard. The following keys are implemented:
W/S—Walk forward/backward
A/D—Strafe left/right
R/F—Fly up/down
Up/Down arrow keys—Pitch
Left/Right arrow keys—Yaw
N/M—Roll
P a r t I I I
Figure 12.8: A screen shot of the camera sample program for this chapter
The implementation of the sample is trivial, since all the work is inside the Camera class, which we have already discussed. We handle user input in the Display function accordingly. Keep in mind that we have instantiated the camera object TheCamera at the global scope. Also notice that we move the camera with respect to the time change (timeDelta); this keeps us moving at a steady speed independent of the frame rate.
bool Display(float timeDelta)
{
if( Device )
{


Building a Flexible Camera Class 211
Device->EndScene(); Device->Present(0, 0, 0, 0);
}
return true;
}
Note: We have updated the d3d namespace with a new function called DrawBasicScene. The function draws the scene in Figure 12.8. We have added it to the d3d namespace because it is convenient to have one function that sets up a basic scene so that in later samples we can concentrate on the code that the sample illustrates rather than irrelevant drawing code. Its declaration in d3dUtility.h is as follows:
//Function references "desert.bmp" internally. This file must
//be in the working directory.
bool DrawBasicScene( |
|
|
IDirect3DDevice9* device, |
// Pass in |
0 for cleanup. |
float scale); |
// uniform |
scale |
The first time the function is called with a valid device pointer it sets up the geometry internally; therefore it is recommended that you call this function first in the Setup function. To clean up the internal geometry, call the function in the Cleanup routine, but pass null for the device pointer. Since this function doesn’t implement anything that we haven’t seen, we leave it to you to examine the code, which can be found in this chapter’s folder in the companion files. Make a note that the function loads the image desert.bmp as a texture. This file can be found in this sample’s folder.
12.4 Summary
|
|
III |
|
We describe the position and orientation of our camera in the world |
|||
coordinate system by maintaining four vectors: a right vector, an up |
t |
||
vector, a look vector, and a position vector. With this description, |
ar |
||
P |
|||
|
|
||
we can easily implement a camera with six degrees of freedom, |
|
||
giving a flexible camera interface that works well for flight simula- |
|
||
tors and games played from the first-person perspective. |
|

Chapter 13
Basic Terrain
Rendering
A terrain mesh is really nothing more than a triangle grid, as Figure 13.1.a shows, but with the heights of each vertex in the grid specified in such a way that the grid models a smooth transition from mountain to valley, simulating a natural terrain (Figure 13.1.b). And of course, we apply a nice texture showing sandy beaches, grassy hills, and snowy mountains (Figure 13.1.c).
Figure 13.1: (a) A triangle grid. (b) A triangle grid with smooth height transitions. (c) A lit and textured terrain that is a screen shot from the sample we create in this chapter.
This chapter walks you through implementing the Terrain class. This class uses a brute force approach. By that we mean it simply stores the entire terrain vertex/index data and then renders it. For games requiring a small terrain, this approach is workable with modern graphics cards that support hardware vertex processing. However, for games requiring larger terrains, you have to do some kind of level of detail or culling because the enormous amount of geometry data needed to model such huge terrains is overwhelming for a brute force approach.
212
