
- •Seagate Crystal Web Reports Server Overview
- •What is the Web Reports Server?
- •Who should use the Web Reports Server?
- •Web Reports Server Features
- •New Features in Version 7
- •The Web Reports Server vs. Active Server Pages
- •Sample Web Sites
- •Implementing the Web Reports Server
- •Choosing a Web Reports Server
- •System Requirements
- •Installing the Web Reports Server
- •Confirming Correct Installation
- •Virtual Directories
- •Creating a Web Site
- •For More Information
- •Crystal Web Reports Server Administration
- •The Web Reports Server Configuration Application
- •Page Server Tab
- •Image Server Tab
- •Report Exporting Tab
- •Server Mappings Tab
- •Report Viewing Tab
- •The Page Server and the Image Server
- •Smart Navigation
- •Drilling Down on Data
- •Database Location
- •Web Reports Server Commands
- •The Crystal Web Reports Server Command Expert
- •Constructing Report Requests
- •Changing Selection Formulas in Web Reports
- •SQL and ODBC Data Sources
- •SQL Stored Procedures and Parameter Fields
- •Report Exporting
- •Refreshing Web Report Data
- •Web Reports Server Architecture
- •The Web Reports Server Extension
- •The Seagate Crystal Web Image Server
- •The Seagate Crystal Web Page Server
- •Report Processing
- •Job Manager Overview
- •Seagate Crystal Report Engine Automation Server
- •Visual InterDev Design-time ActiveX Control
- •Using an Existing Report
- •Building a Report at Runtime
- •Editing Active Server Pages
- •Customizing the Crystal Smart Viewer
- •Modifying the Report
- •Session Timeout
- •Sample Web Site
- •Crystal Smart Viewer Overview
- •Features of the Crystal Smart Viewers
- •Printing from the Crystal Smart Viewers
- •Using Crystal Smart Viewers in Applications
- •Crystal Smart Viewer for HTML
- •Limitations of HTML Reports
- •Crystal Smart Viewer for Java
- •Adding the Viewer to a Web Page
- •Crystal Smart Viewer for ActiveX
- •AuthentiCode Certification
- •Adding the Viewer to a Web Page
- •Downloading the Viewer from the Server
- •ActiveX Viewer Example
- •Introduction to the Crystal Report Engine
- •Before using the Crystal Report Engine in your application
- •Using the Crystal Report Engine
- •Crystal Report Engine API
- •Declarations for the Crystal Report Engine API (REAPI)
- •Using the Crystal Report Engine API
- •The Print-Only Link
- •The Custom-Print Link
- •Working with Parameter Values and Ranges
- •Working with section codes
- •Crystal Report Engine API variable length strings
- •Crystal Report Engine API structures
- •Working with subreports
- •Changing report formats
- •Exporting reports
- •PEExportTo Overview
- •PEExportOptions Structure
- •Considerations when using the export functions
- •Handling Preview Window Events
- •Distributing Crystal Report Engine Applications
- •Additional Sources of Information
- •Using the Crystal Report Engine API in Visual Basic
- •When to Open/Close the Crystal Report Engine
- •Embedded Quotes in Visual Basic Calls to the Crystal Report Engine
- •Passing Dates/Date Ranges in Visual Basic using the Crystal Report Engine API Calls
- •Identifying String Issues in Visual Basic Links to the Crystal Report Engine
- •Hard-coded Nulls in Visual Basic User Defined Types
- •Visual Basic Wrapper DLL
- •Crystal ActiveX Controls
- •Adding the ActiveX Control to your Project
- •Using the ActiveX Controls
- •Upgrading from the Crystal Custom Control
- •Crystal Report Engine Automation Server
- •Adding the Automation Server to your Visual Basic Project
- •Using the Automation Server in Visual Basic
- •Object Name Conflicts
- •Viewing the Crystal Report Engine Object Library
- •Handling Preview Window Events
- •Distributing the Automation Server with Visual Basic Applications
- •Sample Applications
- •Active Data Driver
- •Data Definition Files
- •Using the Active Data Driver
- •Creating Data Definition Files
- •Using ActiveX Data Sources at Design Time
- •Crystal Data Object
- •CDO vs. the Crystal Data Source Type Library
- •Using the Crystal Data Object
- •Crystal Data Object Model
- •Crystal Data Source Type Library
- •Creating a new project and class
- •Adding the type library
- •Implementing the functions
- •Passing the CRDataSource object to the Active Data Driver
- •Crystal Data Source Projects
- •Grid Controls and the Crystal Report Engine
- •Bound Report Driver and Bound Report Files
- •Crystal ActiveX Control Properties
- •Creating a Bound Report using the Crystal ActiveX Control
- •Creating a Formatted Bound Report
- •Creating a Formatted Bound Report at Runtime
- •Sample Application
- •ActiveX designers
- •The Report Designer Component vs. Seagate Crystal Reports
- •Data Access
- •No drag and drop between reports – use copy and paste
- •Conditional Formatting
- •Preview Window
- •Pictures
- •Guidelines
- •Subreports
- •The dual formula environment
- •Application Distribution
- •Installing the Report Designer Component
- •System Requirements
- •Installation
- •Using the Seagate Crystal Report Designer Component
- •Adding the Report Designer Component to a Project
- •Selecting Data
- •The Report Expert
- •Adding the Smart Viewer
- •Running the Application
- •CrystalReport1 - The Report Designer Component
- •CRViewer1 - The Smart Viewer Control
- •The Code
- •Report Packages
- •Working with data
- •ADO and OLEDB
- •Connecting to data with ADO
- •Connecting to data with RDO
- •Connecting to data with DAO
- •Data Environments
- •Data Definition Files
- •Report Templates
- •ODBC, SQL, and PC data sources
- •Report Designer Overview
- •Introduction to the Report Designer Component
- •Report Designer Architecture
- •Report Designer Object Model Programming
- •Report Designer Object Model Introduction
- •Obtaining a Report object
- •Displaying the report in the Smart Viewer
- •Setting a new data source for the report
- •Using ReadRecords
- •Passing fields in the correct order
- •Working with secure data in reports
- •Handling the Format event
- •Changing the contents of a Text object
- •Changing OLE object images
- •Working with Sections
- •Working with the ReportObjects collection
- •Working with the FieldObject object
- •Working with the SubreportObject object
- •Working with the Database and DatabaseTables objects
- •Working with the CrossTabObject object
- •Exporting a report
- •The Application object
- •Report events
- •Microsoft Access Sessions
- •Programmatic ID
- •Report Distribution Considerations
- •Distributing reports as part of the application
- •Saving reports as external files
- •Saving data with reports
- •VCL Component Overview
- •Installation
- •Delphi 2
- •Delphi 3 & 4
- •C++ Builder 3
- •Programming Overview
- •Introduction to the Object Inspector
- •Changing Properties in the Object Inspector
- •Changing Properties at Runtime
- •Delphi Programmers introduction to the SCR Print Engine
- •Dealing with SubClass Objects
- •Consistent Code
- •Using the Retrieve method
- •Working with subreports
- •Other Guidelines
- •Programming Tips
- •Always Set ReportName First
- •Discard Saved Data
- •Verify Database
- •Connecting to SQL Servers
- •Changing Tables & Formulas
- •Changing Groups & Summary fields
- •Using the Send methods
- •Using the JobNumber property
- •TCrpeString
- •Introduction
- •TCrpeString VCL Properties
- •Using the TCrpeString
- •Using Variables with Formulas
- •Introduction
- •Examples
- •About Section Names
- •Introduction
- •Methodology
- •StrToSectionCode
- •C++ Builder 3
- •Introduction
- •Code Syntax
- •Additional Code Examples
- •Known Problems
- •Retrieving ParamFields from a Subreport
- •DialogParent and Temporary Forms
- •Technical Support

Using the Crystal Report Engine API in Visual Basic
This section provides additional information for developers working in Visual Basic. Several features of the Crystal Report Engine must be handled differently in Visual Basic than in other development environments. In addition, some of the topics here are designed to simply assist Visual Basic programmers in the design of applications using the Crystal Report Engine.
The following topics are discussed in this section.
When to Open/Close the Crystal Report Engine, Page 104
Embedded Quotes in Visual Basic Calls to the Crystal Report Engine, Page 104
Passing Dates/Date Ranges in Visual Basic using the Crystal Report Engine API Calls, Page 105
Identifying String Issues in Visual Basic Links to the Crystal Report Engine, Page 106
Hard-coded Nulls in Visual Basic User Defined Types, Page 107
Visual Basic Wrapper DLL, Page 107
When to Open/Close the Crystal Report Engine
In a Visual Basic application, you can either open the Crystal Report Engine when you open your application or when you open a form. As a general rule, it is always best to open the Crystal Report Engine when you open the application and close it when you close the application. Here is why:
●When you open and close a form, the Crystal Report Engine opens every time you open the form and closes every time you close the form. If you print a report, close the form, and later decide to print a report again, the application has to reopen the Crystal Report Engine when you open the form, creating a time delay while running your application.
●When you open and close the application, the Crystal Report Engine opens as you start the application, and stays open as long as the application is open. Once the Crystal Report Engine is open, you can print a report as often as you wish without the need to reopen the Crystal Report Engine every time you print.
Embedded Quotes in Visual Basic Calls to the Crystal Report Engine
When you pass a concatenated string from Visual Basic to the Crystal Report Engine (for example, for a record selection formula), it is important that the resulting string has the exact syntax that the Crystal Report Engine expects. You should pay special attention to embedded quotes in concatenated strings because they are often the source of syntax errors.
Several examples follow. The first example shows code with a common embedded quote syntax error and the last two examples show code using the correct syntax.
Visual Basic Solutions |
104 |

Incorrect syntax
VBNameVariable$ = “John”
Recselct$ = “{file.LASTNAME} = “ + VBNameVariable$
This code results in the string:
{file.LASTNAME} = John
Since John is a literal string, the Formula Checker expects to see it enclosed in quotes. Without the quotes, the syntax is incorrect.
Correct syntax
VBNameVariable$ = “John”
Recselct$ = “{file.LASTNAME} = “ + (chr$(39) + VBNameVariable + chr$(39)
This code results in the string:
{file.LASTNAME} = 'John'
This is the correct syntax for use in a Seagate Crystal Reports record selection formula. This is the syntax you would use if you were entering a selection formula directly into Seagate Crystal Reports.
VBNameVariable$ = “John” Recselct$ = “{file.Lastname} = “ (+ “'” + VBNameVariable + “'”
This code also results in the string:
{file.LASTNAME} = 'John'
Again, the correct syntax.
Passing Dates/Date Ranges in Visual Basic using the Crystal Report Engine API Calls
You may want to pass date or date range information from your Visual Basic application to the Crystal Report Engine for use in formulas, selection formulas, etc. Here is an example showing a way to do it successfully:
1Start by opening a print job and assigning the print job handle to a variable.
JobHandle% = PEOpenPrintJob (“C:\CRW\CUSTOMER.RPT”)
2Create variables that hold the year, month, and day for both the start and end of the range.
StartYear$ = 1992 StartMonth$ = 01 StartDay$ = 01 EndYear$ = 1993 EndMonth$ = 12 EndDay$ = 31
Visual Basic Solutions |
105 |

3Now build a string to pass to the record selection formula. This is done in two steps:
●First, build the starting and ending dates for the date range.
¾Assign the starting date string to the variable StrtSelect$.
¾Assign the ending date string to the variable EndSelect$.
StrtSelect$ = “{filea.STARTDATE} < Date
(“ + StartYear$ + “, “ + StartMonth$ + “, “ + StartDay$ +“)”
EndSelect$ = “{filea.ENDDATE} < Date (“ + EndYear$ + “, “ + EndMonth$ + “, “ + EndDay$ +“)”
● Second, build the selection formula using the StrtSelect$ and EndSelect$ variables.
Recselct$ = StrtSelect$ + “ AND “ + EndSelect$
4Once your formula is built, set the record selection formula for the report.
RetCode% = PESetSelectionFormula
(JobHandle%, RecSelect$)
5Finally, print the report.
RetCode% = PEStartPrintJob (JobHandle, 1)
RetCode% = PEClosePrintJob (JobHandle, 1)
6Modify this code to fit your needs.
Identifying String Issues in Visual Basic Links to the Crystal Report Engine
When passing a string to the Crystal Report Engine as part of the Custom-Print Link, you may think that you are passing one thing when the program, in fact, is passing something entirely different. This can happen easily, for example, when you are passing a concatenated string that you have built using variables. A small syntax error (with embedded quotes, for example) can lead to an error message and a failed call. A simple debugging procedure follows.
To Identify a String Issue (bug)
To identify a string bug, have the program display what it is passing in a message box. To do so, put a line of code similar to the following immediately after the call in question:
MsgBox (variablename)
Visual Basic Solutions |
106 |
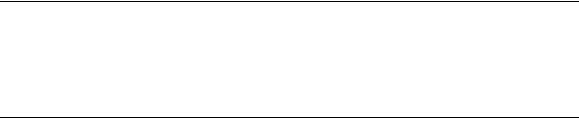
Look at the string that is displayed and make certain that it is exactly what Seagate Crystal Reports expects for
astring.
●If the syntax is incorrect, look for errors in the concatenated string you have built.
●If the syntax is correct, look for other problems that could have caused the call to fail.
●If you are not sure if the syntax is correct, write down the string from the message box, enter it in the Seagate Crystal Reports Formula Editor, and click the Check button. If there is an error in the string, the Formula Checker will identify it for you.
Hard-coded Nulls in Visual Basic User Defined Types
When you assign a string to a user defined type in Visual Basic, it is necessary to hard-code a null immediately after the string. For example:
myStruct.stringField = “Hello” + CHR$(0)
Visual Basic Wrapper DLL
Some of the features of the Crystal Report Engine API are not directly available to Visual Basic programmers, due to restrictions in the Visual Basic language, while others present technological issues that are better handled differently from what was originally designed in the Report Engine API. To avoid problems calling functions in the API, you may want to consider using the Crystal ActiveX Controls, Page 108, or the Crystal Report Engine Automation Server, Page 111. However, if you prefer to work with the API, Seagate Crystal Reports includes the Visual Basic Wrapper DLL, CRWRAP16.DLL (16-bit) and CRWRAP32.DLL (32-bit).
The Visual Basic Wrapper DLL has been designed specifically for programming in the Visual Basic environment and can be used to build Crystal Report Engine applications in Visual Basic 4.0 or later. The CRWRAP.BAS module, installed by default in the \Seagate Software\Crystal Reports directory, redefines many of the functions and structures defined in GLOBAL.BAS. When working with the Crystal Report Engine API, add both modules to your Visual Basic project.
The functions and structures defined in the Visual Basic Wrapper DLL provide an interface for handling export formats, parameter fields, SQL server and ODBC logon information, graphs, printing, and more. For complete information on each of the structures and functions included, search for Visual Basic Wrapper for the Crystal Report Engine in the Developer’s online Help. In most cases, each function or structure has a corresponding function or structure in the original Crystal Report Engine API with a similar name. When working in Visual Basic though, you must use the functions and structures provided by the Visual Basic Wrapper DLL.
Visual Basic Solutions |
107 |