
Java_J2EE_Job_Interview_Companion
.pdf

|
|
Enterprise – RMI |
161 |
||
|
|
|
|
|
|
Q 54: What is the difference between RMI and CORBA? SF |
|
|
|||
A 54: |
|
|
|
|
|
|
RMI |
|
CORBA |
|
|
|
Java only solution. The interfaces, |
|
CORBA was made specifically for interoperability among various |
|
|
|
implementations and the clients are all written |
|
languages. For example the server could be written in C++ and the |
|
|
|
in Java. |
|
business logic can be in Java and the client can be written in COBOL. |
|
|
|
|
|
|
|
|
|
RMI allows dynamic loading of classes at |
|
In a CORBA environment with multi-language support it is not possible to |
|
|
|
runtime. |
|
have dynamic loading. |
|
|
|
|
|
|
|
|
Q 55: What are the services provided by the RMI Object? SF
A 55: In addition to its remote object architecture, RMI provides some basic object services, which can be used in a distributed application. These services are
Object naming/registry service: RMI servers can provide services to clients by registering one or more remote objects with its local RMI registry.
Object activation service: It provides a way for server (i.e. remote) objects to be started on an as-needed basis. Without the remote activation service, a server object has to be registered with the RMI registry service.
Distributed garbage collection: It is an automatic process where an object, which has no further remote references, becomes a candidate for garbage collection.
Q 56: What are the differences between RMI and a socket? SF
A 56:
|
Socket |
RMI |
|
||
|
A socket is a transport mechanism. Sockets are like |
RMI uses sockets. RMI is object oriented. Methods can be |
|
||
|
applying procedural networking to object oriented |
invoked on the remote objects running on a separate JVM. |
|
||
|
environment. |
|
|
||
|
Sockets-based network programming can be laborious. |
RMI provides a convenient abstraction over raw sockets. Can |
|
||
|
|
|
|
send and receive any valid Java object utilizing underlying |
|
|
|
|
|
object serialization without having to worry about using data |
|
|
|
|
|
streams. |
|
|
|
|
|
|
|
Q 57: How will you pass parameters in RMI? |
|
|
|
|
|
SF |
|
|
|||
A 57: |
|
|
|
|
Primitive types are passed by value (e.g. int, char, boolean etc).
References to remote objects (i.e. objects which implement the Remote interface) are passed as remote references that allow the client process to invoke methods on the remote objects.
Non-remote objects are passed by value using object serialization. These objects should allow them to be serialized by implementing the java.io.Serializable interface.
Note: The client process initiates the invocation of the remote method by calling the method on the stub. The stub (client side proxy of the remote object) has a reference to the remote object and forwards the call to the skeleton (server side proxy of the remote object) through the reference manager by marshaling the method arguments. During Marshaling each object is checked to determine whether it implements java.rmi.Remote interface. If it does then the remote reference is used as the Marshaled data otherwise the object is serialized into byte streams and sent to the remote process where it is deserialized into a copy of the local object. The skeleton converts this request from the stub into the appropriate method call on the actual remote object by unmarshaling the method arguments into local stubs on the server (if they are remote reference) or into local copy (if they are sent as serialized objects).
Q 58: What is HTTP tunneling or how do you make RMI calls across firewalls? SFSE
A 58: RMI transport layer generally opens direct sockets to the server. Many Intranets have firewalls that do not allow this. To get through the firewall an RMI call can be embedded within the firewall-trusted HTTP protocol. To get across firewalls, RMI makes use of HTTP tunneling by encapsulating RMI calls within an HTTP POST request.

162 |
Enterprise – RMI |
|
|
HTTP tunnelling |
|
Proxy Server |
Web Server |
|
on port 80 |
||
|
||
HTTP encapsulated RMI call |
call forwarded by CGI script |
|
RMI Client |
RMI Server |
|
|
||
Firewall |
Firewall |
When a firewall proxy server can forward HTTP requests only to a well-known HTTP port: The firewall proxy server will forward the request to a HTTP server listening on port 80, and a CGI script will be executed to forward the call to the target RMI server port on the same machine.
|
|
HTTP tunneling |
|
||
Client |
|
|
Web |
Servlet Container |
|
applets |
I |
Firewall |
|||
|
|||||
servlets |
n |
Server |
Servlet |
||
|
|||||
JMS client |
t |
|
|
|
|
|
e |
|
|
|
|
|
r |
|
|
|
|
|
n |
|
|
|
|
|
e |
|
|
Business Service |
|
|
t |
|
|
RMI |
|
|
|
|
|
||
|
|
|
company |
EJB |
|
|
|
|
network |
Corba |
The disadvantages of HTTP tunneling are performance degradation, prevents RMI applications from using callbacks, CGI script will redirect any incoming request to any port, which is a security loophole, RMI calls cannot be multiplexed through a single connection since HTTP tunneling follows a request/response protocol etc.
Q 59: Why use RMI when we can achieve the same benefits from EJB? SF
A 59: EJBs are distributed components, which use the RMI framework for object distribution. An EJB application server provides more services like transaction management, object pooling, database connection-pooling etc, which RMI does not provide. These extra services that are provided by the EJB server simplify the programming effort at the cost of performance overhead compared to plain RMI. So if performance is important then pure RMI may be a better solution (or under extreme situations Sockets can offer better performance than RMI).
Note: The decision to go for RMI or EJB or Sockets should be based on requirements such as maintainability, ease of coding, extensibility, performance, scalability, availability of application servers, business requirements etc.
Tech Tip #5:
Q. How do you pass a parameter to your JVM?
As JVM arguments:
$> java MyProgram -DallowCache=true
alternatively in your code: |
|
System.setProperty(“allowCache”, Boolean.TRUE); |
// to set the value |
System.getProperty(“allowCache”); |
// to get the value |

Enterprise – EJB 2.x |
163 |
Enterprise – EJB 2.x
There are various persistence mechanisms available like EJB 2.x, Object-to-Relational (O/R) mapping tools like Hibernate, JDBC and EJB 3.0 (new kid on the block) etc. You will have to evaluate the products based on the application you are building because each product has its strengths and weaknesses. You will find yourself trading ease of use for scalability, standards with support for special features like stored procedures, etc. Some factors will be more important to you than for others. There is no one size fits all solution. Let’s compare some of the persistence products:
EJB 2.x |
|
EJB 3.0 |
|
Hibernate |
|
JDBC |
|
|
PROS: |
|
PROS: |
|
PROS: |
|
PROS: |
|
|
Security is provided for free |
|
A lot less artifacts than EJB |
|
Simple to write CRUD |
You have complete control |
|||
for accessing the EJB. |
|
2.x. Makes use of annotations |
|
(create, retrieve, update, |
over |
the |
persistence |
|
Provides declarative |
|
or attributes based |
|
delete) operations. |
because this is the building |
|||
|
programming. |
|
No container or application |
blocks of nearly all other |
||||
transactions. |
|
Narrows the gap between EJB |
|
persistence |
technologies in |
|||
EJBs are pooled and |
|
|
server is required and can be |
Java. |
|
|
||
|
2.x and O/R mapping. |
|
plugged into an existing |
Can call Stored Procedures. |
||||
cached. EJB life cycles are |
|
Do support OO concepts like |
|
container. |
||||
managed by the container. |
|
|
Tools are available to simplify |
Can |
manipulate relatively |
|||
|
|
inheritance. |
|
|||||
Has remote access |
|
|
|
mapping relational data to |
large data sets. |
|||
capabilities and can be |
|
|
|
objects and quick to develop. |
|
|
|
|
clustered for scalability. |
|
|
|
|
|
|
|
|
Cons: |
|
Cons: |
|
|
Cons: |
|
|
|
Cons: |
|
|
Need to understand the |
As of writing, It is still evolving. |
Little or no capabilities for |
You will have to write a lot |
||||||||
intricacies like rolling back |
remote access and |
|
of code to perform a little. |
||||||||
a transaction, granularity |
|
|
|
distributability. |
|
|
Easy to make mistakes in |
||||
etc, infrastructures like |
|
|
|
Mapping schemas can be |
properly |
managing |
|||||
session facades, business |
|
|
|
connections and can cause |
|||||||
delegates, value objects etc |
|
|
|
tedious |
and |
O/R |
mapping |
out of cursors issues. |
|||
and strategies like lazy |
|
|
|
has its tricks like using lazy |
Harder to maintain because |
||||||
loading, dirty marker etc. |
|
|
|
initialization, |
eager |
loading |
|||||
EJBs use lots of resources |
|
|
|
etc. What works for one may |
changes in schemas can |
||||||
|
|
|
not work for another. |
|
cause lot of changes to your |
||||||
and have lots of artifacts. |
|
|
|
Limited clustering |
|
code. |
|
|
|||
Does not support OO |
|
|
|
|
Records need to be locked |
||||||
|
|
|
capabilities. |
|
|
||||||
concepts like inheritance. |
|
|
|
Large data sets can still |
manually (e.g. select for |
||||||
|
|
|
|
|
update). |
|
|||||
|
|
|
|
|
cause memory issues. |
|
|
|
|||
|
|
|
|
|
Support for security at a |
|
|
|
|||
|
|
|
|
|
database level only and no |
|
|
|
|||
|
|
|
|
|
support |
for |
role |
based |
|
|
|
|
|
|
|
|
security without any add on |
|
|
|
|||
|
|
|
|
|
APIs like Aspect |
Oriented |
|
|
|
||
|
|
|
|
|
Programming etc. |
|
|
|
|
||
As a rule of thumb, suitable |
As a rule of thumb, suitable for |
Suitable for records in use |
Where |
possible |
stay away |
||||||
for distributed and |
clustered |
distributed |
and |
clustered |
between 100 and 5000. Watch |
from using JDBC unless you |
|||||
applications, which is heavily |
applications, |
which |
is heavily |
out for memory issues, when |
have compelling |
reason to |
|||||
transaction based. |
Records |
transaction based. |
Records in |
using large data sets. |
|
use it for batch jobs where |
|||||
in use say between 1 and 50. |
use say between 1 and 100. |
|
|
|
|
large amount of data need to |
|||||
|
|
|
|
|
|
|
|
|
be transferred, records in use |
||
|
|
|
|
|
|
|
|
|
greater |
than 5000, required |
|
|
|
|
|
|
|
|
|
|
to use |
Stored |
Procedures |
|
|
|
|
|
|
|
|
|
etc. |
|
|
The stateless session beans and message driven beans have wider acceptance in EJB 2.x compared to stateful session beans and entity beans. Refer Emerging Technologies/Frameworks section for Hibernate and EJB 3.0.
Q 60: What is the role of EJB 2.x in J2EE? SF
A 60: EJB 2.x (Enterprise JavaBeans) is widely adopted server side component architecture for J2EE.
EJB is a remote, distributed multi-tier system and supports protocols like JRMP, IIOP, and HTTP etc.
It enables rapid development of reusable, versatile, and portable business components (i.e. across middleware), which are transactional and scalable.

164 |
Enterprise – EJB 2.x |
EJB is a specification for J2EE servers. EJB components contain only business logic and system level programming and services like transactions, security, instance pooling, multi-threading, persistence etc are managed by the EJB Container and hence simplify the programming effort.
Message driven EJBs have support for asynchronous communication.
Note: Having said that EJB 2.x is a widely adopted server side component, EJB 3.0 is taking ease of development very seriously and has adjusted its model to offer the POJO (Plain Old Java Object) persistence and the new O/R mapping model based on Hibernate. In EJB 3.0, all kinds of enterprise beans are just POJOs. EJB 3.0 extensively uses Java annotations, which replaces excessive XML based configuration files and eliminates the need for the rigid component model used in EJB 1.x, 2.x. Annotations can be used to define the bean’s business interface, O/R mapping information, resource references etc. Refer Q18 in Emerging Technologies/Frameworks section. So, for future developments look out for EJB 3.0 and/or Hibernate framework. Refer Q14 – Q16 in Emerging Technologies/Frameworks section for discussion on Hibernate framework.
EJB - Big Picture
Other J2EE |
|
C++ application |
|
Messaging |
|
Java Applet, |
|
|
HTTP Client |
|||||
Systems |
|
|
|
|
|
|||||||||
|
|
|
Client |
|
Java stand-alone application |
|
(eg: Browser, Wireless etc) |
|||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
HTTP |
|
|
Web Services |
|
|
|
|
|
|
|
|
||||||
|
|
|
|
|
|
|
|
|
|
|||||
(SOAP, UDDI, WSDL, ebXML) |
|
|
|
|
|
|
|
|
|
|
Firewall |
|
|
|
J2EE Server |
|
|
JSP |
|
Servlets |
messaging |
|
(use JavaBeans) |
|
|
|
|
||
(use JavaBeans) |
IIOP |
RMI/IIOP |
Servlets |
|
|
Business Delegate |
|
|
|
|
(use JavaBeans) |
|
(use JavaBeans) |
|
EJB Container (Enterprise Java Beans are deployed) |
|
|
SystemLevel Services like transaction, Security etc are provided by the container |
|
EJB Session Bean |
EJB Message Driven Bean |
EJB Session Bean |
||
EJB Entity Bean |
EJB Session Bean |
EJB Session Bean |
||
BusinessprovidedLogicby the developerthroughEJB |
|
|||
|
|
|
||
SQL |
SQL (fast Lane Reader) |
|
Web Services |
|
|
Connectors (JCA) |
|
|
|
|
(SOAP, UDDI, WSDL, ebXML) |
|||
|
proprietary protocol |
|
|
|
|
Legacy System, |
Message Oriented |
Other J2EE |
|
|
Systems |
|
||
Database |
ERP System etc |
Middleware Topic |
|
|
|
|
|
|
Q 61: What is the difference between EJB and JavaBeans? SF FAQ
A 61: Both EJBs and JavaBeans have very similar names but this is where the similarities end.
JavaBeans |
Enterprise JavaBeans (EJB) |
The components built based on JavaBeans live in a single |
The Enterprise JavaBeans are non-visual distributable |
local JVM (i.e. address space) and can be either visual |
components, which can live across multiple JVMs (i.e. address |
(e.g. GUI components like Button, List etc) or non-visual at |
spaces). |
runtime. |
|
No explicit support exists for services like transactions etc. |
EJBs can be transactional and the EJB servers provide |
|
transactional support. |
JavaBeans are fine-grained components, which can be |
EJBs are coarse-grained components that can be deployed as |
used to assemble coarse-grained components or an |
is or assembled with other components into larger |
application. |
applications. EJBs must be deployed in a container that |
|
provides services like instance pooling, multi-threading, |
|
security, life-cycle management, transactions etc |
Must conform to JavaBeans specification. |
Must conform to EJB specification. |
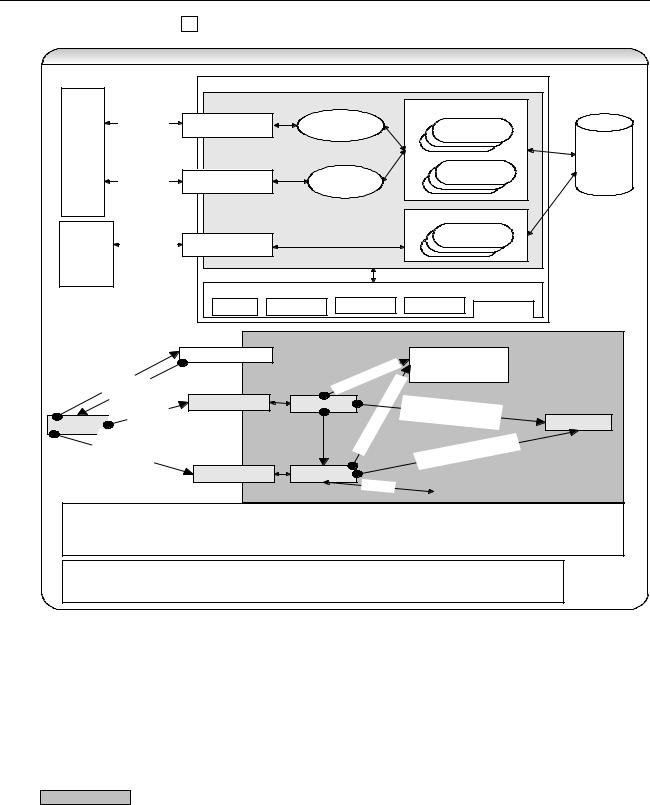



168 |
Enterprise – EJB 2.x |
Container-managed persistence (CMP) - The container is responsible for saving the bean’s state with the help of object-relational mapping tools.
Bean-managed persistence (BMP) – The entity bean is responsible for saving its own state.
If entity beans performance is of concern then there are other persistence technologies and frameworks like JDBC, JDO, Hibernate, OJB and Oracle TopLink (commercial product).
Q 63: What are the different kinds of enterprise beans? SF FAQ
A 63:
Session Bean: is a non-persistent object that implements some business logic running on the server. Session beans do not survive system shut down. There are two types of session beans
Stateless session beans (i.e. each session bean can be reused by multiple EJB clients).
Stateful session beans (i.e. each session bean is associated with one EJB client).
Entity Bean: is a persistent object that represents object views of the data, usually a row in a database. They have the primary key as a unique identifier. Multiple EJB clients can share each entity bean. Entity beans can survive system shutdowns. Entity beans can have two types of persistence
Container-Managed Persistence (CMP) - The container is responsible for saving the bean’s state.
Bean-Managed Persistence (BMP) – The entity bean is responsible for saving its own state.
Message-driven Bean: is integrated with the Java Message Service (JMS) to provide the ability to act as a message consumer and perform asynchronous processing between the server and the message producer.
Q 64: What is the difference between session and entity beans? SF
A 64:
|
Session Beans |
Entity Beans |
|
|||||
|
Use session beans for application logic. |
Use entity beans to develop persistent object model. |
||||||
|
Expect little reuse of session beans. |
Insist on reuse of entity beans. |
||||||
|
Session beans control the workflow and transactions of a |
Domain objects with a unique identity (i.e.-primary key) shared |
||||||
|
group of entity beans. |
by multiple clients. |
||||||
|
Life is limited to the life of a particular client. Handle |
Persist across multiple invocations. Handles database access |
||||||
|
database access for a particular client. |
for multiple clients. |
||||||
|
Do not survive system shut downs or server crashes. |
Do survive system shut downs or server crashes. |
||||||
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|||
Q 65: What is the difference between stateful and stateless session beans? |
|
|
|
|
||||
FAQ |
||||||||
SF |
|
|
||||||
A 65: |
|
|
|
|
|
|
||
|
Stateless Session Beans |
Stateful Session Bean |
|
|||||
|
Do not have an internal state. Can be reused by different |
Do have an internal state. Reused by the same client. |
||||||
|
clients. |
|
|
|
|
|
|
|
|
Need not be activated or passivated since the beans are |
Need to handle activation and passivation to conserve system |
||||||
|
pooled and reused. |
memory since one session bean object per client. |
||||||
|
|
|
|
|
|
|
|
Q 66: What is the difference between Container Managed Persistence (CMP) and Bean Managed Persistence (BMP) entity beans? SF FAQ
A 66:
|
Container Managed Persistence (CMP) |
Bean Managed Persistence (BMP) |
|
|
The container is responsible for persisting state of the bean. |
The bean is responsible for persisting its own state. |
|
|
Container needs to generate database (SQL) calls. |
The bean needs to code its own database (SQL) calls. |
|
|
The bean persistence is independent of its database (e.g. |
The bean persistence is hard coded and hence may not be |
|
|
DB2, Oracle, Sybase etc). So it is portable from one data |
portable between different databases (e.g. DB2, Oracle etc). |
|
|
source to another. |
|
|
|
|
|
|
Q 67: Can an EJB client invoke a method on a bean directly? SF
A 67: An EJB client should never access an EJB directly. Any access is done through the container. The container will intercept the client call and apply services like transaction, security etc prior to invoking the actual EJB.

Enterprise – EJB 2.x |
169 |
Q 68: How does an EJB interact with its container and what are the call-back methods in entity beans? SF A 68: EJB interacts with its container through the following mechanisms
Call-back Methods: Every EJB implements an interface (extends EnterpriseBean) which defines several methods which alert the bean to various events in its lifecycle. A container is responsible for invoking these methods. These methods notify the bean when it is about to be activated, to be persisted to the database, to end a transaction, to remove the bean from the memory, etc. For example the entity bean has the following call-back methods:
public interface javax.ejb.EntityBean {
public void setEntityContext(javax.ejb.EntityContext c); public void unsetEntityContext();
public void ejbLoad(); public void ejbStore(); public void ejbActivate(); public void ejbPassivate(); public void ejbRemove();
}
EJBContext: provides methods for interacting with the container so that the bean can request information about its environment like the identity of the caller, security, status of a transaction, obtains remote reference to itself etc. e.g. isUserInRole(), getUserPrincipal(), isRollbackOnly(), etc
JNDI (Java Naming and Directory Interface): allows EJB to access resources like JDBC connections, JMS topics and queues, other EJBs etc.
Q 69: What is the difference between EJB 1.1 and EJB 2.0? What is the difference between EJB 2.x and EJB 3.0? SF
FAQ
A 69: EJB 2.0 has the following additional advantages over the EJB 1.1
Local interfaces: These are beans that can be used locally, that means by the same Java Virtual Machine, so they do not required to be wrapped like remote beans, and arguments between those interfaces are passed directly by reference instead of by value. This improves performance.
ejbHome methods: Entity beans can declare ejbHomeXXX(…) methods that perform operations related to the EJB component but that are not specific to a bean instance. The ejbHomeXXX(…) method declared in the bean class must have a matching home method XXXX( …) in the home interface.
Message Driven Beans (MDB): is a completely new enterprise bean type, which is designed specifically to handle incoming JMS messages.
New CMP Model. It is based on a new contract called the abstract persistence schema, which will allow the container to handle the persistence automatically at runtime.
EJB Query Language (EJB QL): It is a SQL-based language that will allow the new persistence schema to implement and execute finder methods. EJB QL also used in new query methods ejbSelectXXX(…), which is similar to ejbFindXXXX(…) methods except that it is only for the bean class to use and not exposed to the client (i.e. it is not declared in the home interface)
Let’s look at some of the new features on EJB 2.1
Container-managed timer service: The timer service provides coarse-grained, transactional, time-based event notifications to enable enterprise beans to model and manage higher-level business processes.
Web Service support: EJB 2.1 adds the ability of stateless session beans to implement a Web Service endpoint via a Web Service endpoint interface.
EJB-QL: Enhanced EJB-QL includes support for aggregate functions and ordering of results.
Current EJB 2.x model is complex for a variety of reasons:
You need to create several component interfaces and implement several unnecessary call-back methods.
EJB deployment descriptors are complex and error prone.