
- •About the Authors
- •Contents at a Glance
- •Contents
- •Introduction
- •Goal of the Book
- •How to Use this Book
- •Introduction to the .NET Framework
- •Common Language Runtime (CLR)
- •Class Library
- •Assembly
- •Versioning
- •Exceptions
- •Threads
- •Delegates
- •Summary
- •Introduction to C#
- •Variables
- •Initializing Variables
- •Variable Modifiers
- •Variable Data Types
- •Types of Variables
- •Variable Scope
- •Types of Data Type Casting
- •Arrays
- •Strings
- •Initializing Strings
- •Working with Strings
- •Statements and Expressions
- •Types of Statements
- •Expressions
- •Summary
- •Classes
- •Declaring Classes
- •Inheritance
- •Constructors
- •Destructors
- •Methods
- •Declaring a Method
- •Calling a Method
- •Passing Parameters to Methods
- •Method Modifiers
- •Overloading a Method
- •Namespaces
- •Declaring Namespaces
- •Aliases
- •Structs
- •Enumerations
- •Interfaces
- •Writing, Compiling, and Executing
- •Writing a C# Program
- •Compiling a C# Program
- •Executing a C# Program
- •Summary
- •Arrays
- •Single-Dimensional Arrays
- •Multidimensional Arrays
- •Methods in Arrays
- •Collections
- •Creating Collections
- •Working with Collections
- •Indexers
- •Boxing and Unboxing
- •Preprocessor Directives
- •Summary
- •Attributes
- •Declaring Attributes
- •Attribute Class
- •Attribute Parameters
- •Default Attributes
- •Properties
- •Declaring Properties
- •Accessors
- •Types of Properties
- •Summary
- •Introduction to Threads
- •Creating Threads
- •Aborting Threads
- •Joining Threads
- •Suspending Threads
- •Making Threads Sleep
- •Thread States
- •Thread Priorities
- •Synchronization
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Primary and Foreign Keys
- •Referential Integrity
- •Normalization
- •Designing a Database
- •Low-Level Design
- •Construction
- •Integration and Testing
- •User Acceptance Testing
- •Implementation
- •Operations and Maintenance
- •Summary
- •Creating a New Project
- •Console Application
- •Windows Applications
- •Creating a Windows Application for the Customer Maintenance Project
- •Creating an Interface for Form1
- •Creating an Interface for WorkerForm
- •Creating an Interface for CustomerForm
- •Creating an Interface for ReportsForm
- •Creating an Interface for JobDetailsForm
- •Summary
- •Performing Validations
- •Identifying the Validation Mechanism
- •Using the ErrorProvider Control
- •Handling Exceptions
- •Using the try and catch Statements
- •Using the Debug and Trace Classes
- •Using the Debugging Features of Visual Studio .NET
- •Using the Task List
- •Summary
- •Creating Form1
- •Connecting WorkerForm to the Workers Table
- •Connecting CustomerForm to the tblCustomer Table
- •Connecting the JobDetails Form
- •to the tblJobDetails Table
- •Summary
- •Introduction to the Crystal Reports Designer Tool
- •Creating the Reports Form
- •Creating Crystal Reports
- •Creating the Windows Forms Viewer Control
- •Creating the Monthly Worker Report
- •Summary
- •Introduction to Deploying a Windows Application
- •Deployment Projects Available in Visual Studio .NET
- •Deployment Project Editors
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Populating the TreeView Control
- •Displaying Employee Codes in the TreeView Control
- •Event Handling
- •Displaying Employee Details in the ListView Control
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Adding the Programming Logic to the Application
- •Adding Code to the Form Load() Method
- •Adding Code to the OK Button
- •Adding Code to the Exit Button
- •Summary
- •The Created Event
- •Adding Code to the Created Event
- •Overview of XML
- •The XmlReader Class
- •The XmlWriter Class
- •Displaying Data in an XML Document
- •Displaying an Error Message in the Event Log
- •Displaying Event Entries from Event Viewer
- •Displaying Data from the Summary.xml Document in a Message Box
- •Summary
- •Airline Profile
- •Role of a Business Manager
- •Role of a Network Administrator
- •Role of a Line-of-Business Executive
- •Project Requirements
- •Creation and Deletion of User Accounts
- •Addition of Flight Details
- •Reservations
- •Cancellations
- •Query of Status
- •Confirmation of Tickets
- •Creation of Reports
- •Launch of Frequent Flier Programs
- •Summarizing the Tasks
- •Project Design
- •Database Design
- •Web Forms Design
- •Enabling Security with the Directory Structure
- •Summary
- •Getting Started with ASP.NET
- •Prerequisites for ASP.NET Applications
- •New Features in ASP.NET
- •Types of ASP.NET Applications
- •Exploring ASP.NET Web Applications
- •Introducing Web Forms
- •Web Form Server Controls
- •Configuring ASP.NET Applications
- •Configuring Security for ASP.NET Applications
- •Deploying ASP.NET Applications
- •Creating a Sample ASP.NET Application
- •Creating a New Project
- •Adding Controls to the Project
- •Coding the Application
- •Summary
- •Creating the Database Schema
- •Creating Database Tables
- •Managing Primary Keys and Relationships
- •Viewing the Database Schema
- •Designing Application Forms
- •Standardizing the Interface of the Application
- •Common Forms in the Application
- •Forms for Network Administrators
- •Forms for Business Managers
- •Forms for Line-of-Business Executives
- •Summary
- •The Default.aspx Form
- •The Logoff.aspx Form
- •The ManageUsers.aspx Form
- •The ManageDatabases.aspx Form
- •The ChangePassword.aspx Form
- •Restricting Access to Web Forms
- •The AddFl.aspx Form
- •The RequestID.aspx Form
- •The Reports.aspx Form
- •The FreqFl.aspx Form
- •Coding the Forms for LOB Executives
- •The CreateRes.aspx Form
- •The CancelRes.aspx Form
- •The QueryStat.aspx Form
- •The ConfirmRes.aspx Form
- •Summary
- •Designing the Form
- •The View New Flights Option
- •The View Ticket Status Option
- •The View Flight Status Option
- •The Confirm Reservation Option
- •Testing the Application
- •Summary
- •Locating Errors in Programs
- •Watch Window
- •Locals Window
- •Call Stack Window
- •Autos Window
- •Command Window
- •Testing the Application
- •Summary
- •Managing the Databases
- •Backing Up the SkyShark Airlines Databases
- •Exporting Data from Databases
- •Examining Database Logs
- •Scheduling Database Maintenance Tasks
- •Managing Internet Information Server
- •Configuring IIS Error Pages
- •Managing Web Server Log Files
- •Summary
- •Authentication Mechanisms
- •Securing a Web Site with IIS and ASP.NET
- •Configuring IIS Authentication
- •Configuring Authentication in ASP.NET
- •Securing SQL Server
- •Summary
- •Deployment Scenarios
- •Deployment Editors
- •Creating a Deployment Project
- •Adding the Output of SkySharkDeploy to the Deployment Project
- •Deploying the Project to a Web Server on Another Computer
- •Summary
- •Organization Profile
- •Project Requirements
- •Querying for Information about All Books
- •Querying for Information about Books Based on Criteria
- •Ordering a Book on the Web Site
- •Project Design
- •Database Design
- •Database Schema
- •Web Forms Design
- •Flowcharts for the Web Forms Modules
- •Summary
- •Introduction to ASP.NET Web Services
- •Web Service Architecture
- •Working of a Web Service
- •Technologies Used in Web Services
- •XML in a Web Service
- •WSDL in a Web Service
- •SOAP in a Web Service
- •UDDI in a Web Service
- •Web Services in the .NET Framework
- •The Default Code Generated for a Web Service
- •Testing the SampleWebService Web Service
- •Summary
- •Creating the SearchAll() Web Method
- •Creating the SrchISBN() Web Method
- •Creating the AcceptDetails() Web Method
- •Creating the GenerateOrder() Web Method
- •Testing the Web Service
- •Securing a Web Service
- •Summary
- •Creating the Web Forms for the Bookers Paradise Web Site
- •Adding Code to the Web Forms
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Overview of Mobile Applications
- •The Microsoft Mobile Internet Toolkit
- •Overview of WAP
- •The WAP Architecture
- •Overview of WML
- •The Mobile Web Form
- •The Design of the MobileTimeRetriever Application
- •Creating the Interface for the Mobile Web Forms
- •Adding Code to the MobileTimeRetriever Application
- •Summary
- •Creating the Forms Required for the MobileCallStatus Application
- •Creating the frmLogon Form
- •Creating the frmSelectOption Form
- •Creating the frmPending Form
- •Creating the frmUnattended Form
- •Adding Code to the Submit Button in the frmLogon Form
- •Adding Code to the Query Button in the frmSelectOption Form
- •Adding Code to the Mark checked as complete Button in the frmPending Form
- •Adding Code to the Back Button in the frmPending Form
- •Adding Code to the Accept checked call(s) Button in the frmUnattended Form
- •Adding Code to the Back Button in the frmUnattended Form
- •Summary
- •What Is COM?
- •Windows DNA
- •Microsoft Transaction Server (MTS)
- •.NET Interoperability
- •COM Interoperability
- •Messaging
- •Benefits of Message Queues
- •Limitations
- •Key Messaging Terms
- •Summary
- •Pointers
- •Declaring Pointers
- •Types of Code
- •Implementing Pointers
- •Using Pointers with Managed Code
- •Working with Pointers
- •Compiling Unsafe Code
- •Summary
- •Introduction to the Languages of Visual Studio .NET
- •Visual C# .NET
- •Visual Basic .NET
- •Visual C++ .NET
- •Overview of Visual Basic .NET
- •Abstraction
- •Encapsulation
- •Inheritance
- •Polymorphism
- •Components of Visual Basic .NET
- •Variables
- •Constants
- •Operators
- •Arrays
- •Collections
- •Procedures
- •Arguments
- •Functions
- •Adding Code to the Submit Button
- •Adding Code to the Exit Button
- •Summary
- •Introduction to Visual Studio .NET IDE
- •Menu Bar
- •Toolbars
- •Visual Studio .NET IDE Windows
- •Toolbox
- •The Task List Window
- •Managing Windows
- •Customizing Visual Studio .NET IDE
- •The Options Dialog Box
- •The Customize Dialog Box
- •Summary
- •Index

526 Project 4 CREATING AN AIRLINE RESERVATION PORTAL
This procedure will retrieve the data stored in the fltno, origin, destination, dep-
time, fareexec, farebn, and launchdate columns of the dtFltDetails table. The
retrieved data is displayed in the DataGrid1 control. This procedure will be called from the Page_Load event of the wbFrmSkyShark.aspx page.
The View Ticket Status Option
This option will enable the customer to view the status of her ticket. To view the status,the customer needs to provide the ticket number and e-mail ID. These values are then validated against the values stored in the dtReservations table in the database. If either the ticket number or the e-mail ID provided is incorrect, then a suitable error will be displayed.
You will accept the values from the customer in the txtTicketNo and txtEMail text boxes contained on Panel3. Table 22-3 lists all the controls contained in Panel3.
Table 22-3 Controls in Panel3
Control Type |
ID |
Properties Changed |
TextBox |
txtTicketNo |
None |
TextBox |
txtEMail |
None |
Button |
btnSubmit |
Text=Submit |
|
|
|
The values entered in the text boxes are validated and the corresponding result is displayed on the click of the Submit button. The code for the Click event of the Submit button is given as follows.
private void btnSubmit_Click(object sender, System.EventArgs e)
{
string strSel; int status;
strSel = “Select email, status from dtReservations where TicketNo = @TN”; SqlCommand SelComm;
SelComm = new SqlCommand(strSel, sqlConnection1); sqlDataAdapter1.SelectCommand = SelComm; sqlDataAdapter1.SelectCommand.Parameters.Add(“@TN”, SqlDbType.Char, 10).Value
= txtTicketNo.Text ;
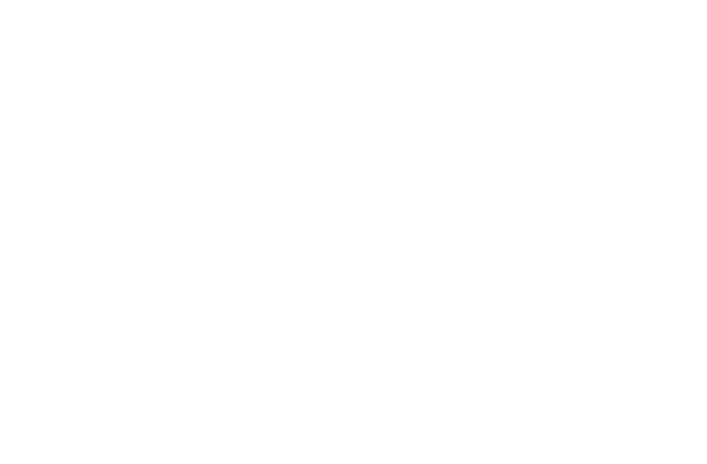
CREATING THE CUSTOMER TRANSACTION PORTAL |
Chapter 22 |
527 |
|
|
|
|
|
SqlDataReader rdrTicket; sqlConnection1.Open();
rdrTicket = sqlDataAdapter1.SelectCommand.ExecuteReader();
if( rdrTicket.Read())
{
if( rdrTicket.GetString(0).Trim() == txtEMail.Text )
{
status = rdrTicket.GetInt32(1);
}
else
{
lblStatus.ForeColor = Color.Red ; lblStatus.Text = “Incorrect EMail ID!!”; return;
}
}
else
{
lblStatus.ForeColor = Color.Red ; lblStatus.Text = “Incorrect Ticket Number!!”; return;
}
sqlConnection1.Close(); if(status >= 0)
{
lblStatus.ForeColor = Color.Blue ; lblStatus.Text = “Your ticket is confirmed”;
}
else
{
lblStatus.ForeColor = Color.Blue ;
lblStatus.Text = “Your ticket is overbooked by “ + Convert.ToString (status);
}
}

528 Project 4 CREATING AN AIRLINE RESERVATION PORTAL
The View Flight Status Option
This option will enable the customer to view the booking status of a flight. To view the status, the customer needs to provide the flight number. The code then searches for the booking status of the flight in the dtFltStatus table and displays the result. If the flight number is incorrect, then an error message is displayed.
You will accept the values from the customer in the txtFlightNo text box contained on Panel2. Table 22-4 lists all the controls contained in Panel2.
Table 22-4 Controls in Panel2
Control Type |
ID |
|
|
|
Properties Changed |
|
|
|
|
Y |
|
|
|
|
L |
||
TextBox |
txtFlightNo |
F |
None |
||
Button |
btnSubmit1 |
Text=Submit |
|||
|
|
M |
|
|
|
The booking status of the flight is displayed on the click of the Submit button. If |
|||||
|
A |
|
|
||
the value entered is incorrect, an error message is displayed. The code for the |
|||||
Click event of the SubmitEbutton is given as follows. |
|||||
|
T |
|
|
|
|
private void btnSubmit1_Click(object sender, System.EventArgs e) |
|||||
{ |
|
|
|
|
|
string strSel; |
|
|
|
|
int status;
strSel = “Select status from dtfltstatus where FltNo = @FN”; SqlCommand SelComm;
SelComm = new SqlCommand(strSel, sqlConnection1); sqlDataAdapter1.SelectCommand = SelComm; sqlDataAdapter1.SelectCommand.Parameters.Add(“@FN”,
SqlDbType.Char, 10).Value = txtFlightNo.Text ; SqlDataReader rdrTicket; sqlConnection1.Open();
rdrTicket = sqlDataAdapter1.SelectCommand.ExecuteReader(); if( rdrTicket.Read())
{
status = rdrTicket.GetInt32(0);
}
Team-Fly®

CREATING THE CUSTOMER TRANSACTION PORTAL |
Chapter 22 |
529 |
|
|
|
|
|
else
{
lblStatus.ForeColor = Color.Red ; lblStatus.Text = “Incorrect Flight Number!!”; return;
}
sqlConnection1.Close(); if(status >= 0)
{
lblStatus.ForeColor = Color.Blue ; lblStatus.Text = “Ticket is available”;
}
else
{
lblStatus.ForeColor = Color.Blue ; lblStatus.Text = “Flight is overbooked by “ +
Convert.ToString(status);
}
}
The Confirm Reservation Option
This option will enable the customer to confirm a reservation. To do so, the customer will need to provide the ticket number and the e-mail ID, which are then validated against the values stored in the dtReservations table of the database. If either of the two values is incorrect, an appropriate error message is displayed.
You will accept the values from the customer in the txtTktNo and txtEml text boxes contained on Panel4. Table 22-5 lists various controls present on Panel4.
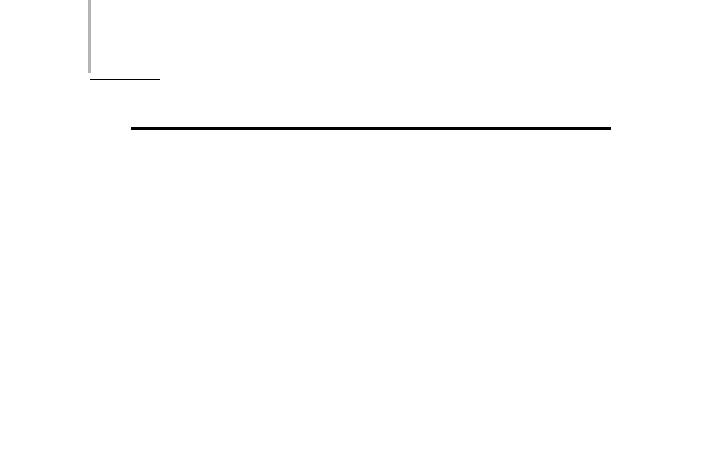
530 Project 4 CREATING AN AIRLINE RESERVATION PORTAL
Table 22-5 Controls in Panel4
Control Type |
ID |
Properties Changed |
TextBox |
txtTktNo |
None |
TextBox |
txtEml |
None |
Button |
btnSubmit2 |
Text=Submit |
|
|
|
These values are validated and the corresponding result is displayed on the click of the Submit button. The code for the Click event of the Submit button is given as follows.
private void btnSubmit2_Click(object sender, System.EventArgs e)
{
string strSel; bool status;
strSel = “Select email, ticketconfirmed from dtReservations where TicketNo = @TN”;
SqlCommand SelComm;
SelComm = new SqlCommand(strSel, sqlConnection1); sqlDataAdapter1.SelectCommand = SelComm; sqlDataAdapter1.SelectCommand.Parameters.Add(“@TN”,
SqlDbType.Char, 10).Value = txtTktNo.Text ;
SqlDataReader rdrTicket;
sqlConnection1.Open();
rdrTicket = sqlDataAdapter1.SelectCommand.ExecuteReader(); if( rdrTicket.Read())
{
if( rdrTicket.GetString(0).Trim() == txtEml.Text )
{
status = rdrTicket.GetBoolean(1);
}
else
{
lblStatus.ForeColor = Color.Red ; lblStatus.Text = “Incorrect EMail ID!!”; return;
}
}
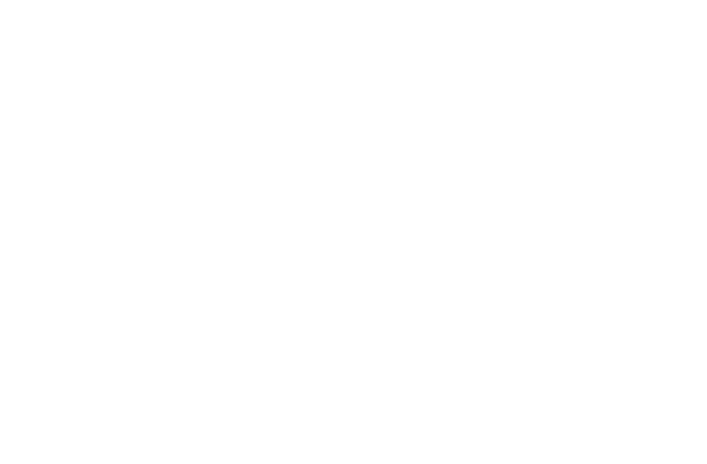
CREATING THE CUSTOMER TRANSACTION PORTAL |
Chapter 22 |
531 |
|
|
|
|
|
else
{
lblStatus.ForeColor = Color.Red ; lblStatus.Text = “Incorrect Ticket Number!!”; return;
}
sqlConnection1.Close(); if(status == true)
{
lblStatus.ForeColor = Color.Blue ;
lblStatus.Text = “Your ticket has already been confirmed!!”;
}
else
{
string UpdStr;
UpdStr= “Update dtReservations set ticketconfirmed = 1 where ticketno = @TN”;
SqlCommand UpdComm;
UpdComm = new SqlCommand(UpdStr, sqlConnection1); sqlDataAdapter1.UpdateCommand = UpdComm; sqlDataAdapter1.UpdateCommand.Parameters.Add(“@TN”,
SqlDbType.Char, 10).Value = txtTktNo.Text ; sqlConnection1.Open(); sqlDataAdapter1.UpdateCommand.ExecuteNonQuery (); sqlConnection1.Close ();
lblStatus.ForeColor = Color.Blue ;
lblStatus.Text = “Your ticket has been confirmed!!”;
}
}
This finishes the code for various options.These codes are executed after the page is loaded. Therefore, I will now list the code for the Page_Load event of the wbFrmSkyShark.aspx page.
private void Page_Load(object sender, System.EventArgs e)
{
// Put user code to initialize the page here Panel2.Style[“left”]=”222px”;
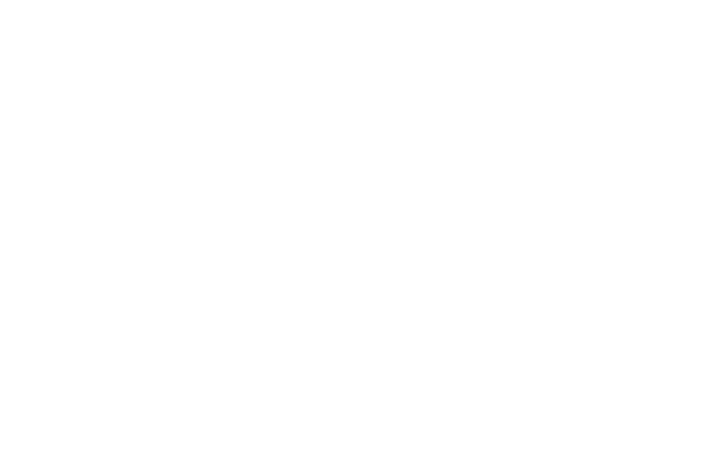
532 |
Project 4 |
CREATING AN AIRLINE RESERVATION PORTAL |
|
|
Panel2.Style[“Top”]=”152px”; |
|
|
|
|
|
Panel3.Style[“left”]=”222px”; |
|
|
Panel3.Style[“Top”]=”152px”; |
|
|
Panel4.Style[“left”]=”222px”; |
|
|
Panel4.Style[“Top”]=”152px”; |
|
|
if(Request.QueryString.Count == 0) |
|
|
{ |
|
|
return; |
|
|
} |
|
|
else |
|
|
{ |
|
|
string param; |
|
|
param = Request.QueryString.Get(0).ToString(); |
|
|
switch(param) |
|
|
{ |
case “VNF”: Display_NewFlights(); break;
case “VTS”: Panel3.Visible = true; break;
case “VFS”: Panel2.Visible = true; break;
case “CR”: Panel4.Visible = true; break;
case “H”: break; default: break;
}
}
}