
- •About the Authors
- •Contents at a Glance
- •Contents
- •Introduction
- •Goal of the Book
- •How to Use this Book
- •Introduction to the .NET Framework
- •Common Language Runtime (CLR)
- •Class Library
- •Assembly
- •Versioning
- •Exceptions
- •Threads
- •Delegates
- •Summary
- •Introduction to C#
- •Variables
- •Initializing Variables
- •Variable Modifiers
- •Variable Data Types
- •Types of Variables
- •Variable Scope
- •Types of Data Type Casting
- •Arrays
- •Strings
- •Initializing Strings
- •Working with Strings
- •Statements and Expressions
- •Types of Statements
- •Expressions
- •Summary
- •Classes
- •Declaring Classes
- •Inheritance
- •Constructors
- •Destructors
- •Methods
- •Declaring a Method
- •Calling a Method
- •Passing Parameters to Methods
- •Method Modifiers
- •Overloading a Method
- •Namespaces
- •Declaring Namespaces
- •Aliases
- •Structs
- •Enumerations
- •Interfaces
- •Writing, Compiling, and Executing
- •Writing a C# Program
- •Compiling a C# Program
- •Executing a C# Program
- •Summary
- •Arrays
- •Single-Dimensional Arrays
- •Multidimensional Arrays
- •Methods in Arrays
- •Collections
- •Creating Collections
- •Working with Collections
- •Indexers
- •Boxing and Unboxing
- •Preprocessor Directives
- •Summary
- •Attributes
- •Declaring Attributes
- •Attribute Class
- •Attribute Parameters
- •Default Attributes
- •Properties
- •Declaring Properties
- •Accessors
- •Types of Properties
- •Summary
- •Introduction to Threads
- •Creating Threads
- •Aborting Threads
- •Joining Threads
- •Suspending Threads
- •Making Threads Sleep
- •Thread States
- •Thread Priorities
- •Synchronization
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Primary and Foreign Keys
- •Referential Integrity
- •Normalization
- •Designing a Database
- •Low-Level Design
- •Construction
- •Integration and Testing
- •User Acceptance Testing
- •Implementation
- •Operations and Maintenance
- •Summary
- •Creating a New Project
- •Console Application
- •Windows Applications
- •Creating a Windows Application for the Customer Maintenance Project
- •Creating an Interface for Form1
- •Creating an Interface for WorkerForm
- •Creating an Interface for CustomerForm
- •Creating an Interface for ReportsForm
- •Creating an Interface for JobDetailsForm
- •Summary
- •Performing Validations
- •Identifying the Validation Mechanism
- •Using the ErrorProvider Control
- •Handling Exceptions
- •Using the try and catch Statements
- •Using the Debug and Trace Classes
- •Using the Debugging Features of Visual Studio .NET
- •Using the Task List
- •Summary
- •Creating Form1
- •Connecting WorkerForm to the Workers Table
- •Connecting CustomerForm to the tblCustomer Table
- •Connecting the JobDetails Form
- •to the tblJobDetails Table
- •Summary
- •Introduction to the Crystal Reports Designer Tool
- •Creating the Reports Form
- •Creating Crystal Reports
- •Creating the Windows Forms Viewer Control
- •Creating the Monthly Worker Report
- •Summary
- •Introduction to Deploying a Windows Application
- •Deployment Projects Available in Visual Studio .NET
- •Deployment Project Editors
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Populating the TreeView Control
- •Displaying Employee Codes in the TreeView Control
- •Event Handling
- •Displaying Employee Details in the ListView Control
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Adding the Programming Logic to the Application
- •Adding Code to the Form Load() Method
- •Adding Code to the OK Button
- •Adding Code to the Exit Button
- •Summary
- •The Created Event
- •Adding Code to the Created Event
- •Overview of XML
- •The XmlReader Class
- •The XmlWriter Class
- •Displaying Data in an XML Document
- •Displaying an Error Message in the Event Log
- •Displaying Event Entries from Event Viewer
- •Displaying Data from the Summary.xml Document in a Message Box
- •Summary
- •Airline Profile
- •Role of a Business Manager
- •Role of a Network Administrator
- •Role of a Line-of-Business Executive
- •Project Requirements
- •Creation and Deletion of User Accounts
- •Addition of Flight Details
- •Reservations
- •Cancellations
- •Query of Status
- •Confirmation of Tickets
- •Creation of Reports
- •Launch of Frequent Flier Programs
- •Summarizing the Tasks
- •Project Design
- •Database Design
- •Web Forms Design
- •Enabling Security with the Directory Structure
- •Summary
- •Getting Started with ASP.NET
- •Prerequisites for ASP.NET Applications
- •New Features in ASP.NET
- •Types of ASP.NET Applications
- •Exploring ASP.NET Web Applications
- •Introducing Web Forms
- •Web Form Server Controls
- •Configuring ASP.NET Applications
- •Configuring Security for ASP.NET Applications
- •Deploying ASP.NET Applications
- •Creating a Sample ASP.NET Application
- •Creating a New Project
- •Adding Controls to the Project
- •Coding the Application
- •Summary
- •Creating the Database Schema
- •Creating Database Tables
- •Managing Primary Keys and Relationships
- •Viewing the Database Schema
- •Designing Application Forms
- •Standardizing the Interface of the Application
- •Common Forms in the Application
- •Forms for Network Administrators
- •Forms for Business Managers
- •Forms for Line-of-Business Executives
- •Summary
- •The Default.aspx Form
- •The Logoff.aspx Form
- •The ManageUsers.aspx Form
- •The ManageDatabases.aspx Form
- •The ChangePassword.aspx Form
- •Restricting Access to Web Forms
- •The AddFl.aspx Form
- •The RequestID.aspx Form
- •The Reports.aspx Form
- •The FreqFl.aspx Form
- •Coding the Forms for LOB Executives
- •The CreateRes.aspx Form
- •The CancelRes.aspx Form
- •The QueryStat.aspx Form
- •The ConfirmRes.aspx Form
- •Summary
- •Designing the Form
- •The View New Flights Option
- •The View Ticket Status Option
- •The View Flight Status Option
- •The Confirm Reservation Option
- •Testing the Application
- •Summary
- •Locating Errors in Programs
- •Watch Window
- •Locals Window
- •Call Stack Window
- •Autos Window
- •Command Window
- •Testing the Application
- •Summary
- •Managing the Databases
- •Backing Up the SkyShark Airlines Databases
- •Exporting Data from Databases
- •Examining Database Logs
- •Scheduling Database Maintenance Tasks
- •Managing Internet Information Server
- •Configuring IIS Error Pages
- •Managing Web Server Log Files
- •Summary
- •Authentication Mechanisms
- •Securing a Web Site with IIS and ASP.NET
- •Configuring IIS Authentication
- •Configuring Authentication in ASP.NET
- •Securing SQL Server
- •Summary
- •Deployment Scenarios
- •Deployment Editors
- •Creating a Deployment Project
- •Adding the Output of SkySharkDeploy to the Deployment Project
- •Deploying the Project to a Web Server on Another Computer
- •Summary
- •Organization Profile
- •Project Requirements
- •Querying for Information about All Books
- •Querying for Information about Books Based on Criteria
- •Ordering a Book on the Web Site
- •Project Design
- •Database Design
- •Database Schema
- •Web Forms Design
- •Flowcharts for the Web Forms Modules
- •Summary
- •Introduction to ASP.NET Web Services
- •Web Service Architecture
- •Working of a Web Service
- •Technologies Used in Web Services
- •XML in a Web Service
- •WSDL in a Web Service
- •SOAP in a Web Service
- •UDDI in a Web Service
- •Web Services in the .NET Framework
- •The Default Code Generated for a Web Service
- •Testing the SampleWebService Web Service
- •Summary
- •Creating the SearchAll() Web Method
- •Creating the SrchISBN() Web Method
- •Creating the AcceptDetails() Web Method
- •Creating the GenerateOrder() Web Method
- •Testing the Web Service
- •Securing a Web Service
- •Summary
- •Creating the Web Forms for the Bookers Paradise Web Site
- •Adding Code to the Web Forms
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Overview of Mobile Applications
- •The Microsoft Mobile Internet Toolkit
- •Overview of WAP
- •The WAP Architecture
- •Overview of WML
- •The Mobile Web Form
- •The Design of the MobileTimeRetriever Application
- •Creating the Interface for the Mobile Web Forms
- •Adding Code to the MobileTimeRetriever Application
- •Summary
- •Creating the Forms Required for the MobileCallStatus Application
- •Creating the frmLogon Form
- •Creating the frmSelectOption Form
- •Creating the frmPending Form
- •Creating the frmUnattended Form
- •Adding Code to the Submit Button in the frmLogon Form
- •Adding Code to the Query Button in the frmSelectOption Form
- •Adding Code to the Mark checked as complete Button in the frmPending Form
- •Adding Code to the Back Button in the frmPending Form
- •Adding Code to the Accept checked call(s) Button in the frmUnattended Form
- •Adding Code to the Back Button in the frmUnattended Form
- •Summary
- •What Is COM?
- •Windows DNA
- •Microsoft Transaction Server (MTS)
- •.NET Interoperability
- •COM Interoperability
- •Messaging
- •Benefits of Message Queues
- •Limitations
- •Key Messaging Terms
- •Summary
- •Pointers
- •Declaring Pointers
- •Types of Code
- •Implementing Pointers
- •Using Pointers with Managed Code
- •Working with Pointers
- •Compiling Unsafe Code
- •Summary
- •Introduction to the Languages of Visual Studio .NET
- •Visual C# .NET
- •Visual Basic .NET
- •Visual C++ .NET
- •Overview of Visual Basic .NET
- •Abstraction
- •Encapsulation
- •Inheritance
- •Polymorphism
- •Components of Visual Basic .NET
- •Variables
- •Constants
- •Operators
- •Arrays
- •Collections
- •Procedures
- •Arguments
- •Functions
- •Adding Code to the Submit Button
- •Adding Code to the Exit Button
- •Summary
- •Introduction to Visual Studio .NET IDE
- •Menu Bar
- •Toolbars
- •Visual Studio .NET IDE Windows
- •Toolbox
- •The Task List Window
- •Managing Windows
- •Customizing Visual Studio .NET IDE
- •The Options Dialog Box
- •The Customize Dialog Box
- •Summary
- •Index
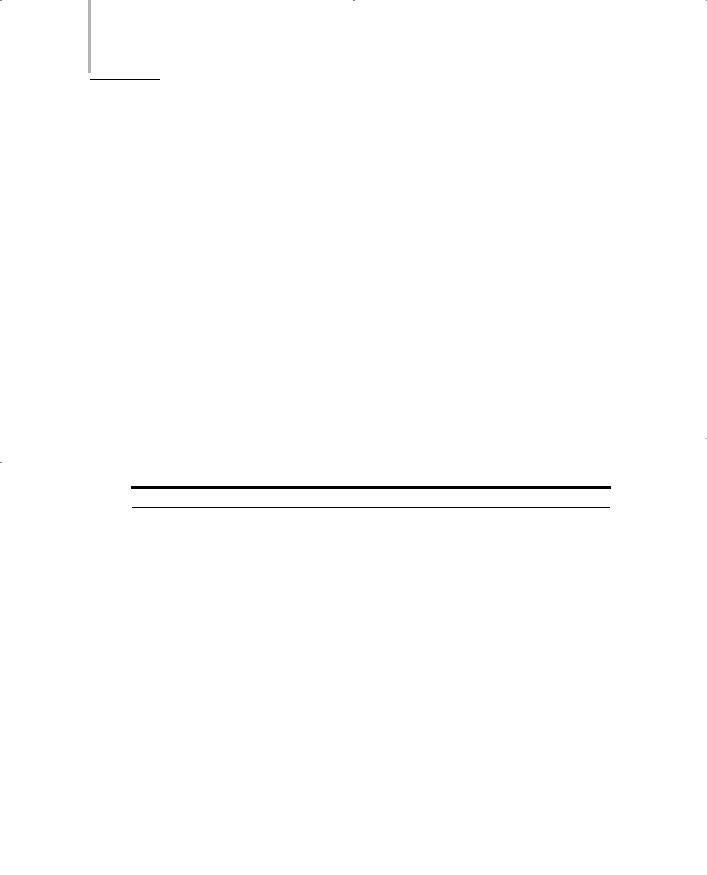
312 Project 2 CREATING THE EMPLOYEE RECORDS SYSTEM PROJECT
In this chapter, you will learn to add nodes to the tree view and items to the list view programmatically. You will also learn to read contents of an XML file. You
will build the Employee Records System application.
Populating the TreeView Control
In order to build the application, the first task you need to complete is populating the TreeView control. You have inserted a TreeView control in the form. However, the TreeView control does not contain any nodes. Now you will learn to add nodes to the control programmatically.
In order to add a node, you first need to initialize a new instance of the TreeNode class. Calling the constructor of the TreeNode class enables you to achieve this.The constructor of the TreeNode class is overloaded, as explained in Table 14-1.
Table 14-1 TreeNode Class Constructors
Constructor Description
public TreeNode(); |
Initializes a new instance of the TreeNode |
|
class |
public TreeNode(string); |
Initializes a new instance of the TreeNode |
|
class with the specified label text |
public TreeNode(string, TreeNode[]); |
Initializes a new instance of the TreeNode |
|
class with the specified label text and |
|
child tree nodes |
public TreeNode(string, int, int); |
Initializes a new instance of the TreeNode |
|
class with the specified label text and |
|
images to be displayed in selected and |
|
unselected state |
public TreeNode(string, int, int, TreeNode[]); |
Initializes a new instance of the TreeNode |
|
class with the specified label text, child |
|
tree nodes, and images to be displayed in |
|
selected and unselected state |
|
|
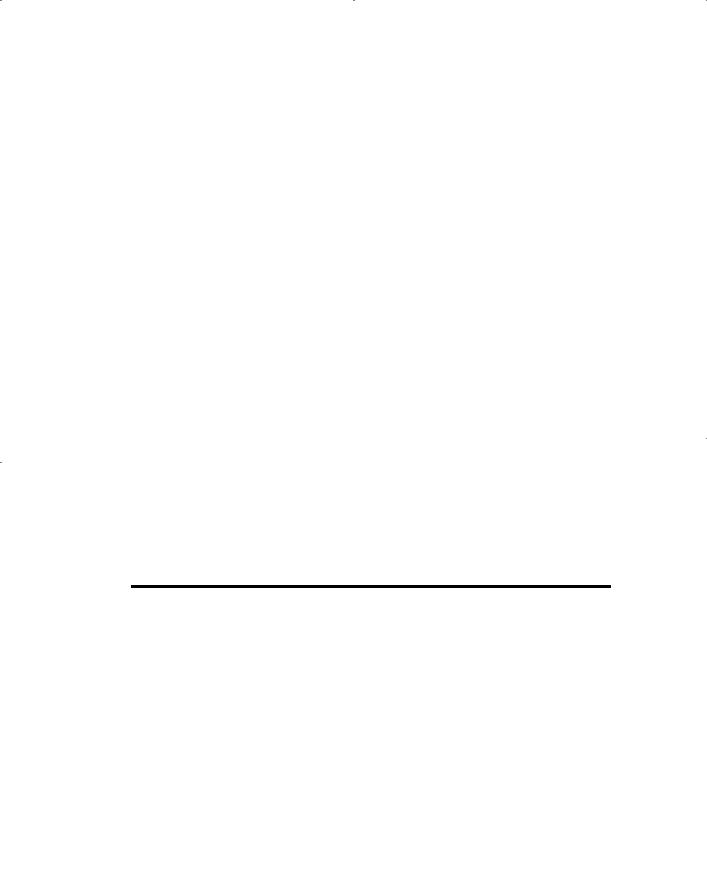
IMPLEMENTING THE BUSINESS LOGIC |
Chapter 14 |
313 |
|
|
|
|
|
Displaying Employee Codes in the TreeView Control
You can add a node to the TreeView control using the Add method, as follows:
treeview1.Nodes.Add(new TreeNode(“Root Node”)
In this code, treeview1 refers to the TreeView control. The Nodes collection contains all child nodes of a particular parent node. The Add method of the TreeNodeCollection class enables you to add nodes to the collection. For example, the following code explains the manner in which you can add a node with the display
text “My Computer”.
tnRootNode = new TreeNode(“My Computer”); tvFolderView.Nodes.Add(tnRootNode);
The previous code assumes that you have added a TreeView control to the form.
The first task that you need to accomplish is to open the XML data store and read the employee records. You will learn about XML in Chapter 17,“Interacting with a Microsoft Word Document and Event Viewer.” The .NET Framework class library provides you with the XmlTextReader class that helps you access a stream of XML data in a fast and noncached manner. I will be using the XmlTextReader class to read the EmpRec.xml file. You have created the EmpRec.xml file in Chapter 13, “Project Case Study and Design.”
The XmlTextReader represents a reader that is advanced using the read methods and properties of the current node. Table 14-2 lists some of the commonly used properties of the XmlTextReader class.
Table 14-2 Properties of the XmlTextReader Class
Properties |
Description |
EOF |
Indicates whether the reader is positioned at the end of the XML stream |
HasAttributes |
Indicates whether current node has any attributes |
HasValue |
Indicates whether current node has any value |
Item |
Obtains the value of the attribute |
LineNumber |
Gets the current line number of the reader |
LinePosition |
Gets the current position of the reader in the line specified |
Name |
Gets the name of the current node |
ReadState |
Gets the state of the reader |
Value |
Gets the text value of the current node |
|
|
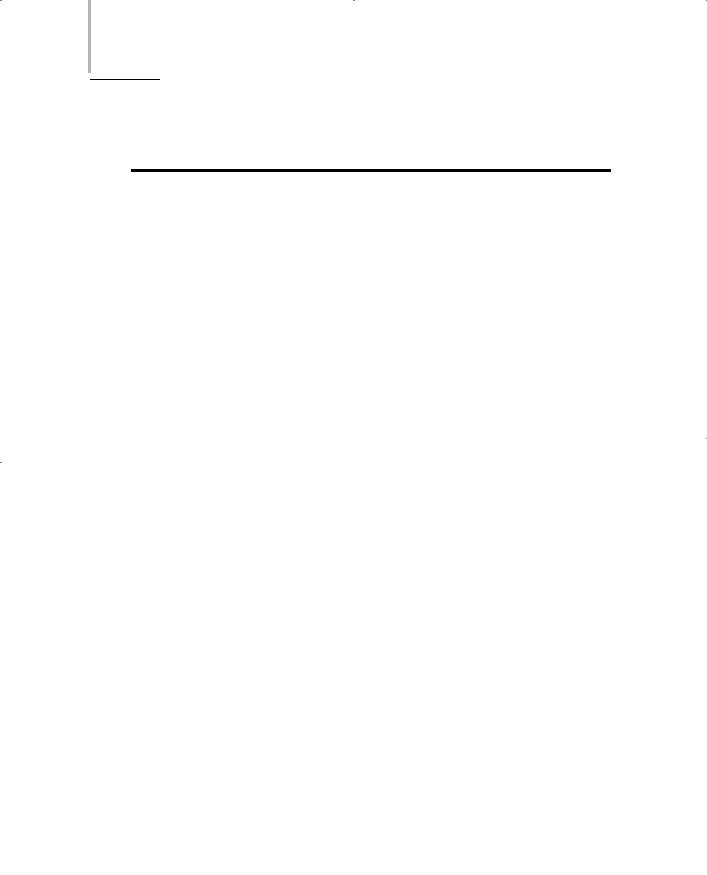
314 Project 2 CREATING THE EMPLOYEE RECORDS SYSTEM PROJECT
Table 14-3 lists some of the commonly used properties of the XmlTextReader class.
Table 14-3 Methods of the XmlTextReader Class
Methods |
Description |
Close |
Changes the ReadState to closed |
GetAttribute |
Gets the value of an attribute |
MoveToAttribute |
Moves to the specified attribute |
MoveToContent |
Checks whether the current node is a content node; if |
|
not, the reader skips to the next node |
MoveToElement |
Moves to the element that contains the current attribute |
|
node |
MoveToFirstAttribute |
Moves to the first attribute |
MoveToNextAttribute |
Moves to the next attribute |
Read |
Reads the next node fr om the stream |
ReadAttributeValue |
Parses the attribute value into one or more Text, |
|
EntityReference, or EndEntity nodes |
ReadInnerXml |
Reads all the content, including markup, as a string |
ReadOuterXml |
Reads the content, including markup, representing the |
|
current node and its child nodes |
ReadString |
Reads the contents of an element or a text node as a |
|
string |
Skip |
Skips the children of the current node |
ToString |
Returns a string that represents the current object |
|
|
Now you need to read the employee codes from the EmpRec.xml file and display them in the tree view control.
You can open the XML file by initializing the XMLTextReader class, as follows:
XmlTextReader reader= new XmlTextReader (“E:\\BookProj\\EmpRec.xml”);
where e:\BookProj\EmpRec.xml represents the path on your hard disk where the EmpRec.xml file is stored.
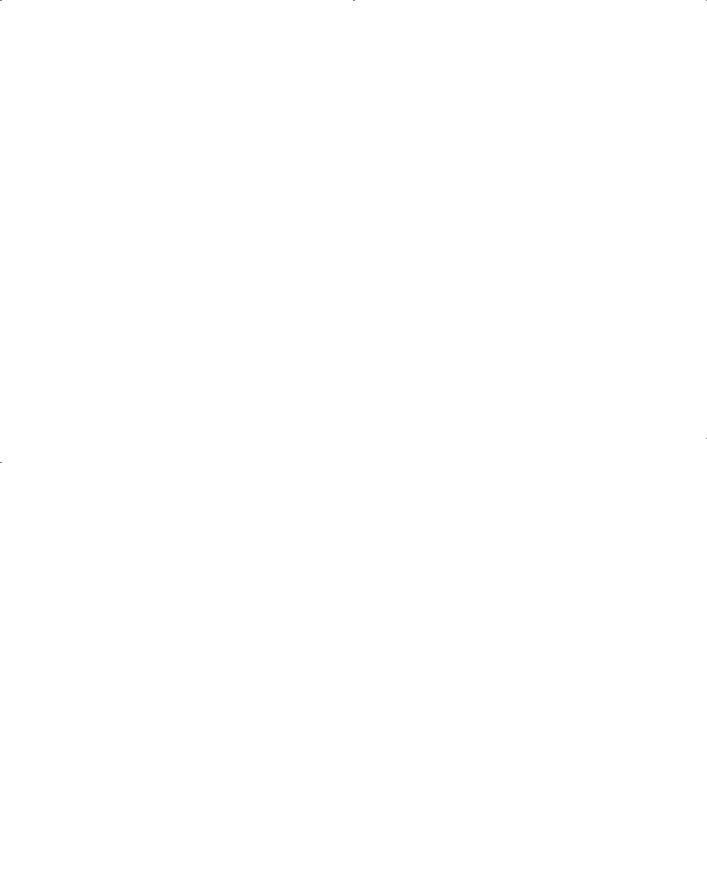
IMPLEMENTING THE BUSINESS LOGIC |
Chapter 14 |
315 |
|
|
|
|
|
I have written the entire code for populating the TreeView control inside a function, PopulateTreeView, as given below.
protected void PopulateTreeView()
{
statusBarPanel1.Text=”Refreshing Employee Codes. Please wait...”; this.Cursor = Cursors.WaitCursor;
treeView1.Nodes.Clear();
tvRootNode=new TreeNode(“Employee Records”); this.Cursor = Cursors.Default; treeView1.Nodes.Add(tvRootNode);
TreeNodeCollection nodeCollect = tvRootNode.Nodes; string strVal=””;
XmlTextReader reader= new XmlTextReader(“E:\\BookProj\\EmpRec.xml”);
reader.MoveToElement(); try
{
while(reader.Read())
{
if(reader.HasAttributes && reader.NodeType==XmlNodeType.Element)
{
reader.MoveToElement(); reader.MoveToElement(); reader.MoveToAttribute(“Id”); strVal=reader.Value; reader.Read(); reader.Read();
if(reader.Name==”Dept”)
{
reader.Read();
}
//create the child nodes
TreeNode EcodeNode = new TreeNode(strVal);