
- •About the Authors
- •Contents at a Glance
- •Contents
- •Introduction
- •Goal of the Book
- •How to Use this Book
- •Introduction to the .NET Framework
- •Common Language Runtime (CLR)
- •Class Library
- •Assembly
- •Versioning
- •Exceptions
- •Threads
- •Delegates
- •Summary
- •Introduction to C#
- •Variables
- •Initializing Variables
- •Variable Modifiers
- •Variable Data Types
- •Types of Variables
- •Variable Scope
- •Types of Data Type Casting
- •Arrays
- •Strings
- •Initializing Strings
- •Working with Strings
- •Statements and Expressions
- •Types of Statements
- •Expressions
- •Summary
- •Classes
- •Declaring Classes
- •Inheritance
- •Constructors
- •Destructors
- •Methods
- •Declaring a Method
- •Calling a Method
- •Passing Parameters to Methods
- •Method Modifiers
- •Overloading a Method
- •Namespaces
- •Declaring Namespaces
- •Aliases
- •Structs
- •Enumerations
- •Interfaces
- •Writing, Compiling, and Executing
- •Writing a C# Program
- •Compiling a C# Program
- •Executing a C# Program
- •Summary
- •Arrays
- •Single-Dimensional Arrays
- •Multidimensional Arrays
- •Methods in Arrays
- •Collections
- •Creating Collections
- •Working with Collections
- •Indexers
- •Boxing and Unboxing
- •Preprocessor Directives
- •Summary
- •Attributes
- •Declaring Attributes
- •Attribute Class
- •Attribute Parameters
- •Default Attributes
- •Properties
- •Declaring Properties
- •Accessors
- •Types of Properties
- •Summary
- •Introduction to Threads
- •Creating Threads
- •Aborting Threads
- •Joining Threads
- •Suspending Threads
- •Making Threads Sleep
- •Thread States
- •Thread Priorities
- •Synchronization
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Primary and Foreign Keys
- •Referential Integrity
- •Normalization
- •Designing a Database
- •Low-Level Design
- •Construction
- •Integration and Testing
- •User Acceptance Testing
- •Implementation
- •Operations and Maintenance
- •Summary
- •Creating a New Project
- •Console Application
- •Windows Applications
- •Creating a Windows Application for the Customer Maintenance Project
- •Creating an Interface for Form1
- •Creating an Interface for WorkerForm
- •Creating an Interface for CustomerForm
- •Creating an Interface for ReportsForm
- •Creating an Interface for JobDetailsForm
- •Summary
- •Performing Validations
- •Identifying the Validation Mechanism
- •Using the ErrorProvider Control
- •Handling Exceptions
- •Using the try and catch Statements
- •Using the Debug and Trace Classes
- •Using the Debugging Features of Visual Studio .NET
- •Using the Task List
- •Summary
- •Creating Form1
- •Connecting WorkerForm to the Workers Table
- •Connecting CustomerForm to the tblCustomer Table
- •Connecting the JobDetails Form
- •to the tblJobDetails Table
- •Summary
- •Introduction to the Crystal Reports Designer Tool
- •Creating the Reports Form
- •Creating Crystal Reports
- •Creating the Windows Forms Viewer Control
- •Creating the Monthly Worker Report
- •Summary
- •Introduction to Deploying a Windows Application
- •Deployment Projects Available in Visual Studio .NET
- •Deployment Project Editors
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Populating the TreeView Control
- •Displaying Employee Codes in the TreeView Control
- •Event Handling
- •Displaying Employee Details in the ListView Control
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Adding the Programming Logic to the Application
- •Adding Code to the Form Load() Method
- •Adding Code to the OK Button
- •Adding Code to the Exit Button
- •Summary
- •The Created Event
- •Adding Code to the Created Event
- •Overview of XML
- •The XmlReader Class
- •The XmlWriter Class
- •Displaying Data in an XML Document
- •Displaying an Error Message in the Event Log
- •Displaying Event Entries from Event Viewer
- •Displaying Data from the Summary.xml Document in a Message Box
- •Summary
- •Airline Profile
- •Role of a Business Manager
- •Role of a Network Administrator
- •Role of a Line-of-Business Executive
- •Project Requirements
- •Creation and Deletion of User Accounts
- •Addition of Flight Details
- •Reservations
- •Cancellations
- •Query of Status
- •Confirmation of Tickets
- •Creation of Reports
- •Launch of Frequent Flier Programs
- •Summarizing the Tasks
- •Project Design
- •Database Design
- •Web Forms Design
- •Enabling Security with the Directory Structure
- •Summary
- •Getting Started with ASP.NET
- •Prerequisites for ASP.NET Applications
- •New Features in ASP.NET
- •Types of ASP.NET Applications
- •Exploring ASP.NET Web Applications
- •Introducing Web Forms
- •Web Form Server Controls
- •Configuring ASP.NET Applications
- •Configuring Security for ASP.NET Applications
- •Deploying ASP.NET Applications
- •Creating a Sample ASP.NET Application
- •Creating a New Project
- •Adding Controls to the Project
- •Coding the Application
- •Summary
- •Creating the Database Schema
- •Creating Database Tables
- •Managing Primary Keys and Relationships
- •Viewing the Database Schema
- •Designing Application Forms
- •Standardizing the Interface of the Application
- •Common Forms in the Application
- •Forms for Network Administrators
- •Forms for Business Managers
- •Forms for Line-of-Business Executives
- •Summary
- •The Default.aspx Form
- •The Logoff.aspx Form
- •The ManageUsers.aspx Form
- •The ManageDatabases.aspx Form
- •The ChangePassword.aspx Form
- •Restricting Access to Web Forms
- •The AddFl.aspx Form
- •The RequestID.aspx Form
- •The Reports.aspx Form
- •The FreqFl.aspx Form
- •Coding the Forms for LOB Executives
- •The CreateRes.aspx Form
- •The CancelRes.aspx Form
- •The QueryStat.aspx Form
- •The ConfirmRes.aspx Form
- •Summary
- •Designing the Form
- •The View New Flights Option
- •The View Ticket Status Option
- •The View Flight Status Option
- •The Confirm Reservation Option
- •Testing the Application
- •Summary
- •Locating Errors in Programs
- •Watch Window
- •Locals Window
- •Call Stack Window
- •Autos Window
- •Command Window
- •Testing the Application
- •Summary
- •Managing the Databases
- •Backing Up the SkyShark Airlines Databases
- •Exporting Data from Databases
- •Examining Database Logs
- •Scheduling Database Maintenance Tasks
- •Managing Internet Information Server
- •Configuring IIS Error Pages
- •Managing Web Server Log Files
- •Summary
- •Authentication Mechanisms
- •Securing a Web Site with IIS and ASP.NET
- •Configuring IIS Authentication
- •Configuring Authentication in ASP.NET
- •Securing SQL Server
- •Summary
- •Deployment Scenarios
- •Deployment Editors
- •Creating a Deployment Project
- •Adding the Output of SkySharkDeploy to the Deployment Project
- •Deploying the Project to a Web Server on Another Computer
- •Summary
- •Organization Profile
- •Project Requirements
- •Querying for Information about All Books
- •Querying for Information about Books Based on Criteria
- •Ordering a Book on the Web Site
- •Project Design
- •Database Design
- •Database Schema
- •Web Forms Design
- •Flowcharts for the Web Forms Modules
- •Summary
- •Introduction to ASP.NET Web Services
- •Web Service Architecture
- •Working of a Web Service
- •Technologies Used in Web Services
- •XML in a Web Service
- •WSDL in a Web Service
- •SOAP in a Web Service
- •UDDI in a Web Service
- •Web Services in the .NET Framework
- •The Default Code Generated for a Web Service
- •Testing the SampleWebService Web Service
- •Summary
- •Creating the SearchAll() Web Method
- •Creating the SrchISBN() Web Method
- •Creating the AcceptDetails() Web Method
- •Creating the GenerateOrder() Web Method
- •Testing the Web Service
- •Securing a Web Service
- •Summary
- •Creating the Web Forms for the Bookers Paradise Web Site
- •Adding Code to the Web Forms
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Overview of Mobile Applications
- •The Microsoft Mobile Internet Toolkit
- •Overview of WAP
- •The WAP Architecture
- •Overview of WML
- •The Mobile Web Form
- •The Design of the MobileTimeRetriever Application
- •Creating the Interface for the Mobile Web Forms
- •Adding Code to the MobileTimeRetriever Application
- •Summary
- •Creating the Forms Required for the MobileCallStatus Application
- •Creating the frmLogon Form
- •Creating the frmSelectOption Form
- •Creating the frmPending Form
- •Creating the frmUnattended Form
- •Adding Code to the Submit Button in the frmLogon Form
- •Adding Code to the Query Button in the frmSelectOption Form
- •Adding Code to the Mark checked as complete Button in the frmPending Form
- •Adding Code to the Back Button in the frmPending Form
- •Adding Code to the Accept checked call(s) Button in the frmUnattended Form
- •Adding Code to the Back Button in the frmUnattended Form
- •Summary
- •What Is COM?
- •Windows DNA
- •Microsoft Transaction Server (MTS)
- •.NET Interoperability
- •COM Interoperability
- •Messaging
- •Benefits of Message Queues
- •Limitations
- •Key Messaging Terms
- •Summary
- •Pointers
- •Declaring Pointers
- •Types of Code
- •Implementing Pointers
- •Using Pointers with Managed Code
- •Working with Pointers
- •Compiling Unsafe Code
- •Summary
- •Introduction to the Languages of Visual Studio .NET
- •Visual C# .NET
- •Visual Basic .NET
- •Visual C++ .NET
- •Overview of Visual Basic .NET
- •Abstraction
- •Encapsulation
- •Inheritance
- •Polymorphism
- •Components of Visual Basic .NET
- •Variables
- •Constants
- •Operators
- •Arrays
- •Collections
- •Procedures
- •Arguments
- •Functions
- •Adding Code to the Submit Button
- •Adding Code to the Exit Button
- •Summary
- •Introduction to Visual Studio .NET IDE
- •Menu Bar
- •Toolbars
- •Visual Studio .NET IDE Windows
- •Toolbox
- •The Task List Window
- •Managing Windows
- •Customizing Visual Studio .NET IDE
- •The Options Dialog Box
- •The Customize Dialog Box
- •Summary
- •Index
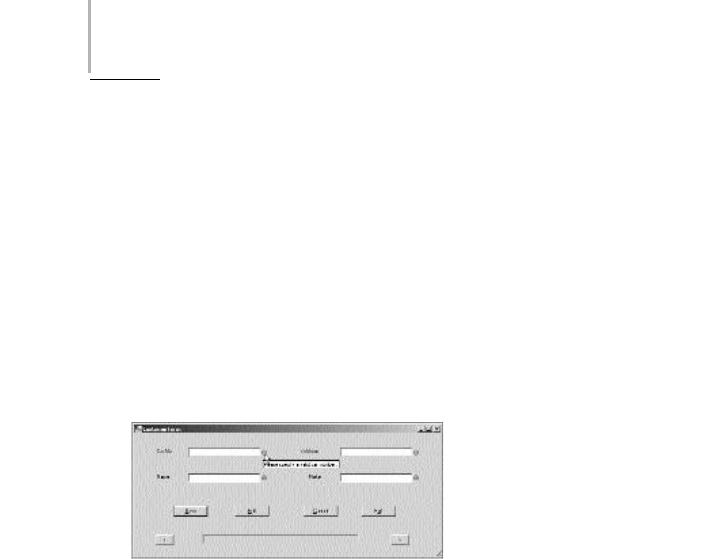
186 Project 1 CREATING A CUSTOMER MAINTENANCE PROJECT
else
{
sqlDataAdapter1.Update(customerDataSet1); MessageBox.Show(“Database updated!”);
}
}
In the preceding code, I have used a variable flag of the bool data type to determine whether any field has been left blank. When a field is blank, the value of the flag variable changes to false and an error message is set on the ErrorProvider control. Similarly, after the user specifies a valid value in the field, the error message associated with the field is cleared.
After writing the preceding code, run the form and check the output. To open CustomerForm, click on Customer on the main menu of the form. If you click on Save without specifying any value in the CustomerForm form,error icons appear for each field in the form, as shown in Figure 9-7.
FIGURE 9-7 Using an ErrorProvider control
Handling Exceptions
Exceptions are abnormal conditions in an application. For example, if you attempt to update records in a database when one or more of the mandatory fields have been left blank, your application will throw an exception.
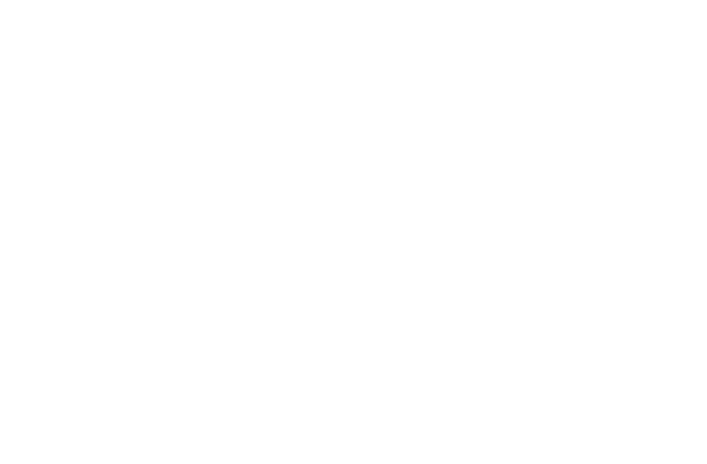
VALIDATIONS AND EXCEPTION HANDLING |
Chapter 9 |
187 |
|
|
|
If exceptions are not handled by your application, your application will terminate abnormally. In this section, you can learn about ways to handle exceptions in the
JobDetails form.
Using the try and catch Statements
The try and catch statements form part of structured exception handling. When you know about certain statements of code that may generate an error, you can place those statements in a try block. For example, when you specify code to update data in a database or convert data from one format to another, your application can throw an exception. Therefore, you should place these statements in a try block.
Whenever statements in a try block throw an exception, the catch block, which follows the try block, catches the exception if the exception is in the same format as that expected by the catch block. For example, if you attempt to supply a string data type variable instead of an int data type, your application will throw an exception of the FormatException class. If the catch block handles exceptions of the FormatException class, the statements of the catch block will be executed.
You may wonder if you need to specify catch statements for each type of exception that your application generates. It is not mandatory to do so. All exception classes are derived from the Exception class of the System namespace. Therefore, unless you want to implement different exception handling logic for different types of exceptions, you can use the Exception class to handle all exceptions generated by your application.
The syntax for the try and catch statements is given as follows:
try
{
//The statements that might generate an error Statement(s);
}
catch (filter)

188 Project 1 CREATING A CUSTOMER MAINTENANCE PROJECT
{
//The statements written here are executed when the statements listed in the Try
//block fail and the filter specified is true.
Statement(s);
}
The code for the Click event of the Update button, after implementing the try and catch statements, is given as follows:
private void btnUpdate_Click(object sender, System.EventArgs e)
{ |
|
|
|
|
Y |
if (editCarNo.Text.Length <6) |
|
||||
{ |
|
|
|
|
|
|
|
|
L |
||
|
|
|
|
||
|
MessageBox.Show(“Please specify a valid car Number”); |
||||
|
editCarNo.Focus(); |
|
F |
||
|
|
M |
|
||
|
return; |
|
|
|
|
} |
|
|
|
|
|
|
|
A |
|
||
try |
|
|
|
||
{ |
|
E |
|
||
|
|
|
|||
|
if (Convert. oInt32(editWorkerId.Text)<1) |
||||
|
|
T |
|
|
{
MessageBox.Show(“Please specify a valid worker ID”); editWorkerId.Focus();
return;
}
if (Convert.ToDateTime(dateTimePicker1.Value) > DateTime.Today)
{
MessageBox.Show(“Please specify a valid date”); dateTimePicker1.Focus();
return;
}
}
catch (Exception exception)
{
MessageBox.Show(exception.Message);
}
}
Team-Fly®
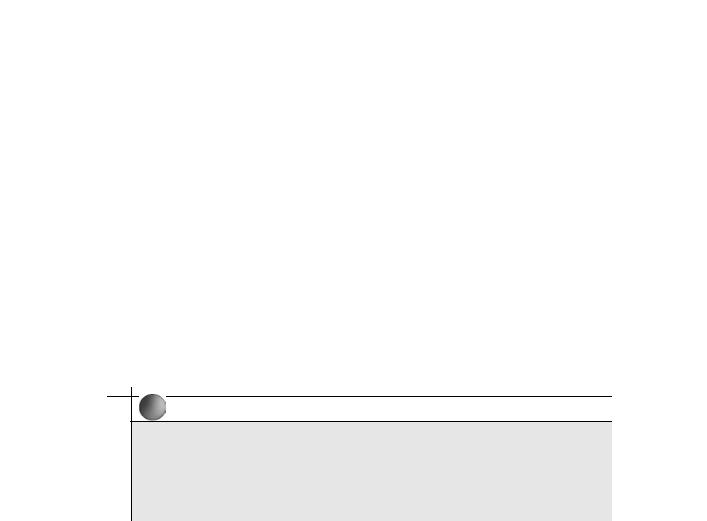
VALIDATIONS AND EXCEPTION HANDLING |
Chapter 9 |
189 |
|
|
|
In the preceding code, I used the try block for converting the values specified by
the user for the editWorkerId and dateTimePicker1 fields to int and date data
types, respectively. If these statements throw any exception, the program control passes to the catch block and the description of the error is displayed to the user.
Using the Debug and Trace Classes
The .NET Framework class library provides the Debug and Trace classes in the System.Diagnostics namespace.The classes can be used to monitor variables in an application. For example, the number of months in a year cannot exceed 12. Therefore, you can use the Debug or Trace classes to monitor the value of a variable, such as a month. Whenever the value of the variable exceeds 12, the application will throw an assertion failure, which will lead to display of the Debug Assertion Failure dialog box.
CAUTION
The Debug and Trace classes provide the same functionality. However, the Debug class is active only in the Debug configuration.Therefore, use the Debug class only to debug your application. Do not change application data by using this class because the logic will not work in the Release configuration.Therefore, if you plan to change application data, use the Trace class.
You can use the Debug and Trace classes anywhere in the JobDetails form. As an example, I have used the Assert method of the Debug class to ensure that the date in the dateTimePicker1 control never exceeds the current date. To do this, complete the following steps:
1.Add a reference to the System.Diagnostics namespace by specifying the following line of code in the JobDetails.cs file:
using System.Diagnostics;
2.Add the following line of code wherever you want to check the value of
the dateTimePicker1 control:
Debug.Assert(Convert.ToDateTime(dateTimePicker1.Value) >
DateTime.Today,”The date has exceeded the current date”);