
- •About the Authors
- •Contents at a Glance
- •Contents
- •Introduction
- •Goal of the Book
- •How to Use this Book
- •Introduction to the .NET Framework
- •Common Language Runtime (CLR)
- •Class Library
- •Assembly
- •Versioning
- •Exceptions
- •Threads
- •Delegates
- •Summary
- •Introduction to C#
- •Variables
- •Initializing Variables
- •Variable Modifiers
- •Variable Data Types
- •Types of Variables
- •Variable Scope
- •Types of Data Type Casting
- •Arrays
- •Strings
- •Initializing Strings
- •Working with Strings
- •Statements and Expressions
- •Types of Statements
- •Expressions
- •Summary
- •Classes
- •Declaring Classes
- •Inheritance
- •Constructors
- •Destructors
- •Methods
- •Declaring a Method
- •Calling a Method
- •Passing Parameters to Methods
- •Method Modifiers
- •Overloading a Method
- •Namespaces
- •Declaring Namespaces
- •Aliases
- •Structs
- •Enumerations
- •Interfaces
- •Writing, Compiling, and Executing
- •Writing a C# Program
- •Compiling a C# Program
- •Executing a C# Program
- •Summary
- •Arrays
- •Single-Dimensional Arrays
- •Multidimensional Arrays
- •Methods in Arrays
- •Collections
- •Creating Collections
- •Working with Collections
- •Indexers
- •Boxing and Unboxing
- •Preprocessor Directives
- •Summary
- •Attributes
- •Declaring Attributes
- •Attribute Class
- •Attribute Parameters
- •Default Attributes
- •Properties
- •Declaring Properties
- •Accessors
- •Types of Properties
- •Summary
- •Introduction to Threads
- •Creating Threads
- •Aborting Threads
- •Joining Threads
- •Suspending Threads
- •Making Threads Sleep
- •Thread States
- •Thread Priorities
- •Synchronization
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Primary and Foreign Keys
- •Referential Integrity
- •Normalization
- •Designing a Database
- •Low-Level Design
- •Construction
- •Integration and Testing
- •User Acceptance Testing
- •Implementation
- •Operations and Maintenance
- •Summary
- •Creating a New Project
- •Console Application
- •Windows Applications
- •Creating a Windows Application for the Customer Maintenance Project
- •Creating an Interface for Form1
- •Creating an Interface for WorkerForm
- •Creating an Interface for CustomerForm
- •Creating an Interface for ReportsForm
- •Creating an Interface for JobDetailsForm
- •Summary
- •Performing Validations
- •Identifying the Validation Mechanism
- •Using the ErrorProvider Control
- •Handling Exceptions
- •Using the try and catch Statements
- •Using the Debug and Trace Classes
- •Using the Debugging Features of Visual Studio .NET
- •Using the Task List
- •Summary
- •Creating Form1
- •Connecting WorkerForm to the Workers Table
- •Connecting CustomerForm to the tblCustomer Table
- •Connecting the JobDetails Form
- •to the tblJobDetails Table
- •Summary
- •Introduction to the Crystal Reports Designer Tool
- •Creating the Reports Form
- •Creating Crystal Reports
- •Creating the Windows Forms Viewer Control
- •Creating the Monthly Worker Report
- •Summary
- •Introduction to Deploying a Windows Application
- •Deployment Projects Available in Visual Studio .NET
- •Deployment Project Editors
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Populating the TreeView Control
- •Displaying Employee Codes in the TreeView Control
- •Event Handling
- •Displaying Employee Details in the ListView Control
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Adding the Programming Logic to the Application
- •Adding Code to the Form Load() Method
- •Adding Code to the OK Button
- •Adding Code to the Exit Button
- •Summary
- •The Created Event
- •Adding Code to the Created Event
- •Overview of XML
- •The XmlReader Class
- •The XmlWriter Class
- •Displaying Data in an XML Document
- •Displaying an Error Message in the Event Log
- •Displaying Event Entries from Event Viewer
- •Displaying Data from the Summary.xml Document in a Message Box
- •Summary
- •Airline Profile
- •Role of a Business Manager
- •Role of a Network Administrator
- •Role of a Line-of-Business Executive
- •Project Requirements
- •Creation and Deletion of User Accounts
- •Addition of Flight Details
- •Reservations
- •Cancellations
- •Query of Status
- •Confirmation of Tickets
- •Creation of Reports
- •Launch of Frequent Flier Programs
- •Summarizing the Tasks
- •Project Design
- •Database Design
- •Web Forms Design
- •Enabling Security with the Directory Structure
- •Summary
- •Getting Started with ASP.NET
- •Prerequisites for ASP.NET Applications
- •New Features in ASP.NET
- •Types of ASP.NET Applications
- •Exploring ASP.NET Web Applications
- •Introducing Web Forms
- •Web Form Server Controls
- •Configuring ASP.NET Applications
- •Configuring Security for ASP.NET Applications
- •Deploying ASP.NET Applications
- •Creating a Sample ASP.NET Application
- •Creating a New Project
- •Adding Controls to the Project
- •Coding the Application
- •Summary
- •Creating the Database Schema
- •Creating Database Tables
- •Managing Primary Keys and Relationships
- •Viewing the Database Schema
- •Designing Application Forms
- •Standardizing the Interface of the Application
- •Common Forms in the Application
- •Forms for Network Administrators
- •Forms for Business Managers
- •Forms for Line-of-Business Executives
- •Summary
- •The Default.aspx Form
- •The Logoff.aspx Form
- •The ManageUsers.aspx Form
- •The ManageDatabases.aspx Form
- •The ChangePassword.aspx Form
- •Restricting Access to Web Forms
- •The AddFl.aspx Form
- •The RequestID.aspx Form
- •The Reports.aspx Form
- •The FreqFl.aspx Form
- •Coding the Forms for LOB Executives
- •The CreateRes.aspx Form
- •The CancelRes.aspx Form
- •The QueryStat.aspx Form
- •The ConfirmRes.aspx Form
- •Summary
- •Designing the Form
- •The View New Flights Option
- •The View Ticket Status Option
- •The View Flight Status Option
- •The Confirm Reservation Option
- •Testing the Application
- •Summary
- •Locating Errors in Programs
- •Watch Window
- •Locals Window
- •Call Stack Window
- •Autos Window
- •Command Window
- •Testing the Application
- •Summary
- •Managing the Databases
- •Backing Up the SkyShark Airlines Databases
- •Exporting Data from Databases
- •Examining Database Logs
- •Scheduling Database Maintenance Tasks
- •Managing Internet Information Server
- •Configuring IIS Error Pages
- •Managing Web Server Log Files
- •Summary
- •Authentication Mechanisms
- •Securing a Web Site with IIS and ASP.NET
- •Configuring IIS Authentication
- •Configuring Authentication in ASP.NET
- •Securing SQL Server
- •Summary
- •Deployment Scenarios
- •Deployment Editors
- •Creating a Deployment Project
- •Adding the Output of SkySharkDeploy to the Deployment Project
- •Deploying the Project to a Web Server on Another Computer
- •Summary
- •Organization Profile
- •Project Requirements
- •Querying for Information about All Books
- •Querying for Information about Books Based on Criteria
- •Ordering a Book on the Web Site
- •Project Design
- •Database Design
- •Database Schema
- •Web Forms Design
- •Flowcharts for the Web Forms Modules
- •Summary
- •Introduction to ASP.NET Web Services
- •Web Service Architecture
- •Working of a Web Service
- •Technologies Used in Web Services
- •XML in a Web Service
- •WSDL in a Web Service
- •SOAP in a Web Service
- •UDDI in a Web Service
- •Web Services in the .NET Framework
- •The Default Code Generated for a Web Service
- •Testing the SampleWebService Web Service
- •Summary
- •Creating the SearchAll() Web Method
- •Creating the SrchISBN() Web Method
- •Creating the AcceptDetails() Web Method
- •Creating the GenerateOrder() Web Method
- •Testing the Web Service
- •Securing a Web Service
- •Summary
- •Creating the Web Forms for the Bookers Paradise Web Site
- •Adding Code to the Web Forms
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Overview of Mobile Applications
- •The Microsoft Mobile Internet Toolkit
- •Overview of WAP
- •The WAP Architecture
- •Overview of WML
- •The Mobile Web Form
- •The Design of the MobileTimeRetriever Application
- •Creating the Interface for the Mobile Web Forms
- •Adding Code to the MobileTimeRetriever Application
- •Summary
- •Creating the Forms Required for the MobileCallStatus Application
- •Creating the frmLogon Form
- •Creating the frmSelectOption Form
- •Creating the frmPending Form
- •Creating the frmUnattended Form
- •Adding Code to the Submit Button in the frmLogon Form
- •Adding Code to the Query Button in the frmSelectOption Form
- •Adding Code to the Mark checked as complete Button in the frmPending Form
- •Adding Code to the Back Button in the frmPending Form
- •Adding Code to the Accept checked call(s) Button in the frmUnattended Form
- •Adding Code to the Back Button in the frmUnattended Form
- •Summary
- •What Is COM?
- •Windows DNA
- •Microsoft Transaction Server (MTS)
- •.NET Interoperability
- •COM Interoperability
- •Messaging
- •Benefits of Message Queues
- •Limitations
- •Key Messaging Terms
- •Summary
- •Pointers
- •Declaring Pointers
- •Types of Code
- •Implementing Pointers
- •Using Pointers with Managed Code
- •Working with Pointers
- •Compiling Unsafe Code
- •Summary
- •Introduction to the Languages of Visual Studio .NET
- •Visual C# .NET
- •Visual Basic .NET
- •Visual C++ .NET
- •Overview of Visual Basic .NET
- •Abstraction
- •Encapsulation
- •Inheritance
- •Polymorphism
- •Components of Visual Basic .NET
- •Variables
- •Constants
- •Operators
- •Arrays
- •Collections
- •Procedures
- •Arguments
- •Functions
- •Adding Code to the Submit Button
- •Adding Code to the Exit Button
- •Summary
- •Introduction to Visual Studio .NET IDE
- •Menu Bar
- •Toolbars
- •Visual Studio .NET IDE Windows
- •Toolbox
- •The Task List Window
- •Managing Windows
- •Customizing Visual Studio .NET IDE
- •The Options Dialog Box
- •The Customize Dialog Box
- •Summary
- •Index
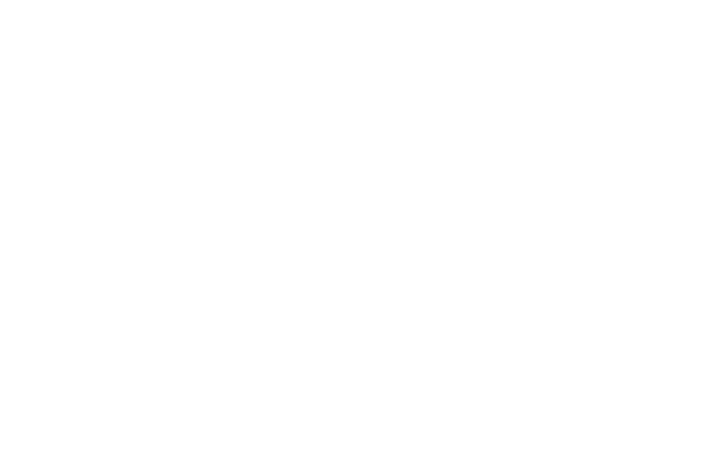
VALIDATIONS AND EXCEPTION HANDLING |
Chapter 9 |
|
183 |
|
|||
|
|
|
|
The preceding code displays an error message if the user specifies incorrect values for the editCarNo and the editWorkerId fields. You will notice that I have not validated the dates and other fields. It is easier to validate these fields by using exception handlers, which will be discussed in the “Handling Exceptions” section of this chapter. Before doing that, the next section will examine the ErrorProvider control of Visual Studio .NET.
Using the ErrorProvider Control
Instead of displaying message boxes each time the user submits an incorrect or incomplete form, you can use the ErrorProvider control to show an icon next to the control (which will be referred to as the error icon in future references) that has an error. When a user moves the mouse pointer over the error icon, the error message associated with the icon is displayed as a ToolTip.
The ErrorProvider control enhances user experience by eliminating the use of message boxes for notifying errors. You have already added the validation code for the JobDetails form.Therefore, in this section, you will validate a different form, CustomerForm, by using the ErrorProvider control. The steps to add the ErrorProvider control to the CustomerForm form are as follows:
1.Open the CustomerForm form in the Design view.
2.Drag the ErrorProvider control from the Toolbox to the form. The ErrorProvider control will be added to the component tray.
3.Change the Name property of ErrorProvider to errCustForm.
4.Click on the textBox1 control that represents the Car No. field.
5.In the Properties window, specify a description of the error message in the Error on errCustForm property, as shown in Figure 9-6.
6.Repeat Steps 4 and 5 to add error descriptions for all text boxes to the
CustomerForm form.

184 Project 1 CREATING A CUSTOMER MAINTENANCE PROJECT
FIGURE 9-6 Adding an ErrorProvider control
TIP
As you add error descriptions to each control in the form, an exclamation point icon appears next to each control.
When you specify an error message with each control during design time, the error icon appears as soon as a user loads the form. To avoid showing an error message even before the user has entered values in the form, you should clear the error message. To clear the error messages associated with controls, use the SetError method of the errCustForm control.The SetError method sets the error message associated with a control. If a blank string is passed to this method, the error message associated with the control is cleared. To clear error messages, add the following code for the Load event
of the CustomerForm form:
private void CustomerForm_Load(object sender, System.EventArgs e)
{
errCustForm.SetError(textBox1,””); errCustForm.SetError(textBox2,””); errCustForm.SetError(textBox3,””); errCustForm.SetError(textBox4,””);
}

VALIDATIONS AND EXCEPTION HANDLING |
Chapter 9 |
185 |
|
|
|
Next, you need to check for the availability of data in each text box when the user clicks on Save.The code for the Click event of the Save button is given as follows:
private void btnSave_Click(object sender, System.EventArgs e)
{
bool flag; flag=true;
if (textBox1.Text==””)
{
errCustForm.SetError(textBox1,”Please specify a valid car number.”); flag=false;
}
else errCustForm.SetError(textBox1,””);
if (textBox2.Text==””)
{
errCustForm.SetError(textBox2,”Please specify a valid name.”); flag=false;
}
else errCustForm.SetError(textBox2,””);
if (textBox3.Text==””)
{
errCustForm.SetError(textBox3,”Please specify a valid address.”); flag=false;
}
else errCustForm.SetError(textBox3,””);
if (textBox4.Text==””)
{
errCustForm.SetError(textBox4,”Please specify a valid make.”); flag=false;
}
else errCustForm.SetError(textBox4,””);
if (flag==false) return;