
- •About the Authors
- •Contents at a Glance
- •Contents
- •Introduction
- •Goal of the Book
- •How to Use this Book
- •Introduction to the .NET Framework
- •Common Language Runtime (CLR)
- •Class Library
- •Assembly
- •Versioning
- •Exceptions
- •Threads
- •Delegates
- •Summary
- •Introduction to C#
- •Variables
- •Initializing Variables
- •Variable Modifiers
- •Variable Data Types
- •Types of Variables
- •Variable Scope
- •Types of Data Type Casting
- •Arrays
- •Strings
- •Initializing Strings
- •Working with Strings
- •Statements and Expressions
- •Types of Statements
- •Expressions
- •Summary
- •Classes
- •Declaring Classes
- •Inheritance
- •Constructors
- •Destructors
- •Methods
- •Declaring a Method
- •Calling a Method
- •Passing Parameters to Methods
- •Method Modifiers
- •Overloading a Method
- •Namespaces
- •Declaring Namespaces
- •Aliases
- •Structs
- •Enumerations
- •Interfaces
- •Writing, Compiling, and Executing
- •Writing a C# Program
- •Compiling a C# Program
- •Executing a C# Program
- •Summary
- •Arrays
- •Single-Dimensional Arrays
- •Multidimensional Arrays
- •Methods in Arrays
- •Collections
- •Creating Collections
- •Working with Collections
- •Indexers
- •Boxing and Unboxing
- •Preprocessor Directives
- •Summary
- •Attributes
- •Declaring Attributes
- •Attribute Class
- •Attribute Parameters
- •Default Attributes
- •Properties
- •Declaring Properties
- •Accessors
- •Types of Properties
- •Summary
- •Introduction to Threads
- •Creating Threads
- •Aborting Threads
- •Joining Threads
- •Suspending Threads
- •Making Threads Sleep
- •Thread States
- •Thread Priorities
- •Synchronization
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Primary and Foreign Keys
- •Referential Integrity
- •Normalization
- •Designing a Database
- •Low-Level Design
- •Construction
- •Integration and Testing
- •User Acceptance Testing
- •Implementation
- •Operations and Maintenance
- •Summary
- •Creating a New Project
- •Console Application
- •Windows Applications
- •Creating a Windows Application for the Customer Maintenance Project
- •Creating an Interface for Form1
- •Creating an Interface for WorkerForm
- •Creating an Interface for CustomerForm
- •Creating an Interface for ReportsForm
- •Creating an Interface for JobDetailsForm
- •Summary
- •Performing Validations
- •Identifying the Validation Mechanism
- •Using the ErrorProvider Control
- •Handling Exceptions
- •Using the try and catch Statements
- •Using the Debug and Trace Classes
- •Using the Debugging Features of Visual Studio .NET
- •Using the Task List
- •Summary
- •Creating Form1
- •Connecting WorkerForm to the Workers Table
- •Connecting CustomerForm to the tblCustomer Table
- •Connecting the JobDetails Form
- •to the tblJobDetails Table
- •Summary
- •Introduction to the Crystal Reports Designer Tool
- •Creating the Reports Form
- •Creating Crystal Reports
- •Creating the Windows Forms Viewer Control
- •Creating the Monthly Worker Report
- •Summary
- •Introduction to Deploying a Windows Application
- •Deployment Projects Available in Visual Studio .NET
- •Deployment Project Editors
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Populating the TreeView Control
- •Displaying Employee Codes in the TreeView Control
- •Event Handling
- •Displaying Employee Details in the ListView Control
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Adding the Programming Logic to the Application
- •Adding Code to the Form Load() Method
- •Adding Code to the OK Button
- •Adding Code to the Exit Button
- •Summary
- •The Created Event
- •Adding Code to the Created Event
- •Overview of XML
- •The XmlReader Class
- •The XmlWriter Class
- •Displaying Data in an XML Document
- •Displaying an Error Message in the Event Log
- •Displaying Event Entries from Event Viewer
- •Displaying Data from the Summary.xml Document in a Message Box
- •Summary
- •Airline Profile
- •Role of a Business Manager
- •Role of a Network Administrator
- •Role of a Line-of-Business Executive
- •Project Requirements
- •Creation and Deletion of User Accounts
- •Addition of Flight Details
- •Reservations
- •Cancellations
- •Query of Status
- •Confirmation of Tickets
- •Creation of Reports
- •Launch of Frequent Flier Programs
- •Summarizing the Tasks
- •Project Design
- •Database Design
- •Web Forms Design
- •Enabling Security with the Directory Structure
- •Summary
- •Getting Started with ASP.NET
- •Prerequisites for ASP.NET Applications
- •New Features in ASP.NET
- •Types of ASP.NET Applications
- •Exploring ASP.NET Web Applications
- •Introducing Web Forms
- •Web Form Server Controls
- •Configuring ASP.NET Applications
- •Configuring Security for ASP.NET Applications
- •Deploying ASP.NET Applications
- •Creating a Sample ASP.NET Application
- •Creating a New Project
- •Adding Controls to the Project
- •Coding the Application
- •Summary
- •Creating the Database Schema
- •Creating Database Tables
- •Managing Primary Keys and Relationships
- •Viewing the Database Schema
- •Designing Application Forms
- •Standardizing the Interface of the Application
- •Common Forms in the Application
- •Forms for Network Administrators
- •Forms for Business Managers
- •Forms for Line-of-Business Executives
- •Summary
- •The Default.aspx Form
- •The Logoff.aspx Form
- •The ManageUsers.aspx Form
- •The ManageDatabases.aspx Form
- •The ChangePassword.aspx Form
- •Restricting Access to Web Forms
- •The AddFl.aspx Form
- •The RequestID.aspx Form
- •The Reports.aspx Form
- •The FreqFl.aspx Form
- •Coding the Forms for LOB Executives
- •The CreateRes.aspx Form
- •The CancelRes.aspx Form
- •The QueryStat.aspx Form
- •The ConfirmRes.aspx Form
- •Summary
- •Designing the Form
- •The View New Flights Option
- •The View Ticket Status Option
- •The View Flight Status Option
- •The Confirm Reservation Option
- •Testing the Application
- •Summary
- •Locating Errors in Programs
- •Watch Window
- •Locals Window
- •Call Stack Window
- •Autos Window
- •Command Window
- •Testing the Application
- •Summary
- •Managing the Databases
- •Backing Up the SkyShark Airlines Databases
- •Exporting Data from Databases
- •Examining Database Logs
- •Scheduling Database Maintenance Tasks
- •Managing Internet Information Server
- •Configuring IIS Error Pages
- •Managing Web Server Log Files
- •Summary
- •Authentication Mechanisms
- •Securing a Web Site with IIS and ASP.NET
- •Configuring IIS Authentication
- •Configuring Authentication in ASP.NET
- •Securing SQL Server
- •Summary
- •Deployment Scenarios
- •Deployment Editors
- •Creating a Deployment Project
- •Adding the Output of SkySharkDeploy to the Deployment Project
- •Deploying the Project to a Web Server on Another Computer
- •Summary
- •Organization Profile
- •Project Requirements
- •Querying for Information about All Books
- •Querying for Information about Books Based on Criteria
- •Ordering a Book on the Web Site
- •Project Design
- •Database Design
- •Database Schema
- •Web Forms Design
- •Flowcharts for the Web Forms Modules
- •Summary
- •Introduction to ASP.NET Web Services
- •Web Service Architecture
- •Working of a Web Service
- •Technologies Used in Web Services
- •XML in a Web Service
- •WSDL in a Web Service
- •SOAP in a Web Service
- •UDDI in a Web Service
- •Web Services in the .NET Framework
- •The Default Code Generated for a Web Service
- •Testing the SampleWebService Web Service
- •Summary
- •Creating the SearchAll() Web Method
- •Creating the SrchISBN() Web Method
- •Creating the AcceptDetails() Web Method
- •Creating the GenerateOrder() Web Method
- •Testing the Web Service
- •Securing a Web Service
- •Summary
- •Creating the Web Forms for the Bookers Paradise Web Site
- •Adding Code to the Web Forms
- •Summary
- •Case Study
- •Project Life Cycle
- •Analyzing Requirements
- •High-Level Design
- •Low-Level Design
- •Summary
- •Overview of Mobile Applications
- •The Microsoft Mobile Internet Toolkit
- •Overview of WAP
- •The WAP Architecture
- •Overview of WML
- •The Mobile Web Form
- •The Design of the MobileTimeRetriever Application
- •Creating the Interface for the Mobile Web Forms
- •Adding Code to the MobileTimeRetriever Application
- •Summary
- •Creating the Forms Required for the MobileCallStatus Application
- •Creating the frmLogon Form
- •Creating the frmSelectOption Form
- •Creating the frmPending Form
- •Creating the frmUnattended Form
- •Adding Code to the Submit Button in the frmLogon Form
- •Adding Code to the Query Button in the frmSelectOption Form
- •Adding Code to the Mark checked as complete Button in the frmPending Form
- •Adding Code to the Back Button in the frmPending Form
- •Adding Code to the Accept checked call(s) Button in the frmUnattended Form
- •Adding Code to the Back Button in the frmUnattended Form
- •Summary
- •What Is COM?
- •Windows DNA
- •Microsoft Transaction Server (MTS)
- •.NET Interoperability
- •COM Interoperability
- •Messaging
- •Benefits of Message Queues
- •Limitations
- •Key Messaging Terms
- •Summary
- •Pointers
- •Declaring Pointers
- •Types of Code
- •Implementing Pointers
- •Using Pointers with Managed Code
- •Working with Pointers
- •Compiling Unsafe Code
- •Summary
- •Introduction to the Languages of Visual Studio .NET
- •Visual C# .NET
- •Visual Basic .NET
- •Visual C++ .NET
- •Overview of Visual Basic .NET
- •Abstraction
- •Encapsulation
- •Inheritance
- •Polymorphism
- •Components of Visual Basic .NET
- •Variables
- •Constants
- •Operators
- •Arrays
- •Collections
- •Procedures
- •Arguments
- •Functions
- •Adding Code to the Submit Button
- •Adding Code to the Exit Button
- •Summary
- •Introduction to Visual Studio .NET IDE
- •Menu Bar
- •Toolbars
- •Visual Studio .NET IDE Windows
- •Toolbox
- •The Task List Window
- •Managing Windows
- •Customizing Visual Studio .NET IDE
- •The Options Dialog Box
- •The Customize Dialog Box
- •Summary
- •Index
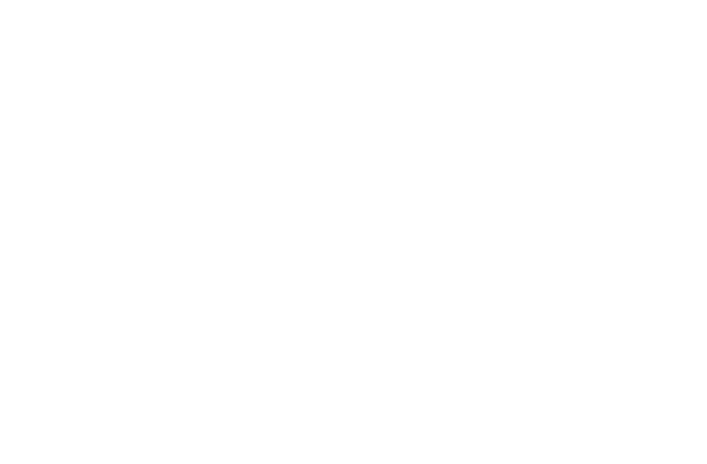
56 |
Part II |
HANDLING DATA |
|
|
|
------------
object1.Add (25, 50)
-------------
Because the type of parameters passed to the Add() method in the previous statement are integers, the C# compiler calls the first Add() method.
Default Parameters
Methods are useful if you want certain parameters of a method not to be explicitly initialized.These parameters then take default values as specified in the body of the method.This feature is provided by the default parameters of C++. C# does not support default parameters. However, to overcome this problem, you can overload methods.
In the previous example, you can create overloads of the method Add() to pass default values to it. The code for the Add() method in the previous section does not specify any value for either x or y. However, you can modify the code as shown following to pass a default value to the method.
public int Add (int x)
{
int z = x + 100; return z;
}
The previous code always adds 100 to the value of integer x that is passed as a parameter when the Add() method is called by any class.
You have learned about classes and the methods used with classes. However, when you create an instance of a user-defined class, there may be more than one class with the same name. Therefore, to avoid this confusion, C# provides you with namespaces.
Namespaces
Namespaces are containers that are used to logically group similar classes that have related functionality. You can also use namespaces to group similar data types. Therefore, when you refer a data type or a class, their names are automatically
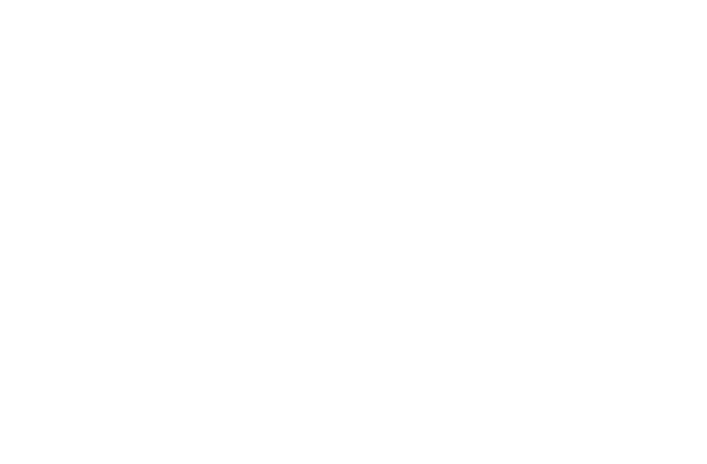
COMPONENTS OF C# |
Chapter 3 |
57 |
|
|
|
prefixed with the name of the namespace. This helps the compiler to understand which class is being referred in your code.
In C#, you need to declare each class in a namespace. However, if you do not explicitly declare a class in a namespace, C# automatically places the class in the default namespace.The default namespace is automatically created with the same name as that of the project. A namespace in C# can have more than one class.
Declaring Namespaces
C# provides you with several classes that you can use in your program code. Most of these classes are a part of the System namespace. You can also declare namespaces in the program code and then add classes to them. A namespace is declared using the namespace keyword. While declaring a namespace, you do not need to prefix the namespace declaration with an access modifier. All namespaces in C# are implicitly public, as they can be used across all programs.
namespace Employee
{
class Employee
}
You can place the Employee class in the Employee namespace. In addition, you can include other namespaces, classes, structs, enumerations, and interfaces in a namespace. You will learn about structs and enumerations later in this chapter.
C# allows the use of nested namespaces.Therefore, to refer to a namespace within another namespace, you use periods (.) to separate the names of the namespaces. For example,
namespace Employee
{
namespace Salary
{
class Salary
}
}
To refer to the Salary class, you need to refer to it as Employee.Salary.Salary.
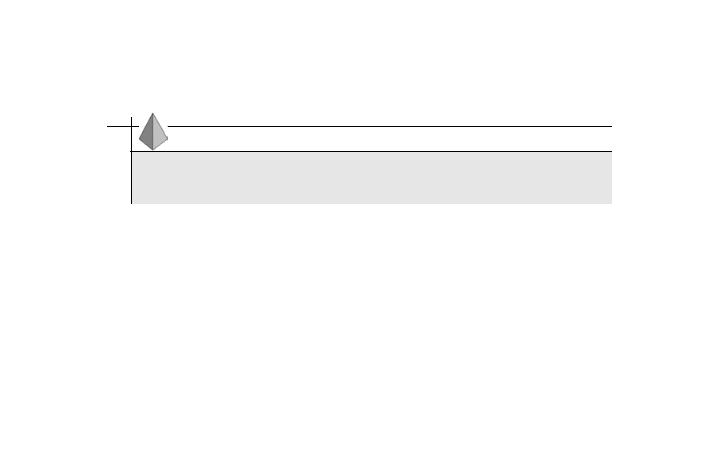
58 |
Part II |
HANDLING DATA |
|
|
|
TIP
You can have two classes with the same name in different namespaces. However, a namespace cannot contain two classes with the same name.
Y After declaring a namespace, you can accessLthe namespace with the using direc-
tive. Therefore, to access the namespaceFEmployee, use the following statement:
M Additionally, as you have seenAearlier, C# allows the use of nested namespaces. It
will, however, be tediousEto write the complete name of a class repeatedly in the
code. To simplify this task, you can declare the class with the keyword in the
T using
beginning of the code, such as:
using Employee.Salary;
In this case, each time you use the class Salary, the compiler can discern that the class Salary within the Salary namespace is being referred.
However, there may be cases when two namespaces contain classes with the same name. To refer to these classes, you need to write the full name in the code. To avoid writing full names in such cases, you can create an alias.
Aliases
C# allows you to create aliases of a class or a namespace with the using keyword. Aliases are short names assigned to classes and namespaces. The syntax of an alias is:
using <alias> = <class>
In the previous example, you can create an alias for the class Salary, such as:
using aliasSalary = Employee.Salary
Now, each time you need to refer to the Salary namespace, you can use the alias name, such as:
aliasSalary.Salary.CalculateSalary()
Team-Fly®