
Assembly Language Step by Step 1992
.pdfWrong question. Silly question. If Michael is still learning, it means that all of us are students and will always be students. It means that the journey is the goal, and as long as we continue to probe and hack and fiddle and try things we never tried before, that over time we will advance the state of the art, and create programs that would have made the pioneers in our field catch their breath in 1977.
For the point is not to conquer the subject, but to live with it, and grow with your knowledge of it. The journey is the goal, and with this book I've tried hard to help those people who have been frozen with fear at the thought of starting the journey, staring at the complexity of it all and wondering where the first brick in that "yellow brick road" might be.
It's here, with nothing more than the conviction that you can do it.
I got out of school in recession year 1974 with a B.A. in English, Summa Cum Laude, and not much in reliable prospects outside of driving a cab. I finessed my way into a job with Xerox Corporation, repairing copy machines. Books were fun, but paperwork makes money—so I picked up a toolbag and had a fine old time for several years, before finessing my way into a computer-programming position.
But I'll never forget that first awful moment when I looked over the shoulder of an accomplished technician at a model 660 copier with its panels off. It looked like a bottomless pit of little cams and gears and drums and sprocket chains, turning and flipping and knocking switch actuators back and forth. Mesmermized by the complexity, I forgot to notice that a sheet of paper had been fed through the machine and turned into a copy of the original document. I was terrified of never learning what all the little cams did, and missed the comforting simplicity of the Big Picture—that a copy machine makes copies.
That's Square One—discover the big picture. Ignore the cams and gears for a bit. You can do it. Find out what's important in holding the big picture together (ask someone if it's not obvious), and study that before getting down to the cams and gears. Locate the processes that happen. Divide the Big Picture into sub pictures. See how things flow.
Only then should you focus on something as small and as lost in the mess as an individual cam or switch.
That's how you conquer complexity, and that's how I've presented assembly language in this book. Some might say I've shorted the instruction set, but covering the instruction set was never the real goal here.
The real goal was to conquer your fear of the complexity of the subject, with some metaphors and some pictures and some funny stories to bleed the tension away. Did it work? You tell me. I'd really like to know.
12.1 Where to Now?
If you've followed me so far, you've probably lost your fear of assembly language, picked up some skills and a good part of the instruction set, and are ready to move on. Here are some other good books to pick up:
Mastering Turbo Assembler by Tom Swan
Howard W. Sams & Co., 1989 ISBN 0-672-48435-8
Tom's intermediate-level assembly volume is a natural next step if you're working with the Borland tools. There's no similarly good book on Microsoft's MASM, but much of what Tom discusses in his book applies to MASM as well.
Mastering Turbo Debugger by Tom Swan
Howard W. Sams & Co, 1990 ISBN 0-672-48454-4
This is the only good book on debugging ever published, and for what I consider an advanced topic it's remarkably approachable. Again, it focuses on the Borland tools, but Tom's higher-level strategies for finding and nuking bugs in your code is absolutely essential reading, no matter what assembler you're using, now or at any time in the future.
PC Magazine Programmer's Technical Reference. The Processor and Coprocessor
Robert L. Hummel Ziff-Davis Press, 1992
ISBN 1-56276-016-5
This is not a tutorial but a reference on all of Intel's x86 processors, and it's by far the best one ever written or likely to be written for some time. It has the best
discussion of that mysterious protected mode that I've ever seen, and its description of the individual assembly instructions is wonderfully crafted. I'm tempted to have my own copy taken apart and rebound as hardcover—if I don't, it's going to fall to pieces any day now!
PC TECHNIQUES
7721 E. Gray Road #204
Scottsdale AZ 85260
(602) 483-0192
This is the programmers' magazine that I own and publish, and we cover assembly language in every issue. Tom Swan writes a column on Windows programming, and Michael Abrash writes about high-performance C and assembly coding. Other industry powers write on their own areas of expertise, and there is probably something of interest to you in every issue.
But don't take my word for it. I only work here. Send the card from the back of this book in right now, and don't miss another issue!
12.2 Stepping Off Square One
OK—with a couple of new books in hand and good night's sleep behind you, strike out on your own a little. Set yourself a goal, and try to achieve it: something tough, say, an assembly-language utility that locates all files anywhere on a hard disk drive with a given ambiguous filename. That's ambitious, and will take some research and study and (perhaps) a few false starts. But you can do it, and once you do it you'll be a real journeyman assembly-language programmer.
Becoming a master takes work, and time. Michael Abrash's massive Zen of Assembly Language (now out of print but to be republished soon) is a compilation of the "secret" knowledge of a programming master. It's not easy reading, but it will give you a good idea where your mind has to be to consider yourself an expert assembly-language programmer.
Keep programming. Michael can show you things that would have taken you years to discover on your own, but they won't stick in your mind unless you use them. Set yourself a real challenge, something that has to be both correct and fast: rotate graphics objects in 3-D, transfer data through a serial port at 19,200 bits per second, things like that.
You can do it.
Coming to believe the truth in that statement is the essence of stepping away from Square One.
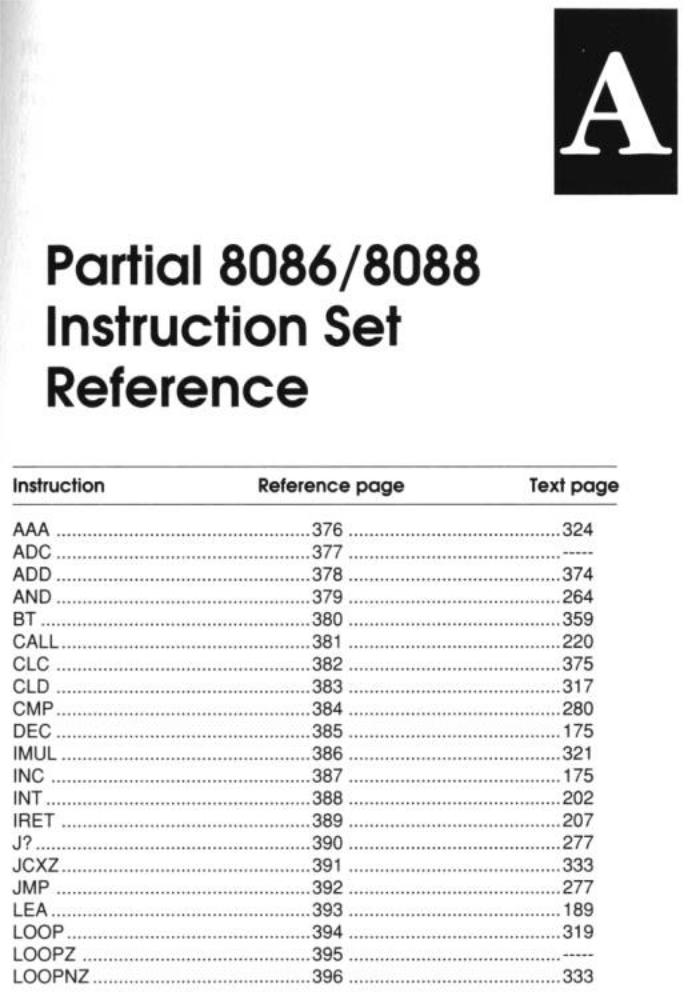

Instruction |
Reference page |
Text page |
MOV................................................ |
397......................................... |
155 |
NEG................................................. |
399......................................... |
169 |
NOP................................................. |
400.............................................. |
|
NOT................................................. |
401 ......................................... |
178 |
OR................................................... |
402......................................... |
266 |
POP................................................. |
403......................................... |
196 |
POPA............................................... |
404......................................... |
348 |
POPF............................................... |
405......................................... |
196 |
PUSH............................................... |
406......................................... |
194 |
PUSHF............................................ |
407......................................... |
194 |
PUSHA............................................ |
408......................................... |
348 |
RET................................................. |
409......................................... |
220 |
ROL................................................. |
410......................................... |
-— |
ROR................................................. |
411 ......................................... |
— |
SBB................................................. |
412......................................... |
----- |
SHL.................................................. |
413......................................... |
269 |
SHR................................................. |
414......................................... |
269 |
STC................................................. |
415.............................................. |
|
STD................................................. |
416......................................... |
317 |
STOS............................................... |
417......................................... |
314 |
SUB................................................. |
418......................................... |
289 |
XCHG.............................................. |
419......................................... |
161 |
XOR., |
...420.. |
...266 |
Notes on the Instruction Set Reference
Instruction operands
When an instruction takes two operands, the destination operand is the one on the left, and the source operand is the one on the right. In general, when a result is produced by an instruction, the result replaces the destination operand. For example, in this instruction:
ADD BX,SI
the BX register is added to the SI register, and the sum is then placed in the BX register, overwriting whatever was in BX before the addition.
Flag results
Each instruction contains a flag summary that looks like this (the asterisks will vary from instruction to instruction):
O D I T S Z A P C |
OF: |
Overflow flag |
TF: |
Trap flag |
|
F F F F F F F F F |
DF: |
Direction flag |
SF: |
Sign flag |
|
* |
* * * * * |
IF. |
interrupt flag |
ZF: |
Zero flag |
AF: Aux carry PF: Parity flag CF: Carry flag
The nine flags are all represented here. An asterisk indicates that the instruction on that page affects that flag. If a flag is affected at all (that is, if it has an asterisk beneath it) it will be affected according to these rules:
OF: Set if the result is too large to fit in the destination operand. DF: Set by the STD instruction; cleared by CLD.
IF: Set by the STI instruction; cleared by CLI.
TF: For debuggers; not used in normal programming and may be ignored.
SF: Set when the sign of the result forces the destination operand to become negative. ZF: Set if the result of an operation is zero. If the result is non-zero, ZF is cleared.
AF: "Auxiliary carry" used for 4-bit BCD math. Set when an operation causes a carry out of a 4-bit BCD quantity.
PF: Set if the number of 1 bits in the low byte of the result is even; cleared if the number of 1 bits in the low byte of the result is odd. Used in data communications applications but little else.
CF: Set if the result of an add or shift operation "carries out" a bit beyond the destination operand; otherwise cleared. May be manually set by STC and manually cleared by CLC when CF must be in a known state before an operation begins.
Some instructions force certain flags to become undefined. When this is the case, it will be noted under "Notes." "Undefined" means don't count on it being in any particular state.
AAA Adjust AL after BCD addition
Flags affected:
ODITSZAPC OF: Overflow flag TF: Trap flag AF: Aux carry
FFFFFFFFF |
DF: |
Direction flag |
SF: Sign flag |
PF: |
Parity flag |
* * |
IF: |
Interrupt flag |
ZF: Zero flag |
CF: |
Carry flag |
Legal forms:
AAA
Examples:
AAA
Notes:
AAA makes an addition "come out right" in AL when what you're adding are BCD values rather than ordinary binary values. Note well that AAA does not perform the arithmetic itself, but is a "postprocessor" after ADD or ADC. AL is an implied operand and may not be explicitly stated—so make sure that the preceding ADD or ADC instruction leaves its results in AL!
A BCD digit is a byte with the high 4 bits set to 0, and the low 4 bits containing a digit from 0 to 9. AAA will yield garbage results if the preceding ADD or ADC acted upon one or both operands with values greater than 09.
After the addition of two legal BCD values, AAA will adjust a non-BCD result (that is, a result greater than 09 in AL) to a value between 0 and 9. This is called a decimal carry, since it is the carry of a BCD digit and not simply the carry of a binary bit.
For example, if ADD added 08 and 04 (both legal BCD values) to produce OC in AL, AAA will take the OC and adjust it to 02. The decimal carry goes to AH, not to the upper 4 bits of AL, which are always cleared to 0 by AAA.
If the preceding ADD or ADC resulted in a decimal carry, (as in the example above) both CF and AF are set to 1 and AH is incremented by 1. Otherwise, AH is not incremented and CF and AF are cleared to 0.
This instruction is subtle. See the detailed discussion in Section 10.3.
r8 |
- |
AL |
AH BL |
BH CL CH DL DH |
r16 |
= AX BX CX DX BP SP SI DI |
|||
sr - |
CS |
DS SS |
ES |
m16 |
- |
16-bit |
memory data |
||
m8 |
- |
8-bit |
memory data |
||||||
18 |
- |
8-bit |
immediate data |
i16 |
- |
16-bit |
immediate data |
||
d8 - 8-bit signed displacement |
d16 - 16-bit signed displacement |
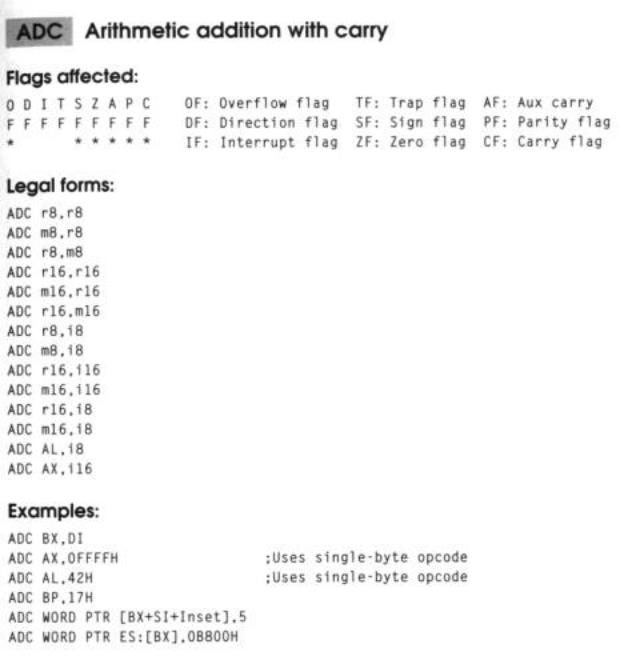
Notes:
ADC adds the source operand and the carry flag to the destination operand, and after the operation the result replaces the destination operand. The add is an arithmetic add, and the carry allows multiple-precision additions across several registers or memory locations. (To add without taking the carry flag into account, use the ADD instruction.) All affected flags are set according to the operation. Most importantly, if the result does not fit into the destination operand, the carry flag is set to 1.
r8 = AL AH BL BH CL CH DL DH |
r16 = AX BX CX DX BP SP SI DI |
|||
sr |
= |
CS DS |
SS ES |
m16 = 16-bit memory data |
m8 |
= |
8-bit |
memory data |
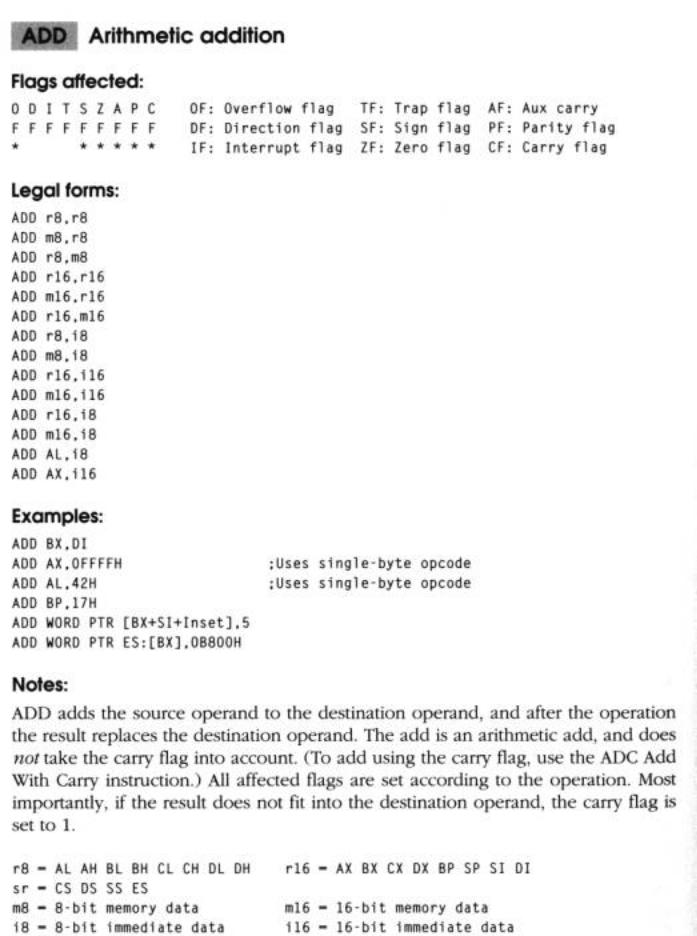
18 = 8-bit immediate data |
116 = 16-bit immediate data |
d8 = 8-bit signed displacement |
d16 =16-bit signed displacement |
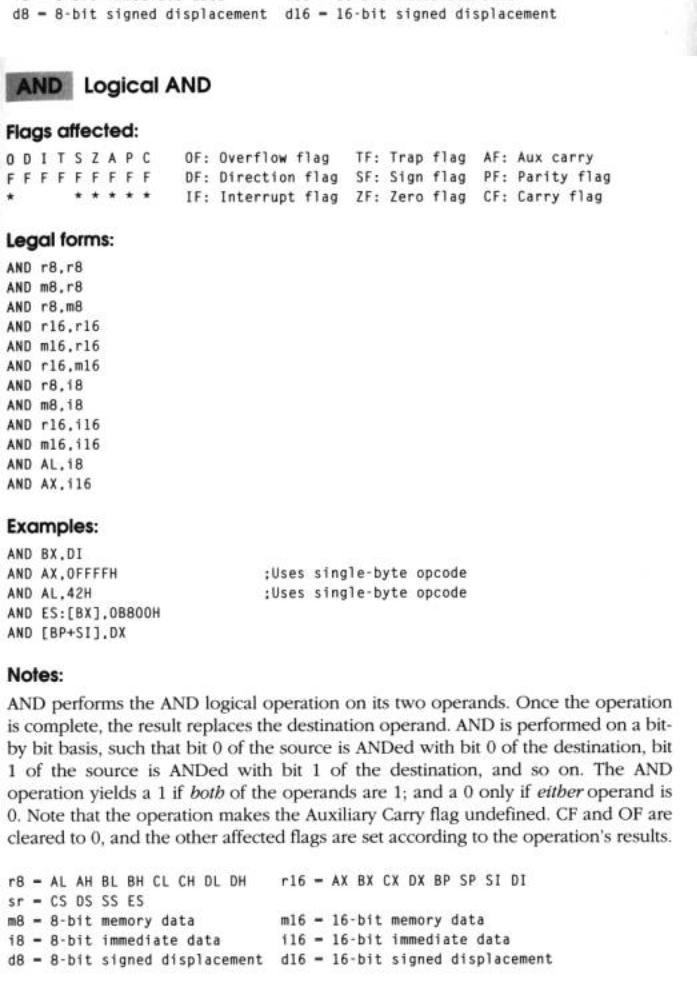