
Visual CSharp 2005 Recipes (2006) [eng]
.pdf
88 C H A P T E R 3 ■ A P P L I C AT I O N D O M A I N S, R E F L E C T I O N, A N D M E TA D ATA
How It Works
The first step in creating an object using reflection is to obtain a Type object that represents the type you want to instantiate. (See recipe 3-10 for details.) Once you have a Type instance, call its GetConstructor method to obtain a ConstructorInfo representing one of the type’s constructors. The most commonly used overload of the GetConstructor method takes a Type array argument and returns a ConstructorInfo representing the constructor that takes the number, order, and type of arguments specified in the Type array. To obtain a ConstructorInfo representing a parameterless (default) constructor, pass an empty Type array (use the static field Type.EmptyTypes or new Type[0]); don’t use null, or GetConstructor will throw a System.ArgumentNullException. If GetConstructor cannot find a constructor with a signature that matches the specified arguments, it will return null.
Once you have the desired ConstructorInfo, call its Invoke method. You must provide an object array containing the arguments you want to pass to the constructor. Invoke instantiates the new object and returns an object reference to it, which you must cast to the appropriate type.
Reflection functionality is commonly used to implement factories in which you use reflection to instantiate concrete classes that either extend a common base class or implement a common interface. Often both an interface and a common base class are used. The abstract base class implements the interface and any common functionality, and then each concrete implementation extends the base class.
No mechanism exists to formally declare that each concrete class must implement constructors with specific signatures. If you intend third parties to implement concrete classes, your documentation must specify the constructor signature called by your factory. A common approach to avoid this problem is to use a default (empty) constructor and configure the object after instantiation using properties and methods.
The Code
The following code fragment demonstrates how to instantiate a System.Text.StringBuilder object using reflection and how to specify the initial content for the StringBuilder (a string) and its capacity (an int):
using System; using System.Text;
using System.Reflection;
namespace Apress.VisualCSharpRecipes.Chapter03
{
class Recipe03_12
{
public static StringBuilder CreateStringBuilder()
{
//Obtain the Type for the StringBuilder class. Type type = typeof(StringBuilder);
//Create a Type[] containing Type instances for each
//of the constructor arguments - a string and an int. Type[] argTypes = new Type[] { typeof(System.String),
typeof(System.Int32) };
//Obtain the ConstructorInfo object.
ConstructorInfo cInfo = type.GetConstructor(argTypes);
// Create an object[] containing the constructor arguments. object[] argVals = new object[] { "Some string", 30 };
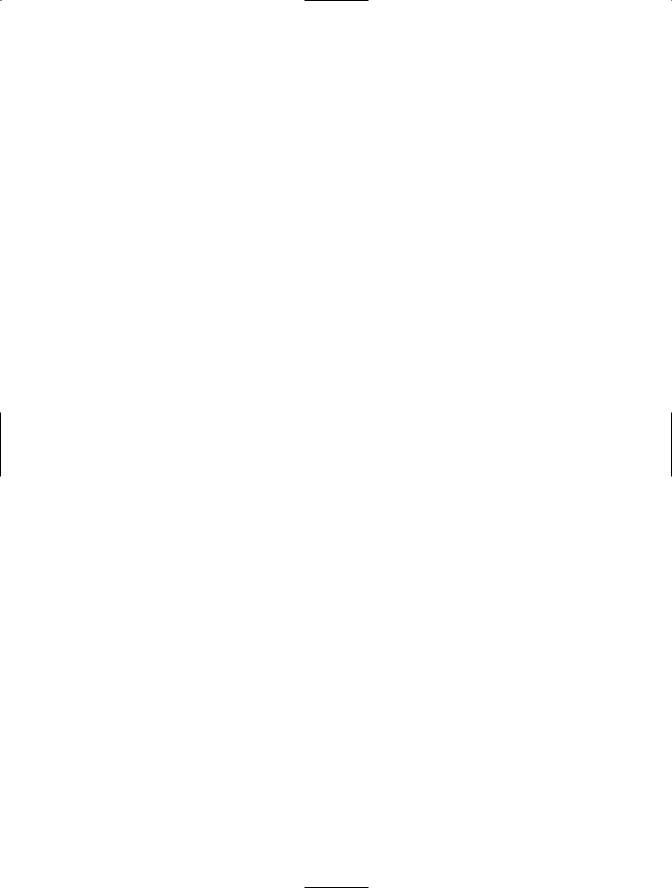
C H A P T E R 3 ■ A P P L I C AT I O N D O M A I N S, R E F L E C T I O N, A N D M E TA D ATA |
89 |
// Create the object and cast it to StringBuilder. StringBuilder sb = (StringBuilder)cInfo.Invoke(argVals);
return sb;
}
}
}
The following code demonstrates a factory to instantiate objects that implement the IPlugin interface (first used in recipe 3-7):
using System;
using System.Reflection;
namespace Apress.VisualCSharpRecipes.Chapter03
{
//A common interface that all plug-ins must implement. public interface IPlugin
{
string Description { get; set; } void Start();
void Stop();
}
//An abstract base class from which all plug-ins must derive. public abstract class AbstractPlugin : IPlugin
{
//Hold a description for the plug-in instance.
private string description = "";
//Sealed property to get the plug-in description. public string Description
{
get { return description; } set { description = value; }
}
//Declare the members of the IPlugin interface as abstract. public abstract void Start();
public abstract void Stop();
}
// A simple IPlugin implementation to demonstrate the PluginFactory class. public class SimplePlugin : AbstractPlugin
{
//Implement Start method. public override void Start()
{
Console.WriteLine(Description + ": Starting...");
}
//Implement Stop method.
public override void Stop()
{
Console.WriteLine(Description + ": Stopping...");
}
}
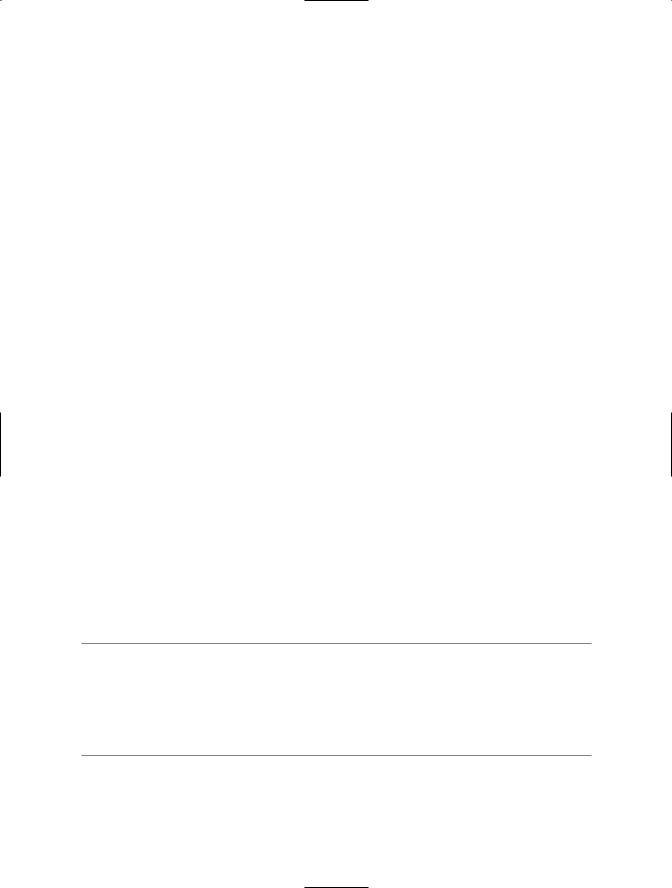
90 C H A P T E R 3 ■ A P P L I C AT I O N D O M A I N S, R E F L E C T I O N, A N D M E TA D ATA
// A factory to instantiate instances of IPlugin. public sealed class PluginFactory
{
public static IPlugin CreatePlugin(string assembly, string pluginName, string description)
{
// Obtain the Type for the specified plug-in.
Type type = Type.GetType(pluginName + ", " + assembly);
// Obtain the ConstructorInfo object.
ConstructorInfo cInfo = type.GetConstructor(Type.EmptyTypes);
//Create the object and cast it to StringBuilder. IPlugin plugin = cInfo.Invoke(null) as IPlugin;
//Configure the new IPlugin.
plugin.Description = description;
return plugin;
}
public static void Main(string[] args)
{
// Instantiate a |
new IPlugin using the PluginFactory. |
|
IPlugin plugin = |
PluginFactory.CreatePlugin( |
|
"Recipe03-12", // Private assembly name |
||
"Apress.VisualCSharpRecipes.Chapter03.SimplePlugin", |
||
// Plug-in |
class name |
|
"A Simple Plugin" |
// Plug-in instance description |
|
); |
|
|
//Start and stop the new plug-in. plugin.Start();
plugin.Stop();
//Wait to continue.
Console.WriteLine("\nMain method complete. Press Enter."); Console.ReadLine();
}

C H A P T E R 3 ■ A P P L I C AT I O N D O M A I N S, R E F L E C T I O N, A N D M E TA D ATA |
91 |
3-13. Create a Custom Attribute
Problem
You need to create a custom attribute.
Solution
Create a class that derives from the abstract base class System.Attribute. Implement constructors, fields, and properties to allow users to configure the attribute. Use System.AttributeUsageAttribute to define the following:
•Which program elements are valid targets of the attribute
•Whether you can apply more than one instance of the attribute to a program element
•Whether the attribute is inherited by derived types
How It Works
Attributes provide a mechanism for associating declarative information (metadata) with program elements. This metadata is contained in the compiled assembly, allowing programs to retrieve it through reflection at runtime without creating an instance of the type. (See recipe 3-14 for more details.) Other programs, particularly the CLR, use this information to determine how to interact with and manage program elements.
To create a custom attribute, derive a class from the abstract base class System.Attribute. Custom attribute classes by convention should have a name ending in Attribute (but this is not essential). A custom attribute must have at least one public constructor—the automatically generated default constructor is sufficient. The constructor parameters become the attribute’s mandatory (or positional) parameters. When you use the attribute, you must provide values for these parameters in the order they appear in the constructor. As with any other class, you can declare more than one constructor, giving users of the attribute the option of using different sets of positional parameters when applying the attribute. Any public nonconstant writable fields and properties declared by an attribute are automatically exposed as named parameters. Named parameters are optional and are specified in the format of name-value pairs where the name is the property or field name. The following example will clarify how to specify positional and named parameters.
To control how and where a user can apply your attribute, apply the attribute
AttributeUsageAttribute to your custom attribute. AttributeUsageAttribute supports the one positional and two named parameters described in Table 3-3. The default values specify the value that is applied to your custom attribute if you do not apply AttributeUsageAttribute or do not specify a value for that particular parameter.
Table 3-3. Members of the AttributeUsage Type
Parameter |
Type |
Description |
Default |
ValidOn |
Positional |
A member of the |
AttributeTargets.All |
|
|
System.AttributeTargets |
|
|
|
enumeration that identifies |
|
|
|
the program elements on |
|
|
|
which the attribute is valid |
|
|
|
|
(Continued ) |
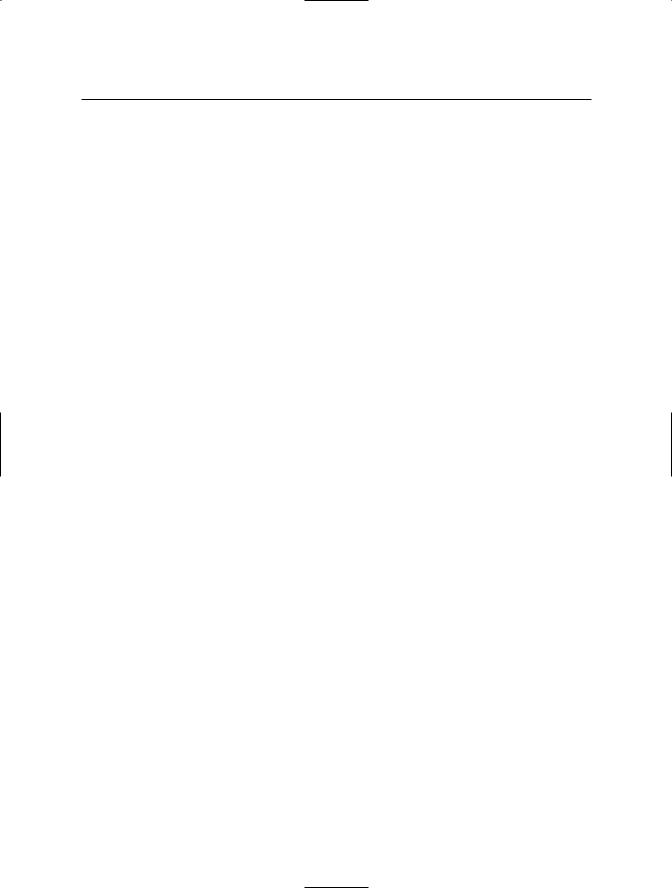
92 C H A P T E R 3 ■ A P P L I C AT I O N D O M A I N S, R E F L E C T I O N, A N D M E TA D ATA
Table 3-3. Continued
Parameter |
Type |
Description |
Default |
AllowMultiple |
Named |
Whether the attribute can be |
False |
|
|
specified more than once for |
|
|
|
a single element |
|
Inherited |
Named |
Whether the attribute is |
True |
|
|
inherited by derived classes |
|
|
|
or overridden members |
|
|
|
|
|
The Code
The following example shows a custom attribute named AuthorAttribute, which you can use to identify the name and company of the person who created an assembly or a class. AuthorAttribute declares a single public constructor that takes a string containing the author’s name. This means users of AuthorAttribute must always provide a positional string parameter containing the author’s name. The Company property is public, making it an optional named parameter, but the Name property is read-only—no set accessor is declared—meaning that it isn’t exposed as a named parameter.
using System;
namespace Apress.VisualCSharpRecipes.Chapter03
{
[AttributeUsage(AttributeTargets.Class | AttributeTargets.Assembly, AllowMultiple = true, Inherited = false)]
public class AuthorAttribute : System.Attribute
{
private |
string |
company; |
// |
Creator's |
company |
private |
string |
name; |
// |
Creator's |
name |
//Declare a public constructor. public AuthorAttribute(string name)
{
this.name = name; company = "";
}
//Declare a property to get/set the company field. public string Company
{
get { return company; } set { company = value; }
}
//Declare a property to get the internal field. public string Name
{
get { return name; }
}
}
}
Usage
The following example demonstrates how to decorate types with AuthorAttribute:

C H A P T E R 3 ■ A P P L I C AT I O N D O M A I N S, R E F L E C T I O N, A N D M E TA D ATA |
93 |
using System;
//Declare Allen as the assembly author. Assembly attributes
//must be declared after using statements but before any other.
//Author name is a positional parameter.
//Company name is a named parameter.
[assembly: Apress.VisualCSharpRecipes.Chapter03.Author("Allen", Company = "Principal Objective Ltd.")]
namespace Apress.VisualCSharpRecipes.Chapter03
{
// Declare a class authored by Allen.
[Author("Allen", Company = "Principal Objective Ltd.")] public class SomeClass
{
// Class implementation.
}
// Declare a class authored by Lena. [Author("Lena")]
public class SomeOtherClass
{
// Class implementation.
}
}
3-14. Inspect the Attributes of a Program Element
Using Reflection
Problem
You need to use reflection to inspect the custom attributes applied to a program element.
Solution
All program elements implement the System.Reflection.ICustomAttributeProvider interface. Call the IsDefined method of the ICustomAttributeProvider interface to determine whether an attribute is applied to a program element, or call the GetCustomAttributes method of the ICustomAttributeProvider interface to obtain objects representing the attributes applied to the program element.
How It Works
All the classes that represent program elements implement the ICustomAttributeProvider interface. This includes Assembly, Module, Type, EventInfo, FieldInfo, PropertyInfo, and MethodBase. MethodBase has two further subclasses: ConstructorInfo and MethodInfo. If you obtain instances of any of these classes, you can call the method GetCustomAttributes, which will return an object array containing the custom attributes applied to the program element. The object array contains only custom attributes, not those contained in the .NET Framework base class library.
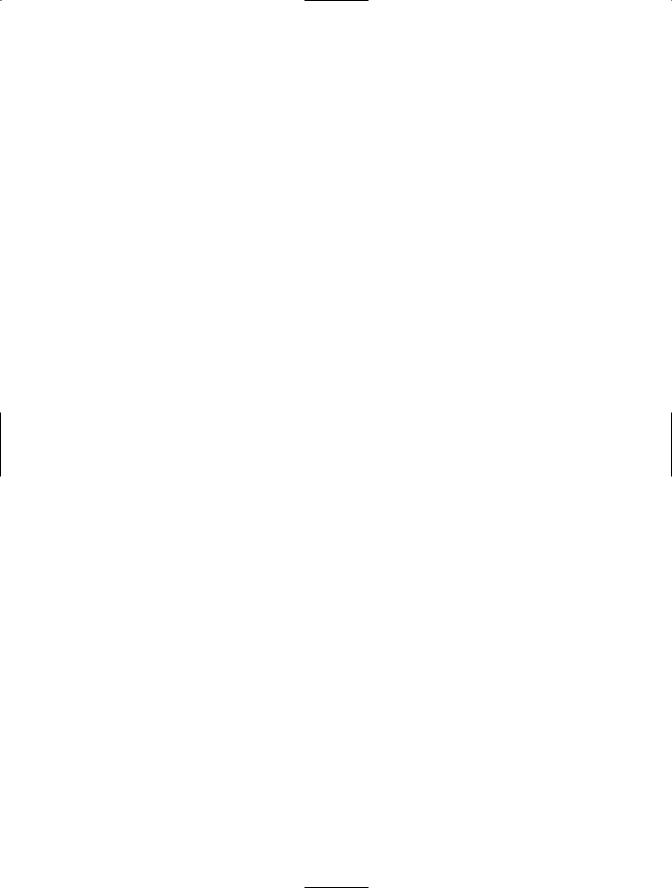
94 C H A P T E R 3 ■ A P P L I C AT I O N D O M A I N S, R E F L E C T I O N, A N D M E TA D ATA
The GetCustomAttributes method provides two overloads. The first takes a bool that controls whether GetCustomAttributes should return attributes inherited from parent classes. The second GetCustomAttributes overload takes an additional Type argument that acts as a filter, resulting in GetCustomAttributes returning only attributes of the specified type.
Alternatively, you can call the IsDefined method. IsDefined provides a single overload that takes two arguments. The first argument is a System.Type object representing the type of attribute you are interested in, and the second is a bool that indicates whether IsDefined should look for inherited attributes of the specified type. IsDefined returns a bool indicating whether the specified attribute is applied to the program element and is less expensive than calling the GetCustomAttributes method, which actually instantiates the attribute objects.
The Code
The following example uses the custom AuthorAttribute declared in recipe 3-13 and applies it to the Recipe03-14 class. The Main method calls the GetCustomAttributes method, filtering the attributes so that the method returns only AuthorAttribute instances. You can safely cast this set of attributes to AuthorAttribute references and access their members without needing to use reflection.
using System;
namespace Apress.VisualCSharpRecipes.Chapter03
{
[Author("Lena")]
[Author("Allen", Company = "Principal Objective Ltd.")] class Recipe03_15
{
public static void Main()
{
//Get a Type object for this class. Type type = typeof(Recipe03_15);
//Get the attributes for the type. Apply a filter so that only
//instances of AuthorAttribute are returned.
object[] attrs = type.GetCustomAttributes(typeof(AuthorAttribute), true);
//Enumerate the attributes and display their details. foreach (AuthorAttribute a in attrs) {
Console.WriteLine(a.Name + ", " + a.Company);
}
//Wait to continue.
Console.WriteLine("\nMain method complete. Press Enter."); Console.ReadLine();
}
}
}
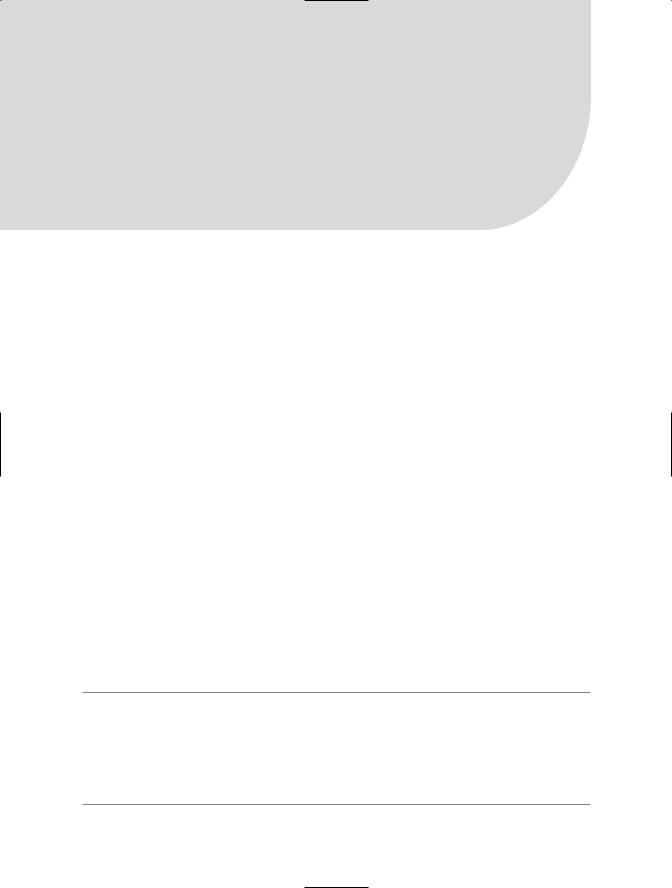
C H A P T E R 4
■ ■ ■
Threads, Processes, and
Synchronization
One of the strengths of the Microsoft Windows operating system is that it allows many programs (processes) to run concurrently and allows each process to perform many tasks concurrently (using multiple threads). When you run an executable application, a new process is created. The process isolates your application from other programs running on the computer. The process provides the application with its own virtual memory and its own copies of any libraries it needs to run, allowing your application to execute as if it were the only application running on the machine.
Along with the process, an initial thread is created that runs your Main method. In single-threaded applications, this one thread steps through your code and sequentially performs each instruction. If an operation takes time to complete, such as reading a file from the Internet or doing a complex calculation, the application will be unresponsive (will block) until the operation is finished, at which point the thread will continue with the next operation in your program.
To avoid blocking, the main thread can create additional threads and specify which code each should start running. As a result, many threads may be running in your application’s process, each running (potentially) different code and performing different operations seemingly simultaneously. In reality, unless you have multiple processors (or a single multicore processor) in your computer, the threads are not really running simultaneously. Instead, the operating system coordinates and schedules the execution of all threads across all processes; each thread is given a tiny portion (or time slice) of the processor’s time, which gives the impression they are executing at the same time.
The difficulty of having multiple threads executing within your application arises when those threads need to access shared data and resources. If multiple threads are changing an object’s state or writing to a file at the same time, your data will quickly become corrupt. To avoid problems, you must synchronize the threads to make sure they each get a chance to access the resource, but only one at a time. Synchronization is also important when waiting for a number of threads to reach a certain point of execution before proceeding with a different task and for controlling the number of threads that are at any given time actively performing a task—perhaps processing requests from client applications.
■Note Although it will not affect your multithreaded programming in Visual C#, it is worth noting that an operating system thread has no fixed relationship to a managed thread. The runtime host—the managed code that loads and runs the common language runtime (CLR)—controls the relationship between managed and unmanaged threads. A sophisticated runtime host, such as Microsoft SQL Server 2005, can schedule many managed threads against the same operating system thread or can perform the actions of a managed thread using different operating system threads.
95
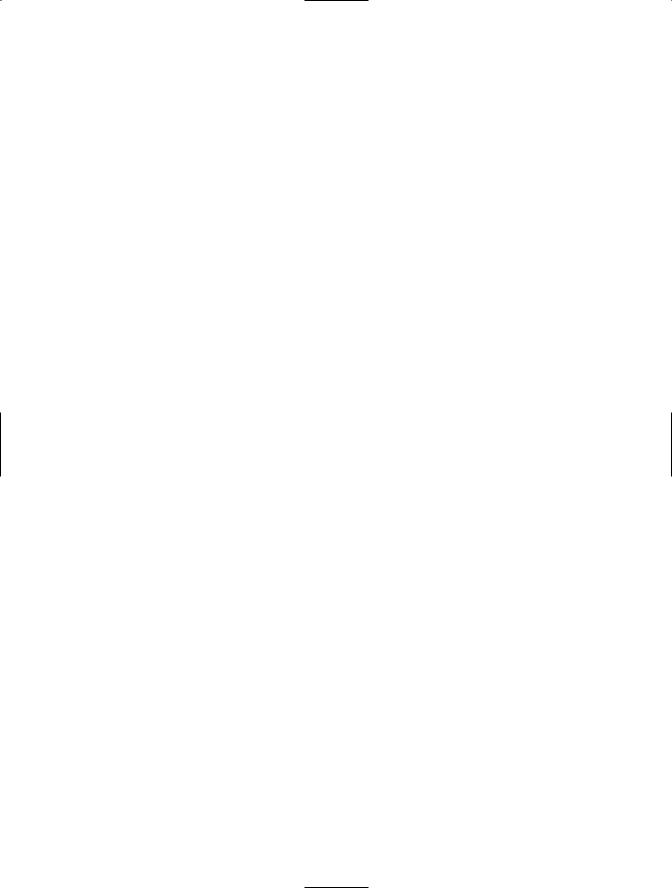
96 C H A P T E R 4 ■ T H R E A D S, P R O C E S S E S, A N D S Y N C H R O N I Z AT I O N
This chapter describes how to control processes and threads in your own applications using the features provided by Visual C# and the Microsoft .NET Framework class library. Specifically, the recipes in this chapter describe how to do the following:
•Execute code in independent threads using features including the thread pool, asynchronous method invocation, and timers (recipes 4-1 through 4-6)
•Synchronize the execution of multiple threads using a host of synchronization techniques including monitors, events, mutexes, and semaphores (recipes 4-7 and 4-11)
•Terminate threads and know when threads have terminated (recipes 4-12 and 4-13)
•Create thread-safe instances of the .NET collection classes (recipe 4-14)
•Start and stop applications running in new processes (recipes 4-15 and 4-16)
•Ensure that only one instance of an application is able to run at any given time (recipe 4-17)
As you will see in this chapter, delegates are used extensively in multithreaded programs to wrap the method that a thread should execute or that should act as a callback when an asynchronous operation is complete. Prior to C# 2.0, it would be necessary to
1.Declare a method that matches the signature of the required delegate,
2.Create a delegate instance of the required type by passing it the name of the method, and
3.Pass the delegate instance to the new thread or asynchronous operation.
C# 2.0 adds two important new features that simplify the code you must write when using delegates:
•First, you no longer need to create a delegate instance to wrap the method you want to execute. You can pass a method name where a delegate is expected, and as long as the method signature is correct, the compiler infers the need for the delegate and creates it automatically.
This is a compiler enhancement only—the intermediate language (IL) generated is as if the appropriate delegate had been instantiated. Recipes 4-1 and 4-2 (along with many others) demonstrate how to use this capability.
•Second, you no longer need to explicitly declare a method for using with the delegate. Instead, you can provide an anonymous method wherever a delegate is required. In effect, you actually write the method code at the point where you would usually pass the method name (or delegate instance). The only difference is that you use the keyword delegate instead of giving the method a name. This approach can reduce the need to implement methods solely for use as callbacks and event handlers, which reduces code clutter, but it can quickly become confusing if the anonymous method is longer than a couple of lines of code. Recipes 4-3 and 4-4 demonstrate how to use anonymous methods.
4-1. Execute a Method Using the Thread Pool
Problem
You need to execute a task using a thread from the runtime’s thread pool.
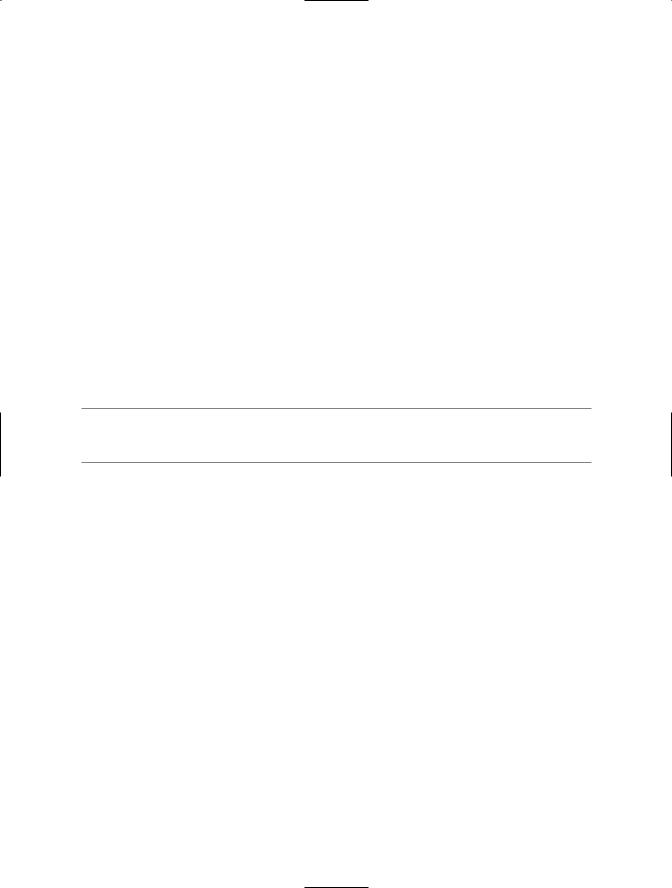
C H A P T E R 4 ■ T H R E A D S, P R O C E S S E S, A N D S Y N C H R O N I Z AT I O N |
97 |
Solution
Declare a method containing the code you want to execute. The method’s signature must match that defined by the System.Threading.WaitCallback delegate; that is, it must return void and take a single object argument. Call the static method QueueUserWorkItem of the System.Threading.
ThreadPool class, passing it your method name. The runtime will queue your method and execute it when a thread-pool thread becomes available.
How It Works
Applications that use many short-lived threads or maintain large numbers of concurrent threads can suffer performance degradation because of the overhead associated with the creation, operation, and destruction of threads. In addition, it is common in multithreaded systems for threads to sit idle a large portion of the time while they wait for the appropriate conditions to trigger their execution. Using a thread pool provides a common solution to improve the scalability, efficiency, and performance of multithreaded systems.
The .NET Framework provides a simple thread-pool implementation accessible through the members of the ThreadPool static class. The QueueUserWorkItem method allows you to execute
a method using a thread-pool thread by placing a work item on a queue. As a thread from the thread pool becomes available, it takes the next work item from the queue and executes it. The thread performs the work assigned to it, and when it is finished, instead of terminating, the thread returns to the thread pool and takes the next work item from the work queue.
■Tip If you need to execute a method with a signature that does not match the WaitCallback delegate, then you must use one of the other techniques described in this chapter. See recipe 4-2 or 4-6.
The Code
The following example demonstrates how to use the ThreadPool class to execute a method named DisplayMessage. The example passes DisplayMessage to the thread pool twice, first with no arguments and then with a MessageInfo object, which allows you to control which message the new thread will display.
using System;
using System.Threading;
namespace Apress.VisualCSharpRecipes.Chapter04
{
class Recipe04_01
{
//A private class used to pass data to the DisplayMessage method when it is
//executed using the thread pool.
private class MessageInfo
{
private int iterations; private string message;
// A constructor that takes configuration settings for the thread. public MessageInfo(int iterations, string message)
{
this.iterations = iterations; this.message = message;
}