
3D Game Programming All In One (2004)
.pdf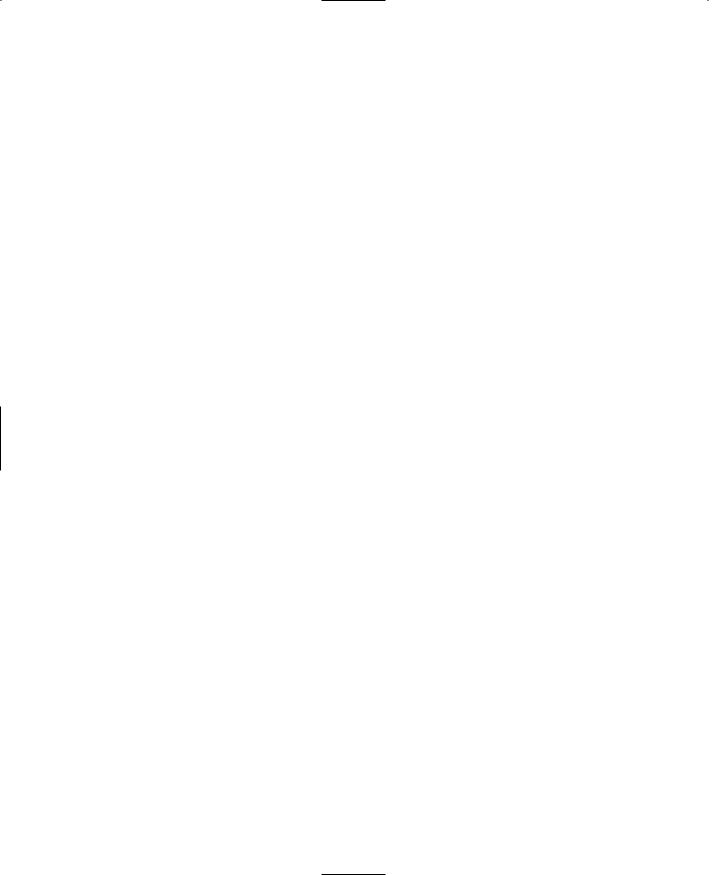
108 |
Chapter 3 ■ 3D Programming Concepts |
||
|
|
// An example function which creates a new TestShape object |
|
|
|
%shape = new Item() { |
|
|
|
datablock = TestShape; |
|
|
|
rotation = "0 0 1 0"; // initialize the values |
|
|
|
|
// to something meaningful |
|
|
}; |
|
|
|
MissionCleanup.add(%shape); |
|
|
|
// Player setup |
|
|
|
%shape.setTransform(" - 90 - 2 20 0 0 1 0"); |
|
|
|
echo("Inserting Shape " @ %shape); |
|
|
|
return %shape; |
|
|
} |
|
|
|
function MoveShape(%shape, %dist) |
||
|
// |
---------------------------------------------------- |
|
|
// |
moves the %shape by %dist amount |
|
|
// ---------------------------------------------------- |
|
|
|
{ |
|
|
|
|
%xfrm = %shape.getTransform(); |
|
|
|
%lx = getword(%xfrm,0); // get the current transform values |
|
|
|
%ly = getword(%xfrm,1); |
|
|
|
%lz = getword(%xfrm,2); |
|
|
|
%lx += %dist; |
// adjust the x axis position |
%shape.setTransform(%lx SPC %ly SPC %lz SPC "0 0 1 0");
}
function DoMoveTest()
// ----------------------------------------------------
//a function to tie together the instantiation
//and the movement in one easy to type function
//call.
// ----------------------------------------------------
{
%ms = InsertTestShape();
MoveShape(%ms,15);
}
In this module there are three functions and a datablock definition. A datablock is a construct that is used to organize properties for objects together in a way that is important for the server. We will cover datablocks in more detail in a later chapter. The datablock begins with the line datablock ItemData(TestShape)—it specifies static properties of the Item class that can't be changed while the game is running. The most important part of the preceding
Team LRN
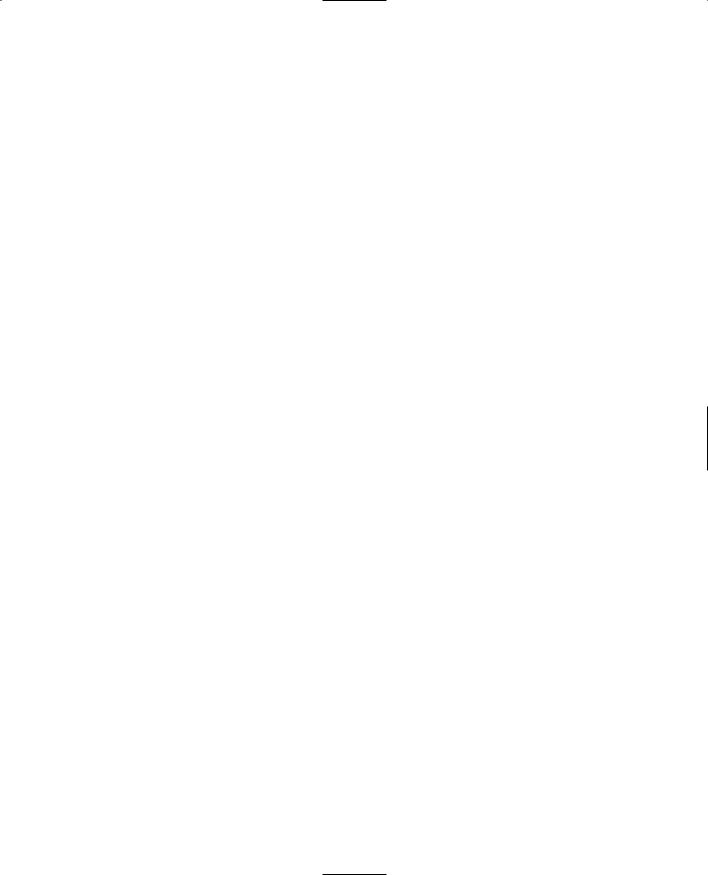
3D Programming |
109 |
datablock is the shapeFile property, which tells Torque where to find the model that will be used to represent the object. The mass and friction values, as mentioned previously, prevent the item from sliding down hills because of the pernicious tug of gravity.
The function InsertTestShape() Item(). It specifies the TestShape tion to some sensible values.
creates a new instance of TestShape with the call to new datablock, defined earlier, and then sets the object's rota-
Next, MissionCleanup.add(%shape); adds the shape instance to a special mission-related group maintained by Torque. When the mission ends, objects assigned to this group are deleted from memory (cleaned up) before a new mission is started.
After that, the program sets the initial location of the object by setting the transform.
Next, the echo statement prints the shape's handle to the console.
Finally, the shape's handle (ID number) is returned from the function. This allows us to save the handle in a variable when we call this function, so that we can refer to this same item instance at a later time.
The function MoveShape accepts a shape handle and a distance as arguments, and uses these to move whatever shape the handle indicates.
First, it gets the current position of the shape using the %shape.getTransform() method of the Item class.
Next, the program employs the getword() function to extract the parts of the transform string that are of interest and store them in local variables. We do this because, for this particular program, we want to move the shape in the X-axis. Therefore, we strip out all three axes and increment the X value by the distance that the object should move. Then we prepend all three axis values to a dummy rotation and set the item's transform to be this new string value. This last bit is done with the %shape.setTransform() statement.
The DoMoveTest() function is like a wrapper folded around the other functions. When we call this function, first it inserts the new instance of the shape object using the InsertTestShape() function and saves the handle to the new object in the variable %ms. It then calls the MoveShape() function, specifying which shape to move by passing in the handle to the shape as the first argument and also indicating the distance with the second argument.
To use the program, follow these steps:
1.Make sure you've saved the file as 3DGPAi1\CH3\moveshape.cs.
2.Run the Chapter 3 demo using the shortcut in the 3DGPAi1 folder.
3.Press the Start button when the CH3 demo screen comes up.
4.Make sure you don't move your player-character after it spawns into the game world.
Team LRN

110 Chapter 3 ■ 3D Programming Concepts
5.Bring up the console window by pressing the Tilde key.
6.Type in the following, and press Enter after the semicolon:
exec("CH3/moveshape.cs");
You should get a response in the console window similar to this:
Compiling CH3/moveshape.cs...
Loading compiled script CH3/moveshape.cs.
This means that the Torque Engine has compiled your program and then loaded it into memory. The datablock definition and the three functions are in memory, waiting with barely suppressed anticipation for your next instruction.
t i p
About those slashes… You've probably noticed that when you see the file names and paths written out, the back slash ("\") is used, and when you type in those same paths in the console window, the forward slash ("/") is used. This is not a mistake. Torque is a cross-platform program that is available for Macintosh and Linux as well as Windows. It's only on Windows-based systems that back slashes are used—everyone else uses forward slashes.
Therefore, the back slashes for Windows-based paths are the exception here. Just thought I'd clear that up!
7. Type the following into the console window:
$tt = InsertTestShape();
You should see the following response:
Inserting Shape 1388
The number you get may be different—that's not an issue. But it will probably be the same. Take note of the number.
You also may see a warning about not locating a texture—that's of no importance here either.
8.Close the console window. You should see a heart on the ground in front of your player.
9.Type the following into the console:
echo($tt);
Torque will respond by printing the contents of the variable $tt to the console window. It should be the same number that you got as a response after using the InsertTestShape instruction above.
10. Type the following into the console:
MoveShape(%tt,50);
Team LRN
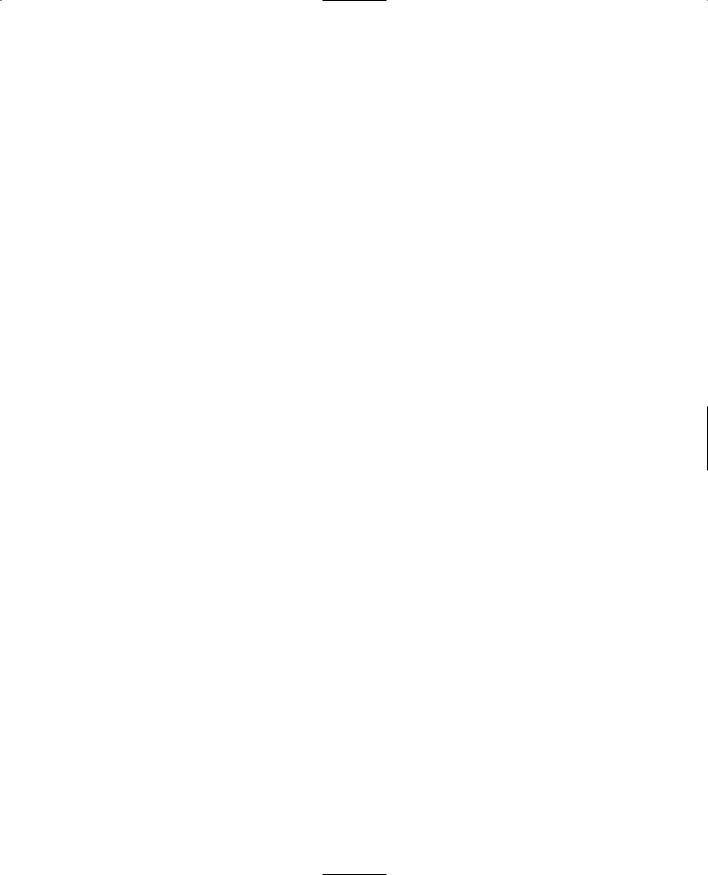
3D Programming |
111 |
11.Press the Tilde key to close the console window. You should see the heart move away from you to the left.
You should be familiar with opening and closing the console window by now, so I won't bother explaining that part in the instruction sequences anymore.
12.Now, type this into the console, and close the console quickly afterward:
DoMoveTest();
What you should see now is the heart dropping from the air to the ground; it then moves away just like the first heart, except not as far this time.
The reason why the heart drops from the air is because the object's initial location was set in the InsertTestShape() function to be -90 -2 20, where the Z value is set to 20 units up. As mentioned earlier, Torque will automatically make objects of the Item class fall under gravity until they hit something that stops the fall. If you don't close the console window quickly enough, you won't see it fall.
Go ahead and experiment with the program. Try moving the item through several axes at once, or try changing the distance.
Programmed Rotation
As you've probably figured out already, we can rotate an object programmatically (or directly, for that matter) using the same setTransform() method that we used to translate an object.
Type the following program and save it as 3DGPAi1\CH3\turnshape.cs.
//========================================================================
//turnshape.cs
//
//This module contains the definition of a test shape.
//It contains functions for placing
//the test shape in the game world and rotating the shape
//========================================================================
datablock ItemData(TestShape)
// ----------------------------------------------------
// Definition of the shape object
// ----------------------------------------------------
{
// Basic Item properties
shapeFile = "~/data/shapes/items/heart.dts"; mass = 1; //we give the shape mass and
friction = 1; // friction to stop the item from sliding
Team LRN

112 Chapter 3 ■ 3D Programming Concepts
// down hills
};
function InsertTestShape()
// ----------------------------------------------------
//Instantiates the test shape, then inserts it
//into the game world roughly in front of
//the player's default spawn location.
// ----------------------------------------------------
{
// An example function which creates a new TestShape object %shape = new Item() {
datablock = TestShape;
rotation = "0 0 1 0"; // initialize the values
// to something meaningful
|
}; |
|
|
MissionCleanup.add(%shape); |
|
|
// Player setup |
|
|
%shape.setTransform(" - 90 - 2 20 0 0 1 0"); |
|
|
echo("Inserting Shape " @ %shape); |
|
|
return %shape; |
|
} |
|
|
function TurnShape(%shape, %angle) |
||
// |
---------------------------------------------------- |
|
// |
turns the %shape by %angle amount. |
|
// ---------------------------------------------------- |
|
|
{ |
|
|
|
%xfrm = %shape.getTransform(); |
|
|
%lx = getword(%xfrm,0); // first, get the current transform values |
|
|
%ly = getword(%xfrm,1); |
|
|
%lz = getword(%xfrm,2); |
|
|
%rx = getword(%xfrm,3); |
|
|
%ry = getword(%xfrm,4); |
|
|
%rz = getword(%xfrm,5); |
|
|
%angle += 1.0; |
|
|
%rd = %angle; |
// Set the rotation angle |
%shape.setTransform(%lx SPC %ly SPC %lz SPC %rx SPC %ry SPC %rz SPC %rd);
}
function DoTurnTest()
Team LRN

3D Programming |
113 |
// ----------------------------------------------------
//a function to tie together the instantiation
//and the movement in one easy to type function
//call.
// ----------------------------------------------------
{
%ts = InsertTestShape();
TurnShape(%ts,30);
}
The program is quite similar to the moveshape.cs program that you were just working with. You can load and run the program in exactly the same way, except that you want to use DoTurnTest() instead of DoMoveTest() and TurnShape() instead of MoveShape().
Things of interest to explore are the variables %rx, %ry, %rz, and %rd in the TurnShape() function. Try making changes to each of these and observing the effects your changes have on the item.
Programmed Scaling
We can also quite easily change the scale of an object using program code.
Type the following program and save it as 3DGPAi1\CH3\sizeshape.cs.
//========================================================================
//Sizeshape.cs
//
//This module contains the definition of a test shape, which uses
//a model of a stylized heart. It also contains functions for placing
//the test shape in the game world and then sizing the shape.
//========================================================================
datablock ItemData(TestShape)
// ----------------------------------------------------
// Definition of the shape object
// ----------------------------------------------------
{
// Basic Item properties
shapeFile = "~/data/shapes/items/heart.dts"; mass = 1; //we give the shape mass and
friction = 1; // friction to stop the item from sliding // down hills
};
function InsertTestShape()
Team LRN

114 Chapter 3 ■ 3D Programming Concepts
// ----------------------------------------------------
//Instantiates the test shape, then inserts it
//into the game world roughly in front of
//the player's default spawn location.
// ----------------------------------------------------
{
// An example function which creates a new TestShape object %shape = new Item() {
datablock = TestShape;
rotation = "0 0 1 0"; // initialize the values
// to something meaningful
};
MissionCleanup.add(%shape);
// Player setup
%shape.setTransform("-90 -2 20 0 0 1 0"); echo("Inserting Shape " @ %shape);
return %shape;
}
function SizeShape(%shape, %scale)
// ----------------------------------------------------
// moves the %shape by %scale amount
// ----------------------------------------------------
{
%shape.setScale(%scale SPC %scale SPC %scale);
}
function DoSizeTest()
// ----------------------------------------------------
//a function to tie together the instantiation
//and the movement in one easy to type function
//call.
// ----------------------------------------------------
{
%ms = InsertTestShape();
SizeShape(%ms,5);
}
The program is obviously similar to the moveshape.cs and turnshape.cs programs. You can load and run the program in exactly the same way, except that you want to use
DoSizeTest() instead of DoMoveTest() and SizeShape() instead of MoveShape().
Team LRN

3D Programming |
115 |
You'll note that we don't call the object's %shape.getScale() function (there is one), because in this case, we don't need to. Also notice that the three arguments to our call to %shape.setScale() all use the same value. This is to make sure the object scales equally in all dimensions. Try making changes to each of these and observing the effects your changes have on the item.
Another exercise would be to modify the SizeShape function to accept a different parameter for each dimension (X, Y, or Z) so that you can change all three to different scales at the same time.
Programmed Animation
You can animate objects by stringing together a bunch of translation, rotation, and scale operations in a continuous loop. Like the transformations, most of the animation in Torque can be left up to an object's class methods to perform. However, you can create your own ad hoc animations quite easily by using the schedule() function.
Type the following program and save it as 3DGPAi1\CH3\animshape.cs.
//========================================================================
//animshape.cs
//
//This module contains the definition of a test shape, which uses
//a model of a stylized heart. It also contains functions for placing
//the test shape in the game world and then animating the shape using
//a recurring scheduled function call.
//========================================================================
datablock ItemData(TestShape)
// ----------------------------------------------------
// Definition of the shape object
// ----------------------------------------------------
{
// Basic Item properties
shapeFile = "~/data/shapes/items/heart.dts"; mass = 1; //we give the shape mass and
friction = 1; // friction to stop the item from sliding // down hills
};
function InsertTestShape()
// ----------------------------------------------------
//Instantiates the test shape, then inserts it
//into the game world roughly in front of
Team LRN
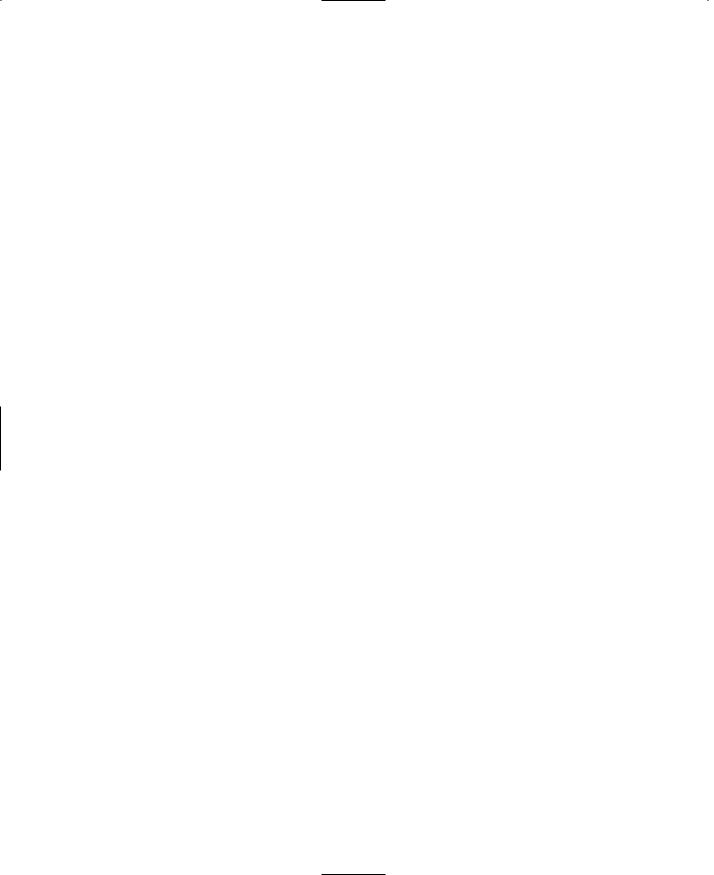
116 |
Chapter 3 ■ 3D Programming Concepts |
|
|
// |
the player's default spawn location. |
|
// |
---------------------------------------------------- |
|
{ |
|
// An example function which creates a new TestShape object %shape = new Item() {
datablock = TestShape;
rotation = "0 0 1 0"; // initialize the values
// to something meaningful
};
MissionCleanup.add(%shape);
// Player setup
%shape.setTransform("-90 -2 20 0 0 1 0"); echo("Inserting Shape " @ %shape);
return %shape;
}
function AnimShape(%shape, %dist, %angle, %scale)
// ----------------------------------------------------
// moves the %shape by %dist amount, and then // schedules itself to be called again in 1/5 // of a second.
// ----------------------------------------------------
{
%xfrm = %shape.getTransform();
%lx = getword(%xfrm,0); // first, get the current transform values
%ly = getword(%xfrm,1); |
|
%lz = getword(%xfrm,2); |
|
%rx = getword(%xfrm,3); |
|
%ry = getword(%xfrm,4); |
|
%rz = getword(%xfrm,5); |
|
%lx += %dist; |
// set the new x position |
%angle += 1.0; |
|
%rd = %angle; |
// Set the rotation angle |
if ($grow) |
// if the shape is growing larger |
{ |
|
Team LRN
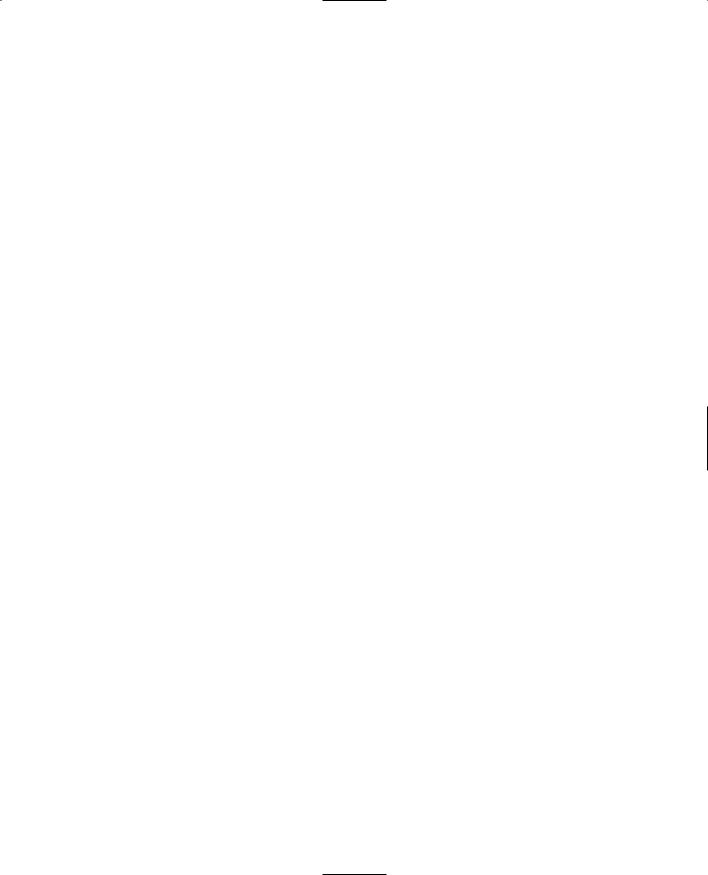
|
3D Programming |
117 |
if (%scale < 5.0) |
// and hasn't gotten too big |
|
%scale += 0.3; |
// make it bigger |
|
else |
|
|
$grow = false; |
// if it's too big, don't let it grow more |
|
} |
|
|
else |
// if it's shrinking |
|
{ |
|
|
if (%scale > 3.0) // and isn't too small |
|
|
%scale -= 0.3; |
// then make it smaller |
|
else |
|
|
$grow = true; |
// if it's too small, don't let it grow smaller |
|
} |
|
|
%shape.setScale(%scale SPC %scale SPC %scale);
%shape.setTransform(%lx SPC %ly SPC %lz SPC %rx SPC %ry SPC %rz SPC %rd); schedule(200,0,AnimShape, %shape, %dist, %angle, %scale);
}
function DoAnimTest()
//----------------------------------------------------
//a function to tie together the instantiation
//and the movement in one easy to type function
//call.
//----------------------------------------------------
{
%as = InsertTestShape(); $grow = true; AnimShape(%as,0.2, 1, 2);
}
This module is almost identical to the MoveShape() module we worked with earlier.
The function AnimShape accepts a shape handle in %shape, a distance step as %dist, an angle value as %angle, and a scaling value as %scale and uses these to transform the shape indicated by the %shape handle.
First, it obtains the current position of the shape using the %shape.getTransform() method of the Item class.
As with the earlier MoveShape() function, the AnimShape() function fetches the transform of the shape and updates one of the axis values.
Then it updates the rotation value stored %rd.
Team LRN