
- •Advanced Bash-Scripting Guide
- •Dedication
- •Table of Contents
- •Part 1. Introduction
- •Advanced Bash-Scripting Guide
- •Chapter 2. Starting Off With a Sha-Bang
- •2.1. Invoking the script
- •2.2. Preliminary Exercises
- •Part 2. Basics
- •Chapter 3. Exit and Exit Status
- •Chapter 4. Special Characters
- •Chapter 5. Introduction to Variables and Parameters
- •5.1. Variable Substitution
- •5.2. Variable Assignment
- •5.3. Bash Variables Are Untyped
- •5.4. Special Variable Types
- •Chapter 6. Quoting
- •Chapter 7. Tests
- •7.1. Test Constructs
- •7.2. File test operators
- •7.3. Comparison operators (binary)
- •7.4. Nested if/then Condition Tests
- •7.5. Testing Your Knowledge of Tests
- •8.1. Operators
- •8.2. Numerical Constants
- •Part 3. Beyond the Basics
- •Chapter 9. Variables Revisited
- •9.1. Internal Variables
- •9.2. Manipulating Strings
- •9.3. Parameter Substitution
- •9.4. Typing variables: declare or typeset
- •9.5. Indirect References to Variables
- •9.6. $RANDOM: generate random integer
- •9.7. The Double Parentheses Construct
- •Chapter 10. Loops and Branches
- •10.1. Loops
- •10.2. Nested Loops
- •10.3. Loop Control
- •10.4. Testing and Branching
- •Chapter 11. Internal Commands and Builtins
- •12.1. Basic Commands
- •12.2. Complex Commands
- •12.3. Time / Date Commands
- •12.4. Text Processing Commands
- •12.5. File and Archiving Commands
- •12.6. Communications Commands
- •12.7. Terminal Control Commands
- •12.8. Math Commands
- •12.9. Miscellaneous Commands
- •Chapter 13. System and Administrative Commands
- •Chapter 14. Command Substitution
- •Chapter 15. Arithmetic Expansion
- •Chapter 16. I/O Redirection
- •16.1. Using exec
- •16.2. Redirecting Code Blocks
- •16.3. Applications
- •Chapter 17. Here Documents
- •Chapter 18. Recess Time
- •Part 4. Advanced Topics
- •Chapter 19. Regular Expressions
- •19.1. A Brief Introduction to Regular Expressions
- •19.2. Globbing
- •Chapter 20. Subshells
- •Chapter 21. Restricted Shells
- •Chapter 22. Process Substitution
- •Chapter 23. Functions
- •23.1. Complex Functions and Function Complexities
- •23.2. Local Variables
- •Chapter 24. Aliases
- •Chapter 25. List Constructs
- •Chapter 26. Arrays
- •Chapter 27. Files
- •Chapter 28. /dev and /proc
- •28.2. /proc
- •Chapter 29. Of Zeros and Nulls
- •Chapter 30. Debugging
- •Chapter 31. Options
- •Chapter 32. Gotchas
- •Chapter 33. Scripting With Style
- •Chapter 34. Miscellany
- •34.2. Shell Wrappers
- •34.3. Tests and Comparisons: Alternatives
- •34.4. Optimizations
- •34.5. Assorted Tips
- •34.6. Oddities
- •34.7. Security Issues
- •34.8. Portability Issues
- •34.9. Shell Scripting Under Windows
- •Chapter 35. Bash, version 2
- •36. Endnotes
- •36.1. Author's Note
- •36.2. About the Author
- •36.3. Tools Used to Produce This Book
- •36.4. Credits
- •List of Tables
- •List of Examples
- •Bibliography
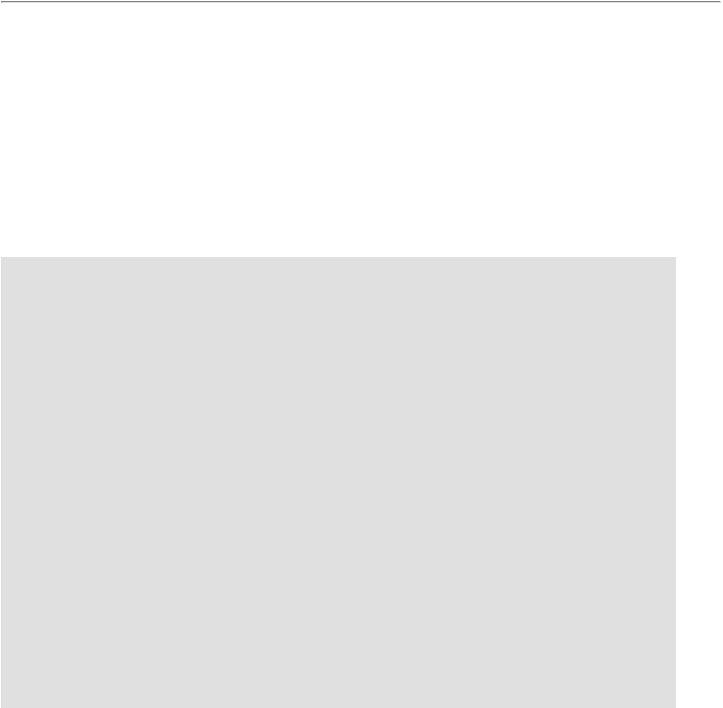
Local Variables
|
Advanced Bash-Scripting Guide: |
|
Prev |
Chapter 23. Functions |
Next |
23.2. Local Variables
What makes a variable "local"?
local variables
A variable declared as local is one that is visible only within the block of code in which it appears. It has local "scope". In a function, a local variable has meaning only within that function block.
Example 23-8. Local variable visibility
#!/bin/bash |
|
func () |
|
{ |
|
local loc_var=23 |
# Declared local. |
echo |
|
echo "\"loc_var\" in function = $loc_var" |
|
global_var=999 |
# Not declared local. |
echo "\"global_var\" in function = $global_var"
}
func
# Now, see if local 'a' exists outside function.
echo
echo "\"loc_var\" outside function = $loc_var"
#"loc_var" outside function =
#Nope, $loc_var not visible globally.
echo "\"global_var\" outside function = $global_var"
#"global_var" outside function = 999
#$global_var is visible globally.
echo
exit 0
http://tldp.org/LDP/abs/html/localvar.html (1 of 4) [7/15/2002 6:35:18 PM]

Local Variables
Before a function is called, all variables declared within the function are invisible outside the body of the function, not just those explicitly declared as local.
#!/bin/bash |
|
func () |
|
{ |
|
global_var=37 |
# Visible only within the function block |
|
#+ before the function has been called. |
} |
# END OF FUNCTION |
echo "global_var = $global_var" # global_var =
# Function "func" has not yet been called, #+ so $global_var is not visible here.
func
echo "global_var = $global_var" # global_var = 37
#Has been set by function call.
23.2.1.Local variables make recursion possible.
Local variables permit recursion, [1] but this practice generally involves much computational overhead and is definitely not recommended in a shell script. [2]
Example 23-9. Recursion, using a local variable
#!/bin/bash |
|
# |
factorial |
# |
--------- |
#Does bash permit recursion?
#Well, yes, but...
#You gotta have rocks in your head to try it.
MAX_ARG=5
E_WRONG_ARGS=65
E_RANGE_ERR=66
if [ -z "$1" ] then
echo "Usage: `basename $0` number" exit $E_WRONG_ARGS
fi
if [ "$1" -gt $MAX_ARG ] then
http://tldp.org/LDP/abs/html/localvar.html (2 of 4) [7/15/2002 6:35:18 PM]

Local Variables
echo "Out of range (5 is maximum)."
#Let's get real now.
#If you want greater range than this,
#rewrite it in a real programming language. exit $E_RANGE_ERR
fi
fact ()
{
local number=$1
#Variable "number" must be declared as local,
#otherwise this doesn't work.
if [ "$number" -eq |
0 ] |
|
|
then |
|
|
|
factorial=1 |
# |
Factorial |
of 0 = 1. |
else |
|
|
|
let "decrnum = |
number - 1" |
|
|
fact $decrnum |
# |
Recursive |
function call. |
let "factorial |
= |
$number * |
$?" |
fi |
|
|
|
return $factorial
}
fact $1
echo "Factorial of $1 is $?."
exit 0
See also Example A-16 for an example of recursion in a script. Be aware that recursion is resource-intensive and executes slowly, and is therefore generally not appropriate to use in a script.
Notes
[1]Herbert Mayer defines recursion as "...expressing an algorithm by using a simpler version of that same algorithm..." A recursive function is one that calls itself.
[2]Too many levels of recursion may crash a script with a segfault.
#!/bin/bash
recursive_function ()
{
(( $1 < $2 )) && f $(( $1 + 1 )) $2;
# As long as 1st parameter is less than 2nd, #+ increment 1st and recurse.
}
recursive_function 1 50000 # Recurse 50,000 levels!
#Segfaults, of course.
#Recursion this deep might cause even a C program to segfault,
http://tldp.org/LDP/abs/html/localvar.html (3 of 4) [7/15/2002 6:35:18 PM]

Local Variables
#+ by using up all the memory allotted to the stack.
# Thanks, S.C.
exit 0 # This script will not exit normally.
Prev |
Home |
Next |
Complex Functions and Function |
Up |
Aliases |
Complexities |
|
|
http://tldp.org/LDP/abs/html/localvar.html (4 of 4) [7/15/2002 6:35:18 PM]