
- •Front Matter
- •Copyright, Trademarks, and Attributions
- •Attributions
- •Print Production
- •Contacting The Publisher
- •HTML Version and Source Code
- •Typographical Conventions
- •Author Introduction
- •Audience
- •Book Content
- •The Genesis of repoze.bfg
- •The Genesis of Pyramid
- •Thanks
- •Pyramid Introduction
- •What Makes Pyramid Unique
- •URL generation
- •Debug Toolbar
- •Debugging settings
- •Class-based and function-based views
- •Extensible templating
- •Rendered views can return dictionaries
- •Event system
- •Built-in internationalization
- •HTTP caching
- •Sessions
- •Speed
- •Exception views
- •No singletons
- •View predicates and many views per route
- •Transaction management
- •Flexible authentication and authorization
- •Traversal
- •Tweens
- •View response adapters
- •Testing
- •Support
- •Documentation
- •What Is The Pylons Project?
- •Pyramid and Other Web Frameworks
- •Installing Pyramid
- •Before You Install
- •Installing Pyramid on a UNIX System
- •Installing the virtualenv Package
- •Creating the Virtual Python Environment
- •Installing Pyramid Into the Virtual Python Environment
- •Installing Pyramid on a Windows System
- •What Gets Installed
- •Application Configuration
- •Summary
- •Creating Your First Pyramid Application
- •Hello World
- •Imports
- •View Callable Declarations
- •WSGI Application Creation
- •WSGI Application Serving
- •Conclusion
- •References
- •Creating a Pyramid Project
- •Scaffolds Included with Pyramid
- •Creating the Project
- •Installing your Newly Created Project for Development
- •Running The Tests For Your Application
- •Running The Project Application
- •Reloading Code
- •Viewing the Application
- •The Debug Toolbar
- •The Project Structure
- •The MyProject Project
- •development.ini
- •production.ini
- •MANIFEST.in
- •setup.py
- •setup.cfg
- •The myproject Package
- •__init__.py
- •views.py
- •static
- •templates/mytemplate.pt
- •tests.py
- •Modifying Package Structure
- •Using the Interactive Shell
- •What Is This pserve Thing
- •Using an Alternate WSGI Server
- •Startup
- •The Startup Process
- •Deployment Settings
- •Request Processing
- •URL Dispatch
- •High-Level Operational Overview
- •Route Pattern Syntax
- •Route Declaration Ordering
- •Route Matching
- •The Matchdict
- •The Matched Route
- •Routing Examples
- •Example 1
- •Example 2
- •Example 3
- •Matching the Root URL
- •Generating Route URLs
- •Static Routes
- •Debugging Route Matching
- •Using a Route Prefix to Compose Applications
- •Custom Route Predicates
- •Route Factories
- •Using Pyramid Security With URL Dispatch
- •Route View Callable Registration and Lookup Details
- •References
- •Views
- •View Callables
- •View Callable Responses
- •Using Special Exceptions In View Callables
- •HTTP Exceptions
- •How Pyramid Uses HTTP Exceptions
- •Custom Exception Views
- •Using a View Callable to Do an HTTP Redirect
- •Handling Form Submissions in View Callables (Unicode and Character Set Issues)
- •Alternate View Callable Argument/Calling Conventions
- •Renderers
- •Writing View Callables Which Use a Renderer
- •Built-In Renderers
- •string: String Renderer
- •json: JSON Renderer
- •JSONP Renderer
- •*.pt or *.txt: Chameleon Template Renderers
- •*.mak or *.mako: Mako Template Renderer
- •Varying Attributes of Rendered Responses
- •Deprecated Mechanism to Vary Attributes of Rendered Responses
- •Adding and Changing Renderers
- •Adding a New Renderer
- •Changing an Existing Renderer
- •Overriding A Renderer At Runtime
- •Templates
- •Using Templates Directly
- •System Values Used During Rendering
- •Chameleon ZPT Templates
- •A Sample ZPT Template
- •Using ZPT Macros in Pyramid
- •Templating with Chameleon Text Templates
- •Side Effects of Rendering a Chameleon Template
- •Debugging Templates
- •Chameleon Template Internationalization
- •Templating With Mako Templates
- •A Sample Mako Template
- •Automatically Reloading Templates
- •Available Add-On Template System Bindings
- •View Configuration
- •Mapping a Resource or URL Pattern to a View Callable
- •@view_defaults Class Decorator
- •NotFound Errors
- •Debugging View Configuration
- •Static Assets
- •Serving Static Assets
- •Generating Static Asset URLs
- •Advanced: Serving Static Assets Using a View Callable
- •Root-Relative Custom Static View (URL Dispatch Only)
- •Overriding Assets
- •The override_asset API
- •Request and Response Objects
- •Request
- •Special Attributes Added to the Request by Pyramid
- •URLs
- •Methods
- •Unicode
- •Multidict
- •Dealing With A JSON-Encoded Request Body
- •Cleaning Up After a Request
- •More Details
- •Response
- •Headers
- •Instantiating the Response
- •Exception Responses
- •More Details
- •Sessions
- •Using The Default Session Factory
- •Using a Session Object
- •Using Alternate Session Factories
- •Creating Your Own Session Factory
- •Flash Messages
- •Using the session.flash Method
- •Using the session.pop_flash Method
- •Using the session.peek_flash Method
- •Preventing Cross-Site Request Forgery Attacks
- •Using the session.get_csrf_token Method
- •Using the session.new_csrf_token Method
- •Using Events
- •An Example
- •Reloading Templates
- •Reloading Assets
- •Debugging Authorization
- •Debugging Not Found Errors
- •Debugging Route Matching
- •Preventing HTTP Caching
- •Debugging All
- •Reloading All
- •Default Locale Name
- •Including Packages
- •pyramid.includes vs. pyramid.config.Configurator.include()
- •Mako Template Render Settings
- •Mako Directories
- •Mako Module Directory
- •Mako Input Encoding
- •Mako Error Handler
- •Mako Default Filters
- •Mako Import
- •Mako Preprocessor
- •Examples
- •Understanding the Distinction Between reload_templates and reload_assets
- •Adding A Custom Setting
- •Logging
- •Sending Logging Messages
- •Filtering log messages
- •Logging Exceptions
- •PasteDeploy Configuration Files
- •PasteDeploy
- •Entry Points and PasteDeploy .ini Files
- •[DEFAULTS] Section of a PasteDeploy .ini File
- •Command-Line Pyramid
- •Displaying Matching Views for a Given URL
- •The Interactive Shell
- •Extending the Shell
- •IPython or bpython
- •Displaying All Application Routes
- •Invoking a Request
- •Writing a Script
- •Changing the Request
- •Cleanup
- •Setting Up Logging
- •Making Your Script into a Console Script
- •Internationalization and Localization
- •Creating a Translation String
- •Using The TranslationString Class
- •Using the TranslationStringFactory Class
- •Working With gettext Translation Files
- •Installing Babel and Lingua
- •Extracting Messages from Code and Templates
- •Initializing a Message Catalog File
- •Updating a Catalog File
- •Compiling a Message Catalog File
- •Using a Localizer
- •Performing a Translation
- •Performing a Pluralization
- •Obtaining the Locale Name for a Request
- •Performing Date Formatting and Currency Formatting
- •Chameleon Template Support for Translation Strings
- •Mako Pyramid I18N Support
- •Localization-Related Deployment Settings
- •Activating Translation
- •Adding a Translation Directory
- •Setting the Locale
- •Locale Negotiators
- •The Default Locale Negotiator
- •Using a Custom Locale Negotiator
- •Virtual Hosting
- •Virtual Root Support
- •Further Documentation and Examples
- •Test Set Up and Tear Down
- •What?
- •Using the Configurator and pyramid.testing APIs in Unit Tests
- •Creating Integration Tests
- •Creating Functional Tests
- •Resources
- •Location-Aware Resources
- •Generating The URL Of A Resource
- •Overriding Resource URL Generation
- •Generating the Path To a Resource
- •Finding a Resource by Path
- •Obtaining the Lineage of a Resource
- •Determining if a Resource is In The Lineage of Another Resource
- •Finding the Root Resource
- •Resources Which Implement Interfaces
- •Finding a Resource With a Class or Interface in Lineage
- •Pyramid API Functions That Act Against Resources
- •Much Ado About Traversal
- •URL Dispatch
- •Historical Refresher
- •Traversal (aka Resource Location)
- •View Lookup
- •Use Cases
- •Traversal
- •Traversal Details
- •The Resource Tree
- •The Traversal Algorithm
- •A Description of The Traversal Algorithm
- •Traversal Algorithm Examples
- •References
- •Security
- •Enabling an Authorization Policy
- •Enabling an Authorization Policy Imperatively
- •Protecting Views with Permissions
- •Setting a Default Permission
- •Assigning ACLs to your Resource Objects
- •Elements of an ACL
- •Special Principal Names
- •Special Permissions
- •Special ACEs
- •ACL Inheritance and Location-Awareness
- •Changing the Forbidden View
- •Debugging View Authorization Failures
- •Debugging Imperative Authorization Failures
- •Creating Your Own Authentication Policy
- •Creating Your Own Authorization Policy
- •Combining Traversal and URL Dispatch
- •A Review of Non-Hybrid Applications
- •URL Dispatch Only
- •Traversal Only
- •Hybrid Applications
- •The Root Object for a Route Match
- •Using *traverse In a Route Pattern
- •Using *subpath in a Route Pattern
- •Corner Cases
- •Registering a Default View for a Route That Has a view Attribute
- •Using Hooks
- •Changing the Not Found View
- •Changing the Forbidden View
- •Changing the Request Factory
- •Using The Before Render Event
- •Adding Renderer Globals (Deprecated)
- •Using Response Callbacks
- •Using Finished Callbacks
- •Changing the Traverser
- •Changing How pyramid.request.Request.resource_url() Generates a URL
- •Changing How Pyramid Treats View Responses
- •Using a View Mapper
- •Creating a Tween Factory
- •Registering an Implicit Tween Factory
- •Suggesting Implicit Tween Ordering
- •Explicit Tween Ordering
- •Displaying Tween Ordering
- •Pyramid Configuration Introspection
- •Using the Introspector
- •Introspectable Objects
- •Pyramid Introspection Categories
- •Introspection in the Toolbar
- •Disabling Introspection
- •Rules for Building An Extensible Application
- •Fundamental Plugpoints
- •Extending an Existing Application
- •Extending the Application
- •Overriding Views
- •Overriding Routes
- •Overriding Assets
- •Advanced Configuration
- •Two-Phase Configuration
- •Using config.action in a Directive
- •Adding Configuration Introspection
- •Introspectable Relationships
- •Thread Locals
- •Why and How Pyramid Uses Thread Local Variables
- •Using the Zope Component Architecture in Pyramid
- •Using the ZCA Global API in a Pyramid Application
- •Disusing the Global ZCA API
- •Enabling the ZCA Global API by Using hook_zca
- •Enabling the ZCA Global API by Using The ZCA Global Registry
- •Background
- •Design
- •Overall
- •Models
- •Views
- •Security
- •Summary
- •Installation
- •Preparation
- •Make a Project
- •Run the Tests
- •Expose Test Coverage Information
- •Start the Application
- •Visit the Application in a Browser
- •Decisions the zodb Scaffold Has Made For You
- •Basic Layout
- •Resources and Models with models.py
- •Views With views.py
- •Defining the Domain Model
- •Delete the Database
- •Edit models.py
- •Look at the Result of Our Edits to models.py
- •View the Application in a Browser
- •Defining Views
- •Declaring Dependencies in Our setup.py File
- •Adding View Functions
- •Viewing the Result of all Our Edits to views.py
- •Adding Templates
- •Viewing the Application in a Browser
- •Adding Authorization
- •Add Authentication and Authorization Policies
- •Add security.py
- •Give Our Root Resource an ACL
- •Add Login and Logout Views
- •Change Existing Views
- •Add permission Declarations to our view_config Decorators
- •Add the login.pt Template
- •Change view.pt and edit.pt
- •See Our Changes To views.py and our Templates
- •View the Application in a Browser
- •Adding Tests
- •Test the Models
- •Test the Views
- •Functional tests
- •View the results of all our edits to tests.py
- •Run the Tests
- •Distributing Your Application
- •SQLAlchemy + URL Dispatch Wiki Tutorial
- •Background
- •Design
- •Overall
- •Models
- •Views
- •Security
- •Summary
- •Installation
- •Preparation
- •Making a Project
- •Running the Tests
- •Exposing Test Coverage Information
- •Initializing the Database
- •Starting the Application
- •Decisions the alchemy Scaffold Has Made For You
- •Basic Layout
- •View Declarations via views.py
- •Content Models with models.py
- •Making Edits to models.py
- •Changing scripts/initializedb.py
- •Reinitializing the Database
- •Viewing the Application in a Browser
- •Defining Views
- •Declaring Dependencies in Our setup.py File
- •Running setup.py develop
- •Changing the views.py File
- •Adding Templates
- •Adding Routes to __init__.py
- •Viewing the Application in a Browser
- •Adding Authorization
- •Adding A Root Factory
- •Add an Authorization Policy and an Authentication Policy
- •Adding an authentication policy callback
- •Adding Login and Logout Views
- •Changing Existing Views
- •Adding the login.pt Template
- •Seeing Our Changes To views.py and our Templates
- •Viewing the Application in a Browser
- •Adding Tests
- •Testing the Models
- •Testing the Views
- •Functional tests
- •Viewing the results of all our edits to tests.py
- •Running the Tests
- •Distributing Your Application
- •Converting a repoze.bfg Application to Pyramid
- •Running a Pyramid Application under mod_wsgi
- •pyramid.authorization
- •pyramid.authentication
- •Authentication Policies
- •Helper Classes
- •pyramid.chameleon_text
- •pyramid.chameleon_zpt
- •pyramid.config
- •pyramid.events
- •Functions
- •Event Types
- •pyramid.exceptions
- •pyramid.httpexceptions
- •HTTP Exceptions
- •pyramid.i18n
- •pyramid.interfaces
- •Event-Related Interfaces
- •Other Interfaces
- •pyramid.location
- •pyramid.paster
- •pyramid.registry
- •pyramid.renderers
- •pyramid.request
- •pyramid.response
- •Functions
- •pyramid.scripting
- •pyramid.security
- •Authentication API Functions
- •Authorization API Functions
- •Constants
- •Return Values
- •pyramid.settings
- •pyramid.testing
- •pyramid.threadlocal
- •pyramid.traversal
- •pyramid.url
- •pyramid.view
- •pyramid.wsgi
- •Glossary
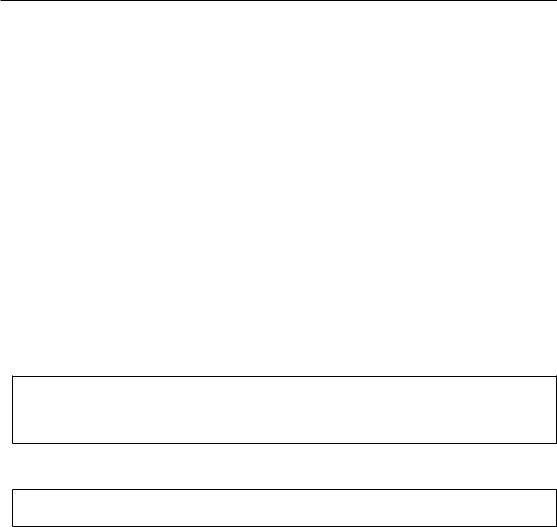
27. SECURITY
27.6 Special Permissions
Special permission names exist in the pyramid.security module. These can be imported for use in ACLs. pyramid.security.ALL_PERMISSIONS
An object representing, literally, all permissions. Useful in an ACL like so: (Allow, ’fred’, ALL_PERMISSIONS). The ALL_PERMISSIONS object is actually a stand-in object that has a __contains__ method that always returns True, which, for all known authorization policies, has the effect of indicating that a given principal “has” any permission asked for by the system.
27.7 Special ACEs
A convenience ACE is defined representing a deny to everyone of all permissions in pyramid.security.DENY_ALL. This ACE is often used as the last ACE of an ACL to explicitly cause inheriting authorization policies to “stop looking up the traversal tree” (effectively breaking any inheritance). For example, an ACL which allows only fred the view permission for a particular resource despite what inherited ACLs may say when the default authorization policy is in effect might look like so:
1
2
3
4
from pyramid.security import Allow from pyramid.security import DENY_ALL
__acl__ = [ (Allow, ’fred’, ’view’), DENY_ALL ]
“Under the hood”, the pyramid.security.DENY_ALL ACE equals the following:
1
2
from pyramid.security import ALL_PERMISSIONS __acl__ = [ (Deny, Everyone, ALL_PERMISSIONS) ]
27.8 ACL Inheritance and Location-Awareness
While the default authorization policy is in place, if a resource object does not have an ACL when it is the context, its parent is consulted for an ACL. If that object does not have an ACL, its parent is consulted for an ACL, ad infinitum, until we’ve reached the root and there are no more parents left.
In order to allow the security machinery to perform ACL inheritance, resource objects must provide location-awareness. Providing location-awareness means two things: the root object in the resource tree must have a __name__ attribute and a __parent__ attribute.
300

27.9. CHANGING THE FORBIDDEN VIEW
1 class Blog(object):
2__name__ = ’’
3__parent__ = None
An object with a __parent__ attribute and a __name__ attribute is said to be location-aware. Location-aware objects define an __parent__ attribute which points at their parent object. The root object’s __parent__ is None.
See pyramid.location for documentations of functions which use location-awareness. See also LocationAware Resources.
27.9 Changing the Forbidden View
When Pyramid denies a view invocation due to an authorization denial, the special forbidden view is invoked. “Out of the box”, this forbidden view is very plain. See Changing the Forbidden View within Using Hooks for instructions on how to create a custom forbidden view and arrange for it to be called when view authorization is denied.
27.10 Debugging View Authorization Failures
If your application in your judgment is allowing or denying view access inappropriately, start your application under a shell using the PYRAMID_DEBUG_AUTHORIZATION environment variable set to 1. For example:
$ PYRAMID_DEBUG_AUTHORIZATION=1 bin/pserve myproject.ini
When any authorization takes place during a top-level view rendering, a message will be logged to the console (to stderr) about what ACE in which ACL permitted or denied the authorization based on authentication information.
This behavior can also be turned on in the application .ini file by setting the pyramid.debug_authorization key to true within the application’s configuration section, e.g.:
1
2
3
[app:main]
use = egg:MyProject pyramid.debug_authorization = true
With this debug flag turned on, the response sent to the browser will also contain security debugging information in its body.
301

27. SECURITY
27.11 Debugging Imperative Authorization Failures
The pyramid.security.has_permission() API |
is used to check security within |
||||||||||
view |
functions |
imperatively. |
It |
returns |
instances of |
objects that are effectively booleans. |
|||||
But these objects are not |
raw |
True |
or |
False |
objects, |
and have |
information |
at- |
|||
tached to |
them |
about why |
the |
permission |
was allowed or |
denied. |
The object |
will |
|||
be |
one |
of |
pyramid.security.ACLAllowed, |
pyramid.security.ACLDenied, |
pyramid.security.Allowed, or pyramid.security.Denied, as documented in pyramid.security. At the very minimum these objects will have a msg attribute, which is a string indicating why the permission was denied or allowed. Introspecting this information in the debugger or via print statements when a call to has_permission() fails is often useful.
27.12 Creating Your Own Authentication Policy
Pyramid ships with a number of useful out-of-the-box security policies (see pyramid.authentication). However, creating your own authentication policy is often necessary when you want to control the “horizontal and vertical” of how your users authenticate. Doing so is a matter of creating an instance of something that implements the following interface:
1 class IAuthenticationPolicy(object):
2""" An object representing a Pyramid authentication policy. """
3
4def authenticated_userid(self, request):
5""" Return the authenticated userid or ‘‘None‘‘ if no
6authenticated userid can be found. This method of the policy
7 should ensure that a record exists in whatever persistent store is 8 used related to the user (the user should not have been deleted); 9 if a record associated with the current id does not exist in a
10 persistent store, it should return ‘‘None‘‘."""
11
12def unauthenticated_userid(self, request):
13""" Return the *unauthenticated* userid. This method performs the
14same duty as ‘‘authenticated_userid‘‘ but is permitted to return the
15userid based only on data present in the request; it needn’t (and
16shouldn’t) check any persistent store to ensure that the user record
17related to the request userid exists."""
18
19def effective_principals(self, request):
20""" Return a sequence representing the effective principals
21including the userid and any groups belonged to by the current
22user, including ’system’ groups such as
302
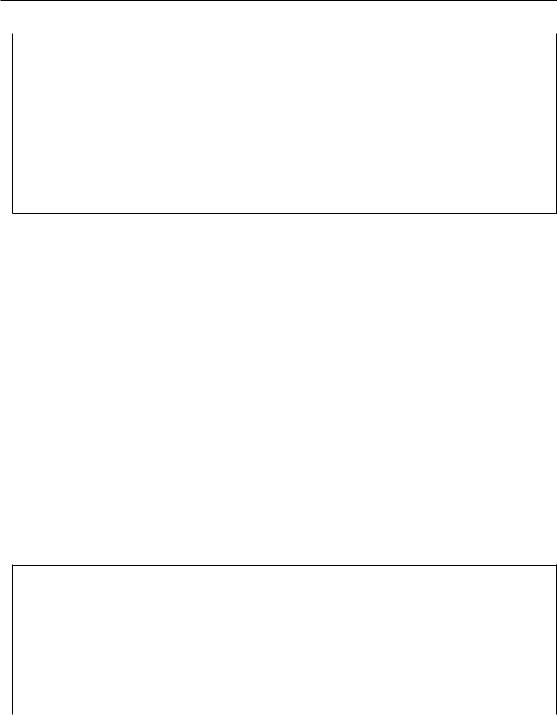
27.13. CREATING YOUR OWN AUTHORIZATION POLICY
23‘‘pyramid.security.Everyone‘‘ and
24‘‘pyramid.security.Authenticated‘‘. """
25
26def remember(self, request, principal, **kw):
27""" Return a set of headers suitable for ’remembering’ the
28principal named ‘‘principal‘‘ when set in a response. An
29individual authentication policy and its consumers can decide
30on the composition and meaning of **kw. """
31
32def forget(self, request):
33""" Return a set of headers suitable for ’forgetting’ the
34current user on subsequent requests. """
After you do so, you can pass an instance of such a class into the set_authentication_policy method configuration time to use it.
27.13 Creating Your Own Authorization Policy
An |
authorization |
policy is |
a policy |
that allows or |
denies |
access |
after a |
user |
has been |
authenticated. |
Most |
Pyramid applications |
will |
use the |
default |
pyramid.authorization.ACLAuthorizationPolicy.
However, in some cases, it’s useful to be able to use a different authorization policy than the default ACLAuthorizationPolicy. For example, it might be desirable to construct an alternate authorization policy which allows the application to use an authorization mechanism that does not involve ACL objects.
Pyramid ships with only a single default authorization policy, so you’ll need to create your own if you’d like to use a different one. Creating and using your own authorization policy is a matter of creating an instance of an object that implements the following interface:
1 class IAuthorizationPolicy(object):
2 """ An object representing a Pyramid authorization policy. """
3def permits(self, context, principals, permission):
4""" Return ‘‘True‘‘ if any of the ‘‘principals‘‘ is allowed the
5‘‘permission‘‘ in the current ‘‘context‘‘, else return ‘‘False‘‘
6"""
7
8def principals_allowed_by_permission(self, context, permission):
9""" Return a set of principal identifiers allowed by the
10 ‘‘permission‘‘ in ‘‘context‘‘. This behavior is optional; if you
303

27. SECURITY
11choose to not implement it you should define this method as
12something which raises a ‘‘NotImplementedError‘‘. This method
13will only be called when the
14‘‘pyramid.security.principals_allowed_by_permission‘‘ API is
15used."""
After you do so, you can pass an instance of such a class into the set_authorization_policy method at configuration time to use it.
304