
- •Front Matter
- •Copyright, Trademarks, and Attributions
- •Attributions
- •Print Production
- •Contacting The Publisher
- •HTML Version and Source Code
- •Typographical Conventions
- •Author Introduction
- •Audience
- •Book Content
- •The Genesis of repoze.bfg
- •The Genesis of Pyramid
- •Thanks
- •Pyramid Introduction
- •What Makes Pyramid Unique
- •URL generation
- •Debug Toolbar
- •Debugging settings
- •Class-based and function-based views
- •Extensible templating
- •Rendered views can return dictionaries
- •Event system
- •Built-in internationalization
- •HTTP caching
- •Sessions
- •Speed
- •Exception views
- •No singletons
- •View predicates and many views per route
- •Transaction management
- •Flexible authentication and authorization
- •Traversal
- •Tweens
- •View response adapters
- •Testing
- •Support
- •Documentation
- •What Is The Pylons Project?
- •Pyramid and Other Web Frameworks
- •Installing Pyramid
- •Before You Install
- •Installing Pyramid on a UNIX System
- •Installing the virtualenv Package
- •Creating the Virtual Python Environment
- •Installing Pyramid Into the Virtual Python Environment
- •Installing Pyramid on a Windows System
- •What Gets Installed
- •Application Configuration
- •Summary
- •Creating Your First Pyramid Application
- •Hello World
- •Imports
- •View Callable Declarations
- •WSGI Application Creation
- •WSGI Application Serving
- •Conclusion
- •References
- •Creating a Pyramid Project
- •Scaffolds Included with Pyramid
- •Creating the Project
- •Installing your Newly Created Project for Development
- •Running The Tests For Your Application
- •Running The Project Application
- •Reloading Code
- •Viewing the Application
- •The Debug Toolbar
- •The Project Structure
- •The MyProject Project
- •development.ini
- •production.ini
- •MANIFEST.in
- •setup.py
- •setup.cfg
- •The myproject Package
- •__init__.py
- •views.py
- •static
- •templates/mytemplate.pt
- •tests.py
- •Modifying Package Structure
- •Using the Interactive Shell
- •What Is This pserve Thing
- •Using an Alternate WSGI Server
- •Startup
- •The Startup Process
- •Deployment Settings
- •Request Processing
- •URL Dispatch
- •High-Level Operational Overview
- •Route Pattern Syntax
- •Route Declaration Ordering
- •Route Matching
- •The Matchdict
- •The Matched Route
- •Routing Examples
- •Example 1
- •Example 2
- •Example 3
- •Matching the Root URL
- •Generating Route URLs
- •Static Routes
- •Debugging Route Matching
- •Using a Route Prefix to Compose Applications
- •Custom Route Predicates
- •Route Factories
- •Using Pyramid Security With URL Dispatch
- •Route View Callable Registration and Lookup Details
- •References
- •Views
- •View Callables
- •View Callable Responses
- •Using Special Exceptions In View Callables
- •HTTP Exceptions
- •How Pyramid Uses HTTP Exceptions
- •Custom Exception Views
- •Using a View Callable to Do an HTTP Redirect
- •Handling Form Submissions in View Callables (Unicode and Character Set Issues)
- •Alternate View Callable Argument/Calling Conventions
- •Renderers
- •Writing View Callables Which Use a Renderer
- •Built-In Renderers
- •string: String Renderer
- •json: JSON Renderer
- •JSONP Renderer
- •*.pt or *.txt: Chameleon Template Renderers
- •*.mak or *.mako: Mako Template Renderer
- •Varying Attributes of Rendered Responses
- •Deprecated Mechanism to Vary Attributes of Rendered Responses
- •Adding and Changing Renderers
- •Adding a New Renderer
- •Changing an Existing Renderer
- •Overriding A Renderer At Runtime
- •Templates
- •Using Templates Directly
- •System Values Used During Rendering
- •Chameleon ZPT Templates
- •A Sample ZPT Template
- •Using ZPT Macros in Pyramid
- •Templating with Chameleon Text Templates
- •Side Effects of Rendering a Chameleon Template
- •Debugging Templates
- •Chameleon Template Internationalization
- •Templating With Mako Templates
- •A Sample Mako Template
- •Automatically Reloading Templates
- •Available Add-On Template System Bindings
- •View Configuration
- •Mapping a Resource or URL Pattern to a View Callable
- •@view_defaults Class Decorator
- •NotFound Errors
- •Debugging View Configuration
- •Static Assets
- •Serving Static Assets
- •Generating Static Asset URLs
- •Advanced: Serving Static Assets Using a View Callable
- •Root-Relative Custom Static View (URL Dispatch Only)
- •Overriding Assets
- •The override_asset API
- •Request and Response Objects
- •Request
- •Special Attributes Added to the Request by Pyramid
- •URLs
- •Methods
- •Unicode
- •Multidict
- •Dealing With A JSON-Encoded Request Body
- •Cleaning Up After a Request
- •More Details
- •Response
- •Headers
- •Instantiating the Response
- •Exception Responses
- •More Details
- •Sessions
- •Using The Default Session Factory
- •Using a Session Object
- •Using Alternate Session Factories
- •Creating Your Own Session Factory
- •Flash Messages
- •Using the session.flash Method
- •Using the session.pop_flash Method
- •Using the session.peek_flash Method
- •Preventing Cross-Site Request Forgery Attacks
- •Using the session.get_csrf_token Method
- •Using the session.new_csrf_token Method
- •Using Events
- •An Example
- •Reloading Templates
- •Reloading Assets
- •Debugging Authorization
- •Debugging Not Found Errors
- •Debugging Route Matching
- •Preventing HTTP Caching
- •Debugging All
- •Reloading All
- •Default Locale Name
- •Including Packages
- •pyramid.includes vs. pyramid.config.Configurator.include()
- •Mako Template Render Settings
- •Mako Directories
- •Mako Module Directory
- •Mako Input Encoding
- •Mako Error Handler
- •Mako Default Filters
- •Mako Import
- •Mako Preprocessor
- •Examples
- •Understanding the Distinction Between reload_templates and reload_assets
- •Adding A Custom Setting
- •Logging
- •Sending Logging Messages
- •Filtering log messages
- •Logging Exceptions
- •PasteDeploy Configuration Files
- •PasteDeploy
- •Entry Points and PasteDeploy .ini Files
- •[DEFAULTS] Section of a PasteDeploy .ini File
- •Command-Line Pyramid
- •Displaying Matching Views for a Given URL
- •The Interactive Shell
- •Extending the Shell
- •IPython or bpython
- •Displaying All Application Routes
- •Invoking a Request
- •Writing a Script
- •Changing the Request
- •Cleanup
- •Setting Up Logging
- •Making Your Script into a Console Script
- •Internationalization and Localization
- •Creating a Translation String
- •Using The TranslationString Class
- •Using the TranslationStringFactory Class
- •Working With gettext Translation Files
- •Installing Babel and Lingua
- •Extracting Messages from Code and Templates
- •Initializing a Message Catalog File
- •Updating a Catalog File
- •Compiling a Message Catalog File
- •Using a Localizer
- •Performing a Translation
- •Performing a Pluralization
- •Obtaining the Locale Name for a Request
- •Performing Date Formatting and Currency Formatting
- •Chameleon Template Support for Translation Strings
- •Mako Pyramid I18N Support
- •Localization-Related Deployment Settings
- •Activating Translation
- •Adding a Translation Directory
- •Setting the Locale
- •Locale Negotiators
- •The Default Locale Negotiator
- •Using a Custom Locale Negotiator
- •Virtual Hosting
- •Virtual Root Support
- •Further Documentation and Examples
- •Test Set Up and Tear Down
- •What?
- •Using the Configurator and pyramid.testing APIs in Unit Tests
- •Creating Integration Tests
- •Creating Functional Tests
- •Resources
- •Location-Aware Resources
- •Generating The URL Of A Resource
- •Overriding Resource URL Generation
- •Generating the Path To a Resource
- •Finding a Resource by Path
- •Obtaining the Lineage of a Resource
- •Determining if a Resource is In The Lineage of Another Resource
- •Finding the Root Resource
- •Resources Which Implement Interfaces
- •Finding a Resource With a Class or Interface in Lineage
- •Pyramid API Functions That Act Against Resources
- •Much Ado About Traversal
- •URL Dispatch
- •Historical Refresher
- •Traversal (aka Resource Location)
- •View Lookup
- •Use Cases
- •Traversal
- •Traversal Details
- •The Resource Tree
- •The Traversal Algorithm
- •A Description of The Traversal Algorithm
- •Traversal Algorithm Examples
- •References
- •Security
- •Enabling an Authorization Policy
- •Enabling an Authorization Policy Imperatively
- •Protecting Views with Permissions
- •Setting a Default Permission
- •Assigning ACLs to your Resource Objects
- •Elements of an ACL
- •Special Principal Names
- •Special Permissions
- •Special ACEs
- •ACL Inheritance and Location-Awareness
- •Changing the Forbidden View
- •Debugging View Authorization Failures
- •Debugging Imperative Authorization Failures
- •Creating Your Own Authentication Policy
- •Creating Your Own Authorization Policy
- •Combining Traversal and URL Dispatch
- •A Review of Non-Hybrid Applications
- •URL Dispatch Only
- •Traversal Only
- •Hybrid Applications
- •The Root Object for a Route Match
- •Using *traverse In a Route Pattern
- •Using *subpath in a Route Pattern
- •Corner Cases
- •Registering a Default View for a Route That Has a view Attribute
- •Using Hooks
- •Changing the Not Found View
- •Changing the Forbidden View
- •Changing the Request Factory
- •Using The Before Render Event
- •Adding Renderer Globals (Deprecated)
- •Using Response Callbacks
- •Using Finished Callbacks
- •Changing the Traverser
- •Changing How pyramid.request.Request.resource_url() Generates a URL
- •Changing How Pyramid Treats View Responses
- •Using a View Mapper
- •Creating a Tween Factory
- •Registering an Implicit Tween Factory
- •Suggesting Implicit Tween Ordering
- •Explicit Tween Ordering
- •Displaying Tween Ordering
- •Pyramid Configuration Introspection
- •Using the Introspector
- •Introspectable Objects
- •Pyramid Introspection Categories
- •Introspection in the Toolbar
- •Disabling Introspection
- •Rules for Building An Extensible Application
- •Fundamental Plugpoints
- •Extending an Existing Application
- •Extending the Application
- •Overriding Views
- •Overriding Routes
- •Overriding Assets
- •Advanced Configuration
- •Two-Phase Configuration
- •Using config.action in a Directive
- •Adding Configuration Introspection
- •Introspectable Relationships
- •Thread Locals
- •Why and How Pyramid Uses Thread Local Variables
- •Using the Zope Component Architecture in Pyramid
- •Using the ZCA Global API in a Pyramid Application
- •Disusing the Global ZCA API
- •Enabling the ZCA Global API by Using hook_zca
- •Enabling the ZCA Global API by Using The ZCA Global Registry
- •Background
- •Design
- •Overall
- •Models
- •Views
- •Security
- •Summary
- •Installation
- •Preparation
- •Make a Project
- •Run the Tests
- •Expose Test Coverage Information
- •Start the Application
- •Visit the Application in a Browser
- •Decisions the zodb Scaffold Has Made For You
- •Basic Layout
- •Resources and Models with models.py
- •Views With views.py
- •Defining the Domain Model
- •Delete the Database
- •Edit models.py
- •Look at the Result of Our Edits to models.py
- •View the Application in a Browser
- •Defining Views
- •Declaring Dependencies in Our setup.py File
- •Adding View Functions
- •Viewing the Result of all Our Edits to views.py
- •Adding Templates
- •Viewing the Application in a Browser
- •Adding Authorization
- •Add Authentication and Authorization Policies
- •Add security.py
- •Give Our Root Resource an ACL
- •Add Login and Logout Views
- •Change Existing Views
- •Add permission Declarations to our view_config Decorators
- •Add the login.pt Template
- •Change view.pt and edit.pt
- •See Our Changes To views.py and our Templates
- •View the Application in a Browser
- •Adding Tests
- •Test the Models
- •Test the Views
- •Functional tests
- •View the results of all our edits to tests.py
- •Run the Tests
- •Distributing Your Application
- •SQLAlchemy + URL Dispatch Wiki Tutorial
- •Background
- •Design
- •Overall
- •Models
- •Views
- •Security
- •Summary
- •Installation
- •Preparation
- •Making a Project
- •Running the Tests
- •Exposing Test Coverage Information
- •Initializing the Database
- •Starting the Application
- •Decisions the alchemy Scaffold Has Made For You
- •Basic Layout
- •View Declarations via views.py
- •Content Models with models.py
- •Making Edits to models.py
- •Changing scripts/initializedb.py
- •Reinitializing the Database
- •Viewing the Application in a Browser
- •Defining Views
- •Declaring Dependencies in Our setup.py File
- •Running setup.py develop
- •Changing the views.py File
- •Adding Templates
- •Adding Routes to __init__.py
- •Viewing the Application in a Browser
- •Adding Authorization
- •Adding A Root Factory
- •Add an Authorization Policy and an Authentication Policy
- •Adding an authentication policy callback
- •Adding Login and Logout Views
- •Changing Existing Views
- •Adding the login.pt Template
- •Seeing Our Changes To views.py and our Templates
- •Viewing the Application in a Browser
- •Adding Tests
- •Testing the Models
- •Testing the Views
- •Functional tests
- •Viewing the results of all our edits to tests.py
- •Running the Tests
- •Distributing Your Application
- •Converting a repoze.bfg Application to Pyramid
- •Running a Pyramid Application under mod_wsgi
- •pyramid.authorization
- •pyramid.authentication
- •Authentication Policies
- •Helper Classes
- •pyramid.chameleon_text
- •pyramid.chameleon_zpt
- •pyramid.config
- •pyramid.events
- •Functions
- •Event Types
- •pyramid.exceptions
- •pyramid.httpexceptions
- •HTTP Exceptions
- •pyramid.i18n
- •pyramid.interfaces
- •Event-Related Interfaces
- •Other Interfaces
- •pyramid.location
- •pyramid.paster
- •pyramid.registry
- •pyramid.renderers
- •pyramid.request
- •pyramid.response
- •Functions
- •pyramid.scripting
- •pyramid.security
- •Authentication API Functions
- •Authorization API Functions
- •Constants
- •Return Values
- •pyramid.settings
- •pyramid.testing
- •pyramid.threadlocal
- •pyramid.traversal
- •pyramid.url
- •pyramid.view
- •pyramid.wsgi
- •Glossary
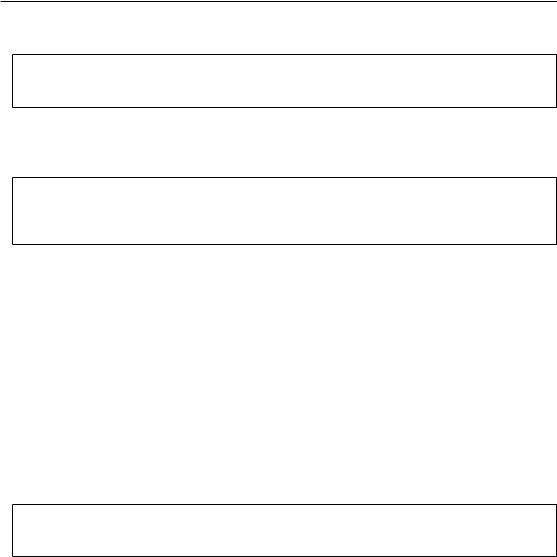
8. URL DISPATCH
If a predicate is a method you’ll need to assign it after method declaration (see PEP 232)
1
2
3
def custom_predicate(): pass
custom_predicate.__text__ = ’my custom method predicate’
If a predicate is a classmethod using @classmethod will not work, but you can still easily do it by wrapping it in classmethod call.
1 def classmethod_predicate():
2pass
3 classmethod_predicate.__text__ = ’my classmethod predicate’ 4 classmethod_predicate = classmethod(classmethod_predicate)
Same will work with staticmethod, just use staticmethod instead of classmethod.
See also pyramid.interfaces.IRoute for more API documentation about route objects.
8.12 Route Factories
Although it is not a particular common need in basic applications, a “route” configuration declaration can mention a “factory”. When that route matches a request, and a factory is attached to a route, the root factory passed at startup time to the Configurator is ignored; instead the factory associated with the route is used to generate a root object. This object will usually be used as the context resource of the view callable ultimately found via view lookup.
1
2
3
config.add_route(’abc’, ’/abc’, factory=’myproject.resources.root_factory’)
config.add_view(’myproject.views.theview’, route_name=’abc’)
The factory can either be a Python object or a dotted Python name (a string) which points to such a Python object, as it is above.
In this way, each route can use a different factory, making it possible to supply a different context resource object to the view related to each particular route.
A factory must be a callable which accepts a request and returns an arbitrary Python object. For example, the below class can be used as a factory:
92

8.13. USING PYRAMID SECURITY WITH URL DISPATCH
1
2
3
class Mine(object):
def __init__(self, request): pass
A route factory is actually conceptually identical to the root factory described at The Resource Tree.
Supplying a different resource factory for each route is useful when you’re trying to use a Pyramid authorization policy to provide declarative, “context sensitive” security checks; each resource can maintain a separate ACL, as documented in Using Pyramid Security With URL Dispatch. It is also useful when you wish to combine URL dispatch with traversal as documented within Combining Traversal and URL Dispatch.
8.13 Using Pyramid Security With URL Dispatch
Pyramid provides its own security framework which consults an authorization policy before allowing any application code to be called. This framework operates in terms of an access control list, which is stored as an __acl__ attribute of a resource object. A common thing to want to do is to attach an __acl__ to the resource object dynamically for declarative security purposes. You can use the factory argument that points at a factory which attaches a custom __acl__ to an object at its creation time.
Such a factory might look like so:
1 class Article(object):
2def __init__(self, request):
3matchdict = request.matchdict
4 article = matchdict.get(’article’, None)
5if article == ’1’:
6self.__acl__ = [ (Allow, ’editor’, ’view’) ]
If the route archives/{article} is matched, and the article number is 1, Pyramid will generate an Article context resource with an ACL on it that allows the editor principal the view permission. Obviously you can do more generic things than inspect the routes match dict to see if the article argument matches a particular string; our sample Article factory class is not very ambitious.
latex-note.png
See Security for more information about Pyramid security and ACLs.
93

8. URL DISPATCH
8.14 Route View Callable Registration and Lookup Details
When a request enters the system which matches the pattern of the route, the usual result is simple: the view callable associated with the route is invoked with the request that caused the invocation.
For most usage, you needn’t understand more than this; how it works is an implementation detail. In the interest of completeness, however, we’ll explain how it does work in the this section. You can skip it if you’re uninterested.
When a view is associated with a route configuration, Pyramid ensures that a view configuration is registered that will always be found when the route pattern is matched during a request. To do so:
•A special route-specific interface is created at startup time for each route configuration declaration.
•When an add_view statement mentions a route name attribute, a view configuration is registered at startup time. This view configuration uses a route-specific interface as a request type.
•At runtime, when a request causes any route to match, the request object is decorated with the route-specific interface.
•The fact that the request is decorated with a route-specific interface causes the view lookup machinery to always use the view callable registered using that interface by the route configuration to service requests that match the route pattern.
As we can see from the above description, technically, URL dispatch doesn’t actually map a URL pattern directly to a view callable. Instead, URL dispatch is a resource location mechanism. A Pyramid resource location subsystem (i.e., URL dispatch or traversal) finds a resource object that is the context of a request. Once the context is determined, a separate subsystem named view lookup is then responsible for finding and invoking a view callable based on information available in the context and the request. When URL dispatch is used, the resource location and view lookup subsystems provided by Pyramid are still being utilized, but in a way which does not require a developer to understand either of them in detail.
If no route is matched using URL dispatch, Pyramid falls back to traversal to handle the request.
8.15 References
A tutorial showing how URL dispatch can be used to create a Pyramid application exists in SQLAlchemy + URL Dispatch Wiki Tutorial.
94