
- •Contents
- •Introduction
- •Who This Book Is For
- •What This Book Covers
- •How This Book Is Structured
- •What You Need to Use This Book
- •Conventions
- •Source Code
- •Errata
- •p2p.wrox.com
- •The Basics of C++
- •The Obligatory Hello, World
- •Namespaces
- •Variables
- •Operators
- •Types
- •Conditionals
- •Loops
- •Arrays
- •Functions
- •Those Are the Basics
- •Diving Deeper into C++
- •Pointers and Dynamic Memory
- •Strings in C++
- •References
- •Exceptions
- •The Many Uses of const
- •C++ as an Object-Oriented Language
- •Declaring a Class
- •Your First Useful C++ Program
- •An Employee Records System
- •The Employee Class
- •The Database Class
- •The User Interface
- •Evaluating the Program
- •What Is Programming Design?
- •The Importance of Programming Design
- •Two Rules for C++ Design
- •Abstraction
- •Reuse
- •Designing a Chess Program
- •Requirements
- •Design Steps
- •An Object-Oriented View of the World
- •Am I Thinking Procedurally?
- •The Object-Oriented Philosophy
- •Living in a World of Objects
- •Object Relationships
- •Abstraction
- •Reusing Code
- •A Note on Terminology
- •Deciding Whether or Not to Reuse Code
- •Strategies for Reusing Code
- •Bundling Third-Party Applications
- •Open-Source Libraries
- •The C++ Standard Library
- •Designing with Patterns and Techniques
- •Design Techniques
- •Design Patterns
- •The Reuse Philosophy
- •How to Design Reusable Code
- •Use Abstraction
- •Structure Your Code for Optimal Reuse
- •Design Usable Interfaces
- •Reconciling Generality and Ease of Use
- •The Need for Process
- •Software Life-Cycle Models
- •The Stagewise and Waterfall Models
- •The Spiral Method
- •The Rational Unified Process
- •Software-Engineering Methodologies
- •Extreme Programming (XP)
- •Software Triage
- •Be Open to New Ideas
- •Bring New Ideas to the Table
- •Thinking Ahead
- •Keeping It Clear
- •Elements of Good Style
- •Documenting Your Code
- •Reasons to Write Comments
- •Commenting Styles
- •Comments in This Book
- •Decomposition
- •Decomposition through Refactoring
- •Decomposition by Design
- •Decomposition in This Book
- •Naming
- •Choosing a Good Name
- •Naming Conventions
- •Using Language Features with Style
- •Use Constants
- •Take Advantage of const Variables
- •Use References Instead of Pointers
- •Use Custom Exceptions
- •Formatting
- •The Curly Brace Alignment Debate
- •Coming to Blows over Spaces and Parentheses
- •Spaces and Tabs
- •Stylistic Challenges
- •Introducing the Spreadsheet Example
- •Writing Classes
- •Class Definitions
- •Defining Methods
- •Using Objects
- •Object Life Cycles
- •Object Creation
- •Object Destruction
- •Assigning to Objects
- •Distinguishing Copying from Assignment
- •The Spreadsheet Class
- •Freeing Memory with Destructors
- •Handling Copying and Assignment
- •Different Kinds of Data Members
- •Static Data Members
- •Const Data Members
- •Reference Data Members
- •Const Reference Data Members
- •More about Methods
- •Static Methods
- •Const Methods
- •Method Overloading
- •Default Parameters
- •Inline Methods
- •Nested Classes
- •Friends
- •Operator Overloading
- •Implementing Addition
- •Overloading Arithmetic Operators
- •Overloading Comparison Operators
- •Building Types with Operator Overloading
- •Pointers to Methods and Members
- •Building Abstract Classes
- •Using Interface and Implementation Classes
- •Building Classes with Inheritance
- •Extending Classes
- •Overriding Methods
- •Inheritance for Reuse
- •The WeatherPrediction Class
- •Adding Functionality in a Subclass
- •Replacing Functionality in a Subclass
- •Respect Your Parents
- •Parent Constructors
- •Parent Destructors
- •Referring to Parent Data
- •Casting Up and Down
- •Inheritance for Polymorphism
- •Return of the Spreadsheet
- •Designing the Polymorphic Spreadsheet Cell
- •The Spreadsheet Cell Base Class
- •The Individual Subclasses
- •Leveraging Polymorphism
- •Future Considerations
- •Multiple Inheritance
- •Inheriting from Multiple Classes
- •Naming Collisions and Ambiguous Base Classes
- •Interesting and Obscure Inheritance Issues
- •Special Cases in Overriding Methods
- •Copy Constructors and the Equals Operator
- •The Truth about Virtual
- •Runtime Type Facilities
- •Non-Public Inheritance
- •Virtual Base Classes
- •Class Templates
- •Writing a Class Template
- •How the Compiler Processes Templates
- •Distributing Template Code between Files
- •Template Parameters
- •Method Templates
- •Template Class Specialization
- •Subclassing Template Classes
- •Inheritance versus Specialization
- •Function Templates
- •Function Template Specialization
- •Function Template Overloading
- •Friend Function Templates of Class Templates
- •Advanced Templates
- •More about Template Parameters
- •Template Class Partial Specialization
- •Emulating Function Partial Specialization with Overloading
- •Template Recursion
- •References
- •Reference Variables
- •Reference Data Members
- •Reference Parameters
- •Reference Return Values
- •Deciding between References and Pointers
- •Keyword Confusion
- •The const Keyword
- •The static Keyword
- •Order of Initialization of Nonlocal Variables
- •Types and Casts
- •typedefs
- •Casts
- •Scope Resolution
- •Header Files
- •C Utilities
- •Variable-Length Argument Lists
- •Preprocessor Macros
- •How to Picture Memory
- •Allocation and Deallocation
- •Arrays
- •Working with Pointers
- •Array-Pointer Duality
- •Arrays Are Pointers!
- •Not All Pointers Are Arrays!
- •Dynamic Strings
- •C-Style Strings
- •String Literals
- •The C++ string Class
- •Pointer Arithmetic
- •Custom Memory Management
- •Garbage Collection
- •Object Pools
- •Function Pointers
- •Underallocating Strings
- •Memory Leaks
- •Double-Deleting and Invalid Pointers
- •Accessing Out-of-Bounds Memory
- •Using Streams
- •What Is a Stream, Anyway?
- •Stream Sources and Destinations
- •Output with Streams
- •Input with Streams
- •Input and Output with Objects
- •String Streams
- •File Streams
- •Jumping around with seek() and tell()
- •Linking Streams Together
- •Bidirectional I/O
- •Internationalization
- •Wide Characters
- •Non-Western Character Sets
- •Locales and Facets
- •Errors and Exceptions
- •What Are Exceptions, Anyway?
- •Why Exceptions in C++ Are a Good Thing
- •Why Exceptions in C++ Are a Bad Thing
- •Our Recommendation
- •Exception Mechanics
- •Throwing and Catching Exceptions
- •Exception Types
- •Throwing and Catching Multiple Exceptions
- •Uncaught Exceptions
- •Throw Lists
- •Exceptions and Polymorphism
- •The Standard Exception Hierarchy
- •Catching Exceptions in a Class Hierarchy
- •Writing Your Own Exception Classes
- •Stack Unwinding and Cleanup
- •Catch, Cleanup, and Rethrow
- •Use Smart Pointers
- •Common Error-Handling Issues
- •Memory Allocation Errors
- •Errors in Constructors
- •Errors in Destructors
- •Putting It All Together
- •Why Overload Operators?
- •Limitations to Operator Overloading
- •Choices in Operator Overloading
- •Summary of Overloadable Operators
- •Overloading the Arithmetic Operators
- •Overloading Unary Minus and Unary Plus
- •Overloading Increment and Decrement
- •Overloading the Subscripting Operator
- •Providing Read-Only Access with operator[]
- •Non-Integral Array Indices
- •Overloading the Function Call Operator
- •Overloading the Dereferencing Operators
- •Implementing operator*
- •Implementing operator->
- •What in the World Is operator->* ?
- •Writing Conversion Operators
- •Ambiguity Problems with Conversion Operators
- •Conversions for Boolean Expressions
- •How new and delete Really Work
- •Overloading operator new and operator delete
- •Overloading operator new and operator delete with Extra Parameters
- •Two Approaches to Efficiency
- •Two Kinds of Programs
- •Is C++ an Inefficient Language?
- •Language-Level Efficiency
- •Handle Objects Efficiently
- •Use Inline Methods and Functions
- •Design-Level Efficiency
- •Cache as Much as Possible
- •Use Object Pools
- •Use Thread Pools
- •Profiling
- •Profiling Example with gprof
- •Cross-Platform Development
- •Architecture Issues
- •Implementation Issues
- •Platform-Specific Features
- •Cross-Language Development
- •Mixing C and C++
- •Shifting Paradigms
- •Linking with C Code
- •Mixing Java and C++ with JNI
- •Mixing C++ with Perl and Shell Scripts
- •Mixing C++ with Assembly Code
- •Quality Control
- •Whose Responsibility Is Testing?
- •The Life Cycle of a Bug
- •Bug-Tracking Tools
- •Unit Testing
- •Approaches to Unit Testing
- •The Unit Testing Process
- •Unit Testing in Action
- •Higher-Level Testing
- •Integration Tests
- •System Tests
- •Regression Tests
- •Tips for Successful Testing
- •The Fundamental Law of Debugging
- •Bug Taxonomies
- •Avoiding Bugs
- •Planning for Bugs
- •Error Logging
- •Debug Traces
- •Asserts
- •Debugging Techniques
- •Reproducing Bugs
- •Debugging Reproducible Bugs
- •Debugging Nonreproducible Bugs
- •Debugging Memory Problems
- •Debugging Multithreaded Programs
- •Debugging Example: Article Citations
- •Lessons from the ArticleCitations Example
- •Requirements on Elements
- •Exceptions and Error Checking
- •Iterators
- •Sequential Containers
- •Vector
- •The vector<bool> Specialization
- •deque
- •list
- •Container Adapters
- •queue
- •priority_queue
- •stack
- •Associative Containers
- •The pair Utility Class
- •multimap
- •multiset
- •Other Containers
- •Arrays as STL Containers
- •Strings as STL Containers
- •Streams as STL Containers
- •bitset
- •The find() and find_if() Algorithms
- •The accumulate() Algorithms
- •Function Objects
- •Arithmetic Function Objects
- •Comparison Function Objects
- •Logical Function Objects
- •Function Object Adapters
- •Writing Your Own Function Objects
- •Algorithm Details
- •Utility Algorithms
- •Nonmodifying Algorithms
- •Modifying Algorithms
- •Sorting Algorithms
- •Set Algorithms
- •The Voter Registration Audit Problem Statement
- •The auditVoterRolls() Function
- •The getDuplicates() Function
- •The RemoveNames Functor
- •The NameInList Functor
- •Testing the auditVoterRolls() Function
- •Allocators
- •Iterator Adapters
- •Reverse Iterators
- •Stream Iterators
- •Insert Iterators
- •Extending the STL
- •Why Extend the STL?
- •Writing an STL Algorithm
- •Writing an STL Container
- •The Appeal of Distributed Computing
- •Distribution for Scalability
- •Distribution for Reliability
- •Distribution for Centrality
- •Distributed Content
- •Distributed versus Networked
- •Distributed Objects
- •Serialization and Marshalling
- •Remote Procedure Calls
- •CORBA
- •Interface Definition Language
- •Implementing the Class
- •Using the Objects
- •A Crash Course in XML
- •XML as a Distributed Object Technology
- •Generating and Parsing XML in C++
- •XML Validation
- •Building a Distributed Object with XML
- •SOAP (Simple Object Access Protocol)
- •. . . Write a Class
- •. . . Subclass an Existing Class
- •. . . Throw and Catch Exceptions
- •. . . Read from a File
- •. . . Write to a File
- •. . . Write a Template Class
- •There Must Be a Better Way
- •Smart Pointers with Reference Counting
- •Double Dispatch
- •Mix-In Classes
- •Object-Oriented Frameworks
- •Working with Frameworks
- •The Model-View-Controller Paradigm
- •The Singleton Pattern
- •Example: A Logging Mechanism
- •Implementation of a Singleton
- •Using a Singleton
- •Example: A Car Factory Simulation
- •Implementation of a Factory
- •Using a Factory
- •Other Uses of Factories
- •The Proxy Pattern
- •Example: Hiding Network Connectivity Issues
- •Implementation of a Proxy
- •Using a Proxy
- •The Adapter Pattern
- •Example: Adapting an XML Library
- •Implementation of an Adapter
- •Using an Adapter
- •The Decorator Pattern
- •Example: Defining Styles in Web Pages
- •Implementation of a Decorator
- •Using a Decorator
- •The Chain of Responsibility Pattern
- •Example: Event Handling
- •Implementation of a Chain of Responsibility
- •Using a Chain of Responsibility
- •Example: Event Handling
- •Implementation of an Observer
- •Using an Observer
- •Chapter 1: A Crash Course in C++
- •Chapter 3: Designing with Objects
- •Chapter 4: Designing with Libraries and Patterns
- •Chapter 5: Designing for Reuse
- •Chapter 7: Coding with Style
- •Chapters 8 and 9: Classes and Objects
- •Chapter 11: Writing Generic Code with Templates
- •Chapter 14: Demystifying C++ I/O
- •Chapter 15: Handling Errors
- •Chapter 16: Overloading C++ Operators
- •Chapter 17: Writing Efficient C++
- •Chapter 19: Becoming Adept at Testing
- •Chapter 20: Conquering Debugging
- •Chapter 24: Exploring Distributed Objects
- •Chapter 26: Applying Design Patterns
- •Beginning C++
- •General C++
- •I/O Streams
- •The C++ Standard Library
- •C++ Templates
- •Integrating C++ and Other Languages
- •Algorithms and Data Structures
- •Open-Source Software
- •Software-Engineering Methodology
- •Programming Style
- •Computer Architecture
- •Efficiency
- •Testing
- •Debugging
- •Distributed Objects
- •CORBA
- •XML and SOAP
- •Design Patterns
- •Index

Delving into the STL: Containers and Iterators
protected: RoundRobin<Host> rr;
};
LoadBalancer::LoadBalancer(const vector<Host>& hosts)
{
// Add the hosts.
for (size_t i = 0; i < hosts.size(); ++i) { rr.add(hosts[i]);
}
}
void LoadBalancer::distributeRequest(NetworkRequest& request)
{
try { rr.getNext().processRequest(request);
} catch (out_of_range& e) { cerr << “No more hosts.\n”;
}
}
The vector<bool> Specialization
The standard requires a partial specialization of vector for bools, with the intention that it optimize space allocation by “packing” the Boolean values. Recall that a bool is either true or false, and thus could be represented by a single bit, which can take on exactly two values. However, most C++ compilers make bools the same size as ints. The vector<bool> is supposed to store the “array of bools” in single bits, thus saving space.
You can think of the vector<bool> as a bit-field instead of a vector. The bitset container described below provides a more full-featured bit-field implementation than does vector<bool>. However, the benefit of vector<bool> is that it can change size dynamically.
In a half-hearted attempt to provide some bit-field routines for the vector<bool>, there is one additional method: flip(). This method can be called on either the container, in which case it negates all the elements in the container, or a single reference returned from operator[] or a similar method, in which case it negates that element.
At this point, you should be wondering how you can call a method on a reference to bool. The answer is that you can’t. The vector<bool> specialization actually defines a class called reference that serves as a proxy for the underlying bool (or bit). When you call operator[], at(), or a similar method, the vector<bool> returns a reference object, which is a proxy for the real bool.
The fact that references returned from vector<bool> are really proxies means that you can’t take their addressees to obtain pointers to the actual elements in the container. The proxy design pattern is covered in detail in Chapter 26.
583

Chapter 21
In practice, the little amount of space saved by packing bools hardly seems worth the extra effort. However, you should be familiar with this partial instantiation because of the additional flip() method, and because of the fact that references are actually proxy objects. Many C++ experts recommend avoiding vector<bool> in favor of the bitset, unless you really need a dynamically sized bit-field.
deque
The deque is almost identical to the vector, but is used far less frequently. The principle differences are:
The implementation is not required to store elements contiguously in memory.
The deque supports constant-time insertion and removal of elements at both the front and the back (the vector supports amortized constant time at just the back).
The deque provides push_front() and pop_front(), which the vector omits.
The deque does not expose its memory management scheme via reserve() or capacity().
Rarely will your applications require a deque, as opposed to a vector or list. Thus, we leave the details of the deque methods to the Standard Library Reference resource on the Web site.
list
The STL list is a standard doubly linked list. It supports constant-time insertion and deletion of elements at any point in the list, but provides slow (linear) time access to individual elements. In fact, the list does not even provide random access operations like operator[]. Only through iterators can you access individual elements.
Most of the list operations are identical to those of the vector, including the constructors, destructor, copying operations, assignment operations, and comparison operations. This section focuses on those methods that differ from those of vector. Consult the Standard Library Reference resource on the Web site for details on the list methods not discussed here.
Accessing Elements
The only methods provided by the list to access elements are front() and back(), both of which run in constant time. All other element access must be performed through iterators.
Lists do not provide random access to elements.
Iterators
The list iterator is bidirectional, not random access like the vector iterator. That means that you cannot add and subtract list iterators from each other, or perform other pointer arithmetic on them.
Adding and Removing Elements
The list supports the same element add and remove methods that does the vector, including push_back(), pop_back(), the three forms of insert(), the two forms of erase(), and clear().
584
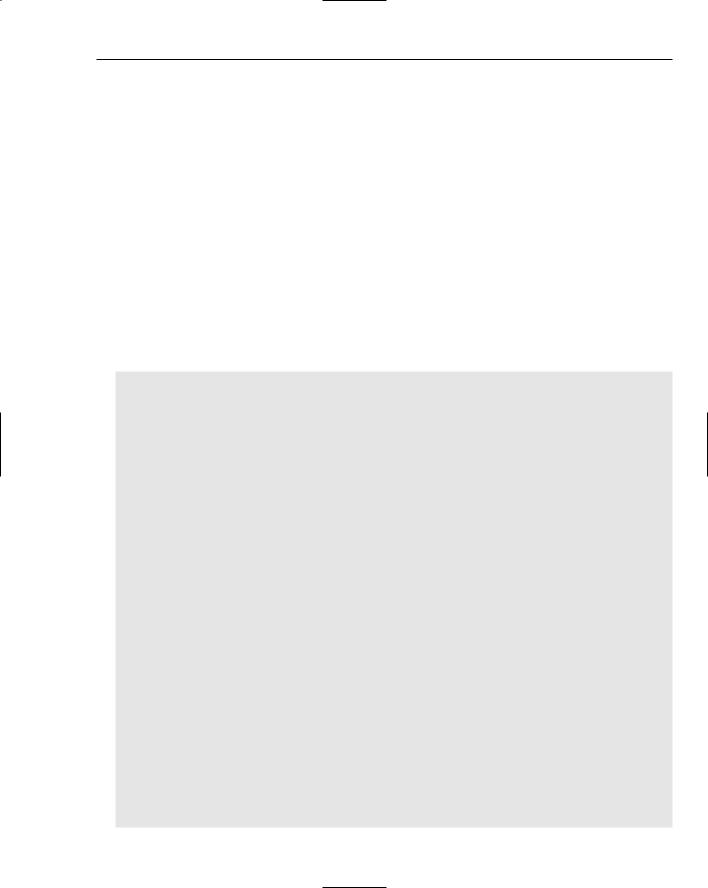
Delving into the STL: Containers and Iterators
Like the deque, it also provides push_front() and pop_front(). The amazing thing about the list is that all these methods (except for clear()) run in constant time, once you’ve found the correct position. Thus, the list is appropriate for applications that perform many insertions and deletions from the data structure, but do not need quick index-based element access.
List Size
Like deques, and unlike vectors, lists do not expose their underlying memory model. Consequently, they support size() and empty(), but not resize() or capacity().
Special List Operations
The list provides several special operations that exploit its quick element insertion and deletion. This section provides an overview and examples. The Standard Library Reference resource on the Web site gives a thorough reference for all the methods.
Splicing
The linked-list characteristics of the list class allow it to splice, or insert, an entire list at any position in another list in constant time. The simplest version of this method works like this:
#include <list> #include <string> #include <iostream> using namespace std;
int main(int argc, char** argv)
{
list<string> dictionary, bWords;
//Add the a words. dictionary.push_back(“aardvark”); dictionary.push_back(“ambulance”); dictionary.push_back(“archive”);
//Add the c words. dictionary.push_back(“canticle”); dictionary.push_back(“consumerism”); dictionary.push_back(“czar”);
//Create another list, of the b words. bWords.push_back(“bathos”); bWords.push_back(“balderdash”); bWords.push_back(“brazen”);
//Splice the b words into the main dictionary. list<string>::iterator it;
int i;
//Iterate up to the spot where we want to insert bs
//for loop body intentionally empty--we’re just moving up three elements. for (it = dictionary.begin(), i = 0; i < 3; ++it, ++i);
//Add in the bwords. This action removes the elements from bWords. dictionary.splice(it, bWords);
585

Chapter 21
// Print out the dictionary.
for (it = dictionary.begin(); it != dictionary.end(); ++it) { cout << *it << endl;
}
return (0);
}
The result from running this program looks like this:
aardvark ambulance archive bathos balderdash brazen canticle consumerism czar
There are also two other forms of splice(): one that inserts a single element from another list and one that inserts a range from another list. See the Standard Library Reference resource on the Web site for details.
Splicing is destructive to the list passed as a parameter: it removes the spliced elements from one list in order to insert them into the other.
More Efficient Versions of Algorithms
In addition to splice(), the list class provides special implementations of several of the generic STL algorithms. The generic forms are covered in Chapter 22. Here we discuss only the specific versions provided by list.
When you have a choice, use the list methods rather than the generic algorithms because the former are more efficient.
The following table summarizes the algorithms for which list provides special implementations as methods. See the Standard Library Reference resource on the Web site and Chapter 22 for prototypes, details on the algorithms, and their specific running time when called on list.
Method |
Description |
|
|
remove() |
Removes certain elements from the list. |
remove_if() |
|
unique() |
Removes duplicate consecutive elements from the list. |
merge() |
Merges two lists. Both lists must be sorted to start. Like splice(), |
|
merge() is destructive to the list passed as an argument. |
|
|
586
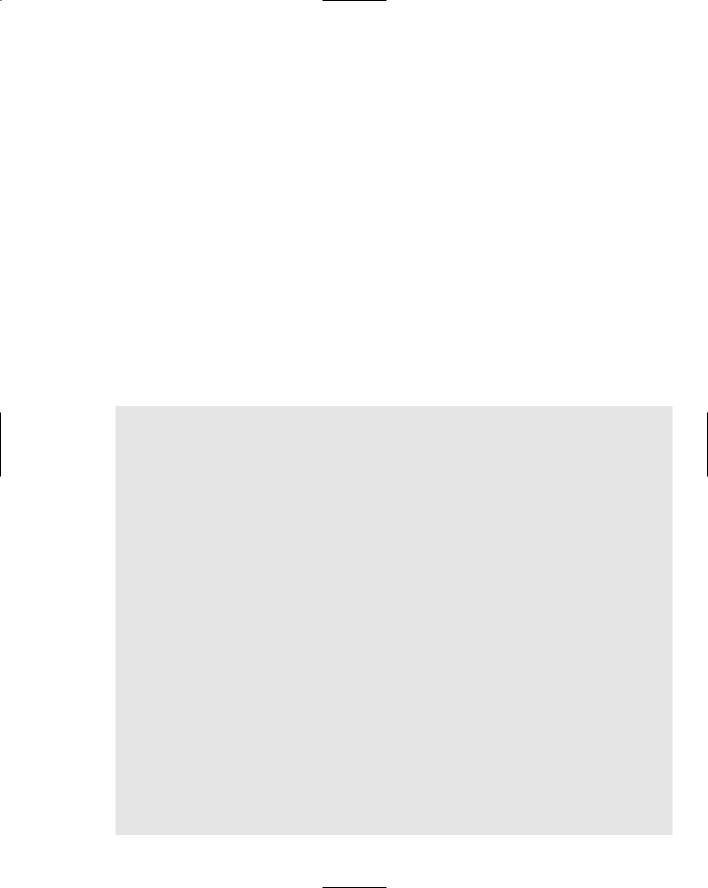
|
|
Delving into the STL: Containers and Iterators |
|
|
|
|
Method |
Description |
|
|
|
|
sort() |
Performs a stable sort on elements in the list. |
|
reverse() |
Reverses the order of the elements in the list. |
|
|
|
The following program demonstrates most of these methods.
List Example: Determining Enrollment
Suppose that you are writing a computer registration system for a university. One feature you might provide is the ability to generate a complete list of enrolled students in the university from lists of the students in each class. For the sake of this example, assume that you must write only a single function that takes a vector of lists of student names (as strings), plus a list of students that have been dropped from their courses because they failed to pay tuition. This method should generate a complete list of all the students in all the courses, without any duplicates, and without those students who have been dropped. Note that students might be in more than one course.
Here is the code for this method. With the power of the STL lists, the method is practically shorter than its written description! Note that the STL allows you to “nest” containers: in this case, you can use a vector of lists.
#include <list> #include <vector> #include <string> using namespace std;
//
//classLists is a vector of lists, one for each course. The lists
//contain the students enrolled in those courses. They are not sorted.
//droppedStudents is a list of students who failed to pay their
//tuition and so were dropped from their courses.
//
//The function returns a list of every enrolled (nondropped) student in
//all the courses.
//
list<string>
getTotalEnrollment(const vector<list<string> >& classLists, const list<string>& droppedStudents)
{
list<string> allStudents;
// Concatenate all the course lists onto the master list. for (size_t i = 0; i < classLists.size(); ++i) {
allStudents.insert(allStudents.end(), classLists[i].begin(), classLists[i].end());
}
//Sort the master list. allStudents.sort();
//Remove duplicate student names (those who are in multiple courses). allStudents.unique();
587