
- •Microsoft C# Programming for the Absolute Beginner
- •Table of Contents
- •Microsoft C# Programming for the Absolute Beginner
- •Introduction
- •Overview
- •Chapter 1: Basic Input and Output: A Mini Adventure
- •Project: The Mini Adventure
- •Reviewing Basic C# Concepts
- •Namespaces
- •Classes
- •Methods
- •Statements
- •The Console Object
- •.NET Documentation
- •Getting into the Visual Studio .Net Environment
- •Examining the Default Code
- •Creating a Custom Namespace
- •Adding Summary Comments
- •Creating the Class
- •Moving from Code to a Program
- •Compiling Your Program
- •Looking for Bugs
- •Getting Input from the User
- •Creating a String Variable
- •Getting a Value with the Console.ReadLine() Method
- •Incorporating a Variable in Output
- •Combining String Values
- •Combining Strings with Concatenation
- •Adding a Tab Character
- •Using the Newline Sequence
- •Displaying a Backslash
- •Displaying Quotation Marks
- •Launching the Mini Adventure
- •Planning the Story
- •Creating the Variables
- •Getting Values from the User
- •Writing the Output
- •Finishing the Program
- •Summary
- •Chapter 2: Branching and Operators: The Math Game
- •The Math Game
- •Using Numeric Variables
- •The Simple Math Game
- •Numeric Variable Types
- •Integer Variables
- •Long Integers
- •Data Type Problems
- •Math Operators
- •Converting Variables
- •Explicit Casting
- •The Convert Object
- •Creating a Branch in Program Logic
- •The Hi Bill Game
- •Condition Testing
- •The If Statement
- •The Else Clause
- •Multiple Conditions
- •Working with The Switch Statement
- •The Switch Demo Program
- •Examining How Switch Statements Work
- •Creating a Random Number
- •Introducing the Die Roller
- •Exploring the Random Object
- •Creating a Random Double with the .NextDouble() Method
- •Getting the Values of Dice
- •Creating the Math Game
- •Designing the Game
- •Creating the Variables
- •Managing Addition
- •Managing Subtraction
- •Managing Multiplication and Division
- •Checking the Answers
- •Waiting for the Carriage Return
- •Summary
- •Chapter 3: Loops and Strings: The Pig Latin Program
- •Project: The Pig Latin Program
- •Investigating The String Object
- •The String Mangler Program
- •A Closer Look at Strings
- •Using the Object Browser
- •Experimenting with String Methods
- •Performing Common String Manipulations
- •Using a For Loop
- •Examining The Bean Counter Program
- •Creating a Sentry Variable
- •Checking for an Upper Limit
- •Incrementing the Variable
- •Examining the Behavior of the For Loop
- •The Fancy Beans Program
- •Skipping Numbers
- •Counting Backwards
- •Using a Foreach Loop to Break Up a Sentence
- •Using a While Loop
- •The Magic Word Program
- •Writing an Effective While Loop
- •Planning Your Program with the STAIR Process
- •S: State the Problem
- •T: Tool Identification
- •A: Algorithm
- •I: Implementation
- •R: Refinement
- •Applying STAIR to the Pig Latin Program
- •Stating the Problem
- •Identifying the Tools
- •Creating the Algorithm
- •Implementing and Refining
- •Writing the Pig Latin Program
- •Setting Up the Variables
- •Creating the Outside Loop
- •Dividing the Phrase into Words
- •Extracting the First Character
- •Checking for a Vowel
- •Adding Debugging Code
- •Closing Up the code
- •Summary
- •Introducing the Critter Program
- •Creating Methods to Reuse Code
- •The Song Program
- •Building the Main() Method
- •Creating a Simple Method
- •Adding a Parameter
- •Returning a Value
- •Creating a Menu
- •Creating a Main Loop
- •Creating the Sentry Variable
- •Calling a Method
- •Working with the Results
- •Writing the showMenu() Method
- •Getting Input from the User
- •Handling Exceptions
- •Returning a Value
- •Creating a New Object with the CritterName Program
- •Creating the Basic Critter
- •Using Scope Modifiers
- •Using a Public Instance Variable
- •Creating an Instance of the Critter
- •Adding a Method
- •Creating the talk() Method for the CritterTalk Program
- •Changing the Menu to Use the talk() Method
- •Creating a Property in the CritterProp Program
- •Examining the Critter Prop Program
- •Creating the Critter with a Name Property
- •Using Properties as Filters
- •Making the Critter More Lifelike
- •Adding More Private Variables
- •Adding the Age() Method
- •Adding the Eat() Method
- •Adding the Play() Method
- •Modifying the Talk() Method
- •Making Changes in the Main Class
- •Summary
- •Introducing the Snowball Fight
- •Inheritance and Encapsulation
- •Creating a Constructor
- •Adding a Constructor to the Critter Class
- •Creating the CritViewer Class
- •Reviewing the Static Keyword
- •Calling a Constructor from the Main() Method
- •Working with Multiple Files
- •Overloading Constructors
- •Viewing the Improved Critter Class
- •Adding Polymorphism to Your Objects
- •Modifying the Critter Viewer in CritOver to Demonstrate Overloaded Constructors
- •Using Inheritance to Make New Classes
- •Creating a Class to View the Clone
- •Creating the Critter Class
- •Improving an Existing Class
- •Introducing the Glitter Critter
- •Adding Methods to a New Class
- •Changing the Critter Viewer Again
- •Creating the Snowball Fight
- •Building the Fighter
- •Building the Robot Fighter
- •Creating the Main Menu Class
- •Summary
- •Overview
- •Introducing the Visual Critter
- •Thinking Like a GUI Programmer
- •Creating a Graphical User Interface (GUI)
- •Examining the Code of a Windows Program
- •Adding New Namespaces
- •Creating the Form Object
- •Creating a Destructor
- •Creating the Components
- •Setting Component Properties
- •Setting Up the Form
- •Writing the Main() Method
- •Creating an Interactive Program
- •Responding to a Simple Event
- •Creating and Adding the Components
- •Adding an Event to the Program
- •Creating an Event Handler
- •Allowing for Multiple Selections
- •Choosing a Font with Selection Controls
- •Creating the User Interface
- •Examining Selection Tools
- •Creating Instance Variables in the Font Chooser
- •Writing the AssignFont() Method
- •Writing the Event Handlers
- •Working with Images and Scroll Bars
- •Setting Up the Picture Box
- •Adding a Scroll Bar
- •Revisiting the Visual Critter
- •Designing the Program
- •Determining the Necessary Tools
- •Designing the Form
- •Writing the Code
- •Summary
- •Chapter 7: Timers and Animation: The Lunar Lander
- •Introducing the Lunar Lander
- •Reading Values from the Keyboard
- •Introducing the Key Reader Program
- •Setting Up the Key Reader Program
- •Coding the KeyPress Event
- •Coding the KeyDown Event
- •Determining Which Key Was Pressed
- •Animating Images
- •Introducing the ImageList Control
- •Setting Up an Image List
- •Looking at the Image Collection
- •Displaying an Image from the Image List
- •Using a Timer to Automate Animation
- •Introducing the Timer Control
- •Configuring the Timer
- •Adding Motion
- •Checking for Keyboard Input
- •Working with the Location Property
- •Detecting Collisions between Objects
- •Coding the Crasher Program
- •Getting Values for newX and newY
- •Bouncing the Ball off the Sides
- •Checking for Collisions
- •Extracting a Rectangle from a Component
- •Getting More from the MessageBox Object
- •Introducing the MsgDemo Program
- •Retrieving Values from the MessageBox
- •Coding the Lunar Lander
- •The Visual Design
- •The Constructor
- •The timer1_Tick() Method
- •The moveShip() Method
- •The checkLanding() Method
- •The theForm_KeyDown() Method
- •The showStats() Method
- •The killShip() Method
- •The initGame() Method
- •Summary
- •Chapter 8: Arrays: The Soccer Game
- •The Soccer Game
- •Introducing Arrays
- •Exploring the Counter Program
- •Creating an Array of Strings
- •Referring to Elements in an Array
- •Working with Arrays
- •Using the Array Demo Program to Explore Arrays
- •Building the Languages Array
- •Sorting the Array
- •Designing the Soccer Game
- •Solving a Subset of the Problem
- •Adding Percentages for the Other Players
- •Setting Up the Shot Demo Program
- •Setting Up the List Boxes
- •Using a Custom Event Handler
- •Writing the changeStatus() Method
- •Kicking the Ball
- •Designing Programs by Hand
- •Examining the Form by Hand Program
- •Adding Components in the Constructor
- •Responding to the Button Event
- •Building the Soccer Program
- •Setting Up the Variables
- •Examining the Constructor
- •Setting Up the Players
- •Setting Up the Opponents
- •Setting Up the Goalies
- •Responding to Player Clicks
- •Handling Good Shots
- •Handling Bad Shots
- •Setting a New Current Player
- •Handling the Passage of Time
- •Updating the Score
- •Summary
- •Chapter 9: File Handling: The Adventure Kit
- •Introducing the Adventure Kit
- •Viewing the Main Screen
- •Loading an Adventure
- •Playing an Adventure
- •Creating an Adventure
- •Reading and Writing Text Files
- •Exploring the File IO Program
- •Importing the IO Namespace
- •Writing to a Stream
- •Reading from a Stream
- •Creating Menus
- •Exploring the Menu Demo Program
- •Adding a MainMenu Object
- •Adding a Submenu
- •Setting Up the Properties of Menu Items
- •Writing Event Code for Menus
- •Using Dialog Boxes to Enhance Your Programs
- •Exploring the Dialog Demo Program
- •Adding Standard Dialogs to Your Form
- •Using the File Dialog Controls
- •Responding to File Dialog Events
- •Using the Font Dialog Control
- •Using the Color Dialog Control
- •Storing Entire Objects with Serialization
- •Exploring the Serialization Demo Program
- •Creating the Contact Class
- •Referencing the Serializable Namespace
- •Storing a Class
- •Retrieving a Class
- •Returning to the Adventure Kit Program
- •Examining the Room Class
- •Creating the Dungeon Class
- •Writing the Game Class
- •Writing the Editor Class
- •Writing the MainForm Class
- •Summary
- •Chapter 10: Chapter Basic XML: The Quiz Maker
- •Introducing the Quiz Maker Game
- •Taking a Quiz
- •Creating and Editing Quizzes
- •Investigating XML
- •Defining XML
- •Creating an XML Document in .NET
- •Creating an XML Schema for Your Language
- •Investigating the .NET View of XML
- •Exploring the XmlNode Class
- •Exploring the XmlDocument Class
- •Reading an Existing XML Document
- •Creating the XML Viewer Program
- •Writing New Values to an XML Document
- •Building the Document Structure
- •Adding an Element to the Document
- •Displaying the XML Code
- •Examining the Quizzer Program
- •Building the Main Form
- •Writing the Quiz Form
- •Writing the Editor Form
- •Summary
- •Overview
- •Introducing the SpyMaster Program
- •Creating a Simple Database
- •Accessing the Data Server
- •Accessing the Data in a Program
- •Using Queries to Modify Data Results
- •Limiting Data with the SELECT Statement
- •Using an Existing Database
- •Adding the Capability to Display Queries
- •Creating a Visual Query Builder
- •Working with Relational Databases
- •Improving Your Data with Normalization
- •Using a Join to Connect Two Tables
- •Creating a View
- •Referring to a View in a Program
- •Incorporating the Agent Specialty Attribute
- •Working with Other Databases
- •Creating a New Connection
- •Converting a Data Set to XML
- •Reading from XML to a Data Source
- •Creating the SpyMaster Database
- •Building the Main Form
- •Editing the Assignments
- •Editing the Specialties
- •Viewing the Agents
- •Editing the Agent Data
- •Summary
- •List of Figures
- •List of Tables
- •List of Sidebars

Figure 11.22: This query limits both the number rows and the number of columns.
In essence, the SELECT statement is used to filter the data to answer some kind of question.
Trap I allowed the user to type in a SELECT statement here simply to keep the code simple. As you can imagine, real users don’t usually know much about SQL, so they probably would really mess this program up. It would be better to build some sort of visual mechanism that creates the SQL statement on the fly than to let the user directly enter SQL. You’ll see some examples of that later in the chapter.
Using an Existing Database
The Query Demo program is based on the existing SimpleSpy database.
I already created the Simple Spy database, so I don’t have to make it again. Simply start a new project, open the server explorer, and the Simple Spy database will still be there. The databases are a function of the entire machine. They are not necessarily tied to any particular project. Drag the Agents table onto the new form, and rename the resulting SqlDataConnection and SqlDataAdapter. Create a new data set from the DataAdapter, and add a data grid to the form. Your form should look something like Figure 11.23.
338

Figure 11.23: The query demo has been attached to the SimpleSpy database.
To finish the connection, set the data grid’s datasource to myDS, and its data member to Agents. Finally, fill the data set in the form’s load method:
private void QueryDemoForm_Load(object sender, System.EventArgs e) {
myAdapter.Fill(myDS); } // end form load
Hint In the last few steps, you have recreated the entire simple spy program. It’s interesting that a procedure that took 20 minutes or more the first time can be done in just a few minutes the second time. With a little practice, you’ll be able to completely hook up a database in a minute or two.
Adding the Capability to Display Queries
The visual layout of the form is not challenging. It contains a data grid called dgSpies, a textbox called txtQuery, and a button called btnQuery. All the action happens in the btnQuery click event:
private void btnQuery_Click(object sender, System.EventArgs e) {
DataSet qDS = new DataSet("results"); myAdapter.SelectCommand.CommandText = txtQuery.Text; myAdapter.Fill(qDS, "results"); dgSpies.SetDataBinding(qDS, "results");
} // end btnQuerey
The code begins by creating a new DataSet object called qDS (for query data set). This new data set has one table, called results. The qDS data set holds the results of the query in its results table. Remember, a data set is a local copy of a database. It must be filled from some sort of data adapter. The new data set gets a subset of the original database. The data adapter class has a SelectCommand property that holds a command for selecting the data. This property holds an SQLCommand object, which has a CommandText property. I can set the CommandText property to a SELECT statement, and this applies to all subsequent fill statements related to the adapter. I use
339

the adapter’s Fill() method to fill qDS with the filtered data. Finally, I used the SetDataBinding() method of the data grid to apply the new Data Set to the data grid and display the results.
Letting the user enter information in a text box is just too risky, so I added an exception handling routine so the program won’t choke up if the user enters a query in some odd format (like “get me all the spies who do sabotage.”)
DataSet qDS = new DataSet("results"); myAdapter.SelectCommand.CommandText =
txtQuery.Text; try {
myAdapter.Fill(qDS, "results"); dgSpies.SetDataBinding(qDS, "results");
}catch (Exception exc){ MessageBox.Show("Something went wrong"); myAdapter.SelectCommand.CommandText =
"Select * from Agents"; myAdapter.Fill(qDS, "results"); dgSpies.SetDataBinding(qDS, "results");
} // end try
}// end btnQuery
Creating a Visual Query Builder
It isn’t reasonable to expect your users to know how to build a SELECT statement. Instead, you may want to prepare some predefined queries for the user, or you might want to build a visual query builder. Figures 11.24 and 11.25 illustrate a program that enables the user to build queries dynamically.
Figure 11.24: The form is just like QueryDemo, but it adds some other controls for building the
340
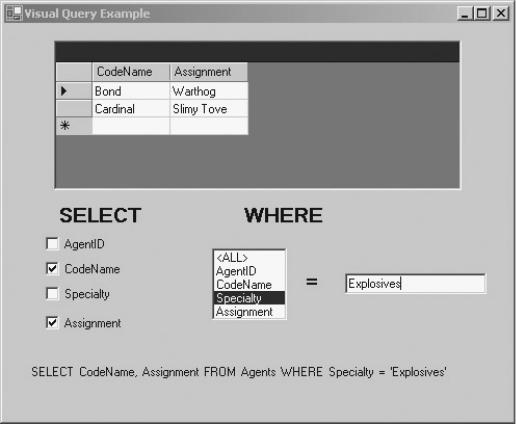
query.
Figure 11.25: If the user types a value into the textbox and chooses a field from the listbox, only records that satisfy the resulting condition are displayed.
The program displays the SELECT statement as it is being built. The query is automatically updated every time any of the screen elements is changed. This program is very easy to use, and it provides a great deal of power to the user.
Creating the Layout for the Visual Query Builder
The Visual Query program uses a number of controls. The most prominent of these is a data grid, which is bound to a data set as you have done several times in this chapter. To create the bound data grid, follow these steps:
1.Locate your database in the server explorer pane.
2.Drag the Agents table to the form.
3.Rename the resulting DataConnection and DataAdapter Objects.
4.Create a data set from the DataAdapter (using the link at the bottom of the property menu).
5.Rename the resulting instance of the data set.
6.Set the DataSource property of the data grid to the data set.
7.Set the DataMember property of the data grid to the Agents table.
8.Call the data adapter’s fill method to fill the data set in the form’s load event.
Hint It might seem crazy to attach to the same database three different times, but it’s really good practice. Repetition is the best way to ensure you know how to attach to a database. Every data application you write starts out something like this, so it’s a good skill to rehearse.
Figure 11.26 shows the Visual Query form in the designer.
341

Figure 11.26: The Visual Query form features checkboxes, list boxes, and a couple of labels.
The check boxes have predictable names (chkAgentID, for example). The list box is called lstField, and I loaded the field names into the list box in design time. The text box is called txtMatch, and the label holding the query is called (cleverly enough) lblQuery. The only class–level variable is a string called theQuery.
Initializing the Code in the Load Event
As usual, there is some initialization in the form’s load event:
private void VisQueryForm_Load(object sender, System.EventArgs e) {
myAdapter.Fill(myDS, "Agents"); lstField.SelectedIndex = 0;
} // end form load
The load event has only two tasks. First, it fills up the data set from the adapter. Second, it selects element 0 in the list box, so there is always some element selected in the list box.
Building a Query
All the elements on the form cooperate to build a SELECT statement (query). Every time the user changes any element (except for the data grid itself) the component fires off a call to the buildQuery() method. This method is essentially a general−purpose event handler called by most of the screen components.
Hint The actual event that triggers the buildQuery() method changes based on the component type, but they were all pretty easy to guess. For example, I called buildQuery() on the CheckChanged event of the check boxes, the SelectedIndexChanged event of the list box, and the TextChanged event of the text box. When I had a "build query" button, I had its Clicked event attached to the buildQuery() method as well.
342

Building the query is a little more involved than it might seem, so I broke it into functions. The SELECT part of the query comes primarily by examining the status of the check boxes. The getSelect() method builds the theQuery string based on which check boxes are currently checked. The getWhere() method takes theQuery and adds a WHERE clause based on which element in the list box is selected and what value is currently in the text box. By the time getWhere() is finished running, theQuery has a legal SQL SELECT statement that can be used to update the data set.
private void buildQuery(object sender, System.EventArgs e) {
getSelect();
getWhere(); lblQuery.Text = theQuery; runQuery();
} // end buildQuery
After returning from the getWhere() method, the program copies the value of theQuery to the label.
Displaying the Query
It isn’t really necessary to display the query, but I did so for two reasons. Most importantly, the entire program is centered around building a query, so having the query visible all the time gave me a quick indication if my algorithms were correct. (They weren’t at first, but with enough feedback and tweaking, I was able to fix them). While I was testing the program, I took the call to runQuery() out of the buildQuery() method altogether, and only ran runQuery() when I pressed a button. Most of the time, I could tell just by looking at the query whether it would work or not. Once I got the algorithm right, I added the call to runQuery(), because the user will always want to see the results of the query.
There’s nothing wrong with providing feedback to the user. If the user already knows some SQL, the statement will be familiar. If not, this basic form of SQL is close enough to English to make sense even to non−technical users.
Getting the SELECT Clause from the Check Boxes
The SELECT clause is used to determine which fields should be displayed. Check boxes seem like a good option for this kind of input, because any number of them can be selected.
private void getSelect(){
//create the SELECT part of the query from check boxes
theQuery = "SELECT "; bool none = true;
if (chkAgentID.Checked){ theQuery += "AgentID, "; none = false;
}// end if
if (chkCodeName.Checked){ theQuery += "CodeName, "; none = false;
} // end if
if (chkSpecialty.Checked){ theQuery += "Specialty, "; none = false;
} // end if
343
if (chkAssignment.Checked){ theQuery += "Assignment, "; none = false;
}// end if if (none){
theQuery += "*, ";
}// end if
//remove the last comma
theQuery = theQuery.Substring(0, theQuery.Length −2);
theQuery += " FROM Agents"; } // end getSelect
The method begins by setting the value of theQuery to "SELECT ". All of the queries created by this program begin with that word. I then created a Boolean variable called none and initialized it to true. The none variable is used to indicate that none of the check boxes have been checked.
The method then looks at each check box to see if it is checked. If so, none is false (because at least one of the check boxes has been checked) and the name of the current field is appended to theQuery. If, after all the check boxes have been examined, the value of none is still true, I use the asterisk (which stands for all fields) as a field name.
Notice that all the field names end with a comma. SQL requires the list of fields to be separated by commas. It’s easy to put a comma after every field, but SQL does not want a comma after the last field. The problem is determining which field is last. I used a little string manipulation magic to solve this problem. I added a comma after every field name, and then once the field names were finished, I always removed the last two characters from the string, eliminating the last comma and space. (Sneaky, huh? You’ve got to be sly when you’re working with secret agents all the time.) In this program, all queries come from the Agents table, so I just added "FROM Agents" to the end of the query.
To make sure you understand how this is working, run the Visual Query program for a while and take a careful look at the query as you select and deselect check boxes.
Getting the WHERE Clause from the List Box and Text Box
The WHERE clause of an SQL statement is usually used to compare the name of a field with some possible value. To automate the creation of this part of the SQL statement, I used a list box to select field names and a text box to input matching values.
private void getWhere(){
//Create WHERE clause of query from list box if (lstField.SelectedIndex != 0){
theQuery += " WHERE "; theQuery += lstField.Text;
if (lstField.Text != "AgentID"){ theQuery += " = '";
theQuery += txtMatch.Text; theQuery += "'";
}else {
theQuery += " = "; try {
int temp = Convert.ToInt32(txtMatch.Text); theQuery += Convert.ToString(temp);
}catch (Exception exc) { theQuery += "0";
}// end try
344