
Pro Visual C++-CLI And The .NET 2.0 Platform (2006) [eng]-1
.pdf
198 C H A P T E R 5 ■ T H E . N E T F R A M E W O R K C L A S S L I B R A R Y
Many classes are contained within the System::Data namespace. Depending on your database needs, you may require the use of many of these classes. Most likely, though, you’ll only have to rely on a few. Table 5-4 provides a list of the more common classes that you may encounter. But don’t despair immediately if the specific database access functionality you require isn’t in this table. Chances are that there’s a class within this namespace that does what you need because System::Data is quite thorough.
Table 5-4. Common System::Data Namespace Classes
Class Name |
Description |
Constraint |
A constraint enforced on a data column—for example, a foreign |
|
key constraint or a unique key constraint. |
DataColumn |
A strong typed column in a data table. |
DataRelation |
A relationship between two data tables within the data set. |
DataRelationCollection |
A collection of all the data relations for a data set. |
DataRow |
A row of data in a data table. |
DataSet |
An in-memory cache of all retrieved data from the data provider. |
DataTable |
An in-memory cache of a single data table within the data set. |
DataTableCollection |
A collection of all data tables within the data set. |
DataView |
A customized view of a data table used for sorting, filtering, |
|
searching, editing, and navigation. This view can be bound to |
|
higher-level constructs such as GUI tables and lists. |
|
|
You will look at the System::Data and its five data provider namespaces when you learn about ADO.NET in great detail in Chapter 12.
System::Deployment
The System::Deployment namespace comprises all of the classes needed to programmatically update an application supporting Microsoft’s ClickOnce deployment model. Being a very specialized namespace, it was placed within its own assembly, System.Deployment.dll. To add the namespace, the following code is required at the top of your source:
#using <System.Deployment.dll>
In a nutshell, you use the class ApplicationDeployment to check for the existence of new updates over the Internet or intranet. If they are available, the class downloads and installs them on the client machine, either synchronously or asynchronously and automatically or user controlled.
System::Diagnostics
Executing a program in the CLR environment has its advantages, one of those being readily available diagnostic information. True, it is possible to code your traditional C++ to capture diagnostic information, but with .NET, you get it virtually free with the classes within the System::Diagnostics namespace. The only catch is that because this namespace is not used that frequently, you need to implement the System.dll assembly:
#using <System.dll>

C H A P T E R 5 ■ T H E . N E T F R A M E W O R K C L A S S L I B R A R Y |
199 |
The diagnostic functionality available ranges from simply allowing viewing of event log files and performance counters to allowing direct interaction with system processes. An added bonus is that this namespace provides classes to handle debugging and tracing.
Two main classes handle event logs in the System::Diagnostics namespace. EventLog provides the ability to create, read, write, and delete event logs or event sources across a network. EntryWrittenEventHandler provides asynchronous interaction with event logs. Numerous supporting classes provide more detailed control over the event logs.
It is possible to monitor system performance using the class PerformanceCounter. It is also possible to set up your own custom performance counters using the class PerformanceCounterCategory. You can only write to local counters, but the restriction is eased when it comes to reading counters. Of course, you need to have the right to access the remote machine from where you want to read the counter.
The System::Diagnostics namespace provides an amazing amount of power when it comes to processes. For example, the Process class has the ability to start, monitor, and stop processes on your local machine. In fact, the Process class can also monitor processes on remote machines. Added to this are the ProcessThread and ProcessModule classes, which allow you to monitor the process’s threads and modules. It is also possible to control how a process runs by having control over things such as arguments and environment variables, and input, output, and error streams using the ProcessStartInfo class.
Almost every programmer uses debug and/or trace statements within his code. So common is the practice that the .NET Framework class library includes the Debug and Trace classes to ease your coding life. Syntactically, the Debug and Trace classes are nearly identical. The difference between them lies in the time of compilation and the current development environment being used. Trace statements are executed no matter what the environment (unless you code otherwise), whereas debug statements are only included and executed while within the Debug environment.
Table 5-5 provides you with a quick lookup table of the classes you might find useful within the
System::Diagnostics namespace.
Table 5-5. Common System::Diagnostics Namespace Classes
Class Name |
Description |
Debug |
Methods and properties to help debug a program |
Debugger |
Provides communication to a debugger |
DefaultTraceListener |
The default output method for Trace |
EntryWrittenEventHandler |
Handler to provide asynchronous interaction with event logs |
EventLog |
Provides interaction with event logs |
PerformanceCounter |
Provides access to system performance counters |
PerformanceCounterCategory |
Creates and provides access to custom performance counters |
Process |
Provides access to local and remote processes and the ability to |
|
start and stop local processes |
ProcessModule |
Provides access to process modules |
ProcessStartInfo |
Provides control over the environment for which a |
|
process starts |
ProcessThread |
Provides access to process threads |
Trace |
Provides methods and properties to help trace a program |
|
|
200 C H A P T E R 5 ■ T H E . N E T F R A M E W O R K C L A S S L I B R A R Y
System::DirectoryServices
System::DirectoryServices is a small namespace providing easy access to Active Directory. Not the most commonly used namespace, it has been placed in its own assembly, System.Directoryservices.dll. To add the namespace, you require the following code at the top of your source:
#using <System.Directoryservices.dll>
It is assumed that you have prior knowledge of Active Directory before you use the class; in a nutshell, here is how to use the class. First, you use the class DirectoryEntry constructor to get access to a node or object within Active Directory. Then, with the DirectoryEntry node and some help classes, you are capable of activities such as creating, deleting, renaming, setting passwords, moving a child node, and enumerating children.
You can use the classes in this namespace with any of the Active Directory service providers. The current providers are
•Internet Information Services (IIS)
•Lightweight Directory Access Protocol (LDAP)
•Novell NetWare Directory Service (NDS)
•Windows NT
Another class that you might find of some use in System::DirectoryServices is the DirectorySearcher class. This class allows you to perform a query against an Active Directory hierarchy. Unfortunately, as of now, only LDAP supports DirectorySearcher.
The book Pro .NET Directory Services Programming by Mikael Freidlitz, Ajit Mungale, Erick Sgarbi, Noel Simpson, and Jamie Vachon (Apress, 2003) covers in extreme detail how to use this namespace. Though the book is written for C# and VB .NET developers, I think you can still use it to get the information you need.
System::Drawing
Computer software without some form of graphics is nearly a thing of the past, especially in the PC world. The .NET Framework relies on a technology named GDI+ to handle graphics. GDI+ is easy to use. It is designed to handle the myriad graphic adapters and printers in a device-independent fashion, thus saving you from having to worry about coding for each graphic device on your own. Of course, this is not a new concept; Windows has had a Graphical Device Interface (GDI) since its earliest versions. Those of you from the GDI world should see a considerable simplification of how you now have to code graphics. But you will also find a huge increase in added functionality.
System::Drawing provides the core graphic classes of GDI+, whereas the following four other child namespaces provide more specialized graphics capabilities:
•System::Drawing::Drawing2D: Adds advanced two-dimensional (2D) and vector graphics
•System::Drawing::Imaging: Adds advanced GDI+ imaging
•System::Drawing::Printing: Adds print-related services
•System::Drawing::Text: Adds advanced GDI+ typography
C H A P T E R 5 ■ T H E . N E T F R A M E W O R K C L A S S L I B R A R Y |
201 |
Every System::Drawing namespace requires that you add the System.Draw.dll assembly to the top of your source:
#using <System.Drawing.dll>
I go into GDI+ software development in detail in Chapter 11, but for those of you who can’t wait that long, here’s a brief summary of the functionality.
The core of all GDI+ classes can be found in the System::Drawing namespace. This large namespace contains classes to handle things ranging from a point on the graphics device all the way up to loading and displaying a complete image in many graphic file formats, including BMP, GIF, and JPEG.
The key to all graphics development is the aptly named Graphics class. This class basically encapsulates the graphics device—for example, the display adaptor or printer. With it you can draw a point, line, polygon, or even a complete image. When you use the Graphics class with other System::Drawing classes, such as Brush, Color, Font, and Pen, you have the means to create amazing and creative images on your display device.
Though you can do almost any 2D work you want with System::Drawing, the .NET Framework class library provides you with another set of classes found within the System::Drawing::Drawing2D that allows for more fine-tuned 2D work. The basic principle is similar to the “connect-the-dots” pictures that you did as a kid. The image you want to draw is laid out in 2D space by drawing straight and curved lines from one point to another. Images can be left open or closed. They can also be filled. Filling and line drawing can be done using a brush and/or using a color gradient.
The System::Drawing namespace can handle most imaging functionality. With the System::Drawing::Imaging namespace, you can add new image formats that GDI+ does not support. You can also define a graphic metafile that describes a sequence of graphics operations that can be recorded and then played back.
GDI+ can display (or, more accurately, print) to a printer. To do so is very similar to displaying to a monitor. The difference is that a printer has many different controls that you will not find on a monitor—for example, a paper source or page feed. All these differences were encapsulated and placed into the System::Drawing::Printing namespace.
Nearly all the functionality to handle text is located within the System::Drawing namespace. The only thing left out and placed in the System::Drawing::Text namespace is the ability to allow users to create and use collections of fonts.
System::EnterpriseServices
The System::EnterpriseServices namespace provides the ability for .NET Framework objects to interface with COM+ objects. COM+ objects are a key component of Microsoft’s enterprise-wide application architecture. You can think of COM+ as an extension of COM into the enterprise environment.
With the System::EnterpriseServices namespace, it is fairly easy to use .NET Framework objects within enterprise applications. Table 5-6 shows some of the more common classes and attributes within the System::EnterpriseServices namespace.
Every System::EnterpriseServices namespace requires that you add the System. Enterpriseservices.dll assembly to the top of your source:
#using <System.Enterpriseservices.dll>
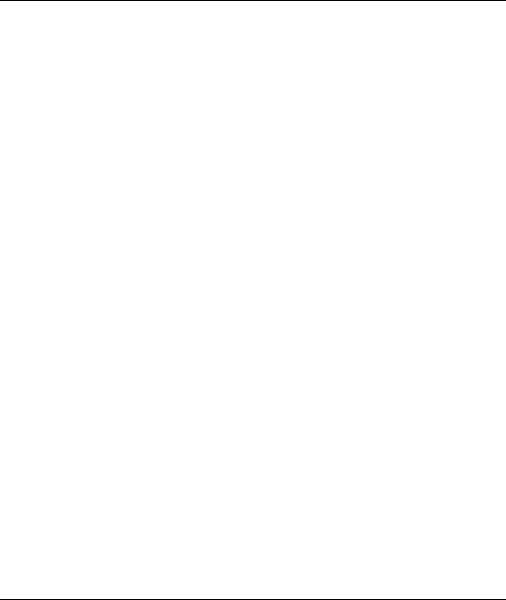
202 C H A P T E R 5 ■ T H E . N E T F R A M E W O R K C L A S S L I B R A R Y
Table 5-6. Common System::EnterpriseServices Namespace Classes and Attributes
Class Name |
Description |
ApplicationActivationAttribute |
An attribute that enables you to specify whether compo- |
|
nents in the assembly run in the creator’s process |
|
(Library) or in a system process (Server) |
ContextUtil |
A class made up several static members from which you |
|
retrieve contextual information about the COM+ object |
EventClassAttribute |
An attribute that specifies that the object is an Event class |
JustInTimeActivationAttribute |
An attribute that turns just-in-time (JIT) activation on |
|
or off |
SecurityIdentity |
A class that contains information regarding the identity |
|
assumed by a COM+ object |
SecurityRoleAttribute |
An attribute that configures a role of an application |
|
or component |
ServicedComponent |
A base class for all classes using COM+ services |
SharedProperty |
A class that provides access to a shared property |
SharedPropertyGroup |
A class that provides access to a collection of |
|
shared properties |
SharedPropertyGroupManager |
A class that controls the access to shared properties |
TransactionAttribute |
An attribute that specifies the type of transaction that is |
|
available to the attributed object (Disabled, NotSupported, |
|
Required, RequiredNew, and Supported) |
|
|
System::Globalization
The System::Globalization namespace contains classes that define culture-related information, such as language, currency, numbers, and calendar. Because globalization is a key aspect of .NET, the namespace was included within the mscorlib.dll assembly.
I cover globalization in Chapter 18, when I go into assembly programming. The CultureInfo class contains information about a specific culture, such as the associated language, the country or region where the culture is located, and even the culture’s calendar. Within the CultureInfo class, you will also find reference to the date, time, and number formats the culture uses. Table 5-7 shows some of the more common classes within the System::Globalization namespace.
Table 5-7. Common System::Globalization Namespace Classes
Class Name |
Description |
Calendar |
Specifies how to divide time into pieces (e.g., weeks, months, |
|
and years) |
CultureInfo |
Contains specific information about a culture |
DateTimeFormatInfo |
Specifies how dates and times are formatted |
NumberFormatInfo |
Specifies how numbers are formatted |
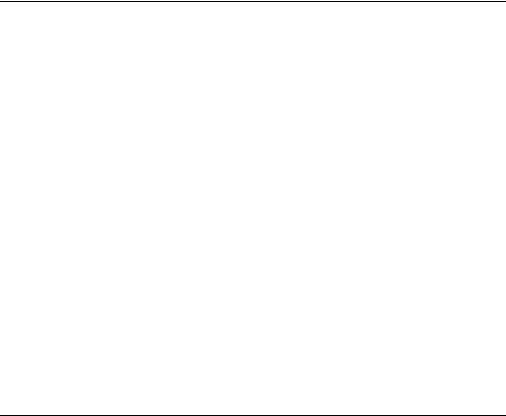
C H A P T E R 5 ■ T H E . N E T F R A M E W O R K C L A S S L I B R A R Y |
203 |
Table 5-7. Common System::Globalization Namespace Classes
Class Name |
Description |
RegionInfo |
Contains information about the country and region |
SortKey |
Maps a string to its sort key |
TextInfo |
Specifies the properties and behaviors of the writing system |
|
|
System::IO
If you are not using sockets or a database to retrieve and store data, then you are most likely using file and/or stream input and output (I/O). Of course, it is completely possible that you are using sockets, a database, and file and stream I/O within the same application. As you can guess by the name System::IO, it handles the .NET Framework library class’s file and stream I/O. To access System::IO, you need to reference the mscorlib.dll assembly:
#using <mscorlib.dll>
Typically when you deal with the System::IO namespace’s classes, you are working with files and directories on your local machine and network, or streams of data probably via the Internet. These, however, are not the only uses of the classes found within the System::IO namespace. For example, it is possible to read data from and write data to computer memory, usually either a string buffer or a specific memory location.
You learn about the .NET Framework class library’s I/O capabilities in some detail in Chapter 8. For now, Table 5-8 shows some of the more common classes that you might come across in the
System::IO namespace.
Table 5-8. Common System::IO Namespace Classes
Class Name |
Description |
BinaryReader |
Reads in .NET primitive types from a binary stream. |
BinaryWriter |
Writes out .NET primitive types to a binary stream. |
Directory |
A collection of static methods for creating, moving, and enumerating |
|
directories. |
DirectoryInfo |
A collection of instance methods for creating, moving, and |
|
enumerating directories. |
File |
A collection of static methods for creating, copying, deleting, moving, |
|
and opening files. It also can be used in the creation of a FileStream. |
FileInfo |
A collection of instance methods for creating, copying, deleting, |
|
moving, and opening files. It also can be used in the creation of a |
|
FileStream. |
FileNotFoundException |
An exception that is thrown when a file on a disk is not found. |
FileStream |
Provides support for both synchronous and asynchronous read |
|
and write operations to a stream. |
FileSystemWatcher |
Monitors and then raises events for file system changes. |
IOException |
An exception that is thrown when an I/O exception occurs. |

204 C H A P T E R 5 ■ T H E . N E T F R A M E W O R K C L A S S L I B R A R Y
Table 5-8. Common System::IO Namespace Classes (Continued)
Class Name |
Description |
MemoryStream |
Provides support for reading and writing a stream of bytes |
|
to memory. |
Path |
Provides support for operations on a String that contains a file |
|
or directory. |
StreamReader |
Reads a UTF-8 encoded byte stream from a TextReader. |
StreamWriter |
Writes a UTF-8 encoded byte stream to a TextWriter. |
StringReader |
Reads a String using a TextReader. |
StringWriter |
Writes a String using a TextWriter. |
TextReader |
An abstract reader class that can represent a sequence |
|
of characters. |
TextWriter |
An abstract writer class that can represent a sequence of characters. |
|
|
System::IO::Ports
The System::IO::Ports namespace provides the developer complete access to the computer’s serial port. System::IO::Ports is primarily made up of the one class SerialPort, which presents assorted functionality like synchronous and event-driven I/O, and access to serial driver properties. The SerialPort class also provides a method to allow stream access to the serial ports of the computer.
To access System::IO::Ports, you need to reference the System.dll assembly near the top of your code:
#using <System.dll>
This was not added until .NET Framework version 2.0, which is a surprising oversight by Microsoft.
System::Management
The System::Management namespace provides access to a large amount of information about the system, devices, and applications maintained within the Windows Management Instrumentation (WMI) infrastructure. The System::Management allows you to query for information like the free space remaining on the disk, CPU utilization, shared device names, and so forth.
You will predominately use classes derived from ManagementObjectSearcher, ManagementQuery, and ManagementEventWatcher to get information for both managed and unmanaged components maintained within the WMI infrastructure.
Though the System::Management namespace appears to be simple, it is actually deceptively tricky to use. In fact, .NET System Management Services by Alexander Golomshtok (Apress, 2003) covers how to use this namespace in great detail, to help you over the learning curve. Though the book is written for C# developers, I think you can still use it to get the information you need.
To access System::Management, you need to reference the System.Management.dll assembly near the top of your code:
#using <System.Management.dll>

C H A P T E R 5 ■ T H E . N E T F R A M E W O R K C L A S S L I B R A R Y |
205 |
Table 5-9 shows some of the more common classes that you might come across in the
System::Management namespace.
Table 5-9. Common System::Management Namespace Classes
Class Name |
Description |
EventQuery |
Represents a WMI event query. Instances of this |
|
class or derived classes from it are used in |
|
ManagementEventWatcher to subscribe to WMI events |
ManagementClass |
Represents a management class |
ManagementEventWatcher |
Used to temporarily subscribe to event notifications |
|
based on a specified EventQuery |
ManagementNamedValueCollection |
Represents a collection of key/value pairs containing |
|
contextual information to WMI operations |
ManagementObject |
Represents a data management class |
ManagementObjectCollection |
Represents a collection data management class retrieved |
|
from WMI |
ManagementObjectSearcher |
This collection retrieves a ManagementObjectCollection |
|
based on a specific query |
ManagementPath |
Used to build and parse paths to a specific WMI object |
ManagementQuery |
An abstract class used to build all management |
|
query objects |
PropertyData |
Represents a specific WMI object property |
PropertyDataCollection |
Represents a collection of properties about a WMI object |
QualifierData |
Represents a specific WMI object qualifier |
QualifierDataCollection |
Represents a collection of qualifiers about a WMI object |
|
|
System::Net
This namespace will be hidden from most Web developers using .NET, because they will most likely use ASP.NET’s higher-level extraction of Internet communication. For those of you who are more familiar with the networks, the .NET Framework class library has provided several namespaces.
To access both the System::Net hierarchy of namespaces, you need to reference the System.dll assembly near the top of your code:
#using <system.dll>
The System::Net namespace provides a simple programming interface for many of today’s network protocols. It enables you to do things such as manage cookies, make DSN lookups, and communicate with HTTP and FTP servers. If that is not intimate enough for you, then the System::Net::Sockets namespace provides you with the ability to program at the sockets level. I cover network programming in detail in Chapter 17.

206 C H A P T E R 5 ■ T H E . N E T F R A M E W O R K C L A S S L I B R A R Y
For those of you who want to program your network at this lower level, Table 5-10 provides a list of all the namespaces that make up the System::Net hierarchy.
Table 5-10. System::Net Hierarchy Namespaces
Namespace |
Description |
System::Net |
A simple programming interface for many of the |
|
protocols used on networks today |
System::Net::Cache |
A set of types used to define cache policies for resources |
|
obtained using the WebRequest and HttpWebRequest |
System::Net::Configuration |
A set of types used to access and update configuration |
|
settings for the System::Net namespace hierarchy |
System::Net::Mail |
A simple programming interface for sending electronic |
|
mail to a Simple Mail Transfer Protocol (SMTP) server |
|
for delivery |
System::Net::Mime |
A set of types used to define Multipurpose Internet |
|
Mail Exchange (MIME) headers |
System::Net::NetworkInformation |
A simple programming interface for retrieving infor- |
|
mation about your network like network traffic data, |
|
statistics, and address change information |
System::Net::Security |
A simple programming interface for secured data |
|
transfer |
System::Net::Sockets |
A simple programming interface for Windows Sockets |
|
(Winsock) |
|
|
System::Reflection
Most of the time when you develop code, it will involve static loading of assemblies and the data types found within. You will know that, to execute properly, application X requires class Y’s method Z. This is pretty standard and most programmers do it without thinking.
This is the normal way of developing with the .NET Framework class library as well. There are times, though, that a developer may not know which class, method, or other data type is needed for successful execution until the time that the application is running. What is needed is dynamic instance creation of data types. With the .NET Framework class library, this is handled by the classes within the System::Reflection namespace found within the mscorlib.dll assembly:
#using <mscorlib.dll>
The System::Reflection namespace provides a class that encapsulates assemblies, modules, and types. With this encapsulation, you can now examine and load classes, structures, methods, and so forth. You can also create dynamically an instance of a type and then invoke one of its methods, or access its properties or member variables.

C H A P T E R 5 ■ T H E . N E T F R A M E W O R K C L A S S L I B R A R Y |
207 |
I explore System::Reflection in more detail when you examine assembly programming in Chapter 18. Table 5-11 shows some of the more common classes that you might use within the
System::Reflection namespace.
Table 5-11. Common System::Reflection Namespace Classes
Class Name |
Description |
Assembly |
Defines an assembly |
AssemblyName |
Provides access to all the parts of an assembly’s name |
AssemblyNameProxy |
A remotable version of AssemblyName |
Binder |
Selects a method, property, and so forth, and converts its actual |
|
argument list to a generic formal argument list |
ConstructorInfo |
Provides access to the constructor’s attributes and metadata |
EventInfo |
Provides access to the event’s attributes and metadata |
FieldInfo |
Provides access to the field’s attributes and metadata |
MemberInfo |
Provides access to the member’s attributes and metadata |
MethodInfo |
Provides access to the method’s attributes and metadata |
Module |
Defines a module |
ParameterInfo |
Provides access to the parameter’s attributes and metadata |
Pointer |
Provides a wrapper class for a pointer |
PropertyInfo |
Provides access to the property’s attributes and metadata |
TypeDelegator |
Provides a wrapper for an object and then delegates all methods to |
|
that object |
|
|
System::Resources
The .NET Framework can handle resources in several different ways: in an assembly, in a satellite assembly, or as external resource files and streams. The handling of resources within the .NET Framework class library for any of these three ways lies in the classes of the System::Resources namespace. Handling resources is a very common task, so it was placed within the mscorlib.dll assembly:
#using <mscorlib.dll>
Resources can be fixed for an application divided by culture. I examine resources programming with assembly programming in Chapter 18. You will be dealing mostly with three classes within the System::Resources namespace, as shown in Table 5-12.