
ASP.NET 2.0 Everyday Apps For Dummies (2006)
.pdf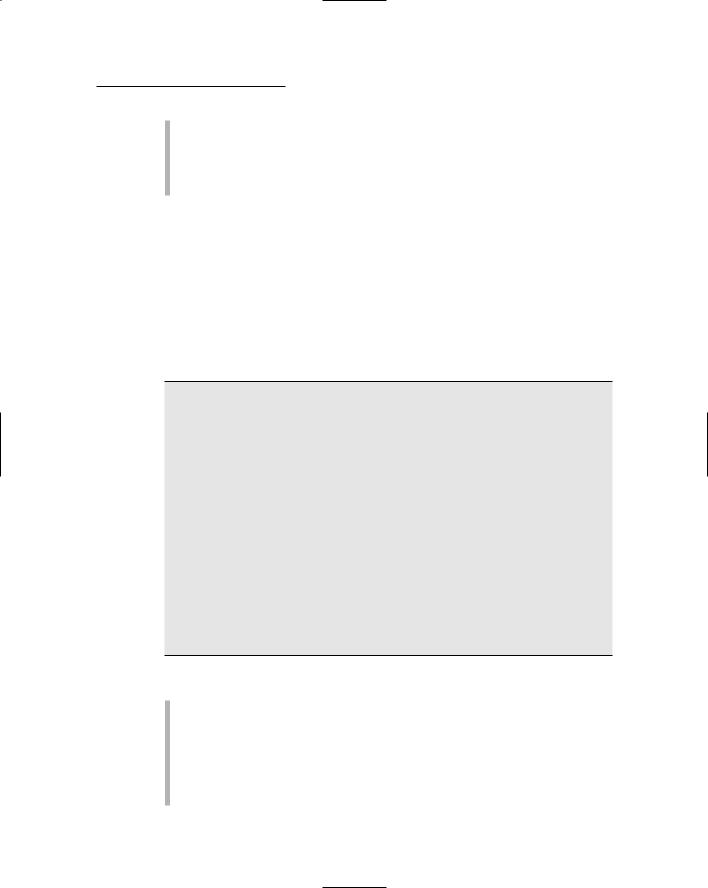
Chapter 9: Building a Content Management System 307
6 This SQL data source is bound to the Repeater control. Its SELECT statement retrieves the deptid and name columns from the Departments table.
7 The second ContentPlaceHolder control provides the main display area for the content of each page.
Building the Login Page
The Login page, shown back in Figure 9-2, is automatically displayed when the user tries to access any other page of the Content Management System without first logging in. Thus, the user must log in before accessing the application. The .aspx code for the Login page is shown in Listing 9-3.
Listing 9-3: The Login page (Login.aspx)
<%@ Page Language=”C#” |
1 |
MasterPageFile=”~/MasterPage.master” |
|
AutoEventWireup=”true” |
|
CodeFile=”Login.aspx.cs” |
|
Inherits=”Login” |
|
Title=”Company Intranet” %> |
|
<asp:Content ID=”Content1” |
2 |
ContentPlaceHolderID=”ContentPlaceHolder1” |
|
Runat=”Server”> |
|
<h1> |
|
Welcome to the Company Intranet |
|
</h1> |
|
</asp:Content> |
|
<asp:Content ID=”Content2” Runat=”Server” |
3 |
ContentPlaceHolderID=”ContentPlaceHolder2” > |
|
<asp:Login ID=”Login1” runat=”Server” |
4 |
DestinationPageUrl=”~/Default.aspx” |
|
TitleText=”Please enter your account information: <br /><br />” />
</asp:Content>
Here’s a more detailed rundown on the numbered parts of this listing:
1 The Page directive indicates that MasterPage.master is used as the master file. If you’re using Visual Basic instead of C#, this directive will indicate VB instead of C# as the language and
AutoEventWireup will be set to false.
2 The first <Content> element defines the content displayed at the top of the page. In this case, the simple heading “Welcome to the Company Intranet” is displayed.
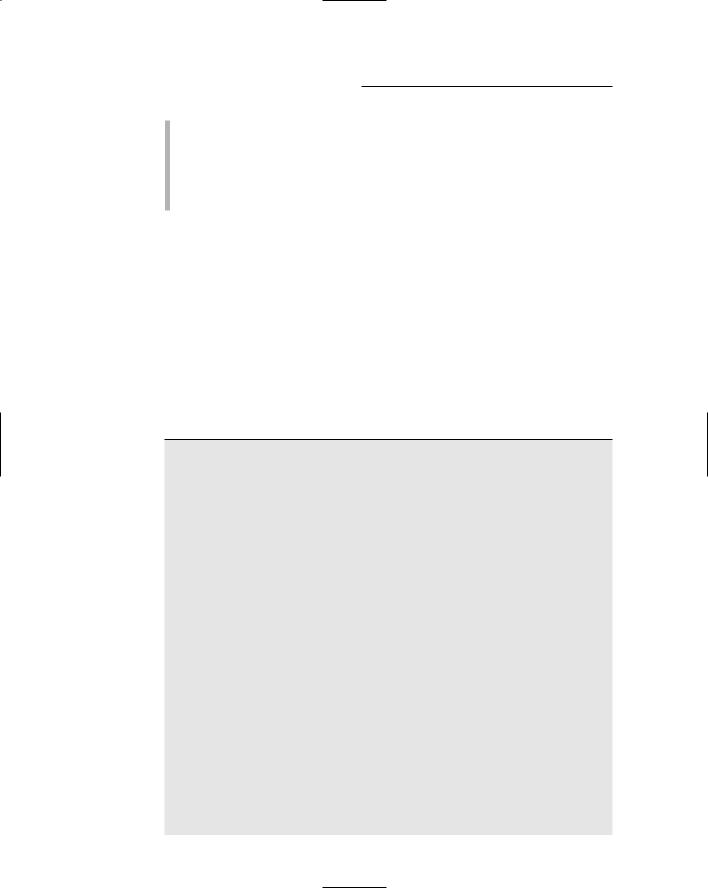
308 Part V: Building Community Applications
3 The second <Content> element provides the content displayed in the main portion of the page. For the Login page, the only item here is the Login control, described in the next paragraph.
4 The Login control displays the labels, text boxes, and buttons necessary to let the user log in. For more information about using the Login control, refer to Chapter 4.
Building the Home Page
The Home page (Default.aspx) displays a greeting and a list of the departments. The department list is a little redundant, since the sidebar in the Master Page also displays a list of the departments. However, the list displayed in the main area of the Home page includes the department descriptions in addition to the names. Listing 9-4 shows the .aspx code for this page. A code-behind file isn’t required. Refer to Figure 9-3 for a refresher of what this page looks like.
Listing 9-4: The Home page (Default.aspx)
<%@ Page Language=”C#” |
1 |
MasterPageFile=”~/MasterPage.master” |
|
AutoEventWireup=”true” |
|
CodeFile=”Default.aspx.cs” |
|
Inherits=”_Default” |
|
Title=”Company Intranet” %> |
|
<asp:Content ID=”Content1” |
2 |
ContentPlaceHolderID=”ContentPlaceHolder1” |
|
Runat=”Server”> |
|
<h1> |
|
Welcome to the Company Intranet |
|
</h1> |
|
</asp:Content> |
|
<asp:Content ID=”Content2” |
3 |
ContentPlaceHolderID=”ContentPlaceHolder2” |
|
Runat=”Server”> |
|
Welcome to our fabulous new company Intranet, where<br /> each department of our growing company can customize<br /> its own page.<br /><br />
Which department would you like to visit?<br /><br /><br
/> |
|
<asp:Repeater ID=”Repeater1” runat=”Server” |
4 |
DataSourceID=”SqlDataSource1” > |
|
<ItemTemplate> |
|
<asp:LinkButton ID=”LinkButton1” |
5 |
runat=”server” |
|
Text=’<% #Eval(“name”) %>’ |
|
PostBackUrl=’<% #Eval(“deptid”, |
|
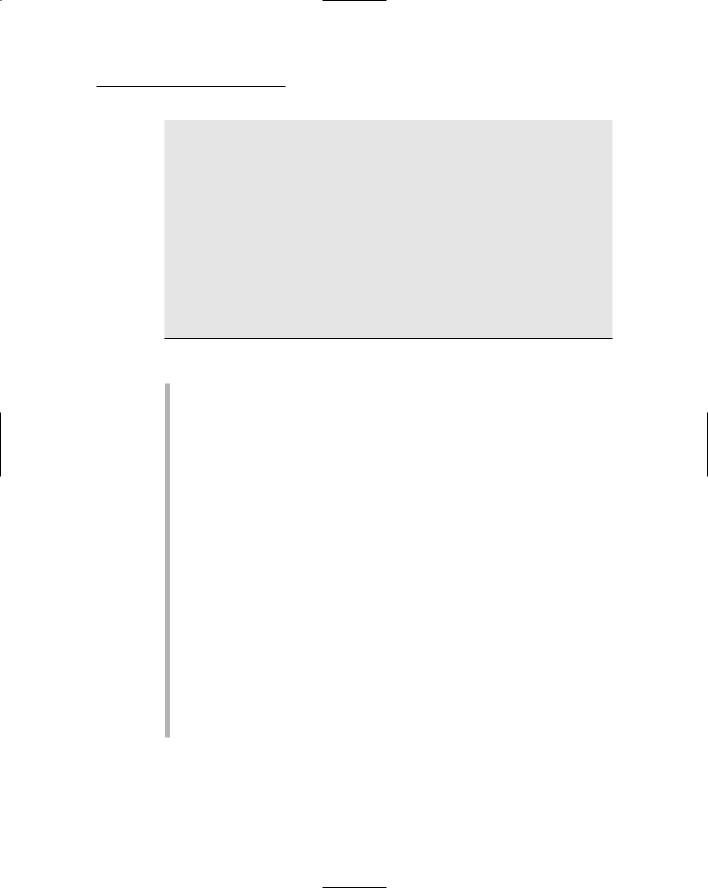

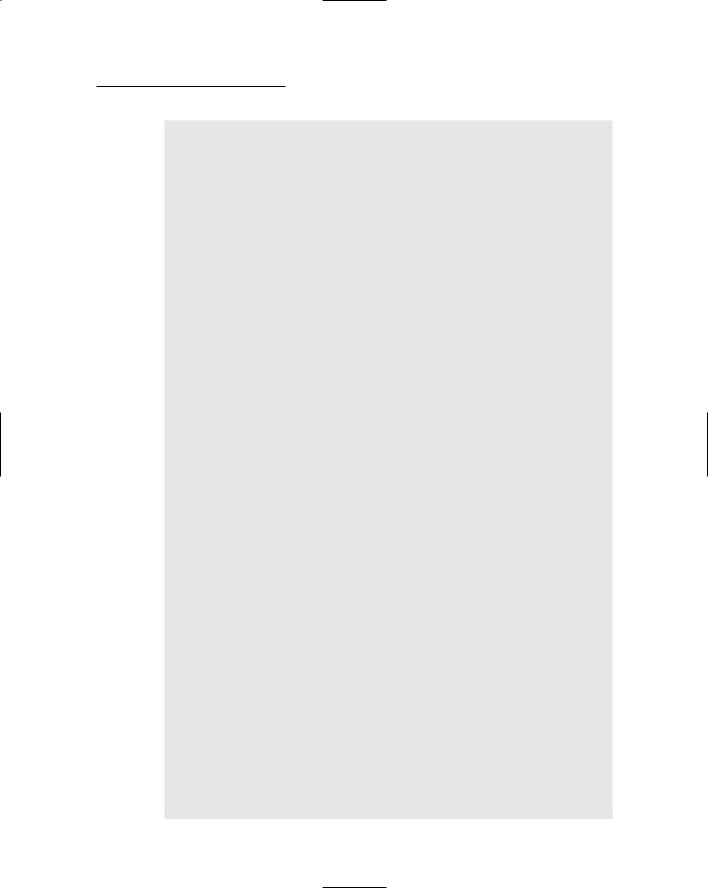
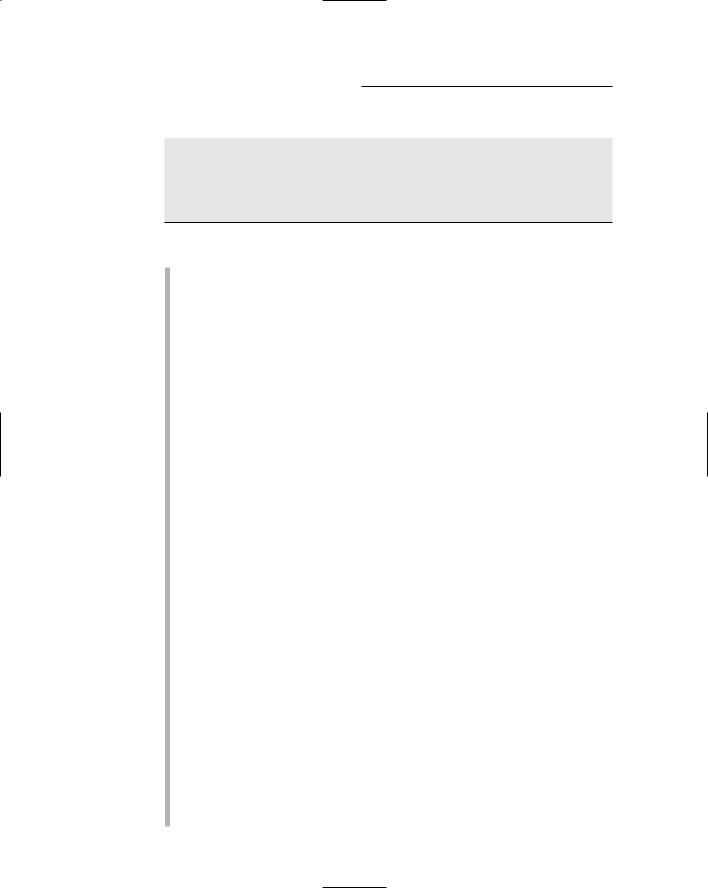
312 Part V: Building Community Applications
Listing 9-5 (continued)
SelectCommand=”SELECT [typeid], [name]
FROM [ContentTypes]
ORDER BY [name]”>
</asp:SqlDataSource>
</asp:Content>
And now, here comes the play-by-play commentary for this listing:
1 The Page directive does its normal job of identifying the Master Page and other details. Some of these details are dependent on the language being used, so you’ll need to change them if you’re working with VB instead of C#.
2 The first <Content> element defines the information that will be displayed at the top of the page. This <Content> element includes a FormView control and a DataView control so it can retrieve the department name from the database and display it in the heading area.
3 This FormView control is bound to a specific SQL data source named SqlDataSource1. It might seem a little strange to use a FormView control to display just one field, but the FormView control is needed to provide a binding context for the Eval method described in Line 4.
4 This Label control displays the name field retrieved by the data source. Notice that this label is sandwiched between <h1> and </h1> tags, so the department name is formatted as a level-1 heading.
5 The first SQL data source for this form retrieves the department information from the Departments table. The WHERE clause in the SELECT statement uses a parameter named deptid to indicate which department to retrieve.
6 The deptid parameter is defined by this <QueryParameter> element, which specifies that the parameter’s value is taken from the query string field named dept. As a result, this data source retrieves the department row indicated by the dept query string field.
7 The second <Content> element provides the main content for the page: the department description and a list of links to the available content types.
8 A FormView control (similar to the one defined in Line 3) displays the name and description fields retrieved by the
SqlDataSource2 data source.
9 This label displays the name field from the data source.
10 Then, another label displays the description field.
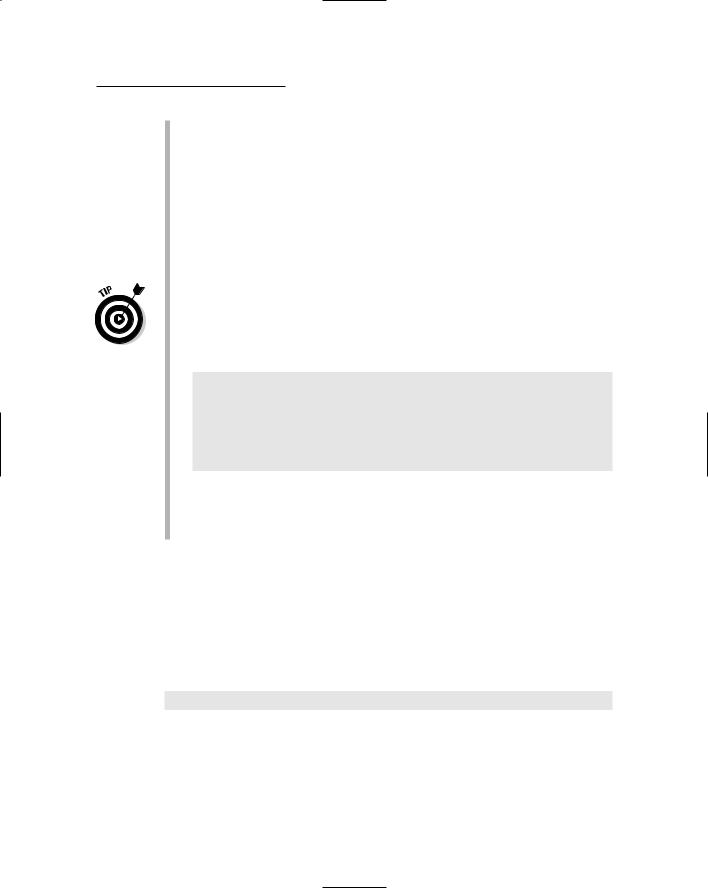
Chapter 9: Building a Content Management System 313
11 A Repeater control displays a list of links for the content types. The Repeater control is bound to a third data source,
SqlDataSource3.
12 The LinkButton control includes a complicated expression that builds the PostBack URL with two query string fields, one named type, the other named dept. For example, if the user has chosen the faq type for the hr department, the PostBack URL will
be List.aspx?type=faq&dept=hr. Notice that Request. QueryString is used to retrieve the value for the dept query string field.
If you’re working in Visual Basic, you’ll need to make two changes to this expression. First, Visual Basic requires continuation characters to break the expression across lines. And second, you’ll need to replace the brackets on the QueryString parameter with parentheses. Thus, the Visual Basic version of this <LinkButton> element should look like this:
<asp:LinkButton ID=”linkContent” runat=”server”
Text=”<% #Eval(“name”) %>’ PostBackUrl=’<% #Eval(“typeid”, _
“List.aspx?type={0}”) _
+“&dept=” _
+Request.QueryString(“dept”) %>’ />
13 This data source retrieves the name and description fields displayed by the FormView control that was defined in Line 8.
14 This data source retrieves all rows from the Types table so they can be displayed by the Repeater control defined in Line 11.
Building the Content List Page
The Content List page, which was pictured back in Figure 9-5, displays a list of the content items for the selected department. Two query string fields are passed to this page — one for the department ID and the other for the type ID. For example, a typical request string for this page would look like this:
List.aspx?type=faq&dept=hr
Here, the FAQ items for the Human Resources department are being requested.
Unlike the other pages of this application presented so far, this page requires a code-behind file. The following sections present the List.aspx file and both the C# and Visual Basic versions of the code-behind file.
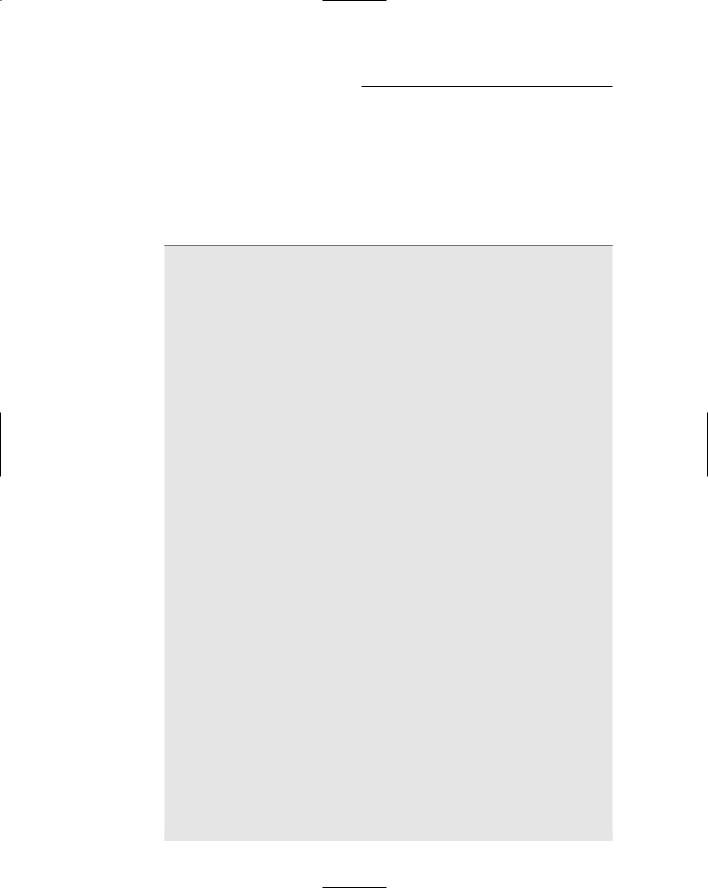
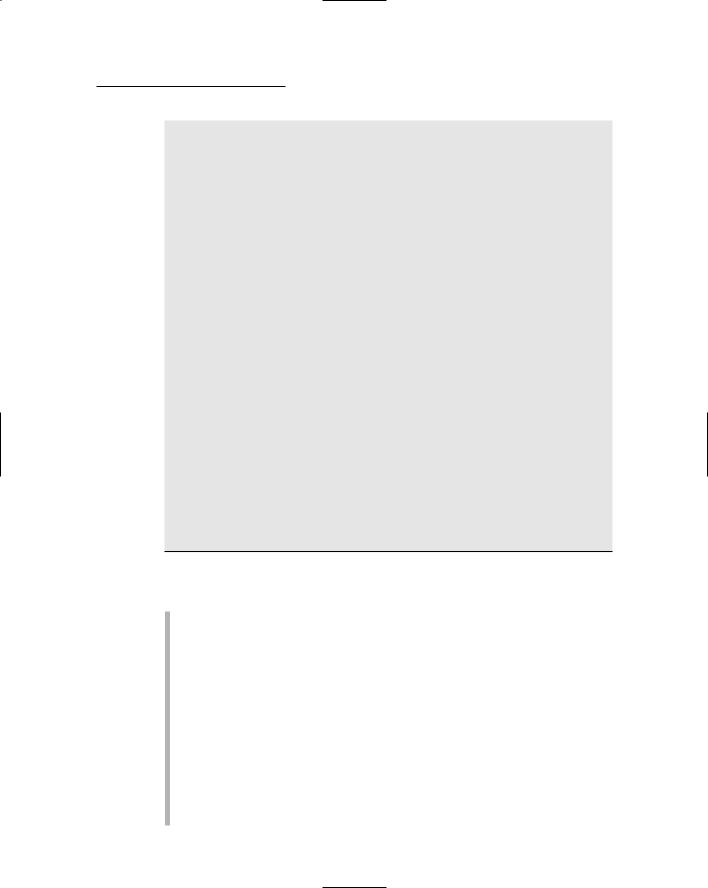
Chapter 9: Building a Content Management System 315
PostBackUrl=’<%# Eval(“contentid”, “Detail.aspx?item={0}”) +
“&dept=” + Request.QueryString[“dept”] + “&type=” + Request.QueryString[“type”] %>’
/> |
|
<br /> |
|
</ItemTemplate> |
|
</asp:Repeater> |
|
<asp:SqlDataSource ID=”SqlDataSource2” |
10 |
runat=”server” |
|
ConnectionString=”<%$ ConnectionStrings: |
|
ConnectionString %>” |
|
SelectCommand=”SELECT [contentid], [title] |
|
FROM [ContentItems] |
|
WHERE [deptid] = @deptid |
|
AND [typeid] = @typeid”> |
|
<SelectParameters> |
|
<asp:QueryStringParameter |
11 |
Name=”deptid” |
|
QueryStringField=”dept” |
|
Type=”String” /> |
|
<asp:QueryStringParameter |
12 |
Name=”typeid” |
|
QueryStringField=”type” |
|
Type=”String” /> |
|
</SelectParameters> |
|
</asp:SqlDataSource> |
|
<br /> |
|
<asp:LinkButton ID=”btnAdd” runat=”server” |
13 |
Text=”Add” |
|
OnClick=”btnAdd_Click” /> </asp:Content>
For your reading pleasure, the following paragraphs summarize the key points that are necessary to understand this page:
1 As usual, you’ll need to modify the Page directive if you want to use the VB version of the code-behind file. In particular, you’ll need to change the Language attribute to VB, the AutoEventWireup attribute to false, and the CodeFile attribute to List.aspx.vb.
2 The first <Content> element displays the department name at the top of the page.
3 This FormView control displays the department name. It’s bound to a SQL data source named SqlDataSource1.
4 This is the Label control that displays the name field, formatted by the <h1> and </h1> tags.
5 The SqlDataSource1 data source retrieves the department name. The deptid parameter indicates which department should be retrieved.
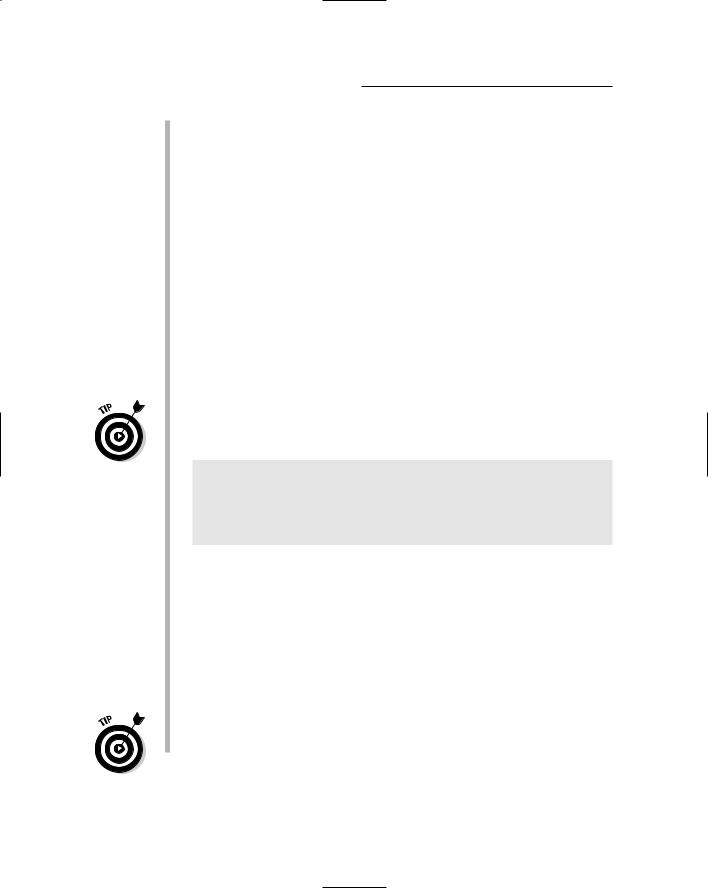
316 Part V: Building Community Applications
6 The <QueryParameter> element defines the deptid parameter. Its value is taken from the dept query string.
7 The second <Content> element provides the main content for the page.
8 A Repeater control is used to display the content types. This control is bound to SqlDataSource2, which is defined in Line 10.
9 The LinkButton control in the Repeater’s item template displays the links to the content types. As you can see, it uses a simple Eval expression to bind the Text attribute to the title field. However, a more complicated expression specifies the PostBack URL. This URL includes three query strings, named item, dept, and type. The item query string’s value comes from
the contented field of the data source; the other two query strings are simply carried forward from the request query string. If (for example) the user selects the content item whose ID is 2 while viewing the FAQ items for the Human Resources department, then the
PostBack URL is Detail.aspx?item=2&dept=hr&type=faq.
If you’re working in Visual Basic, you’ll need to modify this expression so it uses VB continuation characters and parentheses instead of brackets. In that case, the entire <LinkButton> element looks like this:
<asp:LinkButton ID=”Link1” runat=”server” 9 Text=’<%# Eval(“title”) %>’ PostBackUrl=’<%# Eval(“contentid”, _
“Detail.aspx?item={0}”) + _
“&dept=” + Request.QueryString(“dept”) + _ “&type=” + Request.QueryString(“type”) %>’ />
10 The SqlDataSource2 data source retrieves the list of content items for the selected department and type. As you can see, the SELECT statement requires two parameters, deptid and typeid.
11 The deptid parameter is defined with a <QueryString> element, taking its value from the dept query string.
12 The deptid parameter is defined with a <QueryString> element. It takes its value from the dept query string.
13 This LinkButton control displays the Add link, which lets the user add a new content item. Note that the code-behind file hides
this link if the user is not an administrator for the department.
If you’re working in Visual Basic, you should remove the <OnClick> element.