
ASP.NET 2.0 Everyday Apps For Dummies (2006)
.pdf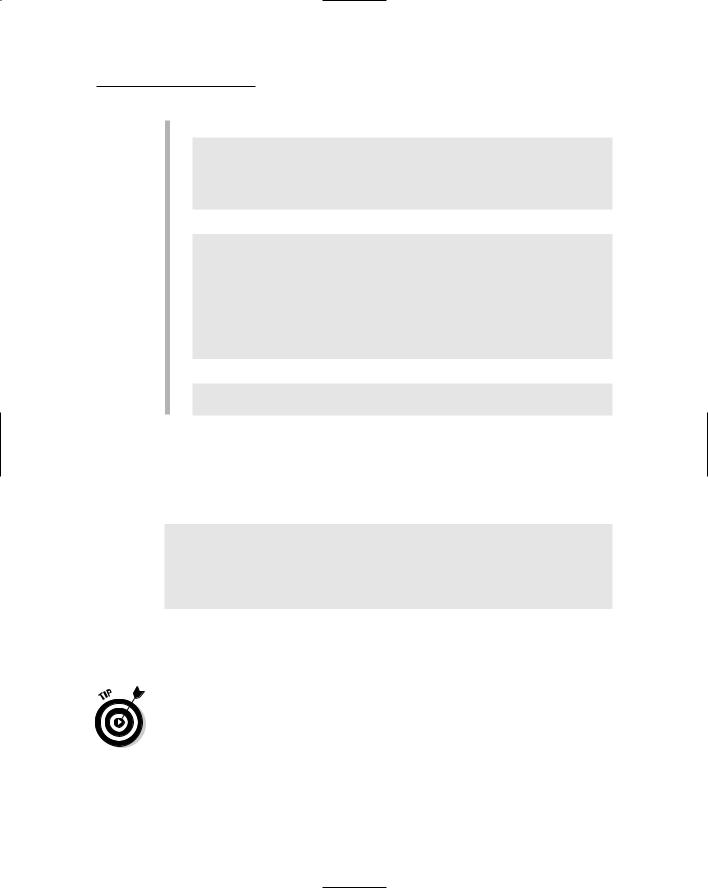
Chapter 7: Building a Product Maintenance Application 237
This INSERT statement inserts a new product:
INSERT INTO [Products]
([productid], [catid], [name], [shorttext], [longtext], [price], [thumbnail], [image])
VALUES (@productid, @catid, @name, @shorttext, @longtext, @price, @thumbnail, @image)
This UPDATE statement updates a product row:
UPDATE [Products]
SET [catid] = @catid, [name] = @name,
[shorttext] = @shorttext, [longtext] = @longtext, [price] = @price, [thumbnail] = @thumbnail, [image] = @image
WHERE [productid] = @original_productid
This DELETE statement deletes a row from the Products table:
DELETE FROM [Products]
WHERE [productid] = @original_productid
Connecting to the database
The Maint database uses a connection string that’s stored in the application’s Web.config file, like this:
<connectionStrings>
<add name=”ConnectionString” connectionString=”Data
Source=localhost\SQLExpress;
Initial Catalog=Maint;Integrated Security=True”/> </connectionStrings>
Because the connection string is the only place that specifies the database and server name, you can move the database to a different server — or use a different database — by changing the connection string specified in the
Web.config file.
If you want to use the database for the Chapter 6 Shopping Cart application rather than the separate Maint database, just change the Initial Catalog setting from Maint to Cart.
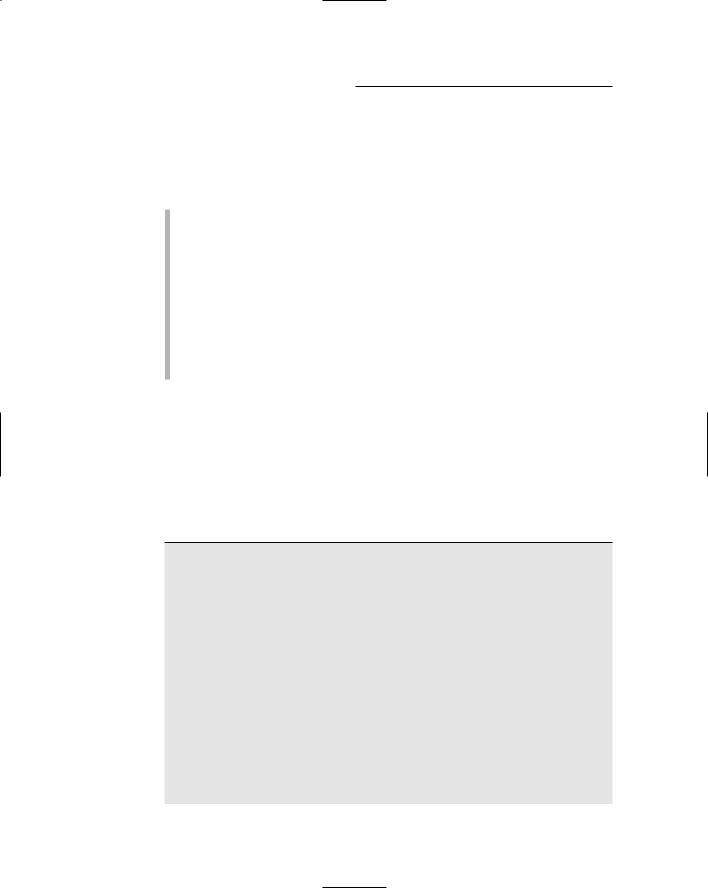
238 Part IV: Building Back-End Applications
The Application’s Folders
Like most ASP.NET applications, the Product Catalog application includes several folders. In particular:
(Root): The application’s root folder contains the application’s three pages (Default.aspx, Product.aspx, and Cart.aspx) as well as the Master Page (Default.master).
App_Data: This folder is created by default by Visual Studio when the application is created. However, the actual database (managed by SQL Server) is stored in a folder that’s not part of the application’s folder hierarchy.
Images: This folder contains the banner graphic that’s displayed by the Master Page. The images referred to in the Thumbnail and Image columns of the Products table aren’t used by this application, so they aren’t included in the Images folder.
Building the Master Page
Listing 7-2 shows the Master Page (MasterPage.master). As with the Master Pages shown in previous chapters, this one simply displays a banner at the top of each page.
Listing 7-2: The Master page (MasterPage.master)
<%@ Master Language=”C#” %> |
1 |
<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.1//EN” “http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd”>
<script runat=”server”>
</script>
<html xmlns=”http://www.w3.org/1999/xhtml” > <head runat=”server”>
<title>Acme Pirate Supply</title> </head>
<body>
<form id=”form1” runat=”server”> <div>
<asp:Image ID=”Image1” runat=”server” 2 ImageUrl=”~/Images/Banner.jpg”/>
<br />
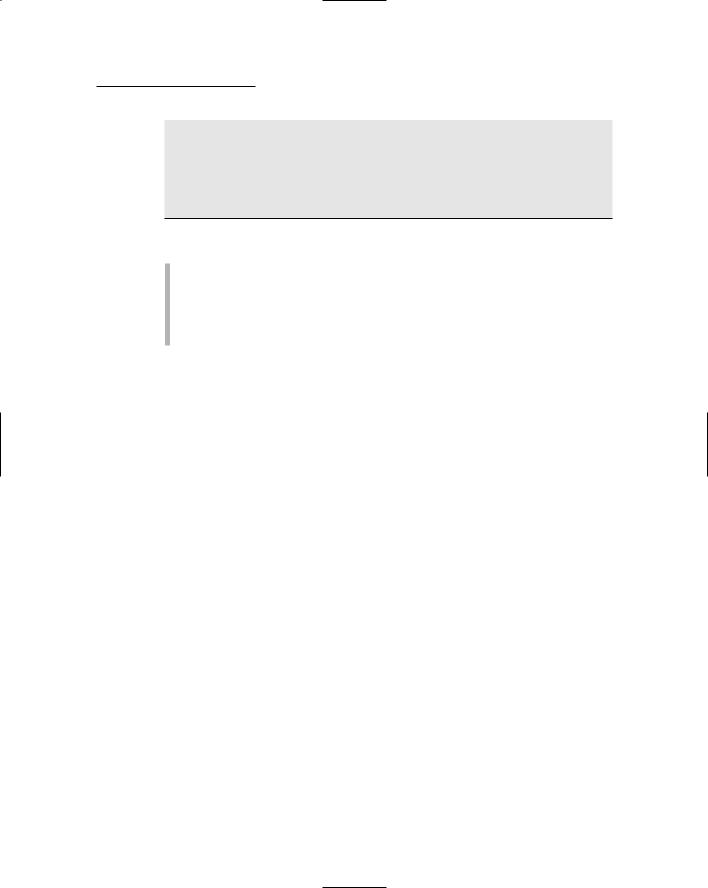
Chapter 7: Building a Product Maintenance Application 239
<asp:contentplaceholder runat=”server” |
3 |
id=”ContentPlaceHolder1” > </asp:contentplaceholder>
</div>
</form>
</body>
</html>
Here are the key points of this listing:
1 The Master directive indicates that the file is a Master Page.
2 The Image control displays the banner image that appears at the top of each page.
3 The ContentPlaceHolder control indicates where the content for each page that uses this master will appear.
Building the Menu Page
The Menu page (Default.aspx) is the default page for the Product Catalog application. It simply displays a pair of links that let the user go to the Category Maintenance page or the Product Maintenance page. Listing 7-3 shows the code for this page.
Listing 7-3: The Menu page (Default.aspx)
<%@ Page Language=”C#” |
1 |
MasterPageFile=”~/MasterPage.master” |
|
AutoEventWireup=”true” |
|
CodeFile=”Default.aspx.cs” |
|
Inherits=”_Default” |
|
Title=”Acme Pirate Supply” %> |
|
<asp:Content ID=”Content1” Runat=”Server” |
2 |
ContentPlaceHolderID=”ContentPlaceHolder1” > |
|
<br /> |
|
Welcome to the Maintenance Application.<br /> |
|
<br /> |
|
<asp:LinkButton ID=”LinkButton1” runat=”server” |
3 |
PostBackUrl=”CatMaint.aspx”> |
|
Maintain Categories |
|
</asp:LinkButton> |
|
<br /><br /> |
|
<asp:LinkButton ID=”LinkButton2” runat=”server” |
4 |
PostBackUrl=”ProdMaint.aspx”> |
|
Maintain Products |
|
</asp:LinkButton> |
|
</asp:Content> |
|
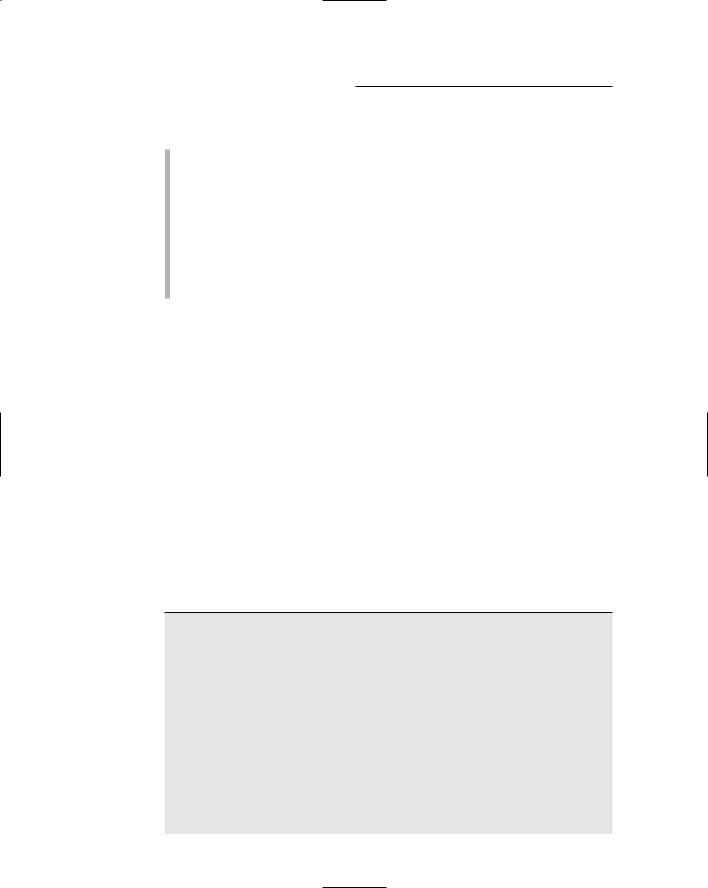
240 Part IV: Building Back-End Applications
Here are some of the key points to note about the code for this page:
1 The Page directive uses the MasterPageFile attribute to specify the name of the Master Page.
2 The <Content> element provides the content that’s displayed for the page.
3 The first <LinkButton> element provides a link to the CatMaint. aspx page. The PostBackUrl attribute is used to post to that page when the user clicks the link.
4 The second <LinkButton> element provides a link that posts back to the ProdMaint.aspx page.
Building the Category Maintenance Page
The Category Maintenance page (CatMaint.aspx) lets the user update or delete existing categories or create new categories. It uses a GridView control to let the user modify or delete existing categories. In addition, it uses three text boxes that let the user enter data to define a new category. The text boxes are required because the GridView control doesn’t provide a way for users to insert rows.
The CatMaint.aspx file
Listing 7-4 shows the .aspx code for the Category Maintenance page. You may want to refer back to Figure 7-3 to see how this page looks on-screen.
Listing 7-4: The Category Maintenance page (CatMaint.aspx)
<%@ Page Language=”C#” |
1 |
MasterPageFile=”~/MasterPage.master” |
|
AutoEventWireup=”true” |
|
CodeFile=”CatMaint.aspx.cs” |
|
Inherits=”CatMaint” |
|
Title=”Acme Pirate Supply” %> |
|
<asp:Content ID=”Content1” Runat=”Server” |
2 |
ContentPlaceHolderID=”ContentPlaceHolder1” > |
|
Category Maintenance<br /> |
|
<br /> |
|
<asp:GridView ID=”GridView1” runat=”server” |
3 |
AutoGenerateColumns=”False” |
|
DataKeyNames=”catid” |
|
DataSourceID=”SqlDataSource1”
OnRowDeleted=”GridView1_RowDeleted”
OnRowUpdated=”GridView1_RowUpdated”>
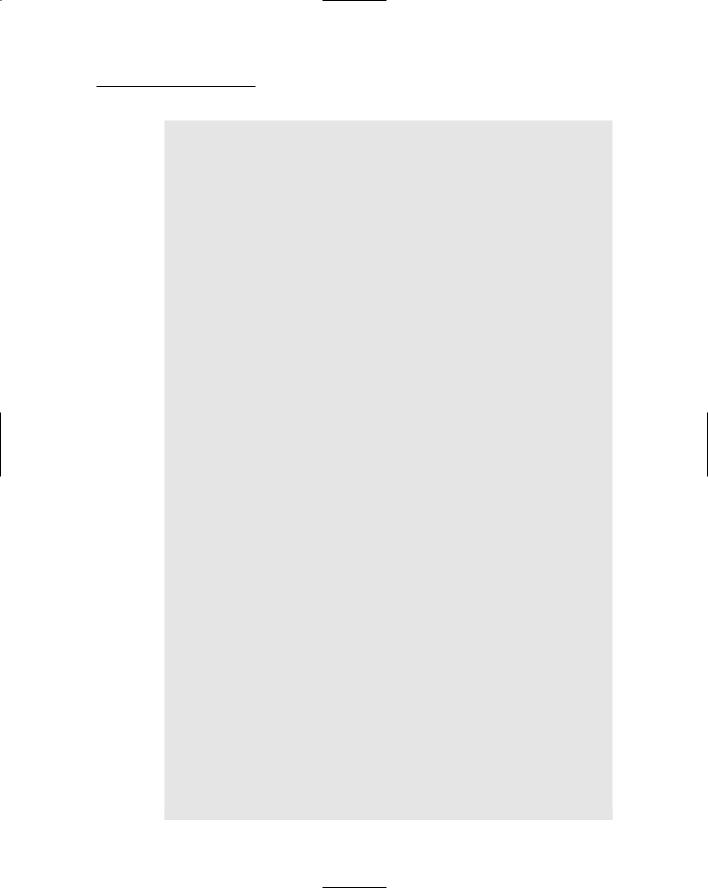
Chapter 7: Building a Product Maintenance Application 241
<Columns>
<asp:BoundField DataField=”catid” HeaderText=”ID” ReadOnly=”True”> <HeaderStyle HorizontalAlign=”Left” /> <ItemStyle Width=”80px” />
</asp:BoundField> <asp:BoundField DataField=”name”
HeaderText=”Name”>
<HeaderStyle HorizontalAlign=”Left” /> <ItemStyle Width=”100px” />
</asp:BoundField> <asp:BoundField DataField=”desc”
HeaderText=”Description”> <HeaderStyle HorizontalAlign=”Left” /> <ItemStyle Width=”400px” />
</asp:BoundField>
<asp:CommandField
CausesValidation=”False” ShowEditButton=”True” />
<asp:CommandField
CausesValidation=”False” ShowDeleteButton=”True” />
</Columns>
</asp:GridView>
<asp:SqlDataSource ID=”SqlDataSource1” runat=”server” ConflictDetection=”CompareAllValues” ConnectionString=
“<%$ ConnectionStrings:ConnectionString %>” OldValuesParameterFormatString=”original_{0}” DeleteCommand=”DELETE FROM [Categories]
WHERE [catid] = @original_catid AND [name] = @original_name AND [desc] = @original_desc”
InsertCommand=”INSERT INTO [Categories] ([catid], [name], [desc])
VALUES (@catid, @name, @desc)” SelectCommand=”SELECT [catid],
[name], [desc]
FROM [Categories] ORDER BY [catid]” UpdateCommand=”UPDATE [Categories]
SET [name] = @name, [desc] = @desc WHERE [catid] = @original_catid
AND [name] = @original_name AND [desc] = @original_desc”>
<DeleteParameters>
<asp:Parameter Name=”original_catid” Type=”String” />
<asp:Parameter Name=”original_name” Type=”String” />
<asp:Parameter Name=”original_desc” Type=”String” />
4
5
6
7
8
9
10
11
12
13
14
(continued)
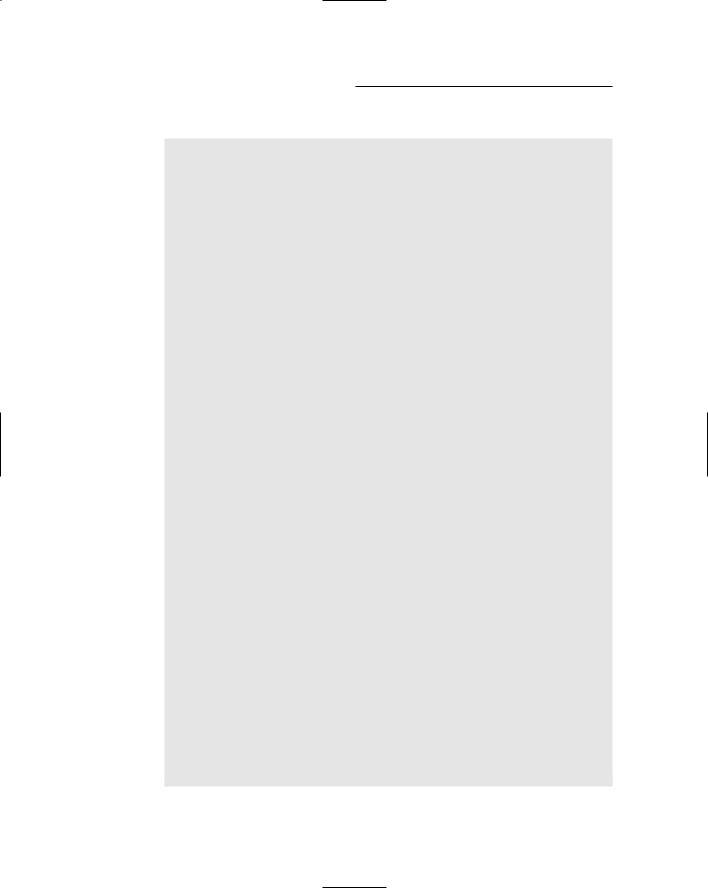
242 Part IV: Building Back-End Applications
Listing 7-4 (continued)
</DeleteParameters>
<UpdateParameters> <asp:Parameter Name=”name”
Type=”String” /> <asp:Parameter Name=”desc” Type=”String” />
<asp:Parameter Name=”original_catid” Type=”String” />
<asp:Parameter Name=”original_name” Type=”String” />
<asp:Parameter Name=”original_desc” Type=”String” />
</UpdateParameters>
<InsertParameters> <asp:Parameter Name=”catid”
Type=”String” /> <asp:Parameter Name=”name” Type=”String” /> <asp:Parameter Name=”desc” Type=”String” />
</InsertParameters>
</asp:SqlDataSource> <br />
<asp:Label ID=”lblMessage” runat=”server” EnableViewState=”False” ForeColor=”Red” /><br />
Enter the category information below to create a new category:<br /><br /> <asp:Label ID=”Label1” runat=”server” BorderStyle=”None” Width=”80px”
Text=”ID:” />
<asp:TextBox ID=”txtID” runat=”server” />
<asp:RequiredFieldValidator ID=”RequiredFieldValidator1” runat=”server” ControlToValidate=”txtID” ErrorMessage=”Required.” />
<br />
<asp:Label ID=”Label2” runat=”server” BorderStyle=”None” Width=”80px” Text=”Name:” />
<asp:TextBox ID=”txtName” runat=”server” />
<asp:RequiredFieldValidator ID=”RequiredFieldValidator2” runat=”server” ControlToValidate=”txtName” ErrorMessage=”Required.” />
<br />
15
16
17
18
19

Chapter 7: Building a Product Maintenance Application 243
<asp:Label ID=”Label3” runat=”server” |
|
BorderStyle=”None” Width=”80px” |
|
Text=”Description:” /> |
|
<asp:TextBox ID=”txtDesc” runat=”server” /> |
20 |
|
|
<asp:RequiredFieldValidator |
|
ID=”RequiredFieldValidator3” runat=”server” |
|
ControlToValidate=”txtDesc” |
|
ErrorMessage=”Required.” /><br /> |
|
<br /> |
|
<asp:Button ID=”btnAdd” runat=”server” |
21 |
OnClick=”btnAdd_Click” |
|
Text=”Add Category” /><br /><br /> |
|
<asp:LinkButton ID=”LinkButton1” |
22 |
runat=”server” |
|
PostBackUrl=”~/Default.aspx”
CausesValidation=”false” > Return to Home Page
</asp:LinkButton>
</asp:Content>
Here’s a detailed look at each of the numbered lines in this listing:
1 The Page directive specifies the Master Page and other information for the page.
To use the Visual Basic version of the code-behind file — shown in Listing 7-6 — you must change the AutoEventWireup attribute to false.
2 The <Content> element provides the content that’s displayed in the <ContentPlaceHolder> element of the Master Page.
3 The GridView control displays the rows from the Categories table. It’s bound to the data source named SqlDataSource1, which is defined in line 9. Notice also that it specifies methods to handle the RowDeleted and RowUpdated events.
To use the Visual Basic version of the code-behind file for this page, you should remove the OnRowDeleted and OnRowUpdated attributes.
4 The elements under the <Columns> element define the columns displayed by the GridView control. This one defines the first column in the grid, which displays the category ID. Notice that this column is read-only. That prevents the user from changing the category ID for a category.
5 The column defined by this <BoundField> element displays the category name.

244 Part IV: Building Back-End Applications
6 This column displays the category description.
7 This line defines a command field that displays an Edit link, which the user can click to edit the row. When the user clicks the Edit link, the labels in the name and desc columns for the row are replaced by text boxes, and the Edit link is replaced by Update and Cancel links.
8 This line defines a command field that displays a Delete link, which lets the user delete a row.
9 This <SqlDataSource> element defines the data source used for the GridView control. The ConflictDetection attribute specifies CompareAllValues, which enables optimistic concurrency checking for the data source. Then the ConnectionString attribute specifies that the connection string for the data source should be retrieved from the Web.config file. Finally, the
OldParameterValuesFormatString attribute specifies the format string that’s used to create the parameter names used to supply the original parameter values. In this case, the
word original_ is simply added to the beginning of each parameter name.
10 The <DeleteCommand> element provides the DELETE statement used to delete rows from the table. Notice that the original values of the catid, name, and desc columns are listed in the WHERE clause. The values @original_catid, @original_name, and
@original_desc parameters are automatically provided by the GridView control when the user deletes a row.
11 The InsertCommand attribute provides the INSERT statement used to insert a row in the Categories table. Note that the GridView control doesn’t use this INSERT statement, as the GridView control doesn’t provide a way for the user to insert rows. Instead, the code that’s executed when the user clicks the Add Category button (defined in line 21) calls this statement.
12 This SELECT statement is used to retrieve the categories from the Categories table.
13 The UPDATE statement updates a row in the Categories table. Notice that the original values are used in the WHERE clause to provide optimistic concurrency checking.
14 The <DeleteParameters> element defines the parameters used by the DELETE statement.
15 The <UpdateParameters> element defines the parameters used by the UPDATE statement.
16 The <InsertParameters> element defines the parameters used by the INSERT statement.
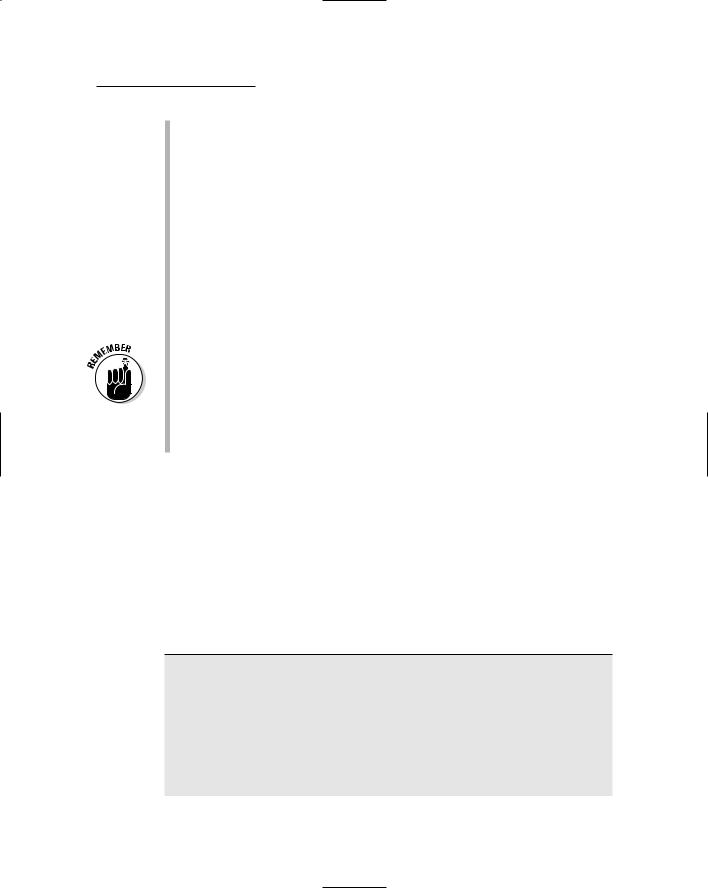
Chapter 7: Building a Product Maintenance Application 245
17 This label is used by the code-behind file to display messages. For example, if an error occurs while trying to update or delete a category, a suitable error message is displayed in this label.
18 This text box lets the user enter the category ID for a new category. Note that a RequiredFieldValidator ensures that the user enters a value into this field.
19 This text box lets the user enter the name for a new category.
A RequiredFieldValidator is used to force the user to enter a name.
20 This text box is where the user enters the description for a new category. Once again, a RequiredFieldValidator is present to require an entry for this text box.
21 The Add Category button lets the user add a new category using the ID, name, and description entered into the text boxes.
If you’re using the Visual Basic version of the code-behind file, you should remove the OnClick attribute.
22 This link button provides a link back to the menu page. Note that CausesValidation is set to false for this button. That way the RequiredFieldValidator isn’t enforced for any of the three text boxes when the user clicks the link.
The code-behind file for the
Catalog Maintenance page
The CatMaint.aspx page requires a code-behind file to handle the buttonclick event for the Add Category button, as well as the RowUpdated and RowDeleted events for the GridView control. Listing 7-5 shows the C# version of this code-behind file, and Listing 7-6 shows the Visual Basic version.
Listing 7-5: The code-behind file for the Catalog Maintenance page (C#)
using System; using System.Data;
using System.Configuration; using System.Collections; using System.Web;
using System.Web.Security; using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts; using System.Web.UI.HtmlControls;
(continued)

246 Part IV: Building Back-End Applications
Listing 7-5 (continued)
public partial class CatMaint : System.Web.UI.Page |
|
{ |
|
protected void btnAdd_Click( |
1 |
object sender, EventArgs e) |
|
{ |
|
setParameter(“catid”, txtID.Text); |
|
setParameter(“name”, txtName.Text); |
|
setParameter(“desc”, txtDesc.Text); |
|
try |
|
{ |
|
SqlDataSource1.Insert(); |
|
txtID.Text = “”; |
|
txtName.Text = “”; |
|
txtDesc.Text = “”; |
|
} |
|
catch (Exception) |
|
{ |
|
lblMessage.Text = |
|
“There is already a category “ |
|
+ “with that ID. Please try another.”; |
|
} |
|
} |
|
private void setParameter(string name, |
2 |
string value) |
|
{ |
|
SqlDataSource1.InsertParameters[name] |
|
.DefaultValue = value; |
|
} |
|
protected void GridView1_RowUpdated( |
3 |
object sender, |
|
GridViewUpdatedEventArgs e) |
|
{ |
|
if (e.Exception != null) |
|
{ |
|
lblMessage.Text = “Incorrect data. “ |
|
+ “Please try again.”; |
|
e.ExceptionHandled = true; |
|
e.KeepInEditMode = true; |
|
} |
|
else if (e.AffectedRows == 0) |
|
{ |
|
lblMessage.Text = “That category could not “ |
|
+ “be updated. Please try again.”; |
|
} |
|
} |
|
protected void GridView1_RowDeleted( |
4 |
object sender, |
|
GridViewDeletedEventArgs e) |
|
|
|