
- •Contents
- •What Is C#?
- •C# Versus Other Programming Languages
- •Preparing to Program
- •The Program Development Cycle
- •Your First C# Program
- •Types of C# Programs
- •Summary
- •Workshop
- •C# Applications
- •Basic Parts of a C# Application
- •Structure of a C# Application
- •Analysis of Listing 2.1
- •Object-Oriented Programming (OOP)
- •Displaying Basic Information
- •Summary
- •Workshop
- •Variables
- •Using Variables
- •Understanding Your Computer’s Memory
- •C# Data Types
- •Numeric Variable Types
- •Literals Versus Variables
- •Constants
- •Reference Types
- •Summary
- •Workshop
- •Types of Operators
- •Punctuators
- •The Basic Assignment Operator
- •Mathematical/Arithmetic Operators
- •Relational Operators
- •Logical Bitwise Operators
- •Type Operators
- •The sizeof Operator
- •The Conditional Operator
- •Understanding Operator Precedence
- •Converting Data Types
- •Understanding Operator Promotion
- •For Those Brave Enough
- •Summary
- •Workshop
- •Controlling Program Flow
- •Using Selection Statements
- •Using Iteration Statements
- •Using goto
- •Nesting Flow
- •Summary
- •Workshop
- •Introduction
- •Abstraction and Encapsulation
- •An Interactive Hello World! Program
- •Basic Elements of Hello.cs
- •A Few Fundamental Observations
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Essential Elements of SimpleCalculator.cs
- •A Closer Look at SimpleCalculator.cs
- •Simplifying Your Code with Methods
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Lexical Structure
- •Some Thoughts on Elevator Simulations
- •Concepts, Goals and Solutions in an Elevator Simulation Program: Collecting Valuable Statistics for Evaluating an Elevator System
- •A Deeper Analysis of SimpleElevatorSimulation.cs
- •Class Relationships and UML
- •Summary
- •Review Questions
- •Programming Exercises
- •The Hello Windows Forms Application
- •Creating and Using an Event Handler
- •Defining the Border Style of the Form
- •Adding a Menu
- •Adding a Menu Shortcut
- •Handling Events from Menus
- •Dialogs
- •Creating Dialogs
- •Using Controls
- •Data Binding Strategies
- •Data Binding Sources
- •Simple Binding
- •Simple Binding to a DataSet
- •Complex Binding of Controls to Data
- •Binding Controls to Databases Using ADO.NET
- •Creating a Database Viewer with Visual Studio and ADO.NET
- •Resources in .NET
- •Localization Nuts and Bolts
- •.NET Resource Management Classes
- •Creating Text Resources
- •Using Visual Studio.NET for Internationalization
- •Image Resources
- •Using Image Lists
- •Programmatic Access to Resources
- •Reading and Writing RESX XML Files
- •The Basic Principles of GDI+
- •The Graphics Object
- •Graphics Coordinates
- •Drawing Lines and Simple Shapes
- •Using Gradient Pens and Brushes
- •Textured Pens and Brushes
- •Tidying up Your Lines with Endcaps
- •Curves and Paths
- •The GraphicsPath Object
- •Clipping with Paths and Regions
- •Transformations
- •Alpha Blending
- •Alpha Blending of Images
- •Other Color Space Manipulations
- •Using the Properties and Property Attributes
- •Demonstration Application: FormPaint.exe
- •Why Use Web Services?
- •Implementing Your First Web Service
- •Testing the Web Service
- •Implementing the Web Service Client
- •Understanding How Web Services Work
- •Summary
- •Workshop
- •How Do Web References Work?
- •What Is UDDI?
- •Summary
- •Workshop
- •Passing Parameters and Web Services
- •Accessing Data with Web Services
- •Summary
- •Workshop
- •Managing State in Web Services
- •Dealing with Slow Services
- •Workshop
- •Creating New Threads
- •Synchronization
- •Summary
- •The String Class
- •The StringBuilder Class
- •String Formatting
- •Regular Expressions
- •Summary
- •Discovering Program Information
- •Dynamically Activating Code
- •Reflection.Emit
- •Summary
- •Simple Debugging
- •Conditional Debugging
- •Runtime Tracing
- •Making Assertions
- •Summary

Working with Operators |
95 |
This will not accomplish what you want. If the person is 21 or older and a female, the statement will not execute. The AND portion will result in being false. To overcome this problem, you can force how the statements are evaluated using the parenthesis punctuator. To accomplish the desired results, you would change the previous example to
if( age >= 21 AND (gender == male OR gender == female)) // statement
The execution always starts with the innermost parenthesis. In this case, the statement (gender == male OR gender == female) is evaluated first. Because this uses OR, this portion of the statement will evaluate to true if either side is true. If this is true, the AND will compare the age value to see whether the age is greater than or equal to
this proves to be true as well, the statement will execute.
Tip
Use parentheses to make sure that you get code to execute in the order you want.
DO
DO use parentheses to make complex math and relational operations easier to understand.
DON’T |
4 |
DON’T confuse the assignment operator (=) with the relational equals operator (==).
Logical Bitwise Operators
There are three other logical operators that you might want to use: the logical bitwise operators. Although the use of bitwise operations is beyond the scope of this book, I’ve included a section near the end of today’s lesson called “For Those Brave Enough.” This section explains bitwise operations, the three logical bitwise operators, and the bitwise shift operators.
The bitwise operators obtain their name from the fact that they operate on bits. A bit is a single storage location that stores either an on or off value (equated to 0 or 1). In the section at the end of today’s lesson, you learn how the bitwise operators can be used to manipulate these bits.
Type Operators
As you begin working with classes and interfaces later in this book, you will have need for the type operators. Without understanding interfaces and classes, it is hard to fully
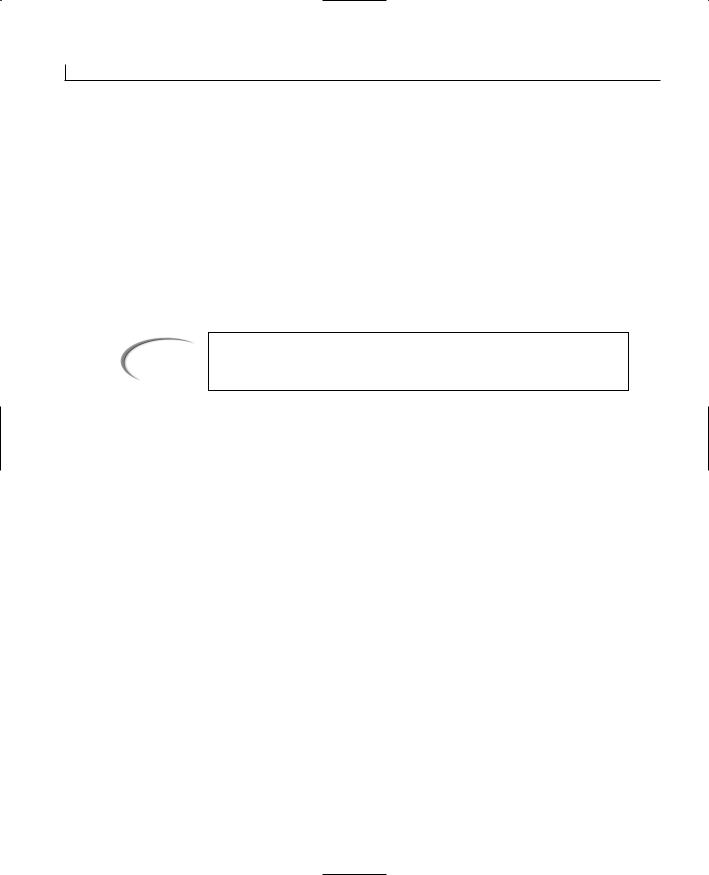
96 |
Day 4 |
understand these operators. For now, be aware that there are a number of operators that you will need later. These type operators are
•typeof
•is
•as
The sizeof Operator
You have already seen the sizeof operator. This sizeof operator is used to determine the size of a value. You saw the sizeof operator in action on Day 3.
Caution |
Because the sizeof operator manipulates memory directly, avoid its use if |
|
possible. |
||
|
The Conditional Operator
There is one ternary operator in C#, the conditional operator. The conditional operator has the following format:
Condition ? if_true_statement : if_false_statement;
As you can see, there are three parts to this operation, with two symbols used to separate them. The first part of the command is a condition. This is just like the conditions you created earlier for the if statement. This can be any condition that results in either true or false.
After the condition is a question mark. The question mark separates the condition from the first of two statements. The first of the two statements executes if the condition is true. The second statement is separated from the first with a colon and is executed if the condition is false. Listing 4.6 presents the conditional operator in action.
The conditional operator is used to create concise code. If you have a simple if statement that evaluates to doing a simple true and simple false statement, the conditional operator can be used. Otherwise, you should avoid the use of the conditional operator.
Because it is just a shortcut version of an if statement, you should just stick with using the if statement itself. Most people reviewing your code will find the if statement easier to read and understand.

Working with Operators |
97 |
LISTING 4.6 cond.cs—The Conditional Operator in Action
1:// cond.cs - The conditional operator
2://----------------------------------------------------
4:class cond
5:{
6:static void Main()
7:{
8:int Val1 = 1;
9:int Val2 = 0;
10:int result;
11:
12: result = (Val1 == Val2) ? 1 : 0; 13:
14:System.Console.WriteLine(“The result is {0}”, result);
15:}
16:}
The result is 0
This listing is very simple. In line 12, the conditional operator is executed and
the result is placed in the variable, result. Line 14 then prints this value. In this 4 case, the conditional operator is checking to see whether the value in Val1 is equal to the
value in Val2. Because 1 is not equal to 0, the false result of the conditional is set. Modify line 8 so that Val2 is set equal to 1 and then rerun this listing. You will see that because 1 is equal to 1, the result will be 1 instead of 0.
|
|
|
Caution |
|
The conditional operator provides a shortcut for implementing an if |
|
statement. Although it is more concise, it is not always the easiest for to |
|
|
|
|
|
|
understand. When using the conditional operator, you should verify that |
|
|
|
|
|
you are not making your code harder to understand. |
|
|
|
Understanding Operator Precedence
You’ve learned about a lot of different operators in today’s lessons. Rarely are these operators used one at a time. Often, multiple operators will be used in a single statement. When this happens, a lot of issues seem to arise. Consider the following:
Answer = 4 * 5 + 6 / 2 – 1;
What is the value of Answer? If you said 12, you are wrong. If you said 44 you are also wrong. The answer is 22.

98 |
Day 4 |
There is a set order in which different types of operators are executed. This set order is called operator precedence. The word precedence is used because some
operators have a higher level of precedence than others. In the example, multiplication and division have a higher level of precedence than addition and subtraction. This means that 4 is multiplied by 5 and 6 is divided by 2, before any addition occurs.
Table 4.3 lists all the operators. The operators at each level of the table are at the same level of precedence. In almost all cases, there is no impact on the results. For example, 5 * 4 / 10 is the same whether 5 is multiplied by 4 first or 4 is divided by 10.
TABLE 4.3 Operator Precedence
Level |
Operator Types |
Operators |
|
|
|
||
1 |
Primary operators |
() . [] x++ x— new |
|||||
|
|
typeof |
sizeof |
checked unchecked |
|||
2 |
Unary |
+ |
- |
! |
~ ++x |
—x |
|
3 |
Multiplicative |
* |
/ |
% |
|
|
|
4 |
Additive |
+ |
- |
|
|
|
|
5 |
Shift |
<< |
>> |
|
|
|
|
6 |
Relational |
< |
> |
<= |
>= |
is |
|
7 |
Equality |
== |
!= |
|
|
|
|
8 |
Logical AND |
& |
|
|
|
|
|
9 |
Logical XOR |
^ |
|
|
|
|
|
10 |
Logical OR |
| |
|
|
|
|
|
11 |
Conditional AND |
&& |
|
|
|
|
|
12 |
Conditional OR |
|| |
|
|
|
|
|
13 |
Conditional |
?: |
|
|
|
|
|
14 |
Assignment |
= |
*= |
/= |
%= |
+= |
-= |
|
|
<<= |
>>= |
&= |
^= |
|= |
|
|
|
|
|
|
|
|
|
Changing Precedence Order
You learned how to change the order of precedence by using parentheses punctuators earlier in today’s lessons. Because parentheses have a higher level of precedence than the operators, what is enclosed in them is evaluated before operators outside of them. Using the earlier example, you can force the addition and subtraction to occur first by using parentheses: