
- •Contents
- •What Is C#?
- •C# Versus Other Programming Languages
- •Preparing to Program
- •The Program Development Cycle
- •Your First C# Program
- •Types of C# Programs
- •Summary
- •Workshop
- •C# Applications
- •Basic Parts of a C# Application
- •Structure of a C# Application
- •Analysis of Listing 2.1
- •Object-Oriented Programming (OOP)
- •Displaying Basic Information
- •Summary
- •Workshop
- •Variables
- •Using Variables
- •Understanding Your Computer’s Memory
- •C# Data Types
- •Numeric Variable Types
- •Literals Versus Variables
- •Constants
- •Reference Types
- •Summary
- •Workshop
- •Types of Operators
- •Punctuators
- •The Basic Assignment Operator
- •Mathematical/Arithmetic Operators
- •Relational Operators
- •Logical Bitwise Operators
- •Type Operators
- •The sizeof Operator
- •The Conditional Operator
- •Understanding Operator Precedence
- •Converting Data Types
- •Understanding Operator Promotion
- •For Those Brave Enough
- •Summary
- •Workshop
- •Controlling Program Flow
- •Using Selection Statements
- •Using Iteration Statements
- •Using goto
- •Nesting Flow
- •Summary
- •Workshop
- •Introduction
- •Abstraction and Encapsulation
- •An Interactive Hello World! Program
- •Basic Elements of Hello.cs
- •A Few Fundamental Observations
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Essential Elements of SimpleCalculator.cs
- •A Closer Look at SimpleCalculator.cs
- •Simplifying Your Code with Methods
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Lexical Structure
- •Some Thoughts on Elevator Simulations
- •Concepts, Goals and Solutions in an Elevator Simulation Program: Collecting Valuable Statistics for Evaluating an Elevator System
- •A Deeper Analysis of SimpleElevatorSimulation.cs
- •Class Relationships and UML
- •Summary
- •Review Questions
- •Programming Exercises
- •The Hello Windows Forms Application
- •Creating and Using an Event Handler
- •Defining the Border Style of the Form
- •Adding a Menu
- •Adding a Menu Shortcut
- •Handling Events from Menus
- •Dialogs
- •Creating Dialogs
- •Using Controls
- •Data Binding Strategies
- •Data Binding Sources
- •Simple Binding
- •Simple Binding to a DataSet
- •Complex Binding of Controls to Data
- •Binding Controls to Databases Using ADO.NET
- •Creating a Database Viewer with Visual Studio and ADO.NET
- •Resources in .NET
- •Localization Nuts and Bolts
- •.NET Resource Management Classes
- •Creating Text Resources
- •Using Visual Studio.NET for Internationalization
- •Image Resources
- •Using Image Lists
- •Programmatic Access to Resources
- •Reading and Writing RESX XML Files
- •The Basic Principles of GDI+
- •The Graphics Object
- •Graphics Coordinates
- •Drawing Lines and Simple Shapes
- •Using Gradient Pens and Brushes
- •Textured Pens and Brushes
- •Tidying up Your Lines with Endcaps
- •Curves and Paths
- •The GraphicsPath Object
- •Clipping with Paths and Regions
- •Transformations
- •Alpha Blending
- •Alpha Blending of Images
- •Other Color Space Manipulations
- •Using the Properties and Property Attributes
- •Demonstration Application: FormPaint.exe
- •Why Use Web Services?
- •Implementing Your First Web Service
- •Testing the Web Service
- •Implementing the Web Service Client
- •Understanding How Web Services Work
- •Summary
- •Workshop
- •How Do Web References Work?
- •What Is UDDI?
- •Summary
- •Workshop
- •Passing Parameters and Web Services
- •Accessing Data with Web Services
- •Summary
- •Workshop
- •Managing State in Web Services
- •Dealing with Slow Services
- •Workshop
- •Creating New Threads
- •Synchronization
- •Summary
- •The String Class
- •The StringBuilder Class
- •String Formatting
- •Regular Expressions
- •Summary
- •Discovering Program Information
- •Dynamically Activating Code
- •Reflection.Emit
- •Summary
- •Simple Debugging
- •Conditional Debugging
- •Runtime Tracing
- •Making Assertions
- •Summary
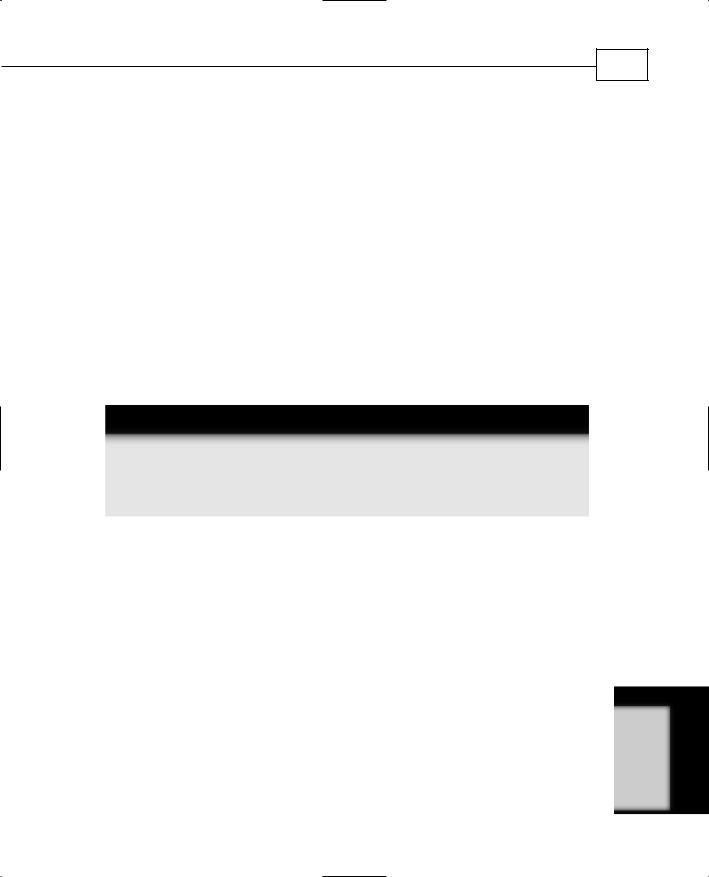
String Manipulation
CHAPTER 25
533
char charResult;
string str1 = “string 1”;
charResult = str1[3];
Console.WriteLine(“str1[3]: {0}”, charResult);
In this example, the indexer extracts the third character from a zero-based count on str1. The result is the character ‘i’.
The StringBuilder Class
For direct manipulation of a string, use the StringBuilder class. It’s the best solution when a lot of work needs to be done to change a string. It’s more efficient for manipulation operations because, unlike a String object, it doesn’t incur the overhead involved in creating a new object on every method call. The StringBuilder class is a member of the
System.Text namespace.
Tip
A String instantiates and returns a new object when its contents are modified. It’s a good idea to consider using a StringBuilder for string modifications to avoid the overhead associated with additional instantiations of modified string objects.
Instance Methods
The StringBuilder class doesn’t have static methods. All of its methods operate on the instance they’re invoked from. The invoking object is referred to in following sections as this StringBuilder.
The Append() Method
The Append() method adds a typed object to this StringBuilder. Here’s an example of how to implement the Append() method:
StringBuilder myStringBuilder;
myStringBuilder = new StringBuilder(“Original”);
myStringBuilder.Append(“Appended”);
Console.WriteLine( “myStringBuilder.Append(\”Appended\”): {0}”, myStringBuilder);
25
TRINGS
ANIPULATIONM

534
Extreme C#
PART IV
This example shows how to append one string to another with the Append() method. The result is “OriginalAppended”. The Append() method has the following overloads:
•Append(bool)
•Append(byte)
•Append(char)
•Append(char[])
•Append(decimal)
•Append(double)
•Append(short)
•Append(int)
•Append(long)
•Append(Object)
•Append(sbyte)
•Append(float)
•Append(ushort)
•Append(uint)
•Append(ulong)
•Append(char, int)
•Append(char[], int, int)
•Append(string, int, int)
In these overloads, each type is converted to its string representation and appended to this StringBuilder. The Append(char, int) overload appends a specified number, int, of char to this StringBuilder. In the last two overloads, the first int specifies the beginning character of either the char[] or string to start appending, and the second int specifies the number of characters to append.
The AppendFormat() Method
The AppendFormat() method can replace multiple format specifications with a properly formatted value. Here’s an example of how to implement the AppendFormat() method:
StringBuilder myStringBuilder;
myStringBuilder = new StringBuilder(“Original”);
myStringBuilder.AppendFormat(“{0,10}”, “Appended”);
Console.WriteLine(
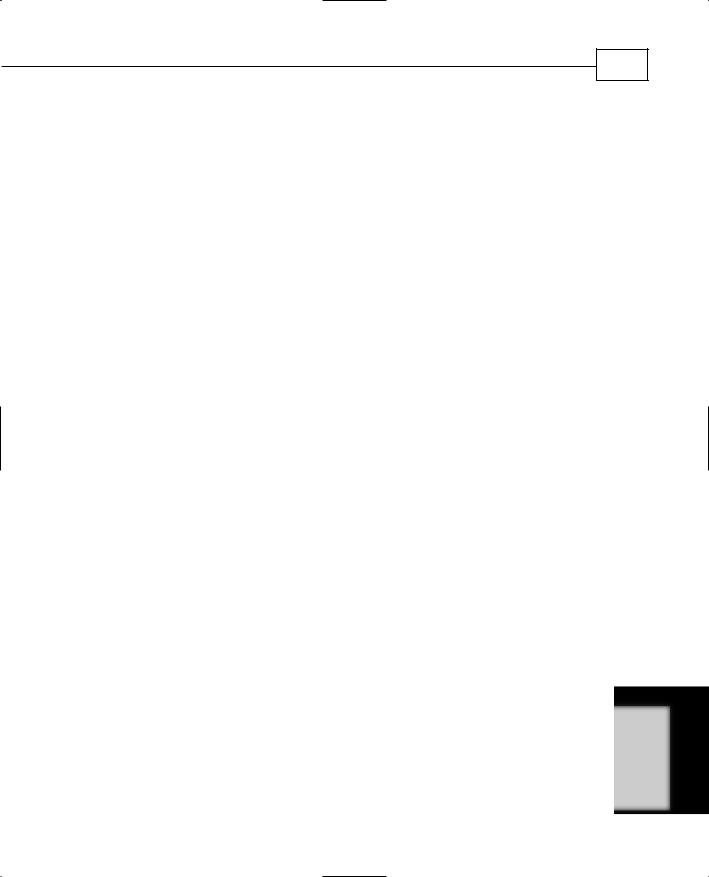
String Manipulation
CHAPTER 25
535
“myStringBuilder.AppendFormat(\”{0,10}\”,\”Appended\”): {0}”, myStringBuilder);
This example uses the AppendFormat() method to format the “Appended” string to 10 characters and then append it to myStringBuilder. The result is “Original Appended”, with two spaces between words because “Appended” was formatted to 10 characters. The AppendFormat() method has the following overloads:
•AppendFormat(string, Object[])
•AppendFormat(IformatProvider, string, Object)
•AppendFormat(string, Object, Object)
•AppendFormat(string, Object, Object, Object)
In these overloads, the string parameter is the format specification. The Object parameters are the object(s) upon which to apply formatting. The IFormatProvider is an interface that is implemented by an object to manage formatting.
The EnsureCapacity() Method
The EnsureCapacity() method guarantees that a StringBuilder will have a specified minimal size. Here’s an example of how to implement the EnsureCapacity() method:
StringBuilder myStringBuilder; int capacity;
myStringBuilder = new StringBuilder();
capacity = myStringBuilder.EnsureCapacity(129);
Console.WriteLine( “myStringBuilder.EnsureCapacity(129): {0}”, capacity);
The example shows the EnsureCapacity() method guaranteeing that myStringBuilder will have at least a 129-character capacity. The result will have an actual capacity setting of 256, because, interestingly, the capacity is set to the lowest power of two greater than the specified capacity. Setting the minimum capacity to 258, for instance, results in an actual capacity setting of 512.
The Equals() Method
The Equals() method compares a given StringBuilder to this StringBuilder. It returns true when both StringBuilders are equal, and false otherwise. Here’s an example of how to implement the Equals() method:
StringBuilder myStringBuilder;
StringBuilder anotherStringBuilder;
25
TRINGS
ANIPULATIONM

536 |
Extreme C# |
|
PART IV |
||
|
bool boolResult;
myStringBuilder = new StringBuilder(“my string builder”); anotherStringBuilder = new StringBuilder(“another string builder”);
boolResult = myStringBuilder.Equals(anotherStringBuilder);
Console.WriteLine( “myStringBuilder.Equals(anotherStringBuilder): {0}”, boolResult);
The Equals() method in this example compares two StringBuilder objects. Since their values are different, the Equals() method returns false.
The Insert() Method
The Insert() method places a specified object into this StringBuilder at a specified location. Here’s an example of how to implement the Insert() method:
StringBuilder myStringBuilder;
myStringBuilder = new StringBuilder(“one, three”);
myStringBuilder.Insert(3, “, two”);
Console.WriteLine(
“myStringBuilder.Insert(3, \”, two\”): {0}”, myStringBuilder);
The example shows how to insert a string into a StringBuilder. The original string, “one, three” becomes “one, two, three”. The Insert() method has the following overloads:
•Insert(int, bool)
•Insert(int, byte)
•Insert(int, char)
•Insert(int, char[])
•Insert(int, decimal)
•Insert(int, double)
•Insert(int, short)
•Insert(int, int)
•Insert(int, long)
•Insert(int, Object)
•Insert(int, sbyte)
•Insert(int, float)
•Insert(int, ushort)

String Manipulation
CHAPTER 25
537
•Insert(int, uint)
•Insert(int, ulong)
•Insert(int, string, countInt)
•Insert(int, char[], beginInt, numberInt)
In the overloads, each type is converted to its string representation and inserted into this StringBuilder at the position specified by int. The countInt specifies the number of strings to insert at int position. The beginInt and numberInt parameters indicate, respectively, where in char[] to begin using characters to insert, and numberInt indicates how many items from char[] to insert.
The Remove() Method
The Remove() method deletes a specified span of characters from this StringBuilder. Here’s an example of how to implement the Remove() method:
StringBuilder myStringBuilder;
myStringBuilder = new StringBuilder(“Jane X. Doe”);
myStringBuilder.Remove(4, 3);
Console.WriteLine( “myStringBuilder.Remove(4, 3): {0}”, myStringBuilder);
As the example shows, the first parameter is the zero-based position to begin removing characters, and the second parameter is the number of characters to remove. Removing three characters transforms “Jane X. Doe” into “Jane Doe”.
The Replace() Method
The Replace() method replaces a specified set of characters with another. Here’s an example of how to implement the Replace() method:
StringBuilder myStringBuilder;
myStringBuilder = new StringBuilder(“Jane X. Doe”);
myStringBuilder.Replace(‘X’, ‘B’);
Console.WriteLine( “myStringBuilder.Replace(‘X’, ‘B’): {0}”, myStringBuilder);
This example shows the Replace() method accepting two string parameters. The first is the character to be replaced, and the second parameter is the character that will replace the first. The result is that “Jane X. Doe” is transformed to “Jane B. Doe”. The Replace() method has the following overloads:
25
TRINGS
ANIPULATIONM

538
Extreme C#
PART IV
•Replace(string, string)
•Replace(char, char, beginInt, numberInt)
•Replace(string, string, beginInt, numberInt)
In these overloads, a second string parameter will replace the first, and a second char parameter will replace the first char parameter. The beginInt parameter references the position in this StringBuilder to begin replacement, and the numberInt indicates the offset from beginInt of where to stop replacing.
The ToString() Method
The ToString() method converts this StringBuilder to a string. Here’s an example of how to implement the ToString() method:
StringBuilder myStringBuilder; string stringResult;
myStringBuilder = new StringBuilder(“my string”);
stringResult = myStringBuilder.ToString();
Console.WriteLine( “myStringBuilder.ToString(): {0}”, stringResult);
The ToString() method has the following overload:
• ToString(beginInt, numberInt)
In the overload, beginInt is the starting position in this StringBuilder to start extracting characters, and numberInt is the number of characters to convert.
Properties and Indexers
The String class has a few properties and an indexer.
The Capacity Property
The Capacity property sets and returns the number of characters this StringBuilder can hold. Here’s an example of how to implement the Capacity property:
StringBuilder myStringBuilder; int intResult;
myStringBuilder = new StringBuilder(“my string”);
intResult = myStringBuilder.Capacity;

String Manipulation
CHAPTER 25
539
Console.WriteLine( “myStringBuilder.Capacity: {0}”, intResult);
The result of this example is 16.
The Length Property
The StringBuilder Length property returns the number of characters in a String. Here’s an example of how to implement the Length property:
StringBuilder myStringBuilder; int intResult;
myStringBuilder = new StringBuilder(“my string”);
intResult = myStringBuilder.Length;
Console.WriteLine( “myStringBuilder.Length: {0}”, intResult);
The result of this example is 9.
The MaxCapacity Property
The MaxCapacity property is a read-only property that returns the maximum number of characters this StringBuilder can hold. Here’s an example of how to implement the
MaxCapacity property:
StringBuilder myStringBuilder; int intResult;
myStringBuilder = new StringBuilder(“my string”);
intResult = myStringBuilder.MaxCapacity;
Console.WriteLine( “myStringBuilder.MaxCapacity: {0}”, intResult);
The result of this example is 2147483647.
The Indexer
The indexer permits reading and writing of a specified character at a certain position. Here’s an example of how to implement the Indexer property:
StringBuilder myStringBuilder; char charResult;
myStringBuilder = new StringBuilder(“my string”);
charResult = myStringBuilder[1];
25
TRINGS
ANIPULATIONM