
- •Contents
- •What Is C#?
- •C# Versus Other Programming Languages
- •Preparing to Program
- •The Program Development Cycle
- •Your First C# Program
- •Types of C# Programs
- •Summary
- •Workshop
- •C# Applications
- •Basic Parts of a C# Application
- •Structure of a C# Application
- •Analysis of Listing 2.1
- •Object-Oriented Programming (OOP)
- •Displaying Basic Information
- •Summary
- •Workshop
- •Variables
- •Using Variables
- •Understanding Your Computer’s Memory
- •C# Data Types
- •Numeric Variable Types
- •Literals Versus Variables
- •Constants
- •Reference Types
- •Summary
- •Workshop
- •Types of Operators
- •Punctuators
- •The Basic Assignment Operator
- •Mathematical/Arithmetic Operators
- •Relational Operators
- •Logical Bitwise Operators
- •Type Operators
- •The sizeof Operator
- •The Conditional Operator
- •Understanding Operator Precedence
- •Converting Data Types
- •Understanding Operator Promotion
- •For Those Brave Enough
- •Summary
- •Workshop
- •Controlling Program Flow
- •Using Selection Statements
- •Using Iteration Statements
- •Using goto
- •Nesting Flow
- •Summary
- •Workshop
- •Introduction
- •Abstraction and Encapsulation
- •An Interactive Hello World! Program
- •Basic Elements of Hello.cs
- •A Few Fundamental Observations
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Essential Elements of SimpleCalculator.cs
- •A Closer Look at SimpleCalculator.cs
- •Simplifying Your Code with Methods
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Lexical Structure
- •Some Thoughts on Elevator Simulations
- •Concepts, Goals and Solutions in an Elevator Simulation Program: Collecting Valuable Statistics for Evaluating an Elevator System
- •A Deeper Analysis of SimpleElevatorSimulation.cs
- •Class Relationships and UML
- •Summary
- •Review Questions
- •Programming Exercises
- •The Hello Windows Forms Application
- •Creating and Using an Event Handler
- •Defining the Border Style of the Form
- •Adding a Menu
- •Adding a Menu Shortcut
- •Handling Events from Menus
- •Dialogs
- •Creating Dialogs
- •Using Controls
- •Data Binding Strategies
- •Data Binding Sources
- •Simple Binding
- •Simple Binding to a DataSet
- •Complex Binding of Controls to Data
- •Binding Controls to Databases Using ADO.NET
- •Creating a Database Viewer with Visual Studio and ADO.NET
- •Resources in .NET
- •Localization Nuts and Bolts
- •.NET Resource Management Classes
- •Creating Text Resources
- •Using Visual Studio.NET for Internationalization
- •Image Resources
- •Using Image Lists
- •Programmatic Access to Resources
- •Reading and Writing RESX XML Files
- •The Basic Principles of GDI+
- •The Graphics Object
- •Graphics Coordinates
- •Drawing Lines and Simple Shapes
- •Using Gradient Pens and Brushes
- •Textured Pens and Brushes
- •Tidying up Your Lines with Endcaps
- •Curves and Paths
- •The GraphicsPath Object
- •Clipping with Paths and Regions
- •Transformations
- •Alpha Blending
- •Alpha Blending of Images
- •Other Color Space Manipulations
- •Using the Properties and Property Attributes
- •Demonstration Application: FormPaint.exe
- •Why Use Web Services?
- •Implementing Your First Web Service
- •Testing the Web Service
- •Implementing the Web Service Client
- •Understanding How Web Services Work
- •Summary
- •Workshop
- •How Do Web References Work?
- •What Is UDDI?
- •Summary
- •Workshop
- •Passing Parameters and Web Services
- •Accessing Data with Web Services
- •Summary
- •Workshop
- •Managing State in Web Services
- •Dealing with Slow Services
- •Workshop
- •Creating New Threads
- •Synchronization
- •Summary
- •The String Class
- •The StringBuilder Class
- •String Formatting
- •Regular Expressions
- •Summary
- •Discovering Program Information
- •Dynamically Activating Code
- •Reflection.Emit
- •Summary
- •Simple Debugging
- •Conditional Debugging
- •Runtime Tracing
- •Making Assertions
- •Summary

Understanding C# Programs |
45 |
Summary
Today’s lesson continued to help build a foundation that will be used to teach you C#. Today you learned about some of the basic parts of a C# application. You learned that comments help make your programs easier to understand.
You also learned about the basic parts of a C# application, including whitespace, C# keywords, literals, and identifiers. Looking at an application, you saw how these parts are
combined to create a complete listing. This included seeing a special identifier used as a 2 starting point in an application—Main().
After you examined a listing, you explored an overview of object-oriented programming, including the concepts of encapsulation, polymorphism, inheritance, and reuse.
Today’s lesson concluded with some basic information on using the
System.Console.WriteLine() and System.Console.Write() routines. You learned that these two routines are used to print information to the screen (console).
Q&A
Q What is the difference between component-based and object-oriented?
AC# has been referred to as component-based by some people. Component-based development can be considered an extension of object-oriented programming. A component is simply a standalone piece of code that performs a specific task.
Component-based programming consists of creating lots of these standalone components that can be reused. You then link them to build applications.
Q What other languages are considered object-oriented?
AOther languages that are considered object-oriented include C++, Java, and SmallTalk. Microsoft’s Visual Basic.NET can also be used for object-oriented programming. There are other languages, but these are the most popular.
Q What is composition? Is it an object-oriented term?
AMany people confuse inheritance with composition, but they are different. With composition, one object is used within another object. Figure 2.1 is a composition of many circles. This is different from the sphere in the previous example. The sphere is not composed of a circle; it is an extension of the circle. To summarize the difference between composition and inheritance: Composition occurs when one class (object) has another within it. Inheritance occurs when one class (object) is an expansion of another.

46 |
Day 2 |
FIGURE 2.1
A snowman made of circles.
Workshop
The Workshop provides quiz questions to help you solidify your understanding of the material covered and exercises to provide you with experience in using what you’ve learned. Try to understand the quiz and exercise answers before continuing to the next day’s lesson. Answers are provided in Appendix A, “Answers.”
Quiz
1.What are the three types of comments you can use in a C# program?
2.How are each of the three types of comments entered into a C# program?
3.What impact does whitespace have on a C# program?
4.Which of the following are C# keywords?
field, cast, as, object, throw, baseball, catch, football, fumble, basketball
5.What is a literal?
6.Which is true:
Expressions are made up of statements. Statements are made up of expressions.
Expressions and statements have nothing to do with each other.
7.What is an empty statement?
8.What are the key concepts of object-oriented programming?
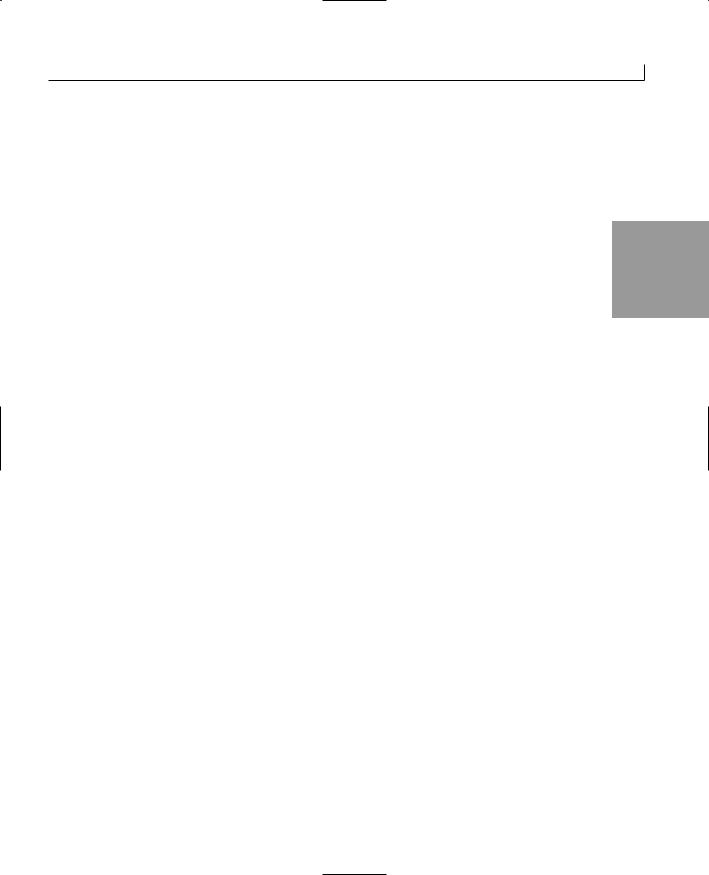
Understanding C# Programs |
47 |
9.What is the difference between WriteLine() and Write()?
10.What is used as a placeholder when printing a value with WriteLine() or
Write()?
Exercises
1. Enter and compile the following program (listit.cs). Remember, you don’t enter the
line numbers. |
2 |
|||
1: // |
ListIT.cs - program to print a listing with line numbers |
|||
|
||||
2: |
// |
----------------------------------------------------------- |
|
|
3: |
|
|
|
4:using System;
5:using System.IO;
7:class ListIT
8:{
9:public static void Main(string[] args)
10:{
11:try
12:{
13:
14:int ctr=0;
15:if (args.Length <= 0 )
16:{
17:Console.WriteLine(“Format: ListIT filename”);
18:return;
19:}
20:else
21:{
22:FileStream f = new FileStream(args[0], FileMode.Open);
23:try
24:{
25: |
StreamReader t = new StreamReader(f); |
26: |
string line; |
27: |
while ((line = t.ReadLine()) != null) |
28: |
{ |
29: |
ctr++; |
30: |
Console.WriteLine(“{0}: {1}”, ctr, line); |
31: |
} |
32: |
f.Close(); |
33:}
34:finally
35:{
36: f.Close();
37:}
38:}
39:}
40:catch (System.IO.FileNotFoundException)
41:{

48 |
Day 2 |
42: Console.WriteLine (“ListIT could not find the file {0}”, args[0]);
43: } 44:
45:catch (Exception e)
46:{
47:Console.WriteLine(“Exception: {0}\n\n”, e);
48:}
49:}
50:}
2.Run the program entered in exercise 1. What happens when you run this program? Run this program a second time. This time enter the following at the command line:
listit listit.cs
What happens this time?
3.Enter, compile, and run the following program. What does it do?
1:// ex0203.cs - Exercise 3 for Day 2
2://-----------------------------------------------
3:
4:class Exercise2
5:{
6:public static void Main()
7:{
8:int x = 0;
9:
10:for( x = 1; x <= 10; x++ )
11:{
12:System.Console.Write(“{0:D3} “, x);
13:}
14:}
15:}
4.BUG BUSTER: The following program has a problem. Enter it in your editor and compile it. Which lines generate error messages?
1:// bugbust.cs
2://-----------------------------------------------
3:
4:class bugbust
5:{
6:public static void Main()
7:{
8:System.Console.WriteLine(“\nA fun number is {1}”, 123 );
9:}
10:}
5.Write the line of code that prints your name on the screen.

WEEK 1
DAY 3
Storing Information with Variables
When you start writing programs, you are going to quickly find that you need to keep track of different types of information. This might be the tracking of your clients’ names, the amounts of money in your bank accounts, or the ages of your favorite movie stars. To keep track of this information, your computer programs need a way to store the values. Today you
•Learn what is a variable
•Discover how to create variable names in C#
•Use different types of numeric variables
•Evaluate the differences and similarities between character and numeric values
•See how to declare and initialize variables