
- •Contents
- •What Is C#?
- •C# Versus Other Programming Languages
- •Preparing to Program
- •The Program Development Cycle
- •Your First C# Program
- •Types of C# Programs
- •Summary
- •Workshop
- •C# Applications
- •Basic Parts of a C# Application
- •Structure of a C# Application
- •Analysis of Listing 2.1
- •Object-Oriented Programming (OOP)
- •Displaying Basic Information
- •Summary
- •Workshop
- •Variables
- •Using Variables
- •Understanding Your Computer’s Memory
- •C# Data Types
- •Numeric Variable Types
- •Literals Versus Variables
- •Constants
- •Reference Types
- •Summary
- •Workshop
- •Types of Operators
- •Punctuators
- •The Basic Assignment Operator
- •Mathematical/Arithmetic Operators
- •Relational Operators
- •Logical Bitwise Operators
- •Type Operators
- •The sizeof Operator
- •The Conditional Operator
- •Understanding Operator Precedence
- •Converting Data Types
- •Understanding Operator Promotion
- •For Those Brave Enough
- •Summary
- •Workshop
- •Controlling Program Flow
- •Using Selection Statements
- •Using Iteration Statements
- •Using goto
- •Nesting Flow
- •Summary
- •Workshop
- •Introduction
- •Abstraction and Encapsulation
- •An Interactive Hello World! Program
- •Basic Elements of Hello.cs
- •A Few Fundamental Observations
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Essential Elements of SimpleCalculator.cs
- •A Closer Look at SimpleCalculator.cs
- •Simplifying Your Code with Methods
- •Summary
- •Review Questions
- •Programming Exercises
- •Introduction
- •Lexical Structure
- •Some Thoughts on Elevator Simulations
- •Concepts, Goals and Solutions in an Elevator Simulation Program: Collecting Valuable Statistics for Evaluating an Elevator System
- •A Deeper Analysis of SimpleElevatorSimulation.cs
- •Class Relationships and UML
- •Summary
- •Review Questions
- •Programming Exercises
- •The Hello Windows Forms Application
- •Creating and Using an Event Handler
- •Defining the Border Style of the Form
- •Adding a Menu
- •Adding a Menu Shortcut
- •Handling Events from Menus
- •Dialogs
- •Creating Dialogs
- •Using Controls
- •Data Binding Strategies
- •Data Binding Sources
- •Simple Binding
- •Simple Binding to a DataSet
- •Complex Binding of Controls to Data
- •Binding Controls to Databases Using ADO.NET
- •Creating a Database Viewer with Visual Studio and ADO.NET
- •Resources in .NET
- •Localization Nuts and Bolts
- •.NET Resource Management Classes
- •Creating Text Resources
- •Using Visual Studio.NET for Internationalization
- •Image Resources
- •Using Image Lists
- •Programmatic Access to Resources
- •Reading and Writing RESX XML Files
- •The Basic Principles of GDI+
- •The Graphics Object
- •Graphics Coordinates
- •Drawing Lines and Simple Shapes
- •Using Gradient Pens and Brushes
- •Textured Pens and Brushes
- •Tidying up Your Lines with Endcaps
- •Curves and Paths
- •The GraphicsPath Object
- •Clipping with Paths and Regions
- •Transformations
- •Alpha Blending
- •Alpha Blending of Images
- •Other Color Space Manipulations
- •Using the Properties and Property Attributes
- •Demonstration Application: FormPaint.exe
- •Why Use Web Services?
- •Implementing Your First Web Service
- •Testing the Web Service
- •Implementing the Web Service Client
- •Understanding How Web Services Work
- •Summary
- •Workshop
- •How Do Web References Work?
- •What Is UDDI?
- •Summary
- •Workshop
- •Passing Parameters and Web Services
- •Accessing Data with Web Services
- •Summary
- •Workshop
- •Managing State in Web Services
- •Dealing with Slow Services
- •Workshop
- •Creating New Threads
- •Synchronization
- •Summary
- •The String Class
- •The StringBuilder Class
- •String Formatting
- •Regular Expressions
- •Summary
- •Discovering Program Information
- •Dynamically Activating Code
- •Reflection.Emit
- •Summary
- •Simple Debugging
- •Conditional Debugging
- •Runtime Tracing
- •Making Assertions
- •Summary

WEEK 1
DAY 2
Understanding C#
Programs
In addition to understanding the basic composition of a program, you also need to understand the structure of creating a C# program. Today you
•Learn about the parts of a C# application
•Understand C# statements and expressions
•Discover the facts about object-oriented programming
•Examine encapsulation, polymorphism, inheritance, and reuse
•Display basic information in your programs
C# Applications
The first part of today’s lesson focuses on a simple C# application. Using Listing 2.1, you will gain an understanding of some of the key parts of a C# application.
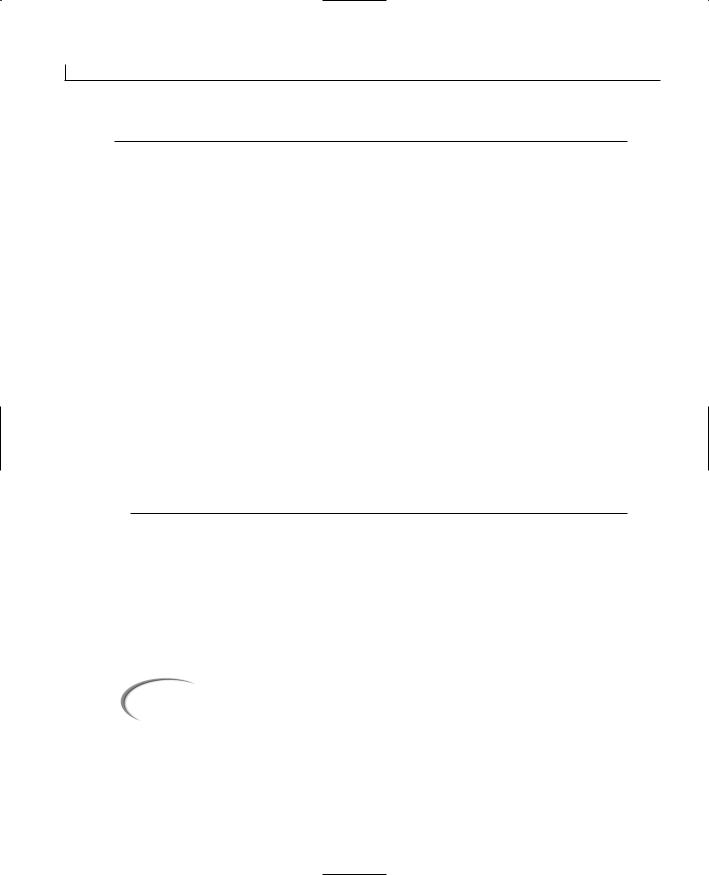
30 |
Day 2 |
LISTING 2.1 app.cs—Example C# Application
1:// app.cs - A sample C# application
2:// Don’t worry about understanding everything in
3:// this listing. You’ll learn all about it later!
4://———————————————————————-
6: using System; 7:
8:class sample
9:{
10:public static void Main()
11:{
12://Declare variables
14:int radius = 4;
15:const double PI = 3.14159;
16:double area;
17:
18: //Do calculation 19:
20: area = PI * radius * radius; 21:
22: //Print the results 23:
24:Console.WriteLine(“Radius = {0}, PI = {1}”, radius, PI );
25:Console.WriteLine(“The area is {0}”, area);
26:}
27:}
You should enter this listing into your editor and then use your compiler to create the program. You can save the program as app.cs. When compiling the program, you enter the following at the command prompt:
csc app.cs
Alternatively, if you are using a visual editor, you should be able to select a compiler from the menu options.
Caution |
Remember, you don’t enter the line numbers or the colons when you are |
|
entering the listing above. The line numbers are to help discuss the listing in |
||
|
||
|
the lessons. |
|
|
|

Understanding C# Programs |
31 |
When you run the program, you get the following output:
OUTPUT |
Radius = 4, PI = 3.14159 |
|
|
The area is 50.3344 |
|
||
As you can see, the output from this listing is pretty straightforward. The value of a |
|
||
radius and the value of PI are displayed. The area of a circle based on these two values is |
|
||
then displayed. |
|
||
2 |
|||
In the following sections you are going to learn about some of the different parts of this |
|||
program. Don’t worry about understanding everything. In the lessons presented on later |
|
||
days, you will be revisiting this information in much greater detail. The purpose of the |
|
||
following sections is to give you a first look. |
|
||
Comments |
|
||
|
|||
The first four lines of Listing 2.1 are comments. Comments are used to enter information |
|
||
in your program that can be ignored by the compiler. Why would you want to enter |
|
||
information that the compiler will ignore? There are a number of reasons. |
|
||
Comments are often used to provide descriptive information about your listing—for |
|
||
example, identification information. Additionally, by entering comments, you can docu- |
|
||
ment what a listing is expected to do. Even though you might be the only one to use a |
|
||
listing, it is still a good idea to put in information about what the program does and how |
|
||
it does it. Although you know what the listing does now—because you just wrote it— |
|
||
you might not be able to remember later what you were thinking. If you give your listing |
|
||
to others, the comments will help them understand what the code was intended to do. |
|
||
Comments can also be used to provide revision history of a listing. |
|
||
The main thing to understand about comments is that they are for programmers using the |
|
||
listing. The compiler actually ignores them. In C#, there are three types of comments you |
|
||
can use: |
|
||
• |
One-line comments |
|
|
• |
Multiline comments |
|
|
• |
Documentation comments |
|
Tip
Comments are removed by the compiler. Because they are removed, there is no penalty for having them in your program listings. If in doubt, you should include a comment.

32 |
Day 2 |
One-Line Comments
Listing 2.1 uses one-line comments in each of lines 1 through 4. Lines 12, 18, and 22 also contain one-line comments. One-line comments have the following format:
// comment text
The two slashes indicate that a comment is beginning. From that point to the end of the current line, everything is treated as a comment.
A one-line comment does not have to start at the beginning of the line. You can actually have C# code on the line before the comments; however, after the two forward slashes, the rest of the line is a comment.
Multiline Comments
Listing 2.1 does not contain any multiline comments, but there are times when you want a comment to go across multiple lines. In this case you can either start each line with the double forward slash (as in lines 1 to 4 of the listing), or you can use multiline comments.
Multiline comments are created with a starting and ending token. To start a multiline comment, you enter a forward slash followed by an asterisk:
/*
Everything after that token is a comment until you enter the ending token. The ending token is an asterisk followed by a forward slash:
*/
The following is a comment:
/* this is a comment */
The following is also a comment:
/* this is
a comment that is on
a number of lines */
You can also enter this comment as the following:
//this is
//a comment that
//is on
//a number of
//lines

Understanding C# Programs |
33 |
The advantage of using multiline comments is that you can “comment out” a section of a code listing by simply adding the /* and */. Anything that appears between the /* and the */ is ignored by the compiler as a comment.
Caution |
You cannot nest multiline comments. This means that you cannot place one |
|
|
multiline comment inside of another. For example, the following is an error: |
|
2 |
|
|
|
||
|
/* Beginning of a comment... |
|
|
|
|
|
|
|
/* with another comment nested */ |
|
|
|
*/ |
|
|
|
|
|
|
|
|
|
|
Documentation Comments
C# has a special type of comment that enables you to create external documentation automatically.
These comments are identified with three slashes instead of the two used for single-line comments. These comments also use Extensible Markup Language (XML) style tags. XML is a standard used to mark up data. Although any valid XML tag can be used, common tags used for C# include <c>, <code>, <example>, <exception>, <list>, <para>,
<param>, <paramref>, <permission>, <remarks>, <returns>, <see>, <seealso>, <summary>, and <value>.
These comments are placed in your code listings. Listing 2.2 shows an example of these comments being used. You can compile this listing as you have earlier listings. See Day 1, “Getting Started with C#,” if you need a refresher.
LISTING 2.2 xmlapp.cs—Using XML Comments
1:// xmlapp.cs - A sample C# application using XML
2: |
// |
documentation |
3: |
// |
----------------------------------------------- |
4: |
|
|
5:/// <summary>
6:/// This is a summary describing the class.</summary>
7:/// <remarks>
8:/// This is a longer comment that can be used to describe
9:/// the class. </remarks>
10:class myApp
11:{
12:/// <summary>
13:/// The entry point for the application.
14:/// </summary>
15:/// <param name=”args”> A list of command line arguments</param>
16:public static void Main(string[] args)

34 |
Day 2 |
LISTING 2.2 continued
17:{
18:System.Console.WriteLine(“An XML Documented Program”);
19:}
20:}
When you compile and execute this listing, you get the following output:
OUTPUT An XML Documented Program
To get the XML documentation, you must compile this listing differently from what you have seen before. To get the XML documentation, add the /doc parameter when you compile at the command line. If you are compiling at the command line, you enter
csc /doc:xmlfile xmlapp.cs
When you compile, you get the same output as before when you run the program. The difference is that you also get a file called xmlfile that contains documentation in XML. You can replace xmlfile with any name you’d like to give your XML file. For Listing 2.2, the XML file is
<?xml version=”1.0”?> <doc>
<assembly>
<name>xmlapp</name>
</assembly>
<members>
<member name=”T:myApp”> <summary>
This is a summary describing the class.</summary> <remarks>
This is a longer comment that can be used to describe the class. </remarks>
</member>
<member name=”M:myApp.Main(System.String[])”> <summary>
The entry point for the application. </summary>
<param name=”args”> A list of command line arguments</param> </member>
</members>
</doc>
Note
It is beyond the scope of this book to cover XML and XML files.