
Expert Service-Oriented Architecture In CSharp 2005 (2006) [eng]
.pdf
96C H A P T E R 5 ■ W E B S E R V I C E S E N H A N C E M E N T S 3 . 0
Listing 5-5. Digitally Signing a SOAP Request Message via the SoapContext
using Microsoft.Web.Services3;
using Microsoft.Web.Services3.Security;
using Microsoft.Web.Services3.Security.Tokens;
//Retrieve the SoapContext for the outgoing SOAP request message StockTraderServiceWse serviceProxy = new StockTraderServiceWse();
//Retrieve the X509 certificate from the CurrentUserStore certificate store X509SecurityToken token = GetSigningToken();
//Add signature element to a security section on the request to sign the request serviceProxy.RequestSoapContext.Security.Tokens.Add( token ); serviceProxy.RequestSoapContext.Security.Elements.Add(
new MessageSignature( token ) );
This concludes the introduction to the WSE 3.0 API. The remainder of this chapter focuses on installation and configuration options for WSE 3.0. The subsequent chapters in the book are dedicated to showing you how to use the WSE API to implement the WSspecifications in your own service-oriented applications.
Install and Configure WSE 3.0
WSE 3.0 is easy to install and to configure. You must install Visual Studio 2005 prior to installing WSE 3.0, since WSE 3.0 will not install with earlier versions. You can install Visual Studio 2005 side by side with Visual Studio .NET 2003 if required.
WSE 3.0 is a package of QuickStart sample applications and documentation that shows you how to use the various classes in the WSE assembly. But the engine of WSE 3.0 is a single assembly called Microsoft.Web.Services3.dll, which is installed by default under C:\Program Files\Microsoft WSE\v3.0. In addition, this assembly gets automatically registered in the Global Assembly Cache (GAC).
In order to use the new assembly in your Web services projects, you will need to register it as a SOAP extension within either the machine.config or web.config files. If you update the machine.config file, the assembly will automatically be registered for all future Web services projects. Otherwise, you will need to update the web.config files for each new project individually.
Listing 5-6 shows the two additional elements that you must update in the web.config file in order for your project to use WSE. You may actually require additional entries, but these are specific to individual WSspecifications such as WS-Security and are only required as needed. Note that you must include each individual element on a single line. In Listing 5-6, elements such as <section> are broken out on multiple lines for clarity only. They must, however, be entered as single lines in the actual web.config file.
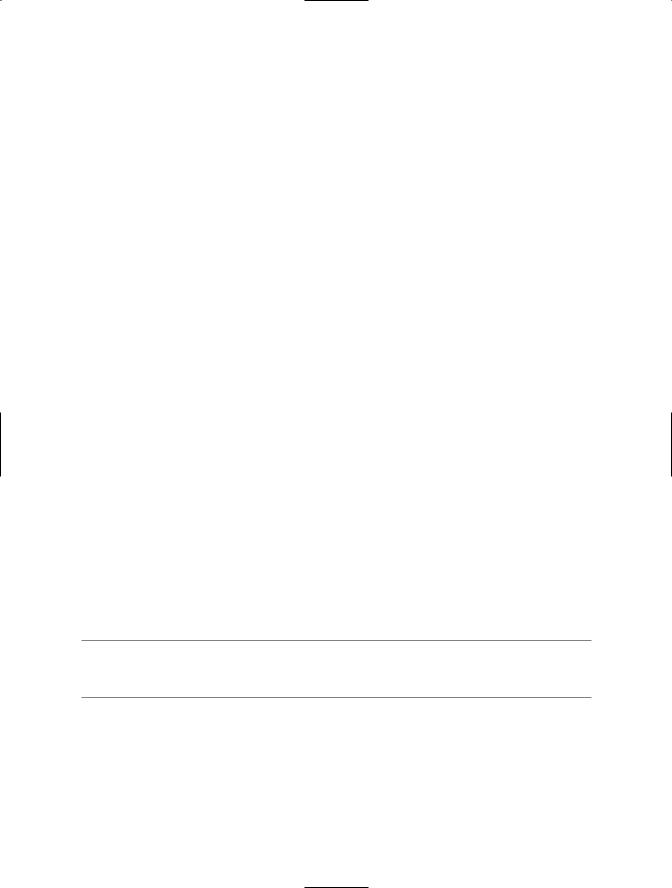
C H A P T E R 5 ■ W E B S E R V I C E S E N H A N C E M E N T S 3 . 0 |
97 |
Listing 5-6. The web.config Updates for a WSE-Enabled Web Service Project
<configuration xmlns="http://schemas.microsoft.com/.NetConfiguration/v2.0"> <configSections>
<section name="microsoft.web.services3" type="Microsoft.Web.Services3.Configuration.WebServicesConfiguration, Microsoft.Web.Services3, Version=3.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" />
</configSections>
<system.web>
<webServices>
< soapServerProtocolFactory type="Microsoft.Web.Services3.WseProtocolFactory, Microsoft.Web.Services3, Version=3.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35"/>
</soapServerProtocolFactory>
<soapExtensionImporterTypes>
<add type="Microsoft.Web.Services3.Description.WseExtensionImporter, Microsoft.Web.Services3, Version=3.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35"/>
</soapExtensionImporterTypes>
</webServices>
</system.web>
</configuration>
Web service client projects do not need to register the SOAP extension, but they do need to register the WebServicesConfiguration class. In addition, the client’s Web service proxy class must inherit from
Microsoft.Web.Services3.WebServicesClientProtocol
Without WSE, the proxy class file inherits from
System.Web.Services.Protocols.SoapHttpClientProtocol
This change is required so that Web service requests get routed through the WSE filters rather than through the standard HTTP-based SOAP filters.
■Note If you want to update the machine.config file, simply copy the <section> element from Listing 5-2 into the machine.config file, under the <configSections> node.
If you prefer to not type these entries manually (and we certainly do!), then you can use the convenient Configuration Editor that ships with WSE 3.0. This tool provides a tabbed GUI interface in which you specify configuration settings for a project and then automatically apply the settings without having to write the code manually. The tool can be accessed directly from within your Visual Studio .NET project, as shown in Figure 5-2.
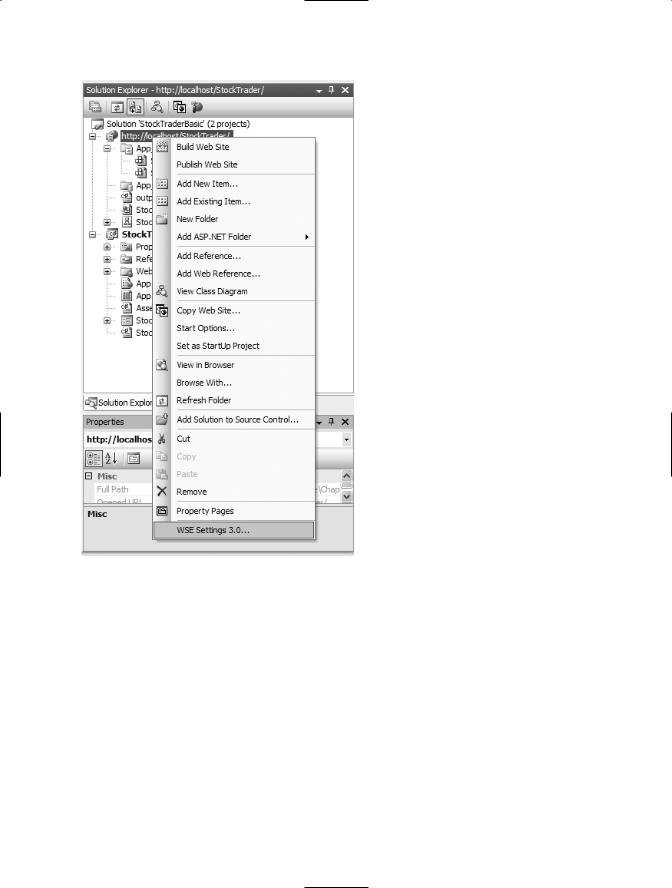
98 C H A P T E R 5 ■ W E B S E R V I C E S E N H A N C E M E N T S 3 . 0
Figure 5-2. Menu access for the WSE 3.0 Configuration Editor
Figure 5-3 shows how you can use the editor to implement the basic settings we have covered so far. You can use the editor for all .NET project types. If you are using it for an ASP.NET Web application or service project, it gives you an additional option to register the SOAP extension class. Otherwise, the second check box in the GUI interface is disabled. The editor settings shown in Figure 5-3 will generate the web.config settings that are shown in Listing 5-6. This is not bad for two simple check box clicks!
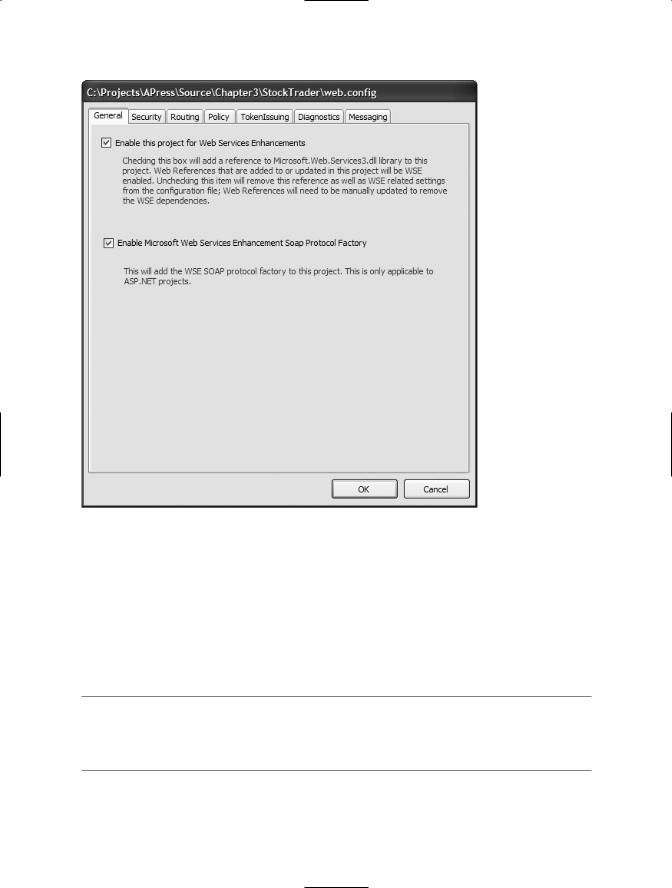
C H A P T E R 5 ■ W E B S E R V I C E S E N H A N C E M E N T S 3 . 0 |
99 |
Figure 5-3. The WSE 3.0 Configuration Editor
When you create a new client application for your WSE-enabled Web service, you can generate the proxy class in two ways. You can either generate it manually from the WSDL document, or you can generate it using Visual Studio .NET’s Add Web Reference Wizard. If you use the wizard, keep in mind that the generated proxy file will contain two separate proxy classes. One inherits from the WebServicesClientProtocol class, which is provided by the Microsoft.Web.Services3 assembly. The other class inherits from the traditional SoapHttpClientProtocol class, which is provided by the System.Web.Services assembly.
■Note The Configuration Editor provides helpful configuration support for several of the WSspecifications, as you can tell from the additional tabs in Figure 5-3. We will discuss the additional support that the Configuration Editor provides in the relevant chapters.
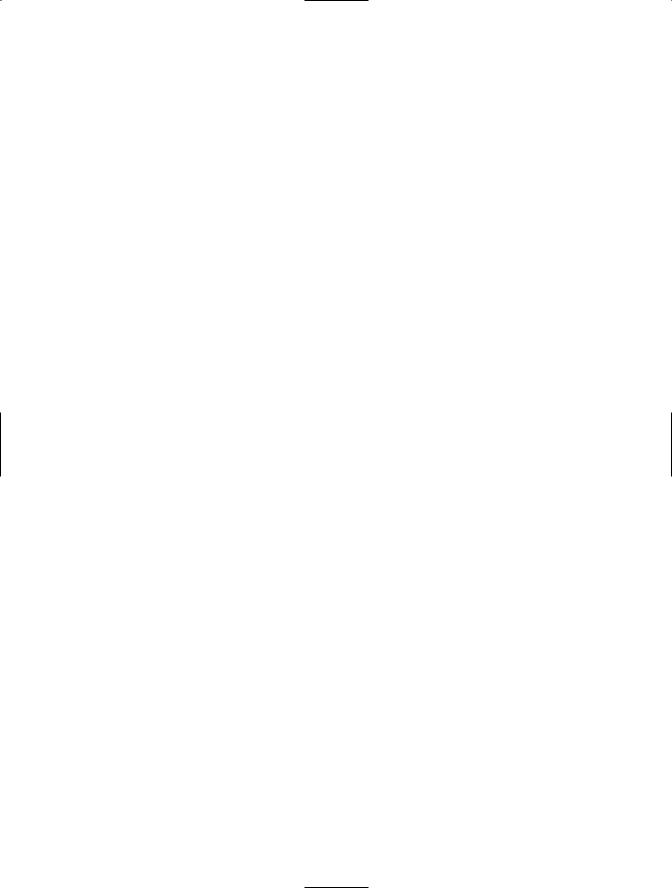
100 C H A P T E R 5 ■ W E B S E R V I C E S E N H A N C E M E N T S 3 . 0
X.509 Certificate Support
Several of the upcoming sample solutions in this book use X.509 digital certificates, which can be used to digitally sign and encrypt SOAP messages (with the help of WSE). In addition, WSE 3.0 uses X.509 digital certificates in its QuickStart sample applications. Certificate installation and configuration can be quite complex, so we felt it was important to provide a section on how to install and configure the X.509 sample certificates.
X.509 Certificates Explained
X.509 digital certificates are widely used as a basis for securing communication between separate endpoints. For example, they are used to support the HTTP Secure Sockets Layer (SSL) protocol, otherwise known as HTTPS.
You will be working directly with the X.509 test certificates that ship with WSE 3.0. You actually have several options for obtaining test certificates:
•Use the WSE 3.0 test certificates (the most convenient option).
•Use the makecert.exe command-line utility to generate test certificates.
•Obtain a test certificate from VeriSign.
Digital certificates are used for asymmetric encryption, also known as public-key encryption. The certificate is used to generate a public-private key pair, whereby the private key is known only to one party, while the public key may be distributed to anyone.
In a service-oriented application that includes a client and a Web service, it is the client that typically procures the certificate and the public-private key pair. This is the model that the sample applications use, so it is important to understand how it works. In an SOA application, certificates and keys are used as follows:
•The client uses the certificate to digitally sign an outgoing SOAP request message (to the Web service).
•The Web service uses the public key to encrypt the outgoing SOAP response message (to the client).
•The client uses the private key to decrypt the incoming SOAP response message (from the Web service).
Chapter 6 provides detailed explanations of how encryption and digital signing work under the hood; but for now this is all you need to know, because it helps you to understand where the certificates and keys need to be registered.
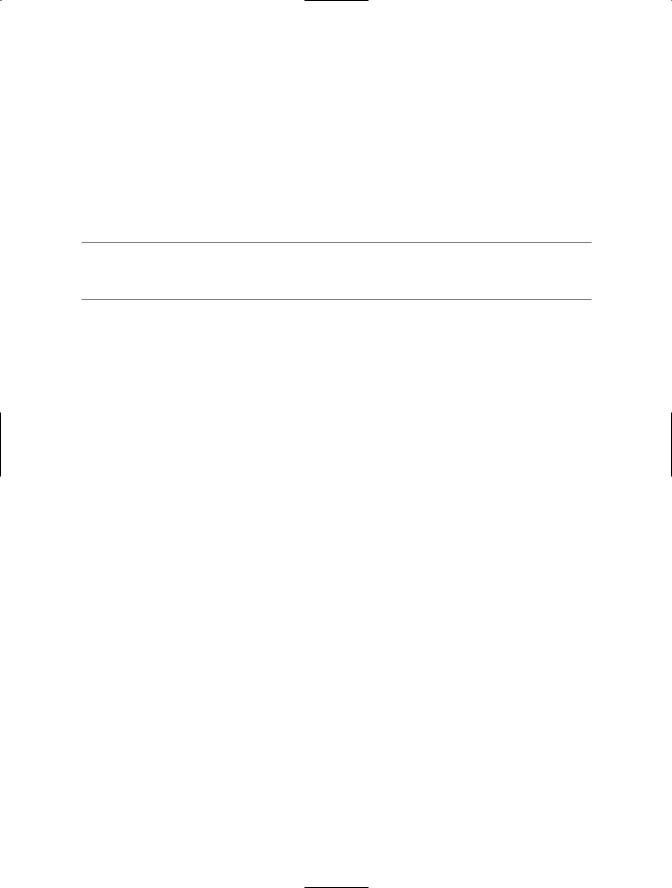
C H A P T E R 5 ■ W E B S E R V I C E S E N H A N C E M E N T S 3 . 0 |
101 |
Installing the X.509 Test Certificates
Web servers such as IIS provide good support tools for installing digital certificates that will be used to support HTTPS. In addition, Windows operating systems provide a Microsoft Management Console (MMC) snap-in called the Certificate Manager for working with certificates.
The sample applications in this book use the X.509 test certificate to support public-key encryption and to support digital signing; therefore, not only do you need the certificate itself, but you also need a public-private key pair that has been generated from the certificate. Luckily, WSE 3.0 ships with these keys already generated, so you are saved one more manual step.
■Caution WSE 3.0 test certificates should not be used in production applications. Their keys are wellknown, so they will not provide any effective security in production applications.
The digital certificate and the keys need to be stored in a location called the certificate store, which you can access using the Certificate Manager snap-in. For testing purposes, most of us use the same machine to run the Web service and the client applications. This requires you to update two certificate stores:
•The Local Computer certificate store: Used by the Web service, this location should store the public key.
•The Current User certificate store: Used by the client, this location should store the certificate and the private key.
You can install the certificates manually, or you can run a preset batch script called Setup.bat that ships with WSE 3.0 and is available in the folder C:\Program Files\Microsoft WSE\v3.0\Samples. The batch script is the easiest way to install the test certificates; however, you will need to know how to install them manually as well for your own development work outside of the QuickStart samples or the code samples that accompany this book.
Here are the installation steps to manually install the certificates:
1.Open a new MMC console by typing mmc in the Run dialog window.
2.Select File Add/Remove Snap-In. Click the Add button and then select Certificates from the available list. You will be prompted to select the type of account that will manage the certificates. Select My User Account and click Finish.
3.Repeat step 2, but this time when you are prompted for an account, select Computer Account and click Finish. Click OK to close out the dialog box for adding certificate stores. You should now be looking at an MMC console view that displays the Current User and the Local Computer certificate stores, as shown in Figure 5-4.
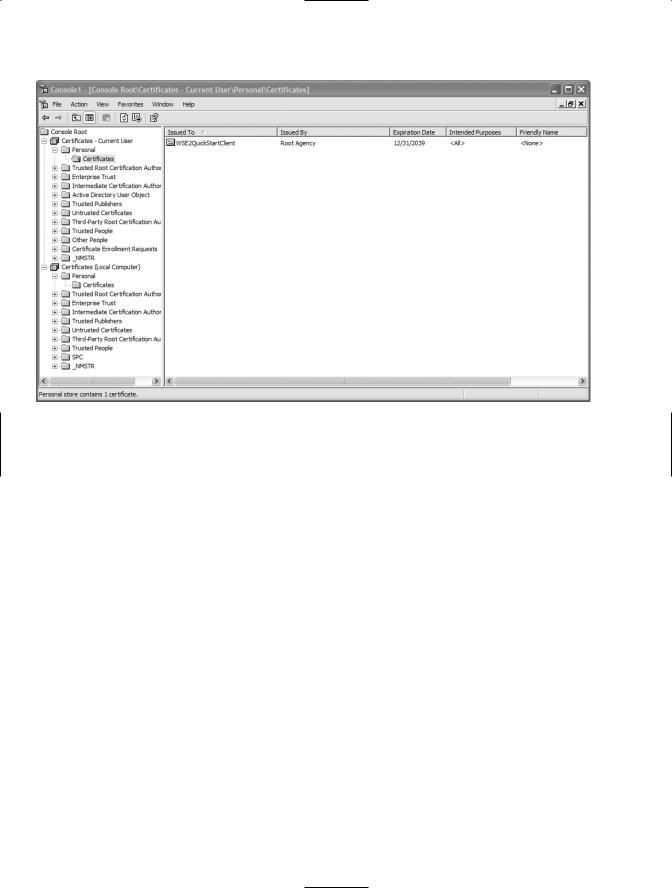
102 C H A P T E R 5 ■ W E B S E R V I C E S E N H A N C E M E N T S 3 . 0
Figure 5-4. MMC console displaying the Current User and the Local Computer certificate stores
4.Expand the Personal folder of the Current User certificate store and then right-click it to select the All Tasks Import menu option. Import the sample personal information exchange file titled Client Private.pfx. The sample certificates and private keys are installed with WSE 3.0, and their default location is C:\Program Files\Microsoft WSE\v3.0\Samples\Sample Test Certificates\. Client Private.pfx is the private key that the Web service client will use to encrypt requests to the Web service. Note that you will be prompted to enter a password for the private key during the import. For the WSE 3.0 test certificates, you can locate this password in a file called readme.htm, which is located in the same folder as the test certificates.
5.Right-click again the Personal folder of the Current User certificate store and select the All Tasks Import menu option. Import the sample test certificate titled Server Public.cer. This is the public key that the client uses to digitally sign requests for the Web service.
6.Expand the Personal folder of the Local Computer certificate store and import the sample test certificate titled Server Public.cer. This is the public key that the Web service uses to decrypt the client’s request.
This completes the installation of the certificates. But in order to use them from within ASP.NET, you will need to adjust permission levels for the ASP.NET worker process.
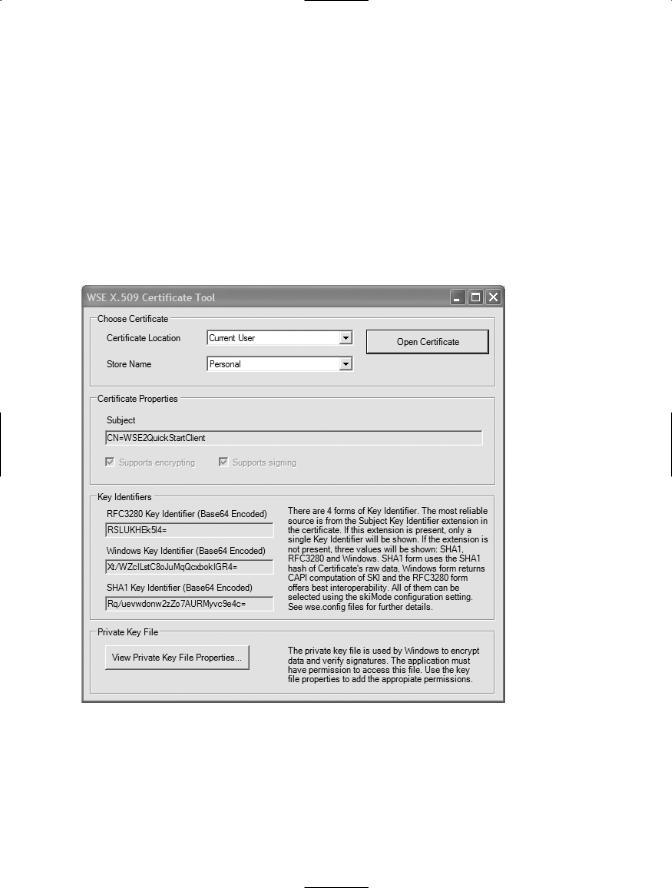
C H A P T E R 5 ■ W E B S E R V I C E S E N H A N C E M E N T S 3 . 0 |
103 |
Set ASP.NET Permissions to Use the X.509 Certificates
WSE 3.0 ships with a useful utility called the X.509 Certificate Tool. You can use this tool for several purposes:
•Browse installed certificates in the Current User and Local Computer certificate stores.
•Set permissions on the keys in the MachineKeys folder, which provides access to Local Computer certificates.
•Retrieve the base64 key identifier for installed certificate keys.
Figure 5-5 shows the X.509 Certificate Tool with a selected certificate, which in this case is the private key certificate for the Local Computer user.
Figure 5-5. The WSE X.509 Certificate Tool
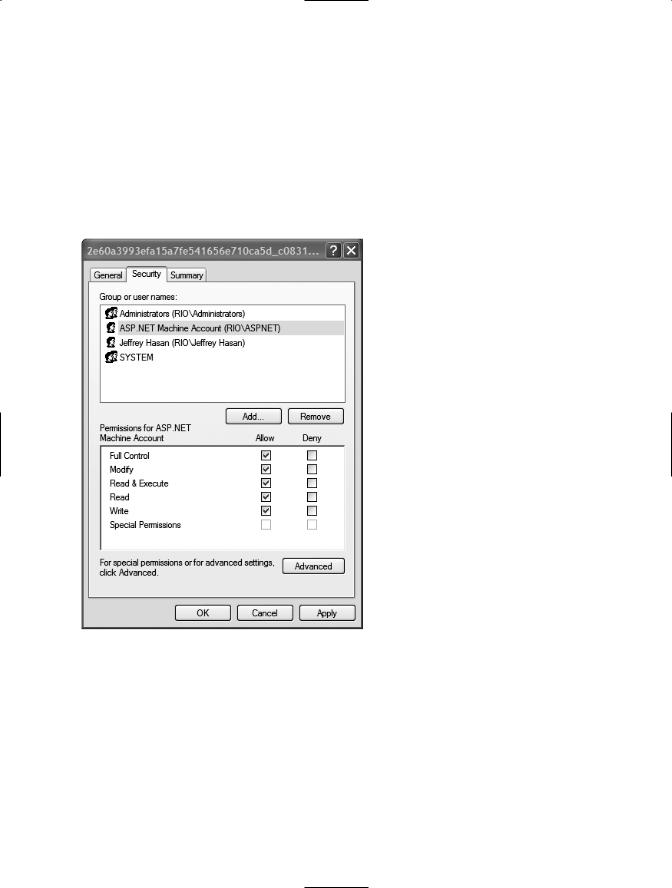
104 C H A P T E R 5 ■ W E B S E R V I C E S E N H A N C E M E N T S 3 . 0
The ASP.NET worker process needs Full Control security-level access to the folder that stores the Local Computer certificates. Click the lower button in the X.509 Certificate Tool page that is labeled View Private Key File Properties to open property pages for the folder.
Switch to the Security tab to display the list of users who have access to the folder. Add the account that is assigned to the ASP.NET worker process and give it Full Control permissions. By default, on Windows XP/2000 under IIS 5.0, the worker process runs under a machine account called ASP.NET. On Windows Server 2003 the worker process usually runs under the NETWORK SERVICE account. Figure 5-6 shows what the Security tab looks like once you have added the ASP.NET worker process account.
Figure 5-6. Security settings for the folder that stores the Local Computer certificates and keys
The X.509 Certificate Tool provides the base64-encoded key identifier for the certificate. You will need this identifier in the code listings in order to retrieve the correct certificate. Listing 5-7 shows you how to retrieve a certificate from the certificate store using its key identifier.
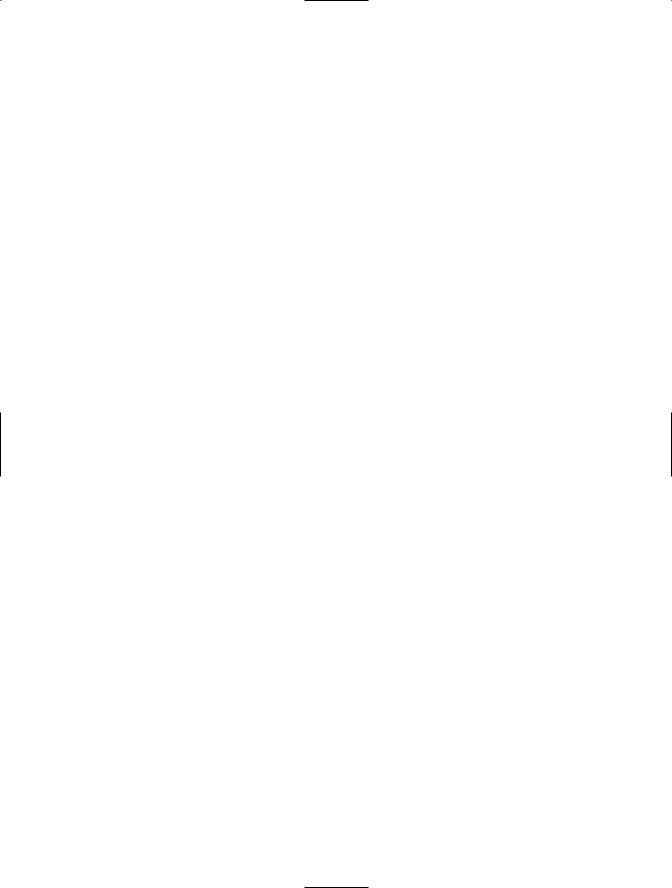
C H A P T E R 5 ■ W E B S E R V I C E S E N H A N C E M E N T S 3 . 0 |
105 |
Listing 5-7. Retrieving a Certificate from the Local Computer Certificate Store Using Its Key Identifier
using Microsoft.Web.Services3.Security.X509;
private X509SecurityToken GetSigningToken()
{
//NOTE: If you use the WSE 3.0 sample certificates then
//you should not need to change these IDs
string ClientBase64KeyId = "Xt/WZcILstC8oJuMqQcxbokIGR4=";
X509SecurityToken token = null;
// Open the CurrentUser Certificate Store X509CertificateStore store;
store = X509CertificateStore.CurrentUserStore( X509CertificateStore.MyStore ); if ( store.OpenRead() )
{
X509CertificateCollection certs = store.FindCertificateByKeyIdentifier( Convert.FromBase64String( ClientBase64KeyId ) );
if (certs.Count > 0)
{
// Get the first certificate in the collection
token = new X509SecurityToken( ((X509Certificate) certs[0]) );
}
}
return token;
}
Certificates require some effort to install and to configure, but it is well worth it. Certificates are easy to use once they are installed and you get a high level of security from asymmetric encryption compared to other methods. Asymmetric encryption does have the drawback of being more processor-intensive than other methods, so it can suffer in performance compared to other methods. But there are workarounds to this. For example, you can implement WS-Secure Conversation, which optimizes the performance of encrypted communication between a Web service and client. WS-Secure Conversation is covered in Chapter 7. Finally, you will learn a lot more about using certificates in your solutions by reading Chapter 6, which focuses on the WS-Security specification.