
- •About the Author
- •Dedication
- •Author’s Acknowledgments
- •Contents at a Glance
- •Table of Contents
- •Introduction
- •Who Should Buy This Book
- •How This Book Is Organized
- •Part I: Programming a Computer
- •Part II: Learning Programming with Liberty BASIC
- •Part III: Advanced Programming with Liberty BASIC
- •Part VI: Internet Programming
- •Part VII: The Part of Tens
- •How to Use This Book
- •Foolish assumptions
- •Icons used in this book
- •Why Learn Computer Programming?
- •How Does a Computer Program Work?
- •What Do I Need to Know to Program a Computer?
- •The joy of assembly language
- •C: The portable assembler
- •High-level programming languages
- •Database programming languages
- •Scripting programming languages
- •The program’s users
- •The target computer
- •Prototyping
- •Choosing a programming language
- •Defining how the program should work
- •The Life Cycle of a Typical Program
- •The development cycle
- •The maintenance cycle
- •The upgrade cycle
- •Writing Programs in an Editor
- •Using a Compiler or an Interpreter
- •Compilers
- •Interpreters
- •P-code: A combination compiler and interpreter
- •So what do I use?
- •Squashing Bugs with a Debugger
- •Writing a Help File
- •Creating an Installation Program
- •Why Learn Liberty BASIC?
- •Liberty BASIC is easy
- •Liberty BASIC runs on Windows
- •You can start using Liberty BASIC today
- •Installing Liberty BASIC
- •Loading Liberty BASIC
- •Your First Liberty BASIC Program
- •Running a Liberty BASIC program
- •Saving a Liberty BASIC program
- •Getting Help Using Liberty BASIC
- •Exiting Liberty BASIC
- •Getting input
- •Displaying output
- •Sending Data to the Printer
- •Storing Data in Variables
- •Creating a variable
- •Assigning a value to a variable
- •Declaring your variables
- •Using Constants
- •Commenting Your Code
- •Using variables
- •Working with precedence
- •Using parentheses
- •Manipulating Strings
- •Declaring variables as strings
- •Smashing strings together
- •Counting the length of a string
- •Playing with UPPERCASE and lowercase
- •Trimming the front and back of a string
- •Inserting spaces
- •Yanking characters out of a string
- •Looking for a string inside another string
- •Using Boolean Expressions
- •Using variables in Boolean expressions
- •Using Boolean operators
- •Exploring IF THEN Statements
- •IF THEN ELSE statements
- •Working with SELECT CASE Statements
- •Checking a range of values
- •Checking a relational operator
- •Boolean expression inside the loop
- •Looping a Fixed Number of Times
- •Counting with different numbers
- •Counting in increments
- •Anatomy of a Computer Bug
- •Syntax Errors
- •Fun with Logic Errors
- •Stepping line by line
- •Tracing through your program
- •Designing a Window
- •Creating a new window
- •Defining the size and location of a window
- •Adding color to a window
- •Putting Controls in a Window
- •Creating a command button
- •Displaying text
- •Creating a check box
- •Creating a radio button
- •Creating text boxes
- •Creating list boxes
- •Creating combo boxes
- •Creating group boxes
- •Storing Stuff in Text Files
- •Creating a new text file
- •Putting stuff in a text file
- •Adding new stuff to an existing text file
- •Retrieving data from a text file
- •Creating a new binary file
- •Saving stuff in a binary file
- •Changing stuff in a binary file
- •Retrieving stuff from a binary file
- •Creating a Graphics Control
- •Using Turtle Graphics
- •Defining line thickness
- •Defining line colors
- •Drawing Circles
- •Drawing Boxes
- •Displaying Text
- •Making Sounds
- •Making a beeping noise
- •Playing WAV files
- •Passing Data by Value or by Reference
- •Using Functions
- •Defining a function
- •Passing data to a function
- •Calling a function
- •Exiting prematurely from a function
- •Using Subroutines
- •Defining a subroutine
- •Passing data to a subroutine
- •Calling a subroutine
- •Exiting prematurely from a subroutine
- •Writing Modular Programs
- •Introducing Structured Programming
- •Sequential instructions
- •Branching instructions
- •Looping instructions
- •Putting structured programming into practice
- •The Problem with Software
- •Ways to Make Programming Easier
- •Breaking Programs into Objects
- •How to use objects
- •How to create an object
- •Creating an object
- •Starting with a Pointer
- •Defining the parts of a linked list
- •Creating a linked list
- •Managing a linked list
- •Making Data Structures with Linked Lists
- •Stacks
- •Queues
- •Trees
- •Graphs
- •Creating a Record
- •Manipulating Data in Records
- •Storing data in a record
- •Retrieving data from a record
- •Using Records with Arrays
- •Making an Array
- •Making a Multidimensional Array
- •Creating Dynamic Arrays
- •Insertion Sort
- •Bubble Sort
- •Shell Sort
- •Quicksort
- •Sorting Algorithms
- •Searching Sequentially
- •Performing a Binary Search
- •Hashing
- •Searching by using a hash function
- •Dealing with collisions
- •Picking a Searching Algorithm
- •Choosing the Right Data Structure
- •Choosing the Right Algorithm
- •Put the condition most likely to be false first
- •Put the condition most likely to be true first
- •Clean out your loops
- •Use the correct data types
- •Using a Faster Language
- •Optimizing Your Compiler
- •Programming Computer Games
- •Creating Computer Animation
- •Making (And Breaking) Encryption
- •Internet Programming
- •Fighting Computer Viruses and Worms
- •Hacking for Hire
- •Participating in an Open-Source Project
- •Niche-Market Programming
- •Teaching Others about Computers
- •Selling Your Own Software
- •Trying Commercial Compilers
- •Windows programming
- •Macintosh and Palm OS programming
- •Linux programming
- •Testing the Shareware and
- •BASIC compilers
- •C/C++ and Java compilers
- •Pascal compilers
- •Using a Proprietary Language
- •HyperCard
- •Revolution
- •PowerBuilder
- •Shopping by Mail Order
- •Getting Your Hands on Source Code
- •Joining a Local User Group
- •Frequenting Usenet Newsgroups
- •Playing Core War
- •Programming a Battling Robot
- •Toying with Lego Mindstorms
- •Index
- •End-User License Agreement

150 Part III: Advanced Programming with Liberty BASIC
In this example, the main program instructions start with line one (NOMAINWIN) and stop with line nine (END). The subprogram, [hackeralert], starts on line 11 ([hacker alert]) and stops on line 18 (RETURN). The only time that the [hackeralert] subprogram runs is if you type an incorrect password in the first Prompt dialog box that asks, What is your password?
Ideally, you want to make all your subprograms small enough to fit on a single screen. The smaller your subprograms are, the easier they are to understand, debug, and modify.
If you use subprograms in a Liberty BASIC program, you’re essentially dividing a larger program into smaller parts, although you still save everything into a single file with a name such as PACMAN.BAS or BLACKJACK.BAS.
Be aware that programming languages often use similar terms to represent different items. If you divide a large program into smaller parts, for example, BASIC calls these smaller parts subprograms. But what BASIC calls a subprogram, the C language calls a function. So if you use a different language, make sure that you use the correct terms for that particular language; otherwise, you may get confused in talking to other programmers.
Using Subroutines
In Liberty BASIC, you can create subprograms, which are miniature programs that perform a specific task. Unfortunately, subprograms work exactly the same every time that they run.
For more flexibility, you may want to create subprograms that can accept data and calculate a new result, basing it on the data that it receives. You may, for example, want to create a subprogram that multiplies two numbers together. In most cases, you don’t want to multiply the same two numbers every time that the subprogram runs. Instead, the subprogram is more useful if you can feed it a different number each time and have the subprogram spit back an answer based on the number that it receives.
In most programming languages such as C/C++, Java, or Pascal, you can create the following two different types of subprograms:
Subroutines: These types of subprograms contain instructions that accept data and use that data for performing certain tasks, such as verifying that the user types a correct password or moving the cursor as the user moves the mouse.

Chapter 11: Writing Large Programs by Using Subprograms 151
Functions: These types of subprograms calculate and return a single value, such as calculating the cube of a number or counting the number of words in a sentence. In Liberty BASIC, the COS(x) and SIN(x) commands are examples of a function.
Defining a subroutine
A subroutine consists of the following two or three parts:
The subroutine’s name
One or more instructions that you want the subroutine to follow
Any data that you want the subroutine to use (optional)
In Liberty BASIC, a subroutine looks as follows:
SUB SubroutineName Data
‘ One or more instructions
END SUB
If a subroutine doesn’t need to accept data from the main program or another subprogram, you can omit the Data portion of the subroutine, as in the following example:
SUB SubroutineName
‘ One or more instructions
END SUB
Passing data to a subroutine
A subroutine acts like a miniature program that performs one or more tasks. Although a subroutine is part of a bigger program, some subroutines can act independently from the rest of the program. You may, for example, have a subroutine that does nothing but display a message on-screen. If a subroutine can act independently from the rest of the program, you need to define only a name for your subroutine and any instructions that you want the subroutine to follow, as in the following example:
SUB SubroutineName
PRINT “This subroutine doesn’t use any data.” END SUB
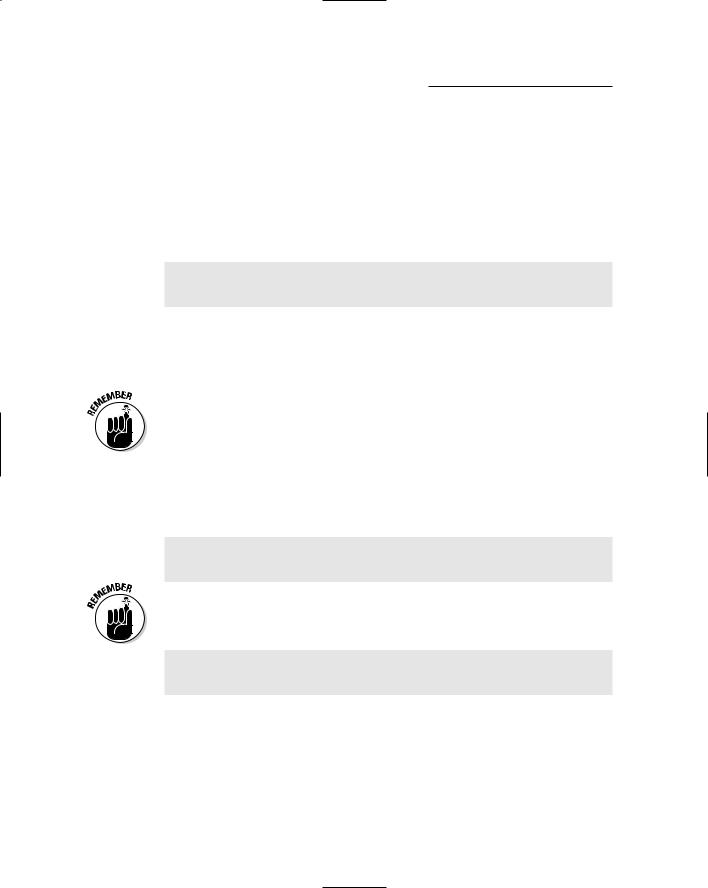
152 Part III: Advanced Programming with Liberty BASIC
The preceding example of a subroutine is similar to a subprogram in Liberty BASIC. As a program runs the preceding subroutine, the subroutine merrily follows its instructions without using any data from any other part of the program.
But in many cases, a subroutine requires outside data to accomplish a given task, such as checking to see whether the user types a valid password. If your subroutine needs data from another part of your program, you need to create a variable to store this data, as in the following example:
SUB SubroutineName Variable
‘ One or more instructions here
END SUB
As another part of the program tells this subroutine to run, it “passes” data to this subroutine, in much the same way that a person may pass a baton to another person. After a subroutine receives data that another part of the program passes to it, the subroutine uses variables (which it traps inside parentheses) to “hold” any data that another part of the program sends to it.
The list of variables, which accepts outside data for a subroutine, is known as a parameter list.
Each variable inside a subroutine’s parameter list specifies one chunk of data, such as a number or string. If another part of the program passes your subroutine two chunks of data, the subroutine’s parameter list must contain exactly two variables to hold this data. The following subroutine example uses three variables in its parameter list:
SUB SubroutineName Variable1, Variable2, Variable3 ‘ One or more instructions here
END SUB
Make sure that you declare string variables by using the dollar sign ($) and number variables without the dollar sign. The following example uses a string and a number variable in its parameter list:
SUB SubroutineName Name$, Age
‘ One or more instructions here
END SUB
The first line of the preceding subroutine defines the name of the subroutine (which is SubroutineName) and creates two variables, Name$ and Age. The Name$ variable represents a string, and the Age variable represents an integer.

Chapter 11: Writing Large Programs by Using Subprograms 153
Calling a subroutine
After you create a subroutine, the final step is to actually run the instructions inside the subroutine. Normally, the subroutine sits around and does absolutely nothing (much like a politician) until the computer specifically tells the subroutine to run its instructions. In technical terms, as you tell the subroutine to run its instructions, you’re calling a subroutine. Essentially, you’re saying, “Hey, stupid subroutine! Start running your instructions now!”
If you want to run the subroutine BurnOutMonitor, you must use the CALL command. Many versions of BASIC enable you to use one of the following two methods:
CALL BurnOutMonitor
If the BurnOutMonitor subroutine needs you to pass data to it for it to run, you just tack on the data to pass to the subroutine as follows:
CALL BurnOutMonitor 45
If you use the CALL command, make sure that you spell your subroutine correctly, including upper and lowercase letters. Liberty BASIC assumes that a subroutine by the name of DisplayMessage is completely different from a subroutine with the name displaymessage.
To help you understand subroutines better, try the following Liberty BASIC program. Notice that the subroutine appears after the END command. This program asks for a name (such as Bill McPherson) and then displays that name to a Notice dialog box by using either the string Bill McPherson must be a moron or Bill McPherson sounds like an idiot to me.
NOMAINWIN
PROMPT “Give me the name of someone you hate:”; enemy$
CALL DisplayMessage enemy$
END
SUB DisplayMessage stuff$
X = INT(RND(1) * 2) + 1
IF X = 1 THEN
NOTICE stuff$ + “ must be a moron.”
ELSE
NOTICE stuff$ + “ sounds like an idiot to me.”
END IF
END SUB