
- •About the Author
- •Dedication
- •Author’s Acknowledgments
- •Contents at a Glance
- •Table of Contents
- •Introduction
- •Who Should Buy This Book
- •How This Book Is Organized
- •Part I: Programming a Computer
- •Part II: Learning Programming with Liberty BASIC
- •Part III: Advanced Programming with Liberty BASIC
- •Part VI: Internet Programming
- •Part VII: The Part of Tens
- •How to Use This Book
- •Foolish assumptions
- •Icons used in this book
- •Why Learn Computer Programming?
- •How Does a Computer Program Work?
- •What Do I Need to Know to Program a Computer?
- •The joy of assembly language
- •C: The portable assembler
- •High-level programming languages
- •Database programming languages
- •Scripting programming languages
- •The program’s users
- •The target computer
- •Prototyping
- •Choosing a programming language
- •Defining how the program should work
- •The Life Cycle of a Typical Program
- •The development cycle
- •The maintenance cycle
- •The upgrade cycle
- •Writing Programs in an Editor
- •Using a Compiler or an Interpreter
- •Compilers
- •Interpreters
- •P-code: A combination compiler and interpreter
- •So what do I use?
- •Squashing Bugs with a Debugger
- •Writing a Help File
- •Creating an Installation Program
- •Why Learn Liberty BASIC?
- •Liberty BASIC is easy
- •Liberty BASIC runs on Windows
- •You can start using Liberty BASIC today
- •Installing Liberty BASIC
- •Loading Liberty BASIC
- •Your First Liberty BASIC Program
- •Running a Liberty BASIC program
- •Saving a Liberty BASIC program
- •Getting Help Using Liberty BASIC
- •Exiting Liberty BASIC
- •Getting input
- •Displaying output
- •Sending Data to the Printer
- •Storing Data in Variables
- •Creating a variable
- •Assigning a value to a variable
- •Declaring your variables
- •Using Constants
- •Commenting Your Code
- •Using variables
- •Working with precedence
- •Using parentheses
- •Manipulating Strings
- •Declaring variables as strings
- •Smashing strings together
- •Counting the length of a string
- •Playing with UPPERCASE and lowercase
- •Trimming the front and back of a string
- •Inserting spaces
- •Yanking characters out of a string
- •Looking for a string inside another string
- •Using Boolean Expressions
- •Using variables in Boolean expressions
- •Using Boolean operators
- •Exploring IF THEN Statements
- •IF THEN ELSE statements
- •Working with SELECT CASE Statements
- •Checking a range of values
- •Checking a relational operator
- •Boolean expression inside the loop
- •Looping a Fixed Number of Times
- •Counting with different numbers
- •Counting in increments
- •Anatomy of a Computer Bug
- •Syntax Errors
- •Fun with Logic Errors
- •Stepping line by line
- •Tracing through your program
- •Designing a Window
- •Creating a new window
- •Defining the size and location of a window
- •Adding color to a window
- •Putting Controls in a Window
- •Creating a command button
- •Displaying text
- •Creating a check box
- •Creating a radio button
- •Creating text boxes
- •Creating list boxes
- •Creating combo boxes
- •Creating group boxes
- •Storing Stuff in Text Files
- •Creating a new text file
- •Putting stuff in a text file
- •Adding new stuff to an existing text file
- •Retrieving data from a text file
- •Creating a new binary file
- •Saving stuff in a binary file
- •Changing stuff in a binary file
- •Retrieving stuff from a binary file
- •Creating a Graphics Control
- •Using Turtle Graphics
- •Defining line thickness
- •Defining line colors
- •Drawing Circles
- •Drawing Boxes
- •Displaying Text
- •Making Sounds
- •Making a beeping noise
- •Playing WAV files
- •Passing Data by Value or by Reference
- •Using Functions
- •Defining a function
- •Passing data to a function
- •Calling a function
- •Exiting prematurely from a function
- •Using Subroutines
- •Defining a subroutine
- •Passing data to a subroutine
- •Calling a subroutine
- •Exiting prematurely from a subroutine
- •Writing Modular Programs
- •Introducing Structured Programming
- •Sequential instructions
- •Branching instructions
- •Looping instructions
- •Putting structured programming into practice
- •The Problem with Software
- •Ways to Make Programming Easier
- •Breaking Programs into Objects
- •How to use objects
- •How to create an object
- •Creating an object
- •Starting with a Pointer
- •Defining the parts of a linked list
- •Creating a linked list
- •Managing a linked list
- •Making Data Structures with Linked Lists
- •Stacks
- •Queues
- •Trees
- •Graphs
- •Creating a Record
- •Manipulating Data in Records
- •Storing data in a record
- •Retrieving data from a record
- •Using Records with Arrays
- •Making an Array
- •Making a Multidimensional Array
- •Creating Dynamic Arrays
- •Insertion Sort
- •Bubble Sort
- •Shell Sort
- •Quicksort
- •Sorting Algorithms
- •Searching Sequentially
- •Performing a Binary Search
- •Hashing
- •Searching by using a hash function
- •Dealing with collisions
- •Picking a Searching Algorithm
- •Choosing the Right Data Structure
- •Choosing the Right Algorithm
- •Put the condition most likely to be false first
- •Put the condition most likely to be true first
- •Clean out your loops
- •Use the correct data types
- •Using a Faster Language
- •Optimizing Your Compiler
- •Programming Computer Games
- •Creating Computer Animation
- •Making (And Breaking) Encryption
- •Internet Programming
- •Fighting Computer Viruses and Worms
- •Hacking for Hire
- •Participating in an Open-Source Project
- •Niche-Market Programming
- •Teaching Others about Computers
- •Selling Your Own Software
- •Trying Commercial Compilers
- •Windows programming
- •Macintosh and Palm OS programming
- •Linux programming
- •Testing the Shareware and
- •BASIC compilers
- •C/C++ and Java compilers
- •Pascal compilers
- •Using a Proprietary Language
- •HyperCard
- •Revolution
- •PowerBuilder
- •Shopping by Mail Order
- •Getting Your Hands on Source Code
- •Joining a Local User Group
- •Frequenting Usenet Newsgroups
- •Playing Core War
- •Programming a Battling Robot
- •Toying with Lego Mindstorms
- •Index
- •End-User License Agreement

186 Part III: Advanced Programming with Liberty BASIC
Saving and Retrieving Data
in a Binary File
Text files can be convenient for storing long strings of information, but they can be painfully cumbersome in retrieving data. Random-access files make retrieving data simple but at the expense of having to organize every chunk of data into records. In case you want to store long bits of information with the convenience of retrieving them quickly, you can save data in a binary file.
Creating a new binary file
Before you can store any data in a binary file, you must first create that binary file. To create a binary file, you use the following Liberty BASIC command:
OPEN “Filename” FOR BINARY AS #Handle
This is what the above Liberty BASIC command tells the computer:
1.The OPEN command tells the computer, “Create a new file and give it the name that “Filename” specifies, which can represent a filename (such as STUFF.BIN) or a drive, directory, and filename (such as C:\WINDOWS\ STUFF.BIN).”
2.The FOR BINARY portion of the command tells the computer, “Get ready to start outputting data into the newly created binary file that “Filename” identifies.”
3.The #Handle is any nickname that you choose to represent the binary file you just created.
If you want to create a file called STUFF.BIN and save it on a floppy disk (the A drive), use the following command:
OPEN “A:\STUFF.BIN” FOR BINARY AS #Secrets
This line tells Liberty BASIC to create the binary file STUFF.BIN on the A drive and assign it the name #Secrets.
Any time that you use the OPEN command to create a new binary file or to open an existing binary file, make sure you use the CLOSE command to shut the binary file. If you don’t use the CLOSE command eventually, your program may cause the computer to crash.
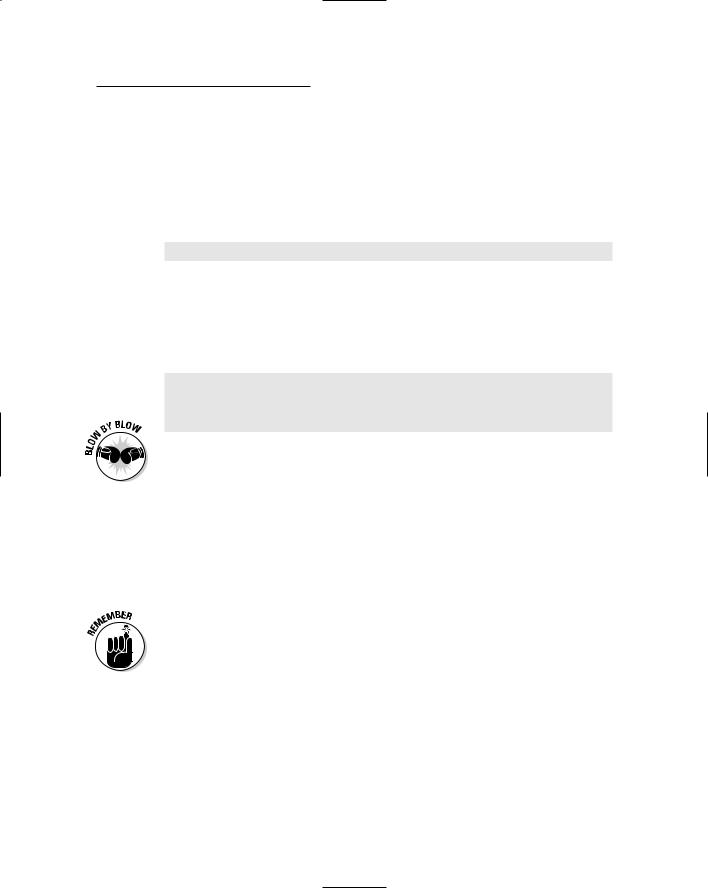
Chapter 13: Saving and Retrieving Stuff in Files 187
Saving stuff in a binary file
After you create a binary file, you can stuff it with data by using the PRINT command. If you use the PRINT command, Liberty BASIC normally displays that data on-screen, so you must tell Liberty BASIC to store the data in your binary file instead by using the handle of that binary file, as in the following command:
PRINT #Secrets, “This line gets stored in the binary file.”
This command tells Liberty BASIC, “Look for a binary file that the name #Secrets identifies and stuff that binary file with the line, This line gets stored in the binary file.”
Putting it all together, you can create a program similar to the following example:
OPEN “A:\STUFF.BIN” FOR BINARY AS #Secrets
PRINT #Secrets, “This line gets stored in the binary file.”
CLOSE #Secrets
END
This Liberty BASIC program tells the computer to do the following:
1.The first line tells the computer, “Open a binary file on the A drive and call this binary file STUFF.BIN. Then give it the handle #Secrets.”
2.The second line tells the computer, “Look for a file that the #Secrets handle identifies and stuff it with the line, This line gets stored in the binary file.”
3.The third line tells the computer, “Close the binary file that the #Secrets handle identifies.”
4.The fourth line tells the computer that the program is at an end.
If you run this program, it simply creates a binary file on your disk but you won’t see anything on the screen.
Changing stuff in a binary file
When you store data in a binary file, you can overwrite that data at any time by using something magical called a file pointer. Because binary files store data as a continuous stream of bytes, the file pointer lets you point at the part of the file that contains the data you want to overwrite.

188 Part III: Advanced Programming with Liberty BASIC
To find the current location of the file pointer, you have to create a variable and assign it the value of the file pointer’s position with the LOC command such as:
FilePointer = LOC(#Filename)
So if #Filename represents a binary file that contains the string, “I love programming with Liberty BASIC,” the value of the FilePointer variable initially would be 38 (the total number of characters in the “I love programming with Liberty BASIC” string).
To move the file pointer, you have to use the SEEK command, such as:
SEEK(#Filename), NewPosition
This command tells Liberty BASIC, “Move the file pointer to the location specified by NewPosition.” So if the value of NewPosition is 3, the file pointer will now point to the third character stored in the binary file. If you stored the string “I hate copycat publishers,” in a binary file, the third position would point to the letter h, which is the first letter of the word “hate”.
To overwrite data in a binary file, you just have to move the file pointer to the location where you want to overwrite the data and then use the ordinary PRINT command such as:
SEEK(#MyStuff), 3
PRINT #MyStuff, “despise copycat publishers.”
If the #MyStuff handle represented a binary file that contained the string, “I hate copycat publishers,” then the above two commands would first move the file pointer to the third position and then overwrite the third position and the rest of the file with the string “despise copycat publishers.”
Be careful when overwriting data in a binary file. Say you have the following string stored in a binary file, “I like cats,” and use the following code:
SEEK(#MyStuff), 1
PRINT #MyStuff, “‘m tired.”
This is what your original binary file contains:
I like cats.
And this is what the overwritten binary file contents now look like:
I’m tired.s.

Chapter 13: Saving and Retrieving Stuff in Files 189
Note that the extra s and period remain because the new string doesn’t completely overwrite the old data in the binary file.
Retrieving stuff from a binary file
Storing data in a binary file is nice, but eventually you may want to retrieve that data out again. To retrieve data from a binary file, you have to move the file pointer to anywhere in the file where you want to start retrieving data, then use the INPUT command such as:
SEEK #MyFile, 0
INPUT #MyFile, RetrieveMe$
In this example, the SEEK #MyFile, 0 command simply moves the file pointer to the beginning of the binary file. If you only wanted to retrieve part of the data in a binary file, you could move the file pointer to a different location such as 8 or 12. #MyFile represents the file handle that you previously used with the OPEN command and RetrieveMe$ is the name of a string variable that temporarily stores the string you stored in that binary file.
If you’re retrieving numeric data stored in a binary file, you would use a numeric variable name that doesn’t have the dollar sign ($) after it, such as:
INPUT #MyFile, RetrieveMe
To see how to store, overwrite and retrieve data in a binary file, take a look at this example:
OPEN “STUFF.BIN” FOR BINARY AS #myfile
PRINT #myfile, “Welcome back home!”
SEEK #myfile, 0
INPUT #myfile, txt$
PRINT “Current Data in binary file: “; txt$
SEEK #myfile, 8
PRINT #myfile, “to my world!”
SEEK #myfile, 0
INPUT #myfile, txt$
PRINT “New Data in binary file: “;txt$
CLOSE #myfile
END
Here’s what’s happening in the preceding example:
1.The first line tells the computer, “Open up the binary file STUFF.BIN, assign it a file handle of #myfile.”

190 Part III: Advanced Programming with Liberty BASIC
2.The second line tells the computer to store the string, “Welcome back home!” in the binary file.
3.The third line tells the computer to move the file pointer to the beginning of the binary file.
4.The fourth line tells the computer, “Read all the data out of the binary file represented by the #myfile handle and store the result in a variable called txt$.”
5.The fifth line tells the computer, “Print the current contents of the binary file in the main window, which means Liberty BASIC prints the string, “Welcome back home!””
6.The sixth line tells the computer, “Move the file pointer to the eight position in the binary file.”
7.The seventh line tells the computer, “Starting with the eighth position in the binary file, overwrite the data and replace it with the string, “to my world!””
8.The eighth line tells the computer, “Move the file pointer back to the front of the binary file.”
9.The ninth line tells the computer, “Read all the data from the binary file represented by the handle #myfile, and store it in a variable called txt$.”
10.The tenth line tells the computer, “Print the contents of the binary file in the main window.”
11.The eleventh line tells the computer, “Close the binary file represented by the #myfile handle.”
12.The twelfth line tells the computer, “This is the end of the program. Now it’s safe for Microsoft Windows to crash without losing any of my valuable data.”