
- •About the Author
- •Dedication
- •Author’s Acknowledgments
- •Contents at a Glance
- •Table of Contents
- •Introduction
- •Who Should Buy This Book
- •How This Book Is Organized
- •Part I: Programming a Computer
- •Part II: Learning Programming with Liberty BASIC
- •Part III: Advanced Programming with Liberty BASIC
- •Part VI: Internet Programming
- •Part VII: The Part of Tens
- •How to Use This Book
- •Foolish assumptions
- •Icons used in this book
- •Why Learn Computer Programming?
- •How Does a Computer Program Work?
- •What Do I Need to Know to Program a Computer?
- •The joy of assembly language
- •C: The portable assembler
- •High-level programming languages
- •Database programming languages
- •Scripting programming languages
- •The program’s users
- •The target computer
- •Prototyping
- •Choosing a programming language
- •Defining how the program should work
- •The Life Cycle of a Typical Program
- •The development cycle
- •The maintenance cycle
- •The upgrade cycle
- •Writing Programs in an Editor
- •Using a Compiler or an Interpreter
- •Compilers
- •Interpreters
- •P-code: A combination compiler and interpreter
- •So what do I use?
- •Squashing Bugs with a Debugger
- •Writing a Help File
- •Creating an Installation Program
- •Why Learn Liberty BASIC?
- •Liberty BASIC is easy
- •Liberty BASIC runs on Windows
- •You can start using Liberty BASIC today
- •Installing Liberty BASIC
- •Loading Liberty BASIC
- •Your First Liberty BASIC Program
- •Running a Liberty BASIC program
- •Saving a Liberty BASIC program
- •Getting Help Using Liberty BASIC
- •Exiting Liberty BASIC
- •Getting input
- •Displaying output
- •Sending Data to the Printer
- •Storing Data in Variables
- •Creating a variable
- •Assigning a value to a variable
- •Declaring your variables
- •Using Constants
- •Commenting Your Code
- •Using variables
- •Working with precedence
- •Using parentheses
- •Manipulating Strings
- •Declaring variables as strings
- •Smashing strings together
- •Counting the length of a string
- •Playing with UPPERCASE and lowercase
- •Trimming the front and back of a string
- •Inserting spaces
- •Yanking characters out of a string
- •Looking for a string inside another string
- •Using Boolean Expressions
- •Using variables in Boolean expressions
- •Using Boolean operators
- •Exploring IF THEN Statements
- •IF THEN ELSE statements
- •Working with SELECT CASE Statements
- •Checking a range of values
- •Checking a relational operator
- •Boolean expression inside the loop
- •Looping a Fixed Number of Times
- •Counting with different numbers
- •Counting in increments
- •Anatomy of a Computer Bug
- •Syntax Errors
- •Fun with Logic Errors
- •Stepping line by line
- •Tracing through your program
- •Designing a Window
- •Creating a new window
- •Defining the size and location of a window
- •Adding color to a window
- •Putting Controls in a Window
- •Creating a command button
- •Displaying text
- •Creating a check box
- •Creating a radio button
- •Creating text boxes
- •Creating list boxes
- •Creating combo boxes
- •Creating group boxes
- •Storing Stuff in Text Files
- •Creating a new text file
- •Putting stuff in a text file
- •Adding new stuff to an existing text file
- •Retrieving data from a text file
- •Creating a new binary file
- •Saving stuff in a binary file
- •Changing stuff in a binary file
- •Retrieving stuff from a binary file
- •Creating a Graphics Control
- •Using Turtle Graphics
- •Defining line thickness
- •Defining line colors
- •Drawing Circles
- •Drawing Boxes
- •Displaying Text
- •Making Sounds
- •Making a beeping noise
- •Playing WAV files
- •Passing Data by Value or by Reference
- •Using Functions
- •Defining a function
- •Passing data to a function
- •Calling a function
- •Exiting prematurely from a function
- •Using Subroutines
- •Defining a subroutine
- •Passing data to a subroutine
- •Calling a subroutine
- •Exiting prematurely from a subroutine
- •Writing Modular Programs
- •Introducing Structured Programming
- •Sequential instructions
- •Branching instructions
- •Looping instructions
- •Putting structured programming into practice
- •The Problem with Software
- •Ways to Make Programming Easier
- •Breaking Programs into Objects
- •How to use objects
- •How to create an object
- •Creating an object
- •Starting with a Pointer
- •Defining the parts of a linked list
- •Creating a linked list
- •Managing a linked list
- •Making Data Structures with Linked Lists
- •Stacks
- •Queues
- •Trees
- •Graphs
- •Creating a Record
- •Manipulating Data in Records
- •Storing data in a record
- •Retrieving data from a record
- •Using Records with Arrays
- •Making an Array
- •Making a Multidimensional Array
- •Creating Dynamic Arrays
- •Insertion Sort
- •Bubble Sort
- •Shell Sort
- •Quicksort
- •Sorting Algorithms
- •Searching Sequentially
- •Performing a Binary Search
- •Hashing
- •Searching by using a hash function
- •Dealing with collisions
- •Picking a Searching Algorithm
- •Choosing the Right Data Structure
- •Choosing the Right Algorithm
- •Put the condition most likely to be false first
- •Put the condition most likely to be true first
- •Clean out your loops
- •Use the correct data types
- •Using a Faster Language
- •Optimizing Your Compiler
- •Programming Computer Games
- •Creating Computer Animation
- •Making (And Breaking) Encryption
- •Internet Programming
- •Fighting Computer Viruses and Worms
- •Hacking for Hire
- •Participating in an Open-Source Project
- •Niche-Market Programming
- •Teaching Others about Computers
- •Selling Your Own Software
- •Trying Commercial Compilers
- •Windows programming
- •Macintosh and Palm OS programming
- •Linux programming
- •Testing the Shareware and
- •BASIC compilers
- •C/C++ and Java compilers
- •Pascal compilers
- •Using a Proprietary Language
- •HyperCard
- •Revolution
- •PowerBuilder
- •Shopping by Mail Order
- •Getting Your Hands on Source Code
- •Joining a Local User Group
- •Frequenting Usenet Newsgroups
- •Playing Core War
- •Programming a Battling Robot
- •Toying with Lego Mindstorms
- •Index
- •End-User License Agreement

Chapter 14
Creating a User Interface
In This Chapter
Creating a window
Adding menus to a window
Placing controls in a window
Using dialog boxes
As the name implies, a user interface acts as the middleman between the user and your program. A user gives commands through your program’s
user interface, which passes those commands to the part of your program that actually does the work. Then your program passes data back to the user interface to display on-screen for the user to see.
Designing a Window
The most common element of a Windows program is a window, which is nothing more than a rectangle that appears on-screen. A window has the following two purposes:
To display your program’s commands, such as pull-down menus or command buttons, so that the user can use them to make selections or input information into the program
To display information on-screen for the user to see, such as a graph of a stock price or text that the user types
Creating a new window
To create a window, you need to use the OPEN command and define the text that you want to appear in the window titlebar, as the following example shows:

192 Part III: Advanced Programming with Liberty BASIC
NOMAINWIN
OPEN “Titlebar text” FOR Window AS #1
PRINT #1, “trapclose [quit]”
WAIT
[quit]
CONFIRM “Are you sure that you want to quit?”; quit$ IF quit$ = “no” THEN WAIT
CLOSE #1 END
This program tells Liberty BASIC to do the following:
1.The first line tells Liberty BASIC not to display the main window.
2.The second line uses the OPEN command to create a new window, display the Titlebar text string in the title bar of the window, and assign the nickname #1 to represent the window that you just created.
3.The third line tells the computer to follow the instructions following the [quit] label if the user tries to close the program. This line uses the PRINT command to detect when the user tries to close the window identified by the #1 nickname, but doesn’t actually print anything on the screen.
4.The fourth line uses the WAIT command to tell your program to wait for the user to do something.
5.The fifth line is the [quit] label.
6.The sixth line displays a Confirm dialog box that asks, Are you sure that you want to quit? The CONFIRM command automatically displays a Yes and No command button in the dialog box. If the user clicks the Yes button, the program stores a value of “yes” in the quit$ variable. If the user clicks the No button, it stores a value of “no” in the quit$ variable.
7.The seventh line checks to see whether the value of the quit$ variable equals ”no.” If so, it runs the WAIT command to wait for the user to do something.
8.The eighth line closes the window that the nickname #1 identifies. This line runs only if the user clicks the Yes button in the Confirm dialog box that the sixth line displays.
9.The ninth line marks the end of the program.
When you run this program, a window appears on-screen and waits for you to click its close box before the program stops running.

Chapter 14: Creating a User Interface 193
Any time that you use the OPEN command to create a new window, you must eventually use the CLOSE command to shut the window. If you don’t use the CLOSE command eventually, your program may cause the computer to crash.
Defining the size and location of a window
Each time that you create a window, Liberty BASIC may display that window on a different part of the screen. If you want to specify the exact location where you want your window to appear, you can use the UpperLeftX and UpperLeftY commands, which define how far from the left (the X coordinate) and the top edge (the Y coordinate) the upper-left corner of your window appears on-screen, as shown in Figure 14-1. If you want your window to appear exactly 225 pixels from the top and 139 pixels from the left edge of the screen, for example, you can use the following program:
NOMAINWIN
UpperLeftX = 139
UpperLeftY = 225
OPEN “Titlebar” FOR Window AS #1
PRINT #1, “trapclose [quit]”
WAIT
[quit]
CONFIRM “Are you sure you want to quit?”; quit$ IF quit$ = “no” THEN WAIT
CLOSE #1 END
The UpperLeftX and UpperLeftY commands define how far the window appears from the left and top edges, respectively. If you want to define the exact size of your window, you can use the WindowHeight and WindowWidth commands to define a size measured in pixels, as follows:
NOMAINWIN
UpperLeftX = 139
UpperLeftY = 225
WindowWidth = 550
WindowHeight = 275
OPEN “Titlebar” FOR Window AS #1
PRINT #1, “trapclose [quit]”
WAIT
[quit]
CONFIRM “Are you sure you want to quit?”; quit$ IF quit$ = “no” THEN WAIT
CLOSE #1 END

194 Part III: Advanced Programming with Liberty BASIC
Title bar
Figure 14-1:
After you create a window, you can define text to appear in that window’s title bar, location on-screen, and the
window size.
You must define the size and location of a window before using the OPEN command that actually creates the window on-screen.
Adding color to a window
Liberty BASIC also enables you to choose the background color for your window by using the BackgroundColor$ command and to set its value to one of the following colors:
White |
Darkblue |
Black |
Red |
Lightgray (or Palegray) |
Darkred |
Darkgray |
Pink |
Yellow |
Darkpink |
Brown |
Green |
Blue |
Darkgreen |
Cyan |
Darkcyan |

Chapter 14: Creating a User Interface 195
If you want to create a window with a pink background, you can use the following commands:
NOMAINWIN
UpperLeftX = 139
UpperLeftY = 225
WindowWidth = 550
WindowHeight = 275
BackgroundColor$ = “Pink”
OPEN “Titlebar” FOR Window AS #1
PRINT #1, “trapclose [quit]”
WAIT
[quit]
CONFIRM “Are you sure you want to quit?”; quit$ IF quit$ = “no” THEN WAIT
CLOSE #1 END
Putting Pull-Down Menus in a Window
Most windows provide pull-down menus so that users can pick a command. To create a pull-down menu, you need to use the MENU command to define the following:
Menu title (typical menu titles are File, Edit, and Help)
Menu commands that appear on the menu, under the menu title (such as Edit, Print, or Cut)
A label to tell your program which instructions to follow after a user chooses a particular menu command
A typical menu command may look as follows:
MENU #WindowHandle, “Menu Title”, “Command1”, [command1]
In this example, #WindowHandle refers to the nickname of the window where you want the menu to appear, Menu Title appears at the top of the window, and Command1 appears after the user clicks the title of the pull-down menu.
To show you a real-life example, take a look at the following program:
NOMAINWIN
MENU #1, “&File”, “&Open”, [asOpen], “&Print”, [asPrint], “E&xit”, [quit]
OPEN “Menu Example” FOR Window AS #1
PRINT #1, “trapclose [quit]”
WAIT
[quit]

196 Part III: Advanced Programming with Liberty BASIC
CONFIRM “Are you sure that you want to quit?”; quit$
IF quit$ = “no” THEN WAIT
CLOSE #1
END
[asOpen]
NOTICE “Open command chosen”
WAIT
[asPrint]
NOTICE “Print command chosen” WAIT
This program works as follows:
1.The first line tells Liberty BASIC not to display the main window.
2.The second line defines a pull-down menu that appears in the window that the file handle #1 designates. The menu title is File and the commands that appear underneath are Open, Print, and Exit.
If you want a letter in a menu title or command to appear underlined, put an ampersand character (&) in front of that letter, such as &Print. Any underlined letter acts as a shortcut or accelerator key so that the user can choose that menu title by pressing Alt plus the underlined letter, such as Alt+F for the File menu. After selecting a menu, the user can then type the underlined letter in a command, such as X, to choose that menu command — for example, Exit.
3.The third line tells the computer, “Create a window with the text Menu Example in the title bar and identify this window by its file handle of #1.”
4.The fourth line tells the computer to follow the instructions following the [quit] label if the user tries to close the program.
5.The fifth line tells the computer to wait for the user to choose a command.
6.The sixth line is the [quit] label.
7.The seventh line displays a Confirm dialog box that asks, “Are you sure that you want to quit?” If the user clicks the Yes button, the program stores a value of “yes” in the quit$ variable. If the user clicks the No button, it stores a value of “no” in the quit$ variable.
8.The eighth line checks to see whether the value of the quit$ variable equals “no”. If so, it runs the WAIT command to wait for the user to do something.
9.The ninth line closes the window that the nickname #1 identifies. This line runs only if the user clicks the Yes button in the Confirm dialog box that the seventh line displays.

Chapter 14: Creating a User Interface 197
10.The tenth line marks the end of the program.
11.The eleventh line identifies the label [asOpen]. After the user chooses the Open command from the File menu, the computer jumps to this line to look for further instructions to follow.
12.The twelfth and thirteenth lines tell the computer, “Display a Notice dialog box with the message, Open command chosen. Then wait for the user to do something else.”
13.The fourteenth line identifies the label [asPrint]. After the user chooses the Print command from the File menu, the computer jumps to this line to look for further instructions to follow.
14.The fifteenth and sixteenth lines tell the computer, “Display a Notice dialog box with the message, Print command chosen. Then wait for the user to do something else.”
To define additional menus for a window, as shown in Figure 14-2, just use multiple MENU commands, as in the following example:
NOMAINWIN
MENU #1, “&File”, “&Open”, [asOpen], “&Print”, [asPrint], “E&xit”, [quit]
MENU #1, “&Help”, “&Contents”, [asContents], “&About”, [asAbout]
OPEN “Menu Example” FOR Window AS #1 PRINT #1, “trapclose [quit]”
WAIT
[quit]
CONFIRM “Are you sure that you want to quit?”; quit$ IF quit$ = “no” THEN WAIT
CLOSE #1 END
[asOpen]
NOTICE “Open command chosen” WAIT
[asPrint]
NOTICE “Print command chosen” WAIT
[asContents]
NOTICE “Contents command chosen” WAIT
[asAbout]
NOTICE “About command chosen” WAIT

198 Part III: Advanced Programming with Liberty BASIC
Each time that you create additional menu commands, you need to create instructions (which a [label] identifies) for the computer to follow whenever the user chooses those commands.
Figure 14-2:
Liberty
BASIC can create pull-down menus.
Making Pop-Up Menus
In addition to pull-down menus, many programs also offer pop-up menus that appear next to the mouse pointer when you right-click the mouse. To create a pop-up menu in Liberty BASIC, you need to use the POPUPMENU command, as follows:
POPUPMENU “command1”, [label1], “command2”, [label2]
To make the POPUPMENU easier to understand, place each command and label on a separate line and use the underscore character (_) at the end of each line. The underscore character tells Liberty BASIC that additional instructions appear on the following line. You can use the underscore character to divide long lines of commands into shorter, separate lines, such as you see in the following example:
POPUPMENU _ “command1”, [label1], _
“command2”, [label2], _ “command3”, [label3]
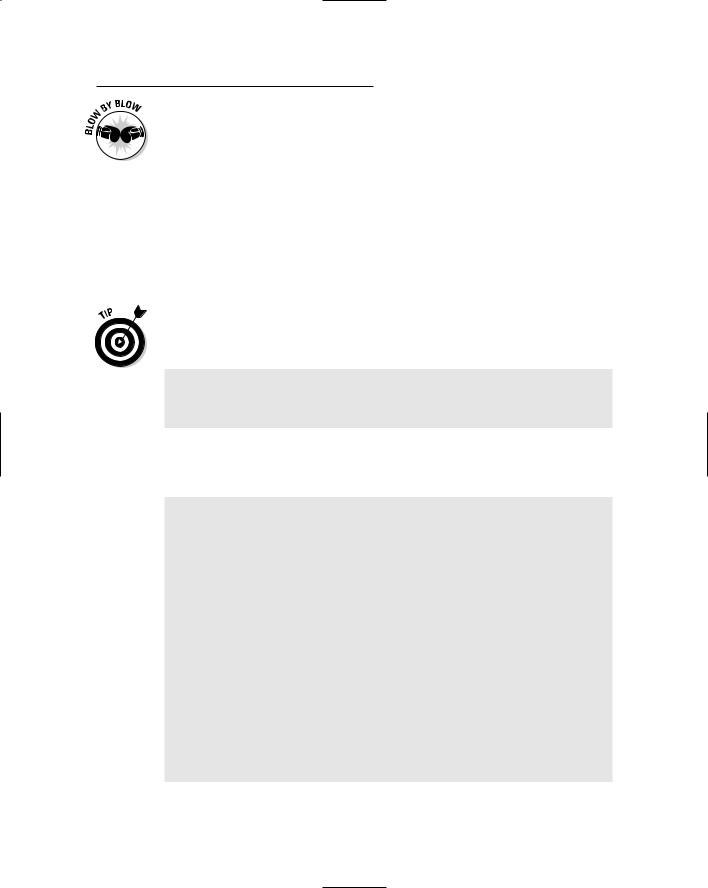
Chapter 14: Creating a User Interface 199
Here’s what the preceding commands do:
1.The POPUPMENU command tells the computer, “Create a pop-up menu next to the current position of the mouse pointer.”
2.The “command1” and “command2“ text are the actual commands that appear on the pop-up menu. You can add additional commands so that your pop-up menus can display 5, 7, or 15 different commands.
3.The [label1] and [label2] labels identify instructions for the computer to follow if the user chooses a particular menu command. So if the user chose “command1”, the computer immediately follows the instructions that the [label1] label identifies.
If you want to add a horizontal line to separate commands on your pop-up menu, just use the vertical line character (|) in place of a command and omit any label following the | character, as shown in the example below.
POPUPMENU _ “command1”, [label1], _
|, _
“command3”, [label3]
To see how pop-up menus work, try the following program, as shown in Figure 14-3. Notice how the vertical line character creates a horizontal line to separate commands on the pop-up menu.
NOMAINWIN
POPUPMENU _
“Destroy the planet”, [one], _ “Poison the environment”, [two], _ | ,_
“Get elected to political office”, [three]
NOTICE “Nothing selected.”
END
[one]
NOTICE “Launching missiles now.” END
[two]
NOTICE “Spilling oil into the oceans.” END
[three]
NOTICE “Fooled the public once more.” END