
Assembly Language Step by Step 1992
.pdfI'll deal with that issue in Chapter 2: Alien Bases) I'm sorry, but that's simply the way the game is played. Assembly language, at the innermost level, is nothing but numbers, and if you hate numbers the way most people hate anchovies, you're going to have a rough time of it.
I should caution you that the Game of Assembly Language represents no real computer processor like the 8088. Also, I've made the names of instructions more clearly understandable than the names of the instructions in 86 assembly language. In the real world, instruction names are typically things like STOSB, DAA, BVC, SBB, and other crypticisms that cannot be understood without considerable explanation. We're easing into this stuff sidewise, and in this chapter I have to sugar-coat certain things a little to draw the metaphors clearly.
Code and Data
Like most board games (including Big Bux), the assembly language board game consists of two broad categories of elements: Game steps and places to store things. The "game steps" are the steps and tests I've been speaking of all along. The places to store things are just that: The cubbyholes into which you can place numbers, with the confidence that those numbers will remain where you put them until you take them out or change them somehow.
In programming terms, the game steps are called code, and the numbers in their cubbyholes (as distinct from the cubbyholes themselves) are called data. The cubbyholes themselves are usually called storage.
The Game of Big Bux works the same way. Look back to Figure 0.1 and note that in the Start a Business detour, there is an instruction that reads Add $850,000 to checking account. The checking account is one of several different kinds of storage in this game, and money values are a type of data. It's no different conceptually from an instruction in the Game of Assembly Language that reads AJDLJ 5 to Register A. An ADD instruction in the code alters a data value stored in a cubbyhole named Register A.
Code and data are two very different kinds of critters, but they interact in ways that make the game interesting. The code includes steps that place data into storage (MOVE instructions) and steps that alter data that is already in storage (INCREMENT and DECREMENT instructions.) Most of the time you'll think of code as being the master of data, in that the code writes data values into storage. Data does influence code as well, however. Among the tests that the code makes are tests that examine data in storage (COMPARE instructions). If a given data value exists in storage, the code may do one
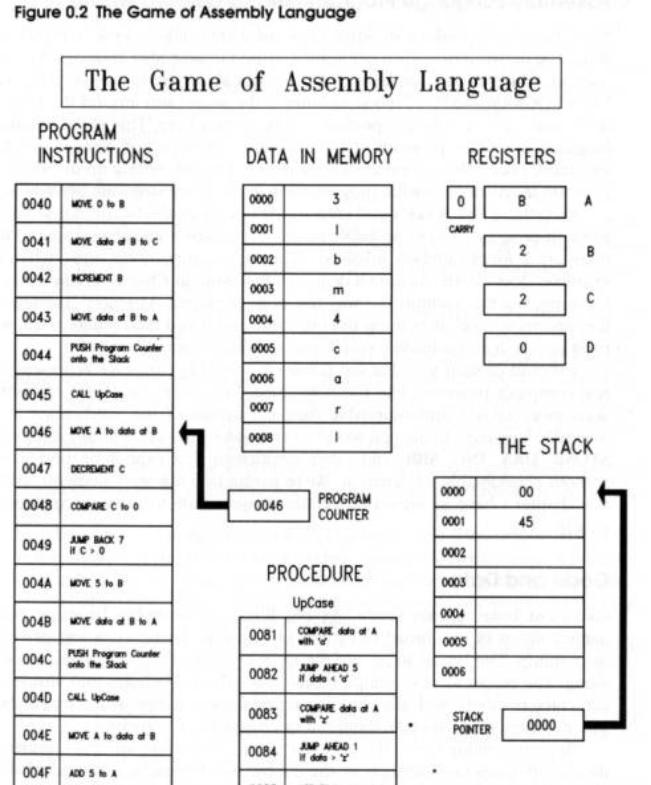
thing; if that value does not exist in storage, the code will do something else, as in the
JUMP BACK and JUMP AHEAD instructions.
The short block of instructions marked PROCEDURE is a detour off the main stream of instructions. At any point in the program you can duck out into the procedure, perform its steps and tests, and then return to the very place from which you left. This allows a sequence of steps and tests that is generally useful and used frequently to exist in only one place rather than exist as a separate copy everywhere it is needed.

Addresses
Another critical concept lies in the funny numbers at the left side of the program step locations and data locations. Each number is unique, in that a location tagged with that number appears only once inside the computer. This location is called an address. Data is stored and retrieved by specifying the data's address in the machine. Procedures are called by specifying the address at which they begin.
The little box (which is also a storage location) marked PROGRAM COUNTER keeps the address of the next instruction to be performed. The number inside the program counter is increased by one (we say, "incremented") each time an instruction is performed unless the instruction tells the program counter to do something else.
Notice the JUMP BACK 7 instruction at address 0049. When this instruction is performed, the program counter will back up by seven counts. This is analogous to the "go back three spaces" concept in most board games.
Metaphor Check!
That's about as much explanation of the Game of Assembly Language as I'm going to offer for now. This is still Chapter 0, and we're still in metaphor territory. People who have had some exposure to computers will recognize and understand more of what Figure 0.2 is doing. (There's a real, traceable program going on in there—I dare you to figure out what it does—and how!) People with no exposure to computer innards at all shouldn’t feel left behind for being utterly lost. I created the Game of Assembly Language solely to put across the following points:
•The individual steps are very simple. One single instruction rarely does more than move a single byte from one storage cubbyhole to another, or compare the value contained in one storage cubbyhole to a value contained in another. This is good news, because it allows you to concentrate on the simple task accomplished by a single instruction without being overwhelmed by complexity. The bad news, however, is that...
•It takes a lot of steps to do anything useful. You can often write a useful program in Pascal or BASIC in five or six lines. A useful assembly language program cannot be implemented in fewer than about fifty lines, and anything challenging takes hundreds or
thousands of lines. The skill of assembly language programming lies in structuring these hundreds or thousands of instructions so that the program can be read and understood. And finally,
• The key to assembly language is understanding memory addresses. In lan-guages like Pascal and BASIC, the compiler takes care of where something is located—you simply have to give that something a name, and call it by that name when you want it. In assembly language, you must always be cognizant of where things are in your computer's memory. So in working through this book, pay special attention to the concept of addressing, which is nothing more than the art of specifying where something is. The Game of Assembly Language is peppered with addresses and instructions that work with addresses. (Such as MOVE data at B to C, which means move the data stored at the address specified by register B to the address specified by register C.) Addressing is by far the trickiest part of assembly language, but master it and you've got the whole thing in your hip pocket.
Everything I've said so far has been orientation. I've tried to give you a taste of the big picture of assembly language and how its fundamental principles relate to the life you've been living all along. Life is a sequence of steps and tests, and so are board games—and so is assembly language. Keep those metaphors in mind as we proceed to "get real" by confronting the nature of computer numbers.

Alien Bases
Getting Your Arms around Binary and Hexadecimal
1.1The Return of the New Math Monster >• 14
1.2Counting in Martian >• 14
1.3Octal: How the Grinch Stole 8 and 9 >• 19
1.4Hexadecimal: Solving the Digit Shortage >• 22
1.5From Hex to Decimal and From Decimal to Hex >• 25
1.6Arithmetic in Hex >• 29
1.7Binary >• 34
1.8Hexadecimal as Shorthand for Binary >• 38
1.1 The Return of the New Math Monster
1966. Perhaps you were there. New Math burst upon the grade school curricula of the nation, and homework became a turmoil of number lines, sets, and alternate bases. Middleclass fathers scratched their heads with their children over questions like, "What is 17 in base 5?" and "Which sets does the Null Set belong to?" In very short order (I recall a period of about two months) the whole thing was tossed in the trash as quickly as it had been concocted by addle-brained educrats with too little to do.
This was a pity, actually. What nobody seemed to realize at the time was that, granted, we were learning New Math—except that Old Math had never been taught at the grade school
level either. We kept wondering of what possible use it was to know what the intersection of the set of squirrels and the set of mammals was. The truth, of course, was that it was no use at all. Mathematics in America has always been taught as applied mathematics— arithmetic—heavy on the word problems. If it won't help you balance your checkbook or proportion a recipe, it ain't real math, man. Little or nothing of the logic of mathematics has ever made it into the elementary classroom, in part because elementary school in America has historically been a sort of trade school for everyday life. Getting the little beasts fundamentally literate is diffi-cult enough. Trying to get them to appreciate the beauty of alternate number systems simply went over the line for practical middle-class America.
I was one of the few who enjoyed fussing with math in the New Age style back in 1966, but I gladly laid it aside when the whole thing blew over. I didn't have to pick it up again until 1976, when, after working like a maniac with a wire-wrap gun for several weeks, I fed power to my COSMAC ELF computer, and was greeted by an LED display of a pair of numbers in base 16!
Mon dieu, New Math redux...
This chapter exists because at the assembly-language level, your computer does not understand numbers in our familiar base 10. Computers, in a slightly schizoid fashion, work in base 2 and base 16—all at the same time. If you're willing to confine yourself to BASIC or Pascal, you can ignore these alien bases altogether, or perhaps treat them as an advanced topic once you get the rest of the language down pat. Not here. Everything in assembly language depends on your thorough understanding of these two number bases. So before we do anything else, we're going to learn how to count all over again—in Martian.
1.2 Counting in Martian
There is intelligent life on Mars.
That is, the Martians are intelligent enough to know from watching our TV programs these past forty years that a thriving tourist industry would not be to their advantage. So they've remained in hiding, emerging only briefly to carve big rocks into the shape of Elvis's face to help the National Enquirer ensure that no one will ever take Mars seriously again. The Martians do occasionally communicate with us science fiction writers, knowing full well that nobody has ever taken us seriously. Hence the information in this section, which involves the way Martians count.
Martians have three fingers on one hand, and only one finger on the other. Male Martians have their three fingers on the left hand, while females have their three fingers on the right
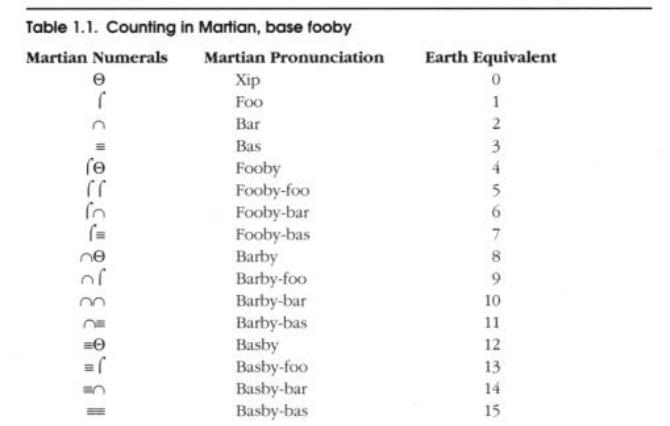
hand. This makes waltzing and certain other things easier.
Like human beings and any other intelligent race, Martians started counting by using their fingers. Just as we used our ten fingers to set things off in groups and powers of ten, the Martians used their four fingers to set things off in groups and powers of four. Over time, our civilization standardized on a set of ten digits to serve our number system. The Martians, similarly, standardized on a set of four digits for their number system. The four digits follow, along with the names of the digits as the Martians pronounce them: Q (Xip) , ó (Foo) , Ç (Bar), º (Bas).
Like our zero, xip is a placeholder representing no items, and while Mar-tians sometimes count from xip, they usually start with foo, representing a single item. So they start counting: Foo, bar, bas...
Now what? What comes after bas? Table 1.1 demonstrates how the Martians count to what we would call twenty-five.
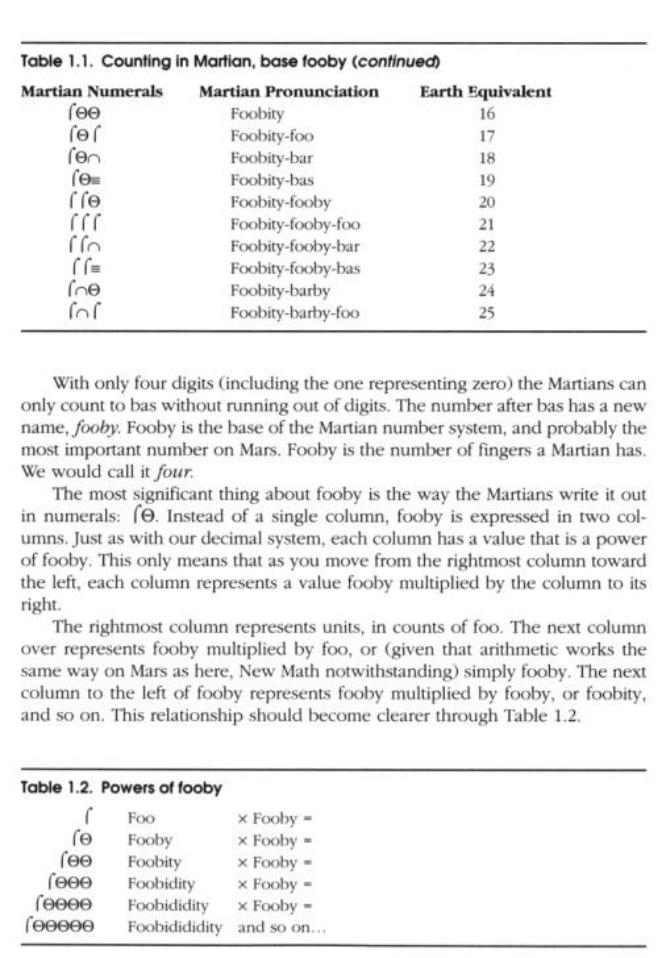

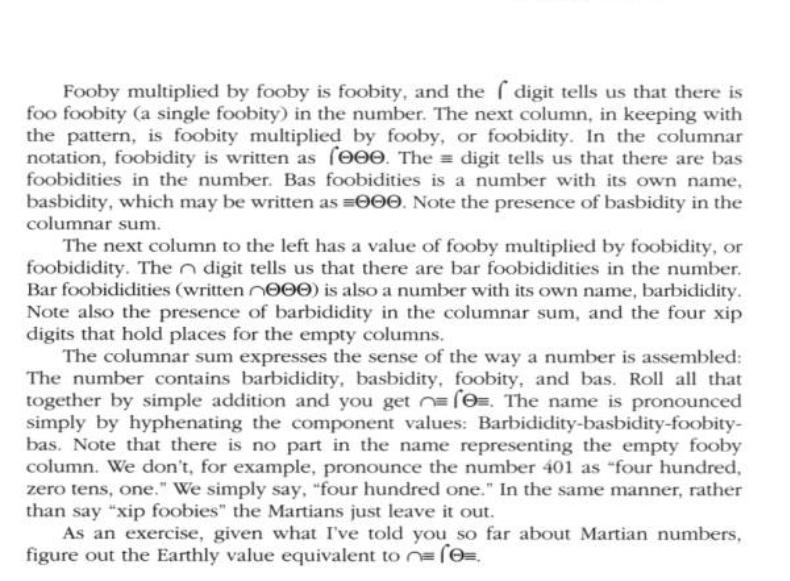
The Essence of a Number Base
Since tourist trips to Mars are unlikely to begin any time soon, of what Earthly use is knowing the Martian numbering system? Just this: it's an excellent way to see the sense in a number base without getting distracted by familiar digits and our universal base 10.
In a columnar system of numeric notation like both ours and the Martians', the base of the number system is the magnitude by which each column of a number exceeds the magnitude of the column to its right. In our base 10 system, each column represents a value ten multiplied by the column to its right. In a base fooby system, each column represents a value fooby multiplied by that of the column to its right. (In case you haven't already caught on, the Martians are actually using base 4—but I wanted you to see it from the Martians' own perspective.) Each has a set of digit symbols, the number of which is equal to the base. In our base 10, we have ten symbols, from 0 through 9. In base 4, there are four digits from 0 through 3. In any given number base, the base itself can never be expressed in a single digit!