
- •Introduction
- •Who This Book Is For
- •What This Book Covers
- •How This Book Is Structured
- •What You Need to Use This Book
- •Conventions
- •Source Code
- •Errata
- •p2p.wrox.com
- •What Are Regular Expressions?
- •What Can Regular Expressions Be Used For?
- •Finding Doubled Words
- •Checking Input from Web Forms
- •Changing Date Formats
- •Finding Incorrect Case
- •Adding Links to URLs
- •Regular Expressions You Already Use
- •Search and Replace in Word Processors
- •Directory Listings
- •Online Searching
- •Why Regular Expressions Seem Intimidating
- •Compact, Cryptic Syntax
- •Whitespace Can Significantly Alter the Meaning
- •No Standards Body
- •Differences between Implementations
- •Characters Change Meaning in Different Contexts
- •Regular Expressions Can Be Case Sensitive
- •Case-Sensitive and Case-Insensitive Matching
- •Case and Metacharacters
- •Continual Evolution in Techniques Supported
- •Multiple Solutions for a Single Problem
- •What You Want to Do with a Regular Expression
- •Replacing Text in Quantity
- •Regular Expression Tools
- •findstr
- •Microsoft Word
- •StarOffice Writer/OpenOffice.org Writer
- •Komodo Rx Package
- •PowerGrep
- •Microsoft Excel
- •JavaScript and JScript
- •VBScript
- •Visual Basic.NET
- •Java
- •Perl
- •MySQL
- •SQL Server 2000
- •W3C XML Schema
- •An Analytical Approach to Using Regular Expressions
- •Express and Document What You Want to Do in English
- •Consider the Regular Expression Options Available
- •Consider Sensitivity and Specificity
- •Create Appropriate Regular Expressions
- •Document All but Simple Regular Expressions
- •Document What You Expect the Regular Expression to Do
- •Document What You Want to Match
- •Test the Results of a Regular Expression
- •Matching Single Characters
- •Matching Sequences of Characters That Each Occur Once
- •Introducing Metacharacters
- •Matching Sequences of Different Characters
- •Matching Optional Characters
- •Matching Multiple Optional Characters
- •Other Cardinality Operators
- •The * Quantifier
- •The + Quantifier
- •The Curly-Brace Syntax
- •The {n} Syntax
- •The {n,m} Syntax
- •Exercises
- •Regular Expression Metacharacters
- •Thinking about Characters and Positions
- •The Period (.) Metacharacter
- •Matching Variably Structured Part Numbers
- •Matching a Literal Period
- •The \w Metacharacter
- •The \W Metacharacter
- •Digits and Nondigits
- •The \d Metacharacter
- •Canadian Postal Code Example
- •The \D Metacharacter
- •Alternatives to \d and \D
- •The \s Metacharacter
- •Handling Optional Whitespace
- •The \S Metacharacter
- •The \t Metacharacter
- •The \n Metacharacter
- •Escaped Characters
- •Finding the Backslash
- •Modifiers
- •Global Search
- •Case-Insensitive Search
- •Exercises
- •Introduction to Character Classes
- •Choice between Two Characters
- •Using Quantifiers with Character Classes
- •Using the \b Metacharacter in Character Classes
- •Selecting Literal Square Brackets
- •Using Ranges in Character Classes
- •Alphabetic Ranges
- •Use [A-z] With Care
- •Digit Ranges in Character Classes
- •Hexadecimal Numbers
- •IP Addresses
- •Reverse Ranges in Character Classes
- •A Potential Range Trap
- •Finding HTML Heading Elements
- •Metacharacter Meaning within Character Classes
- •The ^ metacharacter
- •How to Use the - Metacharacter
- •Negated Character Classes
- •Combining Positive and Negative Character Classes
- •POSIX Character Classes
- •The [:alnum:] Character Class
- •Exercises
- •String, Line, and Word Boundaries
- •The ^ Metacharacter
- •The ^ Metacharacter and Multiline Mode
- •The $ Metacharacter
- •The $ Metacharacter in Multiline Mode
- •Using the ^ and $ Metacharacters Together
- •Matching Blank Lines
- •Working with Dollar Amounts
- •Revisiting the IP Address Example
- •What Is a Word?
- •Identifying Word Boundaries
- •The \< Syntax
- •The \>Syntax
- •The \b Syntax
- •The \B Metacharacter
- •Less-Common Word-Boundary Metacharacters
- •Exercises
- •Grouping Using Parentheses
- •Parentheses and Quantifiers
- •Matching Literal Parentheses
- •U.S. Telephone Number Example
- •Alternation
- •Choosing among Multiple Options
- •Unexpected Alternation Behavior
- •Capturing Parentheses
- •Numbering of Captured Groups
- •Numbering When Using Nested Parentheses
- •Named Groups
- •Non-Capturing Parentheses
- •Back References
- •Exercises
- •Why You Need Lookahead and Lookbehind
- •The (? metacharacters
- •Lookahead
- •Positive Lookahead
- •Negative Lookahead
- •Positive Lookahead Examples
- •Positive Lookahead in the Same Document
- •Inserting an Apostrophe
- •Lookbehind
- •Positive Lookbehind
- •Negative Lookbehind
- •How to Match Positions
- •Adding Commas to Large Numbers
- •Exercises
- •What Are Sensitivity and Specificity?
- •Extreme Sensitivity, Awful Specificity
- •Email Addresses Example
- •Replacing Hyphens Example
- •The Sensitivity/Specificity Trade-Off
- •Sensitivity, Specificity, and Positional Characters
- •Sensitivity, Specificity, and Modes
- •Sensitivity, Specificity, and Lookahead and Lookbehind
- •How Much Should the Regular Expressions Do?
- •Abbreviations
- •Characters from Other Languages
- •Names
- •Sensitivity and How to Achieve It
- •Specificity and How to Maximize It
- •Exercises
- •Documenting Regular Expressions
- •Document the Problem Definition
- •Add Comments to Your Code
- •Making Use of Extended Mode
- •Know Your Data
- •Abbreviations
- •Proper Names
- •Incorrect Spelling
- •Creating Test Cases
- •Debugging Regular Expressions
- •Treacherous Whitespace
- •Backslashes Causing Problems
- •Considering Other Causes
- •The User Interface
- •Metacharacters Available
- •Quantifiers
- •The @ Quantifier
- •The {n,m} Syntax
- •Modes
- •Character Classes
- •Back References
- •Lookahead and Lookbehind
- •Lazy Matching versus Greedy Matching
- •Examples
- •Character Class Examples, Including Ranges
- •Whole Word Searches
- •Search-and-Replace Examples
- •Changing Name Structure Using Back References
- •Manipulating Dates
- •The Star Training Company Example
- •Regular Expressions in Visual Basic for Applications
- •Exercises
- •The User Interface
- •Metacharacters Available
- •Quantifiers
- •Modes
- •Character Classes
- •Alternation
- •Back References
- •Lookahead and Lookbehind
- •Search Example
- •Search-and-Replace Example
- •Online Chats
- •POSIX Character Classes
- •Matching Numeric Digits
- •Exercises
- •Introducing findstr
- •Finding Literal Text
- •Quantifiers
- •Character Classes
- •Command-Line Switch Examples
- •The /v Switch
- •The /a Switch
- •Single File Examples
- •Simple Character Class Example
- •Find Protocols Example
- •Multiple File Example
- •A Filelist Example
- •Exercises
- •The PowerGREP Interface
- •A Simple Find Example
- •The Replace Tab
- •The File Finder Tab
- •Syntax Coloring
- •Other Tabs
- •Numeric Digits and Alphabetic Characters
- •Quantifiers
- •Back References
- •Alternation
- •Line Position Metacharacters
- •Word-Boundary Metacharacters
- •Lookahead and Lookbehind
- •Longer Examples
- •Finding HTML Horizontal Rule Elements
- •Matching Time Example
- •Exercises
- •The Excel Find Interface
- •Escaping Wildcard Characters
- •Using Wildcards in Data Forms
- •Using Wildcards in Filters
- •Exercises
- •Using LIKE with Regular Expressions
- •The % Metacharacter
- •The _ Metacharacter
- •Character Classes
- •Negated Character Classes
- •Using Full-Text Search
- •Using The CONTAINS Predicate
- •Document Filters on Image Columns
- •Exercises
- •Using the _ and % Metacharacters
- •Testing Matching of Literals: _ and % Metacharacters
- •Using Positional Metacharacters
- •Using Character Classes
- •Quantifiers
- •Social Security Number Example
- •Exercises
- •The Interface to Metacharacters in Microsoft Access
- •Creating a Hard-Wired Query
- •Creating a Parameter Query
- •Using the ? Metacharacter
- •Using the * Metacharacter
- •Using the # Metacharacter
- •Using the # Character with Date/Time Data
- •Using Character Classes in Access
- •Exercises
- •The RegExp Object
- •Attributes of the RegExp Object
- •The Other Properties of the RegExp Object
- •The test() Method of the RegExp Object
- •The exec() Method of the RegExp Object
- •The String Object
- •Metacharacters in JavaScript and JScript
- •SSN Validation Example
- •Exercises
- •The RegExp Object and How to Use It
- •Quantifiers
- •Positional Metacharacters
- •Character Classes
- •Word Boundaries
- •Lookahead
- •Grouping and Nongrouping Parentheses
- •Exercises
- •The System.Text.RegularExpressions namespace
- •A Simple Visual Basic .NET Example
- •The Classes of System.Text.RegularExpressions
- •The Regex Object
- •Using the Match Object and Matches Collection
- •Using the Match.Success Property and Match.NextMatch Method
- •The GroupCollection and Group Classes
- •The CaptureCollection and Capture Class
- •The RegexOptions Enumeration
- •Case-Insensitive Matching: The IgnoreCase Option
- •Multiline Matching: The Effect on the ^ and $ Metacharacters
- •Right to Left Matching: The RightToLeft Option
- •Lookahead and Lookbehind
- •Exercises
- •An Introductory Example
- •The Classes of System.Text.RegularExpressions
- •The Regex Class
- •The Options Property of the Regex Class
- •Regex Class Methods
- •The CompileToAssembly() Method
- •The GetGroupNames() Method
- •The GetGroupNumbers() Method
- •GroupNumberFromName() and GroupNameFromNumber() Methods
- •The IsMatch() Method
- •The Match() Method
- •The Matches() Method
- •The Replace() Method
- •The Split() Method
- •Using the Static Methods of the Regex Class
- •The IsMatch() Method as a Static
- •The Match() Method as a Static
- •The Matches() Method as a Static
- •The Replace() Method as a Static
- •The Split() Method as a Static
- •The Match and Matches Classes
- •The Match Class
- •The GroupCollection and Group Classes
- •The RegexOptions Class
- •The IgnorePatternWhitespace Option
- •Metacharacters Supported in Visual C# .NET
- •Using Named Groups
- •Using Back References
- •Exercise
- •The ereg() Set of Functions
- •The ereg() Function
- •The ereg() Function with Three Arguments
- •The eregi() Function
- •The ereg_replace() Function
- •The eregi_replace() Function
- •The split() Function
- •The spliti() Function
- •The sql_regcase() Function
- •Perl Compatible Regular Expressions
- •Pattern Delimiters in PCRE
- •Escaping Pattern Delimiters
- •Matching Modifiers in PCRE
- •Using the preg_match() Function
- •Using the preg_match_all() Function
- •Using the preg_grep() Function
- •Using the preg_quote() Function
- •Using the preg_replace() Function
- •Using the preg_replace_callback() Function
- •Using the preg_split() Function
- •Supported Metacharacters with ereg()
- •Using POSIX Character Classes with PHP
- •Supported Metacharacters with PCRE
- •Positional Metacharacters
- •Character Classes in PHP
- •Documenting PHP Regular Expressions
- •Exercises
- •W3C XML Schema Basics
- •Tools for Using W3C XML Schema
- •Comparing XML Schema and DTDs
- •How Constraints Are Expressed in W3C XML Schema
- •W3C XML Schema Datatypes
- •Derivation by Restriction
- •Unicode and W3C XML Schema
- •Unicode Overview
- •Using Unicode Character Classes
- •Matching Decimal Numbers
- •Mixing Unicode Character Classes with Other Metacharacters
- •Unicode Character Blocks
- •Using Unicode Character Blocks
- •Metacharacters Supported in W3C XML Schema
- •Positional Metacharacters
- •Matching Numeric Digits
- •Alternation
- •Using the \w and \s Metacharacters
- •Escaping Metacharacters
- •Exercises
- •Introduction to the java.util.regex Package
- •Obtaining and Installing Java
- •The Pattern Class
- •Using the matches() Method Statically
- •Two Simple Java Examples
- •The Properties (Fields) of the Pattern Class
- •The CASE_INSENSITIVE Flag
- •Using the COMMENTS Flag
- •The DOTALL Flag
- •The MULTILINE Flag
- •The UNICODE_CASE Flag
- •The UNIX_LINES Flag
- •The Methods of the Pattern Class
- •The compile() Method
- •The flags() Method
- •The matcher() Method
- •The matches() Method
- •The pattern() Method
- •The split() Method
- •The Matcher Class
- •The appendReplacement() Method
- •The appendTail() Method
- •The end() Method
- •The find() Method
- •The group() Method
- •The groupCount() Method
- •The lookingAt() Method
- •The matches() Method
- •The pattern() Method
- •The replaceAll() Method
- •The replaceFirst() Method
- •The reset() Method
- •The start() Method
- •The PatternSyntaxException Class
- •Using the \d Metacharacter
- •Character Classes
- •The POSIX Character Classes in the java.util.regex Package
- •Unicode Character Classes and Character Blocks
- •Using Escaped Characters
- •Using Methods of the String Class
- •Using the matches() Method
- •Using the replaceFirst() Method
- •Using the replaceAll() Method
- •Using the split() Method
- •Exercises
- •Obtaining and Installing Perl
- •Creating a Simple Perl Program
- •Basics of Perl Regular Expression Usage
- •Using the m// Operator
- •Using Other Regular Expression Delimiters
- •Matching Using Variable Substitution
- •Using the s/// Operator
- •Using s/// with the Global Modifier
- •Using s/// with the Default Variable
- •Using the split Operator
- •Using Quantifiers in Perl
- •Using Positional Metacharacters
- •Captured Groups in Perl
- •Using Back References in Perl
- •Using Alternation
- •Using Character Classes in Perl
- •Using Lookahead
- •Using Lookbehind
- •Escaping Metacharacters
- •A Simple Perl Regex Tester
- •Exercises
- •Index

PHP and Regular Expressions
Figure 23-4
How PHP Structures Suppor t for Regular Expressions
Regular expression support in PHP is provided by two different sets of functions. One, ereg() and related functions, has been available in PHP for some time (you can use it with PHP3 Web servers if you wish). The other, Perl Compatible Regular Expressions (PCRE), is more recent and has more regular expression functionality. Most of the descriptions and examples in this chapter will focus on the PCRE functionality.
If you need compatibility with older versions of PHP, the ereg() set of functions may be your only choice. On the other hand, if you need the functionality that is absent from the ereg() family, PCRE may be the most viable option, and if necessary, you may need to upgrade the PHP version on your Web server(s).
The ereg() Set of Functions
The ereg() set of functions is based on POSIX regular expressions. The following table summarizes the functions that relate to the ereg() function.
Function |
Description |
|
|
ereg() |
Attempts to match a regular expression pattern against a string |
|
case sensitively. |
eregi() |
Attempts to match a regular expression pattern against a string |
|
case insensitively. |
ereg_replace() |
Attempts to match a regular expression pattern case sensi- |
|
tively, and if matches are found, they are replaced. |
|
Table continued on following page |
553

Chapter 23
Function |
Description |
|
|
eregi_replace() |
Attempts to match a regular expression pattern case insensi- |
|
tively, and if matches are found, they are replaced. |
split() |
Splits a string into an array, based on matching of a regular |
|
expression, case-sensitive matching. |
spliti() |
Splits a string into an array, based on matching of a regular |
|
expression, case-insensitive matching. |
sql_regcase() |
Creates a valid regular expression pattern to attempt case- |
|
insensitive matching of a specified string. |
|
|
The ereg() Function
The ereg() function matches case sensitively. The ereg() function can be used with two arguments or three. When ereg() is used with two arguments, the first argument is a string value, which is a regular expression pattern. The second argument is a test string.
For example, if you wanted to find whether there is a match for the literal pattern the in the test string
The theatre is a favorite of thespians., you could use the following code:
ereg(‘the’, “The theatre is a favorite of thespians”);
Because ereg() matches case sensitively, there are two matches: the first three characters of theatre and of thespians.
Before looking at how to use ereg() with three arguments, work through the following example, which uses the ereg() function with two arguments. It shows a very simple use of the ereg() function to match a literal regular expression pattern, Hel, against a literal string value, Hello world!.
Try It Out |
A Simple ereg() Example |
1.In a text editor, enter the following code. You can use Notepad if you have no other text editor available.
<html>
<head>
<title>Simple ereg() Regex Test</title> </head>
<body>
<?php
if (ereg(“Hel”, “Hello world!”)) echo “<p>A match was found.</p>” ?>
</body>
</html>
2.Save the file as C:\inetpub\PHP\SimpleRegexTest.php. Modify the location if your Web server is not located on the local machine or you created the PHP directory in a different location.
554

PHP and Regular Expressions
3.In your preferred Web browser, enter the URL http://localhost/PHP/SimpleRegexTest.php; press the Return key; and inspect the Web page that is displayed, as shown in Figure 23-5. The message A match was found. is displayed.
Figure 23-5
How It Works
The file SimpleRegexTest.php includes HTML/XHTML markup and PHP code. The PHP code is very simple:
<?php
if (ereg(“Hel”, “Hello world!”)) echo “<p>A match was found.</p>” ?>
The <?php marks where the PHP code begins, and the ?> marks where the PHP code ends. The middle line is the PHP code itself.
The if statement tests the value returned by the ereg() function. The ereg() function takes two arguments in this example. The first argument is the string Hel, which is interpreted as a literal regular expression pattern. The second argument is the string Hello world!, which is the test string. In other words, the PHP processor attempts to find the pattern Hel in the string Hello world!. Not surprisingly, a match is found, so the ereg() function returns the value 1, indicating the presence of a match. The value 1 is interpreted as equivalent to True, so the code controlled by the if statement is executed:
echo “<p>A match was found.</p>”
Typically, a PHP statement has to end with a semicolon. Because this very simple example has only one line of PHP code, it isn’t necessary to add a semicolon. In other examples in this chapter, your code almost certainly won’t run correctly if you omit the semicolon at the end of PHP statements.
The echo statement causes a string to be inserted into the Web page at the position where the PHP code existed in the Web page SimpleRegexTest.php. That string, which includes HTML/XHTML markup, is inserted on the server between the start tag, <body>, and the end tag, </body>. The source code for the Web page, as delivered to the client, is shown in Figure 23-6.
555
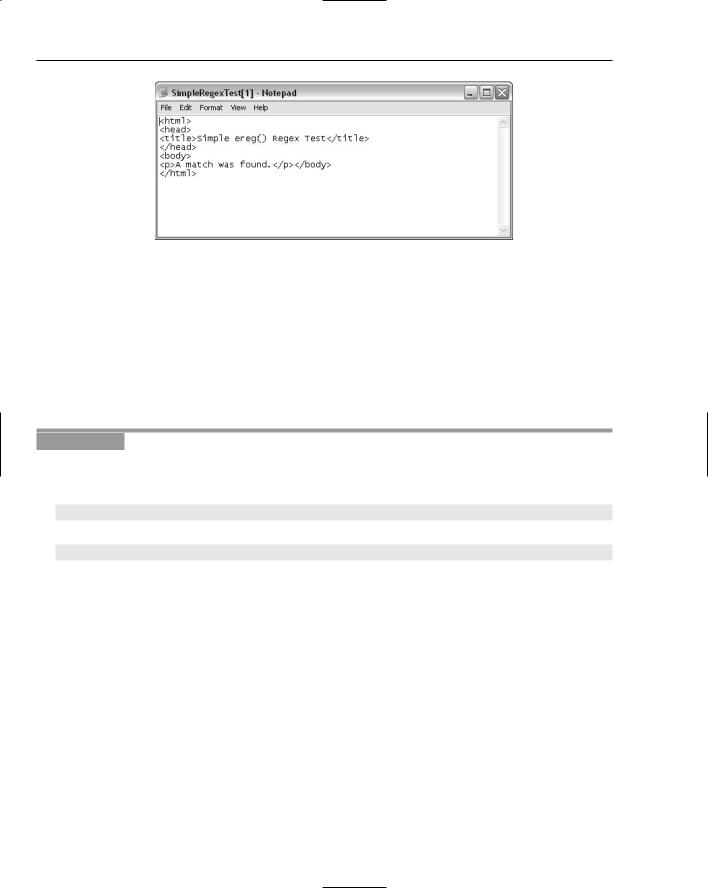
Chapter 23
Figure 23-6
This example simply used the boolean value returned by the ereg() function to control the literal text that was added to the Web page. The next example looks at how the content of individual matches can be manipulated when using the ereg() function.
The ereg() Function with Three Arguments
When the ereg() function is used with three arguments, the first argument is the regular expression pattern expressed as a string value, the second argument is the test string, and the third argument specifies an array where the matches are to be stored.
Try It Out |
The ereg() Function with Three Arguments |
1.In your favorite text editor, enter the following code:
<html>
<head>
<title>Splitting a date using ereg()</title> </head>
<body>
<?php
$myPattern = ‘([12][0-9]{3}) ([01][0-9]) ([0123][0-9])’; $testString = gmdate(“Y m d”);
echo “<p>The date is now: $testString</p>”; $myResult = ereg($myPattern, $testString, $matches); if ($myResult)
{
echo “<p>A match was found when testing case sensitively.</p>”; echo “<p>Expressed in MM/DD/YYYY format the date is now, $matches[2]/$matches[3]/$matches[1].</p>”;
}
else
{
echo “<p>No match was found when testing case sensitively.</p>”;
}
?>
</body>
</html>
2.Save the code as SplitDate.php in the PHP directory in the wwwroot directory of your IIS installation. In other words, save the code as C:\inetpub\wwwroot\PHP\SplitDate.php.
556

PHP and Regular Expressions
3.Enter the URL http://localhost/PHP/SplitDate.php in Internet Explorer, and inspect the displayed results, as shown in Figure 23-7. The code was run on September 22, 2004. Notice that on the final line in the Web browser, that date is displayed as 09/22/2004, having originally been 2004 09 22 when formatted by the gmdate() function.
Figure 23-7
How It Works
First, look at the pattern that is used:
([12][0-9]{3}) ([01][0-9]) ([0123][0-9])
Because the date is created by the PHP gmdate() function, you don’t, realistically, need to worry much about the function returning invalid dates. So you can use a fairly crude pattern here.
This pattern first matches a single numeric digit: a 1 or 2. This makes sense if the dates you are dealing with are in the twentieth and twenty-first centuries. Then three further numeric digits are matched. This part of the pattern is contained in paired parentheses, so it is captured. You will see a little later how the captured group is handled and retrieved. This pattern component matches a four-digit year from the twentieth or twenty-first century.
A single space character follows. Then comes a further captured group beginning with a 0 or 1, which is followed by a numeric digit. This matches the numeric value of the month. Theoretically, it will match “months” such as the 19th month of a year. However, because the gmdate() function is very unlikely ever to return a spurious date like that, it’s an issue you don’t need to worry about in this example.
Then follows a single space character and a further captured group beginning with a 0, 1, 2, or 3 followed by a numeric digit. This matches the numeric value of the day of the month. Theoretically, it will match “days” such as the 39th of January. Again, because the gmdate() function is very unlikely ever to return a spurious date, you don’t need to worry about it.
First, you assign the pattern, as a string, to the $myPattern variable:
$myPattern = ‘([12][0-9]{3}) ([01][0-9]) ([0123][0-9])’;
Then you use the gmdate() function to assign a string value to the $testString variable. The arguments to the gmdate() function specify the formatting of the date returned. In this case, Y specifies that the year is returned as a four-digit form, 2004; the m specifies that the month is expressed as a number between 1 and 12; and the d specifies that the day of the month is expressed as a number from 1 to 31:
557

Chapter 23
$testString = gmdate(“Y m d”);
Then you use the echo statement to display the format in which the current date was returned. If you are used to languages such as JavaScript, you may find it surprising that the $testString variable is just written inside the paired double quotes rather than having literal text inside paired double quotes and using the + concatenation operator. In PHP, you simply write a variable inside the paired quotes, and the PHP processor knows it is intended that the variable’s value be displayed:
echo “<p>The date is now: $testString</p>”;
Then you use the ereg() function with three arguments to assign a value to the $myResult variable. If one or more matches exist, the $myResult variable contains the boolean value True (1), and if there is no match, it contains the boolean value False (0).
The first argument is a pattern, and the second argument is the test string, as before. The third argument is an array containing the components of the matched text. The elements of that array are determined by the capturing parentheses, which were mentioned when you looked at the components of the regular expression pattern:
$myResult = ereg($myPattern, $testString, $matches);
You test to see if there was a match:
if ($myResult)
If there was, you use two echo statements to add content to the Web page:
{
You simply state that a match was found:
echo “<p>A match was found when testing case sensitively.</p>”;
Then you indicate that the date will reorder the date. This is done using
be returned in MM/DD/YYYY format, which means that you must $matches[2]/$matches[3]/$matches[1].
The value of the whole match is in array element 0. The pattern that matched the date contained three pairs of capturing parentheses. The result of the first pair goes in $matches[1], the result of the second pair in $matches[2], and so on. So the year is in $matches[1], the month in $matches[2], and the day in $matches[3].
Because you want to return MM/DD/YYYY, you use the sequence $matches[2]/$matches[3]/ $matches[1], which means return the value in $matches[2] (the month) followed by a literal forwardslash character, followed by the value in $matches[3] (the day), followed by a literal forward-slash character, followed by the value in $matches [1] (the year).
So the value that was originally displayed as 2004 09 20 is transformed into 09/20/2004:
echo “<p>Expressed in MM/DD/YYYY format the date is now,
$matches[2]/$matches[3]/$matches[1].</p>”;
558