
- •Introduction
- •Who This Book Is For
- •What This Book Covers
- •How This Book Is Structured
- •What You Need to Use This Book
- •Conventions
- •Source Code
- •Errata
- •p2p.wrox.com
- •What Are Regular Expressions?
- •What Can Regular Expressions Be Used For?
- •Finding Doubled Words
- •Checking Input from Web Forms
- •Changing Date Formats
- •Finding Incorrect Case
- •Adding Links to URLs
- •Regular Expressions You Already Use
- •Search and Replace in Word Processors
- •Directory Listings
- •Online Searching
- •Why Regular Expressions Seem Intimidating
- •Compact, Cryptic Syntax
- •Whitespace Can Significantly Alter the Meaning
- •No Standards Body
- •Differences between Implementations
- •Characters Change Meaning in Different Contexts
- •Regular Expressions Can Be Case Sensitive
- •Case-Sensitive and Case-Insensitive Matching
- •Case and Metacharacters
- •Continual Evolution in Techniques Supported
- •Multiple Solutions for a Single Problem
- •What You Want to Do with a Regular Expression
- •Replacing Text in Quantity
- •Regular Expression Tools
- •findstr
- •Microsoft Word
- •StarOffice Writer/OpenOffice.org Writer
- •Komodo Rx Package
- •PowerGrep
- •Microsoft Excel
- •JavaScript and JScript
- •VBScript
- •Visual Basic.NET
- •Java
- •Perl
- •MySQL
- •SQL Server 2000
- •W3C XML Schema
- •An Analytical Approach to Using Regular Expressions
- •Express and Document What You Want to Do in English
- •Consider the Regular Expression Options Available
- •Consider Sensitivity and Specificity
- •Create Appropriate Regular Expressions
- •Document All but Simple Regular Expressions
- •Document What You Expect the Regular Expression to Do
- •Document What You Want to Match
- •Test the Results of a Regular Expression
- •Matching Single Characters
- •Matching Sequences of Characters That Each Occur Once
- •Introducing Metacharacters
- •Matching Sequences of Different Characters
- •Matching Optional Characters
- •Matching Multiple Optional Characters
- •Other Cardinality Operators
- •The * Quantifier
- •The + Quantifier
- •The Curly-Brace Syntax
- •The {n} Syntax
- •The {n,m} Syntax
- •Exercises
- •Regular Expression Metacharacters
- •Thinking about Characters and Positions
- •The Period (.) Metacharacter
- •Matching Variably Structured Part Numbers
- •Matching a Literal Period
- •The \w Metacharacter
- •The \W Metacharacter
- •Digits and Nondigits
- •The \d Metacharacter
- •Canadian Postal Code Example
- •The \D Metacharacter
- •Alternatives to \d and \D
- •The \s Metacharacter
- •Handling Optional Whitespace
- •The \S Metacharacter
- •The \t Metacharacter
- •The \n Metacharacter
- •Escaped Characters
- •Finding the Backslash
- •Modifiers
- •Global Search
- •Case-Insensitive Search
- •Exercises
- •Introduction to Character Classes
- •Choice between Two Characters
- •Using Quantifiers with Character Classes
- •Using the \b Metacharacter in Character Classes
- •Selecting Literal Square Brackets
- •Using Ranges in Character Classes
- •Alphabetic Ranges
- •Use [A-z] With Care
- •Digit Ranges in Character Classes
- •Hexadecimal Numbers
- •IP Addresses
- •Reverse Ranges in Character Classes
- •A Potential Range Trap
- •Finding HTML Heading Elements
- •Metacharacter Meaning within Character Classes
- •The ^ metacharacter
- •How to Use the - Metacharacter
- •Negated Character Classes
- •Combining Positive and Negative Character Classes
- •POSIX Character Classes
- •The [:alnum:] Character Class
- •Exercises
- •String, Line, and Word Boundaries
- •The ^ Metacharacter
- •The ^ Metacharacter and Multiline Mode
- •The $ Metacharacter
- •The $ Metacharacter in Multiline Mode
- •Using the ^ and $ Metacharacters Together
- •Matching Blank Lines
- •Working with Dollar Amounts
- •Revisiting the IP Address Example
- •What Is a Word?
- •Identifying Word Boundaries
- •The \< Syntax
- •The \>Syntax
- •The \b Syntax
- •The \B Metacharacter
- •Less-Common Word-Boundary Metacharacters
- •Exercises
- •Grouping Using Parentheses
- •Parentheses and Quantifiers
- •Matching Literal Parentheses
- •U.S. Telephone Number Example
- •Alternation
- •Choosing among Multiple Options
- •Unexpected Alternation Behavior
- •Capturing Parentheses
- •Numbering of Captured Groups
- •Numbering When Using Nested Parentheses
- •Named Groups
- •Non-Capturing Parentheses
- •Back References
- •Exercises
- •Why You Need Lookahead and Lookbehind
- •The (? metacharacters
- •Lookahead
- •Positive Lookahead
- •Negative Lookahead
- •Positive Lookahead Examples
- •Positive Lookahead in the Same Document
- •Inserting an Apostrophe
- •Lookbehind
- •Positive Lookbehind
- •Negative Lookbehind
- •How to Match Positions
- •Adding Commas to Large Numbers
- •Exercises
- •What Are Sensitivity and Specificity?
- •Extreme Sensitivity, Awful Specificity
- •Email Addresses Example
- •Replacing Hyphens Example
- •The Sensitivity/Specificity Trade-Off
- •Sensitivity, Specificity, and Positional Characters
- •Sensitivity, Specificity, and Modes
- •Sensitivity, Specificity, and Lookahead and Lookbehind
- •How Much Should the Regular Expressions Do?
- •Abbreviations
- •Characters from Other Languages
- •Names
- •Sensitivity and How to Achieve It
- •Specificity and How to Maximize It
- •Exercises
- •Documenting Regular Expressions
- •Document the Problem Definition
- •Add Comments to Your Code
- •Making Use of Extended Mode
- •Know Your Data
- •Abbreviations
- •Proper Names
- •Incorrect Spelling
- •Creating Test Cases
- •Debugging Regular Expressions
- •Treacherous Whitespace
- •Backslashes Causing Problems
- •Considering Other Causes
- •The User Interface
- •Metacharacters Available
- •Quantifiers
- •The @ Quantifier
- •The {n,m} Syntax
- •Modes
- •Character Classes
- •Back References
- •Lookahead and Lookbehind
- •Lazy Matching versus Greedy Matching
- •Examples
- •Character Class Examples, Including Ranges
- •Whole Word Searches
- •Search-and-Replace Examples
- •Changing Name Structure Using Back References
- •Manipulating Dates
- •The Star Training Company Example
- •Regular Expressions in Visual Basic for Applications
- •Exercises
- •The User Interface
- •Metacharacters Available
- •Quantifiers
- •Modes
- •Character Classes
- •Alternation
- •Back References
- •Lookahead and Lookbehind
- •Search Example
- •Search-and-Replace Example
- •Online Chats
- •POSIX Character Classes
- •Matching Numeric Digits
- •Exercises
- •Introducing findstr
- •Finding Literal Text
- •Quantifiers
- •Character Classes
- •Command-Line Switch Examples
- •The /v Switch
- •The /a Switch
- •Single File Examples
- •Simple Character Class Example
- •Find Protocols Example
- •Multiple File Example
- •A Filelist Example
- •Exercises
- •The PowerGREP Interface
- •A Simple Find Example
- •The Replace Tab
- •The File Finder Tab
- •Syntax Coloring
- •Other Tabs
- •Numeric Digits and Alphabetic Characters
- •Quantifiers
- •Back References
- •Alternation
- •Line Position Metacharacters
- •Word-Boundary Metacharacters
- •Lookahead and Lookbehind
- •Longer Examples
- •Finding HTML Horizontal Rule Elements
- •Matching Time Example
- •Exercises
- •The Excel Find Interface
- •Escaping Wildcard Characters
- •Using Wildcards in Data Forms
- •Using Wildcards in Filters
- •Exercises
- •Using LIKE with Regular Expressions
- •The % Metacharacter
- •The _ Metacharacter
- •Character Classes
- •Negated Character Classes
- •Using Full-Text Search
- •Using The CONTAINS Predicate
- •Document Filters on Image Columns
- •Exercises
- •Using the _ and % Metacharacters
- •Testing Matching of Literals: _ and % Metacharacters
- •Using Positional Metacharacters
- •Using Character Classes
- •Quantifiers
- •Social Security Number Example
- •Exercises
- •The Interface to Metacharacters in Microsoft Access
- •Creating a Hard-Wired Query
- •Creating a Parameter Query
- •Using the ? Metacharacter
- •Using the * Metacharacter
- •Using the # Metacharacter
- •Using the # Character with Date/Time Data
- •Using Character Classes in Access
- •Exercises
- •The RegExp Object
- •Attributes of the RegExp Object
- •The Other Properties of the RegExp Object
- •The test() Method of the RegExp Object
- •The exec() Method of the RegExp Object
- •The String Object
- •Metacharacters in JavaScript and JScript
- •SSN Validation Example
- •Exercises
- •The RegExp Object and How to Use It
- •Quantifiers
- •Positional Metacharacters
- •Character Classes
- •Word Boundaries
- •Lookahead
- •Grouping and Nongrouping Parentheses
- •Exercises
- •The System.Text.RegularExpressions namespace
- •A Simple Visual Basic .NET Example
- •The Classes of System.Text.RegularExpressions
- •The Regex Object
- •Using the Match Object and Matches Collection
- •Using the Match.Success Property and Match.NextMatch Method
- •The GroupCollection and Group Classes
- •The CaptureCollection and Capture Class
- •The RegexOptions Enumeration
- •Case-Insensitive Matching: The IgnoreCase Option
- •Multiline Matching: The Effect on the ^ and $ Metacharacters
- •Right to Left Matching: The RightToLeft Option
- •Lookahead and Lookbehind
- •Exercises
- •An Introductory Example
- •The Classes of System.Text.RegularExpressions
- •The Regex Class
- •The Options Property of the Regex Class
- •Regex Class Methods
- •The CompileToAssembly() Method
- •The GetGroupNames() Method
- •The GetGroupNumbers() Method
- •GroupNumberFromName() and GroupNameFromNumber() Methods
- •The IsMatch() Method
- •The Match() Method
- •The Matches() Method
- •The Replace() Method
- •The Split() Method
- •Using the Static Methods of the Regex Class
- •The IsMatch() Method as a Static
- •The Match() Method as a Static
- •The Matches() Method as a Static
- •The Replace() Method as a Static
- •The Split() Method as a Static
- •The Match and Matches Classes
- •The Match Class
- •The GroupCollection and Group Classes
- •The RegexOptions Class
- •The IgnorePatternWhitespace Option
- •Metacharacters Supported in Visual C# .NET
- •Using Named Groups
- •Using Back References
- •Exercise
- •The ereg() Set of Functions
- •The ereg() Function
- •The ereg() Function with Three Arguments
- •The eregi() Function
- •The ereg_replace() Function
- •The eregi_replace() Function
- •The split() Function
- •The spliti() Function
- •The sql_regcase() Function
- •Perl Compatible Regular Expressions
- •Pattern Delimiters in PCRE
- •Escaping Pattern Delimiters
- •Matching Modifiers in PCRE
- •Using the preg_match() Function
- •Using the preg_match_all() Function
- •Using the preg_grep() Function
- •Using the preg_quote() Function
- •Using the preg_replace() Function
- •Using the preg_replace_callback() Function
- •Using the preg_split() Function
- •Supported Metacharacters with ereg()
- •Using POSIX Character Classes with PHP
- •Supported Metacharacters with PCRE
- •Positional Metacharacters
- •Character Classes in PHP
- •Documenting PHP Regular Expressions
- •Exercises
- •W3C XML Schema Basics
- •Tools for Using W3C XML Schema
- •Comparing XML Schema and DTDs
- •How Constraints Are Expressed in W3C XML Schema
- •W3C XML Schema Datatypes
- •Derivation by Restriction
- •Unicode and W3C XML Schema
- •Unicode Overview
- •Using Unicode Character Classes
- •Matching Decimal Numbers
- •Mixing Unicode Character Classes with Other Metacharacters
- •Unicode Character Blocks
- •Using Unicode Character Blocks
- •Metacharacters Supported in W3C XML Schema
- •Positional Metacharacters
- •Matching Numeric Digits
- •Alternation
- •Using the \w and \s Metacharacters
- •Escaping Metacharacters
- •Exercises
- •Introduction to the java.util.regex Package
- •Obtaining and Installing Java
- •The Pattern Class
- •Using the matches() Method Statically
- •Two Simple Java Examples
- •The Properties (Fields) of the Pattern Class
- •The CASE_INSENSITIVE Flag
- •Using the COMMENTS Flag
- •The DOTALL Flag
- •The MULTILINE Flag
- •The UNICODE_CASE Flag
- •The UNIX_LINES Flag
- •The Methods of the Pattern Class
- •The compile() Method
- •The flags() Method
- •The matcher() Method
- •The matches() Method
- •The pattern() Method
- •The split() Method
- •The Matcher Class
- •The appendReplacement() Method
- •The appendTail() Method
- •The end() Method
- •The find() Method
- •The group() Method
- •The groupCount() Method
- •The lookingAt() Method
- •The matches() Method
- •The pattern() Method
- •The replaceAll() Method
- •The replaceFirst() Method
- •The reset() Method
- •The start() Method
- •The PatternSyntaxException Class
- •Using the \d Metacharacter
- •Character Classes
- •The POSIX Character Classes in the java.util.regex Package
- •Unicode Character Classes and Character Blocks
- •Using Escaped Characters
- •Using Methods of the String Class
- •Using the matches() Method
- •Using the replaceFirst() Method
- •Using the replaceAll() Method
- •Using the split() Method
- •Exercises
- •Obtaining and Installing Perl
- •Creating a Simple Perl Program
- •Basics of Perl Regular Expression Usage
- •Using the m// Operator
- •Using Other Regular Expression Delimiters
- •Matching Using Variable Substitution
- •Using the s/// Operator
- •Using s/// with the Global Modifier
- •Using s/// with the Default Variable
- •Using the split Operator
- •Using Quantifiers in Perl
- •Using Positional Metacharacters
- •Captured Groups in Perl
- •Using Back References in Perl
- •Using Alternation
- •Using Character Classes in Perl
- •Using Lookahead
- •Using Lookbehind
- •Escaping Metacharacters
- •A Simple Perl Regex Tester
- •Exercises
- •Index
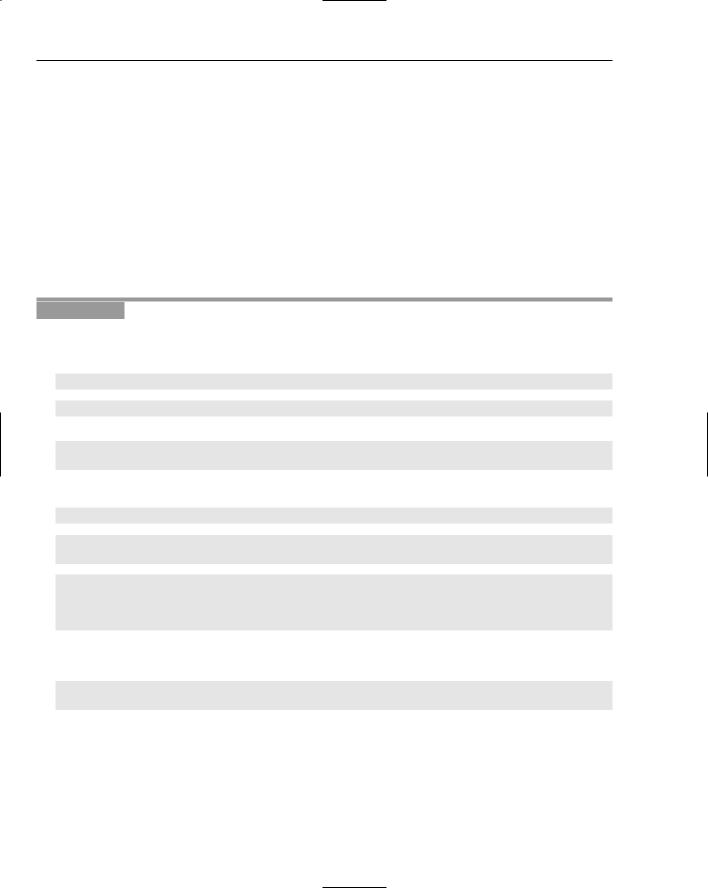
Chapter 25
The matches() Method
The matches() method attempts to match a regular expression pattern against the whole of the test string. It returns a boolean value of true if the whole test string matches the regular expression pattern.
The pattern() Method
The pattern() method takes no argument. It returns a Pattern object. The Pattern object contains the regular expression pattern that the Matcher object uses in matching.
The replaceAll() Method
The replaceAll() method replaces every occurrence of a character sequence in the test string that matches the regular expression pattern with a specified replacement string. The replacement string is the replaceAll() method’s sole argument. The return value is a String.
Try It Out |
Using the replaceAll() Method |
1.Type the following code in a text editor:
import java.util.regex.*;
public class replaceAll{
public static void main(String args[]){ myReplace(args[0]);
} // end main()
public static boolean myReplace(String testString){
String myMatch = “Star”;
Pattern myPattern = Pattern.compile(myMatch);
Matcher myMatcher = myPattern.matcher(testString);
String testResult = myMatcher.replaceAll(“Moon”);
System.out.println(“The test string is: \n’” + testString + “‘.”);
System.out.println();
if (testResult.length() > 0)
{
System.out.println(“After replacement the string is: \n’” + testResult + “‘.” );
}
else
{
System.out.println(“No match was found.”);
}
return true;
} // end myReplace()
}
2.Save the code as replaceAll.java; compile the code; and at the command line, type javac replaceAll.java.
642

Regular Expressions in Java
3.Run the code. At the command line, type java replaceAll “Star training is great. Star Training Company is well known.” Inspect the results, as shown in Figure 25-7. Notice that each occurrence of the character sequence Star has been replaced by the character sequence Moon.
Figure 25-7
How It Works
The code in the main() method calls the myReplace() method, passing a string entered on the command line:
myReplace(args[0]);
In the myReplace() method, a literal pattern, Star, is assigned to the myMatch variable:
String myMatch = “Star”;
Then a Pattern object and a Matcher object are created:
Pattern myPattern = Pattern.compile(myMatch);
Matcher myMatcher = myPattern.matcher(testString);
The replaceAll() method of the Matcher object is called with the replacement string Moon. The result is assigned to the testResult variable:
String testResult = myMatcher.replaceAll(“Moon”);
The original test string and a blank line as a separator are displayed:
System.out.println(“The test string is: \n’” + testString + “‘.”);
System.out.println();
The String class’s length() method is used to determine the length of testResult. If there is a match, the length of testResult will be greater than zero characters; therefore, the test for the if statement returns true, and the information about the replacement string is displayed:
if (testResult.length() > 0)
{
System.out.println(“After replacement the string is: \n’” + testResult + “‘.” );
}
643

Chapter 25
If the length of testResult is zero characters, a message is displayed indicating that no match was found:
else
{
System.out.println(“No match was found.”);
}
return true;
} // end myReplace()
The replaceFirst() Method
The replaceFirst() method replaces the first matching character sequence in the test string with a specified replacement string. The replacement string is the sole argument of the replaceFirst() method. The value returned is a String.
The reset() Method
The reset() method resets state information on a Matcher object. It can be used with no argument or with one argument. When the reset() method is used with no argument, the state information is reset. Any matching takes place from the beginning of the test string. The test string remains the same as previously. The value returned is a Matcher object.
When the reset() method is used with a single argument, the state information is reset. The reset() method’s argument is a String, which then becomes the test string for any future matching. The value returned is a Matcher object.
The start() Method
The start() method takes no argument. It returns an int value, which is the index of the first character in the most recent match.
The start() method can throw an IllegalStateException if matching has not started or if the most recent attempt at matching failed.
The PatternSyntaxException Class
A PatternSyntaxException object is an unchecked exception that indicates that there is an error in the syntax of a regular expression pattern.
The PatternSyntaxException class has methods that allow information about the exception to be accessed. The following table summarizes information about the methods of the PatternSyntax Exception class.
Method |
Description |
|
|
getDescription() |
Retrieves the description of the error |
getIndex() |
Retrieves the error index in the pattern |
getMessage() |
Returns a multiline string that contains the description, pattern, and index |
getPattern() |
Retrieves the regular expression pattern that caused the error |
644

Regular Expressions in Java
Metacharacters Suppor ted in the java.util.regex Package
The java.util.regex package supports an extensive range of metacharacters, which are briefly described in several tables in this section. Some of the metacharacters and character classes are described in more detail later in the section, with examples of how they can be used.
The following table summarizes many of the metacharacters supported in the Java java.util.regex package. The POSIX metacharacters supported in java.util.regex are listed in a later section in this chapter.
Metacharacter |
Description |
|
|
. (period character) |
Matches any character. May or may not match line-terminator |
|
characters. |
\d |
Matches a numeric digit. Equivalent to the character class [0-9]. |
\D |
Matches a character that is not a numeric digit. Equivalent to the |
|
character class [^0-9]. |
\s |
Matches a whitespace character. |
\S |
Matches any character that is not a whitespace character. |
\w |
Matches a word character. Equivalent to the character class |
|
[A-Za-z0-9_]. |
\W |
Matches a character that is not a word character. Equivalent to the |
|
negated character class [^A-Za-z0-9_]. |
[...] |
Character class. Matches any single character contained between the |
|
square brackets. |
[a-d[w-z]] |
The union of two character classes. Equivalent to [a-dw-z]. |
[a-m[h-z]] |
The intersection of two character classes. Equivalent to [h-m]. |
[a-z[^h-m]] |
The intersection of a character class and a negated character class. |
|
The overall effect is subtraction of one character class from another. |
|
Equivalent to [a-gn-z]. |
[^...] |
Negated character class. Matches any single character, except those |
|
characters contained between the square brackets. |
|
|
Using the \d Metacharacter
The \d metacharacter matches a single numeric digit. The following example attempts to match U.S. Zip codes. A simple pattern \d{5}-\d{4}* can match abbreviated and extended U.S. Zip codes.
645

Chapter 25
Try It Out |
sing the \d Metacharacter |
1.Type the following code into a text editor:
import java.util.regex.*;
public class MatchZip{
public static void main(String args[]) throws Exception{
String myTestString = “12345-1234 23456 45678 01234-1234”;
//Attempt to match US Zip codes.
//The pattern matches five numeric digits followed by a hyphen followed by four numeric digits.
String myRegex = “\\d{5}(-\\d{4})*”;
Pattern myPattern = Pattern.compile(myRegex);
Matcher myMatcher = myPattern.matcher(myTestString);
String myMatch = “”;
System.out.println(“The test string was ‘“ + myTestString + “‘.”);
System.out.println(“The pattern was ‘“ + myRegex + “‘.”); while (myMatcher.find())
{
myMatch = myMatcher.group();
System.out.println(“The match ‘“ + myMatch + “‘ was found.”); } // end while
if (myMatch == “”){
System.out.println(“There were no matches.”);
}// end if
}// end main()
}
2.Save the code as MatchZip.java.
3.Compile the code by typing javac MatchZip.java at the command line.
4.Run the code by typing java MatchZip at the command line, and inspect the results, as shown in Figure 25-8.
Figure 25-8
646