
- •Table of Contents
- •Chapter 1. Why Shell Programming?
- •2.1. Invoking the script
- •2.2. Preliminary Exercises
- •Part 2. Basics
- •Chapter 3. Exit and Exit Status
- •Chapter 4. Special Characters
- •Chapter 5. Introduction to Variables and Parameters
- •5.1. Variable Substitution
- •5.2. Variable Assignment
- •5.3. Bash Variables Are Untyped
- •5.4. Special Variable Types
- •Chapter 6. Quoting
- •Chapter 7. Tests
- •7.1. Test Constructs
- •7.2. File test operators
- •7.3. Comparison operators (binary)
- •7.4. Nested if/then Condition Tests
- •7.5. Testing Your Knowledge of Tests
- •Chapter 8. Operations and Related Topics
- •8.1. Operators
- •8.2. Numerical Constants
- •Part 3. Beyond the Basics
- •Chapter 9. Variables Revisited
- •9.1. Internal Variables
- •9.2. Manipulating Strings
- •9.2.1. Manipulating strings using awk
- •9.2.2. Further Discussion
- •9.3. Parameter Substitution
- •9.4. Typing variables: declare or typeset
- •9.5. Indirect References to Variables
- •9.6. $RANDOM: generate random integer
- •9.7. The Double Parentheses Construct
- •Chapter 10. Loops and Branches
- •10.1. Loops
- •10.2. Nested Loops
- •10.3. Loop Control
- •10.4. Testing and Branching
- •Chapter 11. Internal Commands and Builtins
- •11.1. Job Control Commands
- •Chapter 12. External Filters, Programs and Commands
- •12.1. Basic Commands
- •12.2. Complex Commands
- •12.3. Time / Date Commands
- •12.4. Text Processing Commands
- •12.5. File and Archiving Commands
- •12.6. Communications Commands
- •12.7. Terminal Control Commands
- •12.8. Math Commands
- •12.9. Miscellaneous Commands
- •Chapter 13. System and Administrative Commands
- •Chapter 14. Command Substitution
- •Chapter 15. Arithmetic Expansion
- •Chapter 16. I/O Redirection
- •16.1. Using exec
- •16.2. Redirecting Code Blocks
- •16.3. Applications
- •Chapter 17. Here Documents
- •Chapter 18. Recess Time
- •Part 4. Advanced Topics
- •Chapter 19. Regular Expressions
- •19.1. A Brief Introduction to Regular Expressions
- •19.2. Globbing
- •Chapter 20. Subshells
- •Chapter 21. Restricted Shells
- •Chapter 22. Process Substitution
- •Chapter 23. Functions
- •23.1. Complex Functions and Function Complexities
- •23.2. Local Variables
- •23.2.1. Local variables make recursion possible.
- •Chapter 24. Aliases
- •Chapter 25. List Constructs
- •Chapter 26. Arrays
- •Chapter 27. Files
- •Chapter 28. /dev and /proc
- •28.2. /proc
- •Chapter 29. Of Zeros and Nulls
- •Chapter 30. Debugging
- •Chapter 31. Options
- •Chapter 32. Gotchas
- •Chapter 33. Scripting With Style
- •33.1. Unofficial Shell Scripting Stylesheet
- •Chapter 34. Miscellany
- •34.2. Shell Wrappers
- •34.3. Tests and Comparisons: Alternatives
- •34.4. Optimizations
- •34.5. Assorted Tips
- •34.6. Oddities
- •34.7. Portability Issues
- •34.8. Shell Scripting Under Windows
- •Chapter 35. Bash, version 2
- •Chapter 36. Endnotes
- •36.1. Author's Note
- •36.2. About the Author
- •36.3. Tools Used to Produce This Book
- •36.3.1. Hardware
- •36.3.2. Software and Printware
- •36.4. Credits
- •Bibliography
- •Appendix A. Contributed Scripts
- •Appendix C. Exit Codes With Special Meanings
- •Appendix D. A Detailed Introduction to I/O and I/O Redirection
- •Appendix E. Localization
- •Appendix F. History Commands
- •Appendix G. A Sample .bashrc File
- •Appendix H. Converting DOS Batch Files to Shell Scripts
- •Appendix I. Exercises
- •Appendix J. Copyright
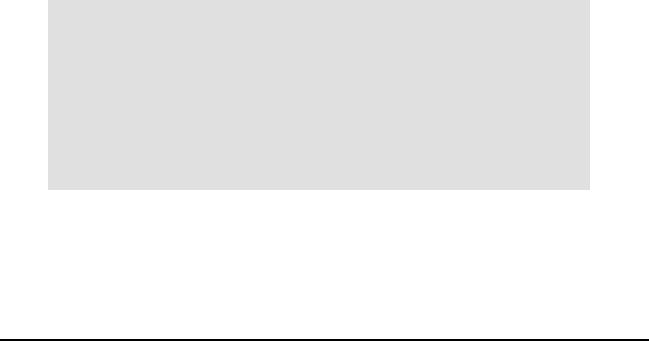
Advanced Bash−Scripting Guide
reliably in Bash scripts. One possible use for it is to have a script self−test whether it is interactive.
$!
PID (process id) of last job run in background
$_
Special variable set to last argument of previous command executed.
Example 9−8. underscore variable
#!/bin/bash |
|
echo $_ |
# /bin/bash |
# Just called /bin/bash |
to run the script. |
|
du >/dev/null |
# So no |
output from command. |
echo $_ |
# du |
|
ls −al |
# So no |
output from command. |
echo $_ |
# −al |
(last argument) |
: |
|
|
echo $_ |
# : |
|
$?
exit status of a command, function, or the script itself (see Example 23−3)
$$
process id of script, often used in scripts to construct temp file names (see Example A−8 , Example 30−5 , and Example 12−23)
9.2. Manipulating Strings
Bash supports a surprising number of string manipulation operations. Unfortunately, these tools lack a unified focus. Some are a subset of parameter substitution, and others fall under the functionality of the UNIX
expr command. This results in inconsistent command syntax and overlap of functionality, not to mention confusion.
String Length
${#string}
expr length $string
expr "$string" : '.*'
9.2. Manipulating Strings |
75 |

Advanced Bash−Scripting Guide
stringZ=abcABC123ABCabc |
|
|
echo ${#stringZ} |
# 15 |
|
echo `expr length $stringZ` |
# |
15 |
echo `expr "$stringZ" : '.*'` |
# |
15 |
Length of Matching Substring at Beginning of String
expr match "$string" '$substring'
$substring is a regular expression.
expr "$string" : '$substring'
$substring is a regular expression.
stringZ=abcABC123ABCabc |
|
|
|
# |
|−−−−−−| |
|
|
echo `expr match "$stringZ" 'abc[A−Z]*.2'` |
# |
8 |
|
echo `expr "$stringZ" : 'abc[A−Z]*.2'` |
# |
8 |
Index
expr index $string $substring
Numerical position in $string of first character in $substring that matches.
|
stringZ=abcABC123ABCabc |
|
|
|
|
|
|
echo `expr index "$stringZ" C12` |
# 6 |
|
|
|
|
|
|
# C position. |
|
|||
|
echo `expr index "$stringZ" 1c` |
# 3 |
|
|
|
|
|
# 'c' (in #3 position) matches before '1'. |
|
|
|
|
|
|
This is the near equivalent of strchr() in C. |
|
|
|
|
|
Substring Extraction |
|
|
|
|
|
|
${string:position} |
|
|
|
|
|
|
|
Extracts substring from $string at $position. |
|
|
|
|
|
|
If the string parameter is "*" or "@", then this extracts the |
positional parameters, [20] starting at |
||||
|
position. |
|
|
|
|
|
${string:position:length} |
|
|
|
|
|
|
|
Extracts $length characters of substring from $string at |
$position. |
stringZ=abcABC123ABCabc
#0123456789.....
#0−based indexing.
9.2. Manipulating Strings |
76 |

|
Advanced Bash−Scripting Guide |
|
|
|
|
echo ${stringZ:0} |
# abcABC123ABCabc |
|
echo ${stringZ:1} |
# bcABC123ABCabc |
|
echo ${stringZ:7} |
# 23ABCabc |
|
echo ${stringZ:7:3} |
# 23A |
|
|
# Three characters of substring. |
|
If the string parameter is "*" or "@", then this extracts a maximum of length positional parameters, starting at position.
echo ${*:2} |
# Echoes second and following positional parameters. |
echo ${@:2} |
# Same as above. |
echo ${*:2:3} |
# Echoes three positional parameters, starting at second. |
expr substr $string $position $length
Extracts $length characters from $string starting at $position.
stringZ=abcABC123ABCabc |
|
|
# |
123456789...... |
|
# |
1−based indexing. |
|
echo `expr substr $stringZ 1 2` |
# ab |
|
echo `expr substr $stringZ 4 3` |
# ABC |
expr match "$string" '\($substring\)'
Extracts $substring at beginning of $string, where $substring is a regular expression.
expr "$string" : '\($substring\)'
Extracts $substring at beginning of $string, where $substring is a regular expression.
stringZ=abcABC123ABCabc |
|
echo `expr match "$stringZ" '\(.[b−c]*[A−Z]..[0−9]\)'` |
# abcABC1 |
echo `expr "$stringZ" : '\(.[b−c]*[A−Z]..[0−9]\)'` |
# abcABC1 |
# Both of the above forms are equivalent. |
|
Substring Removal
${string#substring}
Strips shortest match of $substring from front of $string.
${string##substring}
Strips longest match of $substring from front of $string.
stringZ=abcABC123ABCabc |
|
|
|
# |
|−−−−| |
|
|
# |
|−−−−−−−−−−| |
|
|
echo ${stringZ#a*C} |
# |
123ABCabc |
|
# Strip out shortest match |
between 'a' and 'C'. |
||
|
|
|
|
9.2. Manipulating Strings |
77 |
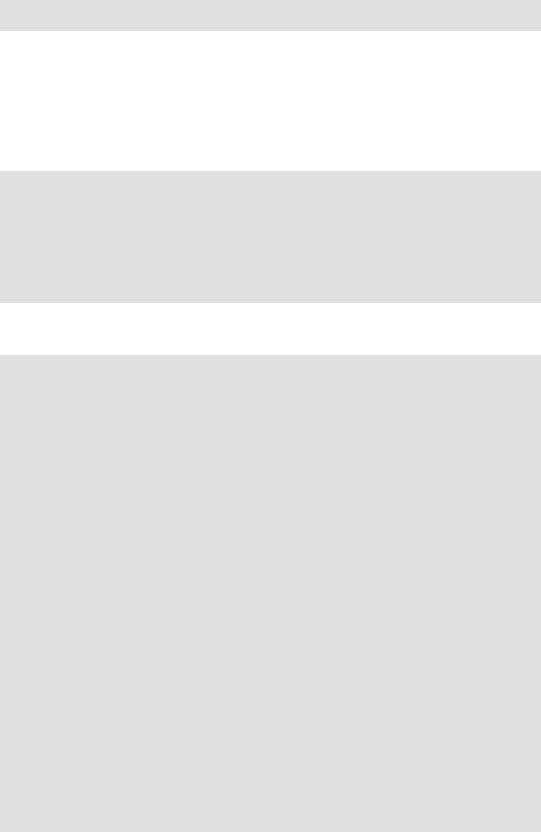
Advanced Bash−Scripting Guide
echo ${stringZ##a*C} |
# abc |
# Strip out longest match between 'a' and 'C'.
${string%substring}
Strips shortest match of $substring from back of $string.
${string%%substring}
Strips longest match of $substring from back of $string. |
|
stringZ=abcABC123ABCabc |
|
# |
|| |
# |
|−−−−−−−−−−−−| |
echo ${stringZ%b*c} |
# |
abcABC123ABCa |
||
# |
Strip out |
shortest match |
between 'b' and 'c', from back of $stringZ. |
|
echo ${stringZ%%b*c} |
# |
a |
||
# |
Strip out |
longest match between 'b' and 'c', from back of $stringZ. |
Example 9−9. Converting graphic file formats, with filename change
#!/bin/bash
#cvt.sh:
#Converts all the MacPaint image files in a directory to "pbm" format.
#Uses the "macptopbm" binary from the "netpbm" package,
#+ which is maintained by Brian Henderson (bryanh@giraffe−data.com).
#Netpbm is a standard part of most Linux distros.
OPERATION=macptopbm |
|
SUFFIX=pbm |
# New filename suffix. |
if [ −n "$1" ] |
|
then |
|
directory=$1 |
# If directory name given as a script argument... |
else |
|
directory=$PWD |
# Otherwise use current working directory. |
fi |
|
#Assumes all files in the target directory are MacPaint image files,
#+ with a ".mac" suffix.
for file in $directory/* |
# Filename globbing. |
do |
|
filename=${file%.*c} |
# Strip ".mac" suffix off filename |
#+ ('.*c' matches everything
#+ between '.' and 'c', inclusive). $OPERATION $file > $filename.$SUFFIX
# Redirect conversion to new filename.
# Delete original files after converting.
# Log what is happening to stdout.
78