
- •Using Your Sybex Electronic Book
- •Acknowledgments
- •Contents at a Glance
- •Introduction
- •Who Should Read This Book?
- •How About the Advanced Topics?
- •The Structure of the Book
- •How to Reach the Author
- •The Integrated Development Environment
- •The Start Page
- •Project Types
- •Your First VB Application
- •Making the Application More Robust
- •Making the Application More User-Friendly
- •The IDE Components
- •The IDE Menu
- •The Toolbox Window
- •The Solution Explorer
- •The Properties Window
- •The Output Window
- •The Command Window
- •The Task List Window
- •Environment Options
- •A Few Common Properties
- •A Few Common Events
- •A Few Common Methods
- •Building a Console Application
- •Summary
- •Building a Loan Calculator
- •How the Loan Application Works
- •Designing the User Interface
- •Programming the Loan Application
- •Validating the Data
- •Building a Math Calculator
- •Designing the User Interface
- •Programming the MathCalculator App
- •Adding More Features
- •Exception Handling
- •Taking the LoanCalculator to the Web
- •Working with Multiple Forms
- •Working with Multiple Projects
- •Executable Files
- •Distributing an Application
- •VB.NET at Work: Creating a Windows Installer
- •Finishing the Windows Installer
- •Running the Windows Installer
- •Verifying the Installation
- •Summary
- •Variables
- •Declaring Variables
- •Types of Variables
- •Converting Variable Types
- •User-Defined Data Types
- •Examining Variable Types
- •Why Declare Variables?
- •A Variable’s Scope
- •The Lifetime of a Variable
- •Constants
- •Arrays
- •Declaring Arrays
- •Initializing Arrays
- •Array Limits
- •Multidimensional Arrays
- •Dynamic Arrays
- •Arrays of Arrays
- •Variables as Objects
- •So, What’s an Object?
- •Formatting Numbers
- •Formatting Dates
- •Flow-Control Statements
- •Test Structures
- •Loop Structures
- •Nested Control Structures
- •The Exit Statement
- •Summary
- •Modular Coding
- •Subroutines
- •Functions
- •Arguments
- •Argument-Passing Mechanisms
- •Event-Handler Arguments
- •Passing an Unknown Number of Arguments
- •Named Arguments
- •More Types of Function Return Values
- •Overloading Functions
- •Summary
- •The Appearance of Forms
- •Properties of the Form Control
- •Placing Controls on Forms
- •Setting the TabOrder
- •VB.NET at Work: The Contacts Project
- •Anchoring and Docking
- •Loading and Showing Forms
- •The Startup Form
- •Controlling One Form from within Another
- •Forms vs. Dialog Boxes
- •VB.NET at Work: The MultipleForms Project
- •Designing Menus
- •The Menu Editor
- •Manipulating Menus at Runtime
- •Building Dynamic Forms at Runtime
- •The Form.Controls Collection
- •VB.NET at Work: The DynamicForm Project
- •Creating Event Handlers at Runtime
- •Summary
- •The TextBox Control
- •Basic Properties
- •Text-Manipulation Properties
- •Text-Selection Properties
- •Text-Selection Methods
- •Undoing Edits
- •VB.NET at Work: The TextPad Project
- •Capturing Keystrokes
- •The ListBox, CheckedListBox, and ComboBox Controls
- •Basic Properties
- •The Items Collection
- •VB.NET at Work: The ListDemo Project
- •Searching
- •The ComboBox Control
- •The ScrollBar and TrackBar Controls
- •The ScrollBar Control
- •The TrackBar Control
- •Summary
- •The Common Dialog Controls
- •Using the Common Dialog Controls
- •The Color Dialog Box
- •The Font Dialog Box
- •The Open and Save As Dialog Boxes
- •The Print Dialog Box
- •The RichTextBox Control
- •The RTF Language
- •Methods
- •Advanced Editing Features
- •Cutting and Pasting
- •Searching in a RichTextBox Control
- •Formatting URLs
- •VB.NET at Work: The RTFPad Project
- •Summary
- •What Is a Class?
- •Building the Minimal Class
- •Adding Code to the Minimal Class
- •Property Procedures
- •Customizing Default Members
- •Custom Enumerations
- •Using the SimpleClass in Other Projects
- •Firing Events
- •Shared Properties
- •Parsing a Filename String
- •Reusing the StringTools Class
- •Encapsulation and Abstraction
- •Inheritance
- •Inheriting Existing Classes
- •Polymorphism
- •The Shape Class
- •Object Constructors and Destructors
- •Instance and Shared Methods
- •Who Can Inherit What?
- •Parent Class Keywords
- •Derived Class Keyword
- •Parent Class Member Keywords
- •Derived Class Member Keyword
- •MyBase and MyClass
- •Summary
- •On Designing Windows Controls
- •Enhancing Existing Controls
- •Building the FocusedTextBox Control
- •Building Compound Controls
- •VB.NET at Work: The ColorEdit Control
- •VB.NET at Work: The Label3D Control
- •Raising Events
- •Using the Custom Control in Other Projects
- •VB.NET at Work: The Alarm Control
- •Designing Irregularly Shaped Controls
- •Designing Owner-Drawn Menus
- •Designing Owner-Drawn ListBox Controls
- •Using ActiveX Controls
- •Summary
- •Programming Word
- •Objects That Represent Text
- •The Documents Collection and the Document Object
- •Spell-Checking Documents
- •Programming Excel
- •The Worksheets Collection and the Worksheet Object
- •The Range Object
- •Using Excel as a Math Parser
- •Programming Outlook
- •Retrieving Information
- •Recursive Scanning of the Contacts Folder
- •Summary
- •Advanced Array Topics
- •Sorting Arrays
- •Searching Arrays
- •Other Array Operations
- •Array Limitations
- •The ArrayList Collection
- •Creating an ArrayList
- •Adding and Removing Items
- •The HashTable Collection
- •VB.NET at Work: The WordFrequencies Project
- •The SortedList Class
- •The IEnumerator and IComparer Interfaces
- •Enumerating Collections
- •Custom Sorting
- •Custom Sorting of a SortedList
- •The Serialization Class
- •Serializing Individual Objects
- •Serializing a Collection
- •Deserializing Objects
- •Summary
- •Handling Strings and Characters
- •The Char Class
- •The String Class
- •The StringBuilder Class
- •VB.NET at Work: The StringReversal Project
- •VB.NET at Work: The CountWords Project
- •Handling Dates
- •The DateTime Class
- •The TimeSpan Class
- •VB.NET at Work: Timing Operations
- •Summary
- •Accessing Folders and Files
- •The Directory Class
- •The File Class
- •The DirectoryInfo Class
- •The FileInfo Class
- •The Path Class
- •VB.NET at Work: The CustomExplorer Project
- •Accessing Files
- •The FileStream Object
- •The StreamWriter Object
- •The StreamReader Object
- •Sending Data to a File
- •The BinaryWriter Object
- •The BinaryReader Object
- •VB.NET at Work: The RecordSave Project
- •The FileSystemWatcher Component
- •Properties
- •Events
- •VB.NET at Work: The FileSystemWatcher Project
- •Summary
- •Displaying Images
- •The Image Object
- •Exchanging Images through the Clipboard
- •Drawing with GDI+
- •The Basic Drawing Objects
- •Drawing Shapes
- •Drawing Methods
- •Gradients
- •Coordinate Transformations
- •Specifying Transformations
- •VB.NET at Work: Plotting Functions
- •Bitmaps
- •Specifying Colors
- •Defining Colors
- •Processing Bitmaps
- •Summary
- •The Printing Objects
- •PrintDocument
- •PrintDialog
- •PageSetupDialog
- •PrintPreviewDialog
- •PrintPreviewControl
- •Printer and Page Properties
- •Page Geometry
- •Printing Examples
- •Printing Tabular Data
- •Printing Plain Text
- •Printing Bitmaps
- •Using the PrintPreviewControl
- •Summary
- •Examining the Advanced Controls
- •How Tree Structures Work
- •The ImageList Control
- •The TreeView Control
- •Adding New Items at Design Time
- •Adding New Items at Runtime
- •Assigning Images to Nodes
- •Scanning the TreeView Control
- •The ListView Control
- •The Columns Collection
- •The ListItem Object
- •The Items Collection
- •The SubItems Collection
- •Summary
- •Types of Errors
- •Design-Time Errors
- •Runtime Errors
- •Logic Errors
- •Exceptions and Structured Exception Handling
- •Studying an Exception
- •Getting a Handle on this Exception
- •Finally (!)
- •Customizing Exception Handling
- •Throwing Your Own Exceptions
- •Debugging
- •Breakpoints
- •Stepping Through
- •The Local and Watch Windows
- •Summary
- •Basic Concepts
- •Recursion in Real Life
- •A Simple Example
- •Recursion by Mistake
- •Scanning Folders Recursively
- •Describing a Recursive Procedure
- •Translating the Description to Code
- •The Stack Mechanism
- •Stack Defined
- •Recursive Programming and the Stack
- •Passing Arguments through the Stack
- •Special Issues in Recursive Programming
- •Knowing When to Use Recursive Programming
- •Summary
- •MDI Applications: The Basics
- •Building an MDI Application
- •Built-In Capabilities of MDI Applications
- •Accessing Child Forms
- •Ending an MDI Application
- •A Scrollable PictureBox
- •Summary
- •What Is a Database?
- •Relational Databases
- •Exploring the Northwind Database
- •Exploring the Pubs Database
- •Understanding Relations
- •The Server Explorer
- •Working with Tables
- •Relationships, Indices, and Constraints
- •Structured Query Language
- •Executing SQL Statements
- •Selection Queries
- •Calculated Fields
- •SQL Joins
- •Action Queries
- •The Query Builder
- •The Query Builder Interface
- •SQL at Work: Calculating Sums
- •SQL at Work: Counting Rows
- •Limiting the Selection
- •Parameterized Queries
- •Calculated Columns
- •Specifying Left, Right, and Inner Joins
- •Stored Procedures
- •Summary
- •How About XML?
- •Creating a DataSet
- •The DataGrid Control
- •Data Binding
- •VB.NET at Work: The ViewEditCustomers Project
- •Binding Complex Controls
- •Programming the DataAdapter Object
- •The Command Objects
- •The Command and DataReader Objects
- •VB.NET at Work: The DataReader Project
- •VB.NET at Work: The StoredProcedure Project
- •Summary
- •The Structure of a DataSet
- •Navigating the Tables of a DataSet
- •Updating DataSets
- •The DataForm Wizard
- •Handling Identity Fields
- •Transactions
- •Performing Update Operations
- •Updating Tables Manually
- •Building and Using Custom DataSets
- •Summary
- •An HTML Primer
- •HTML Code Elements
- •Server-Client Interaction
- •The Structure of HTML Documents
- •URLs and Hyperlinks
- •The Basic HTML Tags
- •Inserting Graphics
- •Tables
- •Forms and Controls
- •Processing Requests on the Server
- •Building a Web Application
- •Interacting with a Web Application
- •Maintaining State
- •The Web Controls
- •The ASP.NET Objects
- •The Page Object
- •The Response Object
- •The Request Object
- •The Server Object
- •Using Cookies
- •Handling Multiple Forms in Web Applications
- •Summary
- •The Data-Bound Web Controls
- •Simple Data Binding
- •Binding to DataSets
- •Is It a Grid, or a Table?
- •Getting Orders on the Web
- •The Forms of the ProductSearch Application
- •Paging Large DataSets
- •Customizing the Appearance of the DataGrid Control
- •Programming the Select Button
- •Summary
- •How to Serve the Web
- •Building a Web Service
- •Consuming the Web Service
- •Maintaining State in Web Services
- •A Data-Driven Web Service
- •Consuming the Products Web Service in VB
- •Summary
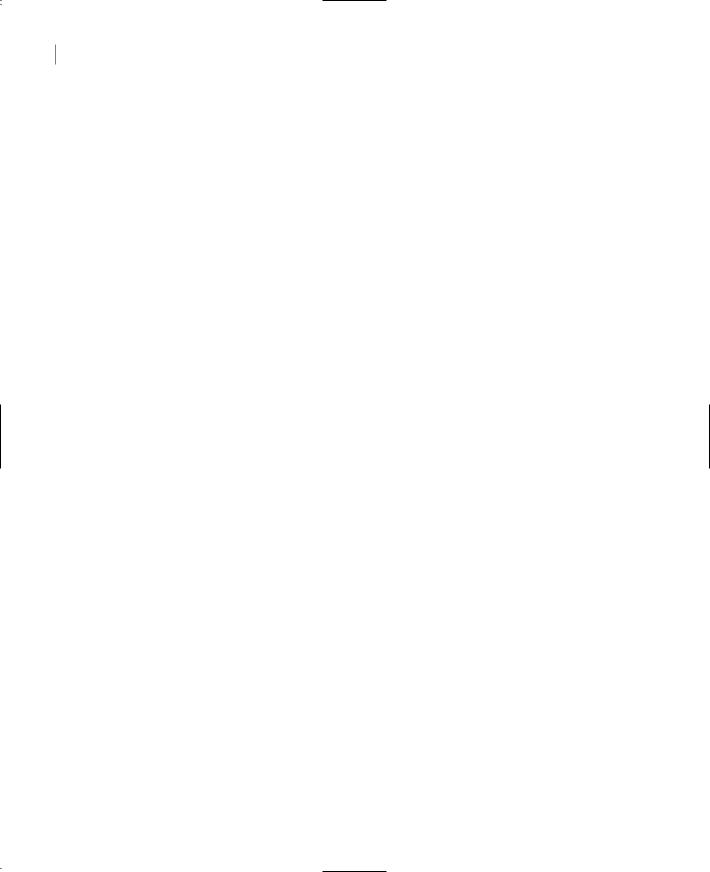
584 Chapter 13 WORKING WITH FOLDERS AND FILES
The syntax of the OpenRead method is:
FStream = fileObj.OpenRead(path)
where fileObj is a properly initialized File variable. The OpenRead method is equivalent to opening an existing file with read-only access with the Open method.
OpenText
This method opens an existing text file for reading and returns a StreamReader object associated with this file. Its syntax is
FStream = File.OpenText(path)
Why do we need an OpenText method in addition to the Open, OpenRead, and OpenWrite methods? The answer is that text can be stored in different formats. It can be plain text (UTF-8 encoding), ASCII text, or Unicode text. The StreamReader object associated with the text file will perform the necessary conversions, and you will always read the correct text from the file. The default encoding for the OpenText method is UTF-8.
OpenWrite
This method opens an existing file in write mode and returns a StreamWriter object associated with this file. You can use this stream to write to the file, as you will see later in this chapter.
The syntax of the OpenRead method is:
FStream = File.OpenWrite(path)
where path is the path of the file.
This ends our discussion of the Directory and File objects, which are the two major objects for manipulating files and folders. In the following section, I will present the DirectoryInfo and FileInfo classes briefly, and then we’ll build an application that puts together much of the information presented so far.
The DirectoryInfo Class
The DirectoryInfo and FileInfo classes are similar to the Directory and File classes, but they must be instantiated before they are used. Their constructors specify the folder or file they will act upon, and you don’t have to specify a folder or file when you call their methods.
To create a new instance of the DirectoryInfo class that references a specific folder, supply the folder’s path in the class’s constructor:
Dim DI As New DirectoryInfo(path)
Methods
The members of the DirectoryInfo class are equivalent to the members of the Directory class, and you will recognize them as soon as you see them in the IntelliSense drop-down list. Here are a couple of methods that are unique to the DirectoryInfo class.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

ACCESSING FOLDERS AND FILES 585
CreateSubdirectory
This method creates a subfolder under the folder specified by the current instance of the class, and its syntax is
CreateSubdirectory(path)
The CreateSubdirectory method returns a DirectoryInfo object that represents the new subfolder. The path argument need not be a single folder’s name. If you specified multiple nested folders, the CreateSubdirectory method will create the appropriate hierarchy, similar to the CreateDirectory method of the Directory class.
GetFileSystemInfos
This method returns an array of FileSystemInfo objects, one for each item in the folder referenced by the current instance of the class. The items can be either folders or files. To retrieve information about all the entries in a folder, create an instance of the DirectoryInfo class and then call its GetFileSystemInfos method:
Dim DI As New DirectoryInfo(path) Dim itemsInfo() As FileSystemInfo itemsInfo = DI.GetFileSystemInfos()
You can also specify an optional search pattern as argument when you call this method:
itemsInfo = DI.GetFileSystemInfos(pattern)
The FileSystemInfo objects expose a few properties, which are not new to you. The Name, FullName, and Extension return a file’s or folder’s name or full path or a file’s extension. The CreationTime, LastAccessTime, and LastWriteTime are also properties of the FileSystemInfo object, as well as the Attributes property.
You will notice that there are no properties that determine whether the current item is a folder or a file. To find out the type of an item, use the Directory member of the Attributes property:
If itemsInfo(i).Attributes And FileAttributes.Directory Then
{current item is a folder }
Else
{current item is a file } End If
The code in Listing 13.7 retrieves all the items in the C:\Program Files folder and prints their name along with the FOLDER and FILE characterization.
Listing 13.7: Processing a Folder’s Items with the FileSystemInfo Object
Dim path As String = “C:\Program Files” Dim DI As New DirectoryInfo(path)
Dim itemsInfo() As FileSystemInfo itemsInfo = DI.GetFileSystemInfos() Dim item As FileSystemInfo
For Each item In itemsInfo
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

586 Chapter 13 WORKING WITH FOLDERS AND FILES
If item.Attributes And FileAttributes.Directory Then
Console.Write(“FOLDER “)
Else
Console.Write(“FILE “)
End If
Console.WriteLine(item.Name)
Next
Notice the similarities and differences between the GetFileSystemInfos method of the DirectoryInfo class and the GetFileSystemEntries of the Directory object. GetFileSystemInfos returns an array of objects that contains information about the current item (file or folder). GetFileSystemEntries returns an array of strings (the names of the folders and files).
The FileInfo Class
The FileInfo class exposes many properties and methods, which are equivalent to the members of the File class, so I’m not going to repeat all of them here. The Copy/Delete/Move methods allow you to manipulate the file represented by the current instance of the FileInfo class, similar to the methods by the same name of the File class. Although there’s substantial overlap between the members of the FileInfo and File classes, the difference is that with FileInfo you don’t have to specify a path. Its members act on the file represented by the current instance of the FileInfo class.
Properties
The FileInfo object exposes a few rather trivial properties, which are mentioned briefly here.
Length
This property returns the size of the file represented by the FileInfo object in bytes. The File class doesn’t provide an equivalent property or method.
CreationTime, LastAccessTime, LastWriteTime
These properties return a date value which is the date on which the file was created, accessed for the last time, or written to for the last time. They are equivalent to the methods of the File object by the same name and the “Get” prefix.
Name, FullName, Extension
These properties return the filename, full path, and extension of the file represented by the current instance of the FileInfo class. They have no equivalents in the File class, because the File class’s methods require that you specify the path of the file, so its path and extension are known.
Methods
The FileInfo object exposes methods for manipulating files, and most of them are equivalent to the methods of the File object.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

ACCESSING FOLDERS AND FILES 587
CopyTo, MoveTo
These two methods copy and move the file represented by the current instance of the FileInfo class. Both methods accept a single argument, which is the destination of the operation (the path to which the file will be copied or moved). If the destination file exists already, you can overwrite it by specifying a second optional argument, which has a True/False value:
FileInfo.CopyTo(path, force)
Both methods return an instance of the FileInfo class, which represents the new file—if the operation completed successfully.
Directory
This method returns a DirectoryInfo value that contains information about the file’s parent directory.
DirectoryName
This method returns a string with the file’s parent directory’s name. The following statements return the two (identical) strings shown in bold:
Dim FI As FileInfo
FI = New FileInfo(“c:\folder1\folder2\folder3\test.txt”) Console.WriteLine(FI.Directory().FullName) c:\folder1\folder2\folder3
Console.WriteLine(FI.DirectoryName())
c:\folder1\folder2\folder3
Of course, the Directory method returns an object, which you can use to retrieve other properties of the parent folder.
The Path Class
The Path class contains an interesting collection of methods, which you can think of as utilities. The Path class’s methods perform simple tasks such as retrieving a file’s name and extension, returning the full path description of a relative path, and so on. The Path class’s members require that you specify the path on which they will act. In other words, there’s no constructor for the Path class that would allow you instantiate a Path object to represent a specific path.
Properties
The Path class exposes the following properties. Notice that none of these members apply to a specific path; they’re general properties that return settings of the operating system.
AltDirectorySeparatorChar
This property returns an alternate directory separator character. For Windows 2000, the AltDirectorySeparatorChar property returns the slash character (/).
DirectorySeparatorChar
This property returns the directory separator character. For Windows 2000, the DirectorySeparatorChar returns the backslash character (\).
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

588 Chapter 13 WORKING WITH FOLDERS AND FILES
InvalidPathChars
This property returns the list of invalid characters in a path as an array of characters. The following statements print the invalid path characters to the Output window; their output is shown in bold below the code:
Dim p As Path
Dim invalidPathChars() As Char invalidPathChars = p.InvalidPathChars Dim c As Char
For Each c In invalidPathChars Console.Write(c & vbtab)
Next
/ \ “ < > |
You can use these characters to validate user input, or pathnames read from a file. If you have a choice, let the user select the files through the Open dialog box, so that their pathnames will always be valid.
PathSeparator
This property returns separator character that may appear between multiple paths. For Windows 2000, this character is the semicolon (;).
VolumeSeparatorChar
This property returns the volume separator character. For Windows 2000, this character is the colon (:).
Methods
The most useful methods exposed by the Path class are like utilities for manipulating file and pathnames, and they described in the following sections. Notice that the methods of the Path class are shared: you must specify the path on which they will act.
ChangeExtension
This method changes the extension of a file, and its syntax is
newExtension = Path.ChangeExtension(path, extension)
The return value is the new extension of the file (a string value). The first argument is the file’s path, and the second argument is the file’s new extension. If you want to remove the file’s extension, set the second argument to Nothing. The following statement changes the extension of the specified file from “BIN” to “DAT”:
Dim path As String = “c:\My Documents\NewSales.bin”
Dim newExt As String = “.dat”
Path.ChangeExtension(path, newExt)
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

ACCESSING FOLDERS AND FILES 589
Combine
This method combines two path specifications into one, and its syntax is
newPath = Path.Combine(path1, path2)
Use this method to combine a folder path with a file path. The following expression will return the string shown in bold:
Path.Combine(“c:\textFiles”, “test.txt”) c:\textFiles\test.txt
Notice that the Combine method inserted the separator, as needed. It’s a simple operation, but if you had to code it yourself, you’d have to examine each path and determine whether a separator must be inserted.
GetDirectoryName
This method returns the directory name of a path. The statement
Path.GetDirectoryName(“C:\folder1\folder2\folder3\Test.txt”)
will return the string
C:\folder1\folder2\folder3
GetFileName, GetFileNameWithoutExtension
These two methods return the filename in a path, with and without its extension, respectively.
GetFullPath
This method returns the full path of the specified path; you can use it to convert relative pathnames to fully qualified pathnames. The following statement returned the string shown in bold on my computer (it will be quite different on your computer, depending on the current directory):
Console.WriteLine(Path.GetFullPath(“..\..\Test.txt”))
C:\Mastering VB.NET\Chapters\Chapter 13\Projects\Test.txt
The pathname passed to the method as argument need not exist. The GetFullPath method will return the fully qualified pathname of a nonexisting file, as long as the path doesn’t contain invalid characters.
GetTempFile, GetTempPath
The GetTempFile method returns a unique filename, which you can use as temporary storage area from within your application. The name of temporary file can be anything, since no user will ever access it. In addition, the GetTempFile method creates a zero-length file on the disk, which you can open with the Open method. A typical temporary filename is the following:
C:\DOCUME~1\TOOLKI~1\LOCALS~1\Temp\tmp105.tmp
which was returned by the following statement on my system:
Console.WriteLine(File.GetTempFile)
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |