
- •Using Your Sybex Electronic Book
- •Acknowledgments
- •Contents at a Glance
- •Introduction
- •Who Should Read This Book?
- •How About the Advanced Topics?
- •The Structure of the Book
- •How to Reach the Author
- •The Integrated Development Environment
- •The Start Page
- •Project Types
- •Your First VB Application
- •Making the Application More Robust
- •Making the Application More User-Friendly
- •The IDE Components
- •The IDE Menu
- •The Toolbox Window
- •The Solution Explorer
- •The Properties Window
- •The Output Window
- •The Command Window
- •The Task List Window
- •Environment Options
- •A Few Common Properties
- •A Few Common Events
- •A Few Common Methods
- •Building a Console Application
- •Summary
- •Building a Loan Calculator
- •How the Loan Application Works
- •Designing the User Interface
- •Programming the Loan Application
- •Validating the Data
- •Building a Math Calculator
- •Designing the User Interface
- •Programming the MathCalculator App
- •Adding More Features
- •Exception Handling
- •Taking the LoanCalculator to the Web
- •Working with Multiple Forms
- •Working with Multiple Projects
- •Executable Files
- •Distributing an Application
- •VB.NET at Work: Creating a Windows Installer
- •Finishing the Windows Installer
- •Running the Windows Installer
- •Verifying the Installation
- •Summary
- •Variables
- •Declaring Variables
- •Types of Variables
- •Converting Variable Types
- •User-Defined Data Types
- •Examining Variable Types
- •Why Declare Variables?
- •A Variable’s Scope
- •The Lifetime of a Variable
- •Constants
- •Arrays
- •Declaring Arrays
- •Initializing Arrays
- •Array Limits
- •Multidimensional Arrays
- •Dynamic Arrays
- •Arrays of Arrays
- •Variables as Objects
- •So, What’s an Object?
- •Formatting Numbers
- •Formatting Dates
- •Flow-Control Statements
- •Test Structures
- •Loop Structures
- •Nested Control Structures
- •The Exit Statement
- •Summary
- •Modular Coding
- •Subroutines
- •Functions
- •Arguments
- •Argument-Passing Mechanisms
- •Event-Handler Arguments
- •Passing an Unknown Number of Arguments
- •Named Arguments
- •More Types of Function Return Values
- •Overloading Functions
- •Summary
- •The Appearance of Forms
- •Properties of the Form Control
- •Placing Controls on Forms
- •Setting the TabOrder
- •VB.NET at Work: The Contacts Project
- •Anchoring and Docking
- •Loading and Showing Forms
- •The Startup Form
- •Controlling One Form from within Another
- •Forms vs. Dialog Boxes
- •VB.NET at Work: The MultipleForms Project
- •Designing Menus
- •The Menu Editor
- •Manipulating Menus at Runtime
- •Building Dynamic Forms at Runtime
- •The Form.Controls Collection
- •VB.NET at Work: The DynamicForm Project
- •Creating Event Handlers at Runtime
- •Summary
- •The TextBox Control
- •Basic Properties
- •Text-Manipulation Properties
- •Text-Selection Properties
- •Text-Selection Methods
- •Undoing Edits
- •VB.NET at Work: The TextPad Project
- •Capturing Keystrokes
- •The ListBox, CheckedListBox, and ComboBox Controls
- •Basic Properties
- •The Items Collection
- •VB.NET at Work: The ListDemo Project
- •Searching
- •The ComboBox Control
- •The ScrollBar and TrackBar Controls
- •The ScrollBar Control
- •The TrackBar Control
- •Summary
- •The Common Dialog Controls
- •Using the Common Dialog Controls
- •The Color Dialog Box
- •The Font Dialog Box
- •The Open and Save As Dialog Boxes
- •The Print Dialog Box
- •The RichTextBox Control
- •The RTF Language
- •Methods
- •Advanced Editing Features
- •Cutting and Pasting
- •Searching in a RichTextBox Control
- •Formatting URLs
- •VB.NET at Work: The RTFPad Project
- •Summary
- •What Is a Class?
- •Building the Minimal Class
- •Adding Code to the Minimal Class
- •Property Procedures
- •Customizing Default Members
- •Custom Enumerations
- •Using the SimpleClass in Other Projects
- •Firing Events
- •Shared Properties
- •Parsing a Filename String
- •Reusing the StringTools Class
- •Encapsulation and Abstraction
- •Inheritance
- •Inheriting Existing Classes
- •Polymorphism
- •The Shape Class
- •Object Constructors and Destructors
- •Instance and Shared Methods
- •Who Can Inherit What?
- •Parent Class Keywords
- •Derived Class Keyword
- •Parent Class Member Keywords
- •Derived Class Member Keyword
- •MyBase and MyClass
- •Summary
- •On Designing Windows Controls
- •Enhancing Existing Controls
- •Building the FocusedTextBox Control
- •Building Compound Controls
- •VB.NET at Work: The ColorEdit Control
- •VB.NET at Work: The Label3D Control
- •Raising Events
- •Using the Custom Control in Other Projects
- •VB.NET at Work: The Alarm Control
- •Designing Irregularly Shaped Controls
- •Designing Owner-Drawn Menus
- •Designing Owner-Drawn ListBox Controls
- •Using ActiveX Controls
- •Summary
- •Programming Word
- •Objects That Represent Text
- •The Documents Collection and the Document Object
- •Spell-Checking Documents
- •Programming Excel
- •The Worksheets Collection and the Worksheet Object
- •The Range Object
- •Using Excel as a Math Parser
- •Programming Outlook
- •Retrieving Information
- •Recursive Scanning of the Contacts Folder
- •Summary
- •Advanced Array Topics
- •Sorting Arrays
- •Searching Arrays
- •Other Array Operations
- •Array Limitations
- •The ArrayList Collection
- •Creating an ArrayList
- •Adding and Removing Items
- •The HashTable Collection
- •VB.NET at Work: The WordFrequencies Project
- •The SortedList Class
- •The IEnumerator and IComparer Interfaces
- •Enumerating Collections
- •Custom Sorting
- •Custom Sorting of a SortedList
- •The Serialization Class
- •Serializing Individual Objects
- •Serializing a Collection
- •Deserializing Objects
- •Summary
- •Handling Strings and Characters
- •The Char Class
- •The String Class
- •The StringBuilder Class
- •VB.NET at Work: The StringReversal Project
- •VB.NET at Work: The CountWords Project
- •Handling Dates
- •The DateTime Class
- •The TimeSpan Class
- •VB.NET at Work: Timing Operations
- •Summary
- •Accessing Folders and Files
- •The Directory Class
- •The File Class
- •The DirectoryInfo Class
- •The FileInfo Class
- •The Path Class
- •VB.NET at Work: The CustomExplorer Project
- •Accessing Files
- •The FileStream Object
- •The StreamWriter Object
- •The StreamReader Object
- •Sending Data to a File
- •The BinaryWriter Object
- •The BinaryReader Object
- •VB.NET at Work: The RecordSave Project
- •The FileSystemWatcher Component
- •Properties
- •Events
- •VB.NET at Work: The FileSystemWatcher Project
- •Summary
- •Displaying Images
- •The Image Object
- •Exchanging Images through the Clipboard
- •Drawing with GDI+
- •The Basic Drawing Objects
- •Drawing Shapes
- •Drawing Methods
- •Gradients
- •Coordinate Transformations
- •Specifying Transformations
- •VB.NET at Work: Plotting Functions
- •Bitmaps
- •Specifying Colors
- •Defining Colors
- •Processing Bitmaps
- •Summary
- •The Printing Objects
- •PrintDocument
- •PrintDialog
- •PageSetupDialog
- •PrintPreviewDialog
- •PrintPreviewControl
- •Printer and Page Properties
- •Page Geometry
- •Printing Examples
- •Printing Tabular Data
- •Printing Plain Text
- •Printing Bitmaps
- •Using the PrintPreviewControl
- •Summary
- •Examining the Advanced Controls
- •How Tree Structures Work
- •The ImageList Control
- •The TreeView Control
- •Adding New Items at Design Time
- •Adding New Items at Runtime
- •Assigning Images to Nodes
- •Scanning the TreeView Control
- •The ListView Control
- •The Columns Collection
- •The ListItem Object
- •The Items Collection
- •The SubItems Collection
- •Summary
- •Types of Errors
- •Design-Time Errors
- •Runtime Errors
- •Logic Errors
- •Exceptions and Structured Exception Handling
- •Studying an Exception
- •Getting a Handle on this Exception
- •Finally (!)
- •Customizing Exception Handling
- •Throwing Your Own Exceptions
- •Debugging
- •Breakpoints
- •Stepping Through
- •The Local and Watch Windows
- •Summary
- •Basic Concepts
- •Recursion in Real Life
- •A Simple Example
- •Recursion by Mistake
- •Scanning Folders Recursively
- •Describing a Recursive Procedure
- •Translating the Description to Code
- •The Stack Mechanism
- •Stack Defined
- •Recursive Programming and the Stack
- •Passing Arguments through the Stack
- •Special Issues in Recursive Programming
- •Knowing When to Use Recursive Programming
- •Summary
- •MDI Applications: The Basics
- •Building an MDI Application
- •Built-In Capabilities of MDI Applications
- •Accessing Child Forms
- •Ending an MDI Application
- •A Scrollable PictureBox
- •Summary
- •What Is a Database?
- •Relational Databases
- •Exploring the Northwind Database
- •Exploring the Pubs Database
- •Understanding Relations
- •The Server Explorer
- •Working with Tables
- •Relationships, Indices, and Constraints
- •Structured Query Language
- •Executing SQL Statements
- •Selection Queries
- •Calculated Fields
- •SQL Joins
- •Action Queries
- •The Query Builder
- •The Query Builder Interface
- •SQL at Work: Calculating Sums
- •SQL at Work: Counting Rows
- •Limiting the Selection
- •Parameterized Queries
- •Calculated Columns
- •Specifying Left, Right, and Inner Joins
- •Stored Procedures
- •Summary
- •How About XML?
- •Creating a DataSet
- •The DataGrid Control
- •Data Binding
- •VB.NET at Work: The ViewEditCustomers Project
- •Binding Complex Controls
- •Programming the DataAdapter Object
- •The Command Objects
- •The Command and DataReader Objects
- •VB.NET at Work: The DataReader Project
- •VB.NET at Work: The StoredProcedure Project
- •Summary
- •The Structure of a DataSet
- •Navigating the Tables of a DataSet
- •Updating DataSets
- •The DataForm Wizard
- •Handling Identity Fields
- •Transactions
- •Performing Update Operations
- •Updating Tables Manually
- •Building and Using Custom DataSets
- •Summary
- •An HTML Primer
- •HTML Code Elements
- •Server-Client Interaction
- •The Structure of HTML Documents
- •URLs and Hyperlinks
- •The Basic HTML Tags
- •Inserting Graphics
- •Tables
- •Forms and Controls
- •Processing Requests on the Server
- •Building a Web Application
- •Interacting with a Web Application
- •Maintaining State
- •The Web Controls
- •The ASP.NET Objects
- •The Page Object
- •The Response Object
- •The Request Object
- •The Server Object
- •Using Cookies
- •Handling Multiple Forms in Web Applications
- •Summary
- •The Data-Bound Web Controls
- •Simple Data Binding
- •Binding to DataSets
- •Is It a Grid, or a Table?
- •Getting Orders on the Web
- •The Forms of the ProductSearch Application
- •Paging Large DataSets
- •Customizing the Appearance of the DataGrid Control
- •Programming the Select Button
- •Summary
- •How to Serve the Web
- •Building a Web Service
- •Consuming the Web Service
- •Maintaining State in Web Services
- •A Data-Driven Web Service
- •Consuming the Products Web Service in VB
- •Summary

600 Chapter 13 WORKING WITH FOLDERS AND FILES
WriteLine(data)
This method is identical to the Write method, but it appends a line break after saving the data to the file (the same as the methods of the Console object by the same name).
You will find examples on using the StreamWriter object after we discuss the methods of the StreamReader object.
The StreamReader Object
The StreamReader object provides the necessary methods for reading from a text file. It exposes methods that match those of the StreamWriter object, methods that can read the information written to the file through the StreamWriter’s Write and WriteLine methods.
The StreamReader object’s constructor is overloaded. You can specify the FileStream object it will use to write data to the file, the encoding scheme, and the buffer size. The simplest form of the constructor is
Dim SR As New StreamReader(FS)
This declaration associates the SR variable with the file on which the FS FileStream object was created. This is the most common form of the StreamReader object’s constructor. To prepare your application for reading the contents of the file C:\My Documents\Meeting.txt, use the following statements:
Dim FS As FileStream
Dim SR As StreamReader
FS = New FileStream(“c:\My Documents\Meeting.txt”, _
System.IO.FileMode.OpenOrCreate, System.IO.FileAccess.Write)
SR = New StreamReader(FS)
You can also create a new StreamReader object directly on a file, with the following form of the constructor:
Dim SR As New StreamReader(path)
To create a StreamReader object and associate it with the file of the previous example, use the statement
Dim SR As New StreamReader(“c:\My Documents\Meeting.txt”)
With both forms of the constructor, you can specify the character encoding with a second argument:
Dim SR As New StreamReader(FS, encoding)
Dim SR As New StreamReader(path, encoding)
You can also specify a third argument with the size of the buffer to be used with the file input/output operations:
Dim SR As New StreamReader(FS, encoding, bufferSize)
Dim SR As New StreamReader(path, encoding, bufferSize)
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

ACCESSING FILES 601
Methods
Close
This method closes the current instance of StreamReader and releases any system resources associated with the StreamReader.
Peek
This method returns the next character without actually removing it from the input stream. The Peek method doesn’t reposition the current position in the stream. If there are no more characters left in the stream, the value –1 is returned. The Peek method will also return –1 if the current stream doesn’t allow peeking.
Read
This method reads a number of characters from the StreamReader object to which it’s applied and returns the number of characters read. This value is usually the same as the number of characters you specified, unless there aren’t as many characters in the file. If you have reached the end of the stream (which is the end of the file), the method returns the value –1. The syntax of the Read method is
charsRead = Read(chars, startIndex, count)
where count is the number of characters to be read. The characters are stored in the chars array of characters, starting at the index specified by the second argument. The return value is the number of characters actually read from the file.
A simpler form of the Read method reads the next character from the stream and returns it as an integer value:
Dim newChar As Integer newChar = SR.Read()
where SR is a properly declared StreamReader object.
ReadBlock
This method reads a number of characters from a text file and stores them in a Character array. It accepts the same arguments as the Read method and returns the number of characters read:
charsRead = SR.Read(chars, startIndex, count)
ReadLine
This method reads the next line from the text file associated with the StreamReader object and returns a string. If you’re at the end of the file, the method returns the Null value. The syntax of the ReadLine method is
Dim txtLine As String txtLine = SR.ReadLine()
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
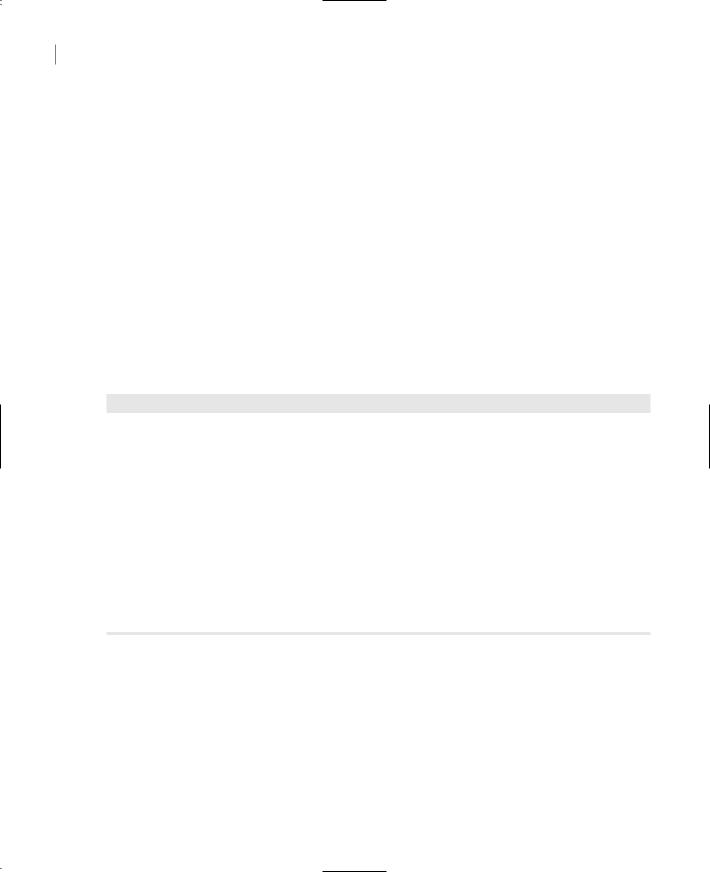
602 Chapter 13 WORKING WITH FOLDERS AND FILES
A text line is a sequence of characters followed by carriage return (\r), or line feed (\n), or carriage return and line feed (\r\n). Notice that the NewLine character you may have specified for the specific file with the StreamWriter object is ignored by the ReadLine method. The string returned by the method doesn’t include the line terminator.
ReadToEnd
The last method for reading characters from a text file reads all the characters from the current position to the end of the file. We usually call this method once to read the entire file with a single statement and store its contents to a string variable. The syntax of the ReadToEnd method is
allText = SR.ReadToEnd()
Sending Data to a File
The statements in Listing 13.14 demonstrate how to send various data types to a file. You can place the statements of this listing to button’s Click event handler and then open the file with Notepad to see its contents. Everything is in text format, including the numeric values. Don’t forget to import the System.IO namespace to your project.
Listing 13.14: Writing Data to a Text File
Dim SW As StreamWriter
Dim FS As FileStream
FS = New FileStream(“C:\TextData.txt”, FileMode.Create)
SW = New StreamWriter(FS)
SW.WriteLine(9.009)
SW.WriteLine(1 / 3)
SW.Write(“The current date is “)
SW.Write(Now())
SW.WriteLine()
SW.WriteLine(True)
SW.WriteLine(New Rectangle(1, 1, 100, 200))
SW.WriteLine(Color.YellowGreen)
SW.Close()
FS.Close()
Here’s the output produced by Listing 13.14:
9.009
0.333333333333333
The current date is 2001-03-16T12:14:02 True
{X=1,Y=1,Width=100,Height=200} Color [YellowGreen]
Notice how the WriteLine method without an argument inserts a new line character in the file. The statement SW.Write(now()) prints the current date but doesn’t switch to another line. The
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

ACCESSING FILES 603
WriteLine method without any arguments starts a new line. The following statements demonstrate a more complicated use of the Write method with formatting arguments:
Dim BDate As Date = #2/8/1960 1:04:00 PM#
SW.WriteLine(“Your age in years is {0}, in months is {1}, “ & _ “in days is {2}, and in hours is {3}.”, _ DateDiff(DateInterval.year, BDate, Now), _ DateDiff(DateInterval.month, BDate, Now), _ DateDiff(DateInterval.day, BDate, Now), _ DateDiff(DateInterval.hour, BDate, Now))
The SW StreamWriter must be declared with the statements at the beginning of Listing 13.14. The day I tested these statements, the following string was written to the file:
Your age in years is 41, in months is 493, in days is 15012.027722980833, and in hours is 360288.66535154.
Of course, the data to be stored to a text file need not be hard-coded in your application. The code of Listing 13.15 stores the contents of a TextBox control to a text file.
Listing 13.15: Storing the Contents of a TextBox Control to a Text File
Dim SW As StreamWriter
Dim FS As FileStream
FS = New FileStream(“C:\TextData.txt”, FileMode.Create)
SW = New StreamWriter(FS)
SW.Write(TextBox1.Text)
To save the contents of a ListBox control to a text file, iterate through its Items collection and store each item to the file. The items of the control will be stored in the file as strings. Neither the StreamWriter nor the BinaryWriter provides a method for storing objects to or reading objects from a file. If you want to store objects, see the discussion of serializing collections in Chapter 11.
The following statements populate a ListBox control with various items:
ListBox1.Items.Add(“First Item”)
ListBox1.Items.Add(New Rectangle(0, 0, 3, 3))
ListBox1.Items.Add(New Point(3.2, 4.01))
ListBox1.Items.Add(“Last Item”)
The code that saves each item to a separate line in the C:\Items.txt file is shown in Listing 13.16.
Listing 13.16: Saving an Items Collection to a Text File
Dim SW As StreamWriter
Dim FS As FileStream
FS = New FileStream(“C:\Items.txt”, FileMode.Create)
SW = New StreamWriter(FS)
Dim itm As Object
For Each itm In ListBox1.Items
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |