
- •Using Your Sybex Electronic Book
- •Acknowledgments
- •Contents at a Glance
- •Introduction
- •Who Should Read This Book?
- •How About the Advanced Topics?
- •The Structure of the Book
- •How to Reach the Author
- •The Integrated Development Environment
- •The Start Page
- •Project Types
- •Your First VB Application
- •Making the Application More Robust
- •Making the Application More User-Friendly
- •The IDE Components
- •The IDE Menu
- •The Toolbox Window
- •The Solution Explorer
- •The Properties Window
- •The Output Window
- •The Command Window
- •The Task List Window
- •Environment Options
- •A Few Common Properties
- •A Few Common Events
- •A Few Common Methods
- •Building a Console Application
- •Summary
- •Building a Loan Calculator
- •How the Loan Application Works
- •Designing the User Interface
- •Programming the Loan Application
- •Validating the Data
- •Building a Math Calculator
- •Designing the User Interface
- •Programming the MathCalculator App
- •Adding More Features
- •Exception Handling
- •Taking the LoanCalculator to the Web
- •Working with Multiple Forms
- •Working with Multiple Projects
- •Executable Files
- •Distributing an Application
- •VB.NET at Work: Creating a Windows Installer
- •Finishing the Windows Installer
- •Running the Windows Installer
- •Verifying the Installation
- •Summary
- •Variables
- •Declaring Variables
- •Types of Variables
- •Converting Variable Types
- •User-Defined Data Types
- •Examining Variable Types
- •Why Declare Variables?
- •A Variable’s Scope
- •The Lifetime of a Variable
- •Constants
- •Arrays
- •Declaring Arrays
- •Initializing Arrays
- •Array Limits
- •Multidimensional Arrays
- •Dynamic Arrays
- •Arrays of Arrays
- •Variables as Objects
- •So, What’s an Object?
- •Formatting Numbers
- •Formatting Dates
- •Flow-Control Statements
- •Test Structures
- •Loop Structures
- •Nested Control Structures
- •The Exit Statement
- •Summary
- •Modular Coding
- •Subroutines
- •Functions
- •Arguments
- •Argument-Passing Mechanisms
- •Event-Handler Arguments
- •Passing an Unknown Number of Arguments
- •Named Arguments
- •More Types of Function Return Values
- •Overloading Functions
- •Summary
- •The Appearance of Forms
- •Properties of the Form Control
- •Placing Controls on Forms
- •Setting the TabOrder
- •VB.NET at Work: The Contacts Project
- •Anchoring and Docking
- •Loading and Showing Forms
- •The Startup Form
- •Controlling One Form from within Another
- •Forms vs. Dialog Boxes
- •VB.NET at Work: The MultipleForms Project
- •Designing Menus
- •The Menu Editor
- •Manipulating Menus at Runtime
- •Building Dynamic Forms at Runtime
- •The Form.Controls Collection
- •VB.NET at Work: The DynamicForm Project
- •Creating Event Handlers at Runtime
- •Summary
- •The TextBox Control
- •Basic Properties
- •Text-Manipulation Properties
- •Text-Selection Properties
- •Text-Selection Methods
- •Undoing Edits
- •VB.NET at Work: The TextPad Project
- •Capturing Keystrokes
- •The ListBox, CheckedListBox, and ComboBox Controls
- •Basic Properties
- •The Items Collection
- •VB.NET at Work: The ListDemo Project
- •Searching
- •The ComboBox Control
- •The ScrollBar and TrackBar Controls
- •The ScrollBar Control
- •The TrackBar Control
- •Summary
- •The Common Dialog Controls
- •Using the Common Dialog Controls
- •The Color Dialog Box
- •The Font Dialog Box
- •The Open and Save As Dialog Boxes
- •The Print Dialog Box
- •The RichTextBox Control
- •The RTF Language
- •Methods
- •Advanced Editing Features
- •Cutting and Pasting
- •Searching in a RichTextBox Control
- •Formatting URLs
- •VB.NET at Work: The RTFPad Project
- •Summary
- •What Is a Class?
- •Building the Minimal Class
- •Adding Code to the Minimal Class
- •Property Procedures
- •Customizing Default Members
- •Custom Enumerations
- •Using the SimpleClass in Other Projects
- •Firing Events
- •Shared Properties
- •Parsing a Filename String
- •Reusing the StringTools Class
- •Encapsulation and Abstraction
- •Inheritance
- •Inheriting Existing Classes
- •Polymorphism
- •The Shape Class
- •Object Constructors and Destructors
- •Instance and Shared Methods
- •Who Can Inherit What?
- •Parent Class Keywords
- •Derived Class Keyword
- •Parent Class Member Keywords
- •Derived Class Member Keyword
- •MyBase and MyClass
- •Summary
- •On Designing Windows Controls
- •Enhancing Existing Controls
- •Building the FocusedTextBox Control
- •Building Compound Controls
- •VB.NET at Work: The ColorEdit Control
- •VB.NET at Work: The Label3D Control
- •Raising Events
- •Using the Custom Control in Other Projects
- •VB.NET at Work: The Alarm Control
- •Designing Irregularly Shaped Controls
- •Designing Owner-Drawn Menus
- •Designing Owner-Drawn ListBox Controls
- •Using ActiveX Controls
- •Summary
- •Programming Word
- •Objects That Represent Text
- •The Documents Collection and the Document Object
- •Spell-Checking Documents
- •Programming Excel
- •The Worksheets Collection and the Worksheet Object
- •The Range Object
- •Using Excel as a Math Parser
- •Programming Outlook
- •Retrieving Information
- •Recursive Scanning of the Contacts Folder
- •Summary
- •Advanced Array Topics
- •Sorting Arrays
- •Searching Arrays
- •Other Array Operations
- •Array Limitations
- •The ArrayList Collection
- •Creating an ArrayList
- •Adding and Removing Items
- •The HashTable Collection
- •VB.NET at Work: The WordFrequencies Project
- •The SortedList Class
- •The IEnumerator and IComparer Interfaces
- •Enumerating Collections
- •Custom Sorting
- •Custom Sorting of a SortedList
- •The Serialization Class
- •Serializing Individual Objects
- •Serializing a Collection
- •Deserializing Objects
- •Summary
- •Handling Strings and Characters
- •The Char Class
- •The String Class
- •The StringBuilder Class
- •VB.NET at Work: The StringReversal Project
- •VB.NET at Work: The CountWords Project
- •Handling Dates
- •The DateTime Class
- •The TimeSpan Class
- •VB.NET at Work: Timing Operations
- •Summary
- •Accessing Folders and Files
- •The Directory Class
- •The File Class
- •The DirectoryInfo Class
- •The FileInfo Class
- •The Path Class
- •VB.NET at Work: The CustomExplorer Project
- •Accessing Files
- •The FileStream Object
- •The StreamWriter Object
- •The StreamReader Object
- •Sending Data to a File
- •The BinaryWriter Object
- •The BinaryReader Object
- •VB.NET at Work: The RecordSave Project
- •The FileSystemWatcher Component
- •Properties
- •Events
- •VB.NET at Work: The FileSystemWatcher Project
- •Summary
- •Displaying Images
- •The Image Object
- •Exchanging Images through the Clipboard
- •Drawing with GDI+
- •The Basic Drawing Objects
- •Drawing Shapes
- •Drawing Methods
- •Gradients
- •Coordinate Transformations
- •Specifying Transformations
- •VB.NET at Work: Plotting Functions
- •Bitmaps
- •Specifying Colors
- •Defining Colors
- •Processing Bitmaps
- •Summary
- •The Printing Objects
- •PrintDocument
- •PrintDialog
- •PageSetupDialog
- •PrintPreviewDialog
- •PrintPreviewControl
- •Printer and Page Properties
- •Page Geometry
- •Printing Examples
- •Printing Tabular Data
- •Printing Plain Text
- •Printing Bitmaps
- •Using the PrintPreviewControl
- •Summary
- •Examining the Advanced Controls
- •How Tree Structures Work
- •The ImageList Control
- •The TreeView Control
- •Adding New Items at Design Time
- •Adding New Items at Runtime
- •Assigning Images to Nodes
- •Scanning the TreeView Control
- •The ListView Control
- •The Columns Collection
- •The ListItem Object
- •The Items Collection
- •The SubItems Collection
- •Summary
- •Types of Errors
- •Design-Time Errors
- •Runtime Errors
- •Logic Errors
- •Exceptions and Structured Exception Handling
- •Studying an Exception
- •Getting a Handle on this Exception
- •Finally (!)
- •Customizing Exception Handling
- •Throwing Your Own Exceptions
- •Debugging
- •Breakpoints
- •Stepping Through
- •The Local and Watch Windows
- •Summary
- •Basic Concepts
- •Recursion in Real Life
- •A Simple Example
- •Recursion by Mistake
- •Scanning Folders Recursively
- •Describing a Recursive Procedure
- •Translating the Description to Code
- •The Stack Mechanism
- •Stack Defined
- •Recursive Programming and the Stack
- •Passing Arguments through the Stack
- •Special Issues in Recursive Programming
- •Knowing When to Use Recursive Programming
- •Summary
- •MDI Applications: The Basics
- •Building an MDI Application
- •Built-In Capabilities of MDI Applications
- •Accessing Child Forms
- •Ending an MDI Application
- •A Scrollable PictureBox
- •Summary
- •What Is a Database?
- •Relational Databases
- •Exploring the Northwind Database
- •Exploring the Pubs Database
- •Understanding Relations
- •The Server Explorer
- •Working with Tables
- •Relationships, Indices, and Constraints
- •Structured Query Language
- •Executing SQL Statements
- •Selection Queries
- •Calculated Fields
- •SQL Joins
- •Action Queries
- •The Query Builder
- •The Query Builder Interface
- •SQL at Work: Calculating Sums
- •SQL at Work: Counting Rows
- •Limiting the Selection
- •Parameterized Queries
- •Calculated Columns
- •Specifying Left, Right, and Inner Joins
- •Stored Procedures
- •Summary
- •How About XML?
- •Creating a DataSet
- •The DataGrid Control
- •Data Binding
- •VB.NET at Work: The ViewEditCustomers Project
- •Binding Complex Controls
- •Programming the DataAdapter Object
- •The Command Objects
- •The Command and DataReader Objects
- •VB.NET at Work: The DataReader Project
- •VB.NET at Work: The StoredProcedure Project
- •Summary
- •The Structure of a DataSet
- •Navigating the Tables of a DataSet
- •Updating DataSets
- •The DataForm Wizard
- •Handling Identity Fields
- •Transactions
- •Performing Update Operations
- •Updating Tables Manually
- •Building and Using Custom DataSets
- •Summary
- •An HTML Primer
- •HTML Code Elements
- •Server-Client Interaction
- •The Structure of HTML Documents
- •URLs and Hyperlinks
- •The Basic HTML Tags
- •Inserting Graphics
- •Tables
- •Forms and Controls
- •Processing Requests on the Server
- •Building a Web Application
- •Interacting with a Web Application
- •Maintaining State
- •The Web Controls
- •The ASP.NET Objects
- •The Page Object
- •The Response Object
- •The Request Object
- •The Server Object
- •Using Cookies
- •Handling Multiple Forms in Web Applications
- •Summary
- •The Data-Bound Web Controls
- •Simple Data Binding
- •Binding to DataSets
- •Is It a Grid, or a Table?
- •Getting Orders on the Web
- •The Forms of the ProductSearch Application
- •Paging Large DataSets
- •Customizing the Appearance of the DataGrid Control
- •Programming the Select Button
- •Summary
- •How to Serve the Web
- •Building a Web Service
- •Consuming the Web Service
- •Maintaining State in Web Services
- •A Data-Driven Web Service
- •Consuming the Products Web Service in VB
- •Summary

270 Chapter 6 BASIC WINDOWS CONTROLS
To iterate through all the selected items in a multiselection ListBox control, use a loop like the following:
Dim itm As Object
For Each itm In ListBox1.SelectedItems
Console.WriteLine(itm)
Next
The itm variable was declared as Object because the items in the ListBox control are objects. They happen to be strings in most cases, but they can be anything. If they’re all of the same type, you can convert them to the specific type and then call their methods. If all the items are of the Color type, you can use a loop like the following to print the red component of each color:
Dim itm As Object
For Each itm In ListBox1.SelectedItems
Console.WriteLine(Ctype(itm, Color).Red)
Next
A common situation in programming the ListBox control is to remove items from one control and add them to another. This is what the ListDemo project of the following section demonstrates, along with the techniques for adding and removing items to single-selection and a multiselection ListBox controls.
Note Even though the ListBox control can store all types of objects, it’s used most frequently for storing strings. Storing objects to a ListBox control requires some extra work, because the ToString method of most objects doesn’t return the type of string we want to display on the control. You will find an example on using the ListBox control with objects in Chapter 8, where you’ll learn how to build custom objects.
VB.NET at Work: The ListDemo Project
The ListDemo application (shown in Figure 6.7) demonstrates the basic operations of the ListBox control. The two ListBox controls on the form operate slightly differently. The first has the default configuration: only one item can be selected at a time, and new items are appended after the existing item. The second ListBox control has its Sorted property set to True and its MultiSelect property set to MultiExtended. This means that the elements of this control are always sorted, and the user can select multiple cells with the mouse.
The code for the ListDemo application contains much of the logic you’ll need in your ListBox manipulation routines. It shows you how to:
Add and remove items
Transfer items between lists
Handle multiple selected items
Maintain sorted lists
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

THE LISTBOX, CHECKEDLISTBOX, AND COMBOBOX CONTROLS 271
Figure 6.7
ListDemo demonstrates most of the operations you’ll perform with ListBoxes.
The Add Item Buttons
The Add Item buttons use the InputBox() function to prompt the user for input, and then they add the user-supplied string to the ListBox control. The code is identical for both buttons (Listing 6.10).
Listing 6.10: The Add Item Buttons
Private Sub bttnAdd1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles bttnAdd1.Click Dim ListItem As String
ListItem = InputBox(“Enter new item’s name”) If ListItem.Trim <> “” Then
sourceList.Items.Add(ListItem) End If
End Sub
Notice that the subroutine examines the data entered by the user to avoid adding blank strings to the list. The code for the Clear buttons is also straightforward; it simply calls the Clear method of the Items collection to remove all entries from the corresponding list.
The Remove Selected Item(s) Buttons
The code for the Remove Selected Item button is different from that for the Remove Selected Items button (both are presented in Listing 6.11). The code for the Remove Selected Items button must scan all the items of the left list and remove the selected one(s). The reason is that the ListBox on the right can have only one selected item, and the other one allows the selection of multiple items. To delete an item, you must have at least one item selected. The code makes sure that the SelectedIndex property is not negative. If no item is selected, the SelectedIndex property is –1 and attempting to remove an item by specifying an invalid index will generate an error.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

272 Chapter 6 BASIC WINDOWS CONTROLS
Listing 6.11: The Remove Buttons
Private Sub bttnRemoveSelDest_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles bttnRemoveSelDest.Click destinationList.Items.Remove(destinationList.SelectedItem)
End Sub
Private Sub bttnRemoveSelSrc_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles bttnRemoveSelSrc.Click Dim i As Integer
For i = 0 To sourceList.SelectedIndices.Count - 1 sourceList.Items.RemoveAt(sourceList.SelectedIndices(0))
Next End Sub
Even though it’s possible to remove an item by name, this is not a safe approach. If two items have the same name, then the Remove method will remove the first one. Unless you’ve provided the code to make sure that no identical items can be added to the list, remove them by their index, which is unique.
Notice that the code removes always the first item in the SelectedIndices collection. If you attempt to remove the item SelectedIndices(i), you will remove the first selected item, but after that you will not remove all the selected items. After removing an item from the selection, the remaining items are no longer at the same locations. The second selected item will take the place of the first selected item, which was just deleted, and so on. By removing the first item in the SelectedIndices collection, we make sure that all selected items, and only they, will be eventually removed.
The code of the Remove Selected Items button uses the Count property of the SelectedIndices collection to repeat the operation as many times as the number of selected items.
The Arrow Buttons
The two Buttons with the single arrows, between the ListBox controls shown in Figure 6.7, transfer selected items from one list to another. The first arrow button can transfer a single element only, after it ensures that the list contains a selected item. Its code is presented in Listing 6.12. First, it adds the item to the second list, and then it removes the item from the original list. Notice that the code removes an item by passing it as argument to the Remove method, because it doesn’t make any difference which one of two identical objects will be removed.
Listing 6.12: The Right Arrow Button
Private Sub bttnMoveDest_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles bttnMoveDest.Click Dim i As Integer
While sourceList.SelectedIndices.Count > 0 destinationList.Items.Add(sourceList.SelectedItems(i)) sourceList.Items.Remove(sourceList.SelectedItems(i))
End While End Sub
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
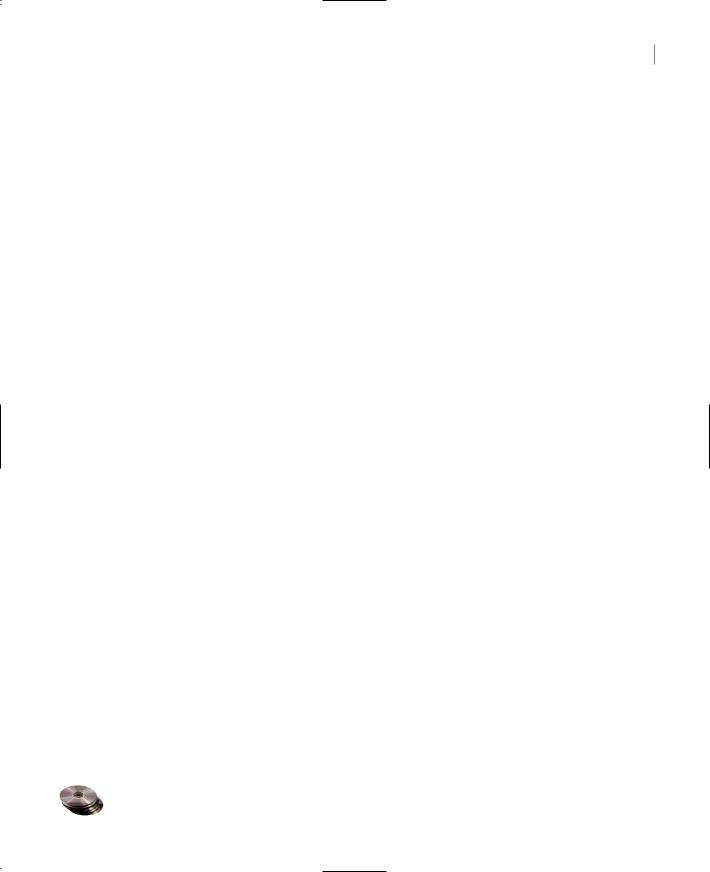
THE LISTBOX, CHECKEDLISTBOX, AND COMBOBOX CONTROLS 273
The second arrow button transfers items in the opposite direction; the code is almost identical to the one presented here, and I need not repeat it. The fact that one list is sorted and the other isn’t doesn’t affect our code. The destination control (the one on the left) doesn’t allow the selection of multiple items, so you could use the SelectedIndex and SelectedItem properties. Since the single element is also part of the SelectedItems collection, you need not use a different approach. The statements that move a single item from the right to the left ListBox are shown next:
sourceList.Items.Add(destinationList.SelectedItem)
destinationList.Items.RemoveAt(destinationList.SelectedIndex)
Before we leave the topic of the ListBox control, let’s examine one more powerful technique: using the ListBox control to maintain a list of keys (the data items used in recalling the information) to an array or random access file with records of related information. We’ll use the ListBox control to store information like names, or book titles, which allows users to select the desired item. The item in the control will be linked to related information, like addresses and phone numbers for people, author and price information for books, and so on. In other words, we’ll use the ListBox control as a lookup mechanism for several pieces of information.
Searching
The single most important enhancement to the ListBox control is that it can now locate any item in the list with the FindString and FindStringExact methods. Both methods accept a string as argument (the item to be located) and a second, optional argument, the index at which the search will begin.
The FindString method locates a string that partially matches the one you’re searching for; FindStringExact finds an exact match. If you’re searching for “Man” and the control contains a name like “Mansfield,” FindString will match the item, but FindStringExact will not.
Note Both the FindString and FindStringExact methods perform case-insensitive searches. If you’re searching for “visual” and the list contains the item “Visual”, both methods will locate it.
The syntax of both methods is the same:
itemIndex = ListBox1.FindString(searchStr As String)
where searchStr is the string you’re searching for. An alternative form of both methods allows you to specify the order of the item at which the search will begin:
itemIndex = ListBox1.FindString(searchStr As String, startIndex As Integer)
The startIndex argument allows you specify the beginning of the search, but you can’t specify where the search will end.
The FindString and FindStringExact methods work even if the ListBox control is not sorted. You need not set the Sorted property to True before you call one of the searching methods on the control. Sorting the list will probably help the search operation a little, but it takes the control less than 100 milliseconds to find an item in a list of 100,000 items, so time spent to sort the list isn’t worth it.
VB.NET at Work: The ListBoxFind Application
The application you’ll build in this section (seen in Figure 6.8) populates a list with a large number of items and then locates any string you specify. Click the button Populate List to populate the
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

274 Chapter 6 BASIC WINDOWS CONTROLS
ListBox control with 10,000 random strings. This process will take a few seconds and will populate the control with different random strings every time. Then, each time you click the Find Item button, you’ll be prompted to enter a string. The code will locate the closest match in the list and select (highlight) this item.
Figure 6.8
The ListBoxFind application
The code (Listing 6.13) attempts to locate an exact match with the FindStringExact method. If it succeeds, it reports the index of the matching element. If not, it attempts to locate a near match, with the FindString method. If it succeeds, it reports the index of the near match (which is the first item on the control that partially matches the search argument) and terminates. If it fails to find an exact match, it reports that the string wasn’t found in the list.
Listing 6.13: Searching the List
Private Sub bttnFind_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles bttnFind.Click
Dim srchWord As String |
|
Dim wordIndex As Integer |
|
srchWord = InputBox(“Enter word to search for”) |
|
wordIndex = ListBox1.FindStringExact(srchWord) |
|
If wordIndex >= 0 Then |
|
MsgBox(“Index = “ & wordIndex.ToString & “ |
=” & _ |
(ListBox1.Items(wordIndex)).ToString, , “EXACT MATCH”) |
|
ListBox1.TopIndex = wordIndex |
|
ListBox1.SelectedIndex = wordIndex |
|
Else |
|
wordIndex = ListBox1.FindString(srchWord) |
|
If wordIndex >= 0 Then |
|
MsgBox(“Index = “ & wordIndex.ToString & “ |
=” & _ |
(ListBox1.Items(wordIndex)).ToString, , “NEAR MATCH”) ListBox1.TopIndex = wordIndex
ListBox1.SelectedIndex = wordIndex Else
MsgBox(“Item “ & srchWord & “ is not in the list”) End If
End If End Sub
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |