
- •Using Your Sybex Electronic Book
- •Acknowledgments
- •Contents at a Glance
- •Introduction
- •Who Should Read This Book?
- •How About the Advanced Topics?
- •The Structure of the Book
- •How to Reach the Author
- •The Integrated Development Environment
- •The Start Page
- •Project Types
- •Your First VB Application
- •Making the Application More Robust
- •Making the Application More User-Friendly
- •The IDE Components
- •The IDE Menu
- •The Toolbox Window
- •The Solution Explorer
- •The Properties Window
- •The Output Window
- •The Command Window
- •The Task List Window
- •Environment Options
- •A Few Common Properties
- •A Few Common Events
- •A Few Common Methods
- •Building a Console Application
- •Summary
- •Building a Loan Calculator
- •How the Loan Application Works
- •Designing the User Interface
- •Programming the Loan Application
- •Validating the Data
- •Building a Math Calculator
- •Designing the User Interface
- •Programming the MathCalculator App
- •Adding More Features
- •Exception Handling
- •Taking the LoanCalculator to the Web
- •Working with Multiple Forms
- •Working with Multiple Projects
- •Executable Files
- •Distributing an Application
- •VB.NET at Work: Creating a Windows Installer
- •Finishing the Windows Installer
- •Running the Windows Installer
- •Verifying the Installation
- •Summary
- •Variables
- •Declaring Variables
- •Types of Variables
- •Converting Variable Types
- •User-Defined Data Types
- •Examining Variable Types
- •Why Declare Variables?
- •A Variable’s Scope
- •The Lifetime of a Variable
- •Constants
- •Arrays
- •Declaring Arrays
- •Initializing Arrays
- •Array Limits
- •Multidimensional Arrays
- •Dynamic Arrays
- •Arrays of Arrays
- •Variables as Objects
- •So, What’s an Object?
- •Formatting Numbers
- •Formatting Dates
- •Flow-Control Statements
- •Test Structures
- •Loop Structures
- •Nested Control Structures
- •The Exit Statement
- •Summary
- •Modular Coding
- •Subroutines
- •Functions
- •Arguments
- •Argument-Passing Mechanisms
- •Event-Handler Arguments
- •Passing an Unknown Number of Arguments
- •Named Arguments
- •More Types of Function Return Values
- •Overloading Functions
- •Summary
- •The Appearance of Forms
- •Properties of the Form Control
- •Placing Controls on Forms
- •Setting the TabOrder
- •VB.NET at Work: The Contacts Project
- •Anchoring and Docking
- •Loading and Showing Forms
- •The Startup Form
- •Controlling One Form from within Another
- •Forms vs. Dialog Boxes
- •VB.NET at Work: The MultipleForms Project
- •Designing Menus
- •The Menu Editor
- •Manipulating Menus at Runtime
- •Building Dynamic Forms at Runtime
- •The Form.Controls Collection
- •VB.NET at Work: The DynamicForm Project
- •Creating Event Handlers at Runtime
- •Summary
- •The TextBox Control
- •Basic Properties
- •Text-Manipulation Properties
- •Text-Selection Properties
- •Text-Selection Methods
- •Undoing Edits
- •VB.NET at Work: The TextPad Project
- •Capturing Keystrokes
- •The ListBox, CheckedListBox, and ComboBox Controls
- •Basic Properties
- •The Items Collection
- •VB.NET at Work: The ListDemo Project
- •Searching
- •The ComboBox Control
- •The ScrollBar and TrackBar Controls
- •The ScrollBar Control
- •The TrackBar Control
- •Summary
- •The Common Dialog Controls
- •Using the Common Dialog Controls
- •The Color Dialog Box
- •The Font Dialog Box
- •The Open and Save As Dialog Boxes
- •The Print Dialog Box
- •The RichTextBox Control
- •The RTF Language
- •Methods
- •Advanced Editing Features
- •Cutting and Pasting
- •Searching in a RichTextBox Control
- •Formatting URLs
- •VB.NET at Work: The RTFPad Project
- •Summary
- •What Is a Class?
- •Building the Minimal Class
- •Adding Code to the Minimal Class
- •Property Procedures
- •Customizing Default Members
- •Custom Enumerations
- •Using the SimpleClass in Other Projects
- •Firing Events
- •Shared Properties
- •Parsing a Filename String
- •Reusing the StringTools Class
- •Encapsulation and Abstraction
- •Inheritance
- •Inheriting Existing Classes
- •Polymorphism
- •The Shape Class
- •Object Constructors and Destructors
- •Instance and Shared Methods
- •Who Can Inherit What?
- •Parent Class Keywords
- •Derived Class Keyword
- •Parent Class Member Keywords
- •Derived Class Member Keyword
- •MyBase and MyClass
- •Summary
- •On Designing Windows Controls
- •Enhancing Existing Controls
- •Building the FocusedTextBox Control
- •Building Compound Controls
- •VB.NET at Work: The ColorEdit Control
- •VB.NET at Work: The Label3D Control
- •Raising Events
- •Using the Custom Control in Other Projects
- •VB.NET at Work: The Alarm Control
- •Designing Irregularly Shaped Controls
- •Designing Owner-Drawn Menus
- •Designing Owner-Drawn ListBox Controls
- •Using ActiveX Controls
- •Summary
- •Programming Word
- •Objects That Represent Text
- •The Documents Collection and the Document Object
- •Spell-Checking Documents
- •Programming Excel
- •The Worksheets Collection and the Worksheet Object
- •The Range Object
- •Using Excel as a Math Parser
- •Programming Outlook
- •Retrieving Information
- •Recursive Scanning of the Contacts Folder
- •Summary
- •Advanced Array Topics
- •Sorting Arrays
- •Searching Arrays
- •Other Array Operations
- •Array Limitations
- •The ArrayList Collection
- •Creating an ArrayList
- •Adding and Removing Items
- •The HashTable Collection
- •VB.NET at Work: The WordFrequencies Project
- •The SortedList Class
- •The IEnumerator and IComparer Interfaces
- •Enumerating Collections
- •Custom Sorting
- •Custom Sorting of a SortedList
- •The Serialization Class
- •Serializing Individual Objects
- •Serializing a Collection
- •Deserializing Objects
- •Summary
- •Handling Strings and Characters
- •The Char Class
- •The String Class
- •The StringBuilder Class
- •VB.NET at Work: The StringReversal Project
- •VB.NET at Work: The CountWords Project
- •Handling Dates
- •The DateTime Class
- •The TimeSpan Class
- •VB.NET at Work: Timing Operations
- •Summary
- •Accessing Folders and Files
- •The Directory Class
- •The File Class
- •The DirectoryInfo Class
- •The FileInfo Class
- •The Path Class
- •VB.NET at Work: The CustomExplorer Project
- •Accessing Files
- •The FileStream Object
- •The StreamWriter Object
- •The StreamReader Object
- •Sending Data to a File
- •The BinaryWriter Object
- •The BinaryReader Object
- •VB.NET at Work: The RecordSave Project
- •The FileSystemWatcher Component
- •Properties
- •Events
- •VB.NET at Work: The FileSystemWatcher Project
- •Summary
- •Displaying Images
- •The Image Object
- •Exchanging Images through the Clipboard
- •Drawing with GDI+
- •The Basic Drawing Objects
- •Drawing Shapes
- •Drawing Methods
- •Gradients
- •Coordinate Transformations
- •Specifying Transformations
- •VB.NET at Work: Plotting Functions
- •Bitmaps
- •Specifying Colors
- •Defining Colors
- •Processing Bitmaps
- •Summary
- •The Printing Objects
- •PrintDocument
- •PrintDialog
- •PageSetupDialog
- •PrintPreviewDialog
- •PrintPreviewControl
- •Printer and Page Properties
- •Page Geometry
- •Printing Examples
- •Printing Tabular Data
- •Printing Plain Text
- •Printing Bitmaps
- •Using the PrintPreviewControl
- •Summary
- •Examining the Advanced Controls
- •How Tree Structures Work
- •The ImageList Control
- •The TreeView Control
- •Adding New Items at Design Time
- •Adding New Items at Runtime
- •Assigning Images to Nodes
- •Scanning the TreeView Control
- •The ListView Control
- •The Columns Collection
- •The ListItem Object
- •The Items Collection
- •The SubItems Collection
- •Summary
- •Types of Errors
- •Design-Time Errors
- •Runtime Errors
- •Logic Errors
- •Exceptions and Structured Exception Handling
- •Studying an Exception
- •Getting a Handle on this Exception
- •Finally (!)
- •Customizing Exception Handling
- •Throwing Your Own Exceptions
- •Debugging
- •Breakpoints
- •Stepping Through
- •The Local and Watch Windows
- •Summary
- •Basic Concepts
- •Recursion in Real Life
- •A Simple Example
- •Recursion by Mistake
- •Scanning Folders Recursively
- •Describing a Recursive Procedure
- •Translating the Description to Code
- •The Stack Mechanism
- •Stack Defined
- •Recursive Programming and the Stack
- •Passing Arguments through the Stack
- •Special Issues in Recursive Programming
- •Knowing When to Use Recursive Programming
- •Summary
- •MDI Applications: The Basics
- •Building an MDI Application
- •Built-In Capabilities of MDI Applications
- •Accessing Child Forms
- •Ending an MDI Application
- •A Scrollable PictureBox
- •Summary
- •What Is a Database?
- •Relational Databases
- •Exploring the Northwind Database
- •Exploring the Pubs Database
- •Understanding Relations
- •The Server Explorer
- •Working with Tables
- •Relationships, Indices, and Constraints
- •Structured Query Language
- •Executing SQL Statements
- •Selection Queries
- •Calculated Fields
- •SQL Joins
- •Action Queries
- •The Query Builder
- •The Query Builder Interface
- •SQL at Work: Calculating Sums
- •SQL at Work: Counting Rows
- •Limiting the Selection
- •Parameterized Queries
- •Calculated Columns
- •Specifying Left, Right, and Inner Joins
- •Stored Procedures
- •Summary
- •How About XML?
- •Creating a DataSet
- •The DataGrid Control
- •Data Binding
- •VB.NET at Work: The ViewEditCustomers Project
- •Binding Complex Controls
- •Programming the DataAdapter Object
- •The Command Objects
- •The Command and DataReader Objects
- •VB.NET at Work: The DataReader Project
- •VB.NET at Work: The StoredProcedure Project
- •Summary
- •The Structure of a DataSet
- •Navigating the Tables of a DataSet
- •Updating DataSets
- •The DataForm Wizard
- •Handling Identity Fields
- •Transactions
- •Performing Update Operations
- •Updating Tables Manually
- •Building and Using Custom DataSets
- •Summary
- •An HTML Primer
- •HTML Code Elements
- •Server-Client Interaction
- •The Structure of HTML Documents
- •URLs and Hyperlinks
- •The Basic HTML Tags
- •Inserting Graphics
- •Tables
- •Forms and Controls
- •Processing Requests on the Server
- •Building a Web Application
- •Interacting with a Web Application
- •Maintaining State
- •The Web Controls
- •The ASP.NET Objects
- •The Page Object
- •The Response Object
- •The Request Object
- •The Server Object
- •Using Cookies
- •Handling Multiple Forms in Web Applications
- •Summary
- •The Data-Bound Web Controls
- •Simple Data Binding
- •Binding to DataSets
- •Is It a Grid, or a Table?
- •Getting Orders on the Web
- •The Forms of the ProductSearch Application
- •Paging Large DataSets
- •Customizing the Appearance of the DataGrid Control
- •Programming the Select Button
- •Summary
- •How to Serve the Web
- •Building a Web Service
- •Consuming the Web Service
- •Maintaining State in Web Services
- •A Data-Driven Web Service
- •Consuming the Products Web Service in VB
- •Summary

642 Chapter 14 DRAWING AND PAINTING WITH VISUAL BASIC
Table 14.4: The HatchStyle Enumeration
Value |
Effect |
BackwardDiagonal |
Diagonal lines from top-right to bottom-left |
Cross |
Vertical and horizontal crossing lines |
DiagonalCross |
Diagonally crossing lines |
ForwardDiagonal |
Diagonal lines from top-left to bottom-right |
Horizontal |
Horizontal lines |
Vertical |
Vertical lines |
|
|
Gradient Brushes
A gradient brush fills a shape with a specified gradient. The LinearGradientBrush fills a shape with a linear gradient, and the PathGradientBrush fills a shape with a gradient that has one starting color and many ending colors. Gradient brushes are discussed in detail in the section “Gradients” later in this chapter.
Textured Brushes
In addition to solid and hatched shapes, you can fill a shape with a texture using a TextureBrush object. The texture is a bitmap that is tiled as needed to fill the shape. Textured brushes are used in creating rather fancy graphics, and we’ll not explore them in this chapter.
Drawing Shapes
In this section, you will learn the drawing methods of the Graphics object. Before getting into the details of the drawing methods, however, let’s write a simple application that draws a couple of simple shapes on a form. First, we must create a Graphics object with the following statement:
Dim G As Graphics
G = Me.CreateGraphics
Everything you draw on the surface represented by the G object will appear on the form. Then, we must create a Pen object to draw with. The following statement creates a Pen object that draws in blue:
Dim P As New Pen(Color.Blue)
You’ve created the two basic objects for drawing: the drawing surface and the drawing instrument. Now you can draw shapes by calling the Graphics object’s methods. The following statement will print a rectangle with its top-left corner near the top-left corner of the form (at a point that’s 10 pixels to the right and 10 pixels down from the form’s corner) and is 200 pixels wide and 150 pixels tall. These are the values you must pass to the DrawRectangle method as arguments, along with the Pen object that will be used to render the rectangle:
G.DrawRectangle(P, 10, 10, 200, 150)
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |
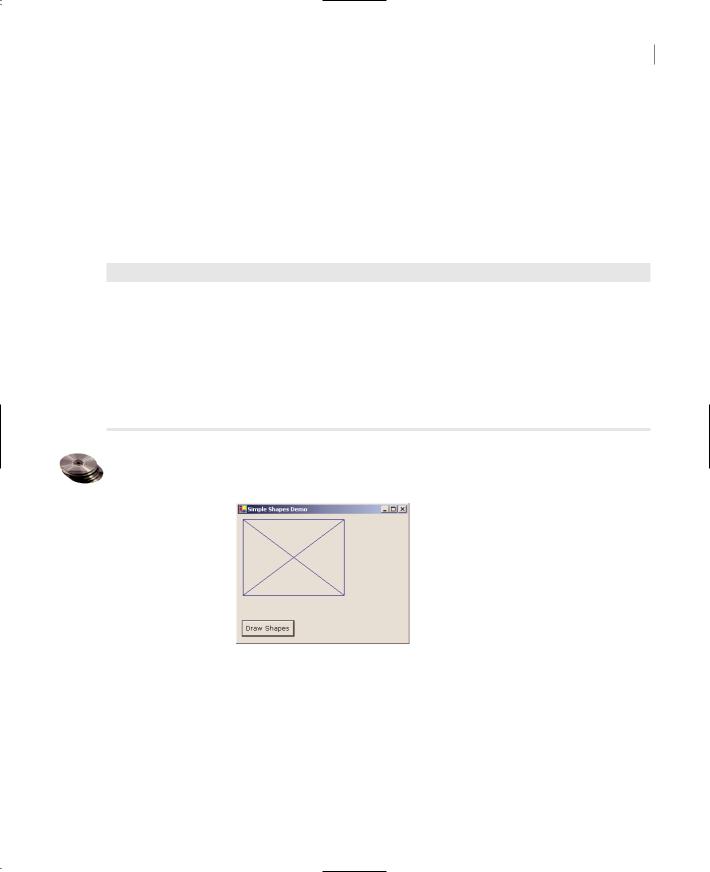
DRAWING WITH GDI+ 643
Let’s add the two diagonals of the rectangle. The diagonals are two lines, one from the top-left to the bottom-right corner of the rectangle and another from top-right to bottom-left. Here are the two statements that draw the diagonals:
G.DrawLine(P, 10, 10, 210, 160)
G.DrawLine(P, 210, 10, 10, 160)
We’ve written all the statements to create a shape on the form, but where do we insert them? Let’s try a Button. Start a new project, place a button on it, and then insert the statements of Listing 14.6 in the Button’s Click event handler.
Listing 14.6: Drawing Simple Shapes
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
Dim G As Graphics
G = Me.CreateGraphics
Dim P As New Pen(Color.Blue)
G.DrawRectangle(P, 10, 10, 200, 150)
G.DrawLine(P, 10, 10, 210, 160)
G.DrawLine(P, 210, 10, 10, 160)
End Sub
Run the application and click the button. You will see the shape shown in Figure 14.10. This figure was created by the SimpleShapes application on the CD.
Figure 14.10
The output of
Listing 14.6
Persistent Drawing
If you switch to the Visual Studio IDE, or any other window, and then return to the form of the SimpleShapes application, you’ll see that the drawing has disappeared! If you’re a VB6 programmer, you’ve recognized that the form’s AutoRedraw property isn’t True. But there’s no AutoRedraw property in VB.NET. Only the bitmap of the PictureBox is persistent. Everything you draw on the Graphics object is temporary. It doesn’t become part of the Graphics object and is visible only while the control, or the form, need not be redrawn. As soon as the form is redrawn, the shapes disappear.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

644 Chapter 14 DRAWING AND PAINTING WITH VISUAL BASIC
So, how do we make the output of the various drawing methods permanent on the form? Microsoft suggests placing all the graphics statements in the OnPaint method, which is activated automatically when the form is redrawn. OnPaint is a method of the form, which is invoked automatically by the operating system and, in turn, it invokes the Paint event. To draw something every time the form is redrawn, place the necessary statements in the OnPaint method.
The OnPaint method accepts a single argument, the e argument, which, among other properties, exposes the form’s Graphics object. You can create a Graphics object in the OnPaint method and then draw on this object. Listing 14.7 is the OnPaint event handler that creates the shape shown in Figure 14.10 and refreshes the form every time it’s totally or partially covered by another form. Delete the code in the button’s Click event handler and insert the subroutine from the listing into the form’s code window.
Listing 14.7: Programming the OnPaint Event
Protected Overrides Sub OnPaint(ByVal e As PaintEventArgs)
Dim G As Graphics
G = e.Graphics
Dim P As New Pen(Color.Blue)
G.DrawRectangle(P, 10, 10, 200, 150)
G.DrawLine(P, 10, 10, 210, 160)
G.DrawLine(P, 210, 10, 10, 160)
End Sub
If you run the application now, it works like a charm. The shapes appear to be permanent, even though they’re redrawn every time you switch to the form. All the samples that come with Visual Studio place the graphics statements in the OnPaint method, so that they’re executed every time the form is redrawn.
This technique is fine for a few graphics elements you want to place on the form to enhance its appearance. But many applications draw something on the form in response to user actions, like the click of a button or a menu commands. Using the OnPaint method in a similar application is out of the question. The drawing isn’t the same, and you must figure out from within your code which shapes you have to redraw at any given time. Consider a drawing application. The current drawing evolves according to the commands you issue. The code in the OnPaint method can’t execute a few drawing commands to regenerate the drawing. Keeping track of the drawing commands that were executed and the order in which they were executed is quite a task. The solution is to make the drawing permanent on the Graphics object, so it won’t have to be redrawn every time the form is hidden or resized.
It is possible to make the graphics permanent by drawing not on the Graphics object, but directly on the control’s (or the form’s) bitmap. The Bitmap object contains the pixels that make up the image and is very similar to the Image object. As you will see, you can create a Bitmap object and assign it to an Image object. In the image-processing application we’ll develop toward the end of this chapter,
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

DRAWING WITH GDI+ 645
you’ll learn how to extract the bitmap from a PictureBox control, process the pixels of a bitmap, and then assign the processed bitmap back to the control’s Image property. In the meantime, you can use the code of Listing 14.7 to create a drawing surface that doesn’t have to be constantly redrawn.
To create this “permanent” drawing surface, you must first create a Bitmap object that has the same dimensions as the PictureBox control you want to draw on:
Dim bmp As Bitmap
bmp = New Bitmap(PictureBox1.Width, PictureBox1.Height)
The bmp variable represents an empty bitmap. Then, we set the control’s Image property to this bitmap with the following statement:
PictureBox1.Image = bmp
Immediately after that, you must set the bitmap to the control’s background color with the Clear method:
G.Clear(PictureBox1.BackColor)
After the execution of this statement, anything we draw on the bmp bitmap is shown on the surface of the PictureBox control and is permanent. All we need is a Graphics object that represents the bitmap, so that we can draw on the control. The following statement creates a Graphics object based on the bmp variable:
Dim G As Graphics
G = Graphics.FromImage(bmp)
Now, we’re in business. We can call the G object’s drawing methods to draw and create permanent graphics on the PictureBox control. You can put all the statements presented so far in a function that returns a Graphics object (Listing 14.8) and use it in your applications.
Listing 14.8: Retrieving a Graphics Object from a PictureBox’s Bitmap
Function GetGraphicsObject(ByVal PBox As PictureBox) As Graphics
Dim bmp As Bitmap
bmp = New Bitmap(PBox.Width, PBox.Height)
PBox.Image = bmp
Dim G As Graphics
G = Graphics.FromImage(bmp)
Return G
End Function
To create permanent drawings on the surface of the PictureBox control, you must call the GetGraphicsObject() function to obtain a Graphics object from the control’s bitmap. The Form object doesn’t expose an Image property, so you must use its BackgroundImage property. Listing 14.9 is the revised GetGraphicsObject() function for the Form object.
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |

646 Chapter 14 DRAWING AND PAINTING WITH VISUAL BASIC
Listing 14.9: Retrieving a Graphics Object from a Form’s Bitmap
Function GetGraphicsObject() As Graphics Dim bmp As Bitmap
bmp = New Bitmap(Me.Width, Me.Height) Dim G As Graphics
Me.BackgroundImage = bmp
G = Graphics.FromImage(bmp) Return G
End Function
Let’s revise the SimpleShapes application so that it draws permanent shapes on the form. Create a new project and place two Button controls on it. Insert the GetGraphicsObject() function in the form’s code window and then the statements shown in Listing 14.10 behind each button. The listing shows the entire code of the SimpleGraphics application, which is on the CD.
Listing 14.10: The SimpleGraphics Application
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click Dim G As Graphics
G = GetGraphicsObject() G.Clear(Color.Silver)
Dim P As New Pen(Color.Blue) G.DrawRectangle(P, 10, 10, 200, 150) G.DrawLine(P, 10, 10, 210, 160) G.DrawLine(P, 210, 10, 10, 160) Me.Invalidate()
End Sub
Private Sub Button2_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button2.Click Me.CreateGraphics.DrawEllipse(Pens.Red, 10, 10, 200, 150)
End Sub
Function GetGraphicsObject() As Graphics Dim bmp As Bitmap
bmp = New Bitmap(Me.Width, Me.Height) Dim G As Graphics
Me.BackgroundImage = bmp
G = Graphics.FromImage(bmp) Return G
End Function
Copyright ©2002 SYBEX, Inc., Alameda, CA |
www.sybex.com |