
ASP.NET 2.0 Everyday Apps For Dummies (2006)
.pdf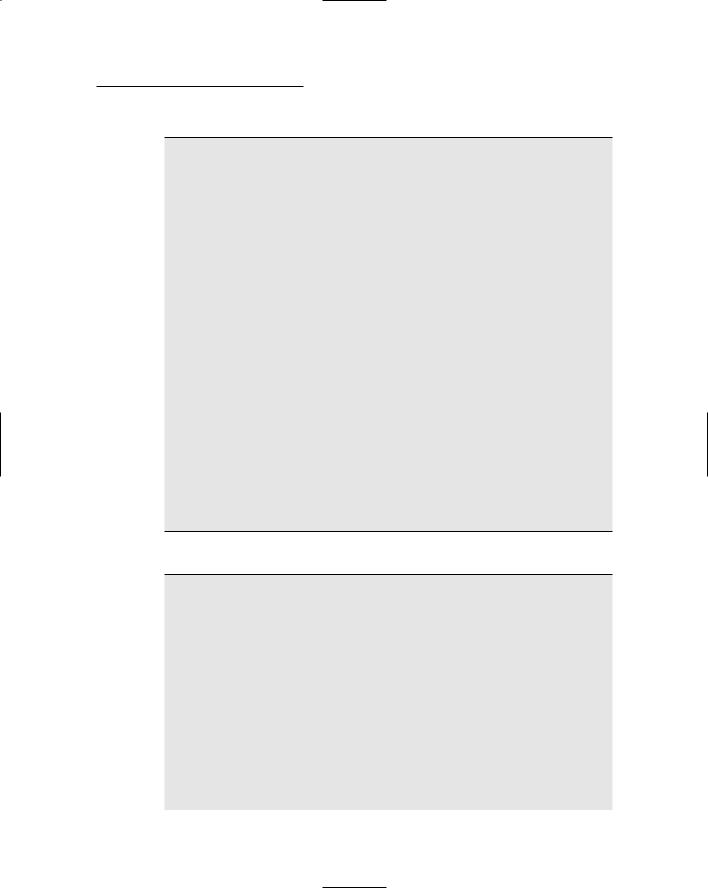
Chapter 6: Building a Shopping Cart Application 167
Listing 6-3: The code-behind file for the Master Page (C#)
using System; using System.Data;
using System.Configuration; using System.Collections; using System.Web;
using System.Web.Security; using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts; using System.Web.UI.HtmlControls;
public partial class MasterPage : System.Web.UI.MasterPage
{
protected void Page_Load(object sender, EventArgs e)
{
ShoppingCart cart = (ShoppingCart)Session[“cart”]; if (cart == null)
lblCart.Text = “Your shopping cart is empty.”; else if (cart.Count == 0)
lblCart.Text = “Your shopping cart is empty.”; else if (cart.Count == 1)
lblCart.Text =
“You have 1 item in your shopping cart.”;
else
lblCart.Text = “You have “
+cart.Count.ToString()
+“ items in your shopping cart.”;
}
}
Listing 6-4: The code-behind file for the Master Page (VB)
Partial Class MasterPage
Inherits System.Web.UI.MasterPage
Protected Sub Page_Load( _
ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles Me.Load
Dim cart As ShoppingCart cart = Session(“cart”) If cart Is Nothing Then
lblCart.Text = “Your shopping cart is empty.” ElseIf cart.Count = 0 Then
lblCart.Text = “Your shopping cart is empty.” ElseIf cart.Count = 1 Then
lblCart.Text =
“You have 1 item in your shopping cart.”
Else
(continued)

168 Part III: Building E-Commerce Applications
Listing 6-4 (continued)
lblCart.Text = “You have “ _
+cart.Count.ToString() _
+“ items in your shopping cart.”
End If
End Sub
End Class
As you can see, the code-behind file has just one method, named Page_Load, which is executed when the page is loaded. It retrieves the shopping cart from session state, casts it as a ShoppingCart object, then sets the label accordingly. If the cart doesn’t exist or is empty, the label is set to “Your shopping cart is empty.” If the cart contains exactly one item, the label is set to “You have 1 item in your shopping cart.” And if the cart has more than one item, the label is set to “You have n items in your shopping cart.”
Modifying the Product Detail Page
The Product Detail page (Product.aspx) is almost identical to the Product Detail page for the Product Catalog application shown in Chapter 5. However, there’s one crucial difference. In the Chapter 5 application, clicking the Add to Cart button simply led the user to a page that indicates that the shopping cart feature hasn’t yet been implemented. But in this application, clicking the Add to Cart button must actually add the product to the shopping cart, then redirect the user to the Cart.aspx page to see the contents of the shopping cart.
To add this feature to the Product Detail page, you must modify the method that’s called when the user clicks the Add to Cart button, btnAdd_Click. The rest of the page is unchanged from the Chapter 5 application.
Listing 6-5 shows the C# version of the btnAdd_Click method, the method that’s called when the user clicks the Add to Cart button. Listing 6-6 shows the Visual Basic version of this method. (To see the .aspx file for the Product Detail page, please refer back to Chapter 5.)
Listing 6-5: The btnAdd_Click method (C#)
protected void btnAdd_Click(object sender, EventArgs e)
{
// get values from data source |
1 |
DataView dv = (DataView)SqlDataSource1.Select(
DataSourceSelectArguments.Empty); DataRowView dr = dv[0];
string ID = (String)dr[“ProductID”]; string Name = (string)dr[“Name”];
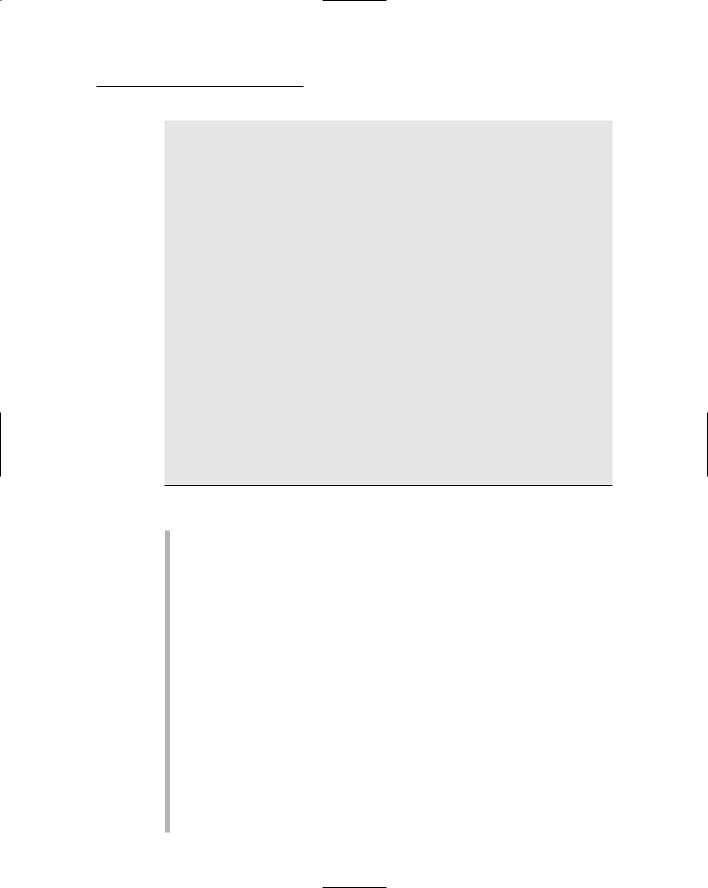
Chapter 6: Building a Shopping Cart Application 169
decimal Price;
if (dr[“SalePrice”] is DBNull) Price = (decimal)dr[“Price”];
else
Price |
= (decimal)dr[“SalePrice”]; |
|
// get or |
create shopping cart |
2 |
ShoppingCart cart;
if (Session[“cart”] == null)
{
cart = new ShoppingCart(); Session[“cart”] = cart;
}
else
{
cart = (ShoppingCart)Session[“cart”];
}
//add item to cart cart.AddItem(ID, Name, Price);
//redirect to cart page
string ProductID = Request.QueryString[“prod”]; string CatID = Request.QueryString[“cat”]; Response.Redirect(“Cart.aspx?prod=” + ProductID
+ “&cat=” + CatID);
}
3
4
The following paragraphs describe the key points of this method:
1 The btnAdd_Click method begins by retrieving the ID, name, and price information for the current product from the data source. You’d think that it would be pretty easy to retrieve the product data displayed by the form, but it turns out to be a little tricky. The easiest technique is to use the Select method of the data source to retrieve a data view object that contains the data retrieved by the data source’s SELECT statement. Because the SELECT statement for this data source retrieves the data for a single product, the resulting data view will have just one row. The indexer for the DataView object lets you retrieve the individual rows of the data view. Thus, index 0 is used to retrieve a DataRowView object for the data view’s only row. Then the individual columns are retrieved from the DataRowView object using the column names as indexes.
Note that if a SalePrice column is present, it is used instead of the Price column for the product’s price.
2 Once the product information has been retrieved from the data source, session state is checked to see if a shopping cart already exists. If so, the shopping cart is retrieved from session state. If not, the application creates a new shopping cart by calling the
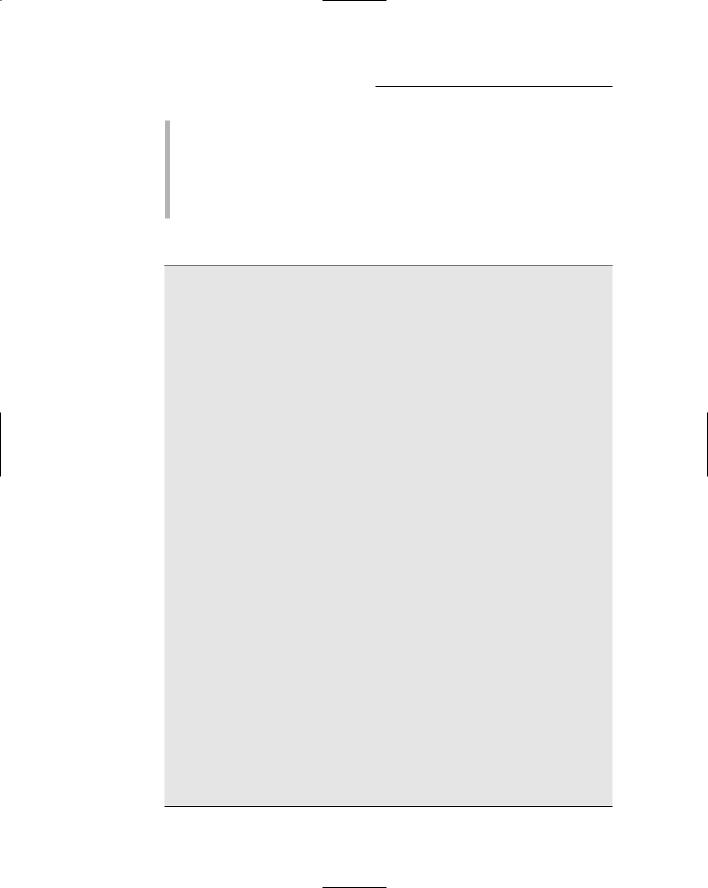
170 Part III: Building E-Commerce Applications
ShoppingCart class constructor. Then the new shopping cart is added to session state under the name “cart.”
3 The AddItem method of the shopping cart is called to add the product to the shopping cart.
4 The user is redirected to the Cart.aspx page, with the product and category IDs passed on as query string fields.
Listing 6-6: The btnAdd_Click method (VB)
Protected Sub btnAdd_Click( _
ByVal sender As Object, _
ByVal e As System.EventArgs) _
Handles btnAdd.Click
‘get values from data source Dim dv As DataView
dv = SqlDataSource1.Select( _ DataSourceSelectArguments.Empty)
Dim dr As DataRowView = dv(0)
Dim ID As String = dr(“ProductID”) Dim name As String = dr(“Name”) Dim Price As Decimal
If TypeOf (dr(“SalePrice”)) Is DBNull Then Price = dr(“Price”)
Else
Price = dr(“SalePrice”) End If
‘get or create shopping cart
Dim cart As ShoppingCart
If Session(“cart”) Is Nothing Then cart = New ShoppingCart() Session(“cart”) = cart
Else
cart = Session(“cart”) End If
‘add item to cart cart.AddItem(ID, name, Price)
‘redirect to cart page
Dim ProductID As String
ProductID = Request.QueryString(“prod”) Dim CatID As String
CatID = Request.QueryString(“cat”) Response.Redirect( _
“Cart.aspx?prod=” + ProductID _ + “&cat=” + CatID)
End Sub
1
2
3
4

Chapter 6: Building a Shopping Cart Application 171
Building the Cart Page
The Cart page (Cart.aspx) displays the user’s shopping cart and lets the user modify the shopping cart by changing the quantity ordered or by deleting items. There are two ways the user can display this page. One is to click the Add to Cart button on the Product Detail page. The other is to click the Go To Cart link that appears beneath the banner at the top of each page of the application. To see what this page looks like, refer to Figure 6-4.
The following sections present the .aspx code for the cart page and the C# and Visual Basic versions of the code-behind file.
The Cart.aspx file
The Cart page is defined by the Cart.aspx file, which is shown in Listing 6-7.
Listing 6-7: The Cart Page (Cart.aspx)
<%@ Page Language=”C#” |
1 |
MasterPageFile=”~/MasterPage.master” |
|
AutoEventWireup=”true” |
|
CodeFile=”Cart.aspx.cs” |
|
Inherits=”Cart” |
|
Title=”Acme Pirate Supply” %> |
|
<asp:Content ID=”Content1” Runat=”Server” |
|
ContentPlaceHolderID=”ContentPlaceHolder1” > |
|
<br /> |
|
<asp:GridView ID=”GridView1” runat=”server” |
2 |
AutoGenerateColumns=”False” |
|
EmptyDataText=”Your shopping cart is empty.” |
|
OnRowDeleting=”GridView1_RowDeleting” |
|
OnRowEditing=”GridView1_RowEditing” |
|
OnRowUpdating=”GridView1_RowUpdating” |
|
OnRowCancelingEdit=”GridView1_RowCancelingEdit”> |
|
<Columns> |
|
<asp:BoundField DataField=”ID” |
3 |
HeaderText=”Product” ReadOnly=”True” > |
|
<HeaderStyle HorizontalAlign=”Left” /> |
|
</asp:BoundField> |
|
<asp:BoundField DataField=”Name” |
4 |
HeaderText=”Name” ReadOnly=”True” > |
|
<HeaderStyle HorizontalAlign=”Left” /> |
|
</asp:BoundField> |
|
<asp:BoundField DataField=”Price” |
5 |
HeaderText=”Price” ReadOnly=”True” |
|
DataFormatString=”{0:c}” > |
|
<HeaderStyle HorizontalAlign=”Left” /> |
|
|
|
(continued)
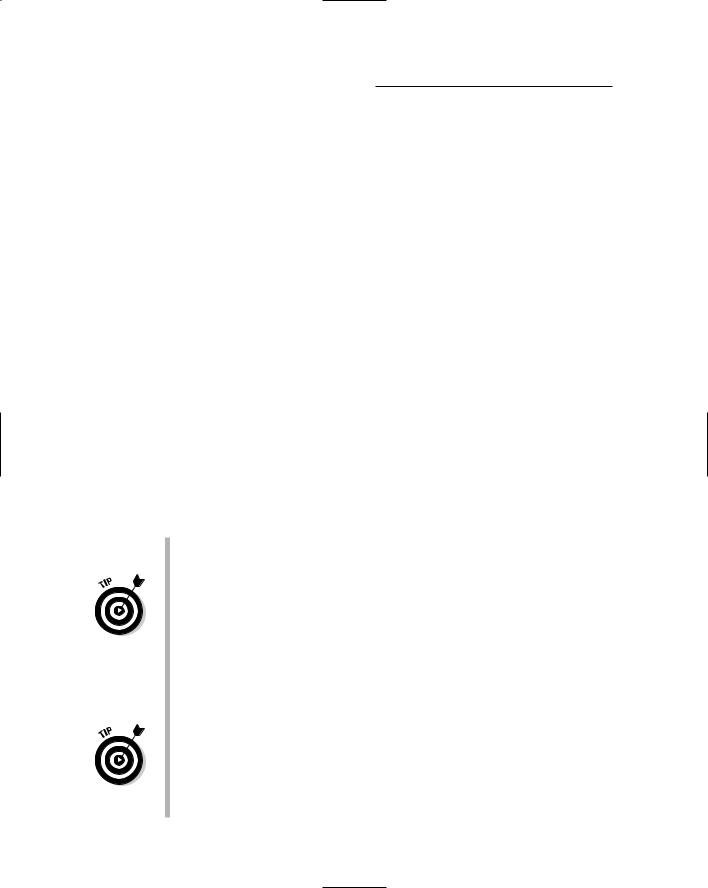
172 Part III: Building E-Commerce Applications
Listing 6-7 (continued)
|
</asp:BoundField> |
|
|
<asp:BoundField DataField=”Quantity” |
6 |
|
HeaderText=”Quantity” > |
|
|
<HeaderStyle HorizontalAlign=”Left” /> |
|
|
</asp:BoundField> |
|
|
<asp:BoundField DataField=”Total” |
7 |
|
HeaderText=”Total” ReadOnly=”True” |
|
|
DataFormatString=”{0:c}” > |
|
|
<HeaderStyle HorizontalAlign=”Left” /> |
|
|
</asp:BoundField> |
|
|
<asp:CommandField EditText=”Change” |
8 |
|
ShowDeleteButton=”True” |
|
|
ShowEditButton=”True” > |
|
|
<ItemStyle BorderStyle=”None” /> |
|
|
<HeaderStyle BorderStyle=”None” /> |
|
|
</asp:CommandField> |
|
|
</Columns> |
|
</asp:GridView> |
|
|
<br /><br /> |
|
|
<asp:Button ID=”btnContinue” runat=”server” |
9 |
|
|
OnClick=”btnContinue_Click” |
|
|
Text=”Continue Shopping” /> |
|
<asp:Button ID=”btnCheckOut” runat=”server” |
10 |
|
|
PostBackUrl=”~/CheckOut.aspx” |
|
|
Text=”Check Out” /> |
|
</asp:Content> |
|
|
The following paragraphs describe the important elements of this listing: |
||
1 |
The Page directive specifies that the page will use MasterPage. |
|
|
master as its Master Page. |
|
|
For the Visual Basic version of this application, be sure to change |
|
|
the AutoEventWireup attribute of the Page directive to false. |
|
|
That enables the Handles clause of the Sub procedures. (If you |
|
|
don’t change this setting, the events for the GridView control |
|
|
included on this page won’t be processed correctly.) |
|
2 The GridView control displays the user’s shopping cart. Notice that unlike the GridView controls used on the Default.aspx page, this GridView control doesn’t specify a data source.
Instead, the data source will be specified at run time, when the Page_Load method is executed.
For the Visual Basic version of this application, you need to remove the four attributes that specify the event handling for this control. Specifically, you need to remove the following four attributes:
•OnRowDeleting
•OnRowEditing
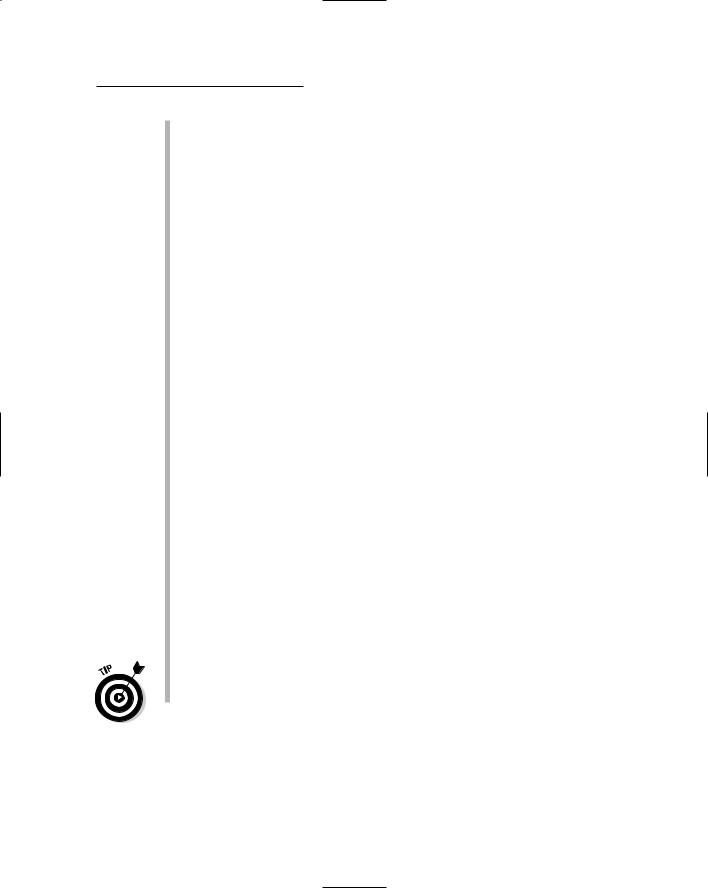
Chapter 6: Building a Shopping Cart Application 173
•OnRowUpdating
•OnRowCancelingEdit
If you don’t remove these attributes, the corresponding events in the Visual Basic code-behind file will be executed twice each time the event is raised.
Notice that AutoGenerateColumns is set to false. Then the GridView control doesn’t automatically create a column for each field in the data source. Instead, you must manually configure the columns by using the <Columns> element.
3 The first column in the GridView control is bound to the data source field named ID. The heading for this column is set to “Product,” and the column is defined as read-only to prevent the user from modifying it.
4 The second column in the GridView control is bound to the Name field. This column is also defined as read-only to prevent the user from modifying it.
5 The next column is bound to the Price field. It uses a format string to display the price in currency format. It too is read-only.
6 Unlike the other columns in the GridView control, the Quantity column isn’t read-only. As a result, the user can modify its contents when the row is placed into Edit mode.
7 The Total column displays the item total (the price times the quantity) in currency format. It is read-only.
8 The last column in the shopping cart GridView control is a command column that lets the user edit or delete a shopping cart row. The ShowEditButton and ShowDeleteButton attributes are required to display the Edit and Delete buttons, and the EditButtonText attribute changes the text displayed in the edit button from the default (“Edit”) to “Change.”
9 The Continue button lets the user continue shopping by returning to the product pages.
10 The Check Out button lets the user proceed to the checkout page.
For the Visual Basic version of this application, you should remove the OnClick attribute for this control.
The code-behind file for the Cart page
Listing 6-8 shows the C# version of the code-behind file for the Cart page, and Listing 6-9 shows the Visual Basic version.

174 Part III: Building E-Commerce Applications
Listing 6-8: The code-behind file for the Cart page (C# version)
using System; using System.Data;
using System.Configuration; using System.Collections; using System.Web;
using System.Web.Security; using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts; using System.Web.UI.HtmlControls;
using System.Collections.Generic;
public partial class Cart : System.Web.UI.Page
{
ShoppingCart cart;
protected void Page_Load(object sender, EventArgs e)
{
CheckTimeStamps();
if (Session[“cart”] == null)
{
cart = new ShoppingCart(); Session[“cart”] = cart;
}
else
{
cart = (ShoppingCart)Session[“cart”];
}
GridView1.DataSource = cart.GetItems(); if (!IsPostBack)
GridView1.DataBind();
btnCheckOut.Enabled = (cart.Count > 0);
}
protected void GridView1_RowDeleting(
object sender, GridViewDeleteEventArgs e)
{
cart.DeleteItem(e.RowIndex);
GridView1.DataBind();
}
protected void GridView1_RowEditing( object sender, GridViewEditEventArgs e)
{
GridView1.EditIndex = e.NewEditIndex; GridView1.DataBind();
1
2
3
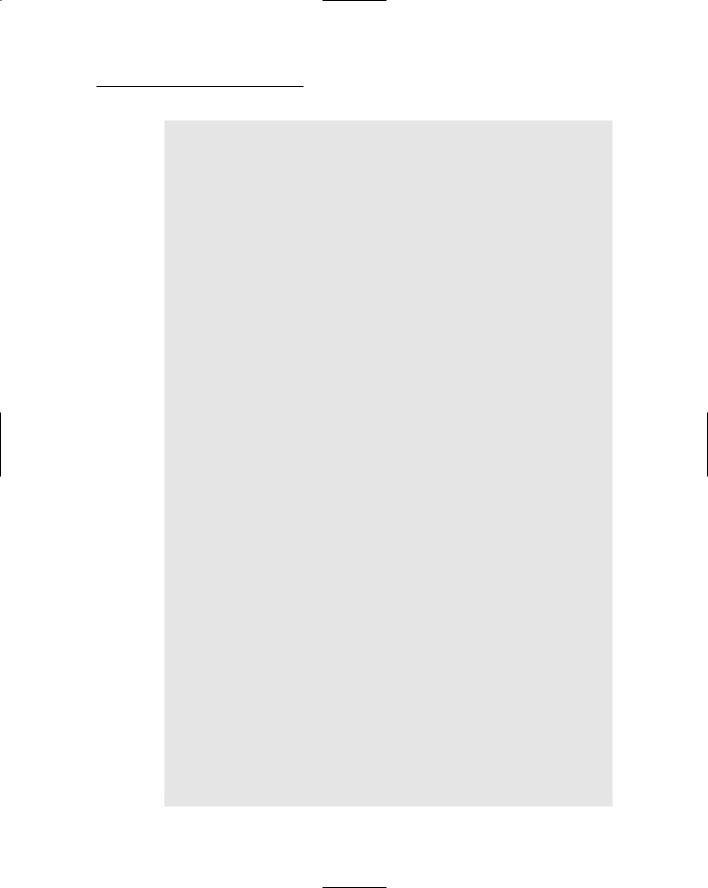
Chapter 6: Building a Shopping Cart Application 175
} |
|
protected void GridView1_RowUpdating( |
4 |
object sender, GridViewUpdateEventArgs e) |
|
{ |
|
DataControlFieldCell cell = |
|
(DataControlFieldCell)GridView1 |
|
.Rows[e.RowIndex].Controls[3]; |
|
TextBox t = (TextBox)cell.Controls[0]; |
|
try |
|
{ |
|
int q = int.Parse(t.Text); |
|
cart.UpdateQuantity(e.RowIndex, q); |
|
} |
|
catch (FormatException) |
|
{ |
|
e.Cancel = true; |
|
} |
|
GridView1.EditIndex = -1; |
|
GridView1.DataBind(); |
|
} |
|
protected void GridView1_RowCancelingEdit( |
5 |
object sender, GridViewCancelEditEventArgs e) |
|
{ |
|
e.Cancel = true; |
|
GridView1.EditIndex = -1; |
|
GridView1.DataBind(); |
|
} |
|
protected void btnContinue_Click( |
6 |
object sender, EventArgs e) |
|
{ |
|
string ProductID = Request.QueryString[“prod”]; string CatID = Request.QueryString[“cat”];
if (ProductID == null) if (CatID == null)
Response.Redirect(“Default.aspx”); else
Response.Redirect(“Default.aspx?cat=” + CatID);
else Response.Redirect(“Product.aspx?prod=”
+ProductID
+“&cat=” + CatID);
}
private void CheckTimeStamps() |
7 |
{ |
|
if (IsExpired()) Response.Redirect(Request.Url.OriginalString);
(continued)
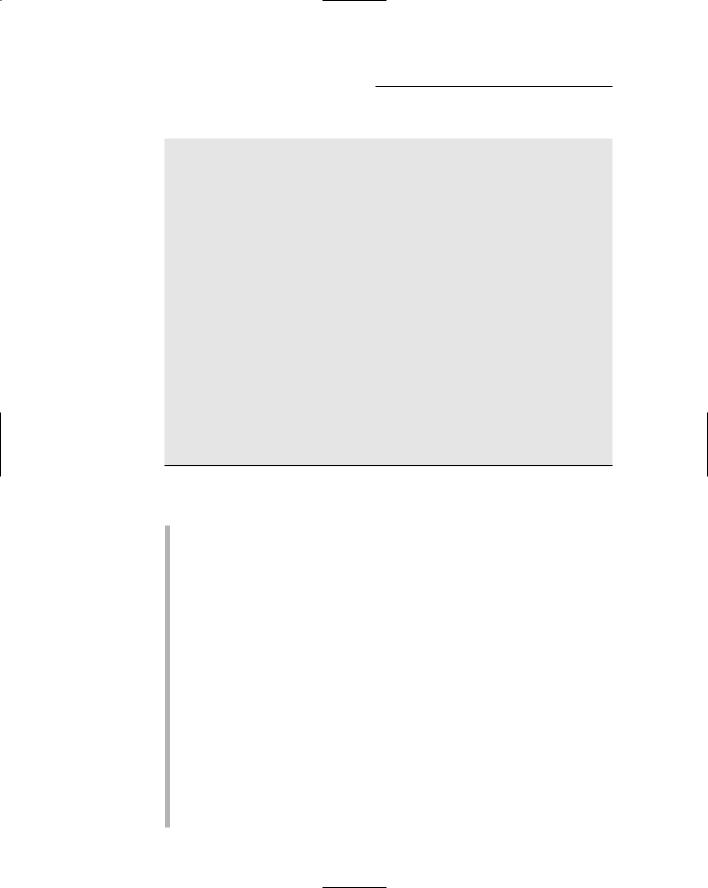
176 Part III: Building E-Commerce Applications
Listing 6-8 (continued)
else
{
DateTime t = DateTime.Now; ViewState.Add(“$$TimeStamp”, t); String page = Request.Url.AbsoluteUri; Session.Add(page + “_TimeStamp”, t);
}
} |
|
private bool IsExpired() |
8 |
{ |
|
String page = Request.Url.AbsoluteUri;
if (Session[page + “_TimeStamp”] == null) return false;
else if (ViewState[“$$TimeStamp”] == null) return false;
else if (Session[page + “_TimeStamp”].ToString() == ViewState[“$$TimeStamp”].ToString())
return false; else
return true;
}
}
The following paragraphs describe the methods in this code-behind file. Note that these comments apply to both the C# and the VB versions.
1 Page_Load: This method is called when the page loads. It begins by calling a method named CheckTimeStamps. I’ll explain how this method works later in this section. For now, just realize that this method forces the page to refresh if the user has come to the page by using the browser’s back button. This approach prevents problems that can occur when the user backs up to a version of the page that shows a shopping cart with contents that differ from the shopping cart that’s stored in session state.
Assuming that the CheckTimeStamps method didn’t force the page to refresh, the Page_Load method next checks to see if session state contains an item named cart. If not, a new shopping cart is created and saved in session state. But if an item named cart does exist, the cart item is retrieved, cast to a ShoppingCart object, and assigned to the cart variable.
Next, the shopping cart’s GetItems method is called. This returns a List object that holds a CartItem object for each item in the shopping cart. This List object is used as the data source for the GridView control. And if this is the first time the page has been posted, the DataBind method of the GridView control is called so it displays the contents of the shopping cart.