
Professional Visual Studio 2005 (2006) [eng]
.pdf
Chapter 10
Resource Files: A Word of Caution
Resource files are the best strategy for storing static application data. Although resource files are linked into the application as part of the compilation process, the contents of the files can easily be extracted and made human readable. As such, resource files are not suitable for storing secure data such as passwords and credit card information.
Custom Resources
Although Visual Studio provides good support for international application development using resource files, there are times when it is not possible to get the level of control required using the default behavior. This section delves a little deeper into how you can serialize custom objects to the resource file and how you can generate designer files, which give you strongly typed accessor methods for resource files you have created.
Visual Studio 2005 enables you to store strings, images, icons, audio files, and other files within a resource file. This can all be done using the rich user interface provided. To store a more complex data type within a resource file, you need to serialize it into a string representation that can be included within the resource file.
The first step in adding any data type into a resource file is to make that data type serializable. This can be achieved easily by marking the class with the Serializable attribute. Once it is marked as serializable, the object can be added to a resource file using an implementation of the IResourceWriter interface — for example, the ResXResourceWriter:
<Serializable()> _ Public Class Person
Public Name As String Public Height As Integer Public Weight As Double
End Class
Dim p As New Person
p.Name = “Bob” p.Height = 167 p.Weight = 69.5
Dim rWriter As New ResXResourceWriter(“foo.resx”) rWriter.AddResource(“DefaultPerson”, p) rWriter.Close()
Serializing an object this way has a couple of drawbacks, however:
Using code to write out this resource file needs to be done prior to the build process so that the resource file can be included in the application. Clearly, this becomes an administrative nightmare, as it is an additional stage in the build process.
Furthermore, the serialized representation of the class is a binary blob and is not human readable. The assumption here is that what is written in the generating code is correct. Unfortunately, this is seldom the case, and it would be easier if the content could be human readable within Visual Studio 2005.
136

XML Resource Files
A workaround for both these issues is to define a TypeConverter for the class and use that to represent the class as a string. This way, the resource can be edited within the Visual Studio resource editor. TypeConverters provide a mechanism through which the framework can determine whether it is possible to represent a class (in this case, a Person class) as a different type (in this case, as a string). The first step is to create a TypeConverter as follows:
Imports System.ComponentModel
Imports System.ComponentModel.Design.Serialization
Imports System.Globalization
Public Class PersonConverter
Inherits TypeConverter
Public Overrides Function CanConvertFrom _
(ByVal context As ITypeDescriptorContext, _ ByVal sourceType As Type) As Boolean
If (sourceType Is GetType(String)) Then Return True
End If
Return MyBase.CanConvertFrom(context, sourceType) End Function
Public Overrides Function CanConvertTo _
(ByVal context As ITypeDescriptorContext, _ ByVal destinationType As Type) As Boolean
If (destinationType Is GetType(InstanceDescriptor)) Then Return True
End If
Return MyBase.CanConvertTo(context, destinationType) End Function
Public Overrides Function ConvertFrom _
(ByVal context As ITypeDescriptorContext, _ ByVal culture As CultureInfo, _
ByVal value As Object) As Object
Dim text1 As String = TryCast(value, String) If (text1 Is Nothing) Then
Return MyBase.ConvertFrom(context, culture, value) End If
Dim text2 As String = text1.Trim If (text2.Length = 0) Then
Return Nothing End If
If (culture Is Nothing) Then
culture = CultureInfo.CurrentCulture End If
Dim ch1 As Char = culture.TextInfo.ListSeparator.Chars(0) Dim chArray1 As Char() = New Char() {ch1}
Dim textArray1 As String() = text2.Split(chArray1) If (textArray1.Length = 3) Then
Dim o As New Person o.Name = textArray1(0)
o.Height = CInt(textArray1(1)) o.Weight = CDbl(textArray1(1)) Return o
137
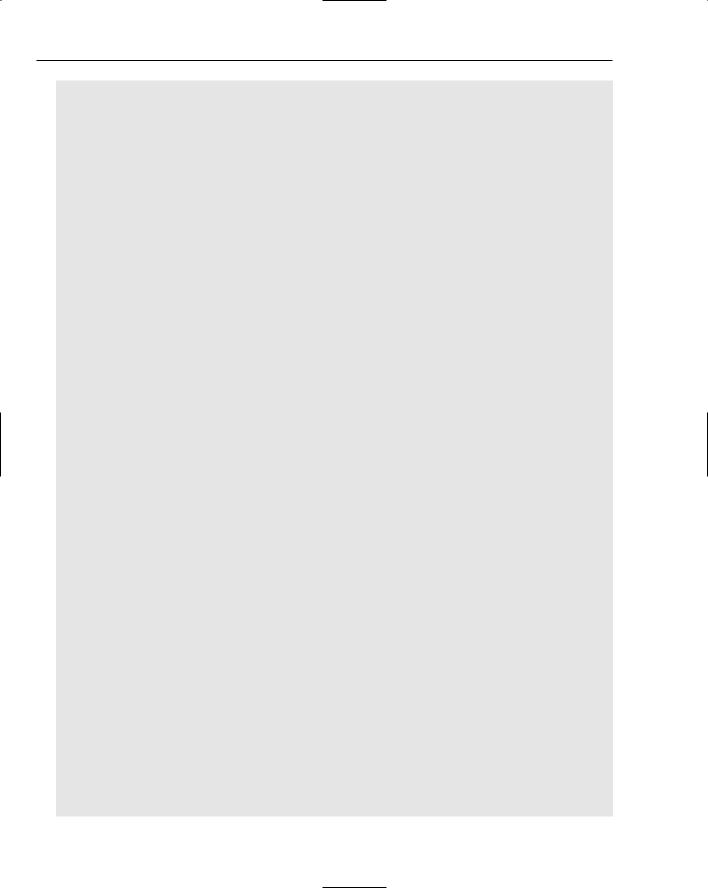
Chapter 10
End If
Throw New ArgumentException(“Unable to convert From”)
End Function
Public Overrides Function GetCreateInstanceSupported _
(ByVal context As ITypeDescriptorContext) As Boolean
Return True
End Function
Public Overrides Function GetProperties _
(ByVal context As ITypeDescriptorContext, _ ByVal value As Object, _
ByVal attributes As Attribute()) As PropertyDescriptorCollection Dim collection1 As PropertyDescriptorCollection = _
TypeDescriptor.GetProperties(GetType(Person), attributes)
Dim textArray1 As String() = New String() {“Name”, “Height”, “Weight”} Return collection1.Sort(textArray1)
End Function
Public Overrides Function ConvertTo _
(ByVal context As ITypeDescriptorContext, _ ByVal culture As CultureInfo, _
ByVal value As Object, _
ByVal destinationType As Type) As Object If (destinationType Is Nothing) Then
Throw New ArgumentNullException(“Unable to convert To”) End If
If TypeOf value Is Person Then
If (destinationType Is GetType(Person)) Then
Dim o As Person = CType(value, Person) If (culture Is Nothing) Then
culture = CultureInfo.CurrentCulture End If
Dim text1 As String = (culture.TextInfo.ListSeparator & “ “) Dim converter1 As TypeConverter = _
TypeDescriptor.GetConverter(GetType(Integer)) Dim textArray1 As String() = New String(2) {} Dim num1 As Integer = 0
textArray1(num1) = _ converter1.ConvertToString(context, culture, o.Name)
num1 += 1 textArray1(num1) = _
converter1.ConvertToString(context, culture, o.Height) num1 += 1
textArray1(num1) = _
converter1.ConvertToString(context, culture, o.Weight) num1 += 1
Return String.Join(text1, textArray1) End If
End If
Return MyBase.ConvertTo(context, culture, value, destinationType) End Function
Public Overrides Function CreateInstance _
138

XML Resource Files
(ByVal context As ITypeDescriptorContext, _ ByVal propertyValues As IDictionary) As Object
If (propertyValues Is Nothing) Then
Throw New ArgumentNullException(“propertyValues”) End If
Dim obj1 As Object = propertyValues.Item(“Name”) Dim obj2 As Object = propertyValues.Item(“Height”) Dim obj3 As Object = propertyValues.Item(“Weight”) If ( _
((obj1 Is Nothing) OrElse _ (obj2 Is Nothing) OrElse _ (obj3 Is Nothing)) _
OrElse _
(Not TypeOf obj1 Is String OrElse _ Not TypeOf obj2 Is Integer OrElse _ Not TypeOf obj3 Is Double) _
) Then
Throw New ArgumentException(“Unable to Create Instance”) End If
Dim o As New Person
o.Name = CType(obj1, String) o.Height = CType(obj2, Integer) o.Weight = CType(obj3, Double) Return o
End Function
Public Overrides Function GetPropertiesSupported _
(ByVal context As ITypeDescriptorContext) As Boolean
Return True
End Function
End Class
The class being represented also needs to be attributed with the TypeConverter attribute:
<System.ComponentModel.TypeConverter(GetType(PersonConverter))> _ <Serializable()> _
Public Class Person Public Name As String
Public Height As Integer
Public Weight As Double End Class
Now this item can be added to a resource file using the string representation of the class. For example, an entry in the resx file might look like this:
<assembly alias=” WindowsApplication1” name=”WindowsApplication1, Version=1.0.0.0, Culture=neutral, PublicKeyToken=null” />
<data name=”Manager” type=” WindowsApplication1.Person, WindowsApplication1”> <value>Joe, 175, 69.5</value>
</data>
139

Chapter 10
Designer Files
The resource generator utility, resgen, has a number of improvements that enable you to build strongly typed wrapper classes for your resource files. When you add a resx file to your application, Visual Studio will automatically create a designer file that wraps the process of creating a resource manager and accessing the resources by name. The accessor properties are all strongly typed and are generated by the designer to reduce the chance of invalid type conversions and references.
Unfortunately, Visual Studio 2005 does not automatically generate the designer file for text resource files, because text resource files cannot be explicitly added to the application. The process of generating a resource file from the text file can be extended to include the generation of the designer file.
A new argument has been added to resgen that facilitates the generation of this designer file:
resgen sample.txt sample.resources /str:vb
Both of the output files need to be added into the application so that the resources are accessible. In order to ensure that the resources can be correctly accessed, it is important to ensure that the naming used within the designer file matches the naming of the compiled resources. Additional parameters can be provided to control the namespace, class name, and output filename:
resgen sample.txt defaultnamespace.sample.resources
/str:vb,defaultnamespace,sample,sample.vb
In this case, the fully qualified output class would be defaultnamespace.sample, and the use of this file would allow access to resources without an exception being raised. Once the correct command has been determined, you can update your prebuild event to include the generation of the designer file. This way, every time the file is modified and saved and the application is compiled, the designer file will be current.
Summar y
This chapter demonstrated how important XML resource files are to building an application that can both access static data and be easily localized into foreign languages and cultures. The rich user interface provided within Visual Studio 2005 allows resources such as images, icons, strings, audio, and other files to be easily added to an application.
The built-in support for localizing forms and generating satellite assemblies empowers developers to write applications that can target a global market. You have also seen that the user interface provided within Visual Studio 2005 is extensible, enabling you to modify it to interact with your own custom resource types.
140
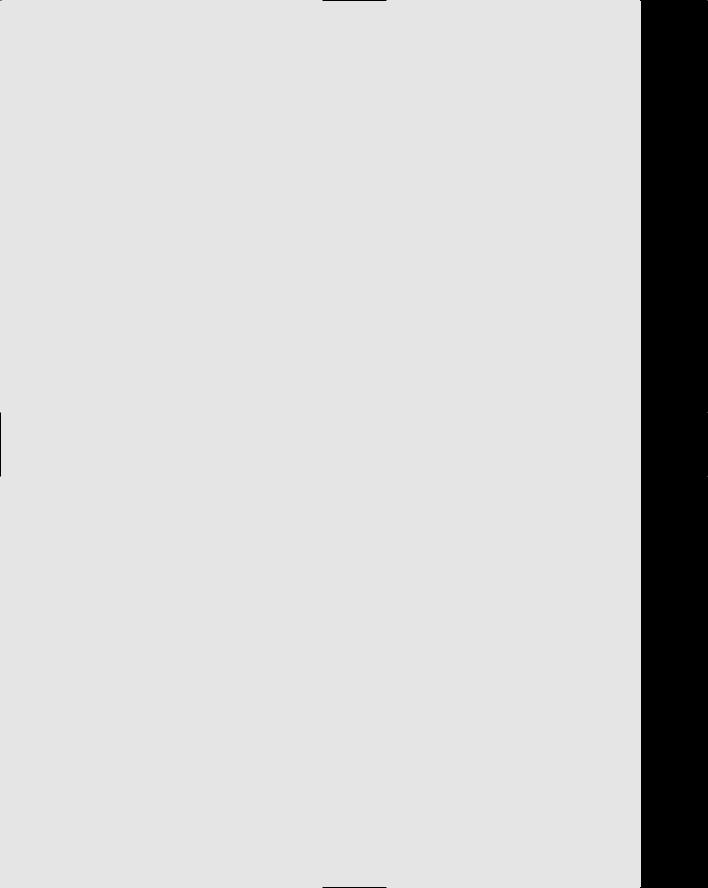
Part III
Documentation and
Research
Chapter 11: Help and Research
Chapter 12: XML Comments
Chapter 13: Control and Document Outline

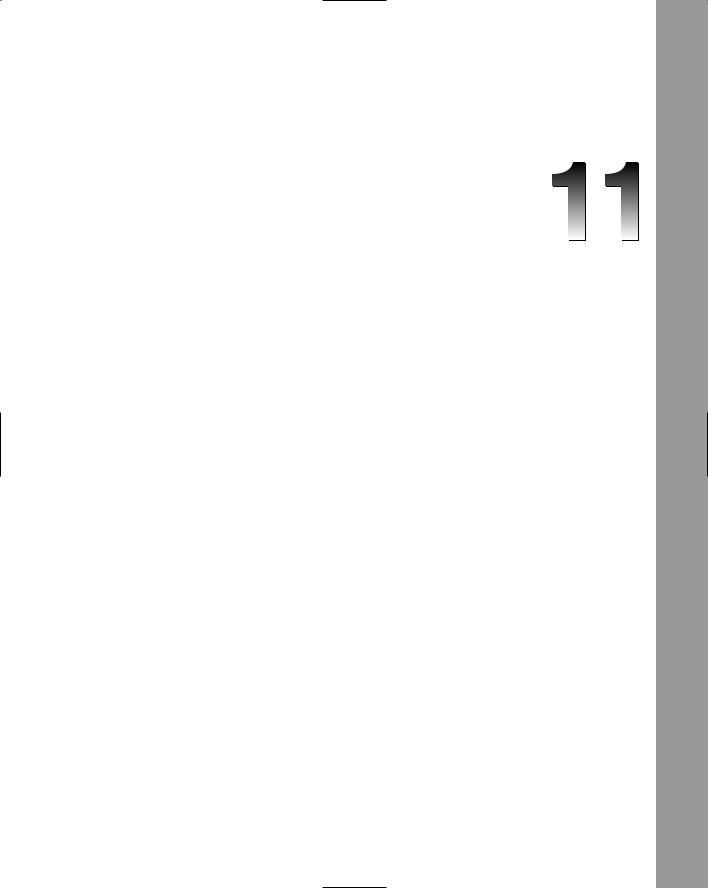
Help and Research
Visual Studio 2005 is an immensely complex development environment that encompasses multiple languages based on an extensive framework of libraries and components. It’s almost impossible to know everything about the IDE, let alone each of the languages or even the full extent of the .NET Framework.
This poses some challenges because all aspects of the development toolset have undergone significant changes since the last version. The .NET Framework has gone through a major revision with 2.0, the languages have evolved to synchronize with the Framework, and important features are introduced. The development environment has also been modified, as shown in Part I of this book.
The huge amount of knowledge required to fully understand every facet of this product is beyond the scope of a single book or course. More than likely, you’ll periodically need to obtain more information on a specific topic. To help you in these situations, Visual Studio 2005 comes with comprehensive documentation in the form of the MSDN Library, Visual Studio 2005 Edition. This chapter walks through the methods of researching documentation associated with developing projects in Visual Studio 2005, and discusses the new interface for help that has been introduced in this latest version.
Accessing Help
The easiest way to get help for Visual Studio 2005 is to use the same method you would use for almost every Windows application ever created — press the F1 key, the universal shortcut key for help. If you do so, the first thing you’ll notice is that help is contextual. For instance, if the cursor were currently positioned on or inside a class definition in a Visual Basic project, the help window would open immediately with a mini tutorial about what the class statement is and how it works, as shown in Figure 11-1.
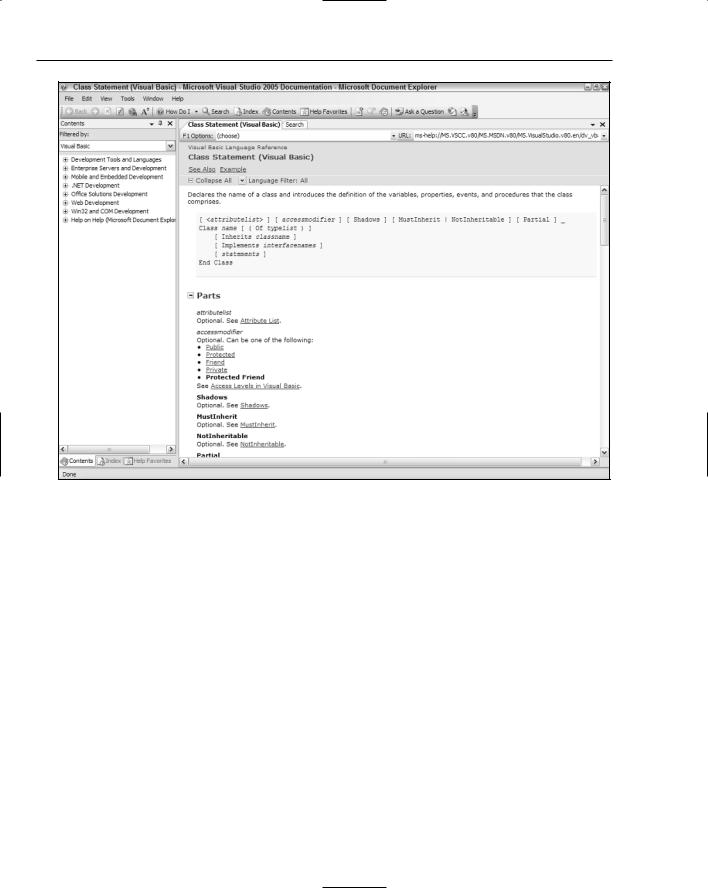
Chapter 11
Figure 11-1
This is incredibly useful because more often than not you can go directly to a help topic in the documentation that deals with the problem you’re currently researching simply by choosing the right context and pressing F1.
However, in some situations you will want to go directly to the table of contents, or the search page within the help system. Visual Studio 2005 provides the capability for doing this through its main Help menu (see Figure 11-2). The following table summarizes the commands related to help documentation (note the different keyboard shortcuts depending on your environment setup):
|
Visual Basic |
|
|
Menu Command |
Shortcut |
C# Shortcut |
Action |
|
|
|
|
How Do I |
Ctrl+F1 |
Ctrl+F1, H |
Opens a special set of documentation |
|
|
|
that introduces basic programming con- |
|
|
|
cepts for the language you’re working in |
Search |
Ctrl+Alt+F3 |
Ctrl+F1, S |
Opens the help Document Explorer, |
|
|
|
with the Search page active and ready |
|
|
|
for you to perform a research action |
|
|
|
|
144

|
|
|
|
|
Help and Research |
|
|
|
|
|
|
|
|
Visual Basic |
|
|
|
|
Menu Command |
Shortcut |
C# Shortcut |
Action |
|
|
|
|
|
|
|
|
Contents |
Ctrl+Alt+F1 |
Ctrl+F1, C |
Makes the Contents tab the active one in |
|
|
|
|
|
the help Document Explorer |
|
|
Index |
Ctrl+Alt+F2 |
Ctrl+F1, I |
Shows the Index tab in the help Docu- |
|
|
|
|
|
ment Explorer |
|
|
Help Favorites |
Ctrl+Alt+F |
Ctrl+F1, F |
Opens the help Document Explorer with |
|
|
|
|
|
the Help Favorites tab active |
|
|
Index Results |
Shift+Alt+F2 |
Ctrl+F1, T |
Opens the help Document Explorer and |
|
|
|
|
|
then opens the Index Results window so |
|
|
|
|
|
you can view the results of a previous |
|
|
|
|
|
search |
|
|
Dynamic Help |
Ctrl+Alt+F4 |
Ctrl+F1, D |
Dynamic Help is a special tool window |
|
|
|
|
|
that dynamically changes its contents |
|
|
|
|
|
based on the context of what you’re |
|
|
|
|
|
doing. |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
Figure 11-2
Document Explorer
The commands shown in the preceding table, with the exception of Dynamic Help, will open the main help documentation for Visual Studio 2005. With this latest version of Visual Studio, Microsoft has introduced a completely new help system, utilizing an interface known as the Document Explorer. Based on a combination of HTML Help, modern web browsers, and the Visual Studio 2005 IDE, the Document Explorer is a feature-rich application in its own right that can perform powerful functions.
Despite the revolutionary changes made to the documentation system, the Document Explorer still presents a familiar interface. It’s constructed using regular Windows application standards: customizable
145