
- •Table of Contents
- •Preface
- •What is ASP.NET?
- •Installing the Required Software
- •Installing the Web Server
- •Installing Internet Information Services (IIS)
- •Installing Cassini
- •Installing the .NET Framework and the SDK
- •Installing the .NET Framework
- •Installing the SDK
- •Configuring the Web Server
- •Configuring IIS
- •Configuring Cassini
- •Where do I Put my Files?
- •Using localhost
- •Virtual Directories
- •Using Cassini
- •Installing SQL Server 2005 Express Edition
- •Installing SQL Server Management Studio Express
- •Installing Visual Web Developer 2005
- •Writing your First ASP.NET Page
- •Getting Help
- •Summary
- •ASP.NET Basics
- •ASP.NET Page Structure
- •Directives
- •Code Declaration Blocks
- •Comments in VB and C# Code
- •Code Render Blocks
- •ASP.NET Server Controls
- •Server-side Comments
- •Literal Text and HTML Tags
- •View State
- •Working with Directives
- •ASP.NET Languages
- •Visual Basic
- •Summary
- •VB and C# Programming Basics
- •Programming Basics
- •Control Events and Subroutines
- •Page Events
- •Variables and Variable Declaration
- •Arrays
- •Functions
- •Operators
- •Breaking Long Lines of Code
- •Conditional Logic
- •Loops
- •Object Oriented Programming Concepts
- •Objects and Classes
- •Properties
- •Methods
- •Classes
- •Constructors
- •Scope
- •Events
- •Understanding Inheritance
- •Objects In .NET
- •Namespaces
- •Using Code-behind Files
- •Summary
- •Constructing ASP.NET Web Pages
- •Web Forms
- •HTML Server Controls
- •Using the HTML Server Controls
- •Web Server Controls
- •Standard Web Server Controls
- •Label
- •Literal
- •TextBox
- •HiddenField
- •Button
- •ImageButton
- •LinkButton
- •HyperLink
- •CheckBox
- •RadioButton
- •Image
- •ImageMap
- •PlaceHolder
- •Panel
- •List Controls
- •DropDownList
- •ListBox
- •RadioButtonList
- •CheckBoxList
- •BulletedList
- •Advanced Controls
- •Calendar
- •AdRotator
- •TreeView
- •SiteMapPath
- •Menu
- •MultiView
- •Wizard
- •FileUpload
- •Web User Controls
- •Creating a Web User Control
- •Using the Web User Control
- •Master Pages
- •Using Cascading Style Sheets (CSS)
- •Types of Styles and Style Sheets
- •Style Properties
- •The CssClass Property
- •Summary
- •Building Web Applications
- •Introducing the Dorknozzle Project
- •Using Visual Web Developer
- •Meeting the Features
- •The Solution Explorer
- •The Web Forms Designer
- •The Code Editor
- •IntelliSense
- •The Toolbox
- •The Properties Window
- •Executing your Project
- •Using Visual Web Developer’s Built-in Web Server
- •Using IIS
- •Using IIS with Visual Web Developer
- •Core Web Application Features
- •Web.config
- •Global.asax
- •Using Application State
- •Working with User Sessions
- •Using the Cache Object
- •Using Cookies
- •Starting the Dorknozzle Project
- •Preparing the Sitemap
- •Using Themes, Skins, and Styles
- •Creating a New Theme Folder
- •Creating a New Style Sheet
- •Styling Web Server Controls
- •Adding a Skin
- •Applying the Theme
- •Building the Master Page
- •Using the Master Page
- •Extending Dorknozzle
- •Debugging and Error Handling
- •Debugging with Visual Web Developer
- •Other Kinds of Errors
- •Custom Errors
- •Handling Exceptions Locally
- •Summary
- •Using the Validation Controls
- •Enforcing Validation on the Server
- •Using Validation Controls
- •RequiredFieldValidator
- •CompareValidator
- •RangeValidator
- •ValidationSummary
- •RegularExpressionValidator
- •Some Useful Regular Expressions
- •CustomValidator
- •Validation Groups
- •Updating Dorknozzle
- •Summary
- •What is a Database?
- •Creating your First Database
- •Creating a New Database Using Visual Web Developer
- •Creating Database Tables
- •Data Types
- •Column Properties
- •Primary Keys
- •Creating the Employees Table
- •Creating the Remaining Tables
- •Executing SQL Scripts
- •Populating the Data Tables
- •Relational Database Design Concepts
- •Foreign Keys
- •Using Database Diagrams
- •Diagrams and Table Relationships
- •One-to-one Relationships
- •One-to-many Relationships
- •Many-to-many Relationships
- •Summary
- •Speaking SQL
- •Reading Data from a Single Table
- •Using the SELECT Statement
- •Selecting Certain Fields
- •Selecting Unique Data with DISTINCT
- •Row Filtering with WHERE
- •Selecting Ranges of Values with BETWEEN
- •Matching Patterns with LIKE
- •Using the IN Operator
- •Sorting Results Using ORDER BY
- •Limiting the Number of Results with TOP
- •Reading Data from Multiple Tables
- •Subqueries
- •Table Joins
- •Expressions and Operators
- •Transact-SQL Functions
- •Arithmetic Functions
- •String Functions
- •Date and Time Functions
- •Working with Groups of Values
- •The COUNT Function
- •Grouping Records Using GROUP BY
- •Filtering Groups Using HAVING
- •The SUM, AVG, MIN, and MAX Functions
- •Updating Existing Data
- •The INSERT Statement
- •The UPDATE Statement
- •The DELETE Statement
- •Stored Procedures
- •Summary
- •Introducing ADO.NET
- •Importing the SqlClient Namespace
- •Defining the Database Connection
- •Preparing the Command
- •Executing the Command
- •Setting up Database Authentication
- •Reading the Data
- •Using Parameters with Queries
- •Bulletproofing Data Access Code
- •Using the Repeater Control
- •More Data Binding
- •Inserting Records
- •Updating Records
- •Deleting Records
- •Using Stored Procedures
- •Summary
- •DataList Basics
- •Handling DataList Events
- •Editing DataList Items and Using Templates
- •DataList and Visual Web Developer
- •Styling the DataList
- •Summary
- •Using the GridView Control
- •Customizing the GridView Columns
- •Styling the GridView with Templates, Skins, and CSS
- •Selecting Grid Records
- •Using the DetailsView Control
- •Styling the DetailsView
- •GridView and DetailsView Events
- •Entering Edit Mode
- •Using Templates
- •Updating DetailsView Records
- •Summary
- •Advanced Data Access
- •Using Data Source Controls
- •Binding the GridView to a SqlDataSource
- •Binding the DetailsView to a SqlDataSource
- •Displaying Lists in DetailsView
- •More on SqlDataSource
- •Working with Data Sets and Data Tables
- •What is a Data Set Made From?
- •Binding DataSets to Controls
- •Implementing Paging
- •Storing Data Sets in View State
- •Implementing Sorting
- •Filtering Data
- •Updating a Database from a Modified DataSet
- •Summary
- •Security and User Authentication
- •Basic Security Guidelines
- •Securing ASP.NET 2.0 Applications
- •Working with Forms Authentication
- •Authenticating Users
- •Working with Hard-coded User Accounts
- •Configuring Forms Authentication
- •Configuring Forms Authorization
- •Storing Users in Web.config
- •Hashing Passwords
- •Logging Users Out
- •ASP.NET 2.0 Memberships and Roles
- •Creating the Membership Data Structures
- •Using your Database to Store Membership Data
- •Using the ASP.NET Web Site Configuration Tool
- •Creating Users and Roles
- •Changing Password Strength Requirements
- •Securing your Web Application
- •Using the ASP.NET Login Controls
- •Authenticating Users
- •Customizing User Display
- •Summary
- •Working with Files and Email
- •Writing and Reading Text Files
- •Setting Up Security
- •Writing Content to a Text File
- •Reading Content from a Text File
- •Accessing Directories and Directory Information
- •Working with Directory and File Paths
- •Uploading Files
- •Sending Email with ASP.NET
- •Configuring the SMTP Server
- •Sending a Test Email
- •Creating the Company Newsletter Page
- •Summary
- •The WebControl Class
- •Properties
- •Methods
- •Standard Web Controls
- •AdRotator
- •Properties
- •Events
- •BulletedList
- •Properties
- •Events
- •Button
- •Properties
- •Events
- •Calendar
- •Properties
- •Events
- •CheckBox
- •Properties
- •Events
- •CheckBoxList
- •Properties
- •Events
- •DropDownList
- •Properties
- •Events
- •FileUpload
- •Properties
- •Methods
- •HiddenField
- •Properties
- •HyperLink
- •Properties
- •Image
- •Properties
- •ImageButton
- •Properties
- •Events
- •ImageMap
- •Properties
- •Events
- •Label
- •Properties
- •LinkButton
- •Properties
- •Events
- •ListBox
- •Properties
- •Events
- •Literal
- •Properties
- •MultiView
- •Properties
- •Methods
- •Events
- •Panel
- •Properties
- •PlaceHolder
- •Properties
- •RadioButton
- •Properties
- •Events
- •RadioButtonList
- •Properties
- •Events
- •TextBox
- •Properties
- •Events
- •Properties
- •Validation Controls
- •CompareValidator
- •Properties
- •Methods
- •CustomValidator
- •Methods
- •Events
- •RangeValidator
- •Properties
- •Methods
- •RegularExpressionValidator
- •Properties
- •Methods
- •RequiredFieldValidator
- •Properties
- •Methods
- •ValidationSummary
- •Properties
- •Navigation Web Controls
- •SiteMapPath
- •Properties
- •Methods
- •Events
- •Menu
- •Properties
- •Methods
- •Events
- •TreeView
- •Properties
- •Methods
- •Events
- •HTML Server Controls
- •HtmlAnchor Control
- •Properties
- •Events
- •HtmlButton Control
- •Properties
- •Events
- •HtmlForm Control
- •Properties
- •HtmlGeneric Control
- •Properties
- •HtmlImage Control
- •Properties
- •HtmlInputButton Control
- •Properties
- •Events
- •HtmlInputCheckBox Control
- •Properties
- •Events
- •HtmlInputFile Control
- •Properties
- •HtmlInputHidden Control
- •Properties
- •HtmlInputImage Control
- •Properties
- •Events
- •HtmlInputRadioButton Control
- •Properties
- •Events
- •HtmlInputText Control
- •Properties
- •Events
- •HtmlSelect Control
- •Properties
- •Events
- •HtmlTable Control
- •Properties
- •HtmlTableCell Control
- •Properties
- •HtmlTableRow Control
- •Properties
- •HtmlTextArea Control
- •Properties
- •Events
- •Index

Summary
categoryList.SelectedItem.Value; comm.Parameters.Add("@SubjectID", System.Data.SqlDbType.Int); comm.Parameters["@SubjectID"].Value =
subjectList.SelectedItem.Value;
comm.Parameters.Add("@Description", System.Data.SqlDbType.NVarChar, 50);
comm.Parameters["@Description"].Value = descriptionTextBox.Text; comm.Parameters.Add("@StatusID", System.Data.SqlDbType.Int); comm.Parameters["@StatusID"].Value = 1;
If you now load the Help Desk page, you’ll see that it works just as it used to, but behind the scenes, it’s making use of a stored procedure. You can verify that this approach works by adding a new help desk request through the web form, then opening the HelpDesk table and checking for your new help desk request.
As you can see, using stored procedures is very easy. Apart for specifying the procedure’s name, you also need to set the CommandType of the SqlCommand object to StoredProcedure. That’s it! Everything else is the same as when working with a parameterized query.
Summary
In this chapter, you learned how to create simple web applications that interact with databases. First, you learned about the various classes included with ADO.NET, such as SqlConnection, SqlCommand, and SqlDataReader. Then, you learned how to use these classes to create simple applications that query the database, insert records into a database, update records within a database, and delete records from a database. You also learned important techniques for querying database data, including using parameters and control binding. Later in the chapter, you learned how to improve application performance through the use of stored procedures.
The next chapter will expand on what we learned here, and introduce a new control that’s often used to display data from a database: the DataList.
399

400

10 |
Displaying Content Using Data |
|
|
|
Lists |
Similar to the Repeater control, the DataList control allows you to bind and customize the presentation of database data. The fundamental difference is that while the Repeater requires you to build the template from scratch (allowing you to customize the generated HTML output in any way you like), the DataList control automatically generates a single-column HTML table for you, like the one shown below:
<table>
<tr>
<td>
<p>Employee ID: 1</p> <p>Name: Zak Ruvalcaba</p> <p>Username: zak</p>
</td>
</tr>
<tr>
<td>
<p>Employee ID: 2</p>
<p>Name: Jessica Ruvalcaba</p> <p>Username: jessica</p>
</td>
</tr>
<tr>
<td>
<p>Employee ID: 3</p>
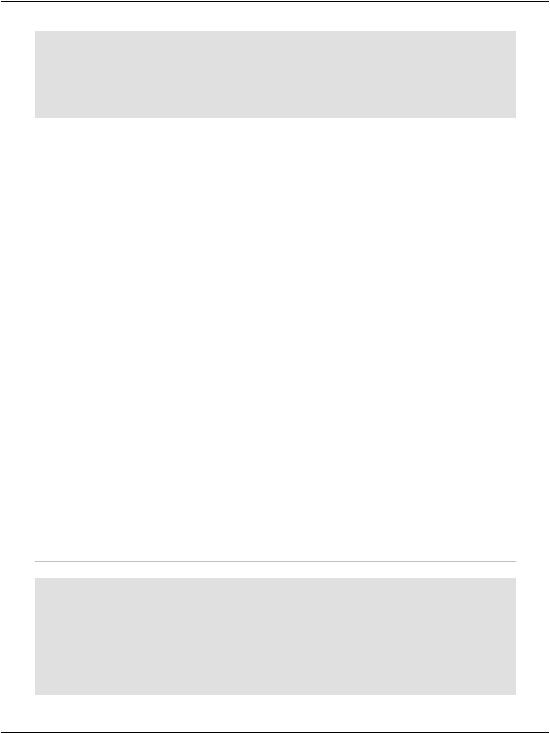
Chapter 10: Displaying Content Using Data Lists
<p>Name: Ted Lindsey</p> <p>Username: ted</p>
</td>
</tr>
</table>
As you can see, DataList has, as the name implies, been designed to display lists of data, and while it’s less flexible than the Repeater, it contains more built-in functionality that can help make the implementation of certain features faster and easier. In the following pages, you’ll learn:
the basics of the DataList control
how to handle DataList events
how to edit DataList items
how to handle the controls inside the DataList templates
how to use Visual Web Developer to edit the DataList
Let’s get started!
DataList Basics
To learn how to use the DataList, we’ll update the Dorknozzle Employee Directory page to use a DataList control instead of a Repeater control. This update will be particularly easy to do because the Employee Directory already has a listlike format.
If you now open EmployeeDirectory.aspx, you’ll see the Repeater control is used like this:
File: EmployeeDirectory.aspx (excerpt)
<asp:Repeater id="employeesRepeater" runat="server"> <ItemTemplate>
Employee ID: <strong><%#Eval("EmployeeID")%></strong><br /> Name: <strong><%#Eval("Name")%></strong><br /> Username: <strong><%#Eval("Username")%></strong>
</ItemTemplate>
<SeparatorTemplate>
402

DataList Basics
<hr /> </SeparatorTemplate>
</asp:Repeater>
You can see the output of this code in Figure 9.9 in Chapter 9. Now, let’s update the employee directory page to use a DataList instead of a Repeater. We can do this simply by replacing the <asp:Repeater> and </asp:Repeater> tags with the tags for a DataList:
File: EmployeeDirectory.aspx (excerpt)
<asp:DataList id="employeesList" runat="server">
<ItemTemplate> Employee ID:
<strong><%#Eval("EmployeeID")%></strong><br /> Name: <strong><%#Eval("Name")%></strong><br /> Username: <strong><%#Eval("Username")%></strong>
</ItemTemplate>
<SeparatorTemplate> <hr />
</SeparatorTemplate>
</asp:DataList>
As we’ve changed the ID for this control, we’ll need to change the name of the control in the code-behind file as well. Locate the following lines of code and change employeesRepeater to employeesList, as shown:
Visual Basic |
File: EmployeeDirectory.aspx.vb (excerpt) |
'Open the connection conn.Open()
'Execute the command
reader = comm.ExecuteReader()
'Bind the reader to the DataList employeesList.DataSource = reader employeesList.DataBind()
'Close the reader
reader.Close()
C# |
File: EmployeeDirectory.aspx.cs (excerpt |
//Open the connection conn.Open();
//Execute the command
reader = comm.ExecuteReader();
// Bind the reader to the DataList employeesList.DataSource = reader; employeesList.DataBind();
403

Chapter 10: Displaying Content Using Data Lists
// Close the reader reader.Close();
As you can see, the changes required to use DataList instead of Repeater are minimal in this case. That’s largely because the Repeater was displaying a basic list of data anyway.
As with the Repeater control, we can feed data into the a DataList control by binding it to a data source. Both Repeater and DataList support the ItemTemplate and SeparatorTemplate templates, but in case of the DataList, the templates specify the content that is to be inserted in the td elements of the table.
At the moment, the output appears very similar to the output we generated using the Repeater, as Figure 10.1 illustrates.
Figure 10.1. The Dorknozzle Employee Directory page
Repeater vs DataList
As a rule of thumb, you’ll use the Repeater when you need total control over the HTML output, and when you don’t need features such as editing, sorting, formatting, or paging for the data you’re displaying. Depending on
404

DataList Basics
the extra features you need, you can use either the DataList control (covered in this chapter), or the GridView or DetailsView controls (which you’ll learn about in Chapter 12).
In this example, we’ve used the ItemTemplate of our DataList. The DataList offers a number of templates:
ItemTemplate
This template is replicated for each record that’s read from the data source. The contents of the ItemTemplate are repeated for each record, and placed inside td elements.
AlternatingItemTemplate
If this template is defined, it will be used instead of ItemTemplate to display every second element.
SelectedItemTemplate
This template is used to display the selected item of the list. The DataList control doesn’t automatically give the user a way to select an item in the list, but you can mark an item as selected by setting the DataLists control’s SelectedIndex property. Setting this property to 0 will mark the first item as selected; setting SelectedIndex to 1 will mark the second item as selected; and so on. Setting SelectedIndex to -1 unselects any selected item.
EditItemTemplate
Similar to SelectedItemTemplate, this template applies to an item that’s being edited. We can set the item being edited using the EditItemIndex property of the DataList, which operates in the same way as the SelectedIndex property. Later in this chapter, you’ll learn how to edit your
DataList using the EditItemTemplate.
HeaderTemplate
This template specifies the content to be used for the list header.
FooterTemplate
This template defines the list footer.
SeparatorTemplate
This template specifies the content to be inserted between two consecutive data items. This content will appear inside its own table cell.
405